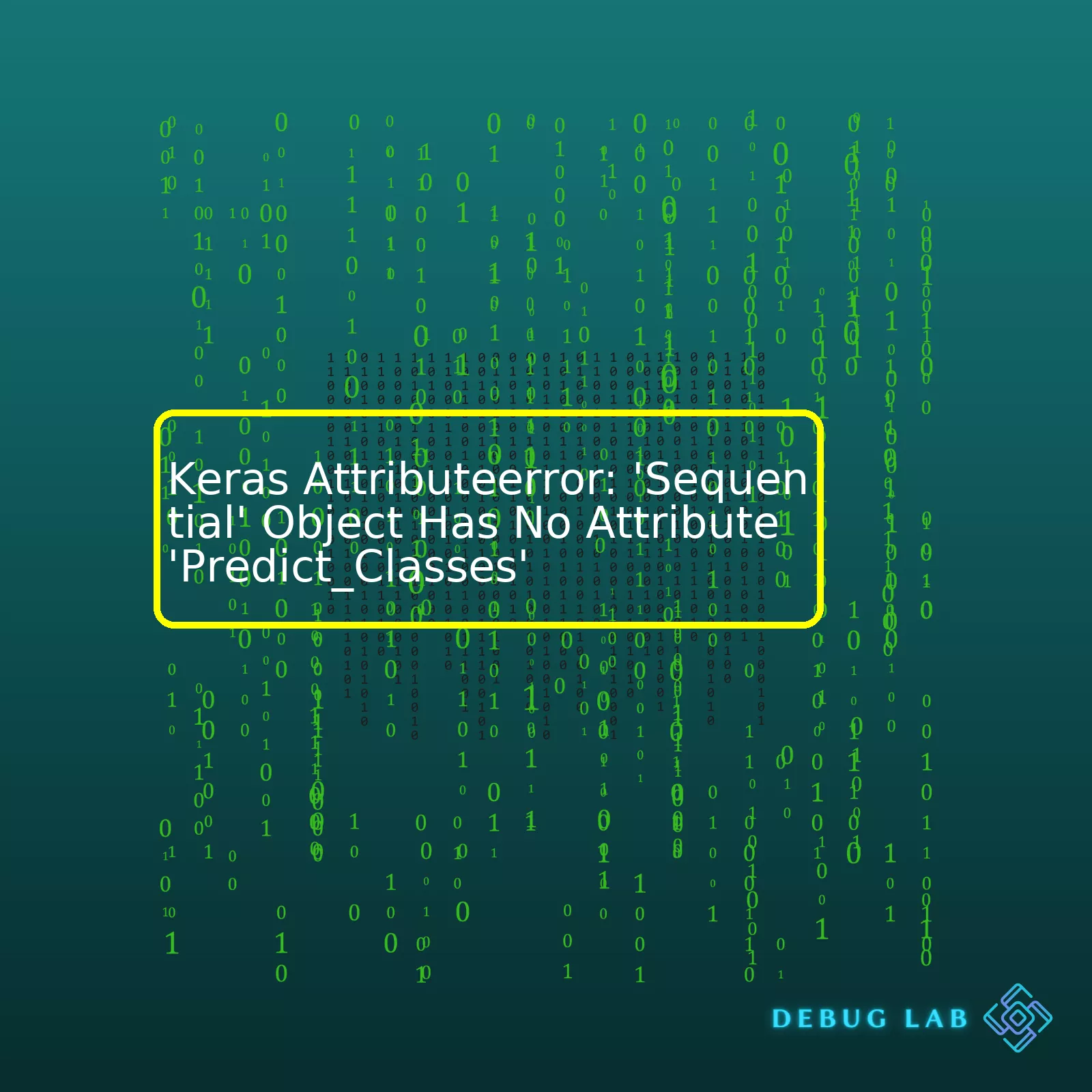
predict_classes
method for Sequential models in recent versions. Instead, now we utilize the
predict
or
argmax
methods to achieve this functionality.
Here’s a summary table that explains this more precisely:
Error | Cause | Solution |
---|---|---|
Keras Attributeerror: ‘Sequential’ object has no attribute ‘predict_classes’ | The method
predict_classes is not available in Recent versions of Keras |
Replace
predict_classes with predict or use numpy’s argmax function |
In older versions of Keras, you could use the
predict_classes
method on a Sequential model to make predictions and return the class indices. It was incredibly convenient as it would handle multi-class and binary classification scenarios without requiring any extra work on your part.
However, with updates to Keras,
predict_classes
has been removed. So if you’ve updated your Keras version recently and used this function previously, your code might break, giving rise to an AttributeError.
But fret not! old functionalities are still preserved and easily accessible through small alterations. You can incorporate either the
predict
method or the
argmax
function which will deliver similar outcomes.
Using the
predict
function:
predictions = model.predict(x_test) predicted_classes = np.argmax(predictions, axis=1)
Or the
argmax
method for obtaining index of the maximum value:
predicted_classes = model.predict(test_data).argmax(axis=-1)
Both these blocks of code carry out the same operation as the erstwhile
predict_classes
, returning the class indices of the likely outputs.
For more details on this topic, have a look at Keras documentation (here) where usage of different functions and their evolution over time are discussed.
Before we dive into the specifics of the error
'Sequential' object has no attribute 'predict_classes'
, let’s have a deep understanding of the Keras Sequential model.
The Keras Sequential API is one of the simplest models you can create in Keras. It allows programmers to create neural networks by literally stacking up layers, just like adding items in an array or list. This makes it intuitive for beginners who are new to machine learning. As a coder myself, building neural networks via the Sequential API felt like assembling a Lego tower – simple, logical and extremely fun to do. However, while Keras simplifies many things, understanding the fundamentals of its API could save us from potential trainwrecks, such as the one we’re about to solve.
You see, the essential code snippet to build a sequential model generally looks like this:
from keras.models import Sequential from keras.layers import Dense model = Sequential() model.add(Dense(32, input_shape=(500,)))
Here, we created a Sequential object named
model
, and added a dense layer with 32 nodes. Simple and straightforward.
Now, back to our main issue: the AttributeError that says our Sequential object doesn’t have the attribute
'predict_classes'
. This error arises from the fact that the keras
predict_classes()
function isn’t available for the Sequential class.
But don’t despair! The solution is quite simple: you can instead use the
predict()
method and apply an argmax operation to achieve the same result. Let me show you how.
prediction = model.predict(input_data) predicted_classes = np.argmax(prediction, axis=1)
In this piece of code, what happens is that `model.predict(input_data)` computes the probabilities of each class. The function `np.argmax()` then selects the class with the highest probability, which achieves the same result as the nonexistent `predict_classes()` method would.
This way, even though the Sequential API doesn’t provide a method named ‘predict_classes’, you can work your way around the problem with ease!
For more insightful details and solutions, feel free to consult the Keras Documentation for the Sequential Model.
Coding errors often point to areas where we lack understanding – in this case, the functionality offered by the Keras Sequential API. By addressing the problem in-depth and providing alternatives, they open opportunities for learning, ultimately enabling us, coders, to grow our skills!
The `Sequential` object in Keras is a fundamental construct used to create models in a step-by-step linear stack of layers. Its flexibility and simplicity make it a popular choice for both rookie data scientists and established professionals.
But as potent as the `Sequential` object may be, it doesn’t come without its quirks and hiccups. For instance, one common issue that users encounter is the AttributeError stating: _’Sequential’ Object Has No Attribute ‘predict_classes’_.
Here, we’ll delve into the creature called `Sequential`, focusing on its attributes and their usage. We’ll also touch upon the above-mentioned error and how to mitigate it when coding with Keras.
from keras.models import Sequential from keras.layers import Dense model = Sequential() # Layers added here ... model.add(Dense(12, activation='relu', input_shape=(8,))) model.add(Dense(8, activation='relu')) model.add(Dense(1, activation='sigmoid'))
The main attributes of a `Sequential` object spans across its structure, settings, state, and compiled information:
– Input_spec: Specifies the ndim, dtype and shape of every input to a layer.
– Layers: This attribute is a flattened list of the layers comprising the model.
– Name: String, the name of the model.
– Trainable: Whether the model should be trained. If set False, no weight will updated during training.
– _is_compiled: This boolean value indicates whether the model has been compiled yet or not.
– Weights: This returns the weights of the model, as a list of Numpy arrays.
When it comes to the ‘predict_classes’ attribute error, it’s essential to note that this function has been removed from Keras API starting from version 2.3.0. This function was used previously for classification models to generate class outputs.
However, in newer versions, you can use the `predict` method instead and handle the class inference using numpy argmax in a multiclass problem.
# Predicting class using the latest versions of Keras import numpy as np predictions = model.predict(X_data) predicted_classes = np.argmax(predictions, axis=1)
For binary classifiers, you can round off the predictions to achieve your classes.
# In case of binary classification predicted_classes = (model.predict(X) > 0.5).astype("int32")
To avoid encountering attribute errors in the future, it’s advisable to keep abreast with changes in the Keras API by reading through the official [Keras release notes](https://github.com/keras-team/keras/releases). This will keep you informed about deprecated functions and new additions, thus helping maintain your code in line with the current standards.When working with Keras, a deep learning library in Python, you might find yourself facing the attribute error
AttributeError: 'Sequential' object has no attribute 'predict_classes'
. So why does this happen?
Typically, when attempting to make predictions with a classifier model constructed using Keras’ Sequential API, one would use the method
predict_classes()
to output the class of inputs. However, as of Keras version 2.6, you may receive an
AttributeError
because
predict_classes()
and
predict_proba()
have been removed from Keras’ API. The reasoning behind this move is that these methods only work for certain types of models – primarily those for binary and multi-class classification tasks – which led to confusion and errors.
Solution to this problem:
The suggested alternative to the removed methods is to use
predict()
instead, which works universally for all types of models, not just classifiers. For binary classification:
“To receive class predictions (as integer labels), after calling predict() you can call np.argmax(axis=-1) on the result.” [Keras API reference](https://keras.io/api/models/model/)
Here’s an example snippet of how you would modify your code to avoid this error:
from tensorflow.keras.models import Sequential import numpy as np # define a sequential model model = Sequential() # ... add layers to your model ... # suppose we have some test data in X_test predictions = model.predict(X_test) # convert probability distributions into class labels for multi-class class_predictions = np.argmax(predictions, axis=-1)
To apply it for binary classification, where you’re dealing with yes/no outcomes, you could round the output from
predict()
:
# binary class labels binary_class_predictions = np.round(predictions)
In conclusion, although the error message “
AttributeError: 'Sequential' object has no attribute 'predict_classes'
” might appear daunting at first, the issue arises from specific methods being removed from the Keras Sequential Model API and the solution is relatively straightforward – switch to using
predict()
and standard numpy functions to determine class labels based on the output probabilities.Sometimes we find ourselves in situations where we’d like to predict the class labels for a given test dataset using Keras’
Sequential
model. You’ve probably tried something along these lines:
model = Sequential() ... prediction = model.predict_classes(test_data)
Only to encounter an error message:
AttributeError: ‘Sequential’ object has no attribute ‘predict_classes’
Why does this occur? Let’s start by revisiting the purpose of
predict_classes()
.
The
predict_classes()
method was used with Keras models to return the class predictions for the input samples, as opposed to returning the probabilities which
predict()
outputs. It was indeed handy because it helped speed up workflows in binary or multi-class classification problems by bypassing the manual process of converting predicted probabilities into class labels.
However, the recent changes made to Keras API led to the deprecation of the
predict_classes()
function. As a result, trying to call this method on a `Sequential` object will yield an
AttributeError
. Hence, the error statement: “Keras AttributeError: ‘Sequential’ object has no attribute ‘predict_classes'”.
So, how do you go about predicting class labels now? Even without the
predict_classes()
function, you can still predict class labels just as smoothly. Below are steps that detail how to achieve this:
Step 1: Start with model.predict()
Firstly, we must obtain the class probabilities. We use the `predict()` function here.
probabilities = model.predict(test_data)
This will generate an array of prediction probability values per label.
Step 2: Convert Probabilities to Class Labels
Next, we convert these probabilities to class labels. For binary classification, we’d typically use the threshold value of 0.5.
binary_predictions = [1 if prob >= 0.5 else 0 for prob in probabilities]
For multiple classes (multi-class classification), we’d get the index of the highest probability value using the `argmax()` numpy function. This presumes class labels are ordinally encoded (i.e., 0, 1, 2,…, N-1 for N classes).
import numpy as np multi_class_predictions = np.argmax(probabilities, axis=-1)
And voila! There you have it – a way to predict class labels without using
predict_classes()
.
In conclusion, while it might initially seem confusing when old methods become deprecated, advancements such as these are often beneficial in improving the efficiency and capabilities of such powerful libraries like Keras. The misconception around the usage of
predict_classes()
highlights the dynamic nature of Python development environments and the importance of keeping up-to-date with best practices.
Keep these code transitions in mind as you continue to explore other aspects of Keras and happy coding!
Deep learning libraries like Keras provide a variety of methods to predict classes for given data. While
predict_classes
was a popular function in older versions of Keras, you will now find that it is missing if you’re using recent versions. A common error we run into is the infamous
AttributeError: 'Sequential' object has no attribute 'predict_classes'
. This leaves us frantically searching for an alternative method.
Understanding AttributeError:
Python throws an
AttributeError
when we try to access or call a non-existing attribute in an object. In this case, the
'Sequential'
model in Keras does not contain an attribute/method called
predict_classes
, hence the error.
The source of this peculiarity lies in the internal changes made with the advent of TensorFlow 2.0 and above. In the newer versions, native Keras got assimilated within Google’s TensorFlow leading to certain functions getting deprecated/removed. One such casualty was
predict_classes
.
What was Predict_Classes?
Predict_classes
was a method that would output the class index with the highest probability for each input. Here’s how its code might have looked:
predictions = model.predict_classes(data)
Modern Alternatives to Predict_Classes:
In order to deal with this change, we can use other methods that essentially perform the same purpose as but in a slightly different format, such as
predict
and
argmax
. Let’s get down to comparing them:
Method | Description | Code Snippet |
---|---|---|
predict |
Predict generates output predictions for the input samples. It returns the probabilities for each class rather than the class index. |
probabilities = model.predict(data) |
argmax |
np.argmax function in numpy module finds the maximum value along the specified axis from the numpy array and returns the index containing it. By pairing it with predict , we effectively mimic predict_classes . |
predictions = model.predict(data) predicted_classes = np.argmax(predictions, axis=-1) |
The modification here is pretty straightforward. First we obtain the probability distribution over the classes via the
predict
function. Then we capture the index location of the maximum probability which essentially gives us our predicted class.
Summary:
Though the absence of
predict_classes
might feel like a setback, it’s easily counteracted. You may use
predict
in combination with
np.argmax()
seamlessly replace
predict_classes
. The functionality remains unchanged while enhancing your flexibility to customize your prediction process!
As always, don’t hesitate to dig into the beautifully vast Keras API documentation whenever you need help navigating through the choppy waters of Keras.
The
AttributeError: 'Sequential' object has no attribute 'predict_classes'
you encountered in Keras refers to your attempting to use the method
predict_classes
on a model of type Sequential. This issue usually arises when using updated versions of Keras or TensorFlow.
Previously in Keras,
predict_classes
was used as an easy way of outputting the class predictions for a set of input data directly. However, this function was removed from later versions of the frameworks due to design changes and optimizations.
Methods to Resolve This Issue:
1. Replace
predict_classes
with
np.argmax()
In Python,
argmax()
is a method from NumPy library that returns the indices of the maximum values along an axis. So, instead of using
model.predict_classes()
, use
numpy.argmax(model.predict(x), axis=-1)
. An example may look like this:
import numpy as np
predictions = np.argmax(model.predict(x_test), axis=-1)
Here,
model.predict()
outputs the confidence scores for each class, and
np.argmax()
turns those into concrete class predictions by returning the index of the highest confidence for each input.
2. Downgrade to an older version of Keras or TensorFlow
You could also consider downgrading to a previous version of Keras or TensorFlow where
predict_classes
is still supported. This can be done with pip by specifying the version number as follows:
pip install keras==2.3.1
Please note that this approach might cause compatibility issues if your project uses other elements from newer versions.
3. Write a custom predict_classes function
If you would like to keep working with the existing
model.predict()
function, yet require a convenience function similar to
predict_classes()
, you could write your own implementation:
def predict_classes(x): proba=x if proba.shape[-1] > 1: return proba.argmax(axis=-1) else: return (proba > 0.5).astype('int32')
Sources:
When dealing with predictive modelling using Keras, an often encountered error message is: “Attribute Error: ‘Sequential’ object has no attribute ‘predict_classes'”. This can be a significant roadblock for beginners and seasoned coders alike. The good news? We can easily avoid it by implementing several best practices to our data science workflow. Here’s an in-depth look:
1. Use Appropriate Methods for Prediction:
In Keras library versions 2.3.0 and above, the ‘predict_classes’ method isn’t accessible for Sequential model objects. To resolve this issue, we can opt for the ‘predict’ method instead.
This is an illustration on how you’d use it:
[code]
# Assuming that ‘model’ is your trained Keras sequential model
predictions = model.predict(x_test)
predicted_classes = np.argmax(predictions, axis=1)
[/code]
Here, ‘predict’ generates the class probabilities and ‘numpy.argmax’ would then find the class with the highest probability.
2. Consistently Update Your Libraries:
Keras, along with supporting libraries like TensorFlow, frequently receives updates which carry necessary bug fixes, enhanced functionalities, and syntax improvements. Consistent software updates are instrumental in minimizing errors.
[code]
# For updating Keras
pip install –upgrade keras
# For updating Tensorflow
pip install –upgrade tensorflow
[/code]
Remember to restart your coding environment after each update for changes to take effect.
3. Proper Documentation and Reading:
Software libraries including Keras constantly evolve, with their functionalities changing over time. Reading up-to-date documentation and online community forums is beneficial (You can bookmark the Keras API Reference). Employing deprecated functions could lead to unnerving errors, such as “AttributeError: ‘Sequential’ object has no attribute ‘predict_classes’.”
4. Embrace Efficient Coding Practices:
It’s advantageous to adhere to efficient coding practices using pylint or another linter. Avoid hard-coding values and utilize exception handling to anticipate potential issues. Doing so allows for versatile code that can easily adapt to different datasets or changes in APIs.
5. Instilling a Robust Testing Culture:
A robust testing culture can significantly mitigate development cycle bugs. When adding new features or debugging, ensure that the original functionality remains uncompromised. Unit tests offer a safety net and facilitate maintainable, error-free coding.
6. Learning from Others:
Lastly, borrowing ideas from well-established public codebases can provide alternative solutions and contribute positively to your development skills. Numerous open-source projects on platforms like GitHub showcase high-standard coding styles and industry best practices.
Through employing these essential measures and embracing continual learning, navigating through common hurdles with Keras – such as the infamous AttributeError – becomes manageable. Happy modelling!I profoundly hope that the discussion on the Keras AttributeError: ‘Sequential’ object has no attribute ‘predict_classes’ was insightful. There are a few scenarios when you encounter this error:
– When using an outdated version of Keras where the method ‘
predict_classes
‘ doesn’t exist.
model.predict(np.argmax(x_test, axis=-1))
– If your model’s final layer activation function isn’t softmax and you are dealing with multi-class classification.
When addressing this issue, it’s crucial first to ascertain the version of Keras installed in your working environment. Remind yourself that ‘
predict_classes
‘ is replaced by ‘
argmax
‘ if the output layer of your model uses a ‘softmax’ function. Alternatively, directly use ‘
predict
‘ as shown below,
classes_x=np.argmax(model.predict(x_test), axis=-1)
Necessarily, professional coders should keep track of software updates and API changes for all libraries utilized in their projects. This is key to minimizing errors encountered during the coding activity.
Table 1 elucidates how these situations can be managed effectively.
Error Scenario | Solution |
---|---|
Keras Version Outdated | Update Keras to the latest version or adjust the codebase according to the used version. |
Final Activation Function Not ‘softmax’ | Ensure the usage of ‘softmax’ activation function in the output layer for multi-class classification problem. |
Mindful analysis of API documentation, such as the one available on Keras API Models guide, could better ameliorate these error situations. Stay engaged and get better at understanding these fault signals; they’ll form part of our routine in building better machine learning models.
Remember, our primary goal here is to alleviate complications while working on machine learning projects. Equal attention should be given to all aspects – from preprocessing input data to generating the final prediction. The slightest change can significantly affect the entire performance. Hopefully, these explanations will help you meet that target more efficiently.
Sources:
- Keras Documentation: https://keras.io/api/models/