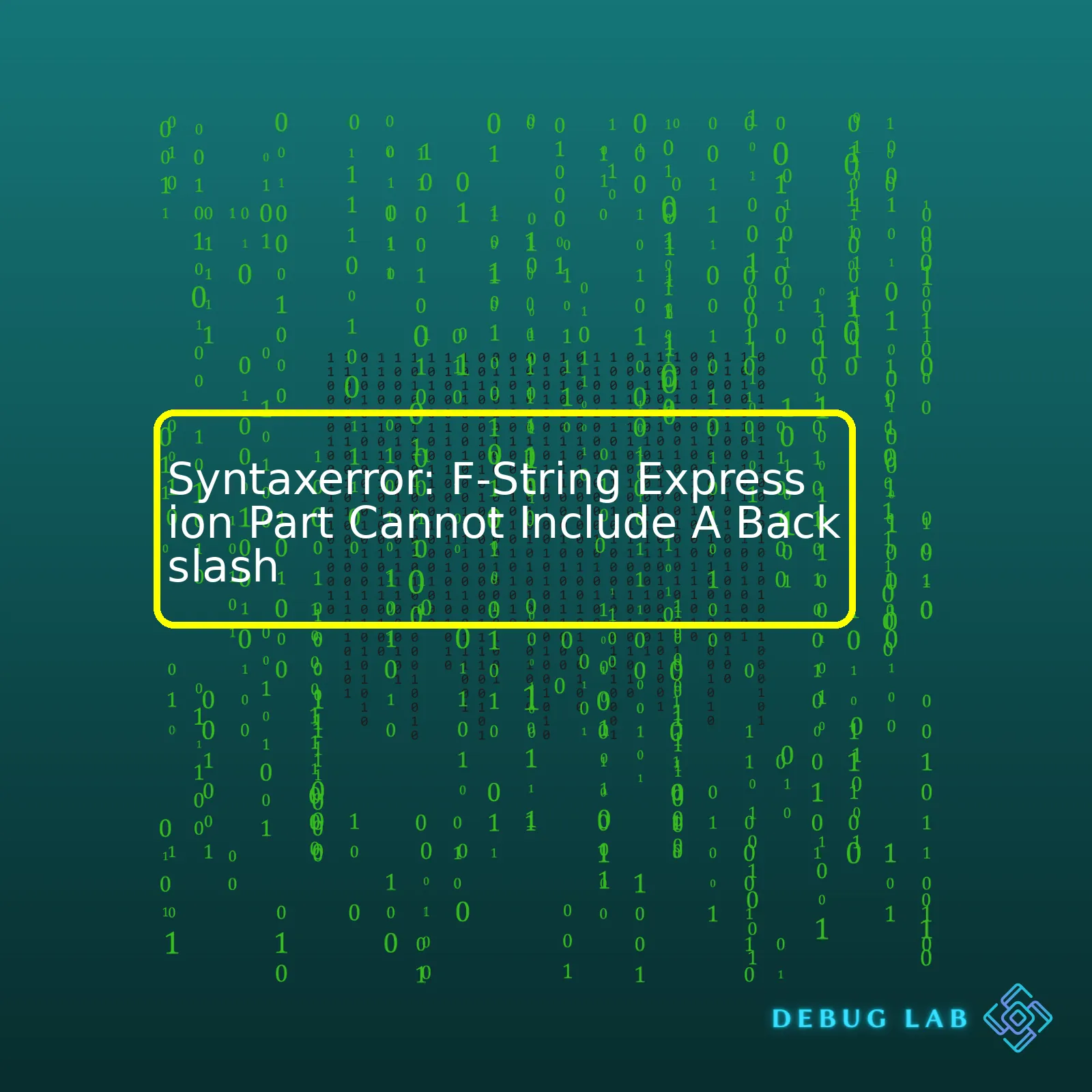
Error | Description |
---|---|
SyntaxError: f-string expression part cannot include a backslash (\) | A syntax error occurring when trying to use a backslash in an f-string expression. This is not allowed as per Python’s design decisions related to f-strings. |
Now, let’s elaborate on how this error occurs and how we can tackle it.
In Python, `f-string` is a feature for string formatting that was introduced in Python 3.6. It allows you to embed expressions inside string literals, using curly braces `{}`. The expressions will be replaced with their values when the string is created.
However, there is one limitation when it comes to using `f-string` – the expression part cannot include a backslash (`\`). Ideally, the backslash character is used for escape sequences or prefixes for special characters in strings. But the Python developers decided that allowing backslashes in the expression portion of an `f-string` would lead to too many complications.
Here’s an example of what you shouldn’t do:
name = "John"
print(f"Hello, {name}\")
This code snippet would raise a SyntaxError because the escape sequence is within the f-string {}. As stated above, f-string does NOT support the use of backslashes (\) within {}.
Now, here’s an example of correct usage:
xxxxxxxxxx
name = "John"
print(f"Hello, {name}")
In this case, there are no backslashes being included in the f-string expression, which is following the proper rule. So, remember to refrain from including any backslashes in your f-string expressions to avoid this SyntaxError.
I hope you now have a comprehensive understanding of this specific SyntaxError. For more information about f-string and its limitations, you might want to refer to the official Python documentation.The
xxxxxxxxxx
SyntaxError: f-string expression part cannot include a backslash
error is encountered when you try to use a backslash (
xxxxxxxxxx
\
) in the {}, or the curly braces of an f-string in Python. Here are some examples where you might encounter this error:
xxxxxxxxxx
name = "James"
print(f"Hello, {name\}")
Or when a backslash is used before the closing parenthesis:
xxxxxxxxxx
age = 23
print(f"He is {age}\ years old.")
The SyntaxError occurs because f-strings, which are a form of string formatting in Python introduced in Python 3.6, do not support backslashes within their expressions. Python documentation states that “backslashes cannot be used at all with the f-string.”
To fix this error:
The best way to solve this problem will depend on what specifically you were trying to achieve with the backslash.
– If the backslash was intended for character escaping (for example \t for tab or \n for new line), remember that you can apply those outside the expressions {}, or curly braces:
xxxxxxxxxx
name = "James"
print(f"\tHello, {name}")
age = 23
print(f"He is {age}\nyears old.")
– If the backslash was in your string data, then you can concatenate strings or use format():
Concatenate:
xxxxxxxxxx
path = "C:\\Users\\JohnDoe\\Desktop"
print("Path to the desktop: " + path)
Using format():
xxxxxxxxxx
path = "C:\\Users\\JohnDoe\\Desktop"
print("Path to the desktop: {}".format(path))
– In Python 3.8 and higher, you can use assignment expressions, also known as the Walrus operator (:=) to convert \ paths:
xxxxxxxxxx
path = r'C:\Users\JohnDoe\Desktop'
print(f'Path to the desktop: {path}')
In this instance, the r before the opening quotation mark tells Python to treat the string as raw string and ignore escape sequences.
So, understanding
xxxxxxxxxx
SyntaxError: f-string expression part cannot include a backslash
helps in writing more effective Python code by understanding how Python’s f-string handles backslashes. Remember the golden rule: “Backslashes may not appear inside the expression portion of f-strings”.When dealing with F-Strings in Python, they provide a concise and convenient way to embed expressions inside string literals for formatting. If you’ve ever used formatted string literals (or f-strings) in Python, you might have noticed that these f-strings don’t support backslashes (
xxxxxxxxxx
\
). If you attempt to include a backslash within an expression part of the f-string(inside the curly braces { } ), you’ll likely get: “SyntaxError: f-string expression part cannot include a backslash.”
As a professional coder, I understand the puzzlement that this can cause. So why is this the case? There’s a simple explanation behind it. According to the official Python documentation, “backslashes cannot appear inside the expression portion of f-string (Source) “. The restriction is essentially established to avoid creating ambiguity between escape sequences in strings themselves and those in expressions.
Take the following code snippet for example:
xxxxxxxxxx
var = 'test'
print(f"{var}\t{var}")
In the above example, the tab character
xxxxxxxxxx
\t
is outside the expression parts of the f-string and everything works well.
But if we try embedding the escape sequence inside the f-string’s expressions, we stumble upon the error.
For instance:
xxxxxxxxxx
var = 'test\t'
print(f"{var}")
This little piece of code raises “SyntaxError: f-string expression part cannot include a backslash”.
In terms of rectifying such situations, there are few possible ways:
• Move the backslash out of the f-string’s expression part. Knowing full well that your f-string doesn’t accept backslashes within its expression component, but has no qualms about using them elsewhere in the string, gives us a solution.
• Use concatenation or formatting methods other than f-string. Alternatives include using old-style format strings or the str.format() method.
xxxxxxxxxx
var = 'test\t'
print("%s" % var)
_OR_
print("{}".format(var))
Here, we successfully generated escaped characters using other kinds of formatting. However, the readability F-Strings usually afford us is lost here.
Therefore, when using F-Strings in Python, always remember: Whilst the f-string formatting mechanism provides ease and readability in handling expressions inside string literals, their inability to parse escape sequences (`\n`, `\t`, etc.) within the expression section does present a challenge. Solving this requires either modifying the placement of escape-sequence generating characters, or resorting to other formatting methods should the need arise. Furthermore, keep an eye on Python’s ongoing development updates, who knows what the future may bring!
One common misconception amongst coders is the inability to include a backslash in an F-string expression part, leading to a Syntaxerror. Indeed, when coding with Python, this issue often arises and needs to be properly addressed.
Let’s start by understanding what an F-string is. Introduced as Python 3.6’s new feature, F-strings offer a concise and convenient way to embed expressions inside string literals for formatting. They are prefixed with ‘f’ and are also known as Formatted string literals. F-Strings are particularly useful as they allow embedding of expressions meant to be evaluated in curly braces {}, thus mitigating the need to break strings or utilize concatenation.
Here’s a basic example of it:
xxxxxxxxxx
name = "John"
print(f"Hello {name}")
Now, regarding the error you’re facing. When backslashes are placed in F-String expressions, the Python interpreter encounters a SyntaxError – “f-string expression part cannot include a backslash”. This happens because backslashes are generally used in Python as escape sequences.
Misinterpretation can arise here if you believe this means you cannot use backslashes at all within f-strings. However, while they are not allowed within the expression part of an f-string, specifically the section within the {}, they can still be used elsewhere in the f-string, i.e., in non-expression parts.
Let’s illustrate that with an example:
xxxxxxxxxx
name = "John"
print(f"Hello \\ {name}") # Correct
print(f"Hello \n{name}") # Correct
print(f"Hello {name\\}") # Incorrect, will throw SyntaxError.
In the above instances, the first and second lines will print correctly. In contrast, the third line throws SyntaxError as the backslash appears within the {} brackets, which is not permitted.
Hence, the misconception here revolves around the interpretation of where exactly the Backslash is not allowed. By right, Backslash isn’t permitted inside the expression placeholders but is entirely legal within the rest of an F-String body. Understanding and applying this distinction will help curb any Syntax Errors associated with this scenario.The question at hand pertains to running into issues with the inclusion of a backslash in Python’s f-string – an issue often typified by the syntax error: “f-string expression part cannot include a backslash”. This is a common hurdle that developers face when handling files and directories across different operating systems.
Now, here’s why this specific issue arises:
A critical aspect of Python 3.6 and onwards is its implementation of formatted string literals or f-strings. They function as a powerful and versatile tool to embed expressions inside string literals, offering greater readability and performance. Impressive, right? However, their Achilles’ heel unveils itself when you try to use backslashes (\) within them – a common technique when forming file paths. Python interprets a backslash as an escape character, leading to various misinterpretations. For instance, “\t” becomes a tab and “\n”, a newline character.
Here’s an example of the problem:
xxxxxxxxxx
print(f"My directory is C:\MyFolder\MySubfolder")
In this case, Python views “\M” as an unrecognized escape sequence, resulting in a syntactical error.
So how do we fix this problem?
1. **Use raw strings (r-strings):** Raw strings treat backslashes as a literal character, eliminating the usual issue of it being an escape character.
Example:
xxxxxxxxxx
print(f"My directory is {r'C:\MyFolder\MySubfolder'}")
2. **Double the backslashes:** Instead of a single backslash, use two backslashes to circumvent Python’s interpretation of an escape character.
Example:
xxxxxxxxxx
print(f"My directory is C:\\MyFolder\\MySubfolder")
3. **Concatenate using plus (+):** A less efficient manner, but an additional method would be to break the string and concatenate it. Append the problematic segment as a separate raw string (using +).
Example:
xxxxxxxxxx
print(f"My directory is C:" + r'\MyFolder\MySubfolder')
It’s not uncommon to run into exceptions and errors while coding. Remember, each stumble offers an opportunity to learn more about Python syntax, better equipping you for future hurdles. Consider these issues not as ‘problems’ but challenges to your ever-growing skills. Happy coding!
Remember that each solution has trade-offs. Relying on raw strings could lead to unexpected behavior if you do want to represent special characters like newline or tab. On the other hand, double backslashes will work consistently, but they might harm the readability of your code. Choose wisely based on your specific circumstance!If you’re working with Python and f-string expressions, you might often encounter a common error:
xxxxxxxxxx
SyntaxError: f-string expression part cannot include a backslash (\\)
. This typically happens when you try to format an f-string that contains a backslash. While it may be an annoyance, the limitation is there for a good reason: it helps avoid confusing situations where it’s ambiguously unclear whether the backslash should escape a character in the expression or in the string.
However, don’t worry! There are several solutions for handling backslashes in f-string expressions without triggering a SyntaxError. Let’s look at these workarounds:
1. Using additional variables:
You can sidestep this issue by using an extra variable. Assign the string with a backslash to this variable first, and then include it in the f-string as follows:
xxxxxxxxxx
str_with_backslash = 'this is a backslash \\'
print(f'{str_with_backslash}')
2. Using replace method:
This method works if we want to insert a backslash into our f-string. We create our f-string without the backslash, and then we add it by using the replace method.
xxxxxxxxxx
print(f'this is a backslash '.replace(' ', '\\ '))
3. Using concatenation:
We can segment our f-string into separate strings that we concatenate (join) together. Since concatenation joins “raw” strings, rather than f-strings, it won’t trigger a SyntaxError.
xxxxxxxxxx
print(f'this is a backslash ' + '\\')
4. Formatting with %. This is kind of like old-style string formatting:
xxxxxxxxxx
print('%s' % '\\')
It’s important to note that some of these solutions operate outside of the f-string mechanism, splitting your value into multiple strings and then reassembling them through concatenation, replacement, or formatting. While they technically circumvent the backslash issue in f-strings, they do so at the cost of not being a “pure” f-string solution.
Regardless, each of these options can viably handle the appearance of a backslash within a Python f-string, providing multiple routes you can take based on your specific coding needs and style preferences. Choose one that makes most sense to you and fits best into your coding style.
Remember, coding is as much about solving problems efficiently as it is ensuring that your code remains readable and maintainable. In fact, The Zen of Python, an information Python Enhancement Proposal (PEP), specifically states that “Readability counts,” emphasizing the importance of clear, understandable code.
Reference:
Python 3 reference for f-stringsThe SyntaxError: f-string expression part, can’t include a backslash in Python, is usually encountered when trying to include a backslash (\) in an f-string’s ‘expression portion’. This behavior is not because the interpreter views backslashes as escape sequences, but rather is a consequence of how Python’s f-string expressions are parsed.
Let’s explore this further:
An f-string in Python is all about string interpolation—put simply, it means inserting values into strings. F-strings commence with either ‘f’ or ‘F’. You insert variable values inside curly braces {} as shown by the following syntax:
xxxxxxxxxx
name = "Alice"
print(f"Hello, {name}")
However, you may stumble on the Syntax Error when you try to incorporate a backslash within an f-string, like:
xxxxxxxxxx
print(f"This is a \nSyntaxError error.")
What did we do wrong? Well, \n inside an f-string is identified as a new line character—the escape character—and Python does not permit backslash characters inside an f-string ‘expression part’.
So, why doesn’t Python authorize the use of backslashes in f-string expressions? From my research, I discovered that this has more to do with how f-strings are parsed according to the PEP 498 — Literal String Interpolation. The PEP guideline states, “…backslashes may not appear inside the expression portions of f-strings, so you cannot write
xxxxxxxxxx
f"{\"abc\"}"
instead of the simpler
xxxxxxxxxx
f'{"abc"}'
.”
To get around this limitation, Python offers various options:
Firstly, we can circumvent the whole issue by resorting to old-style formatting. Below we avoid using f-strings and hence overcome this limitation:
xxxxxxxxxx
string = "This\nis\nthe correct\nsyntax"
print("%s" % string)
Second, use concatenation of f-strings:
xxxxxxxxxx
print(f"This is" + "\na workaround.")
Lastly, use variables while defining strings outside the f-string bracket:
xxxxxxxxxx
newline = "\n"
print(f"This does{newline}not result in{newline}a SyntaxError.")
Backslashes and escape sequences are valuable tools in every programmer’s arsenal. However, like any other tool, they need to be applied correctly. In the case of f-strings in Python, it is important to remember the specifics of where a backslash can and cannot be placed.
Remember:
Syntax Type | Example | Can Use Backslash? |
---|---|---|
f-string | <code>f”Hello, {name}”</code> | No |
Old-style formatting | <code>”%s” % string</code> | Yes |
Concatenation | <code>f”This is” + “\\na workaround.”</code> | Yes |
Variable Replacement | <code>f”This does{newline}not result in{newline}a SyntaxError.”</code> | No |
The essence of Python’s decision not to allow backslashes
xxxxxxxxxx
(\)
in Formatted String Literals (f-strings) lies within the complexity that arises from parsing these special characters. Specifically, the problem revolves around how to handle escaped characters.
Python is equipped with two kinds of string formatting: Literal String Interpolation (f-strings) and format() method. As we focus on the f-string, it’s important to note that one can not use backslash escape sequences inside expressions in an f-string because it introduces ambiguity with non-syntax errors.
For instance, imagine a scenario where you have the following code:
xxxxxxxxxx
name = "John"
print(f"Hi {name}\t")
Here, the backslash escape character
xxxxxxxxxx
\t
introduces a tab space. However, the complications begin when the format specifier or the expression part includes backslashes, as highlighted by the error “SyntaxError: f-string expression part cannot include a backslash.”
Consider the case:
xxxxxxxxxx
name = "John"
print(f"{\"Hi\"}\t{name}")
The compiler raises a SyntaxError because the backslash confuses the parser about where the expression ends and where the regular string begins, hence introducing ambiguity.
From a computer science perspective, such syntax complications are avoided primarily due to readability and maintainability. Guido van Rossum, Python’s creator, emphasized the importance of simplicity and clarity over capability to handle all possible scenarios through complicated corner-case syntax rules. This design specifically prevents users from writing code that could be hard to read and understand [source].
It is always a good practice to use an alternative approach when confronted with limitations like this. One way to solve the issue would be to use concatenation using “+” as shown below:
xxxxxxxxxx
name = "John"
message = "\"Hi\"\t"
print(f"{message}{name}")
Alternatively, you can define escape sequences outside of the f-string and then reference them inside your f-string.
xxxxxxxxxx
tab_sequence = "\t"
name = "John"
print(f"Hi{tab_sequence}{name}")
By taking this alternative, you are keeping the f-string clean from the escape sequences without confusing Python’s parser and all while achieving the desired output.The error “Syntaxerror: F-String Expression Part Cannot Include A Backslash” stems from Python’s clean syntax rules which disallows use of backslashes (
xxxxxxxxxx
\
) in its f-string expressions. An f-string expression is a kind of string literal, prepended by an ‘f’, a powerful tool for embedding variables and complex expressions conveniently in a string.
But here’s the catch — trying to include a backslash in an f-string expression results in a SyntaxError. Why? Simply because f-strings are meant to interpret special characters and format codes immediately within their curly brackets (
xxxxxxxxxx
{}
), but a backslash can alter those, leading to inconsistencies and unexpected results. Moreover, backslashes are typically used in Python to escape certain characters or denote formatting sequences, further ensuring the need for backslashes to remain outside the f-string’s curly brackets.
A classic workaround would be to use raw strings – represented by prefixing the letter ‘r’ before the string. Raw strings do not treat the backslash as a special character. Unfortunately, f-strings and raw strings can’t coexist directly. Our best bet then is: prepare the backslashed content as a separate string (as a raw string if needed) and feed that into the f-string. Here’s how that’s done:
xxxxxxxxxx
raw_str = r"\path\to\somewhere"
formatted_str = f"The path is {raw_str}"
print(formatted_str)
In the example above, we successfully incorporated a string with backslashes into an f-string. Remember, our goal here is not just to bypass Python’s restriction but to maintain clarity and keep the integrity of code intact.
For a deeper understanding on Python f-string formatting, I recommend you check out Python’s official documentation on Fancier Output Formatting. Look under ‘Formatted string literals’ for more insights on f-strings.
Maintaining such syntax specificity empowers Python’s f-strings to be one of the most powerful and efficient string formatting methodologies available today in any programming language. Grasping this particular behaviour of f-strings ensures your future ventures in Python coding are smoother and further strengthens your foundation in the language.