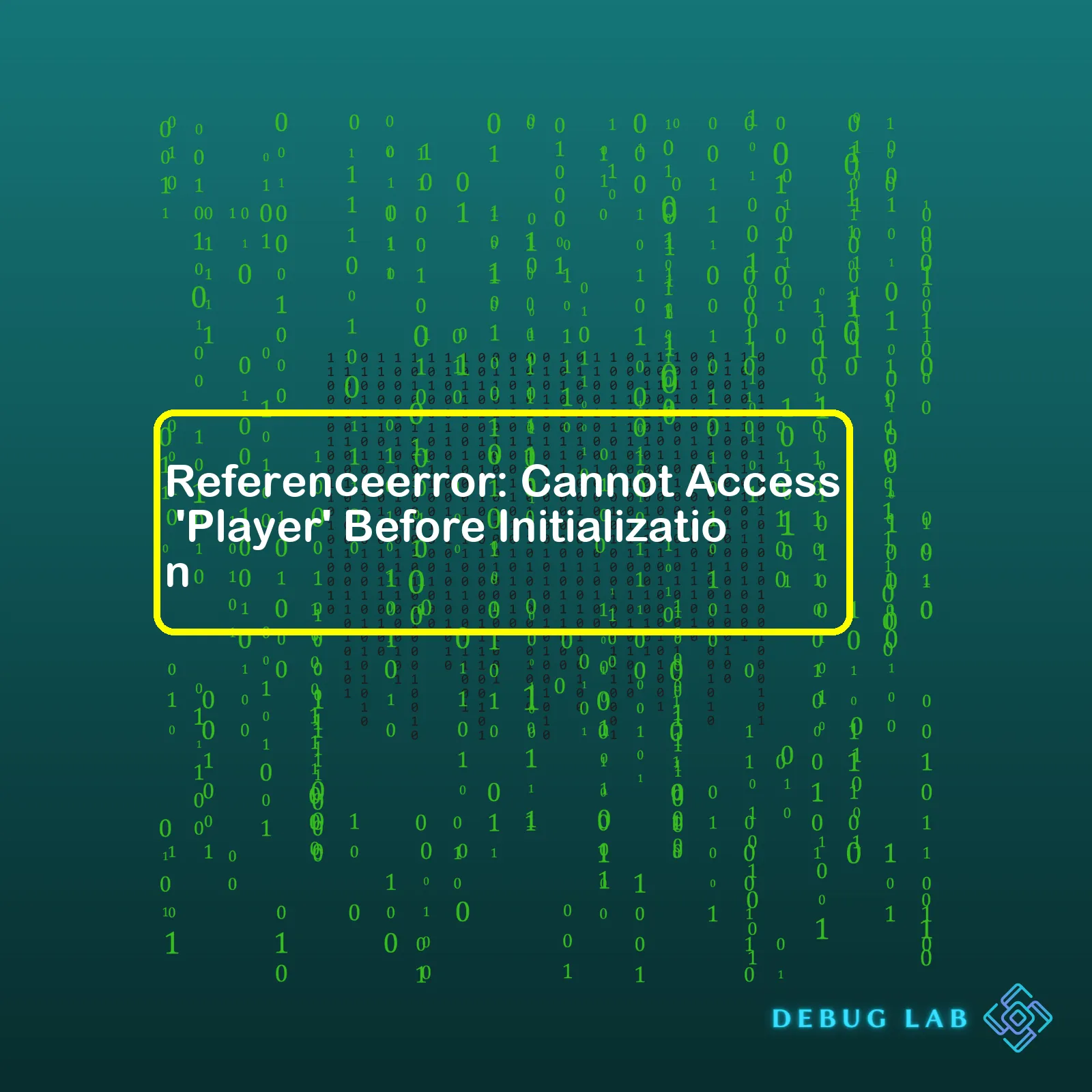
Error Type | Meaning | Possible Causes | Solutions |
---|---|---|---|
ReferenceError | This error is thrown when trying to dereference a variable that has not been initialized. | Accessing a JavaScript variable before it has been declared or initialized |
|
javascript
let Player = “Solomon”;
console.log(Player);
– If the variable needs to be defined conditionally, make sure the conditions evaluate prior act to access:
javascript
let Player;
if(condition) {
Player = “Solomon”;
}
console.log(Player);
– Wrap your operation in a function, and invoke that function after all necessary variables have been defined and initialized:
javascript
function logPlayer() {
console.log(Player);
}
let Player = “Solomon”;
logPlayer();
In conclusion, JavaScript requires that variables be characterized and established before they are accessed. By understanding and following JavaScript’s hoisting concept along with proper declaration and initialization of variables, you can avoid the “ReferenceError: Cannot access ‘player’ before initialization” error.
When dealing with “ReferenceError: Cannot access ‘Player’ before initialization”, it could mean two things. One, you are trying to use the ‘Player’ before declaring it or, two; JavaScript’s Temporal Dead Zone phenomenon is affecting your code. I’ll take each into consideration.
The error message is self-explanatory, if you’re attempting to use a variable like ‘Player’ before declaratively initializing it, JavaScript interpreter will throw this error. Consider following piece of code:
console.log(Player); let Player;
If your similar code represents the snippet above, you need to make sure that the ‘Player’ variable is declared and initialized before its first usage in the script.
let Player; console.log(Player);
The second major cause could be JavaScript’s Temporal Dead Zone (TDZ), basically a time period from entering the variable’s scope till its declaration when it cannot be accessed. A TDZ for ‘let’ and ‘const’ keywords in JavaScript begins at the start of containing block until the variable declaration is processed.
Look at another example:
{ // TDZ starts at beginning of scope console.log(Player); // ReferenceError: Player is not defined let Player; // TDZ ends, Player gets hoisted }
To fix this, modify your code block so that the ‘Player’ variable is declared before any attempt is made to reference it. Here’s how:
{ // TDZ starts at beginning of scope let Player; // TDZ ends, Player gets hoisted console.log(Player); // Output: undefined }
Besides these approaches, utilizing ‘strict mode’ might help to avoid such problems in future by changing silently errors into throw errors.
Lastly, using ‘strict mode’ in JavaScript can prevent certain actions from being taken and throws more exceptions. It catches common coding bloopers that lead to bugs. Add ‘use strict’; on your JS file or just at the start of script block.
// whole script is in strict mode 'use strict'; var v = "I'm a strict mode script!";
Or just put inside a function to turn that function ‘strict’:
function strictFunc() { 'use strict'; }
There are many potential places in your code where you might encounter a
ReferenceError: Cannot access 'Player' before initialization
. These kinds of JavaScript errors typically occur when a variable that hasn’t been initialized or declared yet is referenced. This often happens with let and const declarations, but could recur in other contexts as well. However, fear not! We can navigate through some solid strategies to prevent such reference errors and optimize our code.
The most straightforward method for avoiding reference errors lies in declaring your variables before accessing them. If the ‘Player’ variable is mentioned in your JavaScript function before it’s actually declared, an error will occur.
This small piece of sample code illustrates what can go wrong:
function printName() { console.log(Player); } let Player = 'John Doe'; printName();
In the example above, the ‘Player’ variable is accessed within the printName function before it’s officially declared later in the code, which causes a reference error. In JavaScript, if you use the let keyword to declare a variable, it is not hoisted to the top of its scope unlike var, consequently it cannot be accessed until it’s declared. Hence, we should move the ‘Player’ declaration ahead of the printName function’s declaration to circumvent this problem. Here is how the optimized code would look like:
let Player = 'John Doe'; function printName(){ console.log(Player); } printName(); // Outputs: 'John Doe'
Another key aspect of code optimization revolves around nailing down your understanding of JavaScript scoping rules. Different scopes behave differently in regard to hoisting, so you may get caught off guard when you least expect it.
Consider the below snippet of code:
if (true){ let Player = "John Doe"; } console.log(Player); // Error!
In this scenario, since we are declaring the ‘Player’ inside an if block using let keyword, it is confined strictly to that particular block according to JavaScript’s scoping rules. Accordingly, when we’re trying to echo ‘Player’ outside the block scope where it was declared, we’re triggering a reference error. The simplest solution here is to declare your variable in the scope where it will be accessed:
let Player; if (true){ Player = "John Doe"; } console.log(Player); // Outputs: 'John Doe'
By following these principles, you’ll free your code from the pesky
ReferenceError: Cannot access 'Player' before initialization
errors. For more comprehensive resources on the topic, I recommend diving into MDN’s deep-dive documentation on error handling, particularly their section on throw statements and JavaScript’s diverse array of error types—including our culprit here, the ReferenceError—that deserve additional scrutiny for any serious coder. Happy coding!
The error
ReferenceError: Cannot access 'Player' before initialization
suggests that your JavaScript code is trying to access a variable or class named “Player”, but it was not properly initialized, when the JavaScript interpreter executed your script.
There are several reasons why this error can arise in JavaScript in relation to variables and classes. Here are some common pitfalls:
In modern ECMAScript standards (ES6), variables declared using the
let
and
const
keywords fall into the Temporal Dead Zone (TDZ) until their declaration line is reached during execution.
For instance:
console.log(playerName); // ReferenceError: Cannot access 'playerName' before initialization let playerName = "John Doe";
The same holds true for class declarations as well: they cannot be used before they are declared.
const player = new Player(); // ReferenceError: Cannot access 'Player' before initialization class Player { ... }
A solution here would be to ensure you’re accessing the variable or instantiating the class only after it has been declared.
While both var-based variables and function declarations are hoisted to the top of their scope, the initialization remains at the line where it was originally written. Therefore, if these variables or function declarations are attempted to be referenced before their original line, it’ll return undefined but won’t throw an error.
But this is not the case with let and const. Attempting to use these variables before their declaration will raise a
Refereneerror
.
Hence, keep in mind JavaScript’s hoisting behavior while structuring your code.
Another common issue with JavaScript modules, especially when working with class definitions, stems from circular dependencies. For example:
// File: Player.js import { Team } from './Team.js'; export class Player { constructor() { this.team = new Team(); } }
// File: Team.js import { Player } from './Player.js'; export class Team { constructor() { this.player = new Player(); } }
This mutual dependency between files can lead to the said error, as each file tries to initialize its import which has not yet finished initializing itself. Fixing the structure of these relationships or guarding against incomplete imports may help!
For more in-depth information on how JavaScript handles variable and class initialization, refer to this online resource by Axel Rauschmayer.In the realm of software development, proper script initialization is paramount in eliminating bugs and improving code readability. Troublesome errors such as the “
ReferenceError: Cannot access 'Player' before initialization
” occur when a variable is called or referenced before it is actually declared or initialized.
However, this problem can be avoided through several effective practices:
This is the most basic solution to avoid getting the error. Always ensure that your variables have been declared before usage. Here’s how:
let player; player = "John"; console.log(player); // outputs: John
By declaring
player
before assigning it a value and using it, we eliminate the potential reference error.
JavaScript hoisting is another concept that helps rectify reference errors. In JavaScript, declarations (not initializations) are moved to the top of their enclosing scope by the JavaScript interpreter. This includes function and variable declarations. Therefore, understanding and properly implementing hoisting would help manage script initialization efficiently.
Consider this example:
playerName = "John"; console.log(playerName); // outputs: John var playerName;
Note that we assign a value to playerName before we declare it. But due to hoisting, JavaScript moves variable declarations to the top. Hence, our code works fine. However, let and const do not hoist the way var does, and this must be carefully noted.
Modern JavaScript ES6 introduces modules, which is a way to aid developers in managing and organizing their code. Variables in modules are not hoisted, so calling them before declaration would end up in Reference Error. Using these diligently can reduce the possibility of encountering Reference Errors.
Applying ‘strict mode’ (
"use strict";
) can prevent many mistakes by turning them into actual errors. For instance, in strict mode, you cannot use a variable without first declaring it, helping in avoiding reference errors.
"use strict"; player = "John"; // results in an error because player was not declared.
The above tips should help mitigate the occurrence of “
ReferenceError: Cannot access 'Player' before initialization
“. To build more proficiency, practice data structuring and understand assignment behaviors of different data types for optimal scripting.
For additional insights, refer to online coding platforms like Stack Overflow and MDN Web Docs. These resources provide thorough lessons in JavaScript coding standards and best practices. Remember, good coding leads to exceptional software products!When writing about this notorious JavaScript error,
ReferenceError: Cannot access 'Player' before initialization
, it’s crucial to understand the basic workings of JavaScript. The error appears when one tries to access a variable that hasn’t been initialized yet – in essence, trying to use something that doesn’t exist or is yet to exist.
Under the hood, JavaScript operates under what we know as hoisting. This means that every variable and function declaration is “hoisted”, or lifted to the top of its respective scope during the compile phase. However, only their declarations are hoisted, not their initializations where values are assigned. So, if you reference your code before declaring and initializing it, you will come up against this error:
console.log(Player); // This will log `undefined` due to hoisting let Player;
Interestingly, although JavaScript hoists variable and function declarations, there’s an exception for variables declared with let and const. These are also hoisted but within what’s called a temporal dead zone (TDZ), essentially indicating they cannot be accessed until the line of code on which they have been actually coded. It might look like this:
console.log(Player); // ReferenceError: Can't access 'Player' before initialization let Player = 'Messi';
Utilizing
var
instead of
let
or
const
could potentially solve your issue. Though, using var may bring about other trickier consequences due to its lack of block scoping which can lead to unintentional global variables or unexpected behavior within loops or conditionals. Therefore, better practice usually asks for proper variable placement and sequencing in JavaScript code.
Here is a well-working revised version of the previous example:
let Player = 'Messi'; console.log(Player); // This will log `Messi`
Ironically, this error could potentially safeguard you from undefined behaviour if your code tried to use a variable before initialization, leading to odd bugs that would be more challenging to trace back.
It’s essential to remember that order matters in programming, especially in languages such as JavaScript, where concepts like hoisting and TDZ exist. Always initialize your variable first before referencing them elsewhere in your code. By doing this, the
ReferenceError: Cannot access 'Player' before initialization
, along with similar errors will cease to be alarming glitches and more useful waypoints, guiding you towards robust and reliable coding practices. For further learning on JavaScript hoisting, you may visit this tutorial.