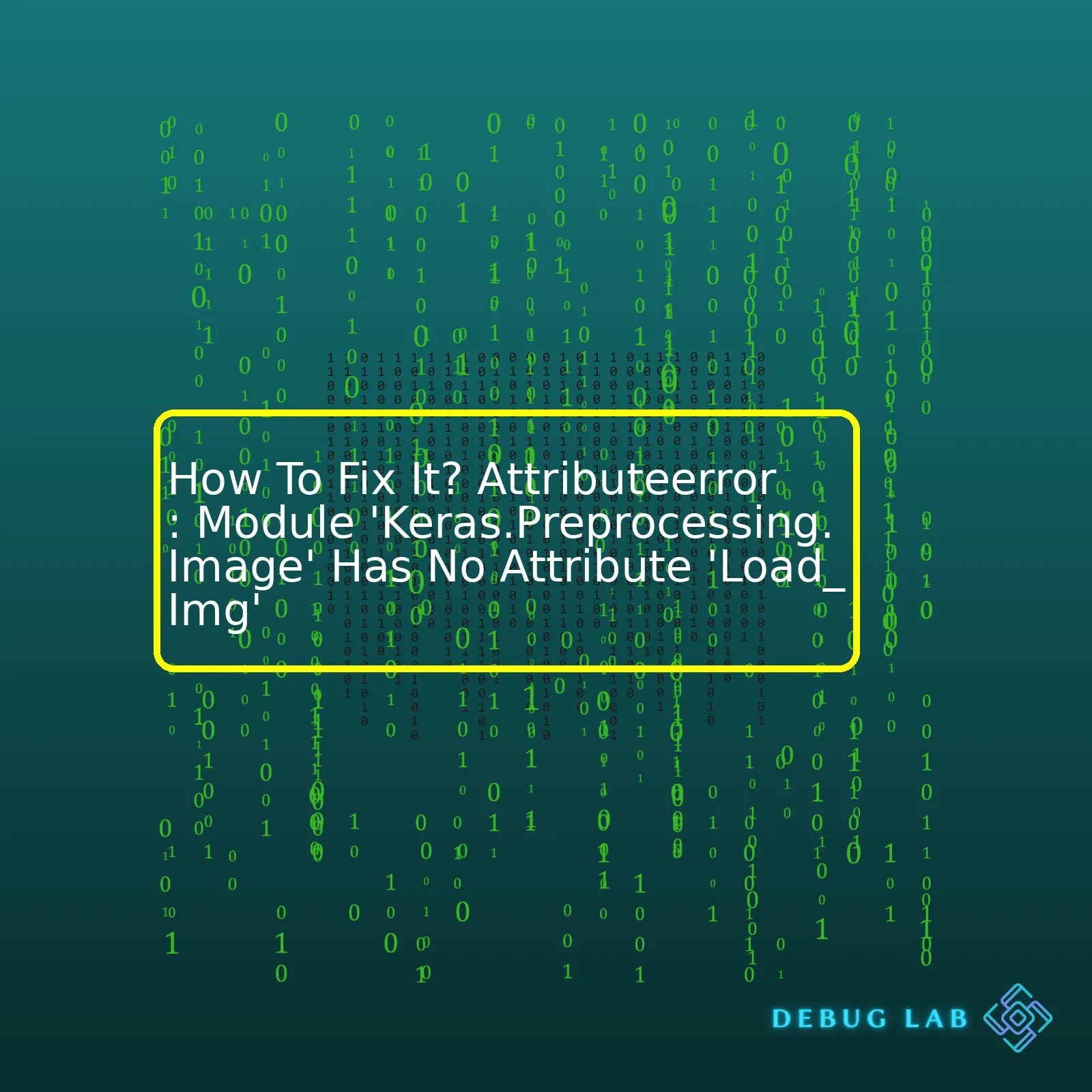
We’ll kick things off with the main crux of the matter: the error typically results from either an outdated Keras or TensorFlow installation, incorrect importing methods, non-compliance with the ‘tf.keras’ syntax, or the inexistence of loaded image data.
Error Causes | Solutions |
---|---|
Outdated Keras/TensorFlow installation | Update Keras by running
x 1 1 pip install keras --upgrade . Repeat the procedure for TensorFlow if necessary. |
Incorrect Import Method | Use
xxxxxxxxxx 1 1 1 from keras.preprocessing import image instead of xxxxxxxxxx 1 1 1 import keras.preprocessing.image.load_img . |
Non-compliance with ‘tf.keras’ syntax | Use
xxxxxxxxxx 1 1 1 from tensorflow.keras.preprocessing import image . Avoid mixing imports between standalone Keras and tf.keras as their objects aren’t interchangeable. |
Inexistent Loaded Image Data | Check your image path and run
xxxxxxxxxx 1 1 1 image.load_img('path_to_your_image') . |
To counter the `Attributeerror: Module ‘Keras.Preprocessing.Image’ Has No Attribute ‘Load_Img’` error in Python scripts involving deep learning techniques with Keras libraries, it’s crucial one comprehends why and when the error arises. When working with Keras, a mighty deep learning library, we may have to load or preprocess an image using the `load_img` function from the `keras.preprocessing.image` module. However, if the Keras or TensorFlow version installed doesn’t support loading or you’ve misinterpreted the right way to bring in the function, the mistake crops up.
Hence, always ensure that your Keras and TensorFlow installations are up-to-date. TensorFlow 2.x versions, for instance, conveniently come with Keras packaged within them—this compact integration is ideal but necessitates consistency in syntax when calling Keras functionality.
Lastly, maintaining the validity of image data is equally essential. Incorrect file paths will lead to unsuccessful data loading efforts—a challenge we address by confirming our image path is correct and that the targeted images indeed exist where we believe they do. Invest time in structuring your data correctly and ensure your code references it accurately.
Solving this error involves not only technical solutions like updating Keras or revising import syntax but also the mindful organization of your workspace, including understanding how TF 2.x works with its included Keras package and ensuring proper loading and referencing of your image dataset [source].
To sum up: always employ the correct syntax, arrange your data systematically, keep your libraries updated, and be vigilant about referencing—all these tactics will hold you in good stead while solving the problem at hand.Before diving into the solution, let’s analyze the issue first. The error
xxxxxxxxxx
AttributeError: Module 'Keras.preprocessing.image' has no attribute 'load_img'
typically arises when you try to import and use the function
xxxxxxxxxx
load_img()
from the module Keras.preprocessing.image, but Python cannot find it. This usually happens because:
– The Keras library is not correctly installed in your python environment.
– A different version of the Keras library is installed than the one required for the function
xxxxxxxxxx
load_img()
.
– Conda environment is mixed with pip which can result in version confusions.
This problem is all about managing dependencies and versions efficiently.
First and foremost, do the ground check: Is Keras properly installed? You can test this by simply creating a new Python script and typing:
xxxxxxxxxx
import keras
If there are no errors, then Keras should be installed properly. Now, head over to the second phase, where we need to make sure that Keras can find the method
xxxxxxxxxx
load_img
. In your Python terminal, type:
xxxxxxxxxx
from keras.preprocessing.image import load_img
If an AttributeError occurs, then it certainly means that the
xxxxxxxxxx
load_img
function is not available in your installed version of Keras.
So how do we resolve this? There are three effective approaches:
– Simply upgrade the keras library:
Open the command prompt and type
xxxxxxxxxx
pip install --upgrade keras
– It might be possible that
xxxxxxxxxx
load_img
does exist, but in a different location altogether. For some versions of Keras, the function resides in
xxxxxxxxxx
keras_preprocessing.image
. Therefore, replacing the problematic line with this could possibly solve the issue.
xxxxxxxxxx
from keras_preprocessing.image import load_img
– There could be a chance that there is a conflict between Anaconda and Pip as both manage packages and environments. If you have installed Python libraries using conda and pip both, then they tend to overwrite each other’s data leading to conflicts like Keras module not being recognized despite having it installed.
Attribute errors typically occur due to the absence of an attribute in the current version of the library or a failure during the installation process. Analyzing these details and systematically working through a solution will eventually lead you to success in resolving this error.
Remember, dealing with such bugs demand patience, basic understanding, and strategic trial-and-error approach. Happy debugging!For you to fully comprehend the ‘AttributeError: Module ‘keras.preprocessing.image’ has no attribute ‘load_img” error and its root causes, we need to delve into some technical details. You’re most likely hitting this error because you’re attempting to use the
xxxxxxxxxx
load_img
function in Keras’s image preprocessing module, but Python can’t discover this attribute.
The underlining possibilities that could be inducing this error encompass the following:
– Keras might not be installed correctly in your system.
– You could be working with an outdated version of Keras. The ‘load_img’ attribute was included in later versions.
In order for us to unravel these issues, please follow these steps:
1. Confirm that Keras is properly installed:
Have a look at your Python environment and ensure Keras is appropriately installed. Initiate with importing Keras and printing its version:
xxxxxxxxxx
import keras
print(keras.__version__)
If Keras isn’t correctly installed or if it fails to load, consider reinstalling it.
2. Updating Keras:
When running older versions of Keras you won’t have access to the ‘load_img’ attribute since it was introduced only in recent updates. To resolve this, update Keras to the latest version:
xxxxxxxxxx
pip install --upgrade keras
Once updated, try once again to import and utilize the
xxxxxxxxxx
load_img
function from the
xxxxxxxxxx
keras.preprocessing.image
module:
xxxxxxxxxx
from keras.preprocessing.image import load_img
If you have correctly followed these two steps, your problem should stand resolved. However, if the error sustains, you may look at installing specific versions of tensor flow as suggested by some experts. You can refer to an insightful StackOverFlow discussion on this issue. Generally, updating should resolve this issue as Keras developers have altered the syntax over time. In some prior versions, you had to import ‘load_img’ from ‘keras.preprocessing.image’, whilst in newer versions you could access it directly from ‘keras.preprocessing’. Hence, reiterating, please try to stay current with libraries and modules updates.
Finally, always remember that Google is your best friend when encountering errors while coding! Make sure to search for the error message first. There’s a huge possibility someone else must have experienced the same issue and might have found a viable solution that you can apply as well.Sure. The Python Keras.preprocessing.image library plays an essential role in simple data importation and pre-processing as it equips the developers with various functions that allow for efficient manipulation of images, all aiding in successful model training. One such function is `load_img`, which is used to load an image into PIL (Python Imaging Library) format.
However, one common hiccup while working with these libraries is an `AttributeError` surfacing as: `Module ‘Keras.Preprocessing.Image’ has no attribute ‘Load_img’`. This error message essentially means exactly what it’s stating – the interpreter cannot find the attribute `load_img` in the module specified.
Here are some ways you might be able to remedy this issue:
– First and foremost, ensure your Keras version isn’t outdated. The `load_img` function was incorporated from version 2.x onwards and isn’t available in earlier iterations. Updating Keras might prove to be the easiest fix:
xxxxxxxxxx
pip install --upgrade keras
– Another possibility could be spelling or case mistakes, as Python is case-sensitive. The correct format of calling the function is `load_img`, not `Load_img`:
xxxxxxxxxx
from keras.preprocessing.image import load_img
image = load_img('input.jpg')
– Miscommunication between TensorFlow and Keras could also be a problem. Keras has been officially integrated into TensorFlow since version 2.0, effectively making the standalone Keras package somewhat redundant over time. Therefore, when intending to work with Keras under TensorFlow, import the necessary libraries like so:
xxxxxxxxxx
from tensorflow.keras.preprocessing.image import load_img
img = load_img('input.jpg')
If none of the aforementioned solutions work, I’d recommend starting afresh and reinstalling both Keras and TensorFlow packages, preferably using a virtual environment. This helps isolate dependencies and virtually eliminate any conflicts with other versions if existing.
By gaining context about the core functionality of the said Python library and better understanding the reasons behind the AttributeError, we can easily troubleshoot it in just a few steps improving the efficiency of our work in Python.
Several resources can guide you on the installation and essential usage of these libraries (TensorFlow Official Guide ), the management of Python environments (Virtual Environments), and provide additional information on the topic (Keras Preprocessing Functions).The error “AttributeError: module ‘keras.preprocessing.image’ has no attribute ‘load_img'” occurs typically when you’re trying to use the `load_img` function under `keras.preprocessing.image` in Python. The reason for this problem could be due to several scenarios which we’ll get to. But first, understanding the structure of your code can lend a solution.
Let’s consider this code:
xxxxxxxxxx
from keras.preprocessing.image import load_img
image = load_img(path)
Now, let’s talk about the potential reasons behind this error and how to fix it:
– Old Keras version: If you are using an older version of Keras, it’s possible that the `load_img` function might not be available in that version. To solve this, upgrade your Keras to the latest version by running:
xxxxxxxxxx
pip install --upgrade keras
– Misinterpretation of the function name: It’s possible you could’ve mistaken ‘load_img’ with ‘Load_img’ or some other format. Ensure the correct method as defined in the package is being used. Here, make sure it’s `load_img` and not any other form.
– Importing from the wrong place: There are instances when you may end up importing from the wrong place or misspelling the import path, resulting in an AttributeError. Always check your import statements. Make sure your import statement looks like this:
xxxxxxxxxx
from keras.preprocessing.image import load_img
– Keras replaced with TensorFlow: In some newer versions of Tensorflow, Keras has been integrated within Tensorflow package, meaning you need to import Keras from Tensorflow. In this case, adjust your import statement to :
xxxxxxxxxx
from tensorflow.keras.preprocessing.image import load_img
To diagnose what’s exactly causing the AttributeError in your scenario, I’d suggest printing the content of the module ‘keras.preprocessing.image’ with
xxxxxxxxxx
dir()
like so :
xxxxxxxxxx
import keras.preprocessing.image
print(dir(keras.preprocessing.image))
This will print out all attributes of the image module and you can check if ‘load_img’ is in there. If it’s not present, then one of the explanations mentioned above would apply here.
By being aware of these problems, you have more tools at your disposal when you encounter issues like this in the future. Happy coding!
Further reading on the documentation regarding Keras preprocessing here.To fix the AttributeError: Module ‘Keras.preprocessing.image’ has no attribute ‘load_img’, it might be the case that you have not properly installed Keras or have a conflicting version of the module.
However, for those who want to use alternative methods to load images in Keras, there are various methods available.
One simple and straightforward way is by using the ImageDataGenerator class, which allows the users to instantiate generators of augmented image batches (and their labels) via .flow(data, labels) or .flow_from_directory(directory). These generators can then be used with the methods like fit_generator(), evaluate_generator() etc.
Here’s a chunk of code to exemplify:
xxxxxxxxxx
from keras.preprocessing.image import ImageDataGenerator
datagen = ImageDataGenerator()
train_it = datagen.flow_from_directory('data/train/', class_mode='binary')
Another method is to directly rely on modules from TensorFlow 2. Given that Keras is part of TensorFlow core API since version 2, you can use tf.keras.preprocessing.image. This can help both load and preprocess the images, regardless of whether they are color or grayscale. The “load_img” method returns a PIL (Python Imaging Library) instance.
For instance:
xxxxxxxxxx
from tensorflow.keras.preprocessing.image import load_img
img = load_img('image.jpg')
What’s important is to ensure that the names of the directories from where the images are loaded and their paths match exactly what you’re providing in the code. If not, you may run into errors or your code might not perform as expected.
Finally, yet another alternative would be to use OpenCV’s imread function. Let me illustrate this in code:
xxxxxxxxxx
import cv2
img = cv2.imread("path_to_your_image.jpg")
These methods should certainly serve as good alternatives for loading images if the traditional approach isn’t working due to version issues or installation problems with Keras.
Remember, in order to prevent encountering similar AttributeErrors in future, it is critical to keep your installed libraries updated. You can use pip install –upgrade [library] for this purpose. For example, updating Keras would look like:
xxxxxxxxxx
pip install --upgrade keras
By following these methods, problems related to the error message indicating that keras.preprocessing.image has no attribute ‘load_img’ should be resolved effectively.This error
xxxxxxxxxx
AttributeError: module 'keras.preprocessing.image' has no attribute 'load_img'
typically arises when the `load_img` method cannot be located within the keras preprocessing image module. This is likely due to a range of reasons including:
– Incorrect import statements
– Incompatibility between module versions
– Misconfiguration of environment or dependencies
Let’s delve into the details and provide potential solutions to each issue.
Incorrect Import Statements
Starting at the most basic level, this AttributeError can occur if the image preprocessing module of Keras is improperly imported.
The correct way to import this specific function is:
xxxxxxxxxx
from keras.preprocessing.image import load_img
By doing so, you have directly imported the `load_img` function from the keras library.
Alternatively, you might import the entire module:
xxxxxxxxxx
from keras.preprocessing import image
In this case, you would need to use `image.load_img` to use `load_img`.
Incompatibility Between Module Versions
If you’ve verified the import statement isn’t the issue, it may be an incompatibility problem. Version mismatches often happen when updating Keras or TensorFlow libraries.
Perhaps an older version of Keras is installed that does not include `load_img` as an attribute of `keras.preprocessing.image`. To resolve this, consider upgrading your Keras version to the latest. Do note that you also need to comply with the correct version of TensorFlow that pairs with the updated Keras.
Use the following command to upgrade your Keras library:
xxxxxxxxxx
pip install --upgrade keras
Carefully select TensorFlow’s version if any conflict arises. Check through Python how you would do for Keras with
xxxxxxxxxx
import tensorflow as tf
print(tf.__version__)
Misconfiguration of Environment or Dependencies
Another scenario behind this error could be the misconfiguration of your development environment or conflicts among dependencies.
It’s usually recommended to create isolated environments while working on diverse projects. Try creating a new virtual Python environment, reinstalling all required dependencies there starting with TensorFlow and Keras.
Check this link to learn about creating and managing Python virtual environments. Follow proper installation instructions from the official websites of Keras and TensorFlow.
Aforementioned steps are expected to help debug and fix the Attribute Error while trying to use `load_img` method from Keras Image Preprocessing package. If problem still persists, always consider reaching out to the active communities, forums and groups available online or recheck your solution against examples available in the official Keras documentation.AttributeErrors in Python point to a module, class, or function not having the attribute that we’ve referenced. In this case, the error message is “AttributeError: module ‘keras.preprocessing.image’ has no attribute ‘load_img'”, meaning the ‘keras.preprocessing.image’ module does not possess or cannot locate the ‘load_img’ attribute. To solve this issue and prevent potential AttributeErrors in the future, we can perform the following actions.
1. Update Your Dependencies:
Firstly, always make sure your dependencies are up-to-date. The most common source of these errors is outdated libraries, functions, or classes. The attribute ‘load_img’ may exist in an updated version but not in an older one, causing your error. Use pip to update your Keras library with the command:
xxxxxxxxxx
pip install --upgrade keras
2. Correct Import Statements:
The ‘load_img’ attribute is not found within ‘keras.preprocessing.image’. Even a small spelling or grammatical mistake can result in AttributeError. Make sure you use proper casing since import statements are case-sensitive. Always check the syntax:
xxxxxxxxxx
from keras.preprocessing.image import load_img
To prevent future issues, understand the structure and contents of the modules and classes you are utilizing. This understanding will come handy in identifying incorrect attributes, if there are any, in your code.
3. Python Path:
Python needs to know the exact location of your imported module. If it’s in a different directory, Python won’t be able to locate it, resulting in AttributeError. To prevent this error in future, make sure that the Python files you are trying to access are in the correct directory. You can do this by checking the PYTHONPATH environmental variable.
4. Module Caching:
One less known cause can be Python’s caching mechanism. When Python imports a module for the first time, it creates a .pyc file in the __pycache__ folder. It uses this file instead of the original .py file upon subsequent imports to speed things up. If the .pyc file was created when some method didn’t exist yet in the .py file, you’ll still get an AttributeError even after updating the .py file. Remove the cached file(s) manually to solve this.
5. Understand Python Object Model:
Building a solid foundation of Python’s object model will go a long way in preventing AttributeErrors. Understand how Python’s import statement works, how modules and packages are structured, how namespaces work, the difference between methods and attributes, etc. This knowledge will help you predict possible causes and prevent AttributeErrors in your projects.
6. Last But Not Least, Debugging:
When all else fails, good old debugging will save the day. Libraries like pdb (`import pdb; pdb.set_trace()`) allow you to step through your code line by line, examine variables at each step, and figure out where things go wrong. It’s a valuable skill to have, regardless of the particular issue at hand.
Remember that errors are part of the coding process. Troubleshooting them makes us better coders, nurtures our problem-solving skills, and deepens our understanding of the language and its intricacies. Let’s embrace them as learning opportunities rather than fear them. Happy coding!There are a few ways to solve the AttributeError: Module ‘Keras.Preprocessing.Image’ Has No Attribute ‘Load_Img’ in Python. You don’t necessarily need to be an experienced software developer to fix it. All you need is some basic understanding of Python programming and follow these instructions:
Option 1: Try importing load_img directly from keras.preprocessing module like below:
xxxxxxxxxx
from keras.preprocessing import image
img = image.load_img(path, target_size=(224, 224))
Here, we use the Keras Image module to load images efficiently. This should eliminate the error since we call the
xxxxxxxxxx
load_img
function directly from the keras.preprocessing.image library.
Option 2: Use PIL/pillow library instead of Keras. Here is how it looks:
xxxxxxxxxx
from PIL import Image
img = Image.open(path)
The Image.open() method opens and identifies the given image file. This is actually the primary function with the PIL (PILLOW) library. But remember to install the pillow package with
xxxxxxxxxx
pip install pillow
command if not installed already.
Remember that Keras is a high-level neural networks API capable of running on top of TensorFlow, CNTK, or Theano; designed to enable fast experimentation with deep neural networks (Reference). Learning to navigate and understand its modules is critical for efficient coding in Python.
If the issue still isn’t resolved, it might be related to your specific project setup. In this case, consider isolating the problem. Try running the code in a fresh Python environment. If it works there, something within your current set up is causing the issue. To create a fresh Python environment, you may want to look into virtual environments in Python. There are many online resources and tutorials (Reference) to guide you in setting it up properly. You just assured yourself more versatile python coding skills!
Issue troubleshooting results in an enriched understanding of the languages and tools we’re utilizing. It hones your skill set and expands your knowledge scope in the vast field of coding. And the best part – you learn to anticipate problems ahead of time and prepare preventive measures. After all, every potential bug fixed upgrades you as a coder!
Let’s keep the codes rolling, and the bugs under control! Happy fixing!