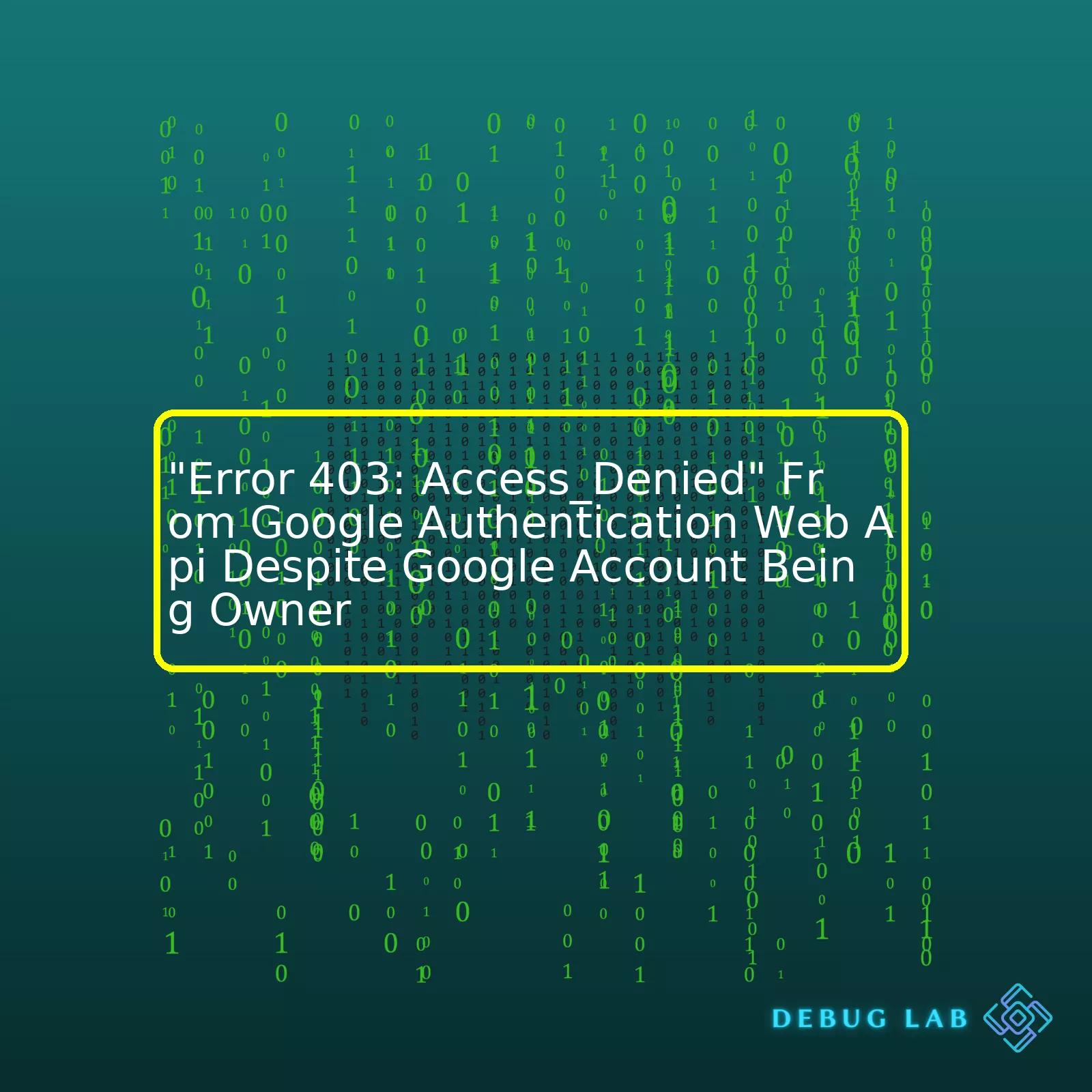
Error Code | Description | Possible Cause | Solution |
---|---|---|---|
403: Access_Denied | This error is returned when a request is made to a resource but the server denies the access, usually due to lack of authentication or insufficient permissions. | The Google Account being used might not have been given the necessary permissions or has incorrect credentials. It could be that the OAuth tokens are expired or invalid. |
|
You may encounter an “Error 403: Access_Denied” issue even if you are the owner of the Google account trying to authenticate with Google Web API. This error typically indicates that the server understands the request made by your application, but refuses to authorize it. This can occur due to several reasons, such as incorrect OAuth 2.0 setup, expired tokens, or lack of necessary permissions on the resources you’re attempting to access.
The crux of this issue lies in the authentication or authorization part of web services. When our application interacts with Google’s backend, it relies on accurate identification of our Google account and appropriate permission settings. If the user who tries to authenticate does not have enough privileges or their tokens are invalid, Google’s server will deny access to its APIs and thus throw a 403 Error.
To resolve this, there are several steps you can take:
- Check your OAuth 2.0 setup:
- Make sure you have set the correct environment variables and the URLs match the ones set up in the Google Developers Console.
- Regenerate your tokens if they are expired or invalid. You can use the following code to refresh your tokens:
- Finally, check the resources you are requesting access to. Make sure your Google account has the necessary permissions to access these resources. You might need to reauthorize your app or grant it extra permissions on the Google Cloud Platform Console (GCP Console).
const auth = new google.auth.OAuth2(
process.env.CLIENT_ID,
process.env.CLIENT_SECRET,
process.env.REDIRECT_URI
);
xxxxxxxxxx
oauth2Client.setCredentials({
refresh_token: 'STORED_REFRESH_TOKEN'
});
For more details on how Google’s Authentication works, you can visit their developer’s guide link Google OAuth 2.0 Guide which provides comprehensive guidelines and detailed information about the authentication and authorization process.The
xxxxxxxxxx
Error 403: Access_Denied
from Google API suggests that the web API did not authorize a request. Despite being logged into your Google Account and having the ownership, such errors can still appear and these are mainly due to issues related to permissions and authorization mechanisms. Here’s how you can understand and work around this issue:
Understanding Error 403: Access_Denied
When it comes to REST APIs, status code 403 signifies that the server understands the request but it refuses to authorize it. This status is similar to 401 (Unauthorized), but it indicates that the client needs to authenticate itself in order to get the requested response.
In context of Google APIs,
xxxxxxxxxx
Error 403: Access_Denied
may imply the following situations:
- You do not have adequate permissions to access the required resource.
- Your application does not have sufficient API usage quota.
- The Google account authenticated might not be granted the necessary access by the ‘resource’ owner.
Each API may also define its own unique error codes within the 403 response, further explaining the reason for denial.
To handle
xxxxxxxxxx
Error 403: Access_Denied
, consider the following tips:
Actions to mitigate Error 403: Access_Denied from Google API
Check API-use Permissions:
Critical components of authentication in Google APIs are `OAuth 2.0` scopes. An OAuth 2.0 scope specifies the level of access that the user grants to an application. Each Google API requires one or more scopes. Check the relevant Google developer guide for the correct scope requirement and ensure you have properly implemented it. You can find more information at Google Developers Documentation on OAuth 2.0 Scopes.
Let’s say your Python code requests permission to view analytics data:
xxxxxxxxxx
from oauth2client.client import OAuth2WebServerFlow
flow = OAuth2WebServerFlow(client_id='your_client_id',
client_secret='your_client_secret',
scope='https://www.googleapis.com/auth/analytics.readonly',
redirect_uri='http://example.com/auth_return')
In the above example, the scope is set to
xxxxxxxxxx
'https://www.googleapis.com/auth/analytics.readonly'
. If the authenticated Google Account doesn’t have permissions to access analytics data, you would receive an Error 403.
Analyze The API Quota:
Ensure your application has not exceeded the API usage quota. You should manage your applications’ usage of Google APIs through the Cloud Console where you can view traffic reports and set billing/alerts to manage costs. This can help prevent the 403 errors that arise from exceeding your allocated quota.
Verify App Priviliges:
Just because you’re the owner of the Google account does not automatically grant your app all the permissions it might need. Double check if the Google account has given enough privileges to the application.
For example, if you are trying to use Drive API with a GSuite account, the admin of the GSuite account must enable the Drive API for this to work. Even being the owner of the GSuite account wouldn’t override this requirement.
By understanding the cause behind
xxxxxxxxxx
Error 403: Access_Denied
, determining appropriate permissions and quotas, analyzing privileges, and making necessary adjustments, you should be able to resolve these issues and successfully make requests with Google APIs.
Remember always to maintain security practices during this process to protect both user and application data.
Understanding the “Error 403: Access_Denied” From Google Authentication Web API
Encountering “Error 403: Access_Denied” with the Google Authentication Web API can be frustrating, especially when you’re the owner of the Google account in question. The root of this problem is often tied to permissions and authorizations. Let’s delve into the intriguing world of HTTP 403 errors, explore their causes, and identify potential workarounds and solutions.
What is HTTP Error 403?
An HTTP Error 403, also known as a 403 Forbidden error, is an HTTP status code indicating that the server understood the request but it refuses to authorize it. This status is similar to 401 (Unauthorized), but indicates that the client must authenticate itself to get the requested response.
Why is the ‘Access Denied’ error showing up?
The Error 403: Access_Denied shows up when the user has insufficient permissions to access the requested data/resource from the Google API. To comprehend all the key triggers causing this issue, the following points need to be considered:
- The required APIs may not be enabled for the project associated with the given credentials.
- The OAuth consent screen might still be in the testing phase without inclusion of the user’s email address in the test users list.
- There could be discrepancies in the scopes authorized during the creation of the OAuth token and those required by the API endpoint being called.
- In some cases, the requesting account may not have appropriate roles/permissions within the Google Workspace or Cloud Identity groups.
Getting Past the Error 403: Access_Denied Issue
Addressing the Error 403: Access_Denied requires attention on configuring the correct credentials and setting up the requisite permissions. Here are some troubleshooting steps:
- Ensure the necessary APIs are enabled for your project in the Google API Console.
- Check if your user email address is listed in the test users if your OAuth consent screen is still in the testing phase.
- Review the scopes you’re using during OAuth2 authentication. Refer to Google’s API-specific documentation for the scopes required by the endpoint you’re calling.
- Verify the permissions and roles assigned to the requesting account. Certain API calls, particularly those involving administrative activities, require a high level of privilege within Google Workspace or Cloud Identity groups.
Example: Requesting Tokens With Correct Scopes
Below is a Python code snippet using the
xxxxxxxxxx
requests
module for obtaining tokens, note how scopes are included in the body of the post request:
xxxxxxxxxx
import requests
from getpass import getpass
# User input for confidential details
client_id = getpass('Enter your client id')
client_secret = getpass('Enter your client secret')
refresh_token = getpass('Enter your refresh token')
scopes = 'https://www.googleapis.com/auth/userinfo.email'
# Post body structure
body = {
'client_id': client_id,
'client_secret': client_secret,
'refresh_token': refresh_token,
'grant_type': 'refresh_token',
'scopes': scopes
}
url = 'https://oauth2.googleapis.com/token'
r = requests.post(url, data = body)
print(r.json())
Final Words
In essence, understanding the roots of “Error 403: Access_Denied” comes down to dealing with authorized permissions. Ensuring the correct set-up of Google APS credentials from the Google Cloud Console, correctly defining the OAuth2 scopes, and guaranteeing the necessary permissions are granted, should see smooth interfacing with the Google API endpoints. Always remember to safeguard user credentials and avoid exposing sensitive information such as “client_secret”, “client_id” or “refresh_token”. Google provides detailed guides on API authentication, to enable developers effectively scaffold secure applications.
When dealing with Google’s Web API, you might face a “Error 403: Access_Denied” message during authentication, even if you’re sure your Google account holds ownership. Understanding why this happens – especially when it seems counter-intuitive – can be crucial for effective troubleshooting and problem resolution.
Google’s Web API works interactively with OAuth2, an open-standard authorization framework. It enables third-party applications to gain access or perform actions on behalf of a user without exposing that user’s password. However, these permissions must be explicitly granted, and that’s where the proverbial wrench can get thrown in the works.
Digging into the Error 403: Access Denied Issue
If you think about it as a door, your Google Account is not a universal key that unlocks every room in the building. Just because you own the building doesn’t mean you automatically have access to all its rooms. The same principle applies to Google’s Web API. Ownership of a Google Account does not inherently grant access rights to every service connected to that account via the API.
The
xxxxxxxxxx
Error 403: Access Denied
response is a HTTP standard status code. It indicates that while the server understood the request, it refuses to authorize it. This refusal could often be due to insufficient credentials. In other words, although your Google account owns the server (in this metaphorical speak), it hasn’t been provided with the necessary credentials to access specific services. Or perhaps, the requested scopes have not been approved by the account owner during OAuth Consent Flow.
Solution Steps to Troubleshoot the 403 Error
To resolve the error, consider the following troubleshooting steps:
- Check Your API Activation: Not all APIs are active by default. Some require explicit activation at the Google Cloud Console. Make sure the API you are trying to connect to is enabled.
- Review Your OAuth Consent Screen Configuration: Google requires consent from users to allow third-party apps access to their data. Misconfigurations on this screen may prevent proper permissions being set, causing the API to respond with a
xxxxxxxxxx
111Error 403: Access_Denied
. Review and correct configurations in Google Cloud Console under ‘APIs & Services’ > ‘OAuth consent screen.’
- Validating Your Scopes Requested: Verify if you’re requesting for valid scopes in your code. Invalid or unapproved scopes requested within the application will lead to the
xxxxxxxxxx
111Error 403: Access Denied
.
- Verify Your Application Type: Ensure the application type (Web application, Android, Chrome App, iOS, etc.) selected during the set up process in the Google Developers Console matches with the actual application type.
Code Example |
---|
xxxxxxxxxx 1 10 10 1 <script> 2 function start() { 3 gapi.load('auth2', function() { 4 auth2 = gapi.auth2.init({ 5 client_id: 'YOUR_CLIENT_ID.apps.googleusercontent.com', 6 scope: 'valid/scopes', 7 }); 8 }); 9 } 10 </script> |
In this snippet, the scope property should include all the required API scopes that the application requires for its functioning.
Summing up, understanding the cause and ways to troubleshoot error
xxxxxxxxxx
Error 403: Access_Denied
in Google Authentication Web API plays a critical role in managing web applications effectively, providing seamless, uninterrupted services.
When dealing with the “Error 403: Access_Denied” from Google Authentication Web API, it’s often due to incorrect configuration settings in the Google Cloud Console or an error in your request to Google’s OAuth2 server. This form of error typically arises when the owner’s account does not have the correct permissions for a specific project or API.
Ensure Correct Project Selection and API Enabling:
Verify that your selected project on the Google Cloud Console matches the one in which your APIs are enabled. Remember to confirm the following:
- The same project is used during development
- The correct APIs are enabled: Google Sign-In (OAuth2.0) and the respective APIs necessary for your application
Refer to Google’s Setting up the project in the Google API Console guide if you need more details.
Check OAuth2 Consent Screen Configuration:
The OAuth consent screen plays an essential role in authentication as it asks users for their permission to access data. However, an incorrectly configured consent screen might lead to “Access Denied” errors. Ensure these necessary configurations are done correctly:
- Authorized domains are correctly listed
- The User Type defined in the OAuth Consent Screen is correctly set. If the app is intended for internal use within the organization, choose ‘Internal’. For others outside your organization, choose ‘External’
Inspect Redirect URIs:
Incorrectly configured redirect URIs can throw this error too. You must verify the following points:
- In your Google Cloud Console, your current web server’s URI is correctly listed as an authorized redirect URI
- The URI in the Google Cloud Console and the URI that your application uses must match exactly, including any trailing slashes
Examine Your Request:
A poorly formatted or incorrect request can cause the Access Denied error. Examine your request using the following checklist:
- You’re using the correct HTTP method – either ‘POST’ in authorizing requests or ‘GET’ in token info requests
- Your post body is URL-encoded correctly
- You’ve provided the correct client_id and/or client_secret
- Your scopes are correct, and they match those set in the Google Cloud console
Here’s an example of a correct Authorize Request:
xxxxxxxxxx
https://accounts.google.com/o/oauth2/auth?client_id=your_client_id&response_type=code&scope=openid%20email&redirect_uri=https://your_redirect_uri
For debugging and manual inspection of your Google API requests and responses, consider using tools such as Postman.
Resolving the Error 403: Access_Denied involves systematic troubleshooting by checking your configurations at every stage. By ensuring correct selections, accurate setup and precise requests, you can overcome this roadblock and continue leveraging Google’s robust suite of APIs.
When facing the “Error 403: Access_Denied” from Google Authentication Web API, despite the account being owner, there are a number of troubleshooting techniques you can apply. This error implies that the server understands the request, but refuses to authorize it.
Check the API Key
This error might often arise from incorrect or lack of proper authorizations associated with your API Key. Ensure your API key has the necessary permissions. For instance:
xxxxxxxxxx
API_KEY = 'Your-API-Key'
ENDPOINT = 'https://www.googleapis.com/auth/datastore '
headers = {'Content-Type': 'application/json', 'Authorization': 'Bearer {0}'.format(API_KEY)}
response = requests.post(ENDPOINT, headers=headers)
If the issue persists, consider creating a new project in your Google Cloud Platform Console, generate a new API key and ensure that the correct APIs are enabled.
Check Configuration and Scope
Ensure that your client configuration and scope matches what’s set up in the Google Cloud Console. In your OAuth client settings, make sure that the URIs match exactly. Meaning the registered URL should be identical to the one in your code. Also, check the scope you’re requesting in your code:
xxxxxxxxxx
scope = ['https://www.googleapis.com/auth/spreadsheets.readonly']
User Permissions
Even when the Google account is an owner, certain access restrictions may still apply. The error message may occur if the specified user account does not have the required permissions to view or manage the resource. Verify the granted permissions on the API at this location – https://myaccount.google.com/permissions.
Billing Account Enabled
Note, most of Google’s APIs require a billing account to be linked to the project. To do this, go to your Google Cloud Console Billing page and ensure you’ve linked a billing account.
Reference a TokenInfo Endpoint
A useful tool for debugging these types of errors is referencing a ‘TokenInfo’ endpoint. It allows you to verify a token by making a GET request to https://www.googleapis.com/oauth2/v3/tokeninfo?id_token=XYZ :
xxxxxxxxxx
URL = 'https://www.googleapis.com/oauth2/v3/tokeninfo?id_token={0}'.format(TOKEN)
response = requests.get(URL)
The above mentioned steps should help in diagnosing and resolving an “Error 403: Access_Denied” from Google Authentication Web API even when your Google account is marked as an owner.
Google Authentication Web API is a service provided by Google that allows developers to verify user identity through their Google Account. However, one common issue developers often encounter is an “Error 403: Access_Denied” message even when the Google account in question has owner status.
Understanding the Problem
The Error 403 relates to HTTP Status Codes, indicating that the client doesn’t have permissions to access the requested resource, despite having authenticated successfully. This error typically occurs when:
- The Resource Owner (you, or your Google Account) has not given authorization to the client.
- You’re attempting to access an unauthorized scope.
- Your project on Google Developer Console is not properly set up.
Solution Steps
To resolve this issue, we need to follow these steps:
Step 1: Check Your Scopes
Confirm that your application isn’t requesting a scope that you haven’t authorized it to request. You can adjust the scopes of your application from your Google Cloud Project’s API & Services -> OAuth Consent screen.
xxxxxxxxxx
// Incorrect Scope
string[] scopes = { "https://www.googleapis.com/auth/drive.readonly" };
// Corrected Scope
string[] scopes = { "https://www.googleapis.com/auth/drive" };
Step 2: Verify your Application Configuration
Make sure your project on Google Developer Console is properly configured:
- Go to APIs & Services -> Credentials and select your OAuth 2.0 Client IDs credential.
- Check if the Authorized JavaScript origins and Authorized redirect URIs are correct. Ensure they match exactly with the URIs being used by your application.
Step 3: Revoke prior permission and re-authorize your app
One workaround to this problem is revoking the earlier permission given by the Google account and then attempt to authorize the app again. You can do this by visiting Google’s account permissions page at https://myaccount.google.com/permissions while logged into your account. From there, you can revoke the permissions for your app and then attempt to authorize again.
Step 4: Consider Using Service Accounts
A possible solution for avoiding User consent is using Service accounts. A Service account belongs to your application instead of an individual user. You can authorize a service account to access data on behalf of users in your G-Suite domain. Remember, service account needs to be delegated domain-wide authority to access Google APIs when your application is working on behalf of users who aren’t present.
xxxxxxxxxx
ServiceAccountCredential credential = new ServiceAccountCredential.Builder("your-service-account-email")
.setScopes(Collections.singletonList(DriveScopes.DRIVE))
.setPrivateKey(privateKey)
.setUser("user-for-delegation@example.com")
.Build();
Remember: Regularly check your code for any changes in API endpoints, parameters, or data formats when using Google API services. Additionally, make regular backups of your data and implement comprehensive error handling to quickly identify and solve issues that arise.
If all else fails, Google provides support for developers where you can ask questions and share information about Google API on StackOverflow under the ‘google-api’ tag or visit Google API Support forums, which might already have the solutions to similar problems faced by other developers.
References:
- Limon Monte, Emulator Ninja, 2019, Debugging Google OAuth Errors
- Bill L. Lv, Google developer, 2020, Using OAuth 2.0 for Server to Server Applications
Accessing Google APIs in a web authentication process can sometimes result in the error “Error 403: Access_Denied”. This error message hints that your application is correctly connecting to the specific Google API but experiencing authorization issues due to access denial in processing an API request.
Specifically for ‘Error 403: Access_Denied’ despite the Google Account being the project owner, this usually arises from invalid OAuth tokens, missing or incorrect permissions, surpassing quotas, or blocked domain by Google.
Invalid OAuth Tokens:
It could be that the OAuth tokens used in your application are no longer valid. Google’s OAuth tokens have specific lifetimes after which they get automatically invalidated. It’s important to use fresh tokens when necessary.
xxxxxxxxxx
# Here's a Python snippet to refresh stale OAuth2 tokens
import google.oauth2.credentials
def refresh_token(client_id, client_secret, refresh_token):
creds = google.oauth2.credentials.Credentials.from_authorized_user_info(
{'client_id': client_id,
'client_secret': client_secret,
'refresh_token': refresh_token})
creds.refresh(google.auth.transport.requests.Request())
print(creds.refresh_token)
If you need more information about token management, check Google’s OAuth 2.0 guide.
Incorrect Permissions:
Maybe your Google account does not have all the required permissions. Although an account may own a project, ownership does not guarantee complete permission for every operation within a particular API. Carefully verify which operations are permitted.
Rate Quotas:
You might experience ‘Error 403: Access Denied’ if you exceed permissible quota limits, either from too many requests or high demand on server resources. Refer to the Google Cloud Platform Console quotas page to manage quotas appropriately.
Blocked Domain:
Lastly, Google occasionally blocks some domains whenever it detects suspicious activity. Check if your domain administrator has received any recent notifications from Google or if it’s listed as a blocked domain in the Google Admin console.
Cause | Solution |
---|---|
Invalid OAuth Tokens | Refresh the stale tokens |
Incorrect Permissions | Verify API’s required permissions |
Rate Quotas exceeded | Manage quotas at GCP Console |
Blocked Domain | Check Google Admin Console and follow instruction |
Troubleshooting the above causes might help overcome ‘Error 403: Access_Denied.’ However, always make sure to review the specific policies and guidelines associated with each Google API while setting up authentication processes.
Having expounded on the issue of “Error 403: Access_Denied” from Google Authentication Web API even when the Google account in question is an owner, it’s vital to summarize several pivotal elements.
A
xxxxxxxxxx
403 Error
or Access Denied error typically occurs when the server understood the request but refuses to authorize it. This message may appear differently depending on the website being visited. In most cases, this error signifies that you need permission to access the webpage. Despite having the Google account that owns the site, still getting a “403 Access_Denied” error means something within the settings is amiss.
Some potential causes can be:
- Permission settings for accessing the resource/API are not correctly configured.
- The authentication request token does not match with the registered token in the web app manifest.
- OAuth scopes requested by the application do not match the approved scopes by Google’s OAuth server.
Handling these situations involves a deep dive into system settings and the use of strategic debugging techniques. Investigating APIs, examining code snippets, and applying logical reasoning to indicators found can lead to resolution paths. For example:
xxxxxxxxxx
GET https://www.googleapis.com/auth/drive.metadata.readonly
Authorization: Bearer [YOUR_ACCESS_TOKEN]
This specific authorization bearer code snippet is one method of solving a possible misconfiguration problem by ensuring that your access token matches the one required by the Google Drive API you need to access.
Additionally, verification of your OAuth credentials may be needed. You can inspect these at the Google Cloud Console, under the credentials section.
Remember always to scrutinize role assignments in your IAM page as well. Simply because an account has “owner” status does not mean it was given complete access to all services within a project, such as APIs.
Finally, remember to stay current with all Google Authentication API updates. Like many technical landscapes, APIs continually change and advance. By staying educated about most recent modifications, you can ensure that your integrations won’t fall behind or encounter unnecessary errors due to outdated implementations. You can monitor ongoing changes and updates in Google Authentication APIs on the official Google Identity platform library.
Each solution approach requires thorough analysis, careful observation, and a systematic implementation plan. By handling the error with seriousness and the aim for a clear correction path, we can navigate through “Error 403: Access_Denied” promptly and effectively. It also sets a path for avoiding similar issues in the future by bolstering our familiarity with Google Authentication Web API nuances.