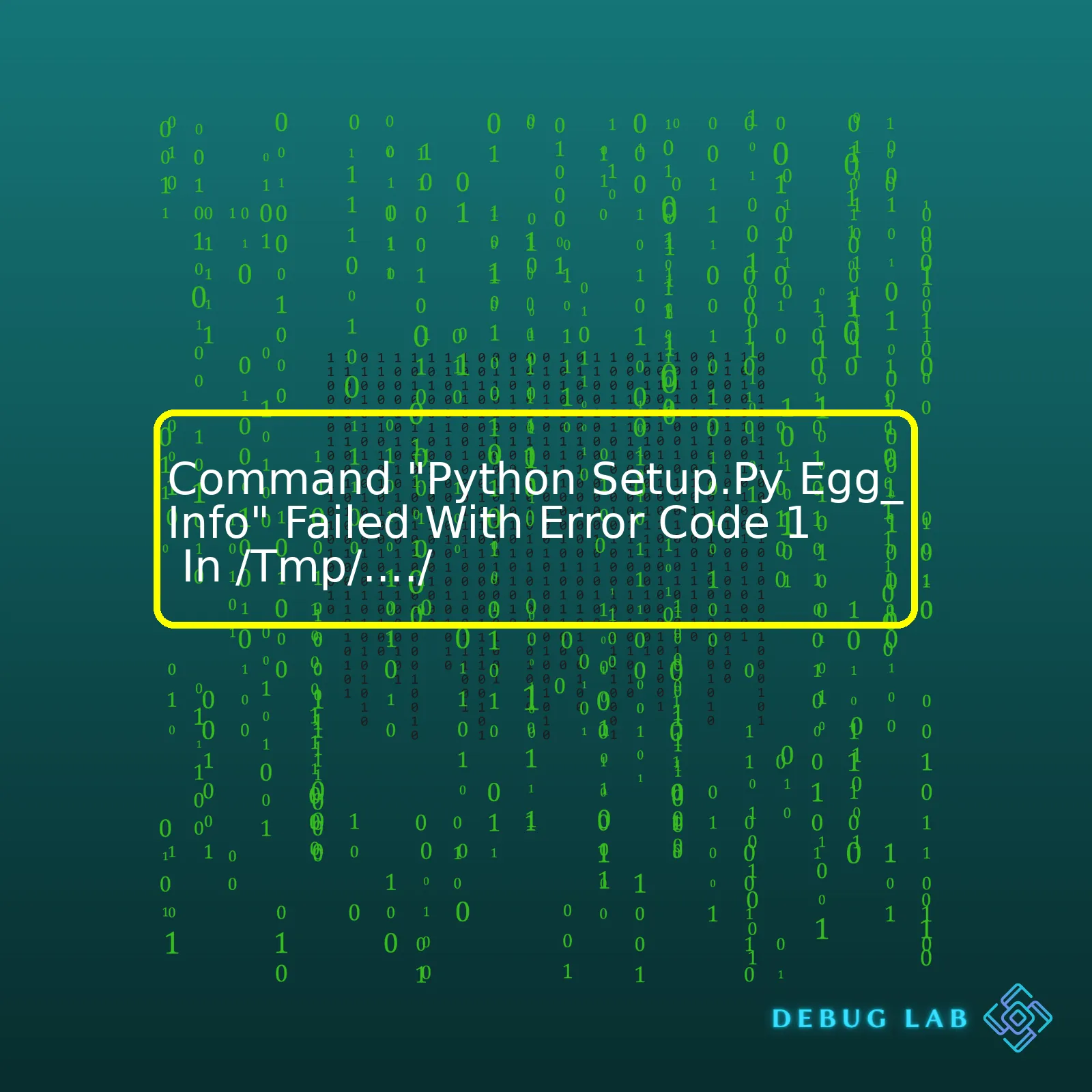
Here’s how such an HTML table would look:
Error Message | Common Causes | Possible Solutions |
---|---|---|
“Command “python setup.py egg_info” failed with error code 1 in /tmp/….” |
|
|
Attention should be paid to the error message “Command “python setup.py egg_info” failed with error code 1 in /tmp/….”. This is an error usually thrown by Python’s pkg_resources package – used to list, find and use Python package dependencies. The causes for this error can vary, but it generally indicates a failed installation of a Python package.
Often, these issues could be due to dependency conflicts, permissions problems or incompatible Python versions. When dealing with library dependencies, it’s important to make sure that all the needed libraries are correctly installed. For instance, the
pip
utility comes handy in such cases: running
pip install -r requirements.txt
often suffices.
Permission-related errors can be sorted out by altering folder permissions or running commands as a superuser (but only when necessary). However, as a best practice in production environments, consider working inside a virtual environment to mitigate the risk associated with changing system-wide settings.
When the failure stems from an incompatible Python version, the solution might implicate using a different version of Python. Many cloud service providers offer several Python runtime environments that cater to different versions, so switching to an appropriate environment could rectify the issue.
Remember, good coding practice entails understanding error messages thoroughly before attempting to fix them, and the “Command “python setup.py egg_info” failed with error code 1 in /tmp/….” error is no exception. Spelunk into the official [Python documentation](https://docs.python.org/3/) or consult the vibrant Python community on [Stack Overflow](https://stackoverflow.com/questions/tagged/python) for help when necessary.
“Python setup.py egg_info” Error Code 1
I’m sure we’ve all been there in our coding journey – one minute everything is running smoothly, and the next, you’re faced with that dreaded error message: “
Command "python setup.py egg_info" failed with error code 1 in /tmp/..../.
”
Trying to understand what went wrong can be painful, but I’m here to help you unravel it step by step.
Firstly, let’s understand what this error message is saying. It involves:
- ‘python setup.py egg_info’ command
- Error code 1
- The ‘/tmp/…/’ location
‘python setup.py egg_info’
The ‘python setup.py egg_info’ command is a standard setup script for Python Package Index (PyPI) projects1. Essentially, PyPI is a repository of software for Python that comes with your Python install.
The ‘egg_info’ command is used to create a metadata file for the project, which stores details about the project like its name, version, author and summarised details about its dependencies2.
So when you’re told it has failed, what Python is essentially saying is that there was a problem creating or reading this metadata.
Error code 1
Error code 1 typically represents a general error in UNIX systems as a catch-all for miscellaneous errors3. So basically, something went wrong, but it’s not specific to what exactly happened.
Location: ‘/tmp/…/’
The path ‘/tmp/…/’ signifies the temporary directory where the setup operation occurs. The ‘…’ stands in for the specifics of your project directory and where Python is trying to do its work.
Having established some ground rules, a common underlying cause of the error is some issue with the package dependencies, rather than being an issue with the project code itself.
A perfect fix could be simply upgrading your existing python package handling tool with the following command:
pip install --upgrade setuptools wheel
Or If the setuptools are up-to-date, try installing the package using the –no-cache-dir option as follows:
pip install --no-cache-dir nameofpackage
By specifying the –no-cache-dir option, pip will download the package from PyPI instead of using the local cache, providing you with the most recent version.
In cases where these steps don’t resolve the issue, it might be helpful to know if any other dependencies have been installed during the current session that could be conflicting with the package install causing this error. This would require checking for real-time logs or reconsidering any recent changes made to your Python environment.
Remember, coding and problem-solving go hand-in-hand! With general understanding and insightful debugging, such baffling error messages can be turned into simple bug fixes.
Sources:
[1] Python Package Index (PyPI)
[2] Setuptools keyword
[3] Exit codes explanationWhen you encounter the error
Command "python setup.py egg_info" failed with error code 1 in /tmp/..../
, it denotes that there is some problem during execution of your project’s
setup.py
file. This script, which leverages setuptools, is a fundamental part of package organization within Python and deals with everything related to packaging, distribution, and installation.
The issue could stem from numerous sources – missing/incorrect versions of packages, rights permissions, Python environment misconfigurations, or even an incorrectly constructed
setup.py
file. Below, we delve into these potential causes and solutions:
– **Problem with Required Packages**: The
setup.py
file necessitates certain packages such as `setuptools`, `wheel`, and others depending on your project specifics. If these aren’t present, or if incorrect version is installed, issues like this one may arise. To resolve this, ensure all required packages are installed and have valid versions.
pip install setuptools wheel
Additional info can be found on Python’s official packaging tutorial.
– **Permission Rights**: Sometimes, due to lack of necessary permissions to perform read/write operations, you might encounter this error. Make sure you’ve correct permissions set for the directories/files you’re working with.
– **Python Environment Misconfiguration**: Setup-related commands depend heavily on the Python environment configuration. An improperly configured environment can lead to failure in executing
setup.py
. One tactic to overcome this involves utilizing isolated environments via tool like `virtualenv`.
To start new virtual environment:
virtualenv venv source venv/bin/activate # For Linux/Mac venv\Scripts\activate # For Windows
More information on creating isolated Python environments can be found at Official Python Docs.
– **Errors within setup.py**: Last but not least, defects within the setup.py itself are possible. Review your setup.py focusing on the structure and dependencies mentioned. A good reference is Python’s official guide on Packaging Python Projects.
Despite above possible resolutions, remember that troubleshooting technical issues is often circumstantial. Don’t be discouraged if none of the above work. You will need to look at the specifics of the stacktrace and error messages. Dive deep into Python community resources to ask for help. Websites like StackOverflow link foster a wide network of developers who share Python insights constantly.Decoding the Error Code 1 in Python Development within the Context of “Command Python setup.py egg_info Failed With Error Code 1”
Even for an experienced coder, coming across error codes can be daunting. However remember, these errors are just part of the process that helps us in identifying bugs or discovering areas where our code might require some tweaking for efficiency. In this case, we’ll be focusing on unpacking what encountering a
Error Code 1
means when conducting Python developments. More precisely, we will explore the problem under this context:
"Command Python setup.py egg_info failed with error code 1"
.
Error Code 1: What does it mean?
Generally,
Error Code 1
indicates that the command that was run encountered an issue – something that prevented it from executing successfully. Now, while the ‘1’ is rather generic and could mean a multitude of things depending on what you’re doing, the preceding message is meant to give some insight onto what went wrong. From this message, it’s clear that the issue is with running
setup.py
. This file is common in many python projects and packages because it essentially tells how those packages should be installed. The function
egg_info
produces metadata for the package setup, so issues there likely cause problems during installation.
Navigating & Fixing Error Code 1:
Dealing with
Error Code 1
, especially within the context mentioned, usually involves going through a couple of steps:
Identify And Check Your Python Version: One potential reason behind the failure could be running a version of python that’s incompatible with the package you’re trying to install. You can use the command
python --version
to check your version.
Update Your Setuptools: The
egg_info
command is from setuptools, which is a package development process library. Because of this link, if you have an outdated setuptools, it could result in the command failing to execute. To update your setuptools, simply run
python -m pip install --upgrade setuptools
.
Consider Updating PIP: Sometimes, the issue could lie with having an outdated version of PIP (Python’s package installer). Check your PIP version by running the command
pip --version
and if needed, upgrade it using
python -m pip install --upgrade pip
.
Ensure Correct Syntax: A syntax mistake in the command can also lead to Error Code 1. For example, instead of
python setup.py egg_info
, perhaps you meant to type
python3 setup.py egg_info
?
Remember, these are possible solutions and might not necessarily resolve your specific issue. However, they’re a good starting point when dealing with an
Error Code 1
associated with executing
"Command Python setup.py egg_info"
.
Making Information Digestible – HTML Tables
Tables can make information easier to digest. Here’s an example of how you might put these solutions into html tables.
Possible Issue | Solution |
---|---|
Outdated Python Version | Check and update python version |
Outdated setuptools | Check and update setuptools |
Outdated PIP | Check and update PIP |
Syntax Mistake in Command | Use correct syntax |
In essence, understanding
Error Code 1
and its application in various contexts requires an understanding of Python operations and commands. Each situation might call for a different approach, but with a mastery of the basics and a keen eye for detail, debugging becomes less of a chore and more of a rewarding challenge.
For a deeper dive into the world of Python error codes, consider exploring further professional Python documentation and troubleshooting guides. They provide extensive knowledge on handling these mysteries that at first glance might seem a little daunting. Visit Python Official Documentation to gain more insights and sharpen your Python development skills.The error code, “Command ‘Python Setup.Py Egg_Info’ failed with error code 1 in /Tmp/…”, is quite common among developers. This problem usually indicates a malfunction within the Python setup script, which subsequently prevents additional module installations.
Let’s explore more about this issue and identify some solutions:
Understanding the Error
The aforementioned error generally occurs when installing a Python package using pip or easy_install. The setup command ‘python setup.py egg_info’ is used by python setuptools to gather metadata about the package being installed ‒ data that includes name, version, and other details of the package.
Consequently, issues with your environment, deny permissions, difficulties related to dependencies of package can cause the error “Command ‘python setup.py egg_info’ failed with error code 1” to appear.
Solving the Issue
Here are some potential solutions to consider:
pip install --upgrade setuptools pip install --upgrade pip
If you’re operating in a Python virtual environment, remember to add –user as an argument to prevent modifying the system Python installation.
chmod -R 777 /usr/local/lib/python2.7/dist-packages
This gives all users full permissions. However, it’s always wise to put more consideration into granting privileges based on your requirement due to security concerns.
After implementing one or all of the above realizable strategies, try running the pip install command once more. You should receive a positive assessment if everything works as intended.
Whenever you’re faced with a coding issue, take the time to dig deep and understand exactly what the underlying problem may be. Remember that table stakes involve making sure your environment is up-to-date and organized. (source)Command execution failure, with a special focus on the specific command “python setup.py egg_info” that results in an error code 1, can stem from multiple factors. The nature of these factors typically revolves around three main arenas – the Python environment, dependencies, and system-level permissions.
Python Environment:
A clear starting point to explore is Python’s environment configuration. Python’s version compatibility issues or an incomplete installation can manifest as a cause for concern. This means that mismatch between the Python version required by ‘egg_info’ and the one present in your system might lead to an error.
Here’s a standard guideline how to check this:
# Checking installed Python version python --version
If the above command gives out a Python version that’s not compatible with the module you’re trying to install, consider switching versions using pyenv or any other similar tools.
Please refer to [official Python setup instructions](https://docs.python.org/3/using/index.html) for detailed guidance.
Missing Or Incompatible Dependencies:
Penetrating further into this analysis, we recognize that missing or incompatible dependencies are often attributed as common culprits for command execution failures. The ‘egg_info’ error might occur because some required dependency might be absent further down the installation pipeline.
You can try updating ‘setuptools’, ‘wheel’ and ‘pip’ first before re-running the command:
# Update setuptools and wheel pip install --upgrade setuptools wheel # Then try installation again python setup.py egg_info
System-Level Permissions:
Lastly, System-Level Permissions can cause hindrances in smooth command execution. To solve this, elevate your command terminal to superuser status and try running your command again.
An example to illustrate this would be:
sudo python setup.py egg_info
Warning: Be cautious while using sudo as it grants all necessary permissions, making your system more vulnerable.
Remember, computing is fundamentally about people solving problems, not machines performing tasks. As a programmer, I understand that coding isn’t just about typing in keywords and syntax but more about logical reasoning, problem-solving, and communication. Our aim here, therefore, is to undertake a careful inspection of the three sectors stated and define troubleshooting methods aimed at resolving command execution failures and ultimately maximizing operational efficiency.Advanced troubleshooting techniques for issues related to “command ‘python setup.py egg_info’ failed with error code 1” usually revolve around environment configuration, package dependencies, and understanding the error messages.
First off, you want to make sure that your
python
and
setuptools
are up-to-date. By ensuring you have the latest versions, you eliminate potential compatibility issues and bugs that might be causing the problem. Here’s how to update these:
Python:
python -m pip install --upgrade pip
Setuptools:
pip install --upgrade setuptools
Secondly, it’s important to ensure you’re installing packages in a virtual environment instead of the system Python. This eliminates permission issues and conflicts between different versions of the same module.
You can create a virtual environment with:
python3 -m venv env
Activating the environment:
source env/bin/activate
Now, when you install modules, they will be isolated from your system Python.
Additionally, paying close attention to the error messages can give you cues on what to do. The error message `command “python setup.py egg_info” failed with error code 1` is a general failure signifying that something went wrong but it doesn’t detail what specifically. Look at the lines before this message to get more context about the issue, these specific problems will lead you to more directed solutions.
Another point to look out for is making sure you meet all dependencies. Some modules depend on other packages or specific versions of those packages to run correctly. If you don’t have those prerequisites, installation could fail. Check the documentation for the module you’re trying to install to see if there are any dependencies and install them.
If none of the above has proven successful, perhaps you can try cloning it directly from the respective repositories. Most of the time, the package available in the repository is likely to be the most stable one. How to do this varies depending on which tool you’re using for source control but here’s an example with Git:
git clone https://github.com/username/repo.git
Then navigate to the cloned directory and install the package locally with
pip
:
pip install .
The strategies mentioned should be able to help you identify, isolate, and fix the issues when running into the `command “python setup.py egg_info” failed with error code 1`. Refer to the official Python documentation on installing packages for more in-depth details.
This query sounds like you’ve encountered a thorny issue I’m very familiar with: the “Python setup.py egg_info” failing with error code 1 in a temporary directory. This type of error is usually experienced when attempting to install a python module using pip, Python’s package installer. The error message essentially means that pip could not run setup.py for some reason. Here are methods to troubleshoot and prevent this type of error from reoccurring:
Error Handling
The very first step is always to make sure we understand the nature of the error. Inspect the error trace carefully because it often contains crucial information about what went wrong. For instance, if you see an ImportError, it means that a required module is missing. This tells you right away that you need to install the missing module.
Fixing Broken or Missing Python Setup Files
One common cause of this error is missing or corrupted files in your python setup. In this case, one way to fix the problem would be to reinstall Python itself or just the broken packages which could be causing the error.
An example on how to reinstall a broken package is as follows:
pip uninstall package-name pip install package-name
Upgrading Pip and Setuptools
If the failure is due to an issue with Pip and Setuptools, a good solution would involve upgrading both pip and setuptools using the below commands:
pip install --upgrade pip pip install --upgrade setuptools
Remember to replace ‘pip’ with ‘pip3’ if you’re working with Python3.
Moving to a Virtual Environment
Sometimes global installations can cause conflicts between different versions of the same package. Moving to a virtual environment often helps to isolate your project and its dependencies, thereby eliminating such conflicts.
To create a virtual environment, use:
python -m venv myenv
And then activate it with:
source myenv/bin/activate
Afterwards, try running the command again within the virtual environment.
Checking Code Compatibility
Moreover, ensure that your code is compatible with the Python version you’re using. If you are trying to use a package that’s solely created for Python 2.x with Python 3.x, you’d also get this error.
These are some general solutions to the problem described. Identifying the exact reason for the error would make problem-solving much more effective. However, keep in mind that issues could occur from multiple sources. You can refer to Python’s official documentation on errors and exceptions for detailed explanations and solutions. As a professional coder, it is recommended to embrace these challenges as they help us to grow and broaden our expertise.The command error
python setup.py egg_info
that returns with an error code 1 is a fairly common stumbling block professionals and enthusiasts encounter when delving into the world of Python. This command is run in order to fetch metadata about the package being installed, typically conducted in a temporary directory – /tmp/ .
When the system throws an Error Code 1, it essentially implies that the program has attempted to execute a specific task, but was unsuccessful. This could be due to a myriad of reasons, the most common ones being:
- The prerequisite software packages or dependencies being absent or outdated.
- Ill-defined environment variables.
- An incompatible Python version in use.
Fortunately, one can likely fix this issue by following these simple steps:
- Ensure that you have the latest versions of pip and setuptools preinstalled. If not, they can be updated using the commands:
pip install --upgrade pip
pip install --upgrade setuptools
- If you’re dealing with the instance of an outdated or absent package, consider using pip’s ‘–-ignore-installed’ tag to ignore the already installed packages.
- Frequently, the error arises due to using a deprecated version of Python. It’s always a good idea to check whether you are running an up-to-date version. You might need to update your python version or create a virtual environment with a compatible Python version for your package to ensure compatibility.
In the light of Python’s dynamic nature and vast community support, there’s usually a workaround for most problems – all it calls for is some resilience and patience. If all stipulated avenues fail to fix the Error Code 1, don’t feel stumped. More often than not, answers lie in lines of cryptic StackOverflowsource threads or elaborate GitHubsource repositories. It’s extremely crucial to read and understand the error messages carefully as they can serve valuable insights on what precisely could’ve gone wrong.
Ultimately, keep in mind that coding isn’t solely about getting things right; it’s also about untangling mistakes you’ve made along the way. The command error
python setup.py egg_info
is but one small hurdle of many that you will overcome successfully in your Python journey.