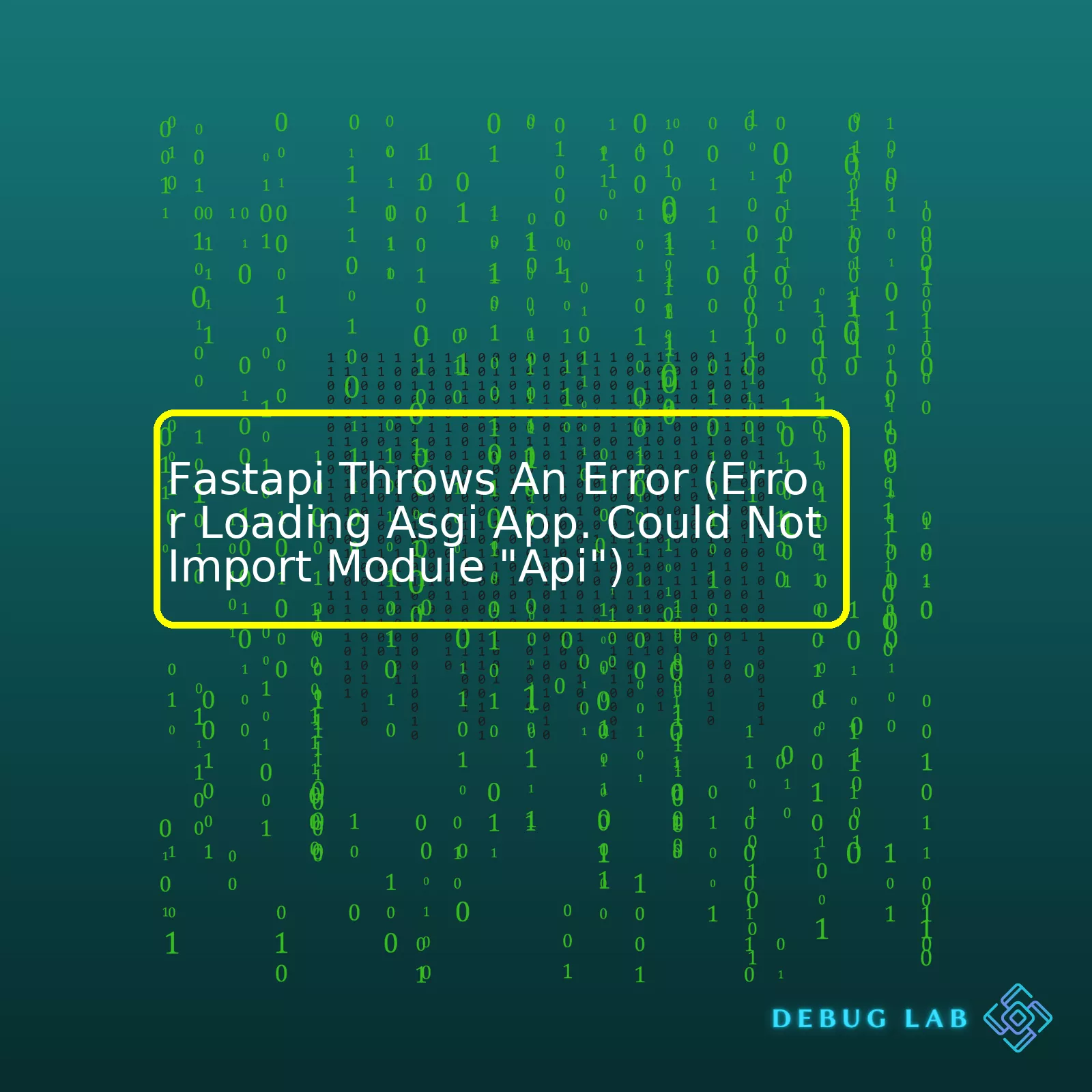
Issue | Reason | Solution |
---|---|---|
FastAPI Throws An Error (Error Loading ASGI App. Could Not Import Module “API”) | The error is usually a consequence of the server’s inability to locate or import a specific module named “API”. Here are some reasons:
|
Here are potential solutions for this problem:
|
The error FastAPI Throwing an ASGI App loading issue and unable to import the module “API” occurs when the deployment environment struggles to find, load or import a specific module labeled “API”. This often suggests that the module is either non-existent, or glitches persist in your application’s directory structure leading to discrepancies between the hierarchy assumed by Python, and what is actually there.
On another note, it could also mean inconsistencies amid parent packages and the current directory format due to how Python manages imports. This can create some complexities, particularly when dealing with intricate packages and their directory structures.
To debug this error, start by affirming whether the ‘API’ module genuinely exists in your application with correct spelling and case accuracy. Subsequently, you may need to scrutinize your import statements; pay close attention to relative imports, ensuring they align with your directory setup. Often, it’s recommended to opt for absolute imports instead, where you specify the complete path from the root of your app to the concerned module.
In particular, this error assimilation and solution strategy involves these key steps:
# Ensure the API module exists
from api import ProjectAPI #replacing 'api' with actual API module name
# Check directory setup
import os
print(os.getcwd()) #check current working directory
# Use absolute imports over relative
from root_project_folder.subfolder import api #sample absolute import statement
Understanding these causes and remedies would come handy in quickly rectifying the given error, and eventually smoothening your FastAPI experience.Debugging the FastAPI ASGI Load Error: An In-Depth Look
The FastAPI ASGI (Asynchronous Server Gateway Interface) load error, particularly the “Error Loading ASGI app. Could not import module ‘api'” can be a real stumbling block for developers. The challenge is to understand this error, pinpoint its potential causes, and figure out ways to solve it.
Understanding the ASGI Load Error
ASGI allows asynchronous applications written in Python to communicate with servers. It’s an essential tool within the FastAPI toolbox, enabling highly efficient handling of HTTP requests. When the error “Error loading ASGI app. Could not import module ‘api’,” rears its head, it simply means that the Python interpreter cannot find or import the specified module, ‘api’.
Main Causes of the FastAPI ASGI Load Error
There are several common culprits behind this error:
- Incorrect File or Directory Names: Python’s import system is case-sensitive. Therefore, if there is a discrepancy between file names or directory names and their corresponding references in the code, imports fail.
- Misconfigured sys.path: The location from which modules are imported needs to be part of Python’s search path (
xxxxxxxxxx
111sys.path
). If ‘api’ isn’t listed, Python won’t know where to look for it.
- Flawed Programming Logic: If there are errors within the ‘api’ module’s code such as indentation errors, missing parentheses, or incorrect syntax, Python will struggle to import it successfully.
Solving the ASGI Load Error
Shared below are some steps that can help to debug the issue properly:
1. Verify Your Filenames and Directories:
Check that your filenames and directories match their references in your code. These should be case-sensitive matches. For instance, if your module file is called
xxxxxxxxxx
API.py
, check that you aren’t importing it as
xxxxxxxxxx
import api
.
2. Check and Adjust Your sys.path:
To see what’s currently in your
xxxxxxxxxx
sys.path
, print it:
xxxxxxxxxx
import sys
print(sys.path)
If your ‘api’ module’s directory isn’t listed, add it using
xxxxxxxxxx
sys.path.append()
. Here is how you do it
xxxxxxxxxx
sys.path.append('/path/to/your/module')
Remember not to permanently alter
xxxxxxxxxx
sys.path
if there are other scripts relying on the existing configuration.
3. Debug Your Code:
Scrutinize the ‘api’ module’s code to flag any syntax or logical errors. Use built-in Python tools such as pylint or online services like pylint.org to facilitate the process.
A Final Note: Absolute vs Relative imports
You may want to consider whether your project favors absolute or relative imports. In cases where directory structure could be causing the problem, switching from one to the other might solve the issue. Consult the Python documentation to learn more about the use and benefits of each.
Remember, debugging is an integral and necessary part of coding. Errors like these can often lead to better comprehension and a cleaner, more functional end product. Happy Debugging!The error message “Error Loading ASGI App: Could not import module ‘api'” in FastAPI is a very common issue that developers face. These error messages generally occur when the ASGI server, such as Uvicorn or Hypercorn, fails to find or load the application instance from the specified python module. ASGI (Asynchronous Server Gateway Interface) is the specification detail of how asynchronous servers communicate with applications. FastAPI is built on top of Starlette for the web parts and Pydantic for the data parts taking full advantage of Python 3.6 type declarations.
Diagnosis of this error message often revolves around two common causes:
1. Incorrect Module Name:
Your application may fail to run if you provide an incorrect module name while trying to start the server. The command typically looks like this:
xxxxxxxxxx
uvicorn main:app
In this command, “main” refers to the filename of your python script where you’ve defined your FastAPI app and “app” is the FastAPI application instance. If your application is defined in a different script file, replace “main” with the actual filename or correct module path. Make sure you do not include the “.py” extension of the Python file.
Moreover, working with larger applications might involve several modules that are nested within directories, hence the module path has to be properly addressed. For example, if your FastAPI application instance is located in “/app/api/main.py”, the command would look like this:
xxxxxxxxxx
uvicorn app.api.main:app
Moving across directories requires “.” whereas moving to a new file requires “:”.
2. Misnamed Application Instance:
The second part of the command after the colon “:” refers to the application instance. A common convention is to name this variable as “app”. However, it can be named anything else based on your application’s code. If the app instance name specified doesn’t match with the one in your code, you will encounter this error. It simply means that the Uvicorn server couldn’t locate an ASGI application instance in your designated python module.
Consider your FastAPI app is defined like this:
xxxxxxxxxx
from fastapi import FastAPI
myapp = FastAPI()
get('/') .
def read_root():
return {'Hello': 'World'}
You should then start the uvicorn server referencing “myapp” instead of “app”:
xxxxxxxxxx
uvicorn main:myapp
In conclusion, paying close attention to the naming of FastAPI application instances and their proper referencing holds key to rectifying these errors. Also important is to maintain careful structuring of your project. A good practice is to keep the FastAPI instance in a standalone file at a high-level location in your directory structure, reducing chances of misnaming and mistargeting.
For more information on FastAPI and handling errors, visit the FastAPI documentation. Here, links to comprehensive guides, tutorials and other resources to understand the framework better are provided.We delve into the world of coding in Python, where blank screens and intriguing mysteries are a day’s work. One such quirk under our microscope today is ASGI (Asynchronous Server Gateway Interface) application load failure often encountered during FastAPI usage. Your coder’s life might have been stirred by this error message:
xxxxxxxxxx
Error loading ASGI app. Could not import module "Api".
Here’s taking a closer gaze at this cryptic message that grates on your nerves when you just want your app to run smoothly.
A probable cause for this discrepancy arises when the system cannot find the referenced API module. You might think, what causes this scenario? Most metropoles draw back to the interplay between import directory structure, PYTHONPATH, and how FastAPI honors these imports leading to this ‘Error loading ASGI app. Could not import module “Api”‘ failure.
Guardrail Principle – The Directory Structure
Our first step to decode this error involves understanding the way our directory structure functions, influencing the process of importing modules. For example, if your directory structure resembles something like this:
xxxxxxxxxx
fastapi-app/
main.py
api/
__init__.py
endpoints/
__init__.py
endpoint_a.py
tests/
__init__.py
The present directory setting allows main.py to import from api. However, if another script tries to import api while residing outside the fastapi-app/ directory, prepare for a name import error to pop up. Verifying the relative position of each file remains crucial.
The All-Knowing PYTHONPATH
PYTHONPATH is an environment variable which instructs Python about which directories should be used for module searching. If the right directory doesn’t make it to the PYTHONPATH list, even absolute imports miss the mark.[1]
Taking the previous directory as an example, if you are running a script from tests/, without having fastapi-app/ in PYTHONPATH, the system wouldn’t know where to find module ‘api’.
Using
xxxxxxxxxx
sys.path.append
is a common practice to append new paths. Your code can look something like this:
xxxxxxxxxx
import sys
sys.path.append("/path/to/fastapi-app")
This adds fastapi-app to PYTHONPATH, making the ‘api’ module accessible for import. But remember, this path alteration is temporary and only effective within the current runtime.[2]
FastAPI’s Import Mechanism
FastAPI uses Starlette’s Route table to match incoming HTTP requests with corresponding callables3. FastAPI’s Uvicorn worker tends to interpret imported strings differently, reducing them to their exact dotted paths. For instance, “api.endpoints.endpoint_a” becomes simply “api”. This difference in interpretation can lead to failed imports.
Fixing this problem might involve restructuring the imports or modifying the PYTHONPATH. Remembering to maintain consistency between relative and absolute imports paves the way for a seamless FastAPI experience.
These pointers put together paint us a clearer picture behind the origins and solutions of the ‘Could not import module “Api”‘ mystery, transforming this cryptic alien message into understandable human language. So get ready to decode your FastAPI errors, rebuild your programs, race across your game plan with flying colors. It’s all about translating problems into solutions, uh- or decoding error messages into plausible explanations, as we coders prefer to say!If you have been using FastAPI and came across the error – Error loading ASGI app. Could not import module “api”, it could be stressful trying to sort it out. Fret not! I am here to walk you through diagnostic steps and troubleshooting techniques to solve this issue.
The error message is a clue in itself that an Application Server Gateway Interface (ASGI) application couldn’t be loaded because it could not import a module named “api”. This can happen mainly due to two reasons:
1. Non-existent or incorrectly positioned “api” module
2. Incorrect configuration setup
Let’s look at them individually.
Non-existent or Incorrectly Positioned Module:
The first thing to ascertain is that your “api” module actually exists. Ensure the Python file where you have implemented your API with FastAPI has the correct name, in this case, it should be “api.py”. If the ‘api’ module does exist, check if it’s located in the correct place. Also ensure that you run your command from the right directory level. A local relative import could fault due to a misplaced directory use.
Here’s a typical structure of a FastAPI project:
xxxxxxxxxx
app/
__init__.py
api/
__init__.py
main.py
Let’s say your FastAPI code resides in
xxxxxxxxxx
main.py
. Then, you need to use the dot delimiter to specify the path to your ASGI app when running your server:
xxxxxxxxxx
uvicorn app.api.main:app --reload
In the above command, we are instructing uvicorn to search for variable ‘app’ inside ‘<%=assistant.firstName()%>‘main’, which is in turn in folder ‘api’, present in the base directory ‘app’. Thus, ‘app’ is where our FastAPI instance is created.
Misconfiguration:
If the module positioning is confirmed to be right, the issue may lie in the configuration. The following two checks could help debug:
1. Check ASGI_APP environment value in UVICORN:
This environment variable in UVICORN should point appropriately to your FastAPI instance. For example:
xxxxxxxxxx
export ASGI_APP=api:app
This will guide UVICORN to the FastAPI instance in the module “api”.
2. Verify the working environment and installed packages
Ensure that all requisite packages and modules are properly installed in your Python environment. You may want to isolate the environment of the project by using virtual environments.
xxxxxxxxxx
pip freeze > requirements.txt
pip install -r requirements.txt
By running these commands you create a snapshot of your current environment settings with
xxxxxxxxxx
pip freeze > requirements.txt
and subsequently ensure that the same packages are installed in any environment with
xxxxxxxxxx
pip install -r requirements.txt
.
For more info on ASGI and its specs, you can refer to ASGI Documentation.
Remember, careful observation of error messages and documentation are key tools for diagnosing issues. So, with these tips, you should be able to troubleshoot FastAPI ‘Error loading ASGI app. Could not import module “api”‘ and similar errors easily. Happy debugging!While using FastAPI, you may occasionally encounter a “Error loading ASGI app. Could not import module “api”” error. Let’s delve into this problem and figure out how we can prevent it in our future coding endeavors.
The aforementioned error implies that FastAPI cannot seem to import your api module. This typically arises from one of these reasons:
• You’ve not created an “api” module.
• You specified incorrect app instance details during execution.
• Your project directory structure isn’t conducive for the imports to work correctly.
• Your current working directory doesn’t align with where your “api” module resides.
• When there is a Python path issue—FastAPI might struggle to locate and load your api module if it isn’t part of the PYTHONPATH.
To resolve the issue consider implementing the following measures:
1. Ensure Module Exists
It seems quite obvious but always ensure the module you’re trying to import exists in the first place. FastAPI will fail to import your ‘api’ module if it is missing or placed in the wrong folder.
xxxxxxxxxx
# Incorrect file structure
.
+-- main.py
# Correct file structure
.
+-- main.py
+-- api.py
2. Verify your ASGI Application Instance
Confirming the correctness of your ASGI application instance and the command used is essential. It should follow the pattern “module:instance”.
For Example:
Assuming you have a api.py file with FastAPI application instance named as app. Then:
xxxxxxxxxx
# Executing FastAPI application
uvicorn api:app --reload
This tells uvicorn to find an ASGI application called ‘app’, within an ‘api’ module.
3. Configure your Project Structure Approprily
Your scripts won’t run if your files aren’t properly organized. Employ directories and subdirectories to keep them tidy. Make certain the topmost level of your directory is discoverable by setting it through sys.path or adding an __init__.py file.
xxxxxxxxxx
import sys
sys.path.insert(0,'/path/to/your/module/')
4. Check Working Directory
Your working directory should be same as your project’s root directory. A simple pwd command assures you are working in the correct directory before running your FastAPI app.
xxxxxxxxxx
pwd
Check if it outputs /path/to/your/project. If not, navigate to the proper directory with cd /path/to/your/project before executing your application.
5. Set Up Python Path
Ensure your projects are automatically added to Python’s site-packages by leveraging .pth files. For example, create my_project.pth file inside your Python’s site-packages directory and add full absolute path of your project(directory) to this .pth file. This would ensure python interpreter is able to locate your ‘api’ module regardless of the location from which the project has been executed.
Nevertheless, efficient debugging plays a vital role in overcoming issues like these. Knowing these tricks, I trust you’ll be better equipped to deal with any similar problems down the line.
Lastly, remember to read the official FastAPI docs frequently as it provides crucial insights about many potential issues and has solutions for most of them.
Experienced coders working with ASGI applications may often come across issues where operations are unsuccessful. One such error that can occur is the “Error loading ASGI app. Could not import module Api” when using FastAPI, a modern, fast (high-performance) web framework for building APIs with Python 3.6+ based on standard Python type hints.
When presented with this error, it’s essential to understand that FastAPI is an ASGI application and hence needs an ASGI server to serve it. The problem arises when the ASGI server cannot locate or identify the FastAPI application instance due to a misconfiguration or misunderstanding of how the ASGI server locates the application object in your code.
Here are a few advanced solutions to consider to handle these scenarios:
Ensure Correct Module Names
The first possible cause that you should look into would be incorrect module names. When running a FastAPI application, you have to provide the ASGI server with the location of the FastAPI application in the format <module>.<object>, like `app:app`. Make sure the module and object names are correctly provided and exist in your project structure.
xxxxxxxxxx
uvicorn main:app
Check for Circular Imports
Cyclical imports in your code might lead to this error. For example, if file A imports from file B and file B imports from file A, it could confuse the interpreter about which to load first, leading to the module not being available at runtime. Review your imports and refactor them as necessary to prevent circular dependencies.
Verify the Working Directory
If your working directory is different from where your FastAPI application resides, an import error might occur because the interpreter cannot find your modules. Hence, you should always ensure that your working directory is the root directory of your FastAPI application, or specify the PYTHONPATH environment variable to include your FastAPI application location.
Analyze Dependency Conflicts
In some instances, the issue might arise due to conflict with dependencies installed globally on your system or within your virtual environment. Creating a separate virtual environment for your project could alleviate this problem by isolating your project dependencies.
Remember, effective debugging requires a deep understanding of the application architecture and the server setup while simultaneously considering the various reasons causing the error. By performing a systematic analysis of the problem, one can gain hints about the real reason behind any failed operation.
For additional information on resolving ASGI Problems, the ASGI Documentation is an excellent place to start for helpful insights into troubleshooting and understanding ASGI apps better.
Here is a snippet of how a typical FastAPI app (`main.py`) looks like:
xxxxxxxxxx
from fastapi import FastAPI
app = FastAPI()
get("/") .
def read_root():
return {"Hello": "World"}
Remember to replace `”main”` and `”app”` in `uvicorn main:app` with your own module and FastAPI application object names respectively. Each project configuration might differ, so it’s crucial to analyze and customize these values according to your specific setup.
Let us know if you need further insights or clarifications, happy to guide you along this journey.While optimizing the performance of FastAPI, you may often encounter a commonly seen error – “Error loading ASGI app. Could not import module ‘API'”. This is typically thrown when FastAPI cannot locate the specific path to your application. Therefore, before getting into performance enhancement, let’s clarify how to deal with this error issue.
FastAPI uses the ASGI (Asynchronous Server Gateway Interface) standard for managing requests and responses. When you run a Uvicorn server instance to serve your FastAPI application, you need to provide the ASGI application instance as an argument. This is usually in the format:
xxxxxxxxxx
<file>:<app>
, where
xxxxxxxxxx
<file>
is the filename containing your FastAPI application, and
xxxxxxxxxx
<app>
is the application instance.
For example, if your file is called main.py and your FastAPI app is instantiated as app:
xxxxxxxxxx
from fastapi import FastAPI
app = FastAPI()
get("/") .
def read_root():
return {"Hello": "World"}
You would refer to it using
xxxxxxxxxx
main:app
when running the Uvicorn server:
xxxxxxxxxx
uvicorn main:app --reload
This error often arises due to one or more of these issues:
- Misnaming or misplacement of your FastAPI application.
- Inappropriate setting of Python environment variables required by your application.
- Error within your actual API code that prevents successful importation.
You should resolve these issues promptly because foundational errors like these can significantly undermine the application’s stability, causing a drastic drop in overall efficiency and hindering reliable performance optimization. Here are some checks you might perform:
- Ensure your FastAPI application instance name is accurately passed to uvicorn. It should be part of a Python executable file in the root project directory.
- Check your PYTHONPATH environment variable is properly set for your environment. This tells Python where to search for modules to import.
- Review your API-related code carefully. Look out for possible syntax errors, missing dependency, broken imports, init leftovers from previous instantiations, etc..
Solving this error could already result in incremental performance improvement. With the underlying issues sorted, let’s dive into unlocking unknown features and tools in FastAPI to optimize performance further. For instance, take advantage of built-in libraries such as:
- Starlette Database Middleware: An asynchronous database package that’s designed for Starlette and compatible with FastAPI. You gain great benefits using this middleware for efficient database handling in your applications.
- Uvicorn server setup with HTTP/2: Enabling HTTP/2 in your Uvicorn server brings about browser compatibility, lower latency, and potential for priority request routing.
- FastAPI Async Tasks: Freshen up knowledge on proper use of async functions and endpoints, which fully benefits FastAPI’s asynchronous power.
Remember that optimal performance isn’t just about faster response times but also streamlined architecture, efficient resource management, and accessible testing and debugging tools. The above enhancements can lead to a well-performing FastAPI application with less downtime, better responsiveness, easier extension, and scalability when your user base expands.
Sources:
Uvicorn,
FastAPI Documentation,
Starlette MiddlewareSo, you’re dealing with an issue where FastAPI throws an error: “Error Loading ASGI App. Could not import module ‘API’.” This error can be a real stumper and may put a wrench in your coding progress for a time, causing understandable frustration and delay.
This error usually means that the
xxxxxxxxxx
ASGI
server (such as Uvicorn or Starlette) could not find and load the specified Python module designated as `API`. It’s almost like trying to read a book that isn’t even in the library – if it’s not there, it can’t be read, and thus, an error occurs.
Here are some potential root causes of error:
- Poorly-defined application structure: There might be something awry within the setup of your project’s directories and files, causing confusion when the server attempts to locate the needed ‘API’ module.
- Misconfigured ASGI application: When configuring the main ASGI application (usually done in
xxxxxxxxxx
111main.py
file), mistakes such as spelling errors, misnamed variable, or using wrong application instance might root from this issue.
- Issues with environment or dependencies: If dependencies aren’t correctly installed or environmental variables aren’t properly configured, they could be the culprit behind the error.
The solution tends to rely on identification and correction of whichever of these underlying issues is causing the problem. Here’s a simple example of how this error might look in code:
xxxxxxxxxx
from fastapi import FastAPI
app = FastApp()
get("/") .
def read_root():
return {"Hello": "World"}
By examining the import statements, configuration, and project’s structure thoroughly, you can typically diagnose where the issue lies and correct it appropriately.
In summary, the FastAPI error message “Error Loading ASGI Application. Could not import module ‘API'” signals an inability to locate and load a specified Python module. You should investigate the possible reasons, which range from malformed project structure, wrong ASGI app configuration, or related dependencies/environmental issues. Once you identify the cause lingering behind your plight, you’ll be only steps away from overcoming this obstacle jamming your way.
Keep these insights as guideposts along your journey to overhaul this common stumbling block thrown by FastAPI, and remember, every error is a new chance to explore and learn more about your code!