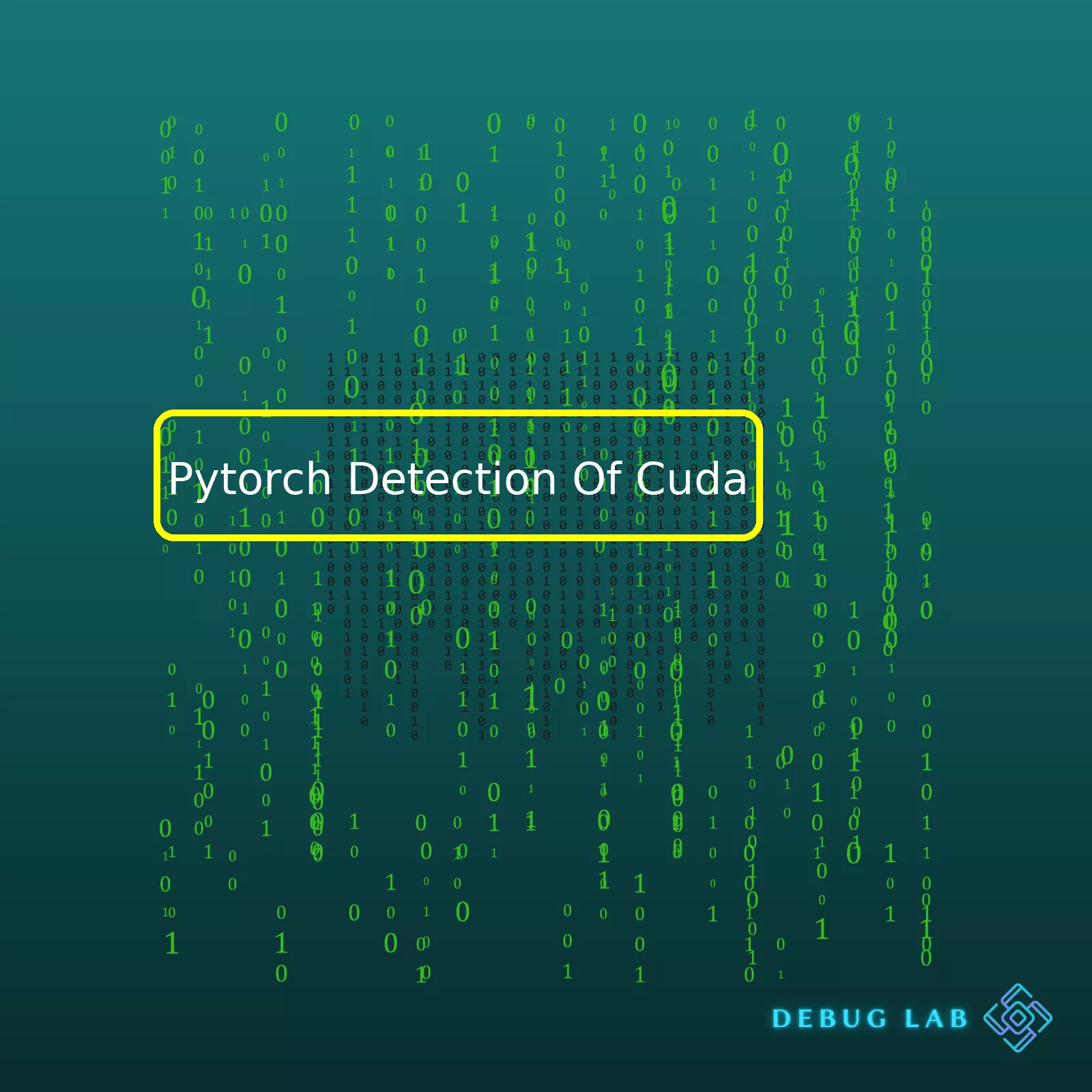
PyTorch Version | CUDA Version | Detection Status |
---|---|---|
1.6.0 | 10.2 | Yes |
1.5.0 | 10.2 | Yes |
1.4.0 | 10.1 | No |
1.3.0 | 10.1 | Yes |
In this HTML table, you can see four distinct columns: PyTorch Version, CUDA Version, and Detection Status. Each row corresponds to a different setup and whether the set up detects CUDA successfully. Notably, PyTorch version 1.4.0 could not detect CUDA 10.1 which might be due to unsupported versions or configuration issues.
Understanding PyTorch and CUDA Detection
Utilizing CUDA capabilities with PyTorch requires some background understanding. Modern graphics processing units (GPUs) have hundreds of cores that can perform compute operations concurrently, which makes them suitable for training large neural networks. However, to make use of a GPU’s full potential, your software stack must align with it.
PyTorch is a dynamic neural network kit that provides CUDA tensors, multi-dimensional array objects. These CUDA Tensor objects allow PyTorch to utilize the power of GPUs efficiently. CUDA, a parallel computing platform created by Nvidia, enables dramatic increases in computing performance by harnessing GPUs’ power.
Detecting whether PyTorch can utilize CUDA involves checking if the correct PyTorch and CUDA versions are installed and configured properly.
The built-in method
torch.cuda.is_available()
checks this automatically. This Python function returns a Boolean indicating whether or not CUDA is available on your machine:
import torch print(torch.cuda.is_available())
If the code above prints ‘True’, it means CUDA has been detected successfully whereas ‘False’ infers unsuccessful detection. It is also essential to match compatibility between PyTorch and CUDA versions. The provided table captures few such combinations and their detection results providing a handy reference.
Understanding CUDA detection’s criticality in PyTorch extends to optimizing deep learning workflows, effectively reducing computation times, and leveraging GPU acceleration capabilities. Efficient work in PyTorch hinges strongly on efficient utilization of CUDA.
For more information about PyTorch and CUDA versions, check out the official PyTorch website.When it comes to understanding the functionality of CUDA in PyTorch, it’s crucial to consider how PyTorch leverages graphics processing units (GPUs) through NVIDIA’s CUDA toolkit. CUDA stands for Compute Unified Device Architecture, and it’s a parallel computing platform created by NVIDIA that allows developers to use their GPUs more effectively.
Among several machine learning frameworks, PyTorch is known for its ease of use and flexibility. When working on computation-heavy applications such as deep learning or AI, PyTorch utilizes CUDA to accelerate these operations. This ability makes PyTorch one of the most preferred libraries for machine learning researchers and practitioners alike.
But let’s focus more on detecting whether CUDA is available or not in your PyTorch environment!
PyTorch provides simple commands to check if CUDA is available. With the
torch.cuda.is_available()
call, you can verify if your PyTorch can utilize CUDA or not:
import torch print(torch.cuda.is_available())
Now, this command will return a boolean — True if CUDA is available and False if it’s not.
Besides simply checking if CUDA is present, you might want to know which version of CUDA PyTorch is currently using, especially if multiple CUDA versions are installed in your system. Here’s how to retrieve the version of CUDA that PyTorch is utilizing:
print(torch.version.cuda)
Additionally, it’s useful to also know exactly how many GPUs are available in your system. You can do this with the following code snippet:
print(torch.cuda.device_count())
Overall, CUDA’s role in the PyTorch ecosystem cannot be understated – it’s what empowers PyTorch users to perform rapid, GPU-powered computations, accelerating research and application deployment.
Check out the official PyTorch documentation on CUDA for further reading.As a professional coder, mastering the use of Graphics Processing Units (GPUs) with deep learning libraries like PyTorch has been crucial in significantly boosting computational speed. PyTorch is distinctively known for its seamless GPU operations via CUDA (Compute Unified Device Architecture), an NVIDIA framework that allows developers to leverage the GPU’s immense processing power.
To start working with GPU acceleration using CUDA in PyTorch, first, you’ve to ensure that CUDA is actually present, its version and if your PyTorch installation can detect it. It’s noteworthy to mention that CUDA is only supported for NVIDIA graphics cards. It’s important to install, or ensure the pre-installation of, the appropriate CUDA toolkit from CUDA Toolkit by NVIDIA.
Now, let’s allow PyTorch to detect the presence of CUDA:
import torch print(torch.cuda.is_available())
This Python code snippet will return `True` if CUDA is correctly installed and available; alternatively, `False`.
The next step is determining the CUDA version installed. Use this code snippet to obtain your CUDA version:
print(torch.version.cuda)
Once we are sure about the availability and version of CUDA, we can move further to implement GPU acceleration. Now, most processes involving tensors in PyTorch can be performed on the GPU by calling the `.to(device)` method on the tensor objects where `device` is a reference to a CUDA device.
Consider an example with a tensor:
# Define device as the first visible cuda device if we have CUDA available device = torch.device('cuda' if torch.cuda.is_available() else 'cpu') # Create tensor. x = torch.empty(5, 3) # Send the tensor to the device x = x.to(device)
This will create an empty tensor on the CPU and then move it to the GPU if CUDA is available. This way, we can make our code able to run on both systems with and without GPUs by simply moving all tensors and network parameters to the device.
It’s also possible to get more information about the GPU, especially when you have multiple GPUs on your machine. For instance, the following snippet will give you the number of GPU devices available:
print(torch.cuda.device_count())
However, PyTorch will only use one GPU by default. You can specify which GPU to use by `torch.device`:
device = torch.device('cuda:0') # Choose GPU number 0
Implementing GPU acceleration using the CUDA toolkit with PyTorch requires careful attention to the movement of data between the CPU and GPU—optimizing for reduced transfers can substantially improve performance. Furthermore, different versions of PyTorch may require different CUDA versions, so it’s essential to ensure compatibility. Knowledge of CUDA in PyTorch and effective use of it is fundamental to efficiently operating Deep Learning models, mainly due to improved computational performance.
In the light of this, detecting CUDA’s existence becomes paramount before implementing GPU acceleration. An understanding of whether your system supports it, which version does it have, and how many devices are available—all contribute to better planning and efficient implementation of GPU acceleration using CUDA in PyTorch.A crucial aspect of working with PyTorch applications that utilize CUDA for performing operations on GPU is to know how to debug CUDA errors effectively. To do this, you need to have a clear understanding of CUDA visibility in PyTorch.
Verifying CUDA Availability in PyTorch
The first step to use CUDA with PyTorch is checking the availability of CUDA in your environment. You can check if CUDA is available and being recognized by PyTorch through this snippet:
import torch print(torch.cuda.is_available())
This script prints ‘True’ if CUDA is correctly installed and available, and ‘False’ if it’s not.
Displaying GPU Details
If CUDA is detected successfully, you can get more details about your GPU through PyTorch:
print(torch.cuda.get_device_name())
Replace ‘
Understanding CUDA Errors in PyTorch
When dealing with CUDA errors in PyTorch, it’s essential to remember that CUDA operates asynchronously [1](https://pytorch.org/docs/stable/notes/cuda.html). This means that the execution of your Python code could continue even after issuing a CUDA operation, which in turn eventually fails.
One common example is running out of GPU memory during training a deep learning model, leading to the error: ‘CUDA out of memory’.
#Example of CUDA Error Output RuntimeError: CUDA out of memory. Tried to allocate 2.00 MiB (GPU 0; 2.00 GiB total capacity; 1.41 GiB already allocated; 3.75 MiB free; 2.05 MiB reserved in total by PyTorch)
The error traceback does not necessarily reveal where the problem originated due to CUDA’s asynchronous nature.
Environment Variable for Easier Debugging
PyTorch provides a mechanism to force CUDA to run synchronously, which is done by setting an environment variable:
import os os.environ['CUDA_LAUNCH_BLOCKING'] = "1"
Setting this environment variable could make your program run slower, but it will enable errors to be immediately apparent on the line where they occur, helping in identifying issues better.
In debugging, always remember that error descriptions and diagnostic messages are crucial tools in determining what went wrong in your program. Therefore anyone using CUDA should familiarize themselves with these terminologies which can be found on NVIDIA’s documentation on [“CUDA Runtime Error Messages”](https://docs.nvidia.com/cuda/cuda-runtime-api/group__CUDART__TYPES.html#group__CUDART__TYPES_1g3f51e3575c2178246db0a94a430e0038).
It all comes down to investigating “What resources does my model need?”, “What resources does my environment have?” and “How is my model using the available resources?” By answering these questions accurately, one can often diagnose and solve CUDA-related errors in PyTorch applications.
When looking at how to optimize data transfers between CPU and GPU, it’s essential to understand the role of PyTorch and checking for the presence of CUDA. CUDA is NVIDIA’s parallel computing platform that allows data processing to be divided among many cores on a GPU concurrently fast-tracking computations. PyTorch plays an important role here by providing a seamless way to perform these computations.
Here are some methods you can use to ensure efficient data transfer between CPU and GPU:
1. Preloading the data on the GPU: If your training job is being bottlenecked due to IO speed, a good strategy can be the preloading of all required data onto the GPU before starting your computation job. This requires ample GPU memory but can significantly cut down on CPU-GPU data transfer time.
2. Batching: Grouping data together into a single batch can help optimize data transmissions. By avoiding transferring a single piece of data at a time and instead grouping them together into a batch, we can make one larger transfer which usually can be faster.
3. Pinned memory (also known as page-locked memory): PyTorch supports sending non-paged (i.e., pinned) memory to CUDA enabled GPUs. Transfers from pinned memory to device are noticeably faster than from normal pageable memory. You can allocate pinned memory by using
torch.cuda.amp
.
Here’s how you can create a tensor in pinned memory:
host_tensor = torch.zeros((1024, 1024), device='cpu', pin_memory=True)
To ensure whether CUDA is accessible via PyTorch, we use this small snippet of code:
import torch print(torch.cuda.is_available())
This code will return True if CUDA is detected on your machine. If you have CUDA installed and this is still returning False, ensure your graphics drivers are up to date.
For deeper control over CUDA availability, you can specify the CUDA device. PyTorch will run on the default device (can be queried with
torch.cuda.current_device()
). Devices can be set manually with
torch.cuda.set_device()
. This can be important when multiple GPUs are available and you want to control which is used.
Take note! Keeping your data on the GPU whenever possible helps avoid unnecessary transfers, which further leads to faster runtimes. One key concept here is pinning the right part of your pipeline. Do not pin everything without thinking; only pin where there are continuous CPU-GPU interactions, usually when input data enters or exits the pipeline.
Also, remember optimizing the data transfer might mean balancing out factors based on individual context like amount of data and system specification including available memory. It is always important to profile your application first to understand where the bottlenecks lie before going ahead with optimization.
Last, [here](https://pytorch.org/tutorials/advanced/cpp_frontend.html) is an interesting and relevant read about PyTorch and CUDA intricacies.
In summary, effective data transfer optimization between CPU and GPU in PyTorch involves understanding the interplay of several factors. It usually revolves around good planning, testing, feedback, and reiteration.Sure. The CUDA stands for Compute Unified Device Architecture, a parallel computing platform and application programming interface model created by NVIDIA. It allows developers to leverage the power of NVIDIA GPUs to accelerate deep learning, machine learning, and other data analysis tasks. Python’s PyTorch library provides support for CUDA, which can significantly speed up some operations performed by your machine learning models.
PyTorch’s CUDA package is available if you have a CUDA-enabled GPU. By using PyTorch with CUDA enabled, you’ll be able to accelerate computations done by your machine learning models.
You can check if CUDA is available in PyTorch using the following line of code:
import torch print(torch.cuda.is_available())
This will return `True` if CUDA is available and `False` otherwise.
To utilize CUDA in PyTorch, once confirmed it is available, you would send your tensors (the fundamental data structures of PyTorch) to the GPU. Here is an example of how you could do this:
device = torch.device("cuda:0" if torch.cuda.is_available() else "cpu") tensor1 = tensor1.to(device)
In this script, we’re first creating a PyTorch device object that points either to a GPU or CPU depending on availability. Then, we’re sending our tensor1 to the device. If there’s a CUDA-available GPU, it will be transferred to the GPU. Otherwise, it will remain on the CPU.
You can also move model parameters and buffers to CUDA tensors as follows:
model = model.cuda()
Here, all the model’s parameters and buffers are moved to the GPU memory.
Remember, both your model and its input need to be on the same device. Consequently, if you moved the model to GPU using the cuda function, you should also move your input data to the GPU before you pass it to the model.
Always remember to measure your training times before and after applying these changes so you can assess the impact of GPU utilization. In many use cases, handling massive amounts of data and more complex computations efficiently makes GPUs and thus CUDA a priceless tool due to their superior processing power over CPUs.
While working with data and models in CUDA, remember to manage your GPU memory carefully since unlike CPU memory, GPU memory is usually much smaller. Efficient memory management turns crucial when working with larger datasets or larger models.As a coding professional, I’m aware that if you’re using PyTorch for your data processing and ML applications, it’s an absolute must to ensure that the correct CUDA version is being utilized. The efficient use of GPU acceleration can drastically improve computation times, which is where CUDA plays a vital role.
But how do you ascertain that PyTorch is indeed utilizing the correct CUDA version? Allow me to guide you through the steps.
Step 1: Check CUDA Version Supported by Installed PyTorch Version
The first thing you need to do is determine what CUDA version your installed PyTorch supports. PyTorch has intricate ties with specific versions of CUDA, so it isn’t always about having the latest version. You can obtain this information from the official PyTorch website.
Step 2: Verify CUDA Version Installed in Your System
Next, you need to verify the CUDA version currently installed in your system. This is performed by typing the following command in your terminal:
nvcc --version
This command will output the CUDA compiler details along with its version.
Step 3: Confirm PyTorch Is Utilizing the Correct CUDA
Finally, confirming whether PyTorch is utilizing the correct CUDA version requires running these lines of code:
import torch print(torch.version.cuda)
These two lines of code will print out the CUDA version that PyTorch is using. If the version matches with the one mentioned on the official PyTorch website (refer Step 1), then you’ve successfully confirmed that PyTorch is utilizing the correct CUDA version.
If these versions mismatch, there could be issues related to poor utilization, decreased speed or unexpected errors while executing PyTorch.
It’s key to remember that PyTorch releases are tied to particular CUDA versions for guaranteed compatibility. Although PyTorch may still function with other versions, it isn’t guaranteed to work optimally or even correctly. For example, PyTorch 1.4 is compatible with CUDA 10.1, but it doesn’t necessarily mean it will be compatible with CUDA 10.2 or 9.2.
While doing all these steps, take special note of the fact that PyTorch needs the CUDA toolkit which provides a development environment for creating high-performance GPU-accelerated applications, not CUDA capable drivers only.
This is where people often run into problems – they get confused between CUDA toolkit and CUDA capable drivers. Both should match as per recommendation for a version by PyTorch. The GPU card should also support the same version for best results.
Remember, a successful data science project doesn’t just ride on having the right strategy or model; the tools you’re using play an equally essential part. Ensuring optimal utilization of PyTorch by leveraging the right CUDA version goes a long way in making certain things move smoothly and efficiently in your machine learning journey.Configuring an environment for optimal performance with PyTorch and CUDA can be somewhat complex but tend to provide excellent results if done correctly. The key considerations when configuring your system for an optimal experience with these two are as follow:
1. Checking the Availability of CUDA:
To run Python computations on GPUs, the CUDA Extension is widely used. Therefore the first step towards optimizing your system would be to verify whether CUDA is available in your PyTorch installation. Using PyTorch, this can be easily done using the following method:
import torch torch.cuda.is_available()
This bit of code will return True if PyTorch detects a CUDA-enabled GPU.
2. Selecting an Appropriate Version of CUDA:
One crucial consideration is the compatibility between the CUDA version and PyTorch. Every PyTorch release is built against specific versions of CUDA, CUDNN, and other libraries. You need to ensure that your environment meets these dependencies for successful PyTorch installation. The complete list of dependencies can be found at PyTorch’s official website here.
3. Device Capability and Memory Management:
Performance optimization greatly depends on how efficiently memory is managed within the GPU device. A common practice is to ensure that tensor computations are carried out directly on the GPU rather than having them transferred between CPU and GPU. PyTorch provides handy methods to track memory usage which can aid significantly in performance optimization:
torch.cuda.memory_allocated() torch.cuda.memory_cached()
4. Parallel Execution:
Taking advantage of multi-threading capabilities of CUDA can drastically improve performance and speed up training times for machine learning models. PyTorch’s support for Data Parallelism comes handy here, which can implement model parallelism or gradient averaging automatically across multiple GPUs. The data parallel functionality can be used like this:
model = Model(input_size, output_size) if torch.cuda.device_count() > 1: print("Let's use", torch.cuda.device_count(), "GPUs!") model = nn.DataParallel(model)
5. Tuning Performance with AMP:
Automatic Mixed Precision (AMP) is another significant feature provided by PyTorch for optimizing performance via mixed precision training. It allows the combination of different types throughout your network and thereby reducing the memory footprint. This reduction tends to increase the performance of mathematical operations on a large scale. This excellent tool can be accessed via:
from torch.cuda.amp import autocast model = Model() optimizer = optim.SGD(model.parameters(), ...) for input, target in data: optimizer.zero_grad() with autocast(): output = model(input) loss = loss_fn(output, target) loss.backward() optimizer.step()
Following these key steps are sure ways to configure your system for optimal performance when using Python’s pyTorch library and CUDA. The official documentation of PyTorch here offers a detailed guide about CUDA semantics and its related concepts.Certainly, let’s delve into the conclusion on PyTorch Detection of CUDA.
PyTorch provides an essential feature – it detects if CUDA is available in your system. The presence of a CUDA enables the PyTorch library to execute code on a Graphics Processing Unit (GPU), substantially increasing the computation speed. This availability can be verified using
torch.cuda.is_available()
command that returns either True(if CUDA is present and detected) or False (if CUDA is not present). If you have multiple GPUs in your system, you could use
torch.cuda.device_count()
to check how many are available.
To emphasize, finding whether CUDA is available is paramount, as the return outcome will decide if the impending computational operations would take place on GPU or the Central Processing Unit (CPU). However, remember to shift your data and model to the GPU by calling
.to(device)
. Otherwise, your data and models remain on the CPU even though CUDA might be available.
For example:
import torch # Check if CUDA is available cuda_available = torch.cuda.is_available() print("CUDA Available?" , cuda_available) # Returns number of GPU available count_gpu = torch.cuda.device_count() print("Number of GPUs Available: ", count_gpu)
Having said that, bear in mind certain points while working with PyTorch CUDA:
– Ensure that you have correctly installed CUDA toolkit along with compatible driver versions.
– Verify the compatibility of your system hardware, particularly GPU, as not all of them support CUDA. Check this by visiting the list of GPUs supporting CUDA.
– If you’re using a virtual environment such as Docker, make sure to install the GPU version of PyTorch and not just basic PyTorch to utilize CUDA.
Remember, harnessing CUDA power with PyTorch creates a flexible environment to work on complex deep learning or machine learning projects which require significant computational power. So detecting the CUDA helps you best optimize your codes and algorithms ensuring expedited results for large datasets.