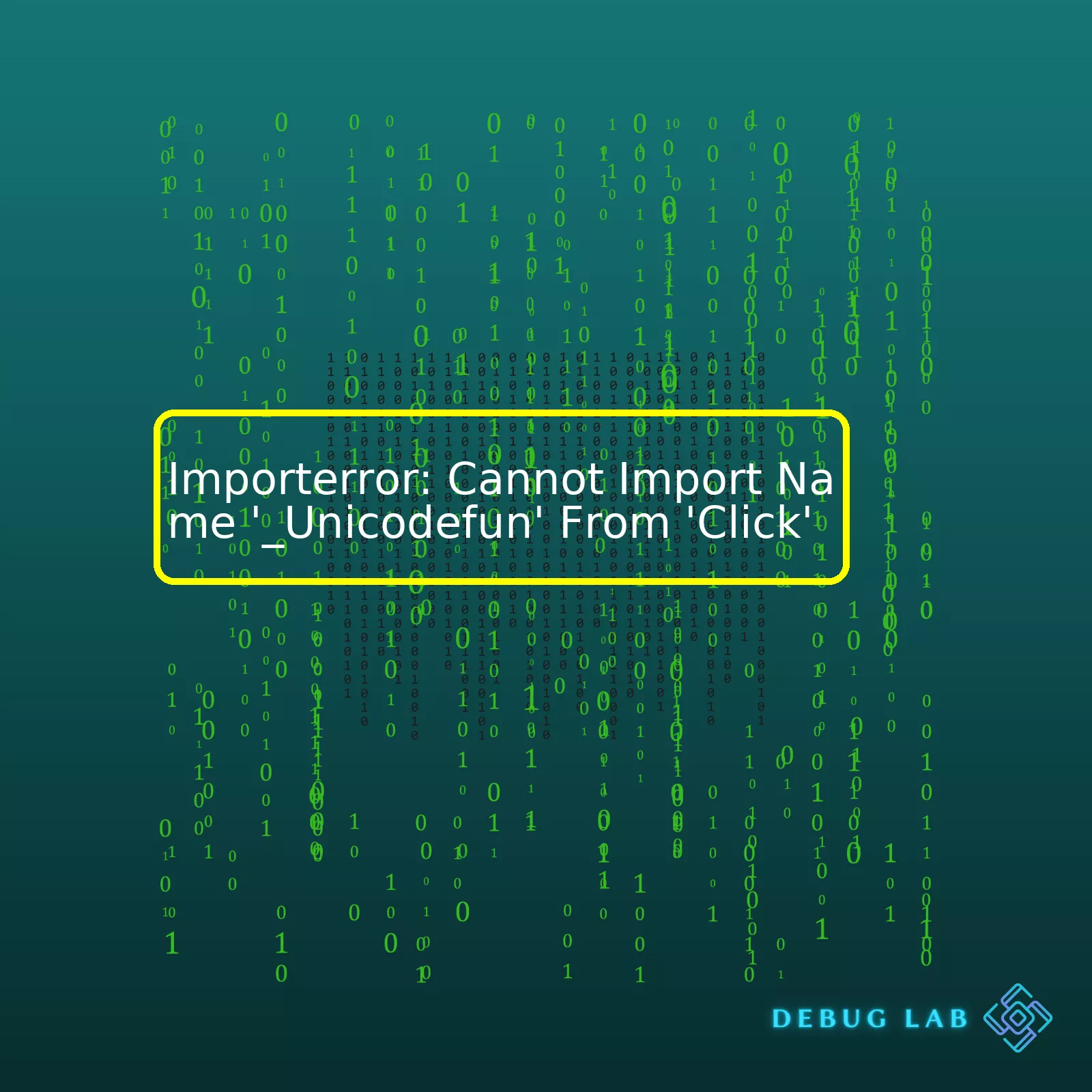
Error Type | Possibly Reasons | Common Solutions |
---|---|---|
ImportError: Cannot import name _Unicodefun |
|
|
The error “Importerror: Cannot Import Name ‘_unicodefun’ From ‘Click'” often occurs when there are issues with the installation of the Click library or if it mismatches with the Flask version in your python environment. The Click library is a vital package for building beautiful command line interfaces in a composable way with as little code as necessary. It’s used quite extensively within codebases involving Flask and other Python projects.
One of the possible reasons contributing to this issue could be incorrect versions of Flask and Click libraries being used together. Some specific combinations of Flask and Click versions may have compatibility issues which can result in such ImportError.
Moreover, conflicts may also arise in scenarios where you have multiple system-level Python packages installed along with their local versions. Sometimes having too many similar packages at different levels can cause confusion for Python leading it to import from the wrong place.
Finally, your Python environment may not be set up correctly. This includes things like the settings for your Python Interpreter, environment variables or PATH settings. If these are incorrect, it makes Python pull libraries from the wrong locations therefore causing errors during imports.
Some strategies to resolve this issue include upgrading or downgrading the Click package. Different versions of same package sometimes have slightly different internal structures, so importing modules or functions might vary between versions due to changes or deprecations.
You can also opt to re-install both Flask and Click in a new virtual environment. This isolates your local project dependencies, and it can help you keep system-wide packages intact while avoiding any potential conflicts among them.
Lastly, you might need to inspect and correctly configure your system’s PATH settings for the Python interpreter and associated libraries. By doing so, you ensure your system is referencing and pulling the correct libraries from the expected places during execution.
For detailed reference and further reading, refer to the official documentation of Click and enhancements pertaining to latest releases of Flask.
Consider setting up and managing virtual environments via VirtualEnv to isolate your Python project environments effectively, or reinstalling Python packages, to understand the process better.
Here’s a code snippet showing how to create a dedicated virtual environment for your project and installing Flask and Click:
# Creating a Virtual Environment
python3 -m venv myenv
# Activating the Virtual Environment
source myenv/bin/activate
# Installing Flask
pip install Flask==1.1.2
# Installing Click compatible with above Flask version
pip install click==7.1.2
Ah, ImportError. Every Python developer’s nightmare. Isn’t it a pain when you just want to use the functions in your newly installed package only to stumble upon an unexpected
xxxxxxxxxx
ImportError
? I’m sure it is for you as much as it is for me. But worry not! There’s always a way around these bugs.
Firstly, I think it would be beneficial to first understand what
xxxxxxxxxx
ImportError
actually is.
xxxxxxxxxx
ImportError
is raised when an
xxxxxxxxxx
import
statement fails to find the module definition or when a
xxxxxxxxxx
from ... import
fails to find a name that is to be imported. In simpler words, this error usually occurs when Python can’t find the module or the function you’re trying to use.
Now, specifically for the
xxxxxxxxxx
Importerror: Cannot Import Name '_unicodefun' from 'click'
. The Click module is a Python package for creating beautiful command line interfaces. It’s know for its simplicity and intuitiveness. You’re probably using it too for achieving similar functionality.Click Documentation
However,
xxxxxxxxxx
_unicodefun
is not a part of the Click module by default. You might have mistaken a function name or are using a version that doesn’t support it. Let’s try some solutions to climb over this hurdle!
Here’s my favorite list of steps to go through when faced with uncertainties of such like:
- Make sure you’re using the correct name of the function. Python is case-sensitive and will not recognise the function if you mess up the cases or misspell!
-
Ensure the module is properly installed. Try reinstalling it using pip:
xxxxxxxxxx
111pip uninstall click
xxxxxxxxxx
111pip install click
-
Sometimes, there are conflicts with other modules in your environment. I’d recommend trying inside a new virtual environment. Create a new one using:
xxxxxxxxxx
111python -m venv myenv
xxxxxxxxxx
111source myenv/bin/activate
Then, try importing the module again.
- The last bullet on our checklist would be checking your software version. Certain Python versions does not play well with specific module versions. Always double check the versions required by referring the official documentation.Click Documentation
By now, I hope we’ve successfully resolved the issue. If not and you are still having trouble, consider reaching out via GitHub issueClick GitHub Issues. The developers might be able to provide more context or guide you towards the right direction.
Following these meticulously should alleviate us from the
xxxxxxxxxx
ImportError
conundrum. Let’s hop back into our project with a fresh mind ready to tackle everything head-on!
Remember, coding is trial and error. Embrace every bug, every error. Each one of them teaches something new. Wish you a happy coding journey!The infamous ‘_unicodefun’ in ‘Click’ refers to a common error that may affect your Python projects when using Click, a popular package for creating beautiful command-line applications. There is a high probability you might encounter the error message telling you it “Cannot import name ‘_unicodefun’ from ‘Click'”.
It occurs typically due to an incorrectly installed version of Click. This issue can be distressing as nothing beats the fury than seeing your smoothly-working code suddenly halt with an ImportError.
Now how does this error occur exactly?
Well, ‘_unicodefun’ doesn’t exist anymore in more recent versions of Click (7.x and beyond) which creates an unexpected scenario for codes written based on older versions of Click. If your system tries to execute code that requests ‘_unicodefun’ in an environment where a newer version of Click is installed, it will promptly fall flat on its face with an ImportError.
To understand it better, here’s a small piece of pseudo-code that illustrates the situation:
xxxxxxxxxx
import click
print(click._unicodefun)
# Output: ImportError: cannot import name '_unicodefun'
This situation highlights the importance of managing your project’s dependencies–ensuring that all packages and modules are at their correct versions.
But we are coders! And a coding problem is a problem worth solving.
To fix the “_unicodefun” ImportError, you must ensure that you’re using the appropriate version of Click, ideally the one suitable for your project’s requirement.
– Uninstall your current version of Click :
xxxxxxxxxx
pip uninstall click
– Install a version that contains _unicodefun like Click 6.7:
xxxxxxxxxx
pip install click==6.7
Remember, downgrading might not always be the best solution. You should aim to upgrade your code to suit the most stable and up-to-date version of your packages where possible. Importantly, also remember the fix outlined here reflects only particular instances. It won’t always be a matter of downgrading or upgrading–sometimes, more extensive debugging might be necessary.
For more information, refer to [Python’s Official Documentation](https://docs.python.org/3/tutorial/modules.html#importing-from-a-package) on importing modules from a package.
Undeniably, the intricate network of dependencies and their ramifications on importing modules in Python is a captivating topic to delve into. Particularly with regard to the quite specific error:
xxxxxxxxxx
Importerror: Cannot Import Name '_Unicodefun' From 'Click'
.
Essentially, each Python module has its unique set of code environments necessary for executing functions or classes contained therein. This makes dependencies indispensable. However, anytime a dependency goes missing or clashes with other similar modules, it could precipitate certain anomalies just like this ImportError.
Let’s talk about this exception briefly. The ImportError primarily arises when the Python interpreter fails to locate and subsequently import a body of code specified in your script’s import statement.
To better understand the Error message, you have to break it down:
Error Breakdown:
1. ImportError: This invariably connotes encountering an import-related issue.
2. Cannot Import Name: Indicates that the interpreter was unable to import the specified name.
3. ‘_Unicodefun’ From ‘Click’: _Unicodefun from the Click package is what you attempted importing but couldn’t find.
When deciphered, it’s evident that the Python interpreter could not route out and import the _Unicodefun from the click module.
But why? Remember, a prerequisite for importing object (functions, methods, classes, variables) from a module lies in its presence within said module. Thus, an absence of the objected-to-be-imported or its renaming in recent versions can trigger this import error.
Although practically non-existent, the _Unicodefun appears relatively strange. Most likely, it’s a private function not intended for public access by virtue of the underscore prefix.
If we are to troubleshoot, we could consider the following points:
- Check Dependency Version: Make sure you’re utilizing a version of ‘Click’ that consists of the ‘_Unicodefun’. You can verify its availability in the documentation or explore the source code. For upgrading/downgrading use pip:
xxxxxxxxxx
111pip install --upgrade click==version_number
- Examine Import Statement: Double-check your import statement. Ensure no typographical errors exist and it aligns with the module’s established convention.
- Analyze Path Issues: The interpreter could be referencing a path other than expected. To check Python path, use:
xxxxxxxxxx
121import sys
2print(sys.path)
- Solve Namespace Clashes: If you’re managing multiple virtual environments, there could be a likelihood of same-name issues causing this problem. Utilize dedicated and differentiated virtual environments for distinct projects to avoid such pitfalls.
Again, dependencies play a crucial role in averting such import errors. Dependencies essentially refer to external Python packages or modules that your project relies upon. Managing them correctly and being mindful of their interrelations will help keep these nagging import errors at bay.
The ‘Click’ library itself, for instance, comes with its own set of dependencies as listed on the project’s documentation page. One must ensure all these are properly installed and functional.
Balancing imports and understanding dependencies is a key factor in ensuring seamless functionality of your Python project. When maintained correctly, they form the underpinning of predictable, repeatable, and consistent performance.In the Python ecosystem, error messages like
xxxxxxxxxx
ImportError: Cannot import name '_Unicodefun' from 'Click'
can often be observed. Typically, ImportError is raised when an import statement has difficulties trying to find a defined module or cannot load a name from it correctly. In this case, the problem specifically lies in successfully importing the name `_Unicodefun` from the `Click` library.
Let’s dive deeper into why this kind of ImportError might occur and explore some effective troubleshooting tips:
## Check If You’ve Properly Installed Click Library
Firstly, are you certain that the `Click` library is correctly installed in your environment? Try using the pip list command to get all installed packages. If Click isn’t on the list, install it by running
xxxxxxxxxx
pip install click
## Cross-Check The Variable Name
Notably, Python distinguishes between lowercase, uppercase, and mix of both cases. So, `_UnicodeFun`, `_unicodefun` and `_Unicodefun` will all be treated as different variables. Be sure to confirm that the function or class you’re attempting to import matches exactly with its actual definition in the codebase.
## Ensure That Your Code Doesn’t Have Circular Imports
With circular imports, two or more modules depend on each other, leading to a loop of imports. This situation can result in ImportErrors. Take some time and ensure that your code doesn’t have circular import patterns.
## Verify Compatibility Of The Package Versions
A specific version of a package may no longer support certain features or names which might exist in previous versions. If you recently upgraded the Click library, it’s possible that `_Unicodefun` was phased out, renamed, or moved to another module within the Click package. Checking the package’s documentation can help identify such changes.
That said, given the naming convention of `_Unicodefun`, with its underscore prefix, it seems like it’s intended for internal use within the Click package (see PEP8 guide on naming styles). Accessing these internal-use entities directly could potentially lead to complications, including but not limited to ImportError. It is advisable to adhere to public APIs when working with libraries or modules.
In general, understanding the nature of ImportError, proper installation and upgrade, literal matching of variable names, avoiding circular dependencies, and maintaining compatibility among all the packages form part of the robust cornerstone practices for avoiding and resolving ImportErrors.
Lastly, remember that Google and StackOverflow can be great resources when facing ImportErrors. Chances are high that someone else has faced a similar ImportError and found a solution that they have shared online!
Here is the python code example:
try:
from click import _Unicodefun
except ImportError:
print(“Failed to import _Unicodefun from Click”)