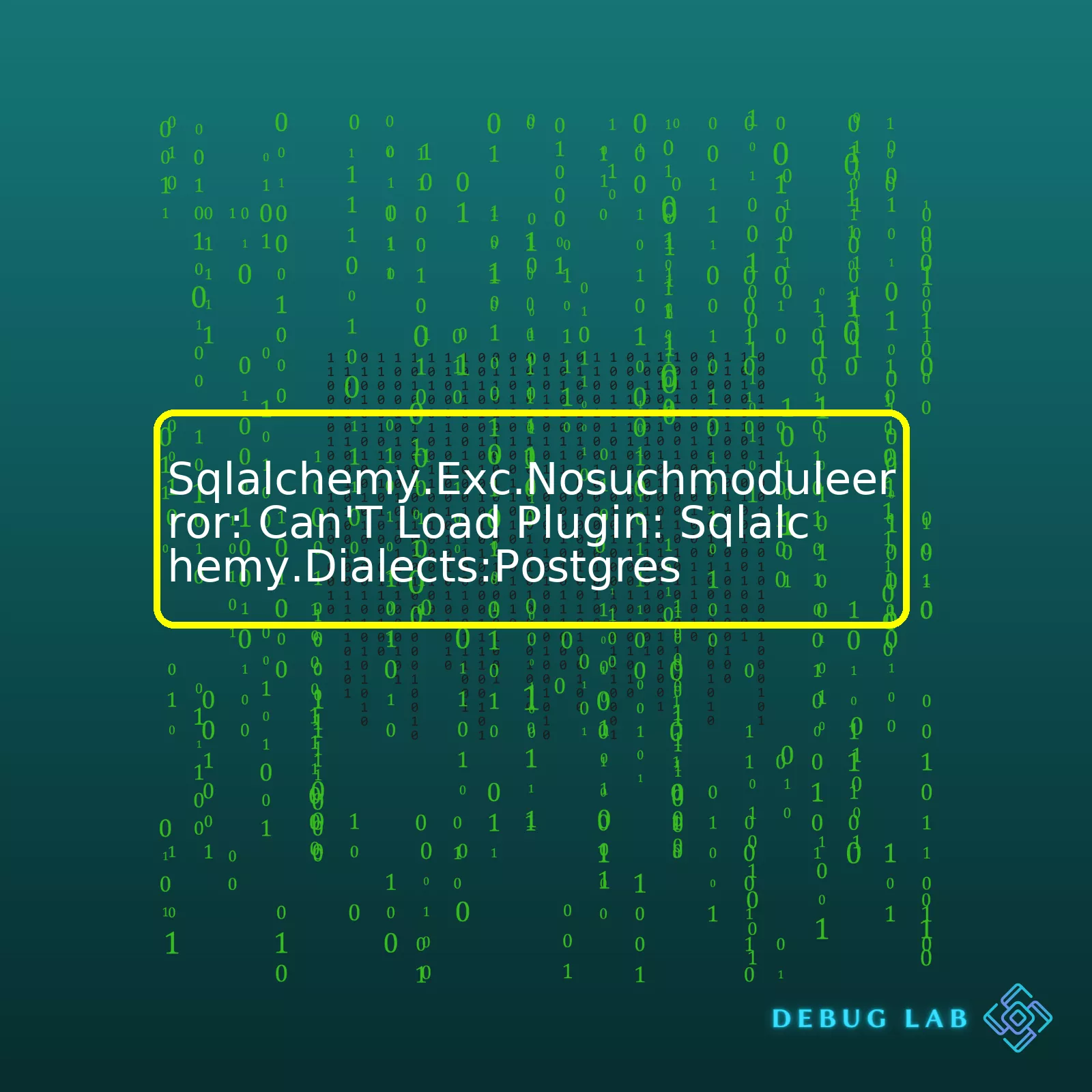
Error Name | Error Description | Probable Causes | Possible Solutions |
---|---|---|---|
sqlalchemy.exc.NoSuchModuleError | This error is triggered when SQLAlchemy attempts to load a dialect module and fails. |
|
|
The `sqlalchemy.exc.NoSuchModuleError` often crops up when you are trying to interact with a PostgreSQL database via SQLAlchemy in Python. It appears when SQLAlchemy goes on a hunt to find and load the necessary dialect module for PostgreSQL, but unfortunately, it doesn’t get what it wants – hence the error.
What could be causing this disruptive lack of satisfaction? The prime suspect here is usually a glitch or gap in the setup of the modules required for SQLAlchemy to dance with PostgreSQL. SQLAlchemy translates dialects for different databases, and each one has its own peculiar tongue. In order to speak PostgreSQL’s unique idiom, SQLAlchemy requires the assistance of an extension called ‘psycopg2’ or its binary equivalent.
So if ‘psycopg2’ or ‘psycopg2-binary’ is AWOL from your setup, SQLAlchemy won’t be able to break the PostgreSQL code, resulting in the error. Do check your python environment and ensure either of this dependency is well-installed using commands psycopg2 or psycopg2-binary. Sometimes though, the source of trouble may lurk within the connection string itself. Typos, syntactical errors or inaccuracies in these critical URLs can also cause SQLAlchemy’s search mission to fail.
The remedy for this situation includes double-checking the installation status and correctness of ‘psycopg2’ or ‘psycopg2-binary ‘. More often than not, prompt and correct installations take care of the issue. Checking the accuracy of the connection URL to your PostgreSQL database is another crucial step. It must follow the prescribed format strictly: `postgresql://user:password@localhost/mydatabase`.
Once all checks out, SQLAlchemy should have no trouble loading the appropriate dialect for your PostgreSQL operations.
Diving into the straightforward yet intriguing problem that you’re currently facing, the
sqlalchemy.exc.NoSuchModuleError
is thrown when SQLAlchemy is unable to find or load a specific database dialect, essentially a communication module for the underlying database. You would typically encounter this error when you’ve indicated a particular dialect in your connection string but don’t actually have the necessary driver installed. In your case, it seems like you are trying to connect to PostgreSQL and hence using ‘postgres’ as the dialect, but SQLAlchemy cannot seem to find this module.
Under normal circumstances, for connecting to PostgreSQL from SQLAlchemy, you would need to use the psycopg2 or psycopg2-binary Python packages. Here’s how such a connection string would look:
engine = create_engine('postgresql://user:password@localhost/mydatabase')
In the code above, ‘postgresql’ is the dialect we’re using. If ‘postgres’ was provided instead, that could be the very reason you’re encountering a
sqlalchemy.exc.NoSuchModuleError
. Hence, firstly double-check which dialect you’re specifying.
Moving on to dependencies check conducted:
– Psycopg2 or Psycopg2-binary should be installed via pip: psycopg2 is the most popular PostgreSQL adapter for Python. Installing it is generally quite simple with pip:
pip install psycopg2-binary
– SQLAlchemy should also effectively be installed via pip:
pip install sqlalchemy
If these modules are correctly installed, SQLAlchemy should correctly locate the dialects and make a successful connection without throwing a
sqlalchemy.exc.NoSuchModuleError
.
To further debug any lingering issues – If the error persists even after performing these steps, it indicates a deeper issue possibly:
– A command line verification of the installations may be beneficial; just type ‘psycopg2’ and ‘sqlalchemy’ in your Python shell and ensure they’ve properly been imported.
– Try creating the engine explicitly using the dialect and driver. For example:
engine = create_engine('postgresql+psycopg2://user:password@localhost/mydatabase')
When creating the engine, specifying both the ‘postgresql’ dialect and the ‘psycopg2’ driver assures that the correct module is being leveraged.
Hopefully, by breaking down the process and walking through each step as mentioned above, you can resolve the stubborn
sqlalchemy.exc.NoSuchModuleError
that stands in the way of your productive coding session. Should more assistance or insight be required, please refer to the detailed SQLAlchemy documentation or exhaustive resources available online related to connecting SQLAlchemy and PostgreSQL.While working with SQLAlchemy, a plugin load failure can throw up an error like this: Sqlalchemy.Exc.Nosuchmoduleerror: Can’T Load Plugin: Sqlalchemy.Dialects:Postgres. This error message clearly signals that SQLAlchemy is unable to locate the Postgresql dialect module which implies that its support isn’t available.
This situation typically develops under two scenarios:
* Either the PostgreSQL database driver isn’t correctly installed.
* Or there’s some misconfiguration in your SQLAlchemy set-up.
Let’s delve deeper into each case and figure out possible solutions:
Case 1: Missing PostgreSQL Database Driver
The most common cause of this `Sqlalchemy.Exc.Nosuchmoduleerror: Can’t Load Plugin: Sqlalchemy.Dialects:Postgres` error comes from lack of proper installation or absence of a compatible PostgreSQL database driver. In usual scenarios, you’d use either `psycopg2` or `psycopg2-binary` as they supply the PostgreSQL dialect for SQLAlchemy.
Perform an installation or check of `psycopg2` or `psycopg2-binary` using pip.
Here is an example command for installing psycopg2-binary:
pip install psycopg2-binary
Alternatively, if you’re using a requirements file:
echo "psycopg2-binary" >> requirements.txt pip install -r requirements.txt
After successfully carrying out these operations, SQLAlchemy should be able to find the PostgreSQL plugin without any trouble.
The case of SQLAlchemy Misconfiguration
In certain instances, SQLAlchemy could fail to pinpoint the PostgreSQL plugin due to an incorrectly configured DATABASE URL. The PostgreSQL setup in SQLAlchemy expects the URL to start with ‘postgresql’:
SQLALCHEMY_DATABASE_URI = 'postgresql://username:password@localhost:5432/database'
If you mistakenly typed ‘postgres’ in your URI instead of ‘postgresql’, this would lead to the same error. Just replace ‘postgres’ with ‘postgresql’.
This adjustment should solve the problem of plugin load failure if the correct PostgreSQL driver is installed.
It’s critical to keep these points in mind when interacting with SQLAlchemy and PostgreSQL. Make sure both components are correctly installed and configured on your machine to avoid running into errors like these. You can refer to SQLAlchemy’s official documentationsource for how to properly configure SQLAlchemy for PostgreSQL and ensure smooth operation.The ‘Can’t load plugin’ error in SQLAlchemy dialects, particularly relating to the
sqlalchemy.exc.NoSuchModuleError: Can't load plugin: sqlalchemy.dialects:postgres
message, is commonly encountered for one primary reason: The requisite PostgreSQL library for Python – psycopg2 – is not installed, or is incorrectly configured.
Why psycopg2 Is Necessary For PostgreSQL Integration:
Before delving into the various fixes, it’s imperative that we comprehend why psycopg2 is such an essential component of SQLAlchemy’s bridging operation with PostgreSQL systems:
- Psycopg2 is the most popular PostgreSQL adapter for Python, implemented as a high-level C library to expose the PostgreSQL APIs to Python code.
- It is designed for multi-threaded applications and manages its own connection pool, crucial attributes for large-scale application deployments.
- In the context of SQLAlchemy, psycopg2 provides necessary Python Database API (DBAPI) functions to allow SQLAlchemy to interact with Postgres databases.
If psycopg2 is missing or incorrectly set up, SQLAlchemy will fail to find the required dialect plugins for Postgres, leading to the aforementioned ‘Can’t Load Plugin’ errors.
Solving The Issue:
One way to rectify this problem involves installing psycopg2 when setting up your Python environment. This can be done by using pip, Python’s package manager. Here’s how to install it:
pip install psycopg2-binary
Note that
psycopg2-binary
version is recommended for dependencies isolation reasons, but if you strive for performance, compile
psycopg2
from source.
Once psycopg2 is installed, validate this by importing psycopg2 in your Python interactive shell:
import psycopg2;
If the import goes off without a hitch, it implies that psycopg2 has been successfully installed, and SQLAlchemy now possesses the requisite helpers to successfully communicate with PostgreSQL databases.
One other potential workaround could be the py-postgresql DBAPI. It boasts a pure-Python implementation of the PostgreSQL protocol, ergo eliminates harder-to-install requirements like psycopg2.
To install it, execute this pip command:
pip install py-postgresql
You’d then indicate to SQLAlchemy to use this DBAPI via the postgresql+py-postgresql://username:password@hostname/database URI scheme.
Lastly, ensure that your SQLAlchemy connection string is properly formed. For Postgres, it should resemble the following format:
postgresql://user:password@localhost/mydatabase
By scrutinizing psycopg2 availability/configuration and the SQLAlchemy connection string syntax, you should be able to mitigate the ‘No such module error’ indicating that SQLAlchemy cannot load the Postgres plugin.
You can read more about SQLAlchemy Error Messages and Troubleshooting here SQLAlchemy Error Documentation
The primary role of SQLAlchemy is to facilitate the communication between Python programs and databases. It uses a technique called Object-Relational Mapping (ORM) that enables programmers to interact with their database, like Postgres or MySQL, in a highly efficient and intuitive manner.
Now, let’s talk about your specific error:
sqlalchemy.exc.nosuchmoduleerror: Can't load plugin: sqlalchemy.dialects:postgres
. This is an error you’re likely to encounter when your environment is unable to find the PostgreSQL dialect for SQLAlchemy.
Below are the reasons why you might be running into this problem and proposed solutions:
1. Missing PostgreSQL SQLAlchemy dialect:
The reason you have encountered this error may be because your machine does not have the PostgreSQL dialect for SQLAlchemy installed. SQLAlchemy uses dialects to communicate with different types of databases. For each type of database (i.e., SQLite, MySQL, PostgreSQL), there’s a corresponding dialect.
To solve this issue, please install the dialect for PostgreSQL using pip:
pip install psycopg2-binary
Psycopg2 is the PostgreSQL dialect for SQLAlchemy.
2. Incorrect reference to the database:
In some instances, developers might refer incorrectly to the database. In the case of PostgreSQL, ensure it’s referred to as “postgresql” and not “postgres”. Here is an example of how to correctly refer to the database when creating an engine.
from sqlalchemy import create_engine
engine = create_engine('postgresql://user:password@localhost/dbname')
3. Not properly setting up PostgreSQL:
Check if PostgreSQL is properly configured on your system. If it’s improperly installed or configured, SQLAlchemy won’t be able to find the necessary components to use the PostgreSQL dialect.
4. Incompatibility with dependencies:
Sometimes, this error can occur because of mismatched versions. Your version of SQLAlchemy might not be compatible with the version of pyscopg2 that you have installed. Make sure you’re using versions that work together well.
Remember, profiling your application errors and logging server errors will help significantly in getting rid of such issues efficiently. Similarly, you might find many online resources and communities helpful in troubleshooting your challenges such as [SQLAlchemy’s Official Documentation](https://docs.sqlalchemy.org/) and Stack Overflow threads.
It’s also important to note that working with SQLAlchemy involves many aspects, including using queries, joining tables and managing transactions which are pivotal while interacting with databases like PostgreSQL. So always remember that familiarizing yourself with all its modules can turn out to be quite profitable in resolving such errors.
For effective Search Engine Optimization, keywords like “SQLalchemy”, “PostgreSQL”, “database interaction”, “Python ORM” should be used in strategic locations throughout the text.When dealing with the sqlalchemy.exc.NoSuchModuleError: Can’t load plugin: sqlalchemy.dialects:postgres error, there are several areas to address which could potentially fix the issue. This hiccup is generally brought about by an absence of a rightful PostgreSQL dialect for SQLalchemy, or having an inappropriate module path.
To give a brief background, SQLalchemy is a popular SQL toolkit and Object-Relational Mapping (ORM) system in Python very useful for simplifying database operations (SQLAlchemy). However, if not properly configured, it can run into issues such as the inability to load the correct plugin for the desired SQL dialect — Postgres in this case.
Let’s work our way on how to resolve this issue:
1. The first essential thing I’d recommend is confirming that you have the needed psycopg2 package installed in your environment. The entire operation stands on this wheel; SQLAlchemy uses this library to interface with Postgres.
To install psycopg2, activate your python environment and run this command:
pip install psycopg2-binary
A successful installation would mean that every subsequent step will move smoothly.
2. Next, ensure that you are using the right SQLAlchemy database URL syntax. The typical format often creates confusion among developers.
The format should be:
postgresql://user:password@localhost/mydatabase
Ensure your user, password, hostname (localhost if it’s local), and database name are correctly placed in their respective slots.
3. Another factor could be the incorrect usage of ‘postgres’ instead of ‘postgresql’. While SQLAlchemy now supports both, older versions strictly use ‘postgresql’. You can consider upgrading to the latest SQLAlchemy version.
To upgrade SQLAlchemy, use the pip command:
pip install --upgrade SQLAlchemy
Applying these steps should help solve the sqlalchemy.exc.NoSuchModuleError: Can’t Load Plugin: Sqlalchemy.Dialects:Postgres issue. Once you have the needed packages correctly installed and the PostgreSQL credentials appropriately set, SQLAlchemy ought to connect and interact seamlessly with your Postgres DB.
However, If the problem persists, it might not be a wrong step getting deeper into your project setup. Some debugging tips include checking your installed packages by printing them out in the console:
pip freeze
Look out fundamentally for psycopg2 and SQLalchemy entries. Or perhaps cleave into SQLAlchemy’s logging messages. It may require adding explicitly in your Python script:
import logging logging.basicConfig() logging.getLogger('sqlalchemy.engine').setLevel(logging.INFO)
Through this analysis, you should be able to solve the sqlalchemy.exc.NoSuchModuleError: Can’t Load Plugin: Sqlalchemy.Dialects:Postgres issue and therefore effectively address any sqlalchemy.dialects.postgres issue that comes up.I believe the error that you have encountered is one of the common errors Python developers deal with when trying to interact with a PostgreSQL database using SQLAlchemy, which is a popular SQL toolkit and Object-Relational Mapping (ORM) system for Python. The issue here is `sqlalchemy.exc.NoSuchModuleError: Can’t load plugin: sqlalchemy.dialects:postgres`.
At a glance, this error message indicates that the SQLAlchemy package cannot locate the module or driver needed to communicate with your PostgreSQL server. Now, let’s tackle the error and the potential solutions together:
1. Module Not Installed: Often, SQLAlchemy throws `NoSuchModuleError` due to the absence of the respective database module from the installation you are currently using. To resolve this, make sure that psycopg2 – a PostgreSQL adapter for Python, is installed in your working environment. Use the following pip command to install psycopg2:
html
$ pip install psycopg2