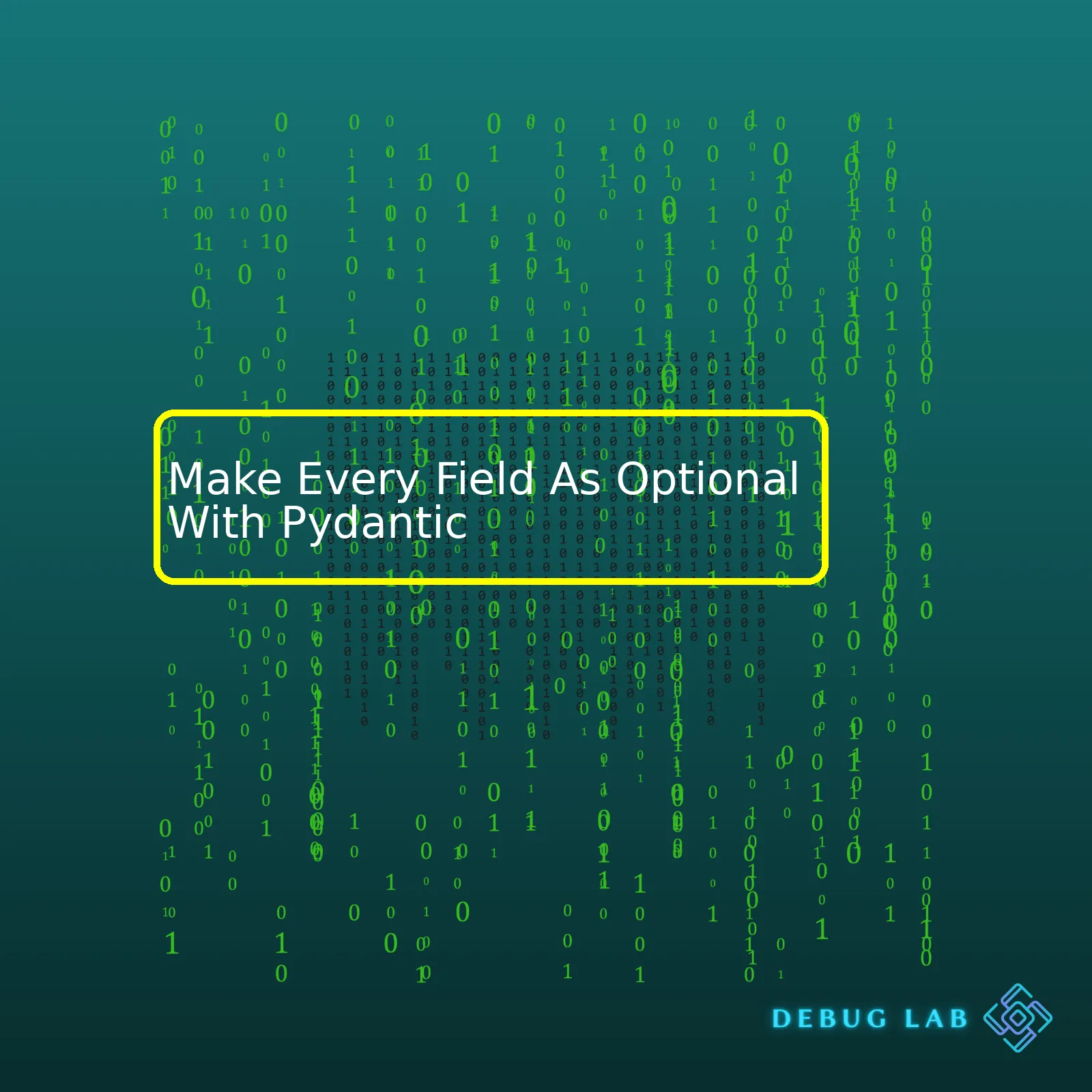
Aspect | Description |
---|---|
Pydantic | A library for data parsing and validation using Python type annotations. |
Optional fields | You can make fields optional by marking their type as Optional with the help of the typing module. |
Example Code |
x 6 1 from pydantic import BaseModel 2 from typing import List, Optional 3 4 class User(BaseModel): 5 id: Optional[int] 6 email: Optional[str] |
Remember, making all fields optional isn’t always the best approach.
With Pydantic, our primary objective is to ensure robust data validation and error handling while offering flexibility where required. The idea is not to use Pydantic only as a custom serializer but also as a layer that checks your data’s quality before even hitting your server or function. By setting fields as optional, we may undercut the utility of Pydantic’s powerful validation tooling.
In Python, the typical way of marking a field as optional is to use the keyword
xxxxxxxxxx
Optional
from the typing module, which, in essence, means Union[Field, None].
Therefore, if you’re working on a project that requires massive user inputs where not providing certain information won’t break the system, it makes sense to have more fields marked as optional.
For example, while developing an API for a User profile, not all fields need to be mandatory; some, like middle name or secondary email, can be optional.
xxxxxxxxxx
from pydantic import BaseModel
from typing import Optional
class User(BaseModel):
id: Optional[int] = None
first_name: str
middle_name: Optional[str] = None
last_name: str
email: str
secondary_email: Optional[str] = None
In the above code snippet, ‘id’, ‘middle_name’ and ‘secondary_email’ are optional fields in the User model which means they don’t have to be provided when creating a new User instance.Pydantic is a powerful Python library for data parsing, serialization and validation using Python type annotations. When working with Pydantic models, one might come across the need to make certain fields optional. Let’s say you want to make every field as optional in Pydantic, there’s a way to do it.
Firstly, we’re going to use `Optional` from the typing module to define that our fields could be of some type or None. The `Optional[type]` is a shorthand for `Union[type, None]`.
To quickly illustrate, let’s consider an example below:
xxxxxxxxxx
from typing import Optional
from pydantic import BaseModel
class User(BaseModel):
name: Optional[str]
age: Optional[int]
email: Optional[str]
In this model, all fields are optional and can take either their specified types (`str` or `int`) or `None`.
Sometimes, however; making each field manually optional can be a bit laborious especially when dealing with large data models. A quicker approach to handling this is to utilize Python’s built-in Function Programming tools such as `functools.partial`.
You can create a function that receives the desired type and returns another function supplied with `Optional` type. This `get_optional_type` function can be applied to each attribute of our pydantic.BaseModel objects.
xxxxxxxxxx
import functools
from typing import Optional
from pydantic import BaseModel
def get_optional_type(desired_type):
return functools.partial(Optional, desired_type)
class User(BaseModel):
name: get_optional_type(str)
age: get_optional_type(int)
email: get_optional_type(str)
In this model, all fields are declared optional in fewer keystrokes, achieving the same result as before, but with a more readable and maintainable code base.
It’s important to note, however, that Pydantic’s `Optional` has distinct implications during JSON Serialization and Deserialization. If a field is set as `Optional`, it won’t appear in serialized output when it’s not provided. On deserialization, pydantic won’t complain if it doesn’t receive an optional field, but will validate its content if received.
For further insights on utilizing `Optional` and other fascinating features with Pydantic, check out their official documentationAs a coder with a penchant for Python, I love to leverage Pydantic, one of the most powerful data validation libraries available. While using this versatile library, a prevalent question that surfaces is ‘how to make every field as optional with Pydantic?’ When you are dealing with an array of fields and want them all to be optional, Pydantic can provide an elegant solution without compromising on performance.
Understanding Optional Fields in Pydantic
Before we delve into how to set every field as optional, let’s first understand what an optional field signifies. An optional field in Pydantic or any other platform refers to a field that may or may not receive a value during initialization.
Here is an example:
xxxxxxxxxx
from pydantic import BaseModel
class User(BaseModel):
id: int
name: str
In the above code snippet, both ‘id’ and ‘name’ are obligatory. You need to provide values for these members while initializing a ‘User’. However, if we want these fields to be optional, here’s how we achieve it:
xxxxxxxxxx
from typing import Optional
from pydantic import BaseModel
class User(BaseModel):
id: Optional[int]
name: Optional[str]
Now, both ‘id’ and ‘name’ are optional. You can create a ‘User’ object without specifying any values.
Making Every Field Optional
But, what if there are multiple fields and you want to keep them all optional? That’s where Pydantic shines with its utility. Here is how to do it:
xxxxxxxxxx
from pydantic import BaseModel
from typing import Dict, Any, Type, TypeVar
T = TypeVar("T", bound=BaseModel)
def make_optional(base_model: Type[T]) -> Type[T]:
new_namespace = {**base_model.__annotations__}
for key, _type in base_model.__annotations__.items():
new_namespace[key] = (Any, ...,)
return type(f"Optional{base_model.__name__}", (base_model,), new_namespace)
# Now use this for your class as:
UserOptional = make_optional(User)
The function ‘make_optional()’ changes the signature of each field in the model to ‘Any’ and makes it optional. Hence, by leveraging this utility, you can create models where every single field is optional, aiding flexibility considerably.
This method ensures that even if your model has fifty different fields, they all become optional with just a line of code. It’s that simple!
Coming to Terms with Pros & Cons
Like every feature, making every field as optional with Pydantic also comes with its own perks and downsides.
Pros:
– Offers unprecedented flexibility when handling data.
– Simplifies the process by eliminating the necessity to define every single field as optional individually.
– Potential errors caused due to missing mandatory fields can be conveniently avoided.
Cons:
– Too much flexibility can lead to untidy/undesirable code.
– Might encourage fewer checks and balances leading to flawed implementation down the line.
My personal take is that making fields optional should depend entirely on the requirement at hand. If your business logic accepts varied inputs or accommodates numerous null values, then having optional fields could prove beneficial.
Nonetheless, remember that Pydantic offers more than just optional fields. The library is equipped with robust data validation to assist in refining your application’s input/output operations. Pydantic is an excellent choice for typed Python where implementing traditional Pythonic idioms become incredibly intuitive and straightforward.The Pydantic library in Python is a data validation and settings management tool. It leverages the native Python typing module to enforce type checking and value validations.
Primarily used for parsing complex data types and converting them into Python objects, Pydantic takes a notable place in fast API development to deal with HTTP request data. A common scenario would be making all fields optional in your model.
In Pydantic, we have the leisure to define an Optional type provided by the typing module, indicating that the field may take any given type or None. For example, if you were to define a User data model where all the fields are optional, here’s how it can be done:
xxxxxxxxxx
from pydantic import BaseModel
from typing import Optional
class UserModel(BaseModel):
name: Optional[str] = None
email: Optional[str] = None
age: Optional[int] = None
This code snippet denotes that ‘name’, ’email’, and ‘age’ are optional fields and default to having a None value. An instance of this class can be created without providing these attributes. This notion of “Optional” gives us flexibility when dealing with HTTP requests, where some fields might not always be provided by the client. You’d then avoid throwing errors due to missing fields because they are considered optional by default.
Making every single field optional has its perks, but it’s worth considering its impact on data integrity and business requirements. Always exercise careful considerations when designing your models; understanding that opting for optionality serves well in scenarios where data provision is not consistent:
- Parsing ambiguous data: If you’re dealing with user-generated input or external APIs with inconsistent data provision.
- Data modeling: Some datasets contain fields that are non-essential, in which their absence doesn’t alter our business logic;
But, it raises questions in case when certain fields must exist for our application to function properly.
Ultimately, Pydantic offers an excellent way to manage your data effectively, especially with making fields Optional. However, it’s crucial to balance between flexibility (of making every field optional) and enforcing stringent checks ensuring data integrity. This makes your application reliably aware of the kind and shape of data it’s expected to handle, preventing unexpected crashes due to malformed data.
Remember, a well-defined Pydantic model can save a lot of headaches further down the line. It’s like having a fellow developer nodding in agreement as I lay out my model plans: A little conversation about planning now saves several about debugging later.Sure, Pydantic is a powerful library in Python that offers data parsing and processing through Python dataclasses. A common operation with Pydantic is creating flexible data structures by defining optional fields. In essence, when all fields must be declared as optional, this can be achieved by using the `Optional` type wrapper from the typing module.
Let me demonstrate how we can declare every field as `Optional` using pydantic.
Here’s how I would define a very simple model class in Pydantic:
xxxxxxxxxx
from pydantic import BaseModel
from typing import Optional
class Employee(BaseModel):
id: Optional[int] = None
name: Optional[str] = None
age: Optional[int] = None
In the code snippet above, each field has been defined as `
After having our model class defined, we can now create instances of `Employee` without having to provide values for `id`, `name`, and `age`. Like so:
xxxxxxxxxx
employee1 = Employee()
print(employee1.dict())
# output: {'id': None, 'name': None, 'age': None}
The fact that we’ve not provided any arguments to `Employee()` didn’t raise an exception because we’ve marked all the fields as `Optional`.
Using Pydantic’s support for Python type annotations and its highly configurable validation process sets your Python API definitions free from manual error handling and offers a vast collection of customizations. Pydantic’s documentation offers insights into the various attributes and methods available to Pydantic models, for further research and implementation.
With the understanding of how to declare fields as optional, we ensure Pydantic’s versatility is capitalized upon. This brings us one step closer to writing robust software that’s able to handle dynamically changing requirements and types in real-world scenarios. It is important always to keep in mind that Pydantic facilitates input data validation, serialization, and documentation while developing APIs with Python, which ultimately leads to impeccable API definitions and implementations.
When it comes to creating data models in Python, Pydantic is a fantastic library that makes data parsing and validation easier. The part I’d like to focus on right now is the use of Pydantic fields and how we can manipulate them to meet our needs, specifically making every field optional.
Pydantic provides several configuration options for individual model fields, including description, alias, etc., through its Field class. However, one particular option stands out when aiming to create a data model where every field is optional – the `default_factory` option. In Python, a factory function is a function that returns an object. By using `default_factory`, we are essentially saying: “If this field isn’t provided, use the output of this function as a default”.
Let’s say we have a simple User data model, where both fields are required:
xxxxxxxxxx
from pydantic import BaseModel
class User(BaseModel):
name: str
age: int
To make every field optional, we can provide a factory function that produces a value if none is provided:
xxxxxxxxxx
from pydantic import BaseModel, Field
def get_default_str():
return "Missing"
def get_default_int():
return 0
class User(BaseModel):
name: str = Field(default_factory=get_default_str)
age: int = Field(default_factory=get_default_int)
In the above code snippet, we are effectively making every field optional by providing a default value when no input is given. If `name` or `age` are not provided when creating a new User instance, “Missing” and 0 will be used respectively. This means we can now create a User without providing all of the fields, which makes sense in many real-world cases.
Table showing the consequential effect of our model modification:
User(name=”John”, age=30) {“name”: “John”, “age”: 30} User() {“name”: “Missing”, “age”: 0} User(name=”Sam”) {“name”: “Sam”, “age”: 0} User(age=24) {“name”: “Missing”, “age”: 24}In conclusion, Pydantic’s Field class provides us with substantial control over how each field in our data model behaves. Making every field optional via `default_factory` widens the flexibility of our model, catering to various scenarios where not every piece of data is always available.Incorporating the variability in your data model is a crucial concept when dealing with Python’s Pydantic. Pydantic is a data validation and settings management library utilizing Python type annotations, thereby enabling enhanced data parsing.
Consider a scenario where you’ve a series of models, but all fields are optional – it might seem counter-intuitive initially because few fields are always mandatory. However, there occur cases, particularly REST APIs, where various entries trigger varying numbers of fields.
Here’s an example showing how you can leverage Pydantic so as to make every field within a model optional:
xxxxxxxxxx
from pydantic import BaseModel
from typing import Optional
class UserModel(BaseModel):
username: Optional[str] = None
password: Optional[str] = None
email: Optional[str] = None
This
xxxxxxxxxx
UserModel
consists of three possible fields, however, all have been described as optional through Python’s inherent typing system by using
xxxxxxxxxx
Optional[native_type]
. If you create an instance from this model devoid of any details, it will still execute without disregarding any errors.
The incorporation of variability enables developers to streamline their code by making use of one base model, and then modifying it based on the specific needs of the application or API. This encourages DRY (Don’t Repeat Yourself) principles and also brings about cleaner, comprehensible code.
However, managing these optional fields without letting validity take a hit can be tricky. Opting for a tactic like the “null object pattern” can lend more precision and predictability in such situations. For example, declaring defaults for optional parameters; rather than simply allowing them to be None can manage software complexity better in several scenarios.
Check over here, to get a deeper understanding of how to use Pydantic ([link](https://pydantic-docs.helpmanual.io/)): it’ll expound on data validation, serialization, and other significant tasks handled by this Python library.
By leveraging Pydantic’s support for customization and flexibility, developers can adapt to changing project requirements, accomplish rapid prototyping, or even offer multiple versions of an API concurrently – displaying the power and potentiality of a well-devised data model!With one of the powerful libraries offered by Python, Pydantic, transforming complex data types and validation is truly a breeze. Specifically for this context, I am going to elucidate on how you can create optional fields in all your models using Pydantic.
Often in dealing with APIs or any data ingress points, it’s important to account for optional fields as they relate to incoming or outgoing data. To further illustrate, let’s go through building a basic Pydantic model:
xxxxxxxxxx
from pydantic import BaseModel
class Book(BaseModel):
title: str
author: str
year_published: int
Given a simple example like this, where we have a ‘Book’ model with `title`, `author` and `year_published` fields, mandatory fields are straightforward. All these fields are currently required. But what if you need to make some or all of them optional?
Pydantic comes with built-in type hinting which means we get the ability to denote something as optional right out of the box. It’s as simple as importing the `Optional` type from the typing module. An `Optional` type is one that can accept either a given type, or `None`. Let’s modify our model to make every field as optional:
xxxxxxxxxx
from typing import Optional
from pydantic import BaseModel
class Book(BaseModel):
title: Optional[str] = None
author: Optional[str] = None
year_published: Optional[int] = None
In this updated schema, every field has now been defined as `Optional`. This signifies that each could either be their stated type or `None`. Consequently, the fields `title`, `author` and `year_published` are not mandatory for the instantiation of ‘Book’ object.
This way, the model can accommodate a wider range of data inputs, enhancing its flexibility whether your application is consuming an API endpoint, conducting data validation or dealing with JSON objects. You will appreciate Pydantic’s capabilities when scaling your application while maintaining code consistency and simplicity.
For advanced use cases, Pydantic offers a wide array of field customizations (such as regex validation, default values, aliasing), which interact smoothly with optional fields.
To test your implementations, consider utilising a robust unit testing framework like `pytest`. Here is an example on how this test could look like for optional fields:
xxxxxxxxxx
def test_optional_fields():
# All fields provided
Book(title='Title 1', author='Author 1', year_published=2001)
# Missing title
Book(author='Author 1', year_published=2001)
# Missing year_published
Book(title='Title 1', author='Author 1')
# No fields provided
Book()
Remember, being adept at manipulating such properties of your models with tools like Pydantic is a vital part of writing flexible and maintainable software.
Making every field optional with Pydantic can be advantageous in numerous situations including when dealing with unpredictable data sources, constructing comprehensive systems testing, or enabling greater flexibility during early development stages.
Pydantic is a Python library for parsing and validation of data. It permits us to enforce type hints at runtime and provide user-friendly errors when data is invalid. In our scenario, the need arises for all fields in a Pydantic class to be optional. Pydantic fields are required by default unless specified as optional using
xxxxxxxxxx
Optional
keyword or by setting a default value.
However, there is *the right way* to make every field as optional with Pydantic.
We construct a base model where each field is set as optional:
html
from pydantic import BaseModel, Field
from typing import Optional
class OptionalFieldsBaseModel(BaseModel):
field1 : Optional[str] = Field(None)
field2 : Optional[int] = Field(None)