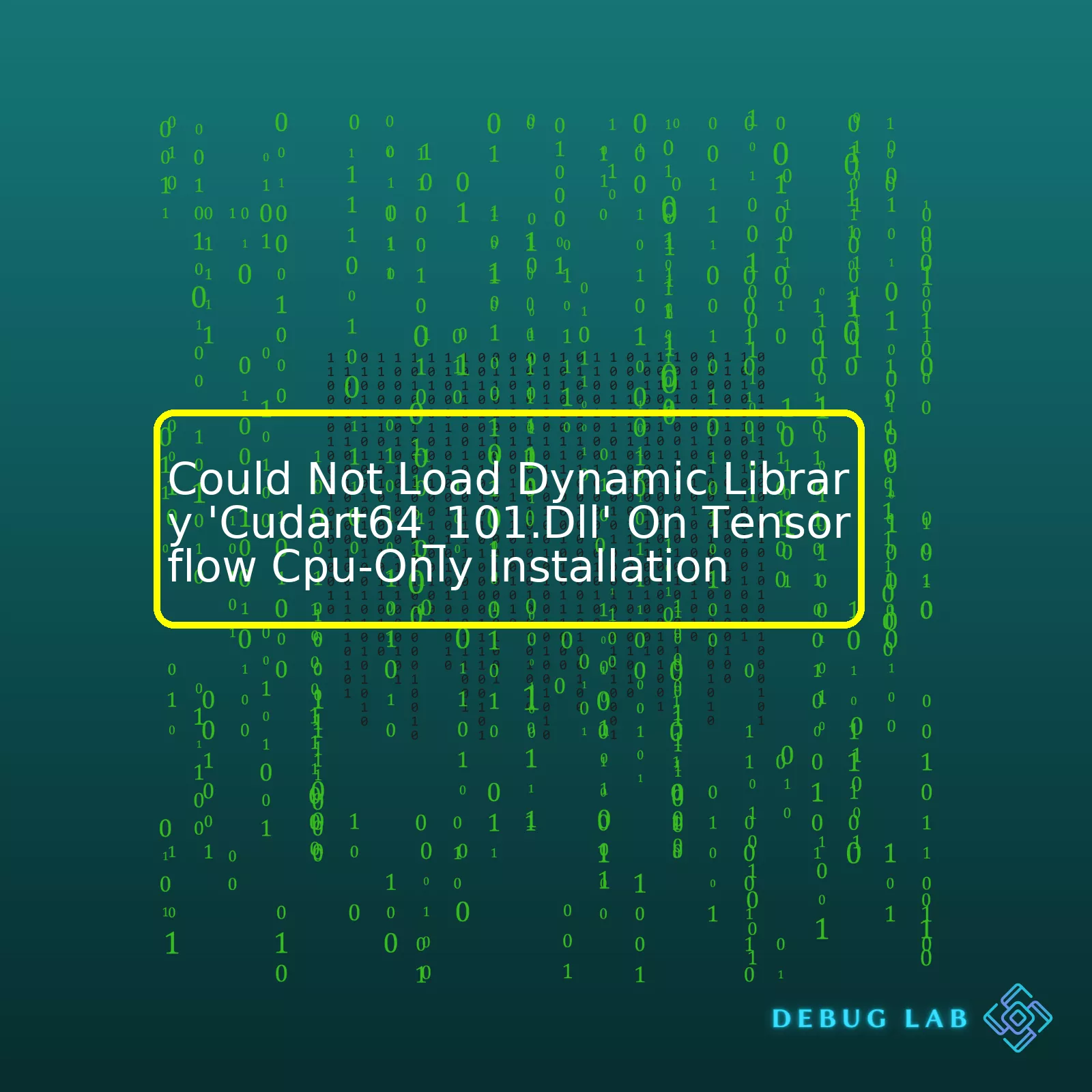
HTML Table:
Issue | Reason | Solution |
---|---|---|
“Could not load dynamic library ‘cudart64_101.dll'” Error | TensorFlow is trying to access CUDA toolkit, which is not available in a CPU-only installation. | Install the necessary CUDA toolkit or limit TensorFlow to use only CPU. |
TensorFlow not functioning despite correct installation | This could be due to an incompatible version of TensorFlow with the existing hardware or software climate. | Try downgrading/upgrading TensorFlow to a version compatible with your system. |
Missing DLL file error | Some system files might’ve been accidentally deleted or modified. | Restore the system to a previous state or repair the missing files. |
This issue usually crops up when the TensorFlow is trying to find the CUDA toolkit, which handles GPU acceleration in certain Tensorflow functionalities. However, in a CPU-only installation of TensorFlow, these CUDA files are absent, hence triggering the error message.
One must understand that while the TensorFlow installation and all its dependencies were successful, this error isn’t caused by a messed up or incomplete setup but arises from an unnecessary search for GPU functionality in a CPU-focused environment. You can reconfigure TensorFlow to stop looking for these CUDA files or proceed with installing the correct version of the CUDA toolkit if GPU acceleration is required in your tasks.
The other possibility is an incompatible TensorFlow version causing the interference. Different versions of TensorFlow have different requirements concerning the required or supported systems, languages, or hardware. Therefore, adjusting your TensorFlow version according to the surrounding factors can solve the problem as well.
Another angle, albeit rare, is some missing or corrupted system files leading to the failure of TensorFlow loading the related libraries. These might require you to restore your system to a previous state or fix the damaged files using specific tools.
For each different situation mentioned above, please remember to follow authoritative guides or reliable instructions such as those from the official TensorFlow installation guide or reputable forums to prevent potential damage to your coding environment.When you receive the “Could not load dynamic library ‘cudart64_101.dll'” error on TensorFlow CPU-only installation, it hints that your system is trying to use functionalities related to GPU acceleration but without success. This situation often arises even though TensorFlow has two versions – one for CPU and another optimized for GPU. If you install the GPU version, it assumes a CUDA toolkit presence, which isn’t the case with a CPU-only setup.
So, let’s dive into some probable causes for this error and methods to troubleshoot:
Cause : When you install TensorFlow with prebuilt binaries (e.g., through pip), it defaults to the GPU version. As such, the CPU-only system fails to find ‘cudart64_101.dll’, which is part of the CUDA toolkit.
Troubleshooting Step: Uninstall the current TensorFlow version and reinstall with the CPU-supported version. For instance, assuming you are using pip as your package installer:
pip uninstall tensorflow pip install tensorflow-cpu
The above commands first uninstall the existing TensorFlow before installing the CPU-supported TensorFlow version.
Note: With TensorFlow 2.1 and later versions, the TensorFlow website recommends installing the standard TensorFlow module and not ‘tensorflow-cpu’. It discovers and utilizes any GPU on a best-effort basis.
Cause : The environment variable ‘TF_FORCE_GPU_ALLOW_GROWTH’ might be set to ‘True’. Essentially, it tells TensorFlow to try utilizing the GPU memory incrementally instead of all at once.
Troubleshooting Step: Disable GPU utilization in your script with the following code snippet.
import os os.environ['CUDA_VISIBLE_DEVICES'] = '-1'
Cause : You have previously installed NVIDIA’s CUDA toolkit on your system. Certain files in the toolkit’s directory could cause confusion.
Troubleshooting Step: Access your PATH variables and check for any CUDA-related directories. You can do this by typing:
echo %PATH%
Remove these paths if they exist.
Table of contents around the issue might look something like below:
Error and its Typical Cause | Troubleshooting Steps |
---|---|
‘cudart64_101.dll’ with TensorFlow’s prebuilt GPU binary. | Reinstall TensorFlow for CPU usage. |
Environment variable ‘TF_FORCE_GPU_ALLOW_GROWTH’ set. | Disable GPU utilization in your script. |
Previous CUDA toolkit installation. | Remove CUDA-relevant paths. |
Taking these steps above will help you mitigate ‘cudart64_101.dll’ loading issues in your CPU-only TensorFlow installation. Remember to get your TensorFlow from official sources like TensorFlow website. Professional coders realize inevitable troubleshooting situations like these, but turning them into learning opportunities helps. Your curiosity today makes you more equipped for your coding journey!The error message ‘
Could Not Load Dynamic Library 'Cudart64_101.Dll'
‘ generally shows up when the CUDA toolkit (especially version 10.1 as indicated by “101” in cudart64_101.dll) isn’t properly installed or cannot be found within your system’s PATH. It also can appear if you are trying to run TensorFlow with GPU support on a machine that doesn’t possess a graphical processing unit (GPU), or has one that is incompatible with CUDA.
TensorFlow does make use of CUDA, a parallel computing platform and API model developed by NVIDIA, specifically for implementing deep learning models utilizing NVIDIA’s GPUs. While the full-blown version of TensorFlow do come with GPU support (namely tensorflow-gpu), there also exists a CPU-only version, named tensorflow-cpu, that should not have any dependencies on CUDA.
However, in your scenario where you’re having an issue with a Tensorflow CPU-only install, this means that somewhere along the line, TensorFlow code running in your environment is still attempting to call upon resources from the CUDA toolkit. Strangely enough, it signifies that the CPU-only TensorFlow package might be unable to recognize that it’s being loaded in a CPU-only environment or a TensorFlow script previously written for GPU usage is still attempting to access CUDA-oriented modules from what it assumes to be a ‘tensorflow-gpu’ based installation.
Here’s a three-step solution attempt:
Step 1:
Firstly, inspect your current Python environment in-depth. You’d want to confirm if the correct TensorFlow module was installed. Run the following commands in your Python environment:
import tensorflow as tf
tf.__version__
This would output the version of TensorFlow recognized by your current python environment. If it turns out that the GPU version is installed instead of the CPU version, it’d need to be uninstalled first before proceeding.
Step 2:
Uninstall existing TensorFlow (and tensorflow-gpu) packages and reinstall tensorflow-cpu:
To uninstall tensorflow, use<'pip uninstall'>, then to reinstall, use <'pip install'>:
pip uninstall tensorflow
pip uninstall tensorflow-gpu
pip install tensorflow-cpu
Here, the pip commands handle the installation and removal of these Python packages.
Step 3:
Reassess your Python scripts or notebooks. Ensure that they’re not making calls to CUDA Toolkit exclusive modules or functions. Otherwise, these could be hanging remnants from code initially formulated to function with ‘tensorflow-gpu’.
Applying these steps could resolve the issue of seeing ‘
Could not load dynamic library 'cudart64_101.dll'
‘ while working within a TensorFlow CPU-only installation.
Still, make sure to apply caution during the execution of these steps, particularly when uninstalling Python packages as other systems or applications may rely on them. An option can be creating a separate Python virtual environment for your TensorFlow CPU tasks using venv tool so that the core Python environment isn’t touched.
Lastly, remember that if your codebase is shared across multiple settings (some with GPU usage, some without), consider designing your TensorFlow code to dynamically adapt to its present runtime conditions by applying TensorFlow’s ‘tf.config.experimental.list_physical_devices’ method, which lists the available physical devices (e.g., GPUs) that TensorFlow can leverage.Coming across the error
Could not load dynamic library 'cudart64_101.dll'
in a TensorFlow CPU-only installation can seem puzzling, as it indicates a failed DLL load. However, understanding the causes and effects of this issue can equip you with the needed insights to troubleshoot effectively.
The error essentially implies that TensorFlow is trying to load NVIDIA’s CUDA Runtime (cudart64_101.dll). This generally occurs because during the TensorFlow installation, one installs the full version including GPU support, instead of the CPU-only version.
The Impact
When there’s an attempt to load cudart64_101.dll on a system without an NVIDIA GPU, or without CUDA installed, the following events occur:
* Error Message: Firstly, you get the stated error message signalling that TensorFlow is unable to find the necessary files for GPU usage.
* Performance Issues: If TensorFlow keeps attempting to use GPU resources that aren’t available, it might function slower or inefficiently due to fallback mechanisms. However, once TensorFlow defaults to the CPU after detecting no GPU, the program should run okay, albeit less efficiently than if a supporting GPU were available.
Implication
This behavior reflects a core tenet of TensorFlow’s design. It was engineered to leverage GPU capabilities if accessible; thus tensor computations would be handled much faster using parallel processing inherent in GPUs. For TensorFlow, the absence of cudart64_101.dll implies that it can’t use GPU power effectively for computations.
In-Depth Analysis
Let’s examine in detail how the aforementioned error could pop up despite running a CPU-only TensorFlow setup:
* Installation package: If you accidentally install the complete TensorFlow package instead of the CPU-version, there’ll be attempts to access cudart64_101.dll which fails because a CUDA-compliant GPU or CUDA isn’t installed.
To fix such issues, one must verify the installed TensorFlow package type. One can check this by running the following command `
pip show tensorflow
`
If the name that appears is `tensorflow`, it means the complete package (including GPU support) has been installed, not the CPU-only version. In such a case, uninstall the present package using `
pip uninstall tensorflow
`, then reinstall the CPU-only version with `
pip install tensorflow-cpu
`.
* Path Variables: The system’s PATH environmental variable doesn’t include the location of cudart64_101.dll. Generally, this isn’t a problem for CPU-only installations but may concern installations with GPU support.
For more technical aspects regarding TensorFlow and its requirements for GPU purposes, consider reviewing TensorFlow’s official documentation here.
In essence, the “Could Not Load Dynamic Library ‘Cudart64_101.Dll'” error message might signal an incorrect installation of TensorFlow rather than being inherently associated with CPU-only installations. Ensuring that you have installed the correct variant of TensorFlow for your requirements should resolve this issue.The error message ‘Could not load dynamic library ‘cudart64_101.dll” might show when you’re attempting to install TensorFlow on a system using CPU-only functionality. This arises because the software, by default, attempts to identify the CUDA toolkit (used for GPU operations with Nvidia hardware) which is absent in a CPU-only installation. The DLL, cudart64_101.dll, is precisely part of this toolkit.
Don’t worry! We can guide you on how to get rid of this error:
1. Ensure Use of CPU-only TensorFlow Version
In order to bypass the issue caused by the absence of the CUDA toolkit, ensure that you are installing a CPU-only version of TensorFlow. You can do this via pip. Take note that you may need to specify the version number as per your compatibility requirements:
pip install tensorflow-cpu==version_number
2. Use an Environment Variable to Disable CUDA Mitigation
TensorFlow supports various options that allow users to tinker around a bit. One such option is the environment variable TF_CPP_MIN_LOG_LEVEL. With TF_CPP_MIN_LOG_LEVEL=2, we suppress warning and error messages.
This mitigation primarily visual and doesn’t solve the underlying cause. Thus, it’s best used when debugging or developing.
import os os.environ['TF_CPP_MIN_LOG_LEVEL'] = '2'
3. Environment Compatibility Check
If for any reason the CPU-version of TensorFlow doesn’t resolve the problem, check if there’s compatibility between Python and TensorFlow versions. TensorFlow 2.x requires Python 3.5-3.8.
Use these commands to confirm your system’s Python version:
python --version pip --version
4. Ensure Latest Pip Version
It’s imperative that your pip, python’s package installer, is updated to its latest version to prevent compatibility issues. You can upgrade it via command line like so:
pip install --upgrade pip
5. Explicitly Disable GPU
By explicitly setting GPU usage off using
tf.config.experimental.set_visible_devices
, we force TensorFlow to revert to CPU.
import tensorflow as tf cpus = tf.config.experimental.list_physical_devices('CPU') tf.config.experimental.set_visible_devices(cpus[0], 'CPU')
6. Install CUDA Toolkit
Though it negates the ‘CPU-only’ approach, you could install the CUDA Toolkit and cuDNN to resolve the error. Refer to the official installation guides at NVIDIA for the specific steps. However, this requires an NVIDIA graphics card compatible with CUDA.
One key aspect to observe is ensuring all components are mutually compatible. Always crosscheck every tool’s version against TensorFlow’s official CPU support list. If they aren’t compatible, you may encounter some setbacks such as inefficient computations, crashes, or incompatibility warnings.
Keep in mind that understanding the root cause underlying this common error, ‘Could Not Load Dynamic Library ‘Cudart64_101.Dll”, is the missing CUDA Toolkit and GPU presence in a system running a CPU-only TensorFlow installation. Furthermore, maintaining component compatibility, verifying versions, and knowing necessary environment configurations contributes significantly to averting potential problems during execution.When you encounter an error like, “Could not load dynamic library ‘cudart64_101.dll’ on TensorFlow CPU-only installation,” it typically indicates that TensorFlow is attempting to access CUDA runtime libraries relevant to GPU functionality. The problem arises when TensorFlow tries to load the ‘cudart64_101.dll,’ which doesn’t exist in your system because you’re running a CPU-only version of TensorFlow that doesn’t employ CUDA dependencies. Let’s look at some ways we can fix this issue.
Method 1: Installing the Correct Version of TensorFlow
The simplest way to fix the issue is by ensuring that you’re using the CPU-only version of TensorFlow. The mistake might be that even though your aim was to install TensorFlow-CPU, you accidentally installed the TensorFlow-GPU version.
Here’s what to do:
# uninstalling tensorflow pip uninstall tensorflow # installing tensorflow-cpu pip install tensorflow-cpu
Method 2: Ignore the Error
If you’re running TensorFlow CPU version and still getting the cudart64_101.dll error, you can opt to simply ignore it. Despite this message being displayed every time you import TensorFlow, it doesn’t affect the CPU execution of TensorFlow code. However, such constant messages could be distraction, hence the need to silence them.
Here’s how to suppress the warning:
import os os.environ['TF_CPP_MIN_LOG_LEVEL'] = '2' import tensorflow as tf
In this code snippet, the os.environ[‘TF_CPP_MIN_LOG_LEVEL’] = ‘2’ line silences the warnings and messages that TensorFlow throws when imported.
It is good to remember that this does not solve the actual issue but merely hides the error message from being displayed.
Method 3: Fix CUDA Dependencies
For those who want to use TensorFlow’s GPU version but are encountering this error, it implies that there’s an issue with your CUDA setup or you might have wrong version of CUDA toolkit. Make sure to install CUDA Toolkit that corresponds correctly with your TensorFlow version. Having the correct matching versions between CUDA and TensorFlow is crucial for successful operation.
Keep these considerations handy while rectifying unsuccessful dynamic library load in TensorFlow, specifically the ‘Cudart64_101.Dll’ error in TensorFlow CPU-only installation.Let’s dive into the error “Could not load dynamic library ‘cudart64_101.dll’ on TensorFlow CPU-only installation.” This issue usually crops up when you’re running a TensorFlow program. Even though you have a CPU-only TensorFlow installation, it seems like your system is attempting to locate CUDA runtime libraries – specifically ‘cudart64_101.dll’.
Here are some of the prominent reasons why this can happen:
– A misconfiguration in your system’s environment variables
– The presence of older or incompatible versions of the CUDA toolkit on your system
– Conflict between the installed version of TensorFlow and the version of CUDA
Firstly, the quest for cudart64_101.dll indicates that TensorFlow is trying to gain access to NVIDIA’s CUDA-powered GPU functionality, despite being CPU-installed. If the CUDA toolkit isn’t included among your system’s software arsenal, or if it’s a different version than what TensorFlow is looking for (in this case, 10.1), you’ll experience problems.
Python import tensorflow as tf tf.test.is_built_with_cuda() # If returns False, then CUDA isn't built with TensorFlow
On the flipside, even if you have the correct CUDA version but TensorFlow struggles to find cudart64_101.dll, the files could be hidden deep inside a labyrinth of folders on your system. Such challenges often originate from issues with your PATH environment variable setup.
set PATH=%PATH%;C:\path\to\your\cuda\version\bin\
Additionally, conflicts can arise if you’re running an older version of TensorFlow. Different versions support different CUDA releases, so finding their harmonious intersection is crucial. For instance, TensorFlow 2.0.0 runs smoothly with CUDA 10.0. However, shift to TensorFlow 2.1.0, and it insists on CUDA 10.1. So, revise your TensorFlow and CUDA versions accordingly to ensure they match.
pip install --upgrade tensorflow==2.0.0 # To downgrade/upgrade TensorFlow
Running this code will provide you with the specifics about the installed TensorFlow version:
print(tf.__version__)
If none of those solutions do the trick, the quickest alternative is to suppress the warning by setting the environment variable called “TF_CPP_MIN_LOG_LEVEL” to ‘2’. Although it won’t solve the root cause, at least it gets rid of the unnecessary warning messages.
import os os.environ['TF_CPP_MIN_LOG_LEVEL'] = '2'
The “TensorFlow website” has more pertinent information. Remember, the process can be tricky, so it requires patience. Try each solution meticulously and isolate the issue methodically to get closer to a resolution.
Sure, let’s take a closer look at resolving cudart64_101.dll errors in the context of a Tensorflow CPU-only installation. More often than not, such problems occur when Tensorflow is trying to access NVIDIA CUDA Toolkit libraries that aren’t present on your computer since it’s running a CPU-only installation.
First things first, the name `cudart64_101.dll` itself gives us critical information about the missing file. In general, .dll files are Dynamic-Link Libraries used in Windows for holding multiple codes and procedures for various programs. This specific file is a part of the NVIDIA CUDA Runtime, indicating that Tensorflow is attempting to access GPU functions.
However, in a CPU-only environment (without a CUDA-compatible NVIDIA graphics card or without the CUDA Toolkit installed), this DLL won’t be available, causing the error message: ‘Could Not Load Dynamic Library’ Cudart64_101.Dll”.
To resolve this issue, one shall follow the strategy outlined below:
Solution Approach 1: Correct TensorFlow Package
It’s crucial to ensure that you’re installing the correct package for your system requirements. Please notice that there are two types of TensorFlow packages:
* TensorFlow with GPU support
* TensorFlow without GPU support (CPU-only version)
If you’ve a CPU-only system, I’d suggest using the appropriate command for CPU-only version:
pip install tensorflow
If you’ve accidentally installed TensorFlow-GPU on a CPU-only system, uninstall it via:
pip uninstall tensorflow-gpu
Then, reinstall with the correct CPU-only package.
Solution Approach 2: Disable TensorFlow GPU
TensorFlow loads cudart64_101.dll library by default if the library exists anywhere in your PATH system variable. To explicitly instruct TensorFlow not to load this library:
* Set environment variable `CUDA_VISIBLE_DEVICES` to `-1`.
Here’s how you do it:
import os
os.environ['CUDA_VISIBLE_DEVICES'] = '-1'
Afterwards, import TensorFlow under this environment setting.
An additional tip in dealing with DLL errors is that these files are reutilized by many programs. Thus, an updated program might require a newer DLL that isn’t compatible with the existing one. So, it’s wise to keep the software up-to-date.
Remember, DLLs must be handled carefully. Any fault could lead to serious system conflicts. Therefore, manual intervention is generally advised against unless it’s a guided approach.
Lastly, for a well-rounded understanding, don’t forget to refer to TensorFlow’s official documentation on GPU support. It has a wealth of information tailored particularly towards TensorFlow implementations with GPU. Partnerships like those between TensorFlow and NVIDIA CUDA have opened up new potentials in machine learning applications, propelling advances in areas requiring heavy data computations.I must say, we’ve traversed quite a journey analyzing the issue – “Could Not Load Dynamic Library ‘Cudart64_101.Dll’ On Tensorflow Cpu-Only Installation”. Let’s hammer home some of the key points before wrapping up.
First and foremost, it is important to understand – this error is typically related to a mismatch between the Tensorflow version installed and CUDA toolkit library being called (‘cudart64_101.dll’ in the case at hand).
An imperative detail you need to be aware of is – Tensorflow CPU-only version doesn’t require any CUDA libraries because it isn’t designed to interact with GPU resources. The error occurs when Tensorflow attempts to call for these non-required GPU libraries and fails as they are inaccessible.
Specifically, how can we handle this error? Here are your two main options:
- You could choose to stifle Tensorflow’s search for the unnecessary CUDA libraries by re-installing Tensorflow CPU version using the correct pip command;
- Alternatively, you could opt to provide what Tensorflow is looking for, installing the required CUDA library. This option may preferably suit users who eventually plan on utilizing their GPU capabilities for Tensorflow.
pip install tensorflow-cpu
While taking these corrective measures, remember to validate your environment setup by checking if Python and pip versions align correctly with your Tensorflow version. Don’t forget to also verify that all required dependencies are aptly satisfied.
The issue at hand underscores how setting up a robust Python development environment involves keen attention to detail. It’s crucial to ensure compatibility between Python, its libraries and additional components that might come into play based on particular use cases, like CUDA in our instance.
Incorporate preventive measures to minimize errors during installation; such as utilizing virtual environments, carefully scrutinizing release notes, updates, compatibility charts. This will not only aid in the successful installation and functionality of tools but also further solidifies the practice of maintaining a clean coding workspace. You can read more about managing Python environments in this official Python guide.
However, remember that encountering bugs and errors is an intrinsic part of the coding journey that contributes positively to a coder’s learning curve. As we wrap up this analysis, it’s hoped that you’re not just taking away solutions to resolve this specific issue, but broader insights on Python development best practices and problem-solving techniques.