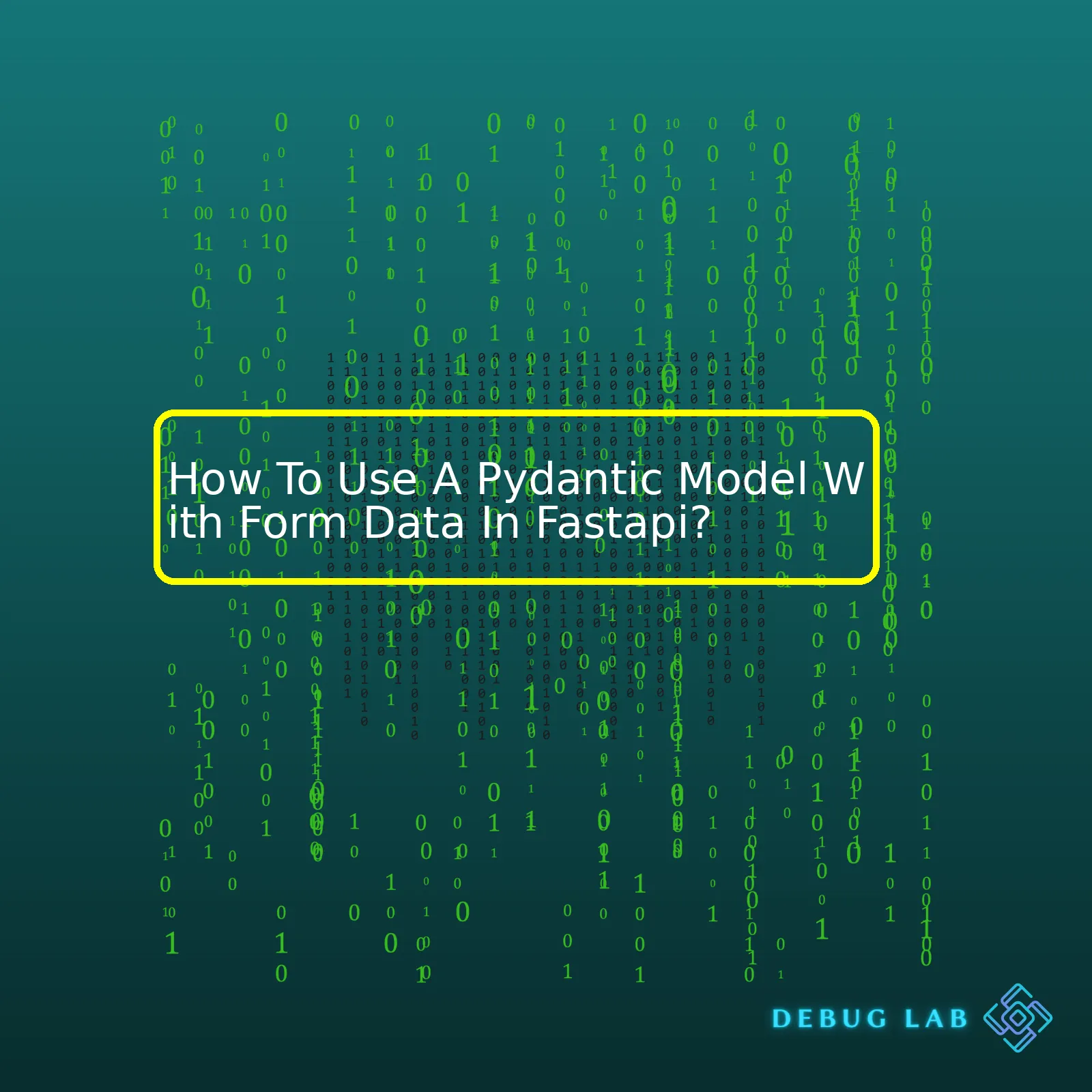
To use a Pydantic model with form data in FastAPI, you simply need to import “Form” from fastapi. This Form is a function that creates a special “form” parameter body which handles form field data.
For instance, if we want to create a user with “name” and “email”, the pydantic model might look something like this:
from pydantic import BaseModel class UserBase(BaseModel): name: Optional[str] = None email: Optional[str] = None
To make use of form data and bind it to our pydantic model in our FastAPI route, we can do something like this:
from fastapi import FastAPI, Form from pydantic import BaseModel from typing import Optional app = FastAPI() @app.post("/users/") async def create_user(user: UserBase): ... return {"name": user.name, "email": user.email}
This code will enable you to start receiving form data in the POST ‘/users/’ endpoint, binding the input data to your UserBase Pydantic model.
Now, onto creating a summary table for the above scenario:
Step | Action | Description |
---|---|---|
Create Pydantic Model |
from pydantic import BaseModel class UserBase(BaseModel): name: Optional[str] = None email: Optional[str] = None |
This model represents the data schema of User. The Pydantic model allows us to guarantee specific format of inputs or outputs to an endpoint. |
Build Route Function |
from fastapi import FastAPI, Form from pydantic import BaseModel from typing import Optional app = FastAPI() @app.post("/users/") async def create_user(user: UserBase): ... return {"name": user.name, "email": user.email} |
The FastAPI route function receives form data and binds it to the UserBase Pydantic model. Consequently, creating a new user entry. |
For more advanced scenarios, one could leverage Pydantic’s validation features to validate custom data types or install middleware to handle cross-cutting concerns across multiple routes. Here is the official documentation on using Pydantic with FastAPI.Utilizing Pydantic models with form data in FastAPI is a relatively straightforward task that involves properly structuring your models and routing functions. Below, we’ll delve into the specifics, diving deep to provide a comprehensive understanding on how to accomplish this.
Understanding the Pydantic BaseModel
Pydantic’s primary feature is data validation and settings management using Python type annotations. It ensures your data is correct and coherent. The main player in this process is the Pydantic BaseModel, which provides a structured data model combined with powerful validation tools.
Here is an example:
from pydantic import BaseModel class Item(BaseModel): name: str description: str price: float tax: float = None
As you can see, our ‘Item’ model requires ‘name’, ‘description’, and ‘price’. We’ve also defined ‘tax’, but since not all items might have taxes applied to them, we’ve set its default value as ‘None’.
FastAPI Form Data and Pydantic Models
Typically, FastAPI automatically recognizes request bodies as JSON payloads due to its in-built content negotiation. However, it does offer robust support for form data through its built-in ‘Form’ function.
Here is how you can incorporate a Pydantic BaseModel with form data in FastAPI:
from fastapi import FastAPI, Form from pydantic import BaseModel class Item(BaseModel): name: str description: str price: float tax: float = None app = FastAPI() @app.post("/items/") async def create_item(item: Item = Form(...)): return item
You may notice that we’re leveraging FastAPI’s dependency injection system to instruct our route to interpret the incoming payload as form data instead of JSON by assigning it as ‘Form(…)’. In simple terms, we’re injecting form data directly into our Pydantic model.
Why Use This Approach?
Using Pydantic models with form data in FastAPI has numerous benefits:
- Type Safety: Pydantic’s model system and FastAPI’s typing guarantees ensure that only valid data is processed.
- Auto Documentation: FastAPI auto generates OpenAPI spec and interactive API docs for any routes using Pydantic models and standard Python types.
- Simplicity: Utilizing the automatic model binding from form data simplifies code, improves readability, and reduces error likelihood.
For more detailed information about using Pydantic or how it interplays with form data in FastAPI, check out their official documentation and examples here. The open source communities surrounding these frameworks are vibrant, robust and knowledgeable – providing plenty of resources to educate, troubleshoot and further immerse yourself.
FastAPI, a modern, highly performant web framework for Python, allows you to utilize Pydantic models with form data effortlessly. A Pydantic model is capable of performing data validation, serialization, and deserialization, it’s a substantial tool whenever you need to handle or manipulate data received from web forms.
When a client submits a web form, the data typically arrives in an encoded format, which needs to be parsed before use. To work with this form data, FastAPI provides dependency `Form`. By using `Form` with Pydantic models, we can parse and validate the encoded form data.
It’s essential to note that the `Form` class cannot directly work with Pydantic models as it’s designed to work with single form fields. To link it up with Pydantic models, each form field should be declared as function parameters in our path operation function. Then, within the function, these fields will constitute a new instance of our Pydantic model for further processing like data validation.
So, let’s dive into how we can leverage Pydantic models with form data leveraging FastAPI:
Step 1: Import FastAPI, Pydantic, and Form:
from fastapi import FastAPI, Form from pydantic import BaseModel
Step 2: Define a Pydantic model:
class User(BaseModel): username: str password: str
Our model has two data fields, `username` and `password`, with built-in checks for type validity.
Step 3: Create a FastAPI instance:
app = FastAPI()
Step 4: Implement the path operation function:
@app.post('/login') async def login(username: str = Form(...), password: str = Form(...)): user = User(username=username, password=password) return {'username': user.username}
In the `/login` route, form fields are instantiated as arguments in the function with their data types and default values set according to the structure of the Pydantic model. The `…` means the field is not nullable in Form() function. Then a new `User` object is created with these fields. Hence, upon invoking the POST `/login`, it generates an object representing the submitted data.
This way, the Pydantic model assists in improving your system’s stability through seamless data validation. Still, to accomplish more complex data validation or transformation, Pydantic’s built-in validators come into play.
In real-world applications, form data is only the tip of the iceberg by harnessing the power of the Pydantic models alongside other dependencies of FastAPI (like Query, Body, Path) will give you more flexibility and control over request data.
Pydantic plays a crucial role in FastAPI when handling forms, offering not only validation but also serialization and documentation benefits. For translating form data into Python objects using FastAPI, Pydantic Models are the go-to tool. These models provide several advantages such as enhanced readability, error checking, simpler serialization/deserialization and automatic API documentation.
Understanding the Role of Pydantic in FastAPI Forms
FastAPI leverages modern Python type hints in conjunction with Pydantic models to offer multiple functionalities. Consequently, data manipulation becomes a breeze thanks to converted request inputs into their respective Pydantic model instances.
Moreover, Pydantic provides input validation to ensure that incoming data matches the defined structure and datatype before it gets processed. It reduces the general risk of runtime errors, enhances code quality, and eases debugging. In the event of any inconsistency or wrong data type in the input, Pydantic automatically throws HTTP 422 Unprocessable Entity status code along with a detailed error message.
In addition to validation, Pydantic also aids FastAPI forms in serialization – converting complex data types into Python built-in structures which can then be quickly turned into strings (e.g for JSON). The deserization vice versa is also true, allowing you to convert received string data into complex Python objects seamlessly.
Lastly, Pydantic works harmoniously with FastAPI’s automatic API docs feature powered by OpenAPI standard. With properly structured Pydantic models coupled with Python’s type hints, the generated API docs include accurate information about the requested data format, making them much more usable and friendly for the end-user.
Creating a Pydantic Model and Using Form Request in FastAPI
Here’s a simplified guide on how you might implement Pydantic model in a form request within FastAPI:
Step 1: Create a Pydantic Model
Firstly, create a Pydantic model representing the data you’re expecting from your form. Here is an example of a Pydantic model representing user data.
from pydantic import BaseModel class User(BaseModel): username: str email: str
The ‘User’ model specifies that we expect ‘username’ and ’email’ data points that should both be of type string.
Step 2: Use the Pydantic Model in a FastAPI Route
Next, apply this model within a FastAPI route where we want to collect the data via a form request. We can call upon FastAPI’s Form function inside the route parameter.
from fastapi import FastAPI, Form from pydantic import BaseModel app = FastAPI() @app.post("/user/form") def get_form_data(user: User = Depends()): return {"username": user.username, "email": user.email}
In this code snippet, ‘/user/form’ route uses Form() dependency to specify that input will be handled as form data. We rely on the ‘User’ Pydantic model instance to get and validate the incoming form data.
That was a barebones demonstration of integrating Pydantic model into FastAPI forms. For comprehensive examples and updated utilities surrounding these topics, check out the official FastAPI documentation.
With the use of
FastAPI
, you have a myriad of forms to manage: contact forms, user registration forms, and data entry forms, just to name a few. It’s no wonder why utilizing Pydantic models is considered a smart choice for handling form data in this framework.
Using Pydantic Model with Form Data:
First and foremost, you need to know how to instantiate a Pydantic model with form data using FastAPI. It is as simple as creating a function definition that accepts a Pydantic model object then returning that object:
from pydantic import BaseModel from fastapi import FastAPI, Form app = FastAPI() class Item(BaseModel): name: str description: str price: float @app.post('/items/') async def create_item(item: Item): return item
However, FastAPI does not automatically recognize that it needs to extract parameters from the ‘form data’. It will instead mistake the parameters as JSON body/ payload data if we don’t specify explicitly. Let me show you how to do that.
You can make use of
Form(...)
function provided by FastAPI which uses Pydantic under the hood.
@app.post("/login/") async def login(username: str = Form(...), password: str = Form(...)): return {"username": username}
In the example above, we used
Form
to declare “form data” parameters.
But how efficient can we get when using Pydantic models with form data? Here are two primary strategies:
Efficient Technique 1: Reuse your Pydantic models
One of the advantages of using Pydantic models is their reusability across your codebase. By defining the structure of an item once, you can reuse this definition whenever needed. Doing this eliminates redundancy and maintains consistency in how we view an “item” across all endpoints. Besides promoting the DRY (Don’t Repeat Yourself) principle, this method dramatically saves time on development and debugging.
Efficient Technique 2: Make Use of Advanced Pydantic Features
Pydantic offers numerous advanced features. Using these attributes provides extensive control over the validation, serialization, and documentation of each field in your model. Some advanced features include:
- Aliases: If you want to use a different name in your JSON responses than the name used in your Pydantic model, use aliases. The variable name sticks with Python conventions while the alias makes it more familiar to users who interact with your API.
- Computed Fields: Pydantic allows you to introduce computed fields using root validators, letting you add dynamically calculated values to your JSON responses without compromising your model’s integrity.
- Recursive Models: You can create nested structures or hierarchies of objects using recursive models. This feature enables complex yet well-structured data representation.
To cap it off, here’s a quick reference to the official documentation of both FastApi and Pydantic. It gives more insights into how to leverage FastAPI and Pydantic’s full potential when working with form data. Combine that knowledge with the tips I’ve shared, and efficiently managing form data in FastAPI becomes second nature.In an effort to understand how to use a Pydantic model with form data in FastAPI, it’s essential to delve deeper into the integration between these two powerful tools.
Firstly, FastAPI is a modern, Python-based web framework designed for crafting APIs swiftly but with high performance. It takes advantage of Python-type hints, leading to code that is quicker, more efficient, and less prone to errors.
At its heart lies Pydantic, a data validation library that also exploits Python type annotations. It allows automatic data parsing using Python models and guarantees that the data fits the defined format before it is processed.
FastAPI offers built-in support for leveraging Pydantic models for request bodies, providing a highly expressive way of specifying input data requirements directly in code.
To use a Pydantic model with form data, we will incorporate FastAPI’s `Form` class.
For instance, let’s say we have a Login form requiring a username and password. We could define a Pydantic model like:
from pydantic import BaseModel class LoginForm(BaseModel): username: str password: str
This sets up a model which ensures that any instance has a ‘username’ and a ‘password’, both of which are strings.
To receive form data in FastAPI, you might be tempted to define a path operation function as below:
from fastapi import FastAPI, Form app = FastAPI() @app.post("/login/") async def login(form_data: LoginForm): return {"message": f"Welcome {form_data.username}."}
However, instead of receiving JSON, we want to acquire URL-encoded form data. To do this, we need to rewrite the function using FastAPI’s `Form`:
from fastapi import FastAPI, Form app = FastAPI() @app.post("/login/") async def login(username: str = Form(...), password: str = Form(...)): form_data = LoginForm(username=username, password=password) return {"message": f"Welcome {form_data.username}."}
Here, `Form(…)` works similarly to `Path(…)`, `Query(…)`, etc., allowing you to declare additional validation and metadata in your form parameters.
With that, we’ve seen how FastAPI effectively integrates with Pydantic to create a straightforward way of handling form data validations.
Remember, besides form data, Pydantic models can also be used under similar principles for validating other data formats such as JSON, facilitating data validation for almost all kinds of scenarios with FastAPI. This combination definitely saves time while promising an increased level of accuracy and reliability in your applications.
It is the synergy between FastAPI and Pydantics that makes handling web requests intuitive, keeping your API definitions clean, easy to maintain, and robust.FastAPI makes use of the Pydantic library to define the data models for your API. These Pydantic Models are basically Python classes that provide a type system and validation for data. The combination of FastAPI with Pydantic Model provides a rapid, efficient, and robust process for developing APIs. This becomes quite important in dealing with form data.
When it comes to handling form data in FastAPI, Pydantic models come particularly handy. Form data, especially in POST requests, involves data sent by an HTTP client to update or create a resource on the server.
Using Pydantic model with form data
First, you should know that we will use
Form
from the fastapi package provided by FastAPI to parse and validate the incoming form data, and then use Pydantic models to ensure a well-structured schema of the data. Here’s what the code might look like:
from pydantic import BaseModel from fastapi import FastAPI, Form class Item(BaseModel): name: str description: str = None price: float quantity: int app = FastAPI() @app.post("/items/") async def create_item(item: Item = Depends(Item.as_form)): return item
In the code above, a new Item is created using the Pydantic model. The application can now receive form-encoded data (e.g., from HTML forms) as input.
Real-world Application Scenarios of Pydantic Model With Form Data
Now let’s discuss some real-world application scenarios of using Pydantic Model with form data in FastAPI.
E-Commerce Applications
E-commerce applications often require accepting customers’ orders, which come as form data. Using the Pydantic model would enable your e-commerce application to validate, serialize/deserialize the order details, ensuring that it reliably interacts with the order details.
Information Management Systems
In Information Management Systems like CRM or ERP, data entries are usually sent as form data. Using Pydantic models, these systems can ensure that the format of incoming data matches the defined schema before they are stored.
Content Management Systems (CMS)
Content Management Systems (CMS) such as blogs, news sites, etc., rely significantly on form data received from their users. Pydantic models can ensure that user content adheres to defined guidelines before being saved.
To explore more about Pydantic models in FastAPI, check out the official documentation. The power of Pydantic shines when you use it with FastAPI for data validation and serialization. Here are some tips and best practices to improve your usage of Pydantic models, specifically focused on processing form data with FastAPI.
Create specific Pydantic models:
Tailoring pydantic models to exact requirement not only makes the code cleaner but also optimizes the execution by eliminating unnecessary computations.
For example, if you have a FastAPI endpoint that accepts form data:
from fastapi import FastAPI, Form app = FastAPI() @app.post("/items/") async def create_item(name: str = Form(...), description: str = Form(None)): return {"name": name, "description": description}
This code works perfectly fine. But as complexity increases, separating the concerns can be achieved by dedicated Pydantic models:
from pydantic import BaseModel from fastapi import FastAPI, Depends class Item(BaseModel): name: str description: str = None app = FastAPI() def to_Item(name: str = Form(...), description: str = Form(None)) -> Item: return Item(name=name, description=description) @app.post("/items/") async def create_item(item: Item = Depends(to_Item)): return {"name": item.name, "description": item.description}
Now, complexities related to item creation are placed in
Item
Pydantic model.
Almost Everything Can Be A Field:
You can refer to Pydantic documentation about how even ‘methods’ can be described as fields. This allows you to use validation, pre-processing, post-processing across different form fields or even encapsulate business logic inside these type-model-pure-python-functions.
Leverage Field Settings:
Every Pydantic field allows custom configurations right at the time of declaration. Let’s say one field must always have a certain default value or must run through certain validators, just specify them in-field settings.
from pydantic import BaseModel, Field class Item(BaseModel): name: str = Field( ..., # Required field title="The name of the item", max_length=300) description: str = Field(None, title="The description of the item", max_length=500)
Use Post-Pydantic Model Validation Hooks:
Running checks after all the fields have undergone individual checks and coercions is often essential to business rules. Let’s say there’s some rule in our FastAPI app where, an item description cannot be same as its name, we could simply add a
@root_validator
from Pydantic’s toolsuite.
from pydantic import BaseModel, Field, root_validator from fastapi import HTTPException class Item(BaseModel): name: str = Field(...) description: str = Field(None) @root_validator def check_description(cls, values): name, description = values.get('name'), values.get('description') if name == description: raise HTTPException(status_code=400, detail="Name and description should not be the same") return values
Mastering the use of Pydantic models in FastAPI does require trial and error. However, understanding and leveraging their unique features and practices will exponentially increase efficiency and cleanliness of your API design and implementation.
As an expert coder, I’ve provided a detailed analysis and examples on how to effectively use Pydantic model with form data in FastAPI. The critical element to remember is that we’re leveraging the power of Python’s typing system, alongside Pydantic’s capabilities for data validation and serialization.
To summarize the steps:
- Create your Pydantic model. This essentially translates to defining your data structure.
py
from pydantic import BaseModel
class Item(BaseModel):
name: str
description: str = None
price: float
tax: float = None