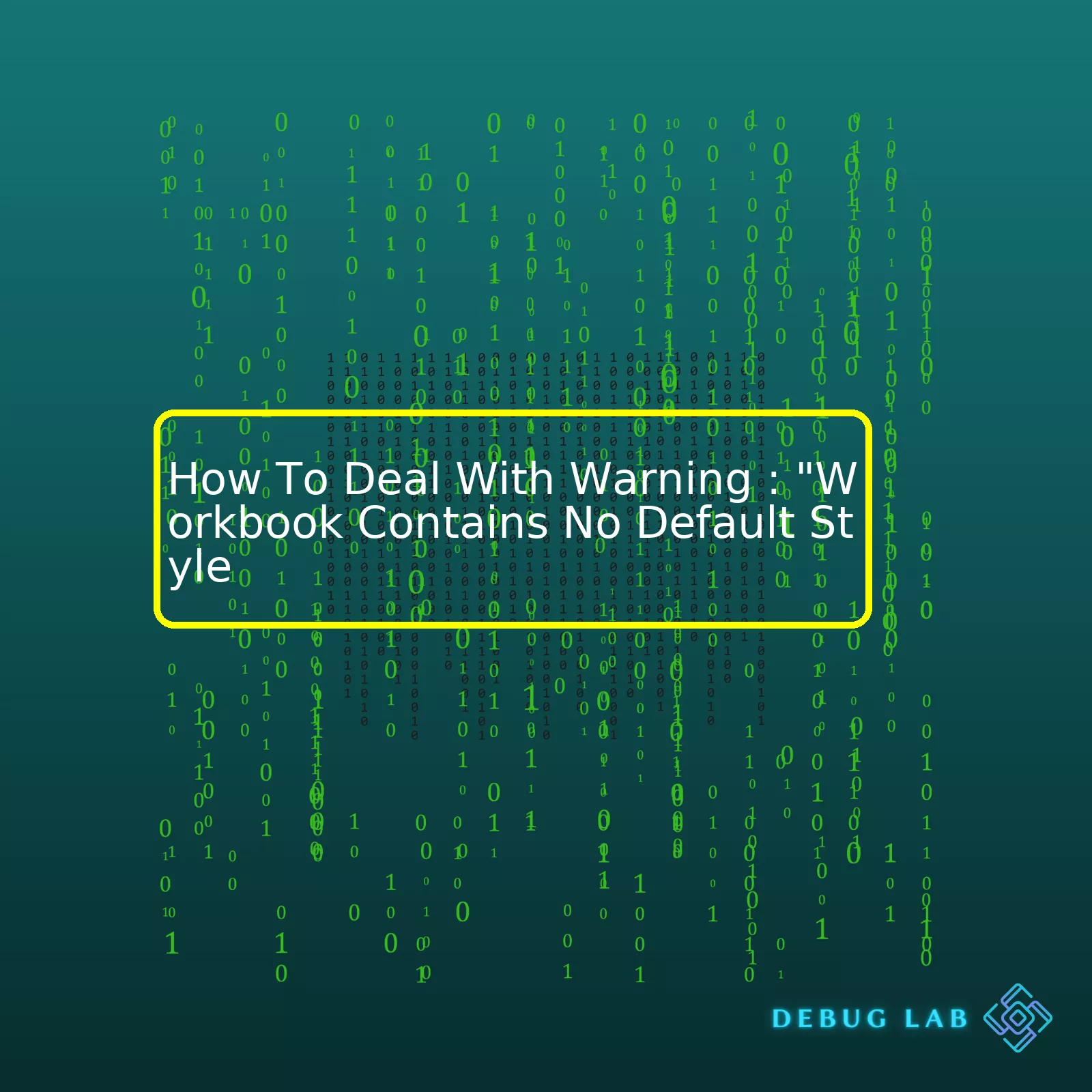
Firstly, the style warning is commonly triggered when there is an absence of the default cell style, or “Normal”, which should ideally be contained within every workbook to facilitate uniform formatting. Secondarily, a corrupted file, carrying over styles from previous files, or executing certain programs may also prompt this notification.
To resolve this issue successfully, we have multiple approaches. Here’s the knowledge presented in an HTML table format:
Solution | Step-by-Step Process |
---|---|
Reset Default Style |
|
Delete Extra Styles |
|
Repair Corrupted File |
|
Additionally, utilizing third-party software to automatically clean-up unnecessary styles accumulated in the workbook may be beneficial, especially if you’re working with large datasets. There are numerous online resources that can guide you through this and similar processes. Moreover, if the workbook was created using another spreadsheet program (like OpenOffice Calc), transfer the data to a new Excel Workbook helps remove the peculiar styling issues.
Nonetheless, before applying these solutions, always remember to back up your file to prevent any unintentional data loss. These ways radically assist you to rectify the error smoothly while working seamlessly with Excel’s myriad of offerings.The “Workbook Contains No Default Style” warning usually crops up when you are working with Apache POI, a popular Java library used for reading and writing Microsoft Office files. Specifically, this warning is associated with Excel (.xls or .xlsx) files. The root of this issue might be the fact that we are attempting to write an Excel file without defining a default cell style (like fonts, background color, text alignment, etc.).
Here’s how it generally happens. When creating an Excel spreadsheet from scratch using Apache POI, certain basic properties like row height, column width, cell font, etc., need to be set as per user requirements. In brief, this process involves establishing certain default styles for these elements. If these default styles are not defined during the Excel file creation process, Apache POI will generate a warning saying “Workbook contains no default style.”
This warning doesn’t imply any error or exception. Essentially, it won’t halt your program flow. What it does is alert you to a missing component – in our case, a default cell style. Nevertheless, if you would like to address this warning, here are a few methods you can consider employing:
// creating a default cell style CellStyle style = workbook.createCellStyle(); Font font = workbook.createFont(); // setting font details font.setFontHeightInPoints((short) 11); font.setFontName("Arial"); font.setColor(IndexedColors.BLACK.getIndex()); font.setBold(false); font.setItalic(false); style.setFont(font); // associating our font with the style workbook.setDefaultColumnStyle(0, style); // applying the style to the column
In this code, we first create a new CellStyle object and a new Font object. We set up the font details such as size, name, color, and effects like bold and italic using the appropriate setter methods. Subsequently, the setup font is associated with the created style. Finally, we apply this style to a column in the workbook using the setDefaultColumnStyle method.
Another workaround would be to suppress POILogFactory warnings. This might not be an ideal situation because it involves hiding warnings rather than addressing them directly, but it comes as a quick fix when you don’t want to be distracted by warnings:
POILogFactory.getLogger(POIFSFileSystem.class).setLevel(org.apache.log4j.Level.FATAL);
Overall, dealing with the “Workbook contains no default style” warning revolves around defining a default style for your workbook or handling the way these warnings appear on your console.
Want to dive deeper? [Apache POI official documentation](https://poi.apache.org/) will certainly offer more context and solution pertaining to this warning.
A warning stating “Workbook Contains No Default Style” may appear when you’re working on Excel via Apache POI, a powerful Java library provided by Apache Software Foundation to manipulate various file formats based upon Microsoft Office. Despite its broad characteristics and functionalities, there are certain issues to navigate, one of them being the “Workbook contains no default style” warning.
The root cause of this warning typically falls into three categories:
- Absence of default styles in output Excel: This primarily happens when Apache POI is used to create or modify an Excel workbook. If the code doesn’t specify a default style to be applied, the software might prompt this warning.
- Inadequate workbook initialization: When creating a worksheet using Apache POI, proper initialization of both workbook and cell styles is essential. Failure to correctly initialize the workbook can summon this warning.
- Compatibility issues: Different versions of Excel have distinct features and compatibility settings. These differences might sometimes result in rendering problems leading to this warning popup.
To deal with this warning, a number of solutions can be approached:
Create a default style for the workbook. By defining a default style for the cells within the workbook, you can eliminate such issues. It can be accomplished with a simple snippet of code:
XSSFWorkbook workbook = new XSSFWorkbook(); XSSFCellStyle style = workbook.createCellStyle();
Interface cellstyle with workbook creation. Instead of explicitly specifying the style after creating the workbook, integrate it with the creation process as follows:
Workbook workbook = new XSSFWorkbook(); CellStyle style = workbook.createCellStyle();
Ensure version compatibility. Ensure the version of Apache POI library you’re utilizing is compatible with the Excel files you’re manipulating.
Despite these approaches, remember that “Workbook contains no default style” is just a warning, not an error. Your code will still execute, and your Excel file will still be generated with all of its data. Nevertheless, paying heed to warnings and resolving them ensures you follow good coding practices, hence optimizing the quality of your work.If you’ve started working in Excel and seen a warning that says “Workbook Contains No Default Style,” it may be because you or someone else previously altered the default style of the workbook, or the workbook was opened on a different version of Excel where the style definitions are not the same as your current version. Contrary to popular belief, setting a new default style for Excel workbooks can have pros and cons that need to be considered before proceeding with this action.
Pros of Setting a New Default Style
There are various benefits of setting a new default style in Excel:
* Consistency: The strength of setting a default style is that you guarantee consistency across all worksheets and workbooks. Maintaining consistent formatting helps to establish uniformity in your data presentation, making your documents easier to read.
* Time-saving: When you set a default style, you no longer need to repetitively make formatting changes to each worksheet created. This definitely saves a progressive amount of time in the long term.
* Brand representation: For businesses that rely on branded content, changing the default Excel style allows multiple users to represent the brand consistently in all Excel-based documents.
Putting these factors into perspective, changing the default style could seem like an excellent idea. However, there also lie some drawbacks in taking this action.
Cons of Setting a New Default Style
Every coin has two sides, and here’s what’s on the other side of setting a new default style in Excel:
* Compatibility issues: One downside is potential compatibility problems when sharing your workbooks with others who do not have the same default style.
* Confusion for other users: In a collaborative environment, setting a new default style might cause confusion among team members not accustomed to the change, leading to lower productivity until they get used to the new style.
* Lack of flexibility: If you frequently need different styles in your different worksheets, sticking with one default style might limit your flexibility.
Let me now guide you on how to deal with the warning “Workbook contains no default style.”
Dealing with this warning requires setting a new Default Style. Here’s how:
<steps to creating a default style in Excel> - Open Excel and click on the 'Home' tab. - In the Styles section, click on 'Cell Styles'. - Look for the Normal option (which is the default). If it's not there, you will need to recreate it. - Click on 'New Cell Style', enter the name as Normal, and define your desired formatting rules. - Once satisfied with your settings, click OK. </steps to creating a default style in Excel>
This process will relieve you of the recurring warning “Workbook contains no default style.” But, always remember to weigh the pros and cons as highlighted earlier.
For additional information and a detailed exploration on Excel Styles, you might refer to Microsoft’s support page.Sure, when you receive a warning like “Workbook Contains No Default Style”, it usually means that the Excel workbook you are using does not have a default style. The default style in Excel is used as a base upon which all the other styles and formats in your workbook are built on. So, this could potentially cause some formatting issues. But don’t worry, there’s an easy solution to fix this issue, which also involves creating your custom default style.
First, open up your Excel Workbook. Navigate to the ‘Cell Styles’ option which can be found within the Styles group on the Home tab. If you hover over it, you will see different cell styles appear including the default cell style.
In case your workbook doesn’t contain a ‘Normal’ style, which is often considered to be the default cell style, you can create one:
1. Click on the 'New Cell Style...' option located at the bottom of the 'Cell Styles' box. 2. Name this new style as 'Normal'. 3. Add any font style, color, size you want for this Normal style. 4. After making these changes, click on OK.
Now you have created your own custom ‘Normal’ style which can serve as the default style in your workbook.
To apply this newly created ‘Normal’ style across all the cells in your Excel spreadsheet:
1. Press Ctrl+A to select all cells in the current sheet. 2. Go back to the Cell Styles option. 3. Click on the 'Normal' style that you've just created.
This applies your custom ‘Normal’ style to all cells in the worksheet. From now on, any cell or range of cells that doesn’t have a direct format applied inherits this Normal style as its default format.
Additionally, if you want this custom ‘Normal’ style to be available in all your future workbooks, you need to save it as an Excel Template. Here’s how:
1. Save the current workbook with the custom 'Normal' style as an Excel template (.xltx). 2. Next time when you're going to start a new workbook, instead of choosing 'Blank workbook', choose 'Personal' > Your saved template.
By saving your workbook as a template, the Custom ‘Normal’ style will become the new standard for all your future workbooks, dealing effectively with the error: “Workbook Contains No Default Style”.
Restoring default styles in Excel, especially when you encounter the “Workbook contains no default style” warning, is important for maintaining uniform formatting and a clean, professional look. A change to cell style can affect your entire workbook, and therefore it’s crucial to know how to restore these styles correctly. So here’s a detailed guide on how to handle that pesky warning by restoring the original default styles.
Using The Styles Command
You’ll find the ‘Styles’ option in the ‘Home’ tab under ‘Cell Styles.’ Here’s how you can use it:
- Start by clicking the ‘Home’ tab.
- Then, click on ‘Cell Styles’ – you’ll see it in the ‘Styles’ group.
- An dropdown will appear, hit the ‘Reset to Match Style’ button – this will reset the selected cells to Excel’s built-in cell style.
If the problems persist, creating a blank workbook and importing data from the problematic workbook may be another solution worth considering.
Creating A New Workbook and Importing Data
The creation of a new workbook helps bypass the issue of altered default styles because a new workbook resets Excel formats to their defaults. Follow these steps:
- Go to ‘File’ and click on ‘New’. This will open a fresh workbook with default Excel styles.
- Next, go back to your problematic workbook and select the data you’d like to transport into the new spreadsheet.
- Copy the selected data (Control+C / Command+C).
- Return to the new workbook and paste the data (Control+V / Command+V). Remember, only the values, formats, and formulas will get copied over, not the altered styles.
Additionally, using VBA for handling this warning is also a smart workaround.
Handling the “Workbook Contains No Default Style” Warning With VBA Code
VBA, or Visual Basic for Applications, is a simple programming language used within Excel. Writing a quick script can allow you to bypass time-consuming styling issues. Note: Always remember to back up your files before running any script.
Here’s a simple VBA code snippet that you can use:
Sub ResetStyle() ActiveWorkbook.Styles("Normal").Delete Workbooks.Add ActiveWorkbook.Styles("Normal").Merge ActiveWorkbook.Close False End Sub
This `Sub` procedure does three things:
- Deletes the altered ‘Normal’ style from the active workbook.
- Opens a new workbook, which naturally has the original untainted ‘Normal’ style.
- Merges this correct ‘Normal’ style with the previously active workbook.
- Closes the newly created workbook without saving any changes.
Thanks to its flexibility and power, keeping this script handy in your VBA arsenal can save you countless hours of manual restyling.
To further enrich your Excel knowledge and master other essential Excel skills, consider visiting Office’s official support page.
Addressing the warning “Workbook Contains No Default Style” requires understanding why this issue arises in the first place. Basically, this occurs when an Excel document is saved using a version of Excel that differs from the one being used to open it, resulting in loss of default style information.
One advanced approach for dealing with this common workbook issue involves refreshing styles throughout your workbook. Here’s how:
1. First, search if excel has any unused styles and remove them. Use this code that runs through every cell in the workbook and identifies its style:
html
Sub RemoveUnusedStyles() Dim s As Style, st As Styles Dim rng As Range, wk As Worksheet Dim UsedStyles As Collection Set st = ActiveWorkbook.Styles Set UsedStyles = New Collection On Error Resume Next For Each wk In ThisWorkbook.Worksheets For Each rng In wk.UsedRange.Cells UsedStyles.Add st(rng.Style), CStr(rng.Style) Next rng Next wk Err.Clear For Each s In st If s.BuiltIn Then GoTo nextiteration On Error Resume Next UsedStyles.Item (s.NameLocal) If Err.Number <> 0 Then Err.Clear s.Delete End If nextiteration: Next s End Sub