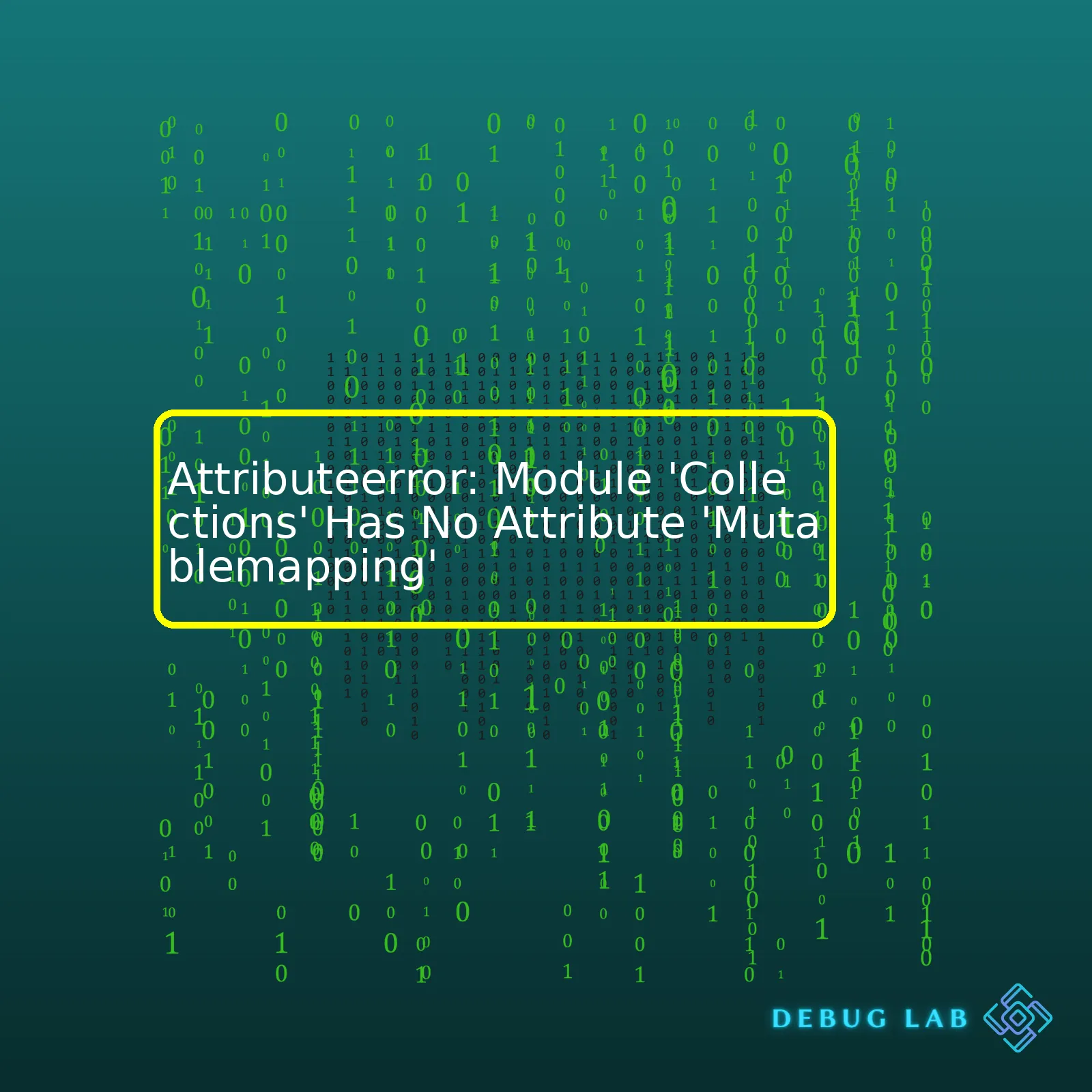
Error type | Description | Causes | Solution |
---|---|---|---|
AttributeError: Module ‘collections’ has no attribute ‘MutableMapping’ | This error explains about the unsuccessful attempt to access the ‘MutableMapping’ attribute from the ‘collections’ module. | Using an outdated Python version or having a conflict in names between your script and the actual ‘collections’ module could cause this error | Upgrade to more recent Python versions will most likely solve this issue. If not, checking for naming conflicts is a good place to start. |
The
AttributeError: Module 'Collections' Has No Attribute 'MutableMapping'
is a common error encountered when there’s been an unsuccessful attempt to access the ‘MutableMapping’ attribute from the ‘collections’ module. This typically indicates that you’re either using an outdated version of Python where the attribute no longer exists in the collections module or there’s been a naming conflict, i.e., your Python file or module is named ‘collections’, hence causing confusion with the built-in Python ‘collections’.
Upgrading to a newer Python version often resolves the problem. However, if a new Python version isn’t compatible with other dependencies in your project, then renaming your Python file or module should do the trick. Make sure to check other files and scripts across your project for potential naming conflicts as well just to ensure successful execution.
For instance, instead of:
xxxxxxxxxx
from collections import MutableMapping
You can use:
xxxxxxxxxx
from collections.abc import MutableMapping
This imports the MutableMapping from the correct location in the collections module which is now within abc, a sub-module within collections. Here it’s important to note if you’re running Python 3.8 and above, the ‘MutableMapping’ has been moved to the sub-package collections.abc (Python Doc Ref). So, ensure you import the right module from the right package.
Sure, I am excited to dive deep into the AttributeError in Python with exclusive focus on the error: Attributeerror: Module ‘Collections’ Has No Attribute ‘Mutablemapping’.
AttributeError in Python
Python is an object-oriented programming language and it defines classes to represent different types of functionality. Each class has attributes which define their characteristics, and methods which define the actions they can perform.
When you try to access an attribute that the object doesn’t have, Python raises an AttributeError. This is a very common error that programmers face while working with classes and objects in Python.
Comprehending this within the spectrum of
xxxxxxxxxx
Attributeerror: Module 'collections' has no attribute 'MutableMapping'
. Sometimes, you might import the module collections intending to use its MutableMapping type, but end up getting the error.
Understanding ‘collections’ module and ‘MutableMapping’
In Python’s standard library, there exists a module called
xxxxxxxxxx
collections
which houses various high-performance container datatypes. One such datatype under collection is MutableMapping.
The reason for having the Immutable and Mutable data types is, as some operations like adding key/value pairs are only supported by mutable mapping, whereas some other operations do not modify the mapping.
The appearance of the Error
If you’re getting the aforementioned error, it implies that Python cannot locate the
xxxxxxxxxx
MutableMapping
attribute within the
xxxxxxxxxx
collections
module. Here’s how an instance of this error occurring might look:
xxxxxxxxxx
from collections import MutableMapping
…raises:
xxxxxxxxxx
AttributeError: module 'collections' has no attribute 'MutableMapping'
A leading cause behind this issue could be your Python version. From Python 3.3 onwards,
xxxxxxxxxx
MutableMapping
(along with others) was moved to
xxxxxxxxxx
collections.abc
.
Here’s how you would import MutableMapping in a Python 3.3+ environment:
xxxxxxxxxx
from collections.abc import MutableMapping
Finding a Solution
Seeing this error doesn’t mean something is wrong with your code, rather it’s a compatibility issue with your existing Python version. There are two methods through which we can rectify this error:
– Update the code to match your Python version: If you’re using Python 3.3+, try changing the import statement to refer to the abc submodule.
xxxxxxxxxx
from collections.abc import MutableMapping
– Use try/except system to handle both cases: To write a code that supports older & newer versions of Python simultaneously, you could import MutableMapping from collections and, if it fails, then import from collections.abc instead.
xxxxxxxxxx
try:
from collections import MutableMapping
except ImportError:
from collections.abc import MutableMapping
I hope this analysis served helpful at unraveling why the
xxxxxxxxxx
Attributeerror: Module 'Collections' has no attribute 'MutableMapping'
error occurs and offered solutions to get past it. All in the spirit of making coding in Python less perplexing and more joyful!Perhaps you’re currently experimenting with the Python’s Collections module and having issues with the Attributeerror: Module ‘Collections’ Has No Attribute ‘Mutablemapping’ bug. Let me clarify this for you.
Python’s collections module is not actually a ordered group of similar objects, rather, it implements several specialized container datatypes providing alternatives to standard Python’s general-purpose built-in containers, dict, list, set, and tuple. [1]
However, when you encounter the error “Attributeerror: Module ‘Collections’ Has No Attribute ‘Mutablemapping'”, it likely implies that you’re trying to access the MutableMapping class through the collections module, which isn’t directly available as an attribute in there.
To utilize this, you actually need to import it from the collections.abc module like so:
xxxxxxxxxx
from collections.abc import MutableMapping
Making use of the above snippet would then enable you to invoke the MutableMapping class without bumping into any AttributeError.
Now, MutableMapping is an ABC (Abstract Base Class) for read-write mapping types, such as dictionary. It has several methods that need to be implemented in order to create a custom mutable mapping datatype, including __getitem__, __setitem__, __delitem__, __iter__, and __len__. [2]
Let’s say you want to create a custom dict subclass that stores all keys in lowercase; here’s how you can employ the MutableMapping abstract base class to achieve this:
xxxxxxxxxx
class LowerDict(MutableMapping):
def __init__(self, initial_dict):
self._data = {key.lower(): value for key, value in initial_dict.items()}
def __getitem__(self, key):
return self._data[key.lower()]
def __setitem__(self, key, value):
self._data[key.lower()] = value
def __delitem__(self, key):
del self._data[key.lower()]
def __iter__(self):
return iter(self._data)
def __len__(self):
return len(self._data)
my_dict = LowerDict({'Foo': 1, 'Bar': 2})
print(my_dict['foo']) # Outputs: 1
In summation, your attribute error happens due to trying to reach the MutableMapping class via the wrong path. But with an understanding of how to correctly import this class, and knowledge on what it is – an Abstract Base Class used in creating flexible, custom mapping types – you’re now equipped to unravel the greater complexities of Python’s collections module and avoid potential pitfalls.
Certainly, it’s such an observation that when encountering the error,
xxxxxxxxxx
AttributeError: 'collections' module has no attribute 'MutableMapping'
, it is most likely that you’re using a version of Python where
xxxxxxxxxx
MutableMapping
has been moved to the
xxxxxxxxxx
collections.abc
module. Originally, in Python versions earlier than 3.3,
xxxxxxxxxx
MutableMapping
was indeed part of the
xxxxxxxxxx
collections
module, but since Python 3.3, it is shifted to the collections.abc package. Additionally, as of Python 3.9, using
xxxxxxxxxx
MutableMapping
from
xxxxxxxxxx
collections
module is completely removed, which means your code will tend to fire this attribute error upon execution.
In older scripts of python where
xxxxxxxxxx
collections.MutableMapping
does not result in errors, we use it to check if an object is an instance of
xxxxxxxxxx
dict
. This method is highly efficient for ensuring native Python dictionaries and dictionary-like objects behave properly while executing operation which alters their length. For example:
xxxxxxxxxx
something = {}
if isinstance(something, collections.MutableMapping):
something['key'] = 'value'
If you encounter the
xxxxxxxxxx
AttributeError: module 'collections' has no attribute 'MutableMapping'
, this error indicates that you need to rewrite or update your import statement with latest python functioning structure.
To prevent this error and work within python updated changes you should make sure that
xxxxxxxxxx
MutableMapping
should now be imported from
xxxxxxxxxx
collections.abc
instead of
xxxxxxxxxx
collections
. Therefore, the correct python 3.9 (and onwards) compatible code would be:
xxxxxxxxxx
from collections.abc import MutableMapping
something = {}
if isinstance(something, MutableMapping):
something['key'] = 'value'
By writing the code in this manner you are ensuring that it is compatible with both Python 3.9 and future releases.
It’s also worth noting that this kind of refactoring isn’t just confined to
xxxxxxxxxx
MutableMapping
, several other classes were also moved to the
xxxxxxxxxx
collections.abc
module to refine the design of the Python Standard Library according to Python Enhancement Proposal. So, it’s fantastic professional practice to keep yourself updated with language updates, changes that assist in avoiding these kind of deprecated feature issues.
Alright, if you’re working with Python and have run into the error “AttributeError: Module ‘collections’ has no attribute ‘MutableMapping'”, then this is worth reading. MutableMapping comes under the collections.abc module, a set of abstract base classes that provide all of the common methods expected on that container type.
Here’s what’s likely happening:
In Python 3.8 and earlier versions, it was possible to import
xxxxxxxxxx
MutableMapping
from the collections module directly like this:
xxxxxxxxxx
from collections import MutableMapping
But from Python 3.9 onwards, MutableMapping needs to be imported from the collections.abc module:
xxxxxxxxxx
from collections.abc import MutableMapping
The reason for this is that importing ABC from `collections` was deprecated in Python 3.4 due to impending removal in Python 3.9. However, this change wasn’t enforced till Python 3.10. To ensure your code works consistently across different version of Python, its best to always import MutableMapping from collections.abc rather than collections.
To give a general idea about MutableMappings, they are a part of python collections abstract base classes. They provide a way to create data structures that are more specific versions of the built-in python collection data types like dict, list, set, and tuple. Mutable mappings allow us to create objects that behave like dictionaries but can have additional methods or behaviors added. It’s quite useful when you want a dictionary-like object with some changes in default property or method behavior.
Here’s an example of a class that extends
xxxxxxxxxx
MutableMapping
and overrides some of its methods:
xxxxxxxxxx
from collections.abc import MutableMapping
class CustomDict(MutableMapping):
def __init__(self, data={}):
self.data = data
def __getitem__(self, key):
return self.data[key]
def __setitem__(self, key, value):
self.data[key] = value
def __delitem__(self, key):
del self.data[key]
def __iter__(self):
return iter(self.data)
def __len__(self):
return len(self.data)
my_dict = CustomDict({'key_one': 'value_one', 'key_two': 'value_two'})
print(my_dict)
This error is relatively straightforward and can be fixed by adjusting your import statement. If you’re working with other parts of the collections module, it’s a good idea to keep an eye on current and future deprecation warnings so you can adjust your code before these changes break it.Often, when you’re debugging or developing in Python, you might encounter the error: ‘AttributeError: module ‘collections’ has no attribute ‘MutableMapping”. This error primarily occurs due to compatibility issues with the Collections Abstract Base Classes (ABCs) in Python 3.3 and above.
Collections and MutableMapping
In Python, the collections module provides alternatives to built-in container types such as list, tuple and dict. One of these provided alternatives is `MutableMapping` which is a subclass of a dictionary and allows values to be set or removed.
However, in Python 3.3 and later versions, the `MutableMapping` class along with other ABCs was moved to `collections.abc`. As a result, attempting to import `MutableMapping` from `collections` directly will raise an `AttributeError`.
Possible Solution
To fix this issue, you’d need to import `MutableMapping` from `collections.abc` rather than `collections`. Here’s how:
xxxxxxxxxx
from collections.abc import MutableMapping
If your code needs to be compatible with both Python 2 and Python 3, you can try importing `MutableMapping` from `collections` first, and if that fails, fallback to `collections.abc`. Here’s how:
xxxxxxxxxx
try:
from collections import MutableMapping # Python 2
except ImportError:
from collections.abc import MutableMapping # Python 3
Checking for Deprecated Classes in Collections
Another helpful approach for troubleshooting this issue involves identifying any deprecated classes in the `collections` module. From Python 3.10 onwards, importing ABCs directly from the `collections` module would no longer be supported. Therefore, it is useful to review your code (especially if it’s been created or maintained by different developers over time) and identify usage of such deprecated classes to update them.
Upgrading the Python Interpreter
If none of these solutions seem to work, you could consider upgrading your Python interpreter. Errors like these often occur due to differences between Python 2 and Python 3. Upgrading to Python 3.3+ ensures that you’ll be working in an environment free of such compatibility issues.
Summary
The ‘AttributeError: module ‘collections’ has no attribute ‘MutableMapping” usually pops up when you attempt to access `MutableMapping` from `collections`, which isn’t possible from Python 3.3 and later. Comparatively, accessing it from `collections.abc` instead should solve the problem. It’s also a good practice to keep an eye out for deprecated classes while reviewing your code. In severer scenarios, you might have to upgrade your Python interpreter to ensure a hassle-free coding experience.The `AttributeError: module ‘collections’ has no attribute ‘MutableMapping’` error message may present itself when you’re working with Python code. It usually points to the fact that you are trying to access or assign an attribute that doesn’t exist in the collections module. A classic use case of this is when you’re using versions of Python where the `MutableMapping` class has shifted from the `collections` module to the `collections.abc` module.
Why does this happen?
Well, such issues stem from updates between different versions of Python. In Python 3.3, the MutableMapping, alongside other abstract base classes, were moved from the collections module into a new module called `collections.abc`. However, to keep backward compatibility, these were still accessible via collections until Python 3.9. As a result, if you’re using Python 3.9 and later, you will encounter the `Attributeerror: module ‘collections’ has no attribute ‘Mutablemapping’`.
So, how can we resolve it? Here are some of the best methods:
Method 1: Update your import statement
The most efficient and straightforward way to tackle this error is directly modifying the import reference.
Here’s how:
xxxxxxxxxx
from collections.abc import MutableMapping
Instead of:
xxxxxxxxxx
from collections import MutableMapping
By doing so, you make sure that whether you’re running the program on Python version lesser than 3.9 or greater, the correct module with the attribute `MutableMapping` is referenced.
Method 2: Using try / except statements
You could also opt for a fallback method using the try/except statements. This method ensures code runs on any Python 3.x version.
Here’s the code snippet:
xxxxxxxxxx
try:
from collections.abc import MutableMapping # 3.3 and beyond
except ImportError:
from collections import MutableMapping # pre-3.3
This code checks if the Python version accepts importing MutableMapping from Collections.abc. If not, it imports MutableMapping from the former available module collections – ensuring smooth execution in any Python 3.x environment.
To determine how these steps assist in eliminating errors or better enhancing your Python coding efforts, more documentation about the architectural change can be found here.
Remember, understanding various Python versions and their unique changes is pivotal to avoid such Attribute errors. And being aware of the classing restructuring, like MutableMapping’s move to collections.abc, plays a big role in that understanding. Happy coding!The error saying
xxxxxxxxxx
AttributeError: module 'collections' has no attribute 'MutableMapping'
occurs when you try using the
xxxxxxxxxx
MutableMapping
class from the
xxxxxxxxxx
collections
module in Python.
This issue crops up because Python’s
xxxxxxxxxx
collections
module was restructured in Python 3.3, and it starts deprecating some features starting Python 3.8. These deprecated attributes include classes like
xxxxxxxxxx
MutableMapping
,
xxxxxxxxxx
Sequence
,
xxxxxxxxxx
Iterable
among others.
From Python 3.3 onwards, for better organization of collections-related functionalities, the
xxxxxxxxxx
collections
module was divided into two main parts:
1. The
xxxxxxxxxx
collections
module – which contains built-in container types like List, Dict, Set, Tuple etc.
2. The
xxxxxxxxxx
collections.abc
module – which houses the abstract base classes like MutableMapping, Sequence, Iterable relating to collections.
To deal with this issue, if you are running Python 3.3 or above, you should change your import statement from
xxxxxxxxxx
from collections import MutableMapping
to
xxxxxxxxxx
from collections.abc import MutableMapping
If you want to see this in practice, consider the following quick example:
xxxxxxxxxx
from collections.abc import MutableMapping
class CustomDict(MutableMapping):
def __init__(self, data={}):
self.data = data
def __getitem__(self, key):
return self.data[key]
def __setitem__(self, key, value):
self.data[key] = value
def __delitem__(self, key):
del self.data[key]
def __iter__(self):
return iter(self.data)
def __len__(self):
return len(self.data)
Here, we define a class
xxxxxxxxxx
CustomDict
that extends the
xxxxxxxxxx
MutableMapping
abstract base class from the
xxxxxxxxxx
collections.abc
module. We then defined the necessary methods to make our custom dictionary-like object.
Stay updated by regularly checking official Python documentation on collections. They keep up-to-date details about all recent changes and best practices. This is important as Python continues to evolve and add, subtract, or reorganize features. A successful coder is also an informed one!In dealing with the AttributeError: Module ‘Collections’ Has No Attribute ‘Mutablemapping’, you may be working with an older version of Python where MutableMapping isn’t directly available under the collections module.
xxxxxxxxxx
import collections
Would raise an AttributeError because, as of Python 3.3, ‘MutableMapping’ and several other classes have been reorganized into a submodule called collections.abc. Now, if you want to import ‘MutableMapping’, you need to modify your code as follows:
xxxxxxxxxx
from collections.abc import MutableMapping
Sometimes, even older scripts can face issues due to the variations in language specifications and library structures over different Python versions. One thing that is particularly relevant here is Python’s decision to move abstract base classes (such as ‘MutableMapping’) into the collections.abc module starting with Python 3.3. This change was done in a bid to avoid cluttering the global namespace, keeping the collections module tidy and better organized.
Even though this update enhances readability and organization, it often leads to a common error when past scripts are executed with newer Python environments without adjusting for the changes. Hence, modernizing the syntax accordingly is key to solving the “AttributeError: module ‘collections’ has no attribute ‘MutableMapping'” error.
If you’re curious why you’re seeing this error despite not using ‘MutableMapping’ in your own script, it may be due to its usage in a third-party library or a built-in Python library. In such cases, ensuring everything is up-to-date (both your Python installation and any libraries you’re using) can help solve the error.
For instance, within the Python official documentation published by Python Software Foundation, you could find out about latest updates related to the collections module, which is quite a handy reference. By diligently keeping track of these changes, it is possible to anticipate potential errors that may occur, such as the above mentioned one. So whether you are a novice or experienced in Python, remember to keep in sync with these modifications and adapt your coding practice accordingly.
Python Version | Syntax |
---|---|
Pre 3.3 |
xxxxxxxxxx 1 1 1 import collections |
Post 3.3 |
xxxxxxxxxx 1 1 1 from collections.abc import MutableMapping |
It’s always crucial to stay up-to-date with not only the changes in Python’s core syntax but also the modifications of the various modules and libraries we use. Being aware of such changes would significantly reduce time spent on debugging and result in a smoother coding experience!