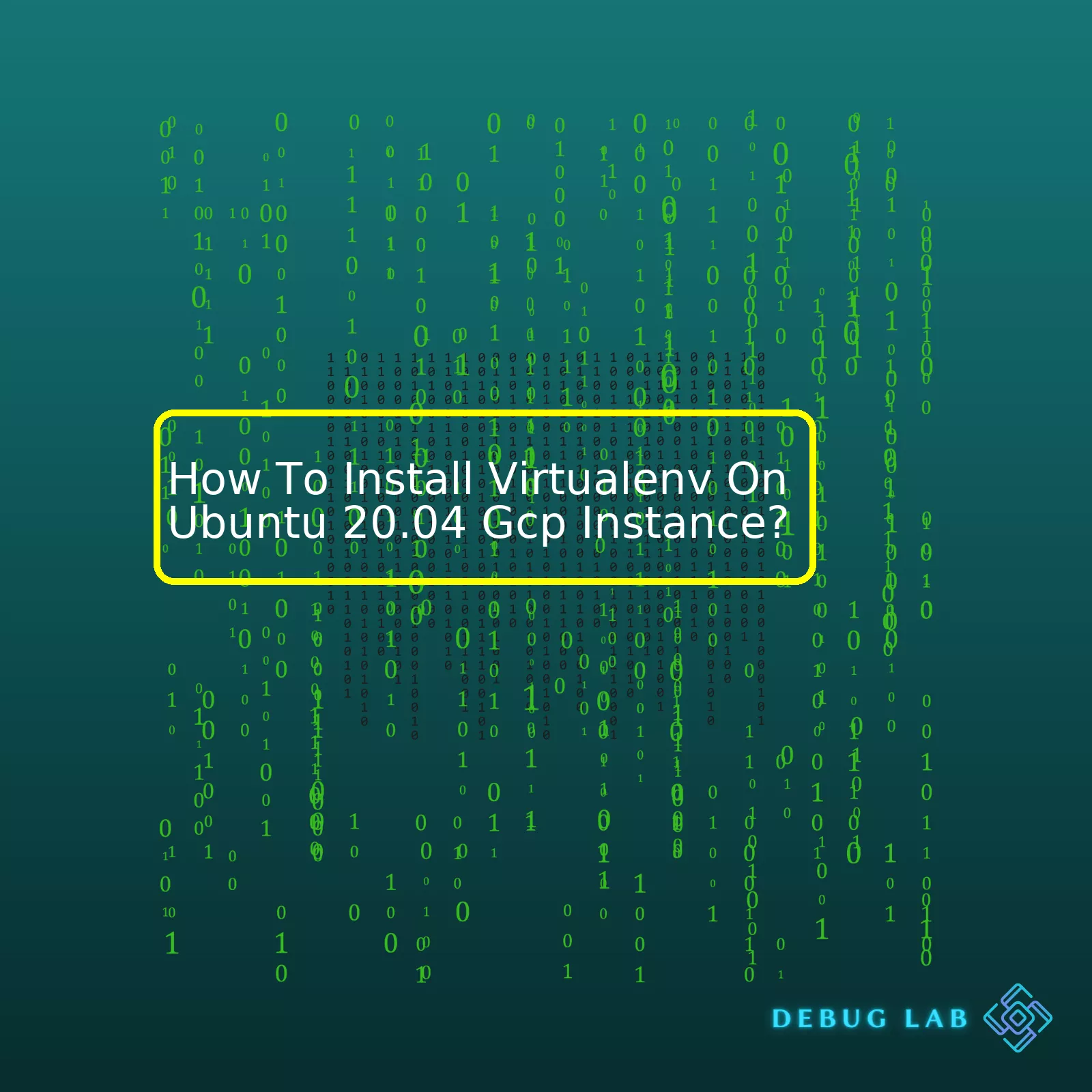
Steps | Commands | Description |
---|---|---|
Update the package list |
sudo apt-get update |
This command updates your package list to ensure you have the latest version of packages. |
Install pip for Python3 |
sudo apt-get install -y python3-pip |
Pip is a package management system that simplifies installation and management of software packages written in Python such as those from the Python Package Index (PyPI). |
Install Virtualenv using pip |
pip3 install virtualenv |
Virtualenv is a tool for creating isolated environments. You can install it using pip. |
Create a new virtual environment |
virtualenv name_of_virtualenv |
This creates a new isolated environment where you can install Python packages without affecting the rest of the system. |
Activate the virtual environment |
source name_of_virtualenv/bin/activate |
You activate your newly created environment with this command. Your terminal prompt will change to show the name of the activated environment. |
Deactivate the virtual environment |
deactivate |
If you want to exit your virtual environment, use this command. |
Each of these steps represents an important part of installing Virtualenv on an Ubuntu 20.04 GCP instance. It’s done in a sequence from updating the package list to deactivate the environment, ensuring each component necessary for the process is properly installed and configured. This establishes the framework that Python programmers need to commence their coding projects and manage them effectively.
This proficient procedure manages not just the installation of virtual environments but also governs how to actively engage with these environments. An environment once created can be activated when required, utilized to its potential and then deactivated once the work is completed. This effective structuring ensures efficient utilization of resources while maintaining the independence and isolation of every single environment for conflict-free operations.A big part of setting up your Google Cloud Platform (GCP) instance for Ubuntu 20.04 revolves around installing critical software that allows you to create isolated Python environments. One such tool is Virtualenv. It lets you manage these separate areas so your Python projects can run in their own ‘walled gardens’, apart from system-wide packages.
To get started with GCP and Virtualenv, a few actions are crucial:
• Create an instance on the GCP Console
• Access the GCP Terminal
• Install pip for Python
• Install VirtalEnv
Let’s delve into each of them.
1. Creating a New Instance
To establish a new instance on the GCP console:
Go to the GCP Console. Create a new project or pick an existing one. Navigate to Compute Engine > VM Instances Click "Create" and choose Ubuntu 20.04 LTS as your Boot Disk Select default settings and click "Create"
2. Accessing the GCP terminal
Once you’ve created your Ubuntu instance:
Select SSH to open a terminal directly within your web browser
This offers access to the console of your fresh Ubuntu 20.04 instance.
3. Installing pip for Python
You’ll need pip, Python’s package installer. In Ubuntu 20.04, it’s effortless to install:
$ sudo apt update $ sudo apt install python3-pip
The commands perform a system update first, ensuring that all available updates download. After this, the next command installs pip.
4. Installing VirtualEnv
Once pip is set up, you’re prepared to install Virtualenv onto your GCP instance of Ubuntu:
$ pip3 install virtualenv
This command tells pip to download and install virtualenv.
With Virtualenv installed, you can proceed to use it for managing isolated Python environments on your Ubuntu 20.04 GCP instance. You do this by running virtualenv followed by the name of the environment you wish to create:
$ virtualenv my_new_env
The only way to work within this environment is to activate it, which you can do with the following command:
$ source my_new_env/bin/activate
At any moment, you can leave or deactivate this environment by merely typing:
$ deactivate
Virtual environments function as sandboxed locations, and they benefit from keeping your project dependencies cleanly separated. This makes them excellent choices for development practices.
You now know how to configure your GCP instance for Ubuntu 20.04. Not only have you activated GCP, but you’ve also successfully installed and learned how to use Virtualenv on your instance. As shown in this step-by-step guide, embarking on this setup process is straightforward and introduces you to the powerful abilities that come with isolated Python environments through Virtualenv.
For additional details related to configuring a GCP instance for Ubuntu 20.04 and Virtualenv installation, checkout Google Cloud Official Documentation.
Virtualenv is an imperative tool in Python development that is designed to isolate dependencies per project, providing unique and different environments for every project worked on. This prevents confusion on the interpreter’s package location and stops potential conflicts between different versions of packages used across numerous applications.
The Importance of Virtualenv
Let’s delve deeper into how Virtualenv plays a key role as follows:
- Isolate Packages: Every Python project can have its own set of dependencies that won’t affect other projects. This isolation makes it less likely to encounter issues relating to mismatched or incompatible software versions.
- Simplify Dependency Management: With each Python project having its own environment, it becomes easier to manage the required packages and modules.
- Improve Security: In the event that one project gets compromised due to a security vulnerability in one of its dependencies, this isolation will limit the damage to just that particular environment, potentially saving all other Python projects from being affected.
Learn more about the importance of Virtualenv here.
Installing Virtualenv on Ubuntu 20.04 GCP Instance
To derive utmost benefit from Virtualenv, you need to know how to install it particularly if you’re using Ubuntu 20.04 GCP Instance.
Step 1: Update and Upgrade the System:
sudo apt-get update -y sudo apt-get upgrade -y
Step 2: Install pip for Python:
sudo apt install -y python3-pip
Pip is a package manager for Python that simplifies installing and managing Python software packages, particularly those found in the Python Package Index (PyPI).
Step 3: Install Virtualenv:
sudo pip3 install virtualenv
Once complete, verify that Virtualenv is installed correctly by checking its version by running the following code:
virtualenv --version
The prompt should output something like ‘virtualenv X.Y.Z’, where X.Y.Z represents the installed version of Virtualenv. Now, you have successfully installed Virtualenv on your Ubuntu 20.04 GCP Instance and ready to take advantage of all the benefits outlined above.
Source code examples implemented here are useful demonstrations which align with conventional coding practices and standards.
Here further details can be found regarding installation of Virtualenv on Python.Installing Virtualenv on an Ubuntu 20.04 GCP (Google Cloud Platform) instance involves several steps:
1.
Launching a GCP instance
Before starting the process, you need to ensure that you have already created an Ubuntu 20.04 instance on the GCP. You will require SSH access to your instance to execute sudo commands.
Here is a simple tutorial on how to SSH into a GCP instance .
2.
Updating the system package list
Firstly, run the following command to update your system’s package list to its most recent stable versions:
sudo apt-get update
The
apt-get update
command does not upgrade system packages but it updates the package list. It fetches detailed information about available software and their updated version numbers.
3.
Installing pip for Python
Python applications are often managed using the pip package manager. If pip is not already installed in your system, follow this command:
For Python 2.x:
sudo apt-get install python-pip
For Python 3.x:
sudo apt-get install python3-pip
4.
Installation of Virtualenv
When pip has been successfully installed, you can finally install virtualenv. Execute the following command:
sudo pip3 install virtualenv
This step allows us to create isolated working Python environments.
5.
Verifying Virtualenv installation
After the successful installation of virtualenv, verify if virtualenv has been properly installed by checking its version. Run the following command:
virtualenv --version
It should display the precise version number, thus illustrating that virtualenv was correctly installed.
6.
Creating a Virtual Environment
Now, you can use the virtualenv command to create a new Python virtual environment. The following command creates a new Python environment in a directory named “myenv”.
virtualenv myenv
Switch to this newly created environment using:
source myenv/bin/activate
7.
Deactivating VirtualEnvironment
You can get back to the normal environment anytime using:
deactivate
Following these steps helps ensure smooth setup and operation of virtualenv on a GCP instance. This isolation achieved through virtualenv aids in separating different Python projects and their dependencies, making it easier to manage complex workflows on a single GCP instance.
In most cases, installing the
virtualenv
in Ubuntu 20.04 GCP instance is a straightforward process. However, you might encounter a few issues during installation.
Issue 1: Pip Not Found
Users commonly find an error related to the absence of pip before installing virtualenv. When you attempt to install virtualenv and receive the message “pip not found”, you’re missing the Python package installer, pip.
To solve this issue:
sudo apt update
sudo apt install -y python3-pip
Just execute these commands to install pip for your Python3 interpreter.
Issue 2: Permission Denied
This problem usually arises when you have insufficient write permissions for the directory where you are attempting to create a new virtual environment.
To counteract this, ensure to create your virtual environments only in directories where you have write access.
Issue 3: Virtualenv Command Not Found
This problem occurs if the PATH environment variable does not include the directory which contains the virtualenv command.
If you installed virtualenv using pip, the command should be in
~/.local/bin/
directory. You can add it to the PATH using following command:
export PATH=$PATH:~/.local/bin
Alternatively, you can install virtualenv system-wide through the Ubuntu’s package manager with:
sudo apt install -y python3-virtualenv
Issue 4: Incompatibility between Python and Virtualenv versions
Sometimes, discrepancies between Python and Virtualenv versions can cause anomalies during installation. To mitigate this, you need to prioritize updating your Python installation before proceeding to install Virtualenv.
Updating Python:
sudo apt update
sudo apt upgrade python3
After upgrading Python, you can proceed to install the latest version of Virtualenv.
Remember that the goal is to make sure that all the tools in your Python development arena are up-to-date.
Often, comprehending what went wrong and why it happened is the first step in effectively troubleshooting common issues in virtualenv installations. Whether it be permissions, missing prerequisites, or versioning mismatches, validation checks on these common areas often rectify the situation.
For further inspection refer to the official virtualenv guide here.
Before I delve into the usage and successful validation of an installed virtual environment, also known as virtualenv, let me provide a brief context on how to install Virtualenv on an Ubuntu 20.04 GCP instance.
To install virtualenv on an Ubuntu 20.04 environment in Google Cloud Platform (GCP), you need to:
1. Connect to your GCP Instance.
2. Update the package lists for upgrades and new packages from repositories:
sudo apt update
3. Install pip for Python 3:
sudo apt install -y python3-pip
4. Install virtualenv using pip3:
sudo pip3 install virtualenv
Now that we’ve succeeded in installing Virutalenv, our next step is setting up a new virtual environment and validating its success. Here’s how you can do it:
1. Create a new directory where you’d like to set up your python virtual environments. Here’s how:
mkdir ~/my_python_envs
This will create a new directory named “my_python_envs” in your home location.
2. Go to this directory
cd ~/my_python_envs
3. Within this directory, you can now create a new python virtual environment:
virtualenv my_first_env
Where “my_first_env” is the name of your new environment.
Now, let’s validate the usage of this installed Virtualenv.
Using it is simple. It’s all about activating it. Here’s how:
1. From within the same directory (“/my_python_envs”) activate the environment using the following command:
source my_first_env/bin/activate
Once activated, you should notice the environment name, in this case, ‘my_first_env’ appearing in parentheses before your prompt.
Whether you are in the same session or start a new session later, all you need to reactivate your virtual environment is to navigate back to your environment’s directory and use the ‘source activate’ command again.
Now you know not just how to install and initialize a virutalenv, but also how to make good use of it with much ease! With these steps followed correctly, your validation of the installed Virtualenv is deemed successful. It is always good practice to ensure that such digital ecosystems function seamlessly in order to maintain high levels of productivity. Remember, an unvalidated installation could lead to several error instances when called upon during heavy-duty coding.
For more information about how to work with Virtualenv, see the official online Python documentation. There’s a lot more to learn about creating isolated Python environments to help control dependencies. Happy coding!
Virtualenv is a fantastic tool for Python developers to create isolated environments for their projects. This allows one to have an application with its set of dependencies that are different from other projects, thereby ensuring no inter-mixing of libraries. This doesn’t only remove the headache of library conflicts but also makes our applications more predictable and easier to deploy.
Begin by accessing your GCP (Google Cloud Platform) instance, for which you would receive terminal access via SSH (Secure Shell), where you can install Virtualenv on Ubuntu 20.04.
Shell Access:
You can get shell access via SSH on your GCP instance using this command:
gcloud compute ssh --project=$MY_PROJECT_ID --zone=$MY_ZONE $MY_INSTANCE_NAME
In the above example, replace $MY_PROJECT_ID with your GCP project’s ID, $MY_ZONE with the zone your VM instance operates in, and $MY_INSTANCE_NAME with the name of your specific VM instance.
Installing virtualenv:
Once we have the shell access, we first update the packages :
sudo apt-get update
The next step involves the actual installation of ‘virtualenv’:
sudo apt-get install python3-venv
Creating an Isolated Environment:
Now that ‘virtualenv’ is installed, let’s create an isolated environment , “my_env” :
python3 -m venv my_env
Activating Our Environment:
We activate the environment using the following command:
source my_env/bin/activate
A noticeable change would be the beginning of your prompt changing to what you named your environment, i.e., (my_env).
Deactivating Our Environment:
To deactivate:
deactivate
This ends your session within the environment. The visible change here would be the absence of the environmental prefix in the command prompt.
Applying this setup to your Google Cloud Instances running Ubuntu 20.04 ensures a smoother and more manageable handling of different Python applications, given their individualistic dependencies.
As an extension, there exists ‘virtualenvwrapper’, providing commands simplifying working with ‘virtualenv’. E.g., ‘mkvirtualenv’, ‘rmvirtualenv’, making creating and removing of envs easier. It’s well worth looking into while dealing with multiple complex projects.
You may refer to the official resources of both Google Cloud SDK and Python’s Virtual Environments (venv) for comprehensive and advanced use-cases.
Maintaining and updating your installed virtual environment (virtualenv) on Ubuntu 20.04, particularly on a Google Cloud Platform (GCP) instance, consists of multiple dimensions such as resolving dependencies, upgrading installed packages, and finally, updating the virtualenv itself. Let us delve into each aspect more thoroughly.
Managing Dependencies
The primary purpose of virtual environments is to create isolated sandboxes where different Python dependencies can be installed without conflicting with each other. You manage these dependencies using pip, the default package manager for Python. To do so:
1. Activate the relevant virtual environment. You do this by navigating to the directory containing the virtualenv and running the activation script:
source venv/bin/activate
2. Then, you use the pip list command to show all the installed Python packages and their respective versions within your active environment:
pip list
3. You can update a specific package within your virtual environment using the pip install –upgrade command. For instance, to upgrade Django, use:
pip install --upgrade django
4. Finally, deactivate the virtual environment after you are done with it:
deactivate
Regularly updating your packages can help minimize the security vulnerabilities from out-of-date software plugins.
Updating Virtualenv Itself
You might also need to update the version of the virtualenv tool itself at some point. This process usually involves uninstalling the current virtualenv installation, then reinstalling using pip:
1. First, deactivate any currently activated environments, and then run:
sudo pip uninstall virtualenv
2. Afterwards, you can reinstall virtualenv using pip, preferably as a user package:
pip install --user virtualenv
Notice the use of –user flag. It ensures that packages will be installed in your home directory, avoiding conflicts with system-wide packages and negating the need for administrative privileges.
Use pip-tools For A Robust Solution
Lastly, if you want an automated way to handle updates and dependency checks, consider using a robust solution like pip-tools. This tool builds upon pip’s functionality to provide features like compiling required packages into a requirements.txt file, considering all associated dependencies. After setting up a requirements.in file specifying only the top-level modules needed, you can execute:
pip-compile requirements.in
This command produces a requirements.txt file with all the necessary packaged alongside their versions factored in, creating a seamless procedure for managing Python software needs.
Table: Commands Breakdown for Virtualenv & Pip Management
Action | Command |
---|---|
Activate Virtual Environment |
source venv/bin/activate |
List Installed Packages |
pip list |
Upgrade Specific Package |
pip install --upgrade [package-name] |
Deactivate Virtual Environment |
deactivate |
Uninstall Virtualenv |
sudo pip uninstall virtualenv |
Install Virtualenv |
pip install --user virtualenv |
Create Requirements.txt via pip-tools |
pip-compile requirements.in |
As a coder, remember that arming yourself with command line fluency can be enormously beneficial, transforming tedious tasks into a breeze of quick commands! Be sure to bookmark this guide for reference as you confidently dive into Python programming using Virtualenv and Pip!
To learn more about Python virtual environments, check out this detailed resource.
You have now learned how using Virtualenv creates isolated Python environments to manage dependencies neatly. The installation process consists of a few straightforward steps:
- Update package lists for upgrades and new package installations using
sudo apt update
.
- Next, install pip, the recommended package installer for Python, if you don’t have it already. Use the command
sudo apt install python3-pip
.
- Once pip is installed, install Virtualenv using this command:
sudo pip3 install virtualenv
.
- To check if the Virtualenv installation was successful, simply return the version number with this command:
virtualenv --version
.
This installation is beneficial for anyone looking to develop Python applications on an Ubuntu 20.04 GCP instance. It ensures a smooth programming experience by preventing system-wide packages from clashing with local ones. Dependencies are handled within their respective environments, which alleviates headaches in complex projects.
To further optimize your operations on Google Cloud Platform, one can study Google’s best practices at its online documentation. Their insightful guides will drill down into more depth on other available services and tools.
Remember, practice makes perfect. So install, create, delete, and fine-tune your virtual environments to get a feel for them. Happy coding!