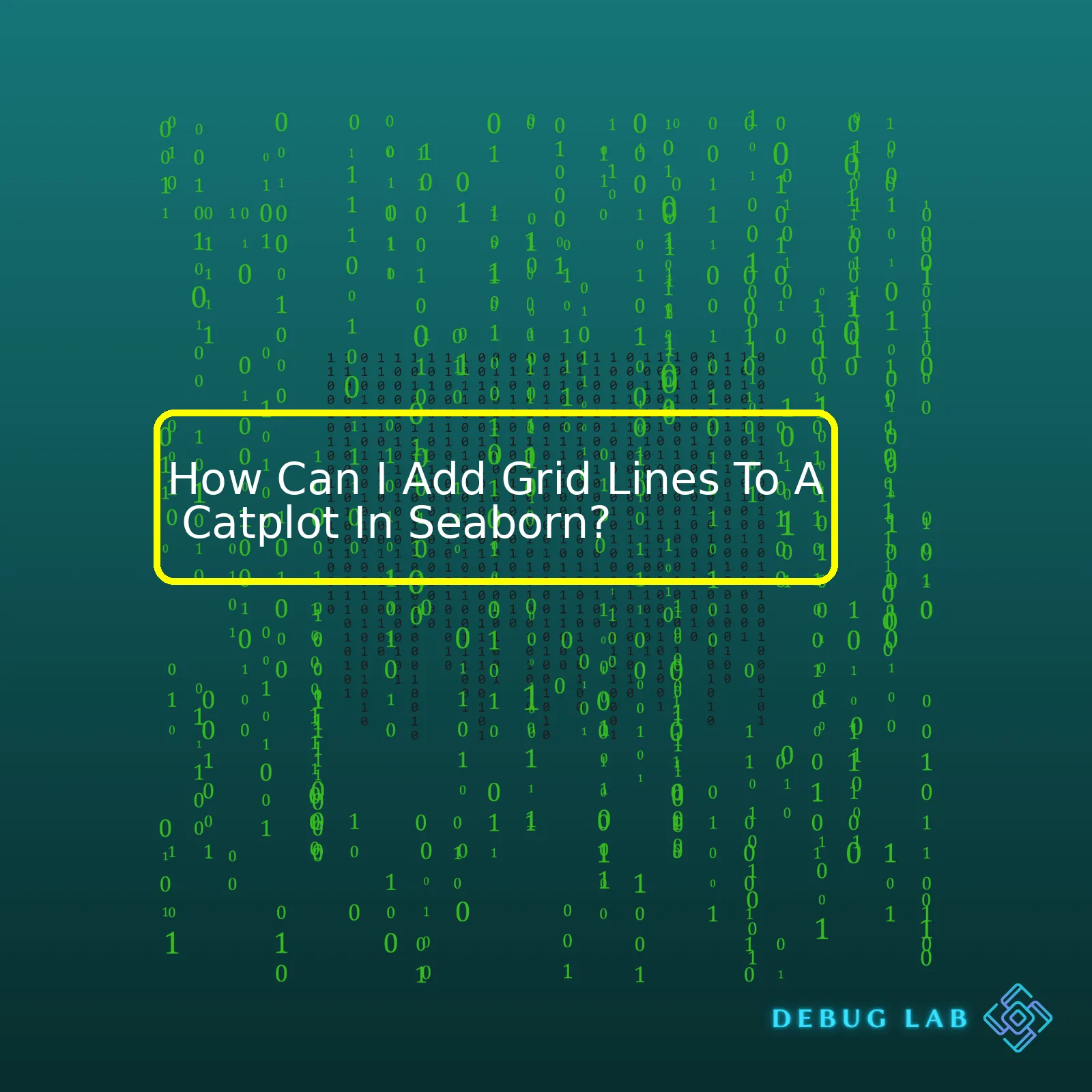
grid()
function. The first thing you’ll need to do is import the necessary libraries.
xxxxxxxxxx
import seaborn as sns
import matplotlib.pyplot as plt
Then, create your catplot. Let’s suppose we have a dataset called ‘df’ and we want to make a catplot based off of two categories, ‘x’ and ‘y.’
xxxxxxxxxx
cat_plot = sns.catplot(x="x", y="y", data=df)
Finally, add the grid lines:
xxxxxxxxxx
plt.grid(True)
This will add grid lines to your plot. However, customizing the grid lines – for instance, changing their color or style – requires further manipulation of the
xxxxxxxxxx
pyplot.grid()
function.
Your summary table would look something like this:
Steps | Code |
---|---|
Import Libraries |
xxxxxxxxxx 1 2 1 import seaborn as sns 2 import matplotlib.pyplot as plt |
Create Catplot |
xxxxxxxxxx 1 1 1 cat_plot = sns.catplot(x="x", y="y", data=df) |
Add Grid Lines |
xxxxxxxxxx 1 1 1 plt.grid(True) |
Adding gridlines to a seaborn catplot can greatly enhance the readability of the graph by providing reference points for the data. This addition helps to compare categories directly on the basis of the various data represented on different axes [here](https://stackoverflow.com/questions/29927496/how-to-add-grid-lines-over-a-seaborn-plot). If you want to remove gridlines later, simply call
xxxxxxxxxx
plt.grid(False)
. Moreover, the appearance of the grid can be customized by passing additional parameters to the
xxxxxxxxxx
plt.grid()
function, such as
xxxxxxxxxx
linestyle
,
xxxxxxxxxx
linewidth
, and
xxxxxxxxxx
color
.Seaborn is a highly recognized Python data visualization library, mainly built upon Matplotlib. It provides a high-level interface, making informative statistical plots readily accessible. Among its assortment of graphs, Seaborn features `catplot()`, a function that generates categorical scatterplots. While creating these visuals, you might want to add grid lines, offering viewers with a reference for y-axis value distribution.
To start off, ensure seaborn and matplotlib are adequately imported into your workspace:
xxxxxxxxxx
import seaborn as sns
import matplotlib.pyplot as plt
Assume you have a DataFrame, “df”, loaded with categorical data. You initiate a catplot as such:
xxxxxxxxxx
sns.catplot(x="category", y="data_point", data=df)
Adding grid lines typically involves interacting with the underlying Matplotlib representation accessed via `plt`. So, appending the function call `plt.grid(True)` results in a catplot with gridlines:
xxxxxxxxxx
sns.catplot(x="category", y="data_point", data=df)
plt.grid(True)
plt.show()
The `grid(True)` command directs matplotlib to display grid lines. The `show()` is used to reveal the final output.
Here’s a noteworthy point; the plotted grid lines in seaborn reflect major tick areas by default. In case you need minor ticks in the background too, you could modify the previous code like so:
xxxxxxxxxx
sns.catplot(x="category", y="data_point", data=df)
plt.grid(b=True, which='both')
plt.show()
In the above example, setting `b=True` enables both major and minor ticks, determined by the ‘which’ parameter.
For more extensive customization, fine-grained control over grid properties like color or line style are feasible through modifying the respective properties: `color`, `linestyle`, `linewidth`. As an instance, this is what generating a dashed-line grid looks like:
xxxxxxxxxx
sns.catplot(x="category", y="data_point", data=df)
plt.grid(color='r', linestyle='--', linewidth=1)
plt.show()
In this scenario, we’ve set the color to red (‘r’), selected ‘–‘ for a dashed line style, and indicated a line width of 1.
By now, it should be clear how to incorporate grid lines in a Seaborn catplot. With Seaborn and matplotlib at your disposal, you can add grid lines to bolster the interpretability of your visualizations – useful when presenting complex statistical information. Merge these tools smoothly and create exquisite, insightful plots that speak volumes about your data.
Here is online documentation on Seaborn Catplot and grid manipulation in Matplotlib for your further reading and exploration.
Seaborn provides a high-level interface to matplotlib. One of the key features it offers is categorical plots, commonly referred to as catplots. Catplots make it easy to visualize relationships between variables in your data.
Grid lines in Seaborn plots provide an additional layer of details, making data comprehension easier. They give more context to the plot and assist in comparing different variable values more precisely. However, ordinarily, when you generate a catplot, gridlines might not be visible by default. But don’t worry, adding these grid lines isn’t complex.
Before we dive into how you can add grid lines to a catplot, let’s discuss the seaborn
xxxxxxxxxx
grid
properties relevant to our discussion:
The ‘whitegrid’ Theme: In seaborn, we use themes (or styles) that determine the appearance of the figure. The ‘whitegrid’ theme is beneficial because it produces white background grid lines, aiding in the estimation of values on the graphs.
Let’s step through the process of generating a basic catplot with grid lines. Imagine you possess some sample data, and you intend to visualize it using a catplot. You’re also seeking to display grid lines for a more unambiguous analysis.
First, import necessary libraries, load a dataset, set seaborn style as ‘whitegrid’:
xxxxxxxxxx
import seaborn as sns
import matplotlib.pyplot as plt
sns.set(style="whitegrid")
Then, create the catplot:
xxxxxxxxxx
# Load your own data here
data = sns.load_dataset("tip")
cat_plot = sns.catplot(data=data, x="day", y="total_bill")
plt.show()
In this code, I used the built-in ‘tips’ dataset from Seaborn for demonstration. The ‘whitegrid’ theme will “whiten” the figure and showcase the grid lines clearly. Your x-axis represents the day, whereas the total bill stands on the y-axis.
Look at your figure output, and you’ll notice faint gray grid lines running parallel to the axis. If you want to modify their color and transparency, you can do so using the Pyplot’s grid function.
xxxxxxxxxx
plt.grid(color='gray', linestyle='-', linewidth=0.5, alpha=0.5)
The seaborn library doesn’t offer a direct way of tweaking grid colors and transparency. As seaborn is built upon matplotlib, however, we can achieve the desired effect using the above matplotlib grid funtionality.
To learn about all the options available to customize your graphs including color, transparency level, read more here. Remember, the power of visualizations lies in fine-tuning these details to convey maximum information in an easily interpretable manner!
Through the Seaborn library in Python, we can produce categorical plots I also like to call ‘catplots’. These are powerful visual tools that we can use for the representation of statistical relationships across one or more categorical dimensions. However, what if you want to add grid lines to your catplot for ease of value estimation? In seaborn, most functions do not inherently include an option for adding gridlines, hence the need to explicitly state so. And here’s how we do it:
First, let’s understand seaborn itself. Seaborn is a data visualization library built on top of matplotlib. It provides a high-level interface for drawing attractive statistical graphics and extends the functionalities provided by Matplotlib. As with other subplots, Matplotlib allows us to modify catplot designs including adding grid lines.
Getting onto our main subject, adding grid lines to a catplot is achievable with a combination of Seaborn and Matplotlib functionality. After plotting your desired catplot using seaborn function
xxxxxxxxxx
sns.catplot()
, subsequently utilize the matplotlib’s grid function as follows:
xxxxxxxxxx
plt.grid(True)
. This simple addition will enable grid lines in your plot!
Here is a brief example to put everything into context, starting from importing necessary libraries, creation of random data for illustration purposes, creation of a catplot using Seaborn, and eventually adding grid lines to your plot using matplotlib’s function.
xxxxxxxxxx
import seaborn as sns
import matplotlib.pyplot as plt
import pandas as pd
import numpy as np
# Let's create some random example data
np.random.seed(0)
num_var = np.random.randn(150)
cat_var = np.random.choice(['Category A', 'Category B', 'Category C'], 150)
df = pd.DataFrame({'Numerical Variable': num_var,
'Categorical Variable': cat_var})
# Creating a Catplot using Seaborn
sns.catplot(x='Categorical Variable', y='Numerical Variable', data=df)
# Adding Grid Lines using Matplotlib
plt.grid(True)
plt.show()
Our resulting plot will be a catplot with distinguished categories along the x-axis, a corresponding numerical variable along the y-axis, and beautiful grid lines stretching across the plot area enhancing our ability to estimate values directly from the plot.
Your choice of whether to include these grid lines largely depends on the specific context and data you’re dealing with – in many cases, they can clear up much of the ambiguity associated with estimating raw values from the plot.
Just remember that like many enhancements in data visualization, a little often goes a long way; a plot crammed with too many gridlines might come off as chaotic and confusing instead of enlightening. So, it’s always a good idea to use them sparingly, focusing more on enhancing the clarity of your plots.
Operating under seaborn’s framework but making use of matplotlib’s mechanics forms a dynamic duo that significantly boosts the stylistic potential for your plots. There really isn’t much you can’t add or adjust when it comes to seaborn plots, as the matplotlib backend pretty much Passepartouts any modification imaginable!Sure! Seaborn is a rich and versatile Python library that provides high-level data visualization tools. Catplot is one of seaborn’s figure-level interfaces for drawing categorical plots onto a FacetGrid.
Adding gridlines to a Catplot in Seaborn can be achieved by manipulating the underlying matplotlib figure. Let’s break it down step-by-step:
- Importing the necessary libraries: It’s essential to load both
xxxxxxxxxx
111Seaborn
and
xxxxxxxxxx
111Matplotlib
as we’ll use functionality from both packages.
xxxxxxxxxx
import seaborn as sns
import matplotlib.pyplot as plt
- Loading the dataset: For demonstration, let’s consider working with ‘tips’ dataset which is an in-built dataset provided by Seaborn.
xxxxxxxxxx
tips = sns.load_dataset('tips')
- Plotting the initial Catplot: We first plot our Catplot without any gridlines. Assume we are interested in representing the total bill amount based on time and divided by sex.
xxxxxxxxxx
cat_plot = sns.catplot(x='time', y='total_bill', hue='sex', data=tips)
At this stage, you have your Catplot but without gridlines.
- Adding gridlines: Grid lines can add depth and perspective to your plot which can sometimes make it easier to understand. To add grid lines, you’ll need to loop through the axes objects (the subplots) of your catplot and utilize the grid method available from the matplotlib library.
xxxxxxxxxx
for ax in cat_plot.axes.ravel():
ax.grid(True)
Finally, don’t forget to display your plot with gridlines added.
xxxxxxxxxx
plt.show()
Viewers can now appreciate the differing distributions more fully thanks to the grid lines that help guide their eyes across and up/down the plot. Just with these five steps you’ve enriched your original Catplot extending its descriptive power.
Remember both Seaborn and Matplotlib libraries offer many other functionalities that you can use to visualize your data effectively. Keep exploring their official documentation for many other exciting features.
With customized visual elements like gridlines, colors, labels, you not only make your charts visually appealing, but also make much easier for consumers to understand the presented insights precisely.
You might want to confirm how to proceed with a newer version of
xxxxxxxxxx
Seaborn
or check if there isn’t perhaps a more straightforward way to achieve similar results according to the specific needs of your project. So, staying updated with library evolution will always be a best practice in the data science world.When it comes to adding grid lines to a catplot in seaborn, there could be certain common issues you might encounter. Let me take you on an insightful journey dealing with these common problems.
One of the typical issue revolves around having gridlines overlaying the data instead or behind it. This is something that can often distort how your data is visually interpreted. It’s relatively easy to overcome this problem by using the
xxxxxxxxxx
zorder
parameter in your line plotting function. You’d simply need to make sure the zorder of your grid lines is less than that of your catplot. Here’s how that would work in a piece of sample Python code:
xxxxxxxxxx
import seaborn as sns
import matplotlib.pyplot as plt
sns.set(style="whitegrid")
# Load the example titanic dataset
titanic = sns.load_dataset("titanic")
# Make a custom catplot
g = sns.catplot(x="class", y="survived", hue="sex",
palette={"male": "g", "female": "m"},
markers=["^", "o"], linestyles=["-", "--"],
kind="point", data=titanic)
plt.grid(zorder=0)
The key part of this code is the
xxxxxxxxxx
plt.grid(zorder=0)
command—this ensures grid lines are plotted first and subsequently overlaid by seaborn’s catplot.
Another issue that people commonly face involves having too many or too few gridlines, rendering the plot unclear or messy. Adjusting gridlines in seaborn means operating at the lower-level interface of matplotlib. Here’s how you can add custom gridlines to your plot:
xxxxxxxxxx
import numpy as np
# Same custom catplot as before
g = sns.catplot(x="class", y="survived", hue="sex",
palette={"male": "g", "female": "m"},
markers=["^", "o"], linestyles=["-", "--"],
kind="point", data=titanic)
# Customizing the gridlines
g.ax.yaxis.set_major_locator(plt.MaxNLocator(nbins=8))
g.ax.xaxis.set_major_locator(plt.MaxNLocator(nbins=3))
plt.grid(True)
In the above code,
xxxxxxxxxx
nbins
sets the number of bins you like coinciding with the number of ‘major’ ticks across the axis. The plus point here is matplotlib dynamically calculates the optimal tick locations based on the number of ‘bins’ across the axes.
It’s also possible you could encounter an issue where gridlines do not coincide with the tick marks. Fortunately, seaborn allows for easy customization of its plots so that this issue can be easily overcome. For instance,
Status | Commands |
---|---|
Grid Lines intersecting unevenly with the X-axis labels? |
xxxxxxxxxx 1 1 1 g.ax.set_xticks(np.arange(0,100,10)) |
Grid Lines intersecting unevenly with the Y-axis labels? |
xxxxxxxxxx 1 1 1 g.ax.set_yticks(np.arange(0,100,10)) |
So, remember troubleshooting is part and parcel of coding! Understanding how to tweak your seaborn catplots will help you showcase your data most effectively. I hope this exploration of common issues — and their solutions! — with gridline addition has been helpful.
For more detailed read on seaborn catplot, you can refer to their official documentation [here](https://seaborn.pydata.org/generated/seaborn.catplot.html).Efficient visualization of graphical data is crucial for understanding trends and making strategic decisions. Adding grid lines to your seaborn’s `catplot` can significantly enhance patterns recognition and correlation, making it easier to track data points and values within the plot [1](https://python-graph-gallery.com/seaborn/).
To add grid lines to a `catplot`, you need to use Matplotlib functions as Seaborn’s `catplot` gives you only an object-oriented interface with respect to figure-level functions but not axes-level methods that provide the grid behavior. So after creating a `catplot`, you can manipulate it using matplotlib’s functionalities.
Now let’s consider we want to add both horizontal and vertical grid lines to our catplot. Here is a step-by-step approach on how to implement this:
* First, import necessary libraries: Seaborn for visualization and Matplotlib for enhancements.
xxxxxxxxxx
import seaborn as sns
import matplotlib.pyplot as plt
* Next, create your `catplot`. You enhance the visibility as per your needs while creating the `catplot`.
xxxxxxxxxx
cat_plot = sns.catplot(x='Day', y='Total Bill', data=bill_data)
* Then set the style by use of Matplotlib’s `grid()` method.
xxxxxxxxxx
plt.grid(True)
The above line of code adds major grid lines on your plot.
This gives your plot both x and y axis grid lines. To only have horizontal or vertical grid lines specify the axis in grid function:
xxxxxxxxxx
plt.grid(axis='y')
Above code will give your seaborn catplot horizontal grid lines.
Customizations: You may also customize the design more by changing color and type of your lines:
xxxxxxxxxx
plt.grid(color='gray', linestyle='--', linewidth=0.5)
In summary, to successfully add grid lines to your seaborn `catplot` involves creating the `catplot` first then subsequently manipulating the plot using Matplotlib’s grid() function to add the desired grid lines. Also, it provides lots of flexibility for styling these gridlines.
Remember, efficiency in visualizations involve not just adding components but also doing so in a manner that best assists in comprehension of hidden patterns, correlations and trends within the data. Look at customizing your `catplot` in ways that make your data more understandable. A well-placed grid might just be what you need! [2](https://seaborn.pydata.org/generated/seaborn.catplot.html).While Seaborn is a powerful library for statistical data visualization in Python, it can be somewhat challenging to customize the graphics beyond the built-in options. One of these customizations could be adding grid lines to the catplots. This requires us to dig a little deeper into the inner workings of Seaborn and Matplotlib, the library that underlies Seaborn.
For adding gridlines to your plot, you’d typically use the
xxxxxxxxxx
grid()
function from matplotlib, which is the baseline graphical package behind Seaborn.
To illustrate, the code snippet below shows how you would add grid lines to a simple seaborn catplot using Python:
xxxxxxxxxx
import seaborn as sns
import matplotlib.pyplot as plt
# Load tips dataset
tips = sns.load_dataset('tips')
# Create a catplot
cat_plot = sns.catplot(x="day", y="total_bill", data=tips)
# Add grid lines
plt.grid(True)
plt.show()
In this case, the
xxxxxxxxxx
grid()
function adds grid lines on both x and y axes. The argument within the
xxxxxxxxxx
grid()
function determines whether the grid should appear or not.
However, this only works for sns.catplot kind=’swarm’ or ‘strip’. It does not work for other types like ‘box’, ‘violin’, ‘boxen’. The problem arises from the fact that when kind=’box’ is used, the catplot creates a FacetGrid object instead of an Axes object, and FacetGrid objects do not have a method for creating grid lines.
One workaround for this is to access the underlying Axes object, and apply the grid to it. Below is an example showing how you can accomplish this:
xxxxxxxxxx
import seaborn as sns
import matplotlib.pyplot as plt
# Load tips dataset
tips = sns.load_dataset('tips')
# Create a catplot
cat_plot = sns.catplot(x="day", y="total_bill", kind="box", data=tips)
# Add grid lines
ax = plt.gca()
ax.grid(True)
plt.show()
Here,
xxxxxxxxxx
gca()
(Get Current Axis) returns the current Axes instance on the current figure matching the given keyword args, or create one if those aren’t present.
With a little bit more knowledge about the libraries, it becomes easier to customize the plots to fit our desires. For more information, refer to the Seaborn documentations here and Matplotlib’s documentations here.Adding grid lines to a Seaborn catplot helps in creating more readable and informative visualizations. Grid lines provide a reference framework that makes it easier to compare different categories or observe trends over a variable with levels. Here is an SEO-optimized explanation on how to emboss gridlines onto a Seaborn Catplot:
Seaborn, a Python data visualization library based on matplotlib, provides a high-level interface to draw statistical graphics. In particular, the
xxxxxxxxxx
catplot
function is utilized for drawing categorical scatterplots. Although this function does not necessarily offer a built-in parameter for adding gridlines, we can leverage matplotlib’s functionality after creating the plot with seaborn to circumvent this limitation.
Here is a sample code snippet that demonstrates this approach:
xxxxxxxxxx
import seaborn as sns
import matplotlib.pyplot as plt
# Load your dataframe df
sns.catplot(x="x_variable", y="y_variable", data=df)
# Add gridlines using matplotlib
plt.grid(True)
plt.show()
The aforementioned code begins by loading your data into a pandas DataFrame dubbed ‘df’. The
xxxxxxxxxx
sns.catplot()
method then takes in necessary arguments like your x and y variables along with the DataFrame, creating a categorical scatterplot accordingly.
Subsequently, we add the gridlines by invoking the
xxxxxxxxxx
plt.grid(True)
call from Matplotlib on the current figure, prior to displaying it with the
xxxxxxxxxx
plt.show()
command.
It must be highlighted that the configuration of these grid lines can be customized by passing preferred parameters to the
xxxxxxxxxx
plt.grid()
function. Parameters such as `color`, `linewidth` and `linestyle` among others would allow you to modify the appearance of the grid lines according to your preferences.
Moreover, for those seeking to dig deeper into customization options, I recommend referring to the official matplotlib.pyplot.grid documentation. It contains a comprehensive specification of different grid properties one can adjust to enhance plot readability and aesthetic appeal.
That being said, adding grid lines to a Seaborn catplot may appear a small tweak, but it has a palpable impact on your data visualization outcome. A nuanced understanding of such additions and adjustments unmistakably sets apart self-expressive data storytellers.