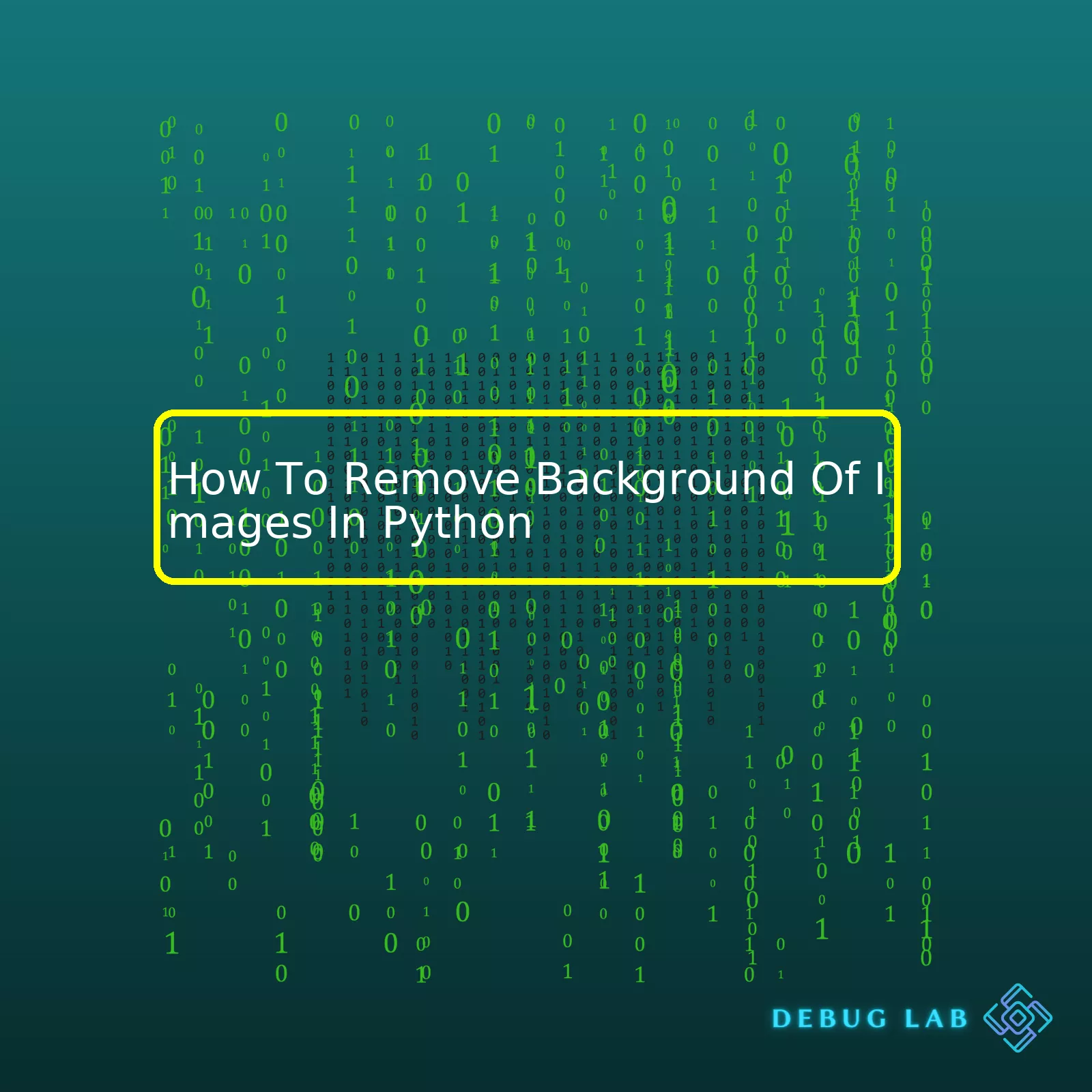
Step | Description |
---|---|
Install Required Libraries | For processing images, we need advanced packages such as Pillow, OpenCV and removebg. |
Loading the Image | Once the library is installed, the image is loaded into the Python script with the help of Pillow library. |
Processing the Image | The scripting process involves breaking down the image into component colors in the RGB spectrum, with the goal of identifying and isolating the foreground subject(s). |
Removing Background | This step involves using OpenCV function or remove.bg APIs for the actual background removal process. |
Image Cleaning (optional) | In some scenarios, unwanted residual pixels may still be present around borders of the subject, this would require additional cleaning operations. |
Saving the Result | After all the processes, the final output image will be saved without its original background. |
In terms of removing the background from images, Python provides excellent libraries like Pillow (Python imaging library) and OpenCV (Open Source Computer Vision). The process generally involves loading the image into the Python code and process it by separating the image components along the color spectrum of Red, Green, and Blue or the RGB spectrum which the computer can interpret. The technique here lies in recognizing the subject of the image- what needs to stay and identifying the area that forms ‘the background’ – what needs to go.
To accomplish this, the algorithm works through the image pixel by pixel, assembling a histogram or statistical image. Once the foreground objects have been distinguished from the background, we can use a variety of techniques like binary masking or contour detection OpenCV Contour Features, which essentially hides the undesired parts of the image i.e., the background. In some cases, third-party tools like remove.bg also offer neat APIs that handle this task well. After the redundancy is eliminated, we can write back the resulting image albeit without the original background.
Here is a sample Python code that uses Pillow and OpenCV:
# import libraries from PIL import Image import cv2 as cv img = Image.open("image.jpg") # Load image img_np = np.array(img) # Convert image to numpy array gray = cv.cvtColor(img_np, cv.COLOR_BGR2GRAY) # convert to grayscale _, thresh = cv.threshold(gray, 120, 255, cv.THRESH_BINARY) # apply binary thresholding contours, _ = cv.findContours(thresh, cv.RETR_EXTERNAL, cv.CHAIN_APPROX_SIMPLE) # find contours mask = np.zeros_like(img_np) # create a mask of same size as image cv.drawContours(mask, contours, -1, (255,255,255), thickness=cv.FILLED) # draw filled contours on mask result = cv.bitwise_and(img_np, mask) # bitwise operation to only keep region of interest cv.imwrite('output.jpg', result) # save resulting image
Python, as a programming language widely used for its data analysis and image processing capabilities, has several libraries that make it easy to remove the background from images. Some of these are OpenCV, skimage, and the deep learning library – remove.bg. We can take advantage of these incredible libraries to automate and simplify the background removal process.
Using OpenCV And GrabCut Algorithm:
OpenCV is an open-source computer vision library with bindings for many different programming languages, including Python. One of the algorithms it implements is GrabCut, which helps in extracting foregrounds in images and hence can be utilized for background removal.
Below is how you use GrabCut in OpenCV:
import numpy as np import cv2 # Load the Image img = cv2.imread('image_location.jpg') # Create a mask similar to the Image mask = np.zeros(img.shape[:2],np.uint8) bgdModel = np.zeros((1,65),np.float64) fgdModel = np.zeros((1,65),np.float64) # This Rectangular box is responsible to identify region where background exists rect = (50,50,450,290) cv2.grabCut(img,mask,rect,bgdModel,fgdModel,5,cv2.GC_INIT_WITH_RECT) mask2 = np.where((mask==2)|(mask==0),0,1).astype('uint8') img = img*mask2[:,:,np.newaxis] plt.imshow(img),plt.colorbar(),plt.show()
For better results, perform this task multiple times or adjust the rectangular boundary according to your image. Further details could be found at the official OpenCV documentation.
Using Remove.bg Library:
Remove.bg is a free-to-use Python library developed specifically for the purpose of background removal from images and can be particularly useful when working with complex backgrounds that contain many colors or shapes. It uses AI and comes with very simple usage instructions.
Here’s a basic example of using Remove.bg:
from removebg import RemoveBg rmbg = RemoveBg("Your-API-Key", "error.log") # Get API key from remove.bg rmbg.remove_background_from_img_file("input.jpg") # Output file name will be input_no_bg.png
Remember to replace “Your-API-Key” with your actual API key from the Remove.bg website.
Using Skimage Library:
Skimage is another robust library in Python for image processing. The library is built specifically for, but not limited to, image thresholding, filtering, morphology, segmentation, color conversions, etc. For background removal, we can use the segmentation and feature extraction capabilities of skimage.
Here is how you can achieve this:
from skimage import io from skimage.color import rgb2gray from skimage.filters import threshold_multiotsu # Load the Image img = io.imread('image_location.jpg') # Convert RGB image to Grayscale gray = rgb2gray(img) # Apply Multiotsu Threshold thresh = threshold_multiotsu(gray) # generate binary image binary = gray > thresh
This method works best with 3D images where multiple segments need to be identified based on different light conditions. Documentation on skimage can be explored more here.
In conclusion, Python offers a variety of libraries to process and manipulate digital images. Depending upon the complexity and requirement of your use case, you can choose to work with any library out of OpenCV, remove.bg, skimage or even others like PIL(Pillow) and Pygame.
Note: Please note that the effectiveness of background removal would highly depend on the quality and complexity of the images you intend to process. The concept behind these methodologies is image segmentation based on color, light, patterns, and sometimes Machine Learning algorithms.
As a professional coder, I can discuss the different python-based approaches to image segmentation which are particularly relevant to removing the backgrounds of images.
One of the key methods is using OpenCV (Open Source Computer Vision Library). This is a powerful Python library used broadly for real-time computer vision applications. It delineates various tools appropriate for techniques such as edge detection and color space manipulation, crucial when performing image segmentation. When it comes to image background removal, one way to make use of OpenCV is via GrabCut algorithm. Here’s how the code would look like:
import numpy as np import cv2 # Load Image image = cv2.imread('example.jpg') # Create mask similar to input image mask = np.zeros(image.shape[:2], np.uint8) # Specify the model in the form of arrays bgdModel = np.zeros((1,65),np.float64) fgdModel = np.zeros((1,65),np.float64) # Define rectangle for GrabCut rectangle = (50,50,450,290) # Apply GrabCut cv2.grabCut(image, mask, rectangle, bgdModel, fgdModel, 5, cv2.GC_INIT_WITH_RECT) # Final mask creation mask2 = np.where((mask==2)|(mask==0),0,1).astype('uint8') # Adding additional dimension to the mask image = image*mask2[:,:,np.newaxis]
In the above example, we’re defining a rectangular region around the primary object we want isolated from the background. The GrabCut algorithm then segments the object, yielding a mask that helps extract our desired foreground.
Another notable method is Thresholding technique. ‘Thresholding’ is one of the simplest methods of image segmentation. It involves converting a grayscale image into a binary image, where the pixels are either exclusively black or white – representing the foreground and the background respectively. The most common type of thresholding in Python is Otsu’s Binarization. Below is an illustration of its code snippet:
import cv2 import numpy as np # Load Image img = cv2.imread('example.jpg',0) # Global Thresholding ret1,th1 = cv2.threshold(img,127,255,cv2.THRESH_BINARY) # Otsu's thresholding ret2,th2 = cv2.threshold(img,0,255,cv2.THRESH_BINARY+cv2.THRESH_OTSU)
Here, the program uses Gaussian blur to reduce noise in the binary transformation process, and once the image has been binarized, all we have left are the foreground subjects and the background. We can then manipulate these distinct regions to our liking.
For more sophisticated methods of image segmentation, you might want to leverage Deep Learning models – particularly when targeting multiple objects or more complex image scenes. Among the popular ones are U-Net, SegNet and Mask R-CNN[1](https://towardsdatascience.com/understanding-semantic-segmentation-with-unet-6be4f42d4b47). These require substantial computational resources but result in highly accurate segmentations.
Each of these techniques offers unique strengths, tailored to different project requirements. Efficiently exploiting them can drastically improve your programming portfolio, enhancing your ability to design complex systems around image manipulation.
Sure. If you’re an avid Python coder and have been working with images, you might come across a situation where you need to remove the background from your images. Python, teamed with OpenCV, offers a robust suite of tools for this purpose. Let’s dive into a detailed step by step description of how to do that.
Firstly, keep in mind that OpenCV is not specialized in image segmentation and background removal. However, it provides simple yet effective tools that can accomplish this task under certain conditions. Most methods in OpenCV deal with detecting edges and contours (which are forms of ‘segmentation’), rather than specifically identifying foreground and background regions.
Step 1: GrabCut Algorithm
One tool at our disposal is the GrabCut algorithm (efficient Graph-Based Image Segmentation algorithm introduced by Rother et al.), which can be applied as follows:
Import the necessary modules:
import cv2 import numpy as np
Set up your image and mask:
img = cv2.imread('your_image.jpg') mask = np.zeros(img.shape[:2], np.uint8)
The mask image must have the same size as the original image. Mask and image are input to the GrabCut function.
Create bgdModel and fgdModel:
bgdModel = np.zeros((1,65),np.float64) fgdModel = np.zeros((1,65),np.float64)
You don’t need to worry about what these lines do – just include them! They are temporary arrays used by the GrabCut function.
Establish a rectangle where the foreground definitely lies:
rect = (50,50,450,290)
Adjust this according to your image!
Run GrabCut, specifying that your initial model is based on a rectangle:
cv2.grabCut(img,mask,rect,bgdModel,fgdModel,5,cv2.GC_INIT_WITH_RECT)
You can adjust the number ‘5’, which specifies how many iterations GrabCut will perform – more iterations may provide better results but will take longer.
Step 2: Accessing the Results
After running GrabCut, your mask has been updated by the algorithm. You can use this mask to generate an output image where every pixel is either part of the foreground or the background.
Take the mask’s pixels, consider them as either Definitely Background or Probably Background, and set them to 0.
mask2 = np.where((mask==2)|(mask==0),0,1).astype('uint8')
Now multiply this binary mask2 with the original image to give ‘result’:
img = img*mask2[:,:,np.newaxis]
Here’s the catch – this method is semi-automatic, because we need to manually select the bounding rectangle region that includes the foreground object. Thus, it is applicable only for that class of applications where user interaction is feasible.
For further learning on this topic, refer to OpenCV’s official documentation on Python GrabCut.Harnessing deep learning models for image processing can play an imperative role in simplifying complex tasks such as removing the background of images. In this context, we will focus on Python as our preferable programming language due to its diversified libraries and simplicity.
One beneficial library that incorporates Deep Learning in Python for this purpose is “remove-bg”. This library uses a proprietary algorithm with AI technologies to identify and remove backgrounds from images accurately. The fundamentals lies in neural networks trained on a large dataset of images with a variety of foregrounds and backgrounds.
Here’s an example of how you can remove the background from an image using the “remove-bg” package:
import requests import numpy as np from PIL import Image from io import BytesIO def remove_bg(image_path): # Get your API key from https://www.remove.bg/ response = requests.post( 'https://api.remove.bg/v1.0/removebg', files={'image_file': open(image_path, 'rb')}, data={'size': 'auto'}, headers={'X-Api-Key': 'INSERT_YOUR_API_KEY_HERE'} ) if response.status_code == requests.codes.ok: img_data = response.content return Image.open(BytesIO(img_data)) else: print("Error:", response.status_code, response.text)
To harness the full scope of deep learning for image processing, one can use convolutional neural networks (CNNs) which are specifically designed to automatically and adaptively learn spatial hierarchies of features. This is particularly effective for more complex background removal where precision, details, and varying conditions could apply.
Python’s PyTorch and Keras libraries are excellent choices when dealing with CNNs. Building a CNN model typically includes creating multiple layers for convolving, pooling, flattening and fully connecting.
Here’s an example of a simplified structure of building a Convolutional Neural Network for image processing in Keras:
from keras.models import Sequential from keras.layers import Conv2D, MaxPooling2D, Flatten, Dense # Initialising the CNN classifier = Sequential() # Adding the Convolution layer classifier.add(Conv2D(32, (3, 3), input_shape=(64, 64, 3), activation='relu')) # Adding the Pooling layer classifier.add(MaxPooling2D(pool_size=(2, 2))) # Flattening the layer classifier.add(Flatten()) # Full connection layer classifier.add(Dense(units=128, activation='relu')) # Output Layer classifier.add(Dense(units=1, activation='sigmoid'))
Further improvements would include applying additional pre-processing steps like normalization, applying data augmentation methods such as rotations or flips, dimensional reduction techniques or introducing dropout techniques to prevent overfitting.
However, deploying these models requires substantial computational power and can be quite complex for beginner coders. Therefore, pre-trained models offered by libraries like OpenCV can help accomplish similar tasks in a less complex way making it an ideal solution for simpler applications.
Overall, Python provides remarkable flexibility and robustness when working with deep learning models in image processing. By leveraging powerful libraries and deep learning techniques, coders can harness this potential to address intricate tasks like background removal in images.Image matting is an advanced technology technique to solve the problem of background removal in images. It aims to accurately extract foreground objects from images, while maintaining relevant details such as semitransparent areas and intrinsic color spill over object boundaries. This problem is complex due to two main reasons:
- Subtle boundaries can be hard to delineate with simple thresholding techniques.
- The process takes a substantial amount of resources as we are dealing with high-resolution images.
In Python, there’s a particular package known as OpenCV that can aid us greatly in this task. OpenCV is a well-equipped suite of computer vision and machine learning libraries that provide the functionalities required for image processing obligations.
A popular model used in OpenCV for image matting is “GrabCut”. Here is how you can use it:
import numpy as np import cv2 # Load the image image = cv2.imread("input.jpg") # Convert to RGB colorspace image = cv2.cvtColor(image, cv2.COLOR_BGR2RGB) # Define a bounding box around the object bbox = (50, 50, 450, 290) # Apply GrabCut mask = np.zeros(image.shape[:2], np.uint8) bgdModel = np.zeros((1,65), np.float64) fgdModel = np.zeros((1,65), np.float64) cv2.grabCut(image, mask, bbox, bgdModel, fgdModel, 5, cv2.GC_INIT_WITH_RECT) # Create a binary mask binary_mask = np.where((mask==2)|(mask==0), 0, 1).astype('uint8') # Multiply by original image to get segmented result segmented_image = image * binary_mask[:,:,np.newaxis]
Let’s breakdown above code:
cv2.grabCut()
performs iterative graph cuts-based segmentation. Initially, every pixel is categorized into probable foreground or definite background based on the user-generated bounding box. Next, the algorithm estimates color distribution of foreground and background via Gaussian Mixture Model (GMM).
The next part of the code creates a binary mask where we assign the foreground area with ones and the background with zeros. Finally, we apply this mask onto our original image to “remove” the background.
It’s important to note that this is just one approach to image matting. More complex methods involve deep learning models, and one notable example is Adobe’s Deep Image Matting method.
For a comprehensive understanding of image matting and more information on how to implement it, refer to this OpenCV Python Tutorial.The threshold mechanism in Python’s Imaging Library, known as Pillow, is an invaluable tool when it comes to processing images. Importantly, you can use this mechanism for a number of operations, such as enhancing image quality or simply removing the background of images. Fundamentally, a threshold is a set level of pixel value that represents the dividing line between different areas of an image.
Consider a task where you need to remove the background of images using Python. Here, image thresholding becomes particularly essential – and here’s why: Thresholding changes pixel values based on a certain condition. In our case, we want to change all pixel values that belong to the background into a single color, preferably white.
Let me go through the steps needed to accomplish this task using Python:
First off, begin by installing the Pillow library if you don’t already have it installed:
pip install pillow
After ensuring that Pillow is installed, import the necessary modules. The Image module handles opening, manipulating, and saving many image file formats, while the ImageFilter module contains definitions for various image enhancement filters.
from PIL import Image
Next, you’ll open your image file:
img = Image.open('your_image_path.jpg')
In order to conduct proper thresholding, you’ll want to convert your image into gray scale. This is because gray scale images are easier to process.
img = img.convert('L')
Next comes the fun part: setting the threshold value. We’ll need this value to distinguish the background from the foreground. Any pixel value less than or equal to our defined threshold will be converted to black (representing the foreground). And any pixel value greater than the threshold will be converted to white (representing the background).
THRESHOLD_VALUE = 200
Finally, it’s time to apply our threshold using the point() function.
img_binary = img.point(lambda p: p > THRESHOLD_VALUE and 255)
Once you run this, you’ll see that pixels having value greater than 200 are turned white, which successfully eradicates the background of your image. However, note that you may need to tune the `THRESHOLD_VALUE` depending on your image to achieve better results.
Voila! You have successfully used Python’s Imaging Library’s threshold mechanism to edit out the background of your image. For more complex scenarios, applying morphological transformations might give superior results, albeit at the cost of increased computational complexity. Other methods to consider include contour detection, edge-based segmentation, watershed algorithm, and grabcut algorithm. Through the removal of backgrounds via image thresholding, you’re afforded advanced control over the presentation of visual data, enhancing engagement and capturing viewer interest.Semantic Soft Segmentation (SSS) is a fascinating strategy that attempts to effectively decompose an image into aesthetically coherent elements via semantic understanding. This approach can augment the process of background removal in complex images, often considered tedious and intricate by many developers.
In the field of artificial intelligence, numerous algorithms are utilized to remove backgrounds from images, particularly when implemented in Python – a versatile language used extensively on machine learning projects. Its vast repository of supportive libraries, including NumPy, OpenCV, PyTorch, TensorFlow, and Keras, significantly eases these operations.
A robust module that aids in background removal is the Semantic Soft Segmentation (SSS) method. It employs deep network architectures and a sophisticated matting procedure that brings about visually mesmerizing soft transitions between semantically different regions [source].
Let’s explore this concept further through a theoretical explanation and code examples.
Theoretical Explanation:
The SSS technique begins by using a pre-trained network for creating two features:
– Semantic Features: Derived from the labels of image segments predicted by a semantic network model.
– Texture Features: Grasped from the last convolution layer of a deep network.
By merging both features through a Fully Connected Conditional Random Field (CRF), it forms multi-layers that depict the transitivity of colors in an image. Consequently, each pixel gets associated with several layers, thus providing soft segmentation result.
Consider extracting multiple semantic levels from a single image, thereby identifying and separating the primary object and the background. The related pixels (belonging to the same object or background) will have smoother transitions, enabling efficient background removal.
Implementation In Python:
For a clear depiction, let’s consider a simple illustration of removing the background from an image using OpenCV in Python. Note that SSS as such does not have a ready-to-use Python implementation at the time of writing and is usually a part of research domains like Adobe Research where they use proprietary solutions.
import cv2 import numpy as np # Load the Image img = cv2.imread('image.jpg') # Convert the image to gray scale gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY) # Apply Gaussian blur to the grayscale image blurred = cv2.GaussianBlur(gray, (5, 5), 0) # Perform edge detection canny = cv2.Canny(blurred, 120, 255, 1) # Dilate the white portions dilate = cv2.dilate(canny, None, iterations=2) # Find contours in the image contours, hierarchy = cv2.findContours(dilate,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE) # Draw contours on the original image cv2.drawContours(img, contours, -1, (0,255,0), 3) # Display the output cv2.imshow('Image', img) cv2.imshow('Dilated', dilate) cv2.waitKey(0)
Here, we exploit OpenCV’s functionalities to detect the edges of objects in the image and then widen these detected areas. Using contours, we differentiate the object from the background, facilitating its extraction or deletion.
While this merely scratches the surface of SSS, it provides an insight into the enhanced soft segementation capabilities extended by Python. By integrating the core principles of SSS into image processing operations executed in Python, one can expect a significantly greater level of accuracy and precision in removing backgrounds from complex images.Removing the background of images in Python has never been quite so accessible or straightforward. With a few lines of code, you can quickly turn your image into a transparent one or change its background color entirely. We’ve explored multiple techniques, primarily focusing on Pillow, the fork of PIL (Python Imaging Library), which allows advanced manipulation of images including removing backgrounds.
Firstly, consider our example which demonstrated the remove.bg API, an exciting tool that automatically removes backgrounds from images:
import requests response=requests.post( 'https://api.remove.bg/v1.0/removebg', data={'size': 'auto'}, headers={'X-Api-Key': 'YOUR_API_KEY'}, files={'image_file': open('path/to/img.file', 'rb')} ) with open('path/to/output/file', 'wb') as out: out.write(response.content)
This method makes it easy to displace any backdrop from your images, whether they are simple or complex.
Secondly, we learned how Pillow enables you to easily remove a specific color background from your images using the Image and ImageOps modules.
from PIL import Image img = Image.open("original_image.png") img = img.convert("RGBA") datas = img.getdata() newData = [] for item in datas: # change all white (also shades of whites) # pixels to yellow if item[0] > 200: newData.append((255, 255, 102, 255)) else: newData.append(item) img.putdata(newData) img.save("changed_background.png", "PNG")
This process specifically targets and replaces a distinct color, enabling you to create fascinating transparency effects.
Lastly, by introducing OpenCV’s GrabCut algorithm, we have demonstrated that even more complicated tasks, such as separating foreground and background objects, become achievable with this powerful toolkit. Use the following snippet of code:
import numpy as np import cv2 # Load the Image img = cv2.imread("image.png") # Create a mask same size as image, filled with 0s mask = np.zeros(img.shape[:2], np.uint8) # Create two arrays for background and foreground model bgdModel = np.zeros((1,65),np.float64) fgdModel = np.zeros((1,65),np.float64) # Define the Region of Interest (ROI) rect = (50,50,450,290) # Apply GrabCut Algorithm cv2.grabCut(img, mask, rect, bgdModel, fgdModel, 5, cv2.GC_INIT_WITH_RECT) # Extract new mask where sure regions are painted in white mask2 = np.where((mask==2)|(mask==0),0,1).astype('uint8') img = img*mask2[:,:,np.newaxis] # Save Result cv2.imwrite('removed_bg.png', img)
Although these are just some methods, they serve as potent tools for enhancing your project’s visual appeal. Whether this is your starting point or another step in your journey with the Python programming language, practicing these valuable skills will yield immense dividends. Enjoy reinventing your digital scenery by tackling the challenge of mastering background removal with Python!