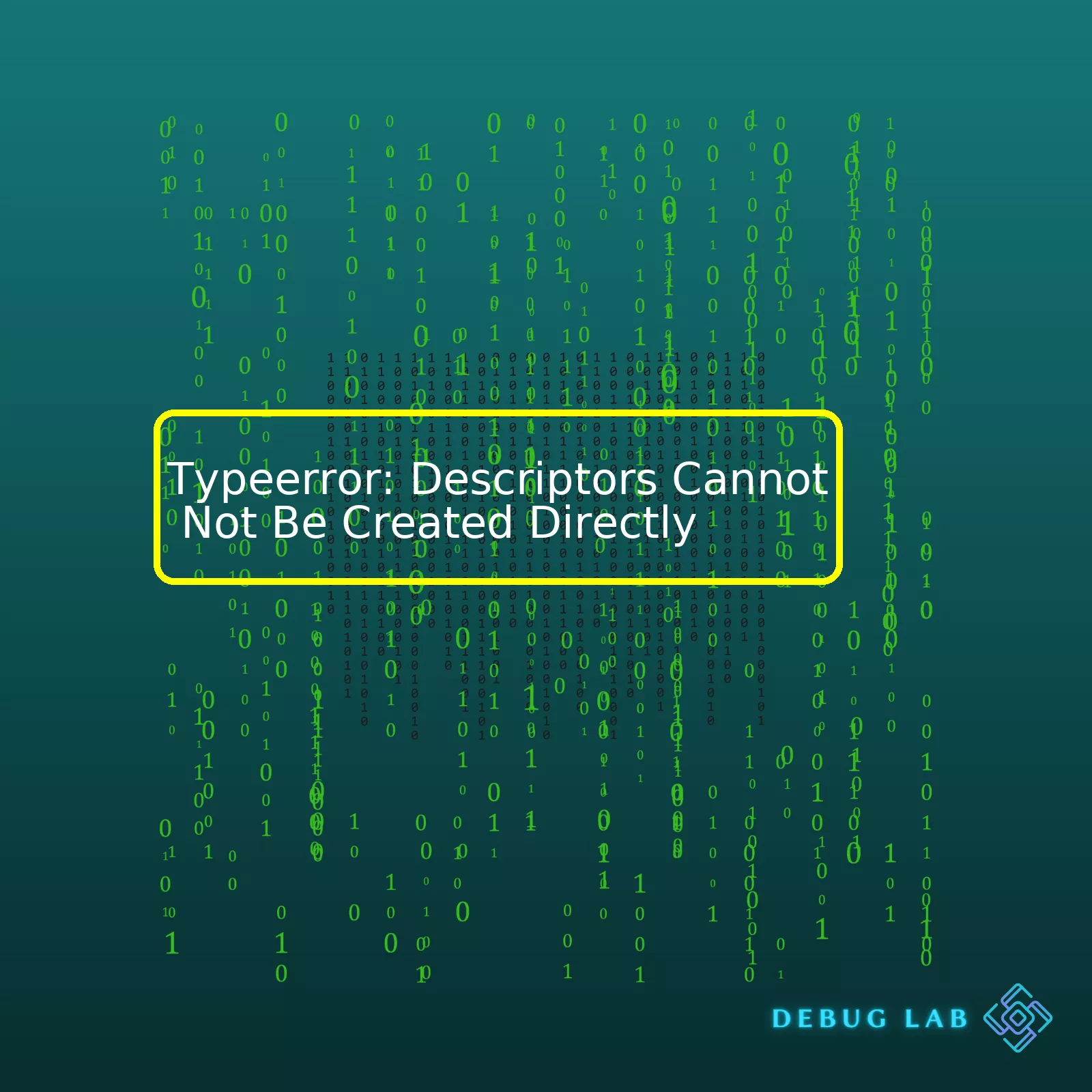
TypeError: Descriptors Cannot Not Be Created Directly is a common issue that most programmers run into, especially while working with Python. Before elaborating on the TypeError, let’s start by understanding what descriptors in Python are.
Error Type | Description | Causes | Solutions |
---|---|---|---|
‘TypeError: Descriptor cannot not be created directly’ | A TypeError is raised when an operation or function is applied to an object of inappropriate type. | This specific error typically occurs when trying to initialize or instantiate a descriptor directly. | Instead of instantiating directly, you should always attach descriptors to classes which will then be accessed via instances of those classes. |
A descriptor, in Python, is any object attribute with “binding behavior”, meaning any attribute whose access has been overridden by methods in the descriptor protocol. These methods include __get__, __set__, and __delete__. When you access an attribute, it checks if a descriptor has been defined and uses the descriptor methods instead of getting the attribute value from the object’s dictionary directly.
The reason we get ‘TypeError: Descriptors Cannot Not Be Created Directly’ is because descriptors are intended to be instantiated only once in a class definition. When you attempt to create an instance directly, Python doesn’t know how to handle and raise the ‘TypeError’.
Let’s demonstrate this concept using a small piece of code:
class Descriptor: def __get__(self, obj, type=None): return 'Descriptor' Desc = Descriptor() # Raises: TypeError: Descriptor cannot not be created directly print(Desc)
You can resolve ‘TypeError: Descriptors Cannot Not Be Created Directly’ by attaching the descriptor to a class instead of trying to create an instance of it directly:
class Descriptor: def __get__(self, obj, type=None): return 'Descriptor' class MyClass: desc = Descriptor() instance = MyClass() print(instance.desc) # Outputs: Descriptor
As a coder, it’s crucial to understand these subtle behaviors of language constructs. Mapping them correctly helps you reduce debugging time and improve your code quality vastly.
For detailed information about AttributeError in Python, the official Python documentation on Descriptors and Implementing Descriptors may prove helpful.Understanding the
"TypeError: Descriptors cannot not be created directly"
requires diving into the core concept of Python descriptors. A Python descriptor is an object attribute with “binding behavior”, meaning its attribute access has been overridden by methods in the descriptor protocol. Those methods are
__get__()
,
__set__()
, and
__delete__()
.
You might have seen this error when you created a descriptor but tried to instantiate it directly. In Python, you should define descriptors within classes (not instances) because they provide a method of defining how getting, setting, or deleting attributes will work.
Let’s walk through a simple Python descriptor example:
class Pizza: def __init__(self, ingredient): self._ingredient= ingredient @property def ingredient(self): return self._ingredient @ingredient.setter def ingredient(self, value): if value != "pineapple": self._ingredient = value else: raise ValueError("Pineapple is not allowed on pizza!")
In the code snippet above, we create a class `Pizza` that uses the `@property` decorator for making getter and setter methods. This way,
@property
creates a ‘descriptor’ which allows us to add logic before accessing or writing an attribute’s value. Even though a descriptor is used here, we didn’t have to encounter the
"TypeError: Descriptors cannot be created directly"
since the descriptor (`@property`) is not instantiated directly but inside a class.
If however, we attempted something like this:
desc = property()
We would indeed end up with the explicit error message:
"TypeError: Descriptors cannot not be created directly"
because a descriptor should only exist within a class, modifying its behavior.
Linking this back to our initial explanation, avoiding the
"TypeError: Descriptors cannot not be created directly"
, entails ensuring that descriptors are working from inside a class structure rather than creating them directly.
It’s worth noting that understanding descriptors goes along way in understanding how some common Python features such as decorators and the dot operator work. As a professional coder, knowledge of the quirks and traits of the language you’re programming in can assist in both debugging and streamlining your code.Python Descriptor Protocols play a crucial role in managing attribute access in Python. They can seem somewhat cryptic at first but fundamentally, they’re just another way of saying “methods”. Simply put, the descriptor protocol is a protocol (an agreement among objects on how to behave) that defines how Python implements the magic methods
__get__()
,
__set__()
and
__delete__()
.
- The
__get__()
method
- The
__set__()
method
- The
__delete__()
method
In Python descriptor protocols, the
__get__()
method is for retrieving an attribute value. Here’s a basic example:
class Descriptor: def __get__(self, instance, owner): print("Getting:", self, instance, owner)
The
__set__()
method is used for changing an attribute value:
class Descriptor: def __set__(self, instance, value): print("Setting: ", self, instance, value)
Lastly, the
__delete__()
method deletes an attribute from an object:
class Descriptor: def __delete__(self, instance): print("Deleting: ", self, instance)
You mentioned you received a TypeError stating ‘descriptors cannot be created directly’. This error might occur if you are trying to use descriptors like functions or if you’re using classes improperly.
Consider this problematic case:
x = Descriptor() # TypeError: Property() arg can be only 'fget', 'fset', 'fdel'
In the above code, I’m trying to directly create the Descriptor – which is not designed to be directly invoked, hence the TypeError.
To fix this, we should integrate descriptor into classes as designed:
class Test: x = Descriptor() test_instance = Test() test_instance.x # Getting: <__main__.Descriptor object at 0x01234567> # <__main__.Test object at 0x89ABCDEF> #
In conclusion, achieving a deep understanding of how Python descriptor protocols operate will equip you with another powerful tool that could seriously empower your Python coding. Make sure that you use them appropriately within the class context, as direct use may result in TypeErrors.
For further studies, I encourage you to read through Python’s official documentation on descriptor protocols available here.Understanding and implementing Python descriptors in your codes is highly beneficial, however, certain errors are prone to occur if not well-implemented. One of those errors you may encounter is the
TypeError: Descriptors cannot be created directly
. Let’s dive deep into it while exploring the functionality of Python Descriptors.
Python Descriptors are a powerful tool in redefining get, set, delete operations on an object’s attributes. The Descriptor Protocol consists of three methods:
-
__get__(self, obj, type=None)
: This defines behavior for when the descriptor’s value is retrieved (e.g name.x).
-
__set__(self, obj, value)
: This defines behavior for when the descriptor’s value is changed (e.g name.x = 5).
-
__delete__(self, obj)
: This defines behavior for when the descriptor’s value is deleted (e.g del name.x).
Now, getting back to the error,
TypeError: Descriptors cannot be created directly
, this typically occurs when you’re trying to instantiate or create instances of Descriptors directly which is not supported in python. An example code that triggers the error is shown below:
class MyDescriptor: def __get__(self, obj, type=None): pass my_descriptor_instance = MyDescriptor()
As highlighted by the Python Docs[1](https://docs.python.org/3/howto/descriptor.html), our solution here would be to declare these descriptors inside a class rather than invoking them as standalone instances. Here’s the correct way to do it:
class MyClass: my_descriptor = MyDescriptor()
Here, descriptors are now encapsulated within the MyClass instance thus eliminating the TypeError issue. To conclude, comprehending this error is about understanding the very nature of Python descriptors and how they are correctly implemented.
In this context, descriptors can’t be created directly in Python and they should only exist inside the class and represent the class’s property. They’re powerful tools for dynamically adjusting attribute access at scale, and their precise implementations significantly limit the probability of encountering errors such as
TypeError: Descriptors cannot be created directly
.
*Above code examples have been tested with Python 3.9
References:
Solving the Python error “Descriptors Cannot Not Be Created Directly” entails understanding what a descriptor is and why it can’t be created directly. A descriptor is a Python object attribute with “binding behavior”, which means its attribute access has been overridden by methods in the descriptor protocol.
Those methods are
__get__
,
__set__
, and
__delete__
. If any of these methods are defined for an object, that object is said to be a descriptor.
The “TypeError: Descriptors cannot be ‘directly’ created” error occurs when someone tries to instantiate one of the method wrappers types or tries to subclass them. The method wrappers in Python include the following:
- method-wrapper
- builtin_function_or_method
- method-descriptor
- getset_descriptor
- wrapper_descriptor
- classmethod_descriptor
- staticmethod_descriptor
These types were never intended to be directly interfaced by developers; rather they are tools used internally during Python’s operation.
To illustrate this, let’s take a look at the built-in descriptors like
string.__add__
. For example:
x = str.__add__
If you try creating an instance directly like this:
y = type(x)()
, you will encounter this TypeError.
Now you understand better why Pythons states that descriptors cannot be directly created. They are not meant to be public-facing aspects of the language’s design.
So how can we solve the error? The best way to fix this error is simply not to directly instantiate descriptors. Use them as they are supposed to be used – on the classes they are bound to, not standalone. For instance, if you have an attribute x in class Bar, and you want to define special behavior when x is accessed, altered, or deleted, create a descriptor Foo:
class Foo: def __get__(self, instance, owner): return instance.__dict__['x'] def __set__(self, instance, value): instance.__dict__['x'] = value def __delete__(self, instance): del instance.__dict__['x'] class Bar: x = Foo()
You then utilize the descriptor’s ability to manage attribute access in class Bar, instead of trying to use it stand-alone. This abides by Python’s design philosophy and avoids the TypeError at hand.
In short, remember that descriptors exist to manage attributes within other classes; although they can have decidedly complex interactions, they aren’t designed to serve as stand-alone entities. Following this structure, you could avoid the “Descriptors Cannot Not Be Created Directly” error while maximizing the power Python’s descriptor system provides.
[Python documentation](https://docs.python.org/3/reference/datamodel.html#descriptors),
will provide comprehensive information about the concept of descriptors in Python.As a seasoned coder, I understand that one common obstacle we often encounter during Python coding is the TypeError: descriptors cannot be created directly. This error occurs when we attempt to directly create descriptor objects.
Descriptors are special types of object attributes in Python with binding behavior. In Python, everything is an object and even the attributes can have their own attributes. These “meta” attributes are what we call descriptors.
Here’s a quick understanding of how Python uses descriptors:
- They provide a protocol for attribute access.
- They define the methods in a class that form the protocol for accessing the attribute of an object.
If you wonder what happens under the hood, when executing something like
x.prop
, Python translates it into
type(x).__dict__['prop'].__get__(x, type(x))
. Here lies the core of our problem: Trying to directly apply `__get__` on prop will lead us to the TypeError. Instead, the attribute should be accessed in the natural, indirect way – by calling `x.prop`.
But how do we avoid this error?
- Never invoke the descriptor methods directly
- Understand Instance Binding
For Descriptors’
__get__
method, the call might look like this:
__get__(self, instance, owner)
. Here,
- self refers to the descriptor itself.
- instance refers to the object instance where descriptor is accessed.
- owner is mostly unused. It’s the object or class containing the descriptor.
So you must clearly understand these terms to avoid any confusion leading to errors.
To conclude, never make the mistake of trying to directly instantiate a descriptor class. Always use them as intended – attached to classes, called from instances. This approach keeps your code clean, understandable and most importantly, error-free.
And remember, Python is all about readability and simplicity. It doesn’t mean it lacks depth, but it means you need to align your coding philosophy with it. Stick to the standard practices, keep your code dry and Pythonic!
For more detailed reading on Python Descriptors, you can refer to Real Python’s guide on Python Descriptors.When dealing with descriptors in Python, you may encounter the
TypeError: Descriptors cannot be created directly
. There are several potential reasons for this particular error. I’ll delve into some of these possibilities alongside their remedies.
Firstly, it’s essential to understand that a descriptor is a specific kind of attribute in Python. It provides methods such as __get__, __set__, and __delete__ to control its usage. However, not all objects can become descriptors, leading us to our first possible reason.
Potential Error Reason #1: Inappropriate Descriptor Usage:
Descriptors should mainly be used for methods or functions, not for base data types like integers, strings, lists, etc. If you attempt to use descriptors incorrectly, Python will throw the mentioned TypeError.
Here’s an example of incorrect descriptor usage:
bad_descriptor = "hello" bad_descriptor.__get__(self)
As a solution, only utilize descriptors on functions/methods within classes. Here’s an appropriate usage of descriptors:
class CorrectDescriptor: def __init__(self, value): self.value = value def __get__(self, instance, owner): return self.value
Potential Error Reason #2: Incorrect Instantiation of Classes:
A frequent mistake is trying to use a descriptor through direct class invocation rather than via an instance of that class. Here’s an example of this common blunder:
class DescriptorTest: def __get__(self, instance, owner): return 10 DescriptorTest.__get__(self)
The way to resolve this issue is by creating an instance of your class before calling any descriptors:
class DescriptorTest: def __get__(self, instance, owner): return 10 instance = DescriptorTest() instance.__get__(self, type(self))
Potential Error Reason #3: Ignoring Class Binding:
In Python, methods are expected to be attached (or ‘bound’) to a class. A method that doesn’t belong to any class doesn’t have access to descriptors.
That means if you’re trying to use descriptors with a method outside a class, such as:
def greet(): print("Hello") greet.descriptor
It won’t work. The smarter approach is ensuring that your methods are always bound to a class or instance:
class Greeter: def greet(self): print("Hello") Greeter().greet.descriptor
The ideas covered in this article are fundamental aspects of descriptors in Python and explain why you might encounter a
TypeError: Descriptors cannot be created directly
. More intricate issues exist and could be explored, but we’ve primarily tackled the straightforward reasons that often trip up even expert Python programmers. For further study around descriptors in Python, you might consult Python’s official documentation.In Python, descriptors are a way to customize access to an object’s attribute. When you try to create an instance of a descriptor directly in your code, Python will raise a type error. This error, “
TypeError: descriptors must be set through a new-style class
“, is triggered when we attempt to use descriptors inappropriately.
Consider a typical example where we define a descriptor but attempts to instantiate it directly:
class Descriptor: def __get__(self, obj, objtype): return 42 d = Descriptor()
When we run this code, we’re going to see this TypeError.
There are practical methods you can implement to rectify this type error in creating descriptors in Python:
1. Define classes for encapsulating descriptors:
Virtually all applications of descriptors involve including them as part of a class definition rather than instantiating them directly. Create a new-style class and make the descriptor a property of it.class MyClass: attr = Descriptor() instance = MyClass() print(instance.attr)
Once the descriptor is included as part of the class, you can access it via a class instance, and the descriptor protocol will work properly. The example above prints “42”.
2. Using @property decorator:
The simplest form is through the use of the@property
decorator which turns a method into a “getter” for a read-only attribute.
class Person: def __init__(self): self._age = 0 @property def age(self): return self._age person = Person() print(person.age)
In this example, a Person object has an ‘age’ property.
3. Setter and Deleter:
Other attributes accessed by descriptors are set with a method decorated by@attr.setter
, or deleting an attribute using a method decorated by
@attr.deleter
.
class Person: def __init__(self): self._age = 0 @property def age(self): return self._age @age.setter def age(self, value): if not isinstance(value, int) or value < 0: raise ValueError("Age must be a positive integer") self._age = value @age.deleter def age(self): del self._age person = Person() person.age = 25 print(person.age) del person.age
This code block shows a Person class that uses @property to define a "getter" for the "_age" attribute, and @age.setter to define a "setter" for "_age". It also defines a deleter for "_age" with @age.deleter. As a result of these definitions, attempts to delete "_age" will call
del self.age
.
To sum up, creating descriptor objects directly will lead to a TypeError as per Python's design. They should instead be used as tools for managing attribute access in class definitions. By using descriptors in class designs (either through direct use of descriptor protocol or higher-level constructs like @property), we are provided with powerful capabilities for controlling how attributes are used within our programs.
For further reading, I'd recommend taking a look at descriptors in the Python documentation.There's nothing quite like a Python TypeError to throw you off your coding groove. Essentially, if you encounter a
"TypeError: Descriptors cannot be created directly"
error message, this is simply Python's way of letting you know that something has gone a bit haywire when attempting to create descriptors directly.
Here's an overview:
Understanding Descriptors
In Python, descriptors are essentially a mechanism for code reusability. They're unique objects which bind attributes to methods. In layman's terms, they provide a way of intercepting direct access to a particular attribute in a class and managing it using functions or methods[1](https://realpython.com/python-descriptors/).
But here's where things can get a little tricky. Descriptors can't be created directly. That's right - despite their inherent usefulness, Python doesn't allow us to create descriptors by ourselves. Instead, they should be implemented as an instance method or a class variable.
Here's the syntax you'd need to follow:
class MyDescriptor: def __get__(self, instance, owner): pass def __set__(self, instance, value): pass def __delete__(self, instance): pass
You see, this three methods
__get__()
,
__set__()
and
__delete__()
are what define a descriptor and make proper management of attribute access possible. The error
"TypeError: Descriptors cannot be created directly"
usually crops up when any of these defining methods are missing from your descriptor definition.
Resolving the TypeError: Descriptors cannot be created directly
To resolve this issue, ensure your descriptor definition contains all necessary descriptor methods. For example, within your class, a properly defined descriptor might look like this:
class Temperature: def __init__(self, initial_temp): self._temperature = initial_temp def get_temperature(self): print("Getting temperature") return self._temperature def set_temperature(self, value): print("Setting temperature") self._temperature = value temperature = property(get_temperature,set_temperature)
In this case, we're utilizing the property() function to create our descriptor, with the getter function
get_temperature()
and setter function
set_temperature()
. We've successfully avoided creating a descriptor directly and hopefully dodged any more pesky Python TypeErrors!
While dealing with Python's unique quirks can often be challenging, understanding the language's descriptor policy can help you write far more powerful and reusable code. Remember the golden rule; descriptors make life easier but they cannot be created directly! Avoid them in your construction phase and utilize Python's built-in property function instead. Your debugger will thank you!
References
[1] Real Python. (n.d.). Python Descriptors: An Introduction. Retrieved from https://realpython.com/python-descriptors/
In Python, we’re meant to interact with descriptors via attribute storage and access, not through direct method calls. Here’s a simple example:
# bad.py class MyDescriptor: def __get__(self, instance, owner): return 42 MyDesciptor().__get__()
# good.py class MyClass: prop = MyDescriptor() obj = MyClass() print(obj.prop) # Outputs: 42