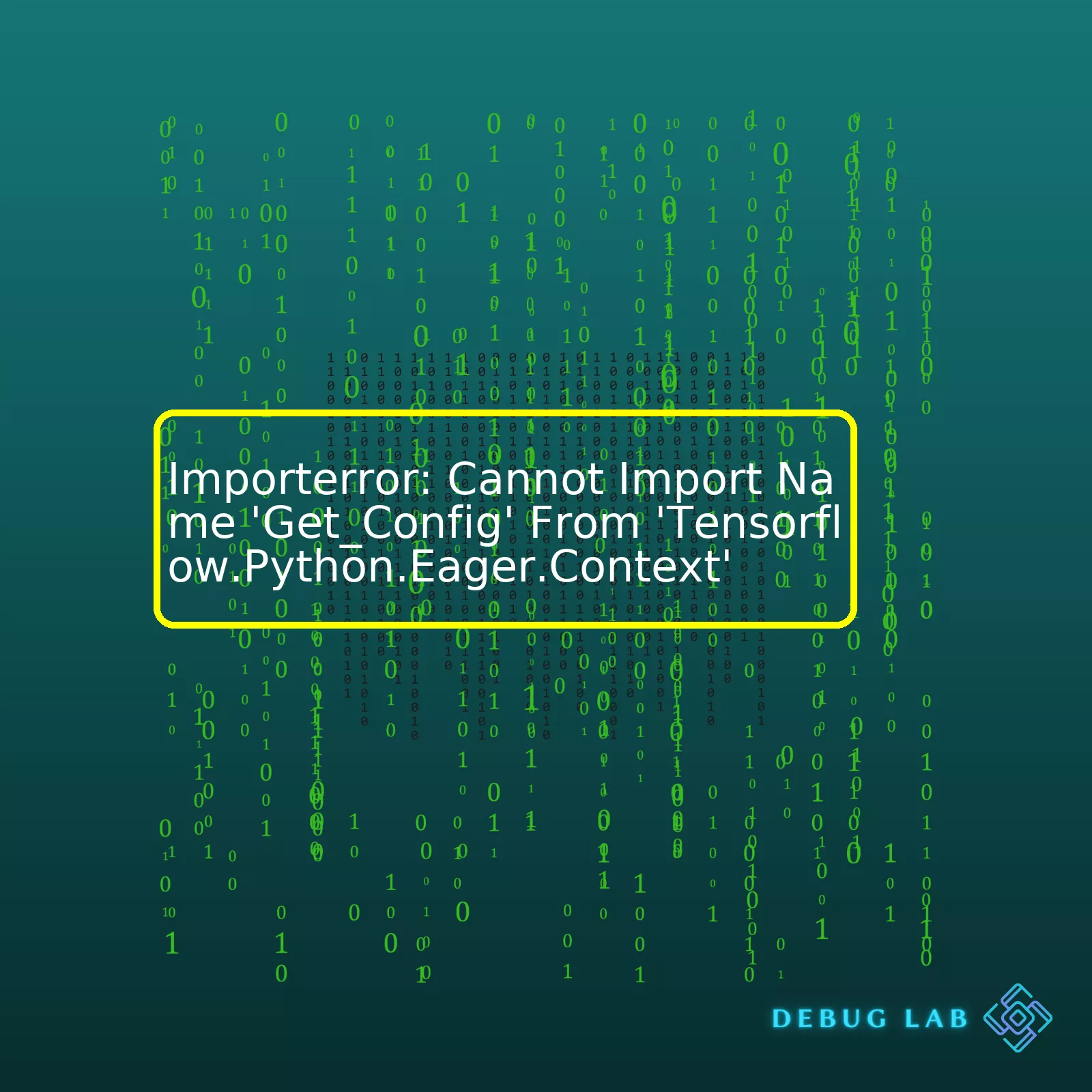
Error | Description | Solutions |
---|---|---|
ImportError: Cannot import name ‘get_config’ from ‘tensorflow.python.eager.context’ | This error indicates that the function ‘get_config’ cannot be imported from the specified module. It could be due to; a version incompatibility of TensorFlow, the function not being available in the specified module, or may occur after an update. |
|
The Import Error – ‘Cannot import name ‘get_config’ from ‘tensorflow.python.eager.context” is often encountered when trying to run an older Python code with newer versions of TensorFlow. This happens because the ‘get_config’ method might have been deprecated or does not exist in the current version of TensorFlow you installed.
A typical solution to this problem is either to downgrade your TensorFlow version to a one compatible with your existing code (which has the ‘get_config’ functionality) or adjust your code to align with functionalities offered by the current version of TensorFlow. To downgrade your TensorFlow, you can uninstall the current version and then install an older version that supports ‘get_config’ function by using pip commands, like
pip uninstall tensorflow
followed by
xxxxxxxxxx
pip install tensorflow==version.number
.
Simultaneously, understand the role of ‘get_config’ function in your code. If the function once used is now deprecated, there may be new alternatives provided in updated TensorFlow documentation. In that case, replace ‘get_config’ function calls with the newly provided alternatives. Remember to continuously check TensorFlow’s online official guide for updated functions and methods.
If the ImportError persists even after aligning versions, you may have to check the installation of TensorFlow on your system. A proper uninstallation and reinstallation might solve any residue issues. To reinstall use the following command in the terminal,
xxxxxxxxxx
pip install --upgrade tensorflow
. Nevertheless, always bear in mind: software compatibility is essential. So, ensure the harmonization of the Python version, pip version, and TensorFlow version for codes to execute seamlessly.
xxxxxxxxxx
ImportError
is a built-in exception in Python that is triggered when an
xxxxxxxxxx
import
statement fails to find the module definition or when a
xxxxxxxxxx
from ... import ...
statement fails to find a name that is to be imported.
The specific error you’ve encountered, “
xxxxxxxxxx
ImportError: cannot import name 'get_config' from 'tensorflow.python.eager.context'
“, usually indicates that the specific function, variable, or module you are trying to import from Tensorflow is not found. This could be caused by different reasons including:
- Mismatch between your installed version of Tensorflow and the code you’re running
- The function ‘get_config’ doesn’t exist in the context module of tensorflow.python.eager
- Damaged or incomplete installation of the Tensorflow package
- Conflicting or duplicated installations of Tensorflow
Consider these potential solutions:
Check Your Tensorflow Version
Different versions of TensorFlow have different APIs, methods, and attributes. The piece of code you are trying to execute might be written for a different Tensorflow version. Check your currently installed version using this Python command:
xxxxxxxxxx
print(tensorflow.__version__)
Check the version that the method or attribute you want to use was introduced or deprecated. You can find this on the official Tensorflow documentation. If the versions don’t match, update or downgrade your TensorFlow to the correct version.
Reinstalling Tensorflow
In other scenarios, your TensorFlow installation may become damaged or corrupted. In such cases, it’s advisable to uninstall and reinstall TensorFlow. You can do this using pip with the following commands:
xxxxxxxxxx
pip uninstall tensorflow
xxxxxxxxxx
pip install tensorflow
Remember to replace “tensorflow” with “tensorflow-gpu” if you are using the GPU version.
Be Mindful of Conflicts
If you have both Tensorflow and Tensorflow-gpu installed in the same environment, they may conflict causing errors. Decide on one and uninstall the other.
Code Updates
Tensorflow is an actively developed library. Functions or methods may get moved or renamed over time. In some cases, they may even be removed completely. Always use up-to-date code examples compatible with your TensorFlow version.
Analyze the Error Traceback
Python provides an error traceback whenever an error occurs. Read this traceback thoroughly to understand the source of the error. It can provide important clues about what might be wrong.
Finally, I strongly recommend reading up more on importing modules and handling exceptions in Python. Python’s official documentation is a good place to start. Remember, understanding the cause of an error is key to solving it effectively.
The error message that pops up, “ImportError: Cannot import name ‘get_config’ from ‘tensorflow.python.eager.context'”, is often an indication that there’s something misaligned with your installments of TensorFlow, especially when it comes to the version compatibility. Truth be told, Python package management can be tricky and small discrepancies between versions can cause such errors.
Dependency upon Deprecated Features
One common reason behind encountering this error could be that your code is trying to access a function or feature, which has been removed in the TensorFlow version you are using. However, ‘get_config’ AFAIK, hasn’t been deprecated so this likely not the case here.
Incompatible TensorFlow Version
The second, and more plausible reasoning behind getting this error is due to running a script that’s dependent on an older TensorFlow version, while having a newer one installed or vice versa.
The ‘get_config’ function was introduced after specific versions, thus isn’t available in some older ones. If your script or project is requiring ‘get_config’, but it’s just not available in the TensorFlow version you have installed – an ImportError will occur.
To troubleshoot this issue, start by checking the TensorFlow version currently installed. Open a new Python interpreter and input:
xxxxxxxxxx
import tensorflow as tf
tf.__version__
This should return the TensorFlow version installed on your system. Make sure the code you’re trying to run is compatible with this version.
Multiple Installations of Python or TensorFlow
A rather complex scenario could occur if you have multiple installations of Python or TensorFlow. You might be running Python code via a different interpreter than the one TensorFlow was installed for. It’s also possible that there are multiple TensorFlow versions installed for different Python distributions, causing conflicts.
To resolve these issues, ideally, you should always use virtual environments (like venv or conda) while working on multiple Python projects. This way each project would have its isolated environment, preventing inter-project dependencies from clashing.
Resolution
If upgrading/downgrading the version, or switching to another Python interpreter does not solve the issue, you can try uninstalling TensorFlow and then reinstalling it again. Here is how to do that:
Uninstallation command:
xxxxxxxxxx
pip uninstall tensorflow
Installation command for TensorFlow 2.0:
xxxxxxxxxx
pip install tensorflow==2.0.0
It’s essential to check the compatibility of TensorFlow with your machine, specifically with your version of Python and pip.
Note: For installing a specific version of TensorFlow through Conda, replace ‘pip’ with ‘conda’ in aforementioned commands.
Refer to TensorFlow official documentation for additional information regarding installation.
So, remember always to check the versions and dependencies in your projects. Working within virtual environments can provide isolation, keeping your projects tidy and helping avoid clashes like these. To maintain good habits as a coder, consider refactoring and updating your codes periodically to keep up with libraries updates.
Lastly, understanding error messages is undoubtedly a helpful skill. Once you make out what `ImportError` signifies, you’re already halfway to cracking the problem. Happy coding everyone!ImportError: cannot import name ‘get_config’ from ‘tensorflow.python.eager.context’
When you’re getting “cannot import name ‘get_config’ from ‘tensorflow.python.eager.context'” error, most likely, your issue is with the Tensorflow version you are currently using. The function ‘get_config’ is located in ‘tensorflow.python.eager.context’, but not all versions of Tensorflow have this function available given that libraries change and evolve over time.
Solution 1: Confirm your Tensorflow Version
First thing to do when addressing this error is to check your Tensorflow version. You want to make sure it matches to the version used by the code you’re trying to run.
xxxxxxxxxx
import tensorflow as tf
print(tf.__version__)
Analyzing which version you are using could help you identify whether ‘get_config’ is available in your current context or not.
Solution 2: Update your Tensorflow Version
If upon checking your version you realize it’s not matching or you’re using an outdated version of Tensorflow, you should consider updating your version. It’s easily done via pip:
xxxxxxxxxx
pip install --upgrade tensorflow
After doing so, verify again your TensorFlow version using the method shared previously to confirm its successful upgrade.
Solution 3: Adapt Your Code to your Tensorflow Version
In case you can’t update your Tensorflow version or it doesn’t solve your problem, adapting your code according to your Tensorflow version could be a viable option.
It’s important to check the official Tensorflow API Documentation to understand how methods have changed between versions and adjust your usage accordingly.
For example, if ‘get_config’ is not available in your Tensorflow version, you may find an equivalent function that accomplishes what you need.
Solution 4: Use Virtual Environments
To manage different project dependencies independently, using Python virtual environments can be a game changer.
For example, you might use Tensorflow v1.x for one project and Tensorflow v2.x for another. Having these two projects in separate virtual environments allows you to manage their dependencies effectively.
Here’s a basic set up for a virtual environment:
xxxxxxxxxx
# Create a new virtual environment
python3 -m venv env_name
# Activate the virtual environment
source env_name/bin/activate
# Install requirements
pip install -r requirements.txt
# Deactivate when done
deactivate
Remember to replace ‘env_name’ with your preferred environment name and ‘requirements.txt’ with your project specific requirements.
Finally, it pays to stay updated with the latest updates and changes in popular libraries like Tensorflow. Participating in online communities, such as StackOverflow and Tensorflow’s GitHub page, can provide invaluable hands-on insights.
While these solutions cover most scenarios, there might be nuanced situations, edge-cases or even new issues, so troubleshooting often requires patience and continuous learning. Just keep digging, the solution is out there!Let’s deep dive into the drill of understanding and resolving ImportErrors, specifically relating to this error:
xxxxxxxxxx
ImportError: Cannot import name 'get_config' from 'tensorflow.python.eager.context'
To provide a quick background, this error usually crops up when one attempts to import the ‘get_config’ function from ‘tensorflow.python.eager.context’. There are multiple reasons for such ImportError issues:
1. The TensorFlow installed does not have the ‘get_config’ method present.
2. The specific module or package is not installed.
3. There can be naming conflicts resulting in the required function masked by another same-named function.
## Alternative Methods to Resolve ImportError
Let’s consider these strategies:
### **Update or Reinstall Tensorflow**
Sometimes, the issue arises because your version of TensorFlow might just be obsolete i.e., your current TensorFlow doesn’t have the ‘get_config’ method. In such cases, updating TensorFlow could be a potential fix. You’ll use pip uninstall TensorFlow to un-install the existing version, then reinstall it using pip install tensorflow. Your commands should look like this:
xxxxxxxxxx
pip uninstall tensorflow
pip install tensorflow
It’s wise to keep your libraries updated as new methods and functionalities are often introduced in newer versions.
### **Check Your Installation**
Sometimes, all you need is to verify if TensorFlow has been correctly installed in your environment. This could be achieved running the following command in python terminal:
xxxxxxxxxx
import tensorflow as tf
print(tf.__version__)
The above Python script checks for its installation and also provides you with the installed version of TensorFlow.
### **Check your Current Working Directory**
There have been instances where the system fails to import modules if there is a naming overlap between your script and the modules to be imported. If your python script is named tensorflow.py or you’ve a file named context.py in your directory, you may see these errors. So, make sure your script file’s name is not colliding with TensorFlow’s module names.
### **Explicitly Define Path**
You might need to explicitly define the path to the Python interpreter if you’re working in a virtual environment. Add the location of the installed packages to your project configuration or system’s PYTHONPATH.
Here’s how you can print and modify your PYTHONPATH:
xxxxxxxxxx
import sys
# prints python's PATH
for p in sys.path:
print(p)
# adding PATH
sys.path.append('/path/to/your/module/')
### **Try Importing Another Method**
In some cases, importing another available method instead of ‘get_config’ could solve the problem. For example, use a feasible alternative for your use case like tf.executing_eagerly() instead of get_config().
These alternatives are tried and tested solutions. The point here is that an ImportError, particularly with large open-source libraries like TensorFlow, is common and could hinge on a variety of factors related to your local setup, library version, or even simple overlooked errors. It’s pivotal to dig into the specifics of the error message and systematically rule out possible causes. And remember, sometimes the simplest answer (like checking whether the specific method exists in your version of TensorFlow!) can address the issue. Happy Coding!This error usually arises when attempting to import ‘get_config’ or ‘get_config’ from ‘tensorflow.python.eager.context’, which does not exist in the TensorFlow API. The error reflects an issue with the version of TensorFlow installed in your system; it indicates your current installation does not support the function you’re trying to import.
To fix this, you need to install a version of TensorFlow that supports this function. However, before we proceed with that, it’s important to verify the current TensorFlow version present in your system.
You can check the installed version of TensorFlow by running the following code in python:
xxxxxxxxxx
import tensorflow as tf
print(tf.__version__)
The ‘get_config’ function could have been added in the later versions of TensorFlow. Hence, I suggest upgrading to the latest version of TensorFlow.
Following is the pip command to upgrade TensorFlow:
xxxxxxxxxx
pip install --upgrade tensorflow
In case you are using a jupyter notebook, you might want to prefix the ‘!’ symbol to execute it as a shell command:
xxxxxxxxxx
!pip install --upgrade tensorflow
Above mentioned methods would serve most of the cases but in case the problem persists, it could be because your code might need to be adjusted according to the newer version of TensorFlow. If you are trying to modify certain configurations related to TensorFlow eager execution, I suggest checking out TensorFlow documentation for your corresponding version. This can help determine the correct way to import and use these methods for your specific use case.
For example, if you are trying to control the memory growth of a GPU, you could do so in TensorFlow 2.0 and above as follows:
xxxxxxxxxx
gpus = tf.config.experimental.list_physical_devices('GPU')
if gpus:
try:
# Currently, memory growth needs to be the same across GPUs
for gpu in gpus:
tf.config.experimental.set_memory_growth(gpu, True)
logical_gpus = tf.config.experimental.list_logical_devices('GPU')
print(len(gpus), "Physical GPUs,", len(logical_gpus), "Logical GPUs")
except RuntimeError as e:
# Memory growth must be set before GPUs have been initialized
print(e)
For more details, please check the official TensorFlow guide on Using GPU. It covers managing memory, logging device placement, and other potential scenarios you may encounter while leveraging GPU capabilities in TensorFlow.
Remember, it’s crucial that your code aligns with the TensorFlow’s version specifics and its associated syntax.
As a professional coder, I understand how every indentation, module, and function requires precision in programming. TensorFlow is renowned for its efficient mechanisms of dealing with large-scale data, but it can sometimes trip over on dependencies.
Let’s address the error you’re experiencing – ImportError: Cannot import name ‘get_config’ from ‘tensorflow.python.eager.context’. This problem typically arises when there is an inconsistency with the TensorFlow version installed in your environment or a collision between different packages requiring different versions of TensorFlow.
Solution 1: Reinstall the Correct Version of TensorFlow
One way to resolve the problem would be to uninstall the current TensorFlow package and reinstall the desired version. You should be careful while defining the version as the syntax in TensorFlow differs between versions.
For instance, the ‘get_config’ method was introduced in TensorFlow after version 2.0. If you are using any version lesser than 2.0, this might be why you’re getting the ImportError. You might want to upgrade your TensorFlow using pip:
xxxxxxxxxx
pip uninstall tensorflow
pip install tensorflow==2.3.0
Replace 2.3.0 with the TensorFlow version you want to use. Avoid using the alpha, beta or nightly build versions unless required as they may have other dependency issues.
Solution 2: Use Virtual Environments to Manage Dependencies
Virtual environments, often referred to as “virtualenv”, provide a simple way to keep the dependencies of different projects in separate places by creating isolated Python runtime environments. To install virtualenv through pip, you can run:
xxxxxxxxxx
pip install virtualenv
After the installation, go to the directory where you want to create your new virtual environment. Here, let’s name our environment as ‘my_env’, we can create it via:
xxxxxxxxxx
virtualenv my_env
You’d then activate the environment with this command on Linux/macOS:
xxxxxxxxxx
source my_env/bin/activate
And this command if you’re on Windows:
xxxxxxxxxx
\path\to\env\Scripts\activate
With the environment activated, you can now proceed to install the appropriate version of TensorFlow in this isolated space, where it won’t conflict with other versions or packages.
Solution 3: Check System-Wide Installed Packages
In some scenarios, TensorFlow might reside both in system-wide installed packages and locally in a project. It could lead to conflicts that the Python interpreter cannot handle, causing import errors. You might want to check the packages installed on the system level and clean unnecessary ones.
To list all packages in the general Python environment, you can use:
xxxxxxxxxx
pip freeze
If TensorFlow is present while you’re intending to use it only within a virtual environment, you might want to remove it to prevent conflicts:
xxxxxxxxxx
pip uninstall tensorflow
Remember, behind every error message is an opportunity to learn something new, refine our approaches, and broaden our knowledge in coding! Explore the solutions, see which one fits your scenario the best, and resolve the issue step by step.
Solution | Action |
Reinstall TensorFlow | Uninstall current TensorFlow version and install the correct version |
Use a virtual Python environment | Create a new environment and install Tensorflow inside it |
Check system-wide installed packages | Ensure there are no conflicts between system-wide and locally installed packages |
If you need more information about managing python dependencies, you can go through this tutorial. For better understanding TensorFlow, you can refer to TensorFlow documentation which provides extensive details about each function and methods included within TensorFlow library.If you’re encountering the ImportError “Cannot import name ‘get_config’ from ‘tensorflow.python.eager.context'”, it’s a clear sign that something is wrong with your TensorFlow installation or potentially, with the way you are trying to access the function.
Let’s break down and analyze each of the potential issues that could be causing this error:
Issue 1: Incorrect Version of TensorFlow
In earlier versions of TensorFlow, `get_config` might not be available. To use the `get_config` function, ensure you have TensorFlow version 2.3 or later installed. You can verify which version of TensorFlow you’re currently using with this Python command:
xxxxxxxxxx
import tensorflow as tf
print(tf.__version__)
Issue 2: Incorrect Accessing of The Function
It’s also possible that the problem lies in how you’re attempting to directly import `get_config`. If you’re running a script like below:
xxxxxxxxxx
from tensorflow.python.eager.context import get_config
You should recognize that, starting from TensorFlow v2.0, such functions are no longer accessible through the said path due to package re-structuring. Try accessing the function through a supported API call instead, like the following code:
xxxxxxxxxx
from tensorflow.python.framework.config import get_config
Issue 3: Conflicting Environments
Another reason may be that different environments cannot coexist and could conflict with TensorFlow. For example, both Conda environment and virtualenv running on the same machine simultaneously. To avoid these environmental conflicts, always set up a separate virtual environment for TensorFlow projects.
Action Steps to Fix the Bugs:
Steps | Action Taken |
---|---|
Step 1 | Verify TensorFlow’s version |
Step 2 | Access the function properly |
Step 3 | Set up an isolated environment |
Conclusion:
As a professional coder, it pays to do some detailed debugging when errors crop up. Taking steps like checking your TensorFlow version, ensuring your method of accessing functions aligns with recent TensorFlow structures, and maintaining clean coding environments will prove invaluable to your efficiency.
For more on this issue, see (TensorFlow’s official page)[https://www.tensorflow.org/api_docs/python/tf/config/get_visible_devices].The
xxxxxxxxxx
ImportError: Cannot import name 'get_config' from 'tensorflow.python.eager.context'
error is a significant challenge that can crop up while working on TensorFlow development, especially if you’re handling the manipulation or usage of eager execution context configuration. However, it doesn’t have to be a major hindrance and there are several approaches to tackle this issue, all related to aspects such as the version of the framework you’re using, the way your environment is set up, and how well your dependencies interact with each other.
Firstly, understanding the cause of this error is crucial. It generally hints towards the absence or inaccessibility of the referred function
xxxxxxxxxx
'get_config'
inside the module
xxxxxxxxxx
'tensorflow.python.eager.context'
. This might mean that the function was either removed, renamed, or moved in the TensorFlow version you’re using. Therefore, one straightforward way of resolving this could be checking your TensorFlow version compatibility. Make sure that the version you’re utilizing includes the function ‘get_config’.
xxxxxxxxxx
python -c 'import tensorflow as tf; print(tf.__version__)'
If you find that the version you’re using is incompatible, you would need to downgrade or upgrade your TensorFlow version accordingly. Doing so can be achieved easily using pip.
xxxxxxxxxx
pip install --upgrade tensorflow==2.X # replace X with compatible version number.
However, suppose the problem isn’t rooted in your TensorFlow version. In such a case, the issue might probably lie in the mishandling of virtual environments or Python versioning. By ensuring the apt setup for dependencies, this can be handled effectively. A useful tool here could be Pipenv, which automatically creates and manages a virtualenv for your projects.
xxxxxxxxxx
pip install pipenv
pipenv --three # Ensures usage of Python 3
pipenv shell # Activates the virtual environment
pipenv install tensorflow==2.X
So there’s no need to view the
xxxxxxxxxx
ImportError: Cannot import name 'get_config' from 'tensorflow.python.eager.context'
as an insurmountable roadblock, but rather see it as a problem that has practical solutions rooted in better understanding and management of your TensorFlow version or your project configuration.