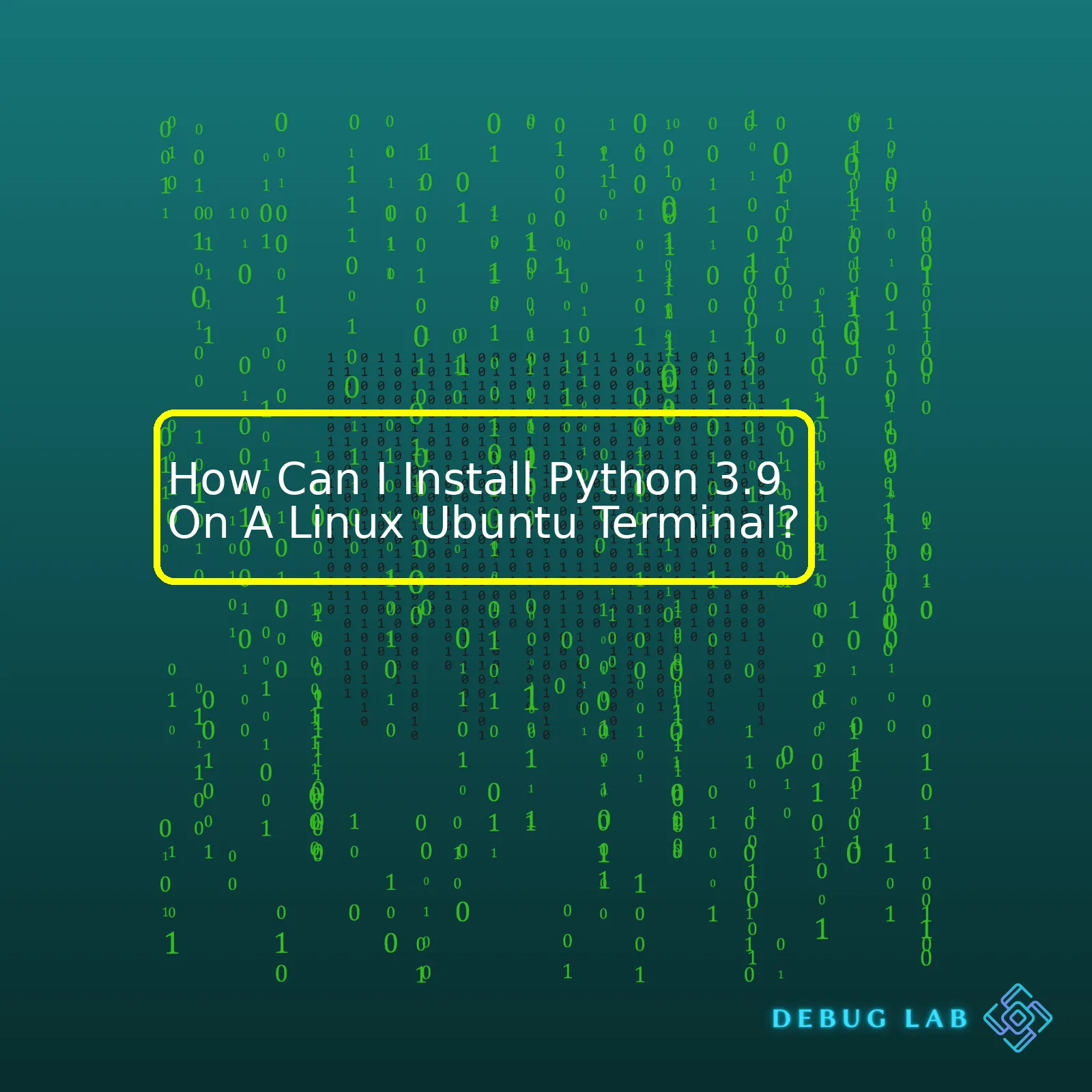
Let’s start by creating an HTML table where each row of the table will represent one step in the installation process.
Action | Command |
Update package list |
sudo apt update |
Install prerequisites |
sudo apt install software-properties-common |
Add deadsnakes PPA to your sources’ list |
sudo add-apt-repository ppa:deadsnakes/ppa |
Update package list again |
sudo apt update |
Install Python 3.9 |
sudo apt install python3.9 |
Check Python version |
python3.9 --version |
Now, let us go through the rows of above table one by one in more detail:
The first step when installing anything new is to make sure that the system’s package list is updated, so we run the
sudo apt update
. Next, we install additional software that enables us to easily manage our repositories using the
sudo apt install software-properties-common
command.
In order to get Python 3.9, we’ll want to add a third-party repository known as the deadsnakes PPA to our sources’ list. This can be achieved by running
sudo add-apt-repository ppa:deadsnakes/ppa
.
Once we’ve added the repository, we do another update with
sudo apt update
to include packages from the newly added repository in the package list.
With everything set up correctly, we finally install Python 3.9 by executing
sudo apt install python3.9
.
Finally, to confirm successful installation, you can check the installed Python version by running command
python3.9 --version
. This should display the installed version of Python which should be Python 3.9.
References
- [Python Download and Installation Guide](https://www.python.org/downloads/)
- [Ubuntu Software Packages for Python](https://packages.ubuntu.com/search?keywords=python3)
Sure, here’s a dive into the features and enhancements that come with Python 3.9 and a guide on how to install it on a Linux Ubuntu terminal.
Python 3.9: Features and Enhancements
Python version 3.9 debuted with various enhancements and new features that make coding much efficient for developers. Here are some powerful features:
String methods: Python 3.9 introduced two string methods –
removeprefix()
and
removesuffix()
. These help to eliminate the need of slicing strings or using the replace() method when one needs to remove substrings from the start or end of the main string.
For example,
str = 'pythonista' print(str.removeprefix('py')) # outputs 'thonista' print(str.removesuffix('ista')) # outputs 'python'
Dictionary update: The union operators
|
and
|=
are now allowed in the dictionary. This facilitates merging dictionaries without employing complex codes.
For example,
# Merging dictionaries using | dict1 = { 'a': 1, 'b': 2 } dict2 = { 'b': 3, 'c': 4 } merged_dict = dict1 | dict2 print(merged_dict) # outputs {'a': 1, 'b': 3, 'c': 4}
Parameter Specification in Decorators: In Python 3.9, you can specify parameters in decorators. This will enhance the readability of your code.
Improved Time Zone Handling: Python 3.9 has brought improvements in time zone handling with the addition of an IANA time zone database in the Standard Library.
Installing Python 3.9 on Linux(Ubuntu)
To install Python on a Linux (Ubuntu) Terminal, follow these steps:
– Start by updating package lists for upgrades and new installations. Run this command in the terminal:
sudo apt update
– Then download required packages necessary for the installation to be processed correctly. Use this command:
sudo apt install build-essential zlib1g-dev libncurses5-dev libgdbm-dev libnss3-dev libssl-dev libreadline-dev libffi-dev libsqlite3-dev wget libbz2-dev
– Now, fetch Python 3.9 source archive:
wget https://www.python.org/ftp/python/3.9.0/Python-3.9.0.tgz
– Extract the gzipped archive using following command:
tar -xf Python-3.9.0.tgz
– Switch into the directory as follows:
cd Python-3.9.0
– Now run configure script which automatically checks whether all the dependencies needed to build the application are present or not. It creates a Makefile based on the detected and available components:
./configure --enable-optimizations
– Lastly, Run this command to complete the installation process:
sudo make altinstall
– Once done, verify it by checking the version installed:
python3.9 --version
That’s pretty much about how one can leverage Python 3.9 and its enhancing features. Also, you can see how easy it is to install Python 3.9 on a Linux Ubuntu terminal. These steps provide a hands-on approach for installing Python while exploring other additional tools and their utilization. Easy peasy, huh?
For reference, more details on Python 3.9 and its features can be found on the Python documentation page here.
Installing Python, an increasingly essential language for many developers and systems administrators, on your Ubuntu device is an easy task if you know the right commands. One key thing to note though is that Ubuntu 20.04 and other versions come preinstalled with Python 2.7 and Python 3.8; however, some of the latest applications might require Python 3.9 for their dependencies.
Streamlining Python 3.9 Installation on Linux Ubuntu Terminal
To streamline this process within the terminal, let’s break it down into manageable steps:
Step 1: Update Package List
Before anything else, update your package list. This ensures you get the latest version of Python when you install it:
sudo apt update
Step 2: Software Properties Installation
Next, install software properties common package. This helps in managing your distribution and independent software vendor software sources:
sudo apt install software-properties-common
Step 3: Adding Deadsnakes PPA
Deadsnakes is a PPA (Personal Package Archive) that allows you to install multiple versions of Python on Ubuntu. It contains Python packages not included in the Ubuntu repositories and updates them more frequently.
Add Deadsnakes PPA to your system by running this command:
sudo add-apt-repository ppa:deadsnakes/ppa
Press enter when it prompts. Now, update your package list again to include packages from the newly added Deadsnakes PPA:
sudo apt update
Step 4: Install Python 3.9
Now you are ready to install Python 3.9:
sudo apt install python3.9
Congratulations – you’ve installed Python 3.9 on your Ubuntu machine!
Verify the Installation
After you’ve done all these, always make sure to verify the installation. This step is important to ensure that Python 3.9 has been successfully installed and is working as expected. Checking can be done using the following command which outputs the version installed:
python3.9 --version
Making Python 3.9 as Default Version
If you intend to use Python 3.9 as your default Python version, you need to update-alternatives via the following set of commands:
sudo update-alternatives --install /usr/bin/python3 python3 /usr/bin/python3.8 1 sudo update-alternatives --install /usr/bin/python3 python3 /usr/bin/python3.9 2
The command
update-alternatives
maintains symbolic links determining default commands. The –install option is followed by link, name, path and priority.
- Link: symbolic link pointing to the file.
- Name: name of the master link.
- Path: alternative being introduced for the master link.
- Priority: order in which alternatives are considered if there’s no manual override.
Certain applications still rely on older versions of Python and might malfunction if Python 3.9 is set as the default version. Therefore, do this only if necessary.
Remember that constant practice not only improves your efficiency in working with Ubuntu but also enhances your understanding. So, take every opportunity to learn by doing various installations and configurations on your own.
Even though Python comes pre-installed in most Linux distributions, knowing how to manually install a specific version is a crucial skill for any Linux user, especially those interested in web development and data science where newer versions of Python may be required.
For more detailed documentation on Python installation process and management, please refer to the official Python documentation page.
Further reading:
- Official Ubuntu documentation: Managing packages link.
- Python.org: Docs for Python installation and usage link.
Installing Python 3.9 on a Linux Ubuntu terminal involves very specific prerequisites and steps to ensure the successful execution of the installation process. By understanding these conditions and the required process, you can effectively use the terminal to install Python 3.9 without any hitches.
Enlightening on the prerequisites for installing Python 3.9 on Linux Ubuntu:
– A working Linux Ubuntu system: This serves as the platform where the Python version will be installed. An apt update and upgrade is recommended before initiating the Python installation to have the system equipped with the latest software patches.
sudo apt-get update && sudo apt-get upgrade
– Sufficient disk space: The appropriate storage space should be made available in your Linux Ubuntu environment for the Python 3.9 software and its libraries.
– Terminal Access: You need access to the Linux terminal command line interface (CLI) because Python 3.9 installation occurs through the command input in this interface.
– Basic command-line knowledge: The command-line tool requires fundamental knowledge on navigating Linux Ubuntu using commands since the entire installation process is facilitated through the terminal commands.
Having mentioned the prerequisites, let’s focus on the exact steps required to install Python 3.9 on Linux Ubuntu terminal:
– First you need to refresh your local package index so you are installing the most recent version:
sudo apt-get update
– Next, Install the dependencies which are necessary for python installation:
sudo apt-get install -y make build-essential libssl-dev zlib1g-dev libbz2-dev \ libreadline-dev libsqlite3-dev wget curl llvm gettext libncurses5-dev \ libncursesw5-dev xz-utils tk-dev libffi-dev liblzma-dev python-openssl git
– Download the Python version by using wget command:
wget https://www.python.org/ftp/python/3.9.0/Python-3.9.0.tgz
– Extract the downloaded file using tar command:
tar -xf Python-3.9.0.tar.xz
– CD into the directory:
cd Python-3.9.0
– Execute a configure script which checks the system whether the dependencies required to run python are installed:
./configure --enable-optimizations
– Initiate build process:
make
– Finally, install the built program:
sudo make install
If you followed the steps correctly, Python 3.9 should be installed successfully on your Ubuntu terminal. To verify this:
python3.9 --version
This should display “Python 3.9.x” which verifies that the version has been installed properly.
Please note, without a basic understanding of Linux commands, this process can be challenging; therefore, it’s important to familiarize yourself with basic Linux commands (Ubuntu Terminal Basics). Also ensure you have permissions and rights within Linux to execute these commands, as insufficient rights could cause the installation to fail.It’s a breeze to install Python 3.9 on your Linux Ubuntu terminal! You’re a few commands away from enjoying the latest features, speed enhancements, and optimizations that Python 3.9 can offer for your coding efforts.
Firstly, you will need to update your system’s package list with the following command:
sudo apt update
Then, upgrade the available packages as needed:
sudo apt upgrade
Following this, you’ll want to ensure that the necessary software which lets us download packages over HTTPS is installed:
sudo apt install software-properties-common
With the prerequisites done, it’s time for an important step: adding the deadsnakes PPA to your system’s source list. This repository has multiple Python versions that we can use. Run this command:
sudo add-apt-repository ppa:deadsnakes/ppa
After pressing enter for the prompt, your system now knows where to grab Python 3.9. Let the actual installation proceed by running this command:
sudo apt install python3.9
That should do it! A process of downloading and installing Python 3.9 begins. Using the ‘python3.9 –version’ command allows you to confirm its successful installation once done.
python3.9 --version
If everything went smoothly, you’ll see the Python version (3.9.x) printed in the terminal.
Worth mentioning is that to use the new Python in the Linux terminal, you’ll likely want to use the command ‘python3.9’ specifically, as ‘python’ or ‘python3’ may still refer to older versions you have installed.
Now, you’ve successfully installed Python 3.9 on your Ubuntu system, ready for development. Happy Coding!
Reference:
Python Software FoundationInstalling Python 3.9 on a Linux Ubuntu Terminal may seem like a daunting task for many, but with the right approach, it can be simplified and a number of common errors circumvented. Here’s how you go about it, along with some strategies to counteract frequent pitfalls:
Firstly, Ubuntu systems generally come with a version of Python pre-installed. You can check this by typing
python3 --version
. If it already exists, you may need to update it or install Python 3.9 alongside the existing installation.
The steps mentioned below will take you through the process of installing Python 3.9:
1. Update Package Lists
Before we dive into the installation, it’s crucial to ensure all package lists are up-to-date on your Linux system.This minimizes the chances of encountering issues due to older versions of packages.
sudo apt update
2. Library Pre-requisites
Before installing Python 3.9, you need to ensure certain necessary libraries and utilities are present on your Ubuntu machine. Installation error can occur if these prerequisites aren’t met.
sudo apt install -y build-essential zlib1g-dev libncurses5-dev libgdbm-dev libnss3-dev libssl-dev libreadline-dev libffi-dev wget libbz2-dev
3. Download Python 3.9
Next, you need to download Python 3.9. This step can fail in case of internet connectivity issues or if the specific Python URL has been moved or removed.
wget https://www.python.org/ftp/python/3.9.7/Python-3.9.7.tgz
4. Extract the file
This step involves extracting the tar ball file downloaded in the previous step.
tar -xf Python-3.9.7.tgz
5. Build and Install Python 3.9
Finally, configure and make the extracted source code files and then install Python 3.9.
cd Python-3.9.7 ./configure --enable-optimizations make sudo make altinstall
Bear in mind that during the ‘make’ stage, a variety of compilation errors can surface, especially if system libraries, packages, or dependencies are missing or outdated.
To append Python 3.9 into your system’s path, execute this:
nano ~/.bashrc
And add
alias python=python3.9
at the end. Press ‘Ctrl+X’, then ‘Y’, and finally ‘Enter’.
At this point, it’s fair to say the installation has led you onto a safer road. But remember! From internet connectivity issues leading to partial downloads, to missing packages causing failed dependencies, to permission errors requiring super user rights – any number of potential obstacles can deter a smooth Python installation.
Fret not though! They may sound complicated, but error messages provide vital information about what may have gone wrong during installation. Cross-verifying every step against these detailed instructions should equip you with the means of overcoming such common errors.
By keeping the foresaid precautions in mind, you’ll find yourself a step closer towards mastering Python 3.9 on the Linux Ubuntu Terminal. For more comprehensive resources on Python installations, head over to its official documentation.
Python is a highly versatile language and one of the key benefits of Python is the plurality and diversity of its libraries. When it comes to compatibility, the majority of open-source libraries are compatible with Python 3.9. Libraries such as NumPy, pandas, SciPy, Scikit-learn, etc., are all compatible with Python 3.9. It’s always essential to regularly check library compatibility because an incompatible Python library might render your code unusable.
However, your query seems to focus on how you can install Python 3.9 on a Linux Ubuntu terminal first. Linux distros, including Ubuntu, usually come preinstalled with python. However, they may not always ship with the latest version and that’s where manual installations become necessary. Here’s how you can manually install Python 3.9 on Ubuntu:
sudo apt update sudo apt install software-properties-common sudo add-apt-repository ppa:deadsnakes/ppa sudo apt install python3.9
The above steps ensure that Python gets updated in your system. To confirm the successful installation and your Python version, type in the command:
python3.9 --version
This should display the installed version which should be Python 3.9.
Once you have your Python environment ready, you can start installing Python libraries. For instance, to install a library like NumPy use:
pip install numpy
Pip is a package manager for Python and is used to install and manage software packages/libraries written in Python. Note, however, that different libraries have different requirements and installation processes. Ensure to refer to their respective documentation for more information.
To conclude, while installing Python 3.9 manually on Ubuntu isn’t a trivial process, the value it delivers, especially when working with recent versions of Python libraries, is immense. Be sure to keep tabbing the Python libraries’ documentation for compatibility issues, updates, and changes. You can also refer to the PyPI website for additional details about specific Python packages.
When you’re a programmer, it’s crucial that you stay updated about Python and its different versions. An excellent example of this is Python 3.9, the latest in the line of Python’s releases. But, why should you choose Python 3.9?
Python 3.9: Key Features
- More accurate typing:
dict[str, int]
is equivalent to
typing.Dict[str, int]
now.
- New String methods:
removeprefix()
and
removesuffix()
for managing prefixes and suffixes conveniently.
- New Parser: Python 3.9 introduces a new parser, called PEG-Based parser, which results in better error messages and facilitates the introduction of new syntax features.
- Zoneinfo module: It makes support for Iana time zones available.
- Merging dictionaries: Python 3.9 allows two dictionaries to be merged using the union operator(‘|’).
All these attributes of Python 3.9 make the new version a more powerful language than its previous iterations. The strong type indications, better errors loggings, zoneinfo module, and merging dictionaries, enable developers to optimize their coding duties.
Coming to the question of how to install Python 3.9 on Linux Ubuntu terminal. It can be achieved quite conveniently by following these steps:
1. Update package lists for upgrades and new installations from repositories:
sudo apt update
2. Install prerequisites using the command:
sudo apt install software-properties-common
3. Add deadsnakes PPA to your system’s Software Source:
sudo add-apt-repository ppa:deadsnakes/ppa
4. Finally install Python 3.9 with:
sudo apt install python3.9
Please note that Linux distributions like Ubuntu might still use an older version as the default Python installation. This means that when you type python into your terminal, it might not invoke Python 3.9. To access Python 3.9, you might have to use
python3.9
instead.
So, keeping up-to-date with Python releases such as Python 3.9 can be hugely advantageous both in professional programming and hobbyist projects. Its advanced features offer an impressive array of functionalities that enrich the Python ecosystem, while also being more efficient and responsive.
References:
- What’s New In Python 3.9 — Python 3.9.0 documentation
- How to Install Python 3.9 on Ubuntu 20.04 | Linuxize
Alright, let’s dive into the details of installing Python 3.9 on a Linux Ubuntu terminal.
Start by updating packages index to ensure you have grabbed the latest version with:
sudo apt update
Next, proceed to install prerequisites for Python before installing it:
sudo apt install software-properties-common
Add Deadsnakes PPA to your system’s sources list by typing:
sudo add-apt-repository ppa:deadsnakes/ppa
You will receive a prompt asking for your confirmation to add the outlined repos. Press
Enter
to proceed. Now that you have added all necessary resources, you can now move to the installation step. Type:
sudo apt install python3.9
To confirm your successful installation, you need to check your Python version. To do so, run the command:
python3.9 --version
If the installation was successful, you would see something similar to Python 3.9.x to signify the installed version.
As such, you’ve taken the proper steps to install Python 3.9 on a Linux Ubuntu Terminal efficiently.
Command | Description |
---|---|
sudo apt update |
Update package lists for upgrades and new package installations. |
sudo apt install software-properties-common |
Install package with necessary libraries for python installation. |
sudo add-apt-repository ppa:deadsnakes/ppa |
Add Deadsnakes PPA to your source list for accessing python versions. |
sudo apt install python3.9 |
Install Python 3.9 using the updated package lists. |
python3.9 --version |
Check whether Python has been installed successfully. |
This knowledge is instrumental not only in setting up your Linux Ubuntu terminal but also in creating an environment conducive for Python development. Remember, ensuring that your core tools are set correctly helps prevent future mistakes in the design process, irrespective of what level of Python programming you’re at; beginner, intermediate, or expert.
Keep an eye out for regular updates to Python; Python.org provides relevant information about the latest versions available for download. It’s always advisable to employ the most recent stable release to utilize all new features and security fixes present.
Installing Python on a Linux Ubuntu terminal sound complex? Don’t fret! Follow this guide, stay patient and you’ll have Python 3.9 running smoothly on your Linux Ubuntu terminal. Keep coding!