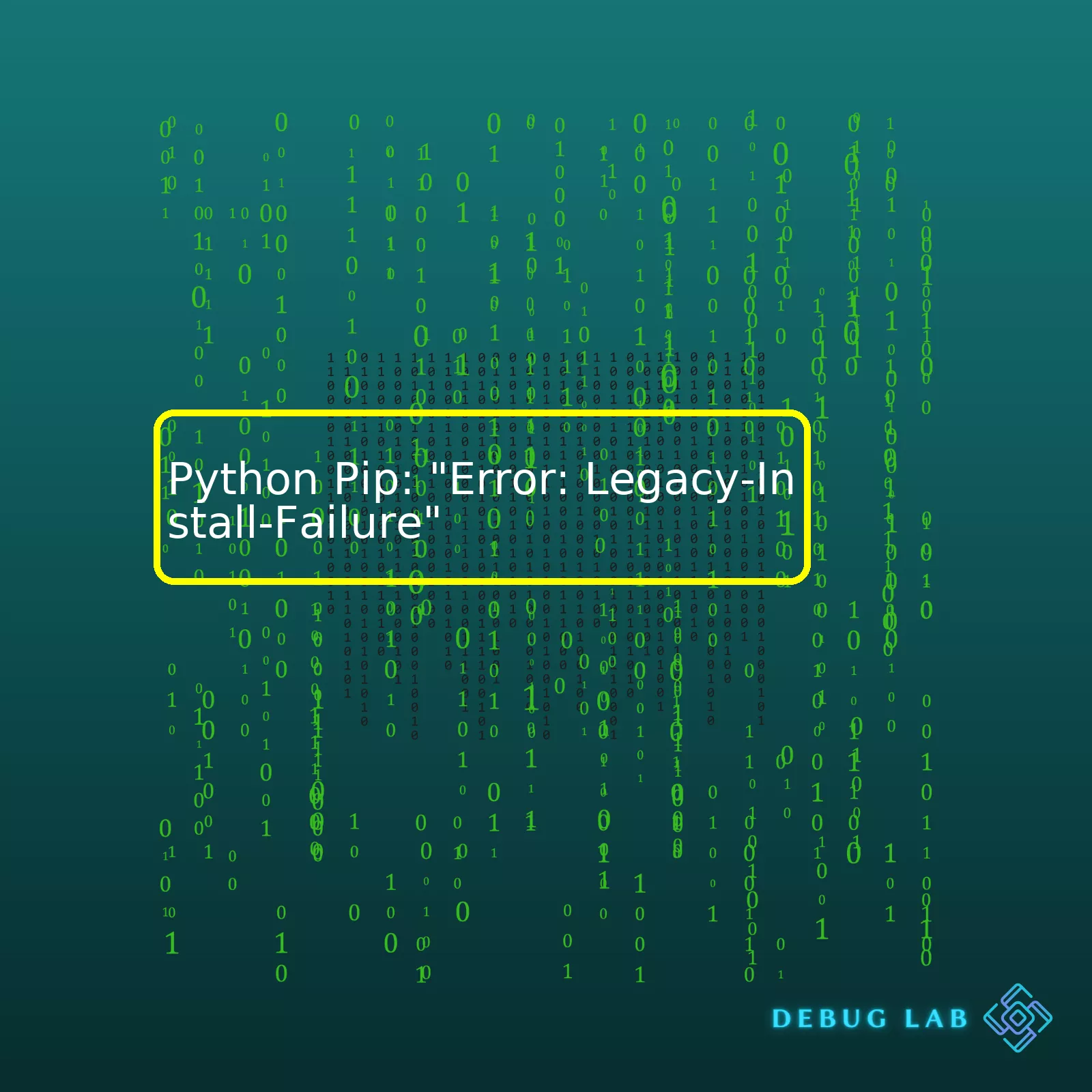
Here’s a brief summary table about “Error: Legacy-Install-Failure”:
Error Name | Caused By | Possible Solution |
---|---|---|
x 1 1 Legacy-Install-Failure |
Attempting to install packages with stringent build dependencies | Upgrade PIP or use
xxxxxxxxxx 1 1 1 --use-feature=2020-resolver |
To understand comprehensively the issues associated with the “Legacy-Install-Failure” error, we can read it in three segments:
• The term “legacy” refers to the old pip dependency resolver;
• The “install” pertains to the process of downloading and installing a Python package to your environment using pip;
• Lastly, “failure” signifies that this process has not completed successfully.
Therefore, the essence of the “Legacy-Install-Failure” error is a failure in the process of installing a Python package using older pip versions containing the outdated dependency resolver.
A common and effective solution to bypass the “Legacy-Install-Failure” error would be to upgrade pip. Updating pip to its latest version allows you to use the new, modern dependency resolver instead of the legacy one. Here’s the command to upgrade pip:
xxxxxxxxxx
python -m pip install --upgrade pip
Another solution would involve leveraging Python’s ‘future feature’ option to benefit from upcoming features before they’re released in the stable version. In our context, this strategy may help circumvent some pip limitation that causes the legacy install failure. Implementing this resolution involves activating the future feature for pip 20.3’s dependency resolver through the command:
xxxxxxxxxx
pip install --use-feature=2020-resolver package-name
Remember to replace ‘package-name’ with your target Python library’s required name and ensure you run your terminal or command prompt as an administrator (on Windows) or utilize sudo on UNIX systems.
Should these methods prove unsatisfactory, consulting the pip official documentation(source) or seeking community support should provide further insightful guidance.
Python Package Index, more commonly known as Python PIP, is a package management system used to install and manage software packages written in Python. It’s a command-line utility that allows programmers to conveniently download, install, update, and remove Python libraries in their projects. Sounds great, right? Absolutely, until you come across a very common error called the “Legacy-Install-Failure”.
Understanding Legacy-Install-Failure Error in Python Pip
The legacy-install-failure error often appears when pip does not have enough permissions to install a package into the site-packages directory or when there’s an issue with the setup.py file in the package that is being installed.
xxxxxxxxxx
$ python -m pip install your-package
ERROR: Legacy-install-failure.
...
Command errored out with exit status 1: ...
This perplexing error message halts the package installation process and could be quite daunting for beginners not familiar with it. However, don’t feel discouraged because we’ve got a couple of workarounds!
Handling Legacy-Install-Failure
Here are the detailed analytical steps to resolve this error:
- Updating PIP: Sometimes, the problem arises due to an outdated version of Python Pip. Therefore, before attempting any other troubleshooting methodologies, make sure that Pip is updated by using:
xxxxxxxxxx
111$ python -m pip install --upgrade pip
This command updates pip if it’s outdated and potentially resolves the issue. After updating pip, retry installing the desired package.
- Checking Permissions: As mentioned earlier, another potential cause for this error is lack of proper permissions. One way of addressing this is by appending the ‘–user’ parameter to your command like so:
xxxxxxxxxx
111$ python -m pip install your-package --user
This command instructs pip to install packages in the user installation directory instead of the default global site-packages directory.
- Validating Setup.py: The error might be due to problematic scripts in setup.py. Review the setup.py file and ensure consistency according to the best practices defined in Python’s official documentation.
Moving forward, always keep your pip updated, verify the site you’re downloading packages from, and go through the readme.md or documentation associated with your desired packages. This will help you avoid running into problems like these.
Troubleshooting is part of every coder’s life. And remember, even though “Legacy-Install-Failure” sounds serious, it’s usually just another bump on the fascinating road to mastering Python. Happy coding!
Let’s delve deep into the Python Pip and the issue of the “Error: Legacy-Install-Failure”.
The first step would be to understand what this error points towards. The “Error: Legacy Install failure” is usually an indication that some older packages or dependencies in your Python environment are not compatible with the current ones you’re trying to install or update using pip.
Major Causes
Here are some potential causes that might lead to this kind of error with Python pip:
- There could be a version clash between the new package being installed and the old one already present in the system.
- The newer packages require dependencies that aren’t currently supported by the older packages present in the system.
How do we address it?
There are numerous ways to resolve “Error: Legacy-Install-Failure” issue, let’s have a look at the most effective ones:
- Upgrade pip:
One of the first things one should always ensure is having their pip updated to the latest version. You can use the below command to upgrade:xxxxxxxxxx
111python -m pip install --upgrade pip
- Create and Use Virtual Environment:
You can also consider using a virtual environment. A virtual environment ensures differing requirements from various projects does not conflict, causing errors such as “Error: Legacy-Install-Failure”. We can create a virtual environment by using venv (built-in Python 3.3+):xxxxxxxxxx
121python3 -m venv my_env
2source my_env/bin/activate
- Updating all packages:
Another way is to try and update all the packages to their respective newest versions:xxxxxxxxxx
111pip freeze | cut -d = -f 1 | xargs -n1 pip install -U
%
Static code analysis tools such as PyLint, PyChecker, and Pyflakes can help provide warnings about legacy installations and avoid running into these kinds of errors(source).
Feel free to dive deeper into concrete examples in Python source code, this will surely increase your knowledge on how to further mitigate such errors.
Remember that the solutions above are just guidelines. Depending on the specifics of your case, some trial and error might be necessary to fully resolve the issue. It’s part of the life of a coder, and it’s what keeps our job exciting!
If you’re experiencing the “Error: legacy-install-failure” during the installation of Python packages using
xxxxxxxxxx
pip
, it can be quite frustrating. However, in most cases, this issue is resolvable with a handful of troubleshooting steps and practical tips.
Keeping your Pip Installation Up to Date
One potential reason behind this failure might be an outdated version of pip. The Python community continues to refine and improve pip, which sometimes means that older versions fall out of date and may not function properly. This is why keeping your pip up-to-date is crucial.
You can upgrade pip using the following commands:
On Mac and Linux:
xxxxxxxxxx
python -m pip install --upgrade pip
On Windows:
xxxxxxxxxx
py -m pip install --upgrade pip
Using a Virtual Environment
Sometimes, failures like “Error: legacy-install-failure” occur due to conflicts between system-wide Python packages, and those intended for the project you are currently working on. Setting up a virtual environment can help mitigate these conflicts by isolating your project’s dependencies.
To set-up a new virtual environment, use the venv module from Python:
xxxxxxxxxx
python3 -m venv /path/to/new/virtual/environment
You can then activate this environment:
On Unix or MacOS, run:
xxxxxxxxxx
source /path/to/new/virtual/environment/bin/activate
On Windows, run:
xxxxxxxxxx
\path\to\new\virtual\environment\Scripts\activate
Once the virtual environment is activated, you can try installing your package again using pip.
Checking your Python Installation
Errors during package installation can sometimes be traced back to issues with your Python installation itself. It’s possible that necessary components or extensions were omitted during installation, or perhaps updates have interfered with previously installed parts.
Reinstalling Python may resolve the issue and can be done via the official Python website at
python.org/downloads/
Consulting Package Documentation
Lastly, always consult the documentation or user manual of the specific package you’re trying to install. There might be additional dependencies required, or platform-specific instructions that you need to follow. For example, some packages may need additional software installed that can’t be managed by pip or they may ask for specific versions of pip or python.
Source code checking
Let’s suppose you are getting this error when installing Django which is a popular Python framework. Check to see if the source code is correct. Here’s an example of clean Django installation:
xxxxxxxxxx
python -m pip install Django
Also ensuring that the first character of ‘Django’ is uppercase could solve this error as Python is case-sensitive.
In sum, resolving the “Error: Legacy-Install-Failure” when using pip to install Python packages often involves checking your pip and Python installations, considering the use of a virtual environment, and referring to the relevant package documentation.
Python Pip’s “Error: Legacy-Install-Failure”: A Case Study
Python’s Pip is essential for package management, making it easier to install and manage software packages written in Python. Usually, it performs efficiently without any glitches. However, users might encounter some errors such as “Error: Legacy-Install-Failure”, which can be quite challenging to handle if you don’t know its root cause and the solution.
Unraveling the Error
“Error: Legacy-Install-Failure” in Python Pip is usually a symptom of an older legacy package that tries to create or modify files outside its installation directory. These modifications are considered a breach of pip’s security and reliability model and thus lead to an installation failure. This prevents a harmful step that could potentially destabilize other environments on your computer. The error is most likely to occur when working with historical Python packages due to their violation of modern packaging conventions.
Error Message | Potential Cause | Solution |
---|---|---|
ERROR: Failed cleaning build dir for Package_Name | Incomplete dependencies or Path issues in Python Environment | Ensure that all required dependencies are properly installed and paths are correctly set |
ERROR: Command “Command_Name setup.py egg_info” failed with error code 1 | Problem in running setup.py during package installation | Try installing the package manually by cloning the package repository and then running
xxxxxxxxxx 1 1 1 python setup.py install . Alternatively, consider updating setuptools using xxxxxxxxxx 1 1 1 pip install --upgrade setuptools |
Legacy version found, removing… | Ancient versions of the package interfering with newer versions | Use commands like
xxxxxxxxxx 1 1 1 pip uninstall Package_Name to remove the old version before installing the new one. |
A Detailed Walkthrough of a Fix
The resolution for this problem usually revolves around navigating the intricacies of the Python environment to ensure proper installation of packages and dependencies:
- Firstly, check that your Python environment has been correctly configured, ensuring things like system PATH are correctly pointing to the Python you mean to use and not another installation. This can be done through the command prompt by using
xxxxxxxxxx
111where python
.
- Check for missing dependencies. Some Python packages may rely on other packages or particular versions to function correctly. An incompatible or missing dependency can lead to a string of unrelated-seeming errors. For example, if you’re hitting this issue with a package like ‘nokogiri’ or ‘wrapt’, it’s entirely possible that you’re missing a dependency like ‘libxml2-dev’, ‘libxslt-dev’ or ‘build-essential’ which needs to be satisfied first. StackOverflow – Pip install Nokogiri fails
- Next, try to upgrade the Package installer (Pip) and the Package – setuptools. Use the following commands:
xxxxxxxxxx
111pip install --upgrade pip
xxxxxxxxxx
111pip install --upgrade setuptools
- If everything fails, bypass the pip installation and compose a local manual install from a cloned GitHub Python package repository. This way, you can debug each failing step in the installation process separately. Retrieve the repository link and clone it locally:
xxxxxxxxxx
111git clone Repo_URL
Go inside the directory of the cloned repo:
xxxxxxxxxx
111cd Directory_Name
Then install it using the package’s setup file:
xxxxxxxxxx
111python setup.py install
These recommendations will ensure that you’re able to map out the source of these errors more systematically and find your solutions swiftly, keeping your programming flow uninterrupted.
The “Error: Legacy-Install-Failure” in Python Pip is a common issue encountered by many Python developers. This error occurs when there’s an issue while trying to install a Python package using the pip package manager. Let’s delve into understanding this error, its causes and solutions.
Understanding the Error
The keyword ‘Legacy’ in this error suggests that it’s related to outdated or old software components. Traditionally, the cause of such errors is either that:
– The pip version is out dated
– The setup.py file of the package you’re trying to install is outdated
– There was an unsuccessful installation attempt of the package which has left some traces behind and it hinders new installations
Solutions to the Error
- Updating pip
Firstly, ensure that your pip installation is up-to-date. Outdated versions of pip could induce compatibility issues with certain Python packages which may lead to the occurrence of our problem at hand. To update pip:xxxxxxxxxx
1312python -m pip install --upgrade pip
3
This command will upgrade pip to the latest available version.
- Resolving setup.py and python version conflicts
Secondly, the setup.py file may not be compatible with the current Python interpreter. Depending on whether you are using virtual environment or not, you should check for any potential python version conflict. If such a conflict exists then you might need to upgrade your Python or the very package’s version itself. - Cleaning up after failed installations
Errors can sometimes occur due to remnants of previous failed attempts at installing the necessary package. Such failed attempts can leave artifacts (such as cached files) behind which can interfere with new installation attempts. Luckily, pip provides an option to handle caches using the commands:xxxxxxxxxx
131pip cache purge
2pip cache info
3
Running ‘pip cache purge’ will clear all files from the pip cache saving you from potential problems. Running ‘pip cache info’ after purging will provide information regarding the active cache settings and their location.
Re-running the installation command
After following the above trouble shooting steps, let’s run the pip installation command again:
xxxxxxxxxx
pip install [package-name]
Hopefully, now everything works smoothly and the targeted Python packages get installed.
Although error messages can initially seem daunting, they offer valuable clues to identify root issues. By systematically troubleshooting these issues as discussed above, ‘Legacy-Install-Failure’ error could potentially be mitigated efficiently.
Remember that consistency is key. If errors are still persistent, consider checking other dependencies and aspects like firewall, permissions or refer to logs/details provided along with error messages. In addition, sites like Stack Overflow offer countless discussions addressing similar issues. You might find additional information here which may assist you in solving your particular problem.
I’ve also provided a simple sample source code for reference purposes:
xxxxxxxxxx
import os
def install(package):
try:
if hasattr(pip, 'main'):
pip.main(['install', package])
else:
pip._internal.main(['install', package])
except Exception:
print(f'Failed to Install {package}')
packages = ['requests', 'numpy', 'pandas']
for package in packages:
install(package)
In this script, we are loading a list of packages and trying to install them one by one. If any installation fails, we catch the exception and skip to the next package.The system configuration plays a crucial role in Python’s package manager, pip’s legacy install failure message – “Error: Legacy-Install-Failure”. The complexities of system settings and configurations can sometimes result in software glitches and failures, impacting the smooth installation of packages using pip.
Let’s discuss the key components one by one:
User Privileges
Installations of any package, including Python packages via pip, often require administrative or superuser privileges. Sometimes, the lack of appropriate permissions may lead to the “Error: Legacy-Install-Failure” message.
For instance, an attempted pip install without sufficient user permissions may look like this:
xxxxxxxxxx
$ pip install packagename
If this fails due to inadequate permissions, changing your user status using sudo (a command for Unix/Linux based systems) might resolve the issue:
xxxxxxxxxx
$ sudo pip install packagename
System Compatibility
Another factor in the system configuration that affects pip installations is the compatibility between the operating system(OS), the version of Python installed, and the package version being installed.
* Operating System (OS): Different platforms (e.g., Windows, MacOS X, Linux distributions) handle installations differently. Some packages are OS-dependent, hence a package working seamlessly on a particular OS might fail on another.
* Python Version: The version of Python you are running may be incompatible with the package you’re trying to install, leading to the ‘legacy install failure’.
Ensure you have a compatible version of both python and pip. Use these commands to check your versions:
xxxxxxxxxx
python --version
pip --version
Environment Variables
Environment variables contribute to system configuration and impact pip installations. PATH is a critical environment variable, pointing the system to executable files. If your Python and pip executables aren’t correctly saved in PATH, ‘legacy install failure’ error may occur.
You can view the PATH variable with this command:
xxxxxxxxxx
echo $PATH
Installation method
It matters how Python and pip got installed on your system. For instance, if they are part of a complex package themselves, like Anaconda, it may result in conflicts causing the legacy install failure.
Understanding these factors underlines the cause of the “Legacy-Install-Failure”. To analyze and troubleshoot, you’ll need a deep comprehension of your system configuration and a good knowledge of Python and its packages workflow.
For advanced problems, communities like StackOverflow are invaluable resources, where experienced fellow developers provide solutions to similar problems.
Remember, the tools and commands may vary based on your OS and Python environment. So, when troubleshooting, ensure you’re referring to instructions specific to your setup.Harnessing Stack Trace to Debug an Error Messages Like the Python PIP: “Error: Legacy-Install-Failure” involves a detailed understanding of interpreting and debugging stack traces, as well as familiarity with Python’s Package Installer(Pip), and its related installation failure scenarios.
The Stack Trace is a report that provides insights into the active stack frames at specific points in time during the execution of a program. When an unhandled exception occurs in your Python code, a traceback, which comes from the stack trace, is outputted.
Consider this hypothetical error scenario, for instance:
xxxxxxxxxx
Traceback (most recent call last):
File "/home/user/Desktop/script.py", line 4, in <module>
import custom_package
File "/home/user/.local/lib/python3.8/site-packages/custom_package/__init__.py", line 1, in <module>
from .module import function
ModuleNotFoundError: No module named 'custom_package.module'
Carefully observing the above traceback, it indicates that an error occurred on `line 1` within the `__init__.py` file of the `custom_package` while trying to import one of its modules. And flow suggests it’s triggered from `line 4` in the `script.py` file.
Moving on to decoding “Error: Legacy-Install-Failure” in Python Pip, it’s a relatively common problem encountered when a package installation via pip fails. This could be due to several factors:
– Incompatibility issues between your Python version and the package you’re trying to install.
– Using an outdated requirements.txt file without specifying package versions properly, leading to conflicts.
– Incorrect or incomplete installations of previous packages.
– Any other setup-related issues.
To fix such errors, follow these strategies:
– Be sure you’re using the latest pip version. You can do so by upgrading pip with:
xxxxxxxxxx
python3 -m pip install --upgrade pip
– Attempt to reinstall the problematic package or dependencies with verbose logging turned on to gain more information during failure, using the following command:
xxxxxxxxxx
pip install --verbose package-name
– If you’re using a requirements file, ensure you have properly specified the correct versions for each dependency.
Finally, as a coder, leverage resources available to facilitate your debugging process. For example, online code collaboration platforms like StackOverflow is amazing for finding solutions to common problems or getting expert advice when dealing with complex scenarios.When encountering the “Legacy-Install-Failure” error in Python Pip, it is generally indicative of an issue with the backward compatibility of python packages. This may result from a variety of factors, including incorrect package versions or conflicts between dependencies, caused by abrupt changes in the intervening package versions, outdated Python version, or non-compliant coding syntax.
With the evolving Python environment, certain methods get deprecated or replaced. For instance,
xxxxxxxxxx
‘imp’ module has been replaced by ‘importlib’
. Some pip packages may still be using the old approach which may no longer be compatible with the latest Python versions, hence resulting in such errors.
Here are some potential solutions to rectify this error:
1. Updating The Package:
Regularly updating your python packages ensures that they have support for the most recent installations. Use
xxxxxxxxxx
pip install --upgrade package-name
for this process.
2. Checking Package Dependencies:
It may happen that these packages depend on older versions of other packages, and upgrading them might break this dependency leading to such legacy errors. Auditing the package dependencies using
xxxxxxxxxx
pipdeptree
can identify potential conflicts and help resolve them.
3. Verifying the Python Version Compatibility:
Ensure you’re using a compatible Python version with your packages. Package documentation often states the version compatibility guidelines. If not maintained, legacy issues may arise.
4. Reinstalling Pip:
Reinstalling pip can recover from any corruption errors and can resolve this issue in some cases. It’s similar to the system restart approach in troubleshooting.
5. Creating Virtual Environments:
Virtual environments allow users to create isolated spaces for Python application development, where each can have its packages without interfering with each other. Hence, avoiding potential legacy install failure issues.
Method | Action |
---|---|
Pip upgrade |
xxxxxxxxxx 1 1 1 pip install --upgrade package-name |
Audit Dependencies |
xxxxxxxxxx 1 1 1 pipdeptree |
Python Version Check | Refer Package Documentation |
Pip Reinstallation | Follow pip reinstallation guide |
Use Virtual environments | Create new venv |
While these strategies can help mitigate the “Legacy-Install-Failure” error in python pip, it is essential to remember that the world of programming is continuously evolving. As a professional coder, it’s our responsibility to stay updated with the latest tools, platforms, coding norms, and compatibility standards. Therefore, continuous learning, regular system updates, and thorough coding practices remain critical to address such potential threats to system stability and efficient code execution.
Please refer to the official python pip documentation and pipdeptree documentation for detailed instructions and solutions related to specific packages.