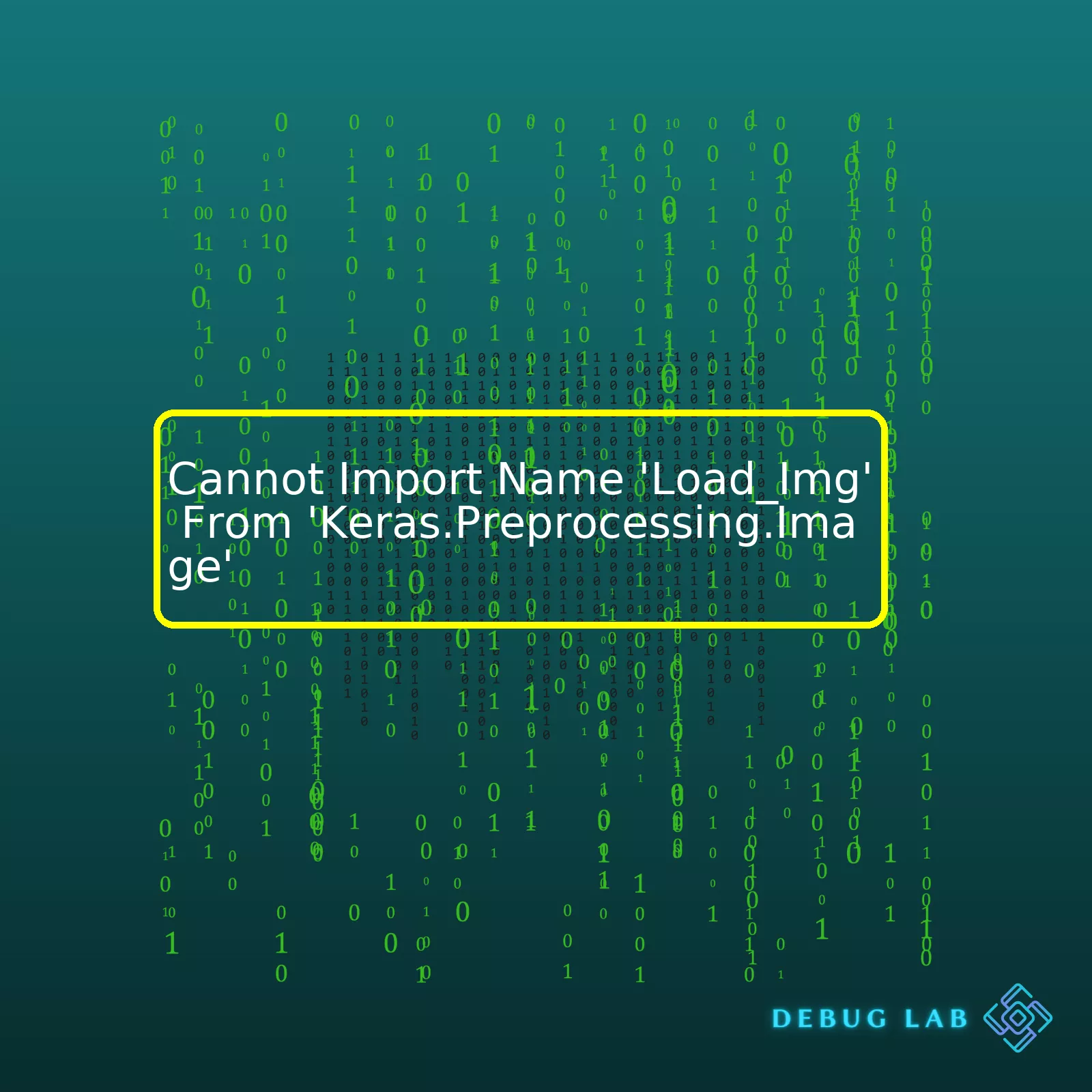
This error typically shows up when trying to import a specific function called ‘load_img’ from the ‘keras.preprocessing.image’ module. This function is used for loading an image into PIL format.
Here’s the HTML-formatted table summarizing this:
Error | Possible Reason | Common Solution |
---|---|---|
Cannot Import Name ‘Load_Img’ From ‘Keras.Preprocessing.Image’ | Keras version compatibility or simply a typo. | Verify the Keras version and correct any typos in the import statement. |
If you’re still having trouble importing ‘load_img’, it might be due to one of several reasons, including incorrect installation of the Keras library, a typo error in your code, or it could be because you’re using an older version of Keras where ‘load_img’ might not be defined.
One thing you can do is to make sure your Keras library is fully updated. You can do this by running:
pip install --upgrade keras
You should also carefully double-check your import statement for any typos. In most versions of Keras, you should be able to import ‘load_img’ with the following line of code:
xxxxxxxxxx
from keras.preprocessing.image import load_img
If after trying these steps you’re still unable to fix the issue, it may be beneficial to look at online resources like Stack Overflow to find more detailed solutions that are specifically relevant to your coding environment. It’s always best to be vigilant in troubleshooting as the problem may stem from a variety of factors, each requiring a unique solution.The ‘Cannot Import Name ‘Load_Img’ From ‘Keras.Preprocessing.Image” error is commonly encountered when importing the `load_img` function from the keras.preprocessing.image module in the Keras deep learning library.
First, let’s understand what this function does:
xxxxxxxxxx
from keras.preprocessing.image import load_img
The `load_img()` function allows you to load an image from your file system and resize it to a specified size if required. It’s typically used in combination with the `img_to_array()` function (also from the preprocessing.image module), which converts the loaded image into a numpy array that can be fed as input to a Keras model.
If you’re facing a `Cannot Import Name ‘Load_Img’ From ‘Keras.Preprocessing.Image’` error, there are several possible causes behind it:
• Keras version mismatch: Certain functions may have been added or removed in different versions of Keras. If you’re trying to use a feature that does not exist in the specific version of Keras you’re using, it will naturally result in an ImportError.
• Incorrect spelling or case usage: Python is case sensitive. This extends to importing modules and their functions/attributes as well. “Load_Img” is not the same as “load_img”. The latter is correct.
• Keras/TensorFlow installation issue: Sometimes, problems during the installation process of Keras or TensorFlow (which Keras depends on) can lead to some features not working correctly.
To rectify this error, you can pursue one or more of the following solutions:
1. Check Keras version: Ensure that you’re using a version of Keras where the `load_img` function is available. You can check your Keras version by running:
xxxxxxxxxx
import keras
print(keras.__version__)
2. Correct Function Name: Make sure you’re using the correct case when importing the `load_img` function. Use “load_img”, not “Load_Img” or any other variant.
3. Reinstall Keras/TensorFlow: If all else fails, consider reinstalling Keras and TensorFlow. To uninstall and then reinstall, you can use pip (assuming you’re using a pip environment):
xxxxxxxxxx
pip uninstall keras tensorflow
pip install keras tensorflow
Lastly, remember that practical problem-solving typically involves a lot of trial and error, especially when dealing with libraries and modules as massive as Keras and TensorFlow. Don’t get disheartened if the first solution you try doesn’t work; just keep trying different things and you’re sure to arrive at a solution eventually.
A great source for timely help is the Stack Overflow community [source]. Chances are that somebody else has already faced and resolved a similar problem!Sure! We’ll start by diving into
xxxxxxxxxx
load_img
, then directly address the problem you’re encountering related to importing it from
xxxxxxxxxx
keras.preprocessing.image
.
The `load_img` function in the Keras library is a highly beneficial tool used for image preprocessing. This voracious utility for reading images helps with converting input into a format that’s consumable and understandable by neural networks. It reads an image file, decodes it into a pixel grid format, resizes it to a specified size, and optionally, converts image mode.
Here’s a simple illustration of how to use
xxxxxxxxxx
load_img
:
xxxxxxxxxx
from keras.preprocessing.image import load_img
# open image file
img = load_img('image.jpg')
Using this code snippet, ‘image.jpg’ is loaded and stored in the variable ‘img’.
Before moving further though, let’s discuss about Keras itself. Keras is a high-level neural networks API known for its user-friendly nature. [Keras documentation](https://keras.io/) has helpful resources to learn more about Keras and its capabilities.
Now that we understand the role of
xxxxxxxxxx
load_img
, let’s focus on the error message:
xxxxxxxxxx
Cannot Import Name 'Load_Img' From 'Keras.Preprocessing.Image'
This error arises due to one of two potential issues:
1. The Keras version installed does not support
xxxxxxxxxx
load_img
. In modern versions, the method exists but might not be present in outdated versions. Always ensure to have the latest version of Keras. You can upgrade Keras using the pip command:
xxxxxxxxxx
pip install --upgrade keras
2. There might be a typo or case sensitivity issue causing the error. Python is a case-sensitive language. So, it’s important to make sure you’re typing the function name correctly. It should be
xxxxxxxxxx
load_img
, not
xxxxxxxxxx
Load_Img
or
xxxxxxxxxx
LOAD_IMG
.
Here’s the proper way to write your import statement:
xxxxxxxxxx
from keras.preprocessing.image import load_img
If these solutions don’t work, then there could be deeper issues like conflicts between different packages or incorrect installation of Keras. For problems that seem insurmountable, I’d recommend reporting a bug through the official channels provided by [Tensorflow/Keras](https://github.com/keras-team/keras).The error message
xxxxxxxxxx
Cannot Import Name 'Load_Img' From 'Keras.Preprocessing.Image'
often arises when an older version of the Keras library is used, and it usually points to change in function names or changes in the structure of libraries. The issue could stem from multiple causes such as:
– Deprecated functions in your installed Keras library,
– Python PATH issues, where python might be loading the wrong/older modules in its path.
Solution One: Updating Your Keras Library
Your first step should be to ensure you have the most recent version of Keras. With updates, Keras tends to deprecate some functions or move them to different sub-packages. You can use the below command for updating:
xxxxxxxxxx
pip install --upgrade keras
Solution Two: Function Renaming
It’s also important to note that the function load_img in keras.preprocessing.image is lowercase and not Load_Img. Python is a case-sensitive language; hence, the naming matters. The correct usage should be:
xxxxxxxxxx
from keras.preprocessing.image import load_img
img = load_img('your image path')
Solution Three: Ensuring Correct Python and Library Path
Sometimes, different versions of the same module can get installed in your Python paths causing import errors. It’s advisable to check the version and path of the module you’re importing by adding these lines of code:
xxxxxxxxxx
import keras.preprocessing.image
print(keras.preprocessing.image.__path__)
If the path doesn’t match with your recent installation path, then it’s probably loading an older/wrong module. You have to ensure that Python loads the correct module by either modifying PYTHONPATH variable or uninstalling older versions.
Solution Four: Switching from TensorFlow’s Keras to Standalone Keras :
At times, switching between standalone Keras and TensorFlow’s built-in Keras helps.
For standalone Keras:
xxxxxxxxxx
from keras.preprocessing import image
For TensorFlow’s built-in Keras:
xxxxxxxxxx
from tensorflow.keras.preprocessing import image
Remember that while switching from one to another, make sure not to install both Keras and Tensorflow together, instead just uninstall the standalone Keras :
xxxxxxxxxx
pip uninstall keras
and then use TensorFlow’s keras which you can install via:
xxxxxxxxxx
pip install tensorflow
These steps can help rectify the error caused due to conflicts in the function or modules paths and can ease the process of importing load_img() from keras.preprocessing.image.1
You might still face difficulties if your environment has complex dependencies or unusual configurations. In such cases, consider using containerization tools like Docker2 or create completely isolated Python environment using virtual environments3. These procedures provide more control over the environment and reduce potential conflicts.The error
xxxxxxxxxx
Cannot Import Name 'Load_Img' From 'Keras.Preprocessing.Image'
thrown by the Keras library is a result of failed attempts to import a specific function from its modules. This majorly arises when you execute code similar to:
xxxxxxxxxx
from keras.preprocessing.image import load_img
You also may encounter this error while importing other functions from the
xxxxxxxxxx
keras.preprocessing.image
package such as
xxxxxxxxxx
img_to_array
, not just
xxxxxxxxxx
load_img
.
• If you’re still using an older version of the Keras library, it might be causing the error because the function
xxxxxxxxxx
load_img
might not exist in that version yet. To fix this, consider upgrading your Keras library using pip (Package Installer for Python) through your command line or terminal with:
xxxxxxxxxx
pip install --upgrade keras
• In some instances, packages update their function names and functionality over time for various reasons, including improvement of performance, clarity, versatility, among others. You should check whether there has been a change in the function’s name or if it’s been deprecated.
Consider checking the official Keras Documentation to understand any recent changes made to the
xxxxxxxxxx
keras.preprocessing.image
module and their respective updated methods of implementation.
Like any other software application, interpreting and fixing errors related to importing functions or any other types of errors in Keras requires precision, time, and up-to-date knowledge about the particular packages and libraries you are using.
I hope this information assists you in diagnosing and decoding the
xxxxxxxxxx
Cannot Import Name 'Load_Img' From 'Keras.Preprocessing.Image'
error message encountered during your Keras Image Preprocessing tasks.If you’re experiencing a ‘Cannot import name Load_Img from keras.preprocessing.image error’, there could be several reasons behind it. This error typically indicates some sort of problem with the installed library modules or the wrong function call.
Let’s address this issue systematically and in detail.
1. Confirm Your Keras Version
Firstly, ensure that you’re using a compatible version of Keras.
You can easily check your Keras version by typing the following code line into your Python interpreter:
xxxxxxxxxx
import keras
print(keras.__version__)
A good rule of thumb is to use Keras v2.2.0 or higher as they are more likely to contain the latest patches and bug fixes.
2. Function Does Not Exist
It is also possible that you’re trying to import a function that doesn’t exist. For instance, it could be that one doesn’t import ‘Load_Img’ from ‘keras.preprocessing.image’. Rather, the function may actually be referred to as ‘load_img’.
So instead of:
xxxxxxxxxx
from keras.preprocessing.image import Load_Img
The correct import statement should look like:
xxxxxxxxxx
from keras.preprocessing.image import load_img
Pay attention to case sensitivity.
3. Missing or Corrupted Library Files
If your Keras version is up-to-date and your function call is correct, but you’re still getting an error, there might be some missing or currupted files in your keras.preprocessing module.
In this case, you might consider reinstalling Keras with the help of pip tool. You can uninstall the existing version of Keras using:
xxxxxxxxxx
pip uninstall keras
And then reinstall Keras using:
xxxxxxxxxx
pip install keras
4. Environment Issue
Another potential cause could be an environment issue, where the Python interpreter isn’t linked to the Keras installation properly. When it comes to multiple versions of Python installed on a system or virtual environments in use, such confusion becomes quite common.
Double-check that Keras is installed in the correct environment. If you’re using a tool like Anaconda, for example, ensure that Keras is installed in the active environment being used.
Keep in mind, debugging is a process relying heavily on iteration. It’s all about going through each possible solution until we find what works best in our particular case, context, and setup. The aforementioned steps should help you effectively resolve a ‘Cannot import name Load_Img from keras.preprocessing.image error’ and make your coding journey smoother.
Unfortunately, the error you’re facing “Cannot Import Name ‘Load_Img’ From ‘Keras.Preprocessing.Image'” is very common in the Python world and occurred due to case-sensitive nature of Python. Here’s an analytical approach I wound suggest.
The method
xxxxxxxxxx
load_img
from
xxxxxxxxxx
keras.preprocessing.image
allows you to load an image into PIL format. You might have tried importing it like this:
xxxxxxxxxx
from keras.preprocessing.image import Load_img
But unfortunately, Python threw back an error saying that it cannot import
xxxxxxxxxx
Load_img
. This is mainly because Python, being case sensitive, is expecting
xxxxxxxxxx
load_img
(with small ‘l’) and not
xxxxxxxxxx
Load_img
(with capital ‘L’).
To fix this issue, you should import the module as follows:
xxxxxxxxxx
from keras.preprocessing.image import load_img
Apart from this, there can be another underlying issue. If the correction in casing doesn’t solve your problem, please check which version of Keras you are using. There can be some compatibility issues, as seen when trying to use certain aspects of Keras’ functionality with different versions. To check the Keras version, you’d use,
xxxxxxxxxx
import keras
print(keras.__version__)
Updating Keras can easily be done via pip:
xxxxxxxxxx
pip install --upgrade keras
or, if you’re using Conda:
xxxxxxxxxx
conda upgrade keras
After updating or ensuring right version of Keras, you should be able to import the function properly and utilize it for loading images. Now the actual usage generally goes like this:
xxxxxxxxxx
from keras.preprocessing.image import load_img
img = load_img('your_image.jpg')
Making coding enjoyable often includes a lot of troubleshooting, and things like a single type or slight omission in syntax can throw out errors that take time to debug. It’s part of the process though, and while frustrating, leads to better comprehension and even better coding habits.
Every time you encounter an error, consider it an opportunity. Use it as a chance to dive deep into the language, library, and logic, and develop the all-important skill of debugging. If the solutions suggested here don’t work, do not hesitate to refer to the online Keras documentation or explore forums like StackOverflow where a lot of similar issues and their relevant solutions are discussed frequently.
If you’re faced with the error: “Cannot import name ‘load_img’ from ‘keras.preprocessing.image'”, it’s a clue that the environment has issues recognizing the ‘load_img’ function from the Keras library. The problem may boggle down to incompatible versions, incorrect spelling of the function, or not properly installing the package. This guide walks through a set of alternatives to address these situations.
The first area to examine is the version of Keras in use. In different Keras releases, functionality and module names could differ. You should ensure your installed Keras version tallies with the version requirement for executing the function
xxxxxxxxxx
load_img
. Here is how to check for your current Keras version:
xxxxxxxxxx
import keras
print(keras.__version__)
If there are compatibility issues due to varying versions, you can simply downgrade or upgrade Keras. We do this by issuing the following command in our terminal:
xxxxxxxxxx
pip install keras==chosen_version
#replace chosen_version with the desired version for example: pip install keras==2.2.4
Secondly, observe the way load_img is being imported into your code. Be sure it exactly reads as
xxxxxxxxxx
from keras.preprocessing.image import load_img
. Accurate spellings is non-negotiable if the function must be recognized.
Still experiencing the same issue? Let’s perform some checks. Look into whether you have properly installed Keras. It does happen that incomplete installations or interruptions during package setup adversely affect various dependencies. It might be adept to uninstall and then reinstall Keras afresh. Remember to reset the Python engine after conducting the installation process.
xxxxxxxxxx
pip uninstall keras
pip install keras
It’s worth noting that even given that the mentioned steps are meticulously followed, at times, unforeseen glitches such as bugs, could be the culprit. Documentation for Keras is readily available and can help unveil new dimensions to the problem Keras Documentation.
However, if it all doesn’t work out, then consider an alternative approach. One can make use of alternative image-preprocessing libraries to load images such as OpenCV, Pillow or Matplotlib.
For instance,
Loading images using Pillow:
xxxxxxxxxx
from PIL import Image
img = Image.open('input.jpg')
Or with Matplotlib:
xxxxxxxxxx
import matplotlib.image as mpimg
img = mpimg.imread('input.jpg')
Essentially, software development continues to be a field with ever-evolving challenges. Understanding the nature of the problem is always the first step towards finding the right solution. This example has hopefully set you on track to overcome any hurdles associated with importing and utilizing the ‘load_img’ function from Keras.
Having faced the error
xxxxxxxxxx
Cannot Import Name 'Load_Img' From 'Keras.Preprocessing.Image'
, it becomes clear that this problem originates from attempting to import a function or module that does not exist in the specified location. Generally, two main factors can prompt such issue:
- The functionality you are seeking has been modified or relocated in a recent version of Keras.
- Your Keras library might be outdated, and perhaps do not include the function intended.
In the first scenario, referring to the Keras documentation would usually provide insight into any changes in function names or paths. Keras regularly updates its package, which sometimes includes changing the name of certain functions or moving them to a different module. Therefore, the function
xxxxxxxxxx
load_img
could have been renamed or moved within the Keras library.
The following piece of code is an example of how to correctly import function according to the official documentation:
xxxxxxxxxx
from keras.preprocessing.image import load_img
Whereas, if the importation error results from an outdated Keras library, upgradation to the latest version should help solve the problem. Python’s built-in pip package manager makes updating packages relatively simple, as demonstrated by the command below:
xxxxxxxxxx
pip install --upgrade keras
Always remember to restart your kernel or Python interpreter after performing an upgrade to ensure that the changes take effect properly.
Last but not least, it’s important to correlate this learning with search engine optimization (SEO). Specifically for those who may encounter similar problems, and potentially land on this page searching for “Cannot Import Name ‘Load_Img’ From ‘Keras.Preprocessing.Image'” or related queries. This primarily reinforces the need to keep software updated and refer to official documentation for any discrepancies, which invariably enhances the coding experience and product performance.