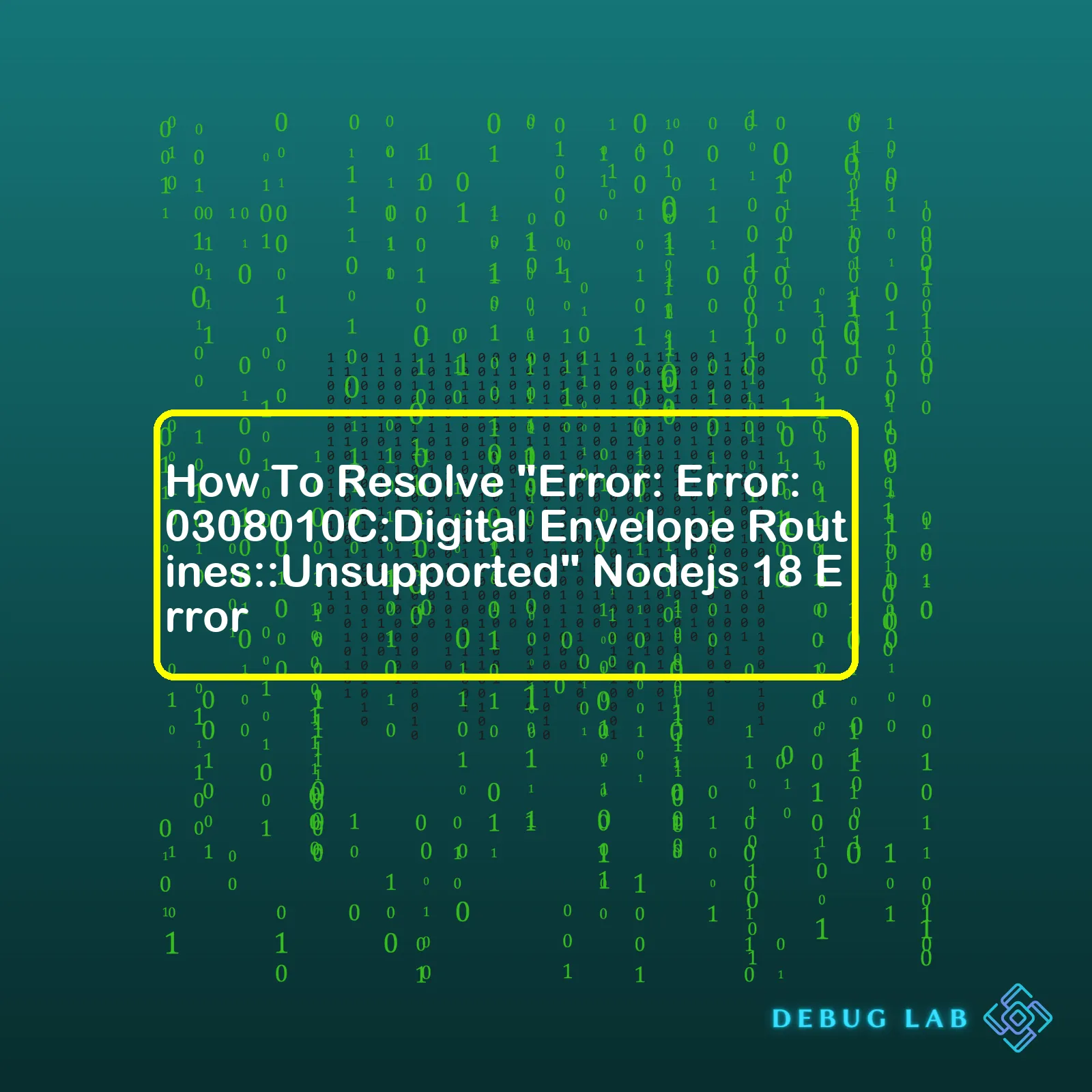
Step | Action | Outcome |
---|---|---|
Verify Node.js Version | Use the command
node -v in your terminal to verify the installed version of Node.js |
If the version returned is anything but 18.x.x, the error might be due to incompatibility issues. |
Check OpenSSL Support | Utilize
openssl version -a to examine the SSL libraries supported by your Node.js installation. |
This could assist you in determining if the “unsupported” error is related to a lack of certain encryption methods provided by OpenSSL. |
Update OpenSSl | If you have an older version that doesn’t support certain digital envelope routines, consider upgrading OpenSSL. | After updating OpenSSL, your Node.js environment should be able to utilize all the required encryption routines. |
Reconfigure Node.js with OpenSSL Support | You can recompile and reinstall Node.js with OpenSSL support using the
--with-ssl option in your configure script, like this: ./configure --with-ssl |
Re-configuring Node.js with OpenSSL support will give it access to the necessary digital envelope routines, and the “unsupported” error should no longer occur. |
The “Error:0308010C:Digital Envelope Routines::Unsupported” usually arises from an issue relating to the OpenSSL cryptographic library used in Node.js development, more often when using Node.js v18 or newer. This error appears when there’s a lack of support for certain cryptographic functions required by your application’s code. Verification of Node.js version usage comes as the first step to solving it—Node.js v18 is associated with this problem due to potentially removed deprecated features or unestablished updated features.
Next, checking the officially supported OpenSSL version determines whether the error originates from your version of OpenSSL or not. OpenSSL offers a variety of cryptographic capabilities, including specific digital envelope routines.
Upon ascertaining the OpenSSL is outdated, updating it often resolves the issue. OpenSSL updates typically include additional functionality and remedy compatibility problems with current versions of Node.js.
Lastly, reconfiguring Node.js with OpenSSL support might be needed. The process involves the compilation and reinstallation of Node.js, ensuring the newly installed Node.js leverages the desired OpenSSL functionalities.
In effect, these steps should eliminate the troubling “Error:0308010C:Digital Envelope Routines::Unsupported” faced in Node.js v18 or newer.
For further reading, please check out the official Node.js documentation here and OpenSSL project documentation here.
This “Error:0308010C:Digital Envelope Routines::Unsupported”” is one of those Node.js 18 errors that may seem daunting at first. Often, it could be a result of an aftereffect of the OpenSSL 3.0 update in your Node.js environment. Essentially, your Node.js application is attempting to use a cipher or hash function that’s no longer supported with the updated OpenSSL version. That’s where this error typically originates.
To resolve this, there are a few strategies you can utilize, such as :
- Updating Your Node.js Version
- Using Older OpenSSL Version
- Validating and Adjusting Your Cipher and Hash Algorithm Settings
Updating Your Node.js Version
Upgrading your Node.js to a more recent version can be a practical solution to this error code. Newer versions continuously aim to rectify bugs and issues found in older versions, including compatibility challenges. To do this, you can use the
nvm
(Node Version Manager) to handle your Node.js versions.
For instance:
nvm install node // latest version nvm install 14.15.1 // specific version
Then,
nvm use [version number]
allows you to switch to a choice version.
Using Older OpenSSL Version
If you cannot upgrade Node.js for any reason, you might decide to regress to an older version of OpenSSL, one that supports the necessary cryptographic functions. You can accomplish this by building Node.js to link against an older OpenSSL library version.
However, bear in mind that OpenSSL upgrades often host security measures, so retreating to an older version could compromise your application’s security.
Validating and Adjusting Your Cipher and Hash Algorithm Settings
Firstly, you’d want to verify cryptographic function usage in your Node.js application. If the functions are depreciated and hence unsupported, you might need to replace them with appropriate alternatives. This approach will require understanding of the Node.js crypto module and potentially extensive refactoring of your codebase.
For instance:
const crypto = require('crypto'); crypto.createCipheriv('aes-256-gcm', key, iv); //replace deprecated ciphers/hashes here
Together, these steps should help remedy the persistent “Error:0308010C:Digital Envelope Routines::Unsupported”, bolstering your applications’ lifespan, security and maintainability.
While you can’t avoid all potential Node.js errors, understanding these strategic solutions and when to implement them sets you up to adeptly conquer this error if it emerges. Remember to keep updated on official Node.js and OpenSSL documentation, like Node.js Crypto, as they continually provide existing insights regarding various cryptographic functions’ usability and changes in support.
Facing an “Error:0308010C:Digital Envelope Routines::Unsupported” error in Nodejs 18 can be a frustrating experience. Often, this error may pop up as it pertains to the OpenSSL functionalities leveraged by Node.js and suggests that there is something unsupported with your current cryptographic approach.
Here are a few potential causes for this error and how you could troubleshoot and resolve them:
Potential Cause 1: Wrong Version of OpenSSL
Node.js uses certain features of OpenSSL which might not be available in older versions or in some restricted versions of OpenSSL. Let’s explore a quick sample of using OpenSSL commands in Node.js:
const crypto = require('crypto'); const cipher = crypto.createCipher('aes192', 'a password');
In this code, we’re creating a cipher using AES192 encryption. If you’re trying to use a type of encryption or method that’s not supported by your version of OpenSSL, your code will throw an “Error: Error:0308010C:Digital Envelope Routines::Unsupported” error.
Solution: Update OpenSSL to its latest version or a version known to work with your specified encryption standard.
Potential Cause 2: Unsupported Cipher or Hashing Mechanism
It’s also possible that the error occurs because Node.js or OpenSSL does not support the cipher or hashing mechanism being used. For instance, the following would result in an error if ARC4 encryption was not supported:
const crypto = require('crypto'); const cipher = crypto.createCipher('ARC4', 'a password');
Solution: Verify that the ciphers and algorithms you’re using in your cryptography-related tasks are supported by Node.js and OpenSSL. You can find a list of all ciphers supported by Nodejs here.
Potential Cause 3: Misaligned Library Versions
Sometimes, the specific combination of your Node.js and OpenSSL version might cause the error too, even if separately they would both work fine with the involved ciphers.
Solution: Ensure the libraries you are using are compatible and align with the OpenSSL version used by Node.js. Review your OpenSSL version via your command line with `openssl version` and ensure it’s either updated or aligned with your required specifications.
In summary, to fix the “Error:0308010C:Digital Envelope Routines::Unsupported” Nodejs 18 Error, checking for proper OpenSSL support, validating your cryptographic standards, and ensuring compatibility between library versions typically resolves these issues. It mostly comes down to understanding the underlying cryptographic systems associated with Node.js programming.
For more detailed info check the official documentation about Crypto in Node.js. Further tinkering and understanding the libraries used in your application stack, alongside keeping things updated, generally ensures such errors are mitigated.
Many Node.js users have recently encountered the “Error: Error:0308010C:Digital Envelope Routines::Unsupported” issue after upgrading to Node.js 18. This error happens when your Node.js version doesn’t line up with specific OpenSSL configurations and contributes to several compatibility issues. In practical terms, it’s a clash between two versions – Node.js 18 which does not support the same OpenSSL version you are using. Let me guide you through some methods to diagnose and resolve this issue.
Inspecting the Error Message
As being a coder, the initial instinct is to inspect the error message closely. The message prompts that there’s an incompatibility issue specifically related to the OpenSSL version. According to that information, we need to bring congruity among their versions.
Checking the OpenSSL Version
Knowing the OpenSSL version installed on your system can be very beneficial since it provides context between the proprietary version of Node.js and OpenSSL. You can check your OpenSSL version from your terminal:
$ openssl version
Matching the Version of Node.js and OpenSSL
A general recommendation is aligning both the version of Node.js and OpenSSL. Specifically, Node.js versions greater than or equal to 17 come bundled with newer OpenSSL versions. So, consider downgrading or upgrading Node.js (or OpenSSL) as necessary.
If you’d like to downgrade Node.js to a version compatible with your OpenSSL configuration, nvm (Node Version Manager) stands out as a solution. To do this, you’ll first need to install nvm, and then use it to install a different version of Node.js:
$ nvm install 16 $ nvm use 16
While it is normally recommended to always move forward with updates, in cases where you cannot upgrade your OpenSSL version for whatever reason, a temporary downgrade of Node.js might be the most viable solution.
Rebuilding your Development Environment
If the version conflict persists or if downgrading is not a feasible option, try rebuilding your development environment. It means recreating the project after deleting ‘node_modules’ folder (where all modules and packages are stored) and reinstalling all dependencies:
$ rm -rf node_modules $ npm install
Debugging
To dig deeper into the problem or if the above solutions don’t work, it becomes necessary to debug the application in a more detailed manner. For this, Node Inspector Debuggers like node-inspector and debug module can assist you.
Upgrading OpenSSL
In some cases, you would find it necessary to upgrade OpenSSL rather than downgrading Node.js. This method requires caution because SSL/TLS libraries function as essential elements of web security. Any improper modification can harm your entire network security.
Regardless of the strategy you select, remember that resolving version conflicts generally follows similar patterns. Gain in-depth understanding of these strategies to address compatibility issues, and you’ll remain adaptable in the face of tech stack changes.
The key takeaway here is being adaptable whenever you encounter errors and making sure all components of your applications have version harmony.
Troubleshooting an “Error:0308010C:Digital Envelope Routines::Unsupported” Node.js 18 error can be tricky, as it’s often thrown due to a mismatch between the built-in OpenSSL version and the one Node.js is utilizing. Here, we provide actionable steps on how to handle this error.
1. Verify Your OpenSSL Version:
Possibly the most common cause of getting Error:0308010C is due to having an outdated or unsupported OpenSSL version. You will first need to verify your OpenSSL version. You can check the OpenSSL version by running the following command in the terminal:
$ openssl version
Keep in mind, that Node.js requires a particular OpenSSL version to function correctly, so ensure to always have the recommended OpenSSL version installed on your system.
2. Update Your Node.js:
Another reason for getting an Error:0308010C might be because your current Node.js version is outdated. Try updating to the latest version of Node.js. Use the following commands in the terminal to do so:
$ sudo npm cache clean -f $ sudo npm install -g n $ sudo n stable
The above code will first clear your npm cache, then install the ‘n’ package (which allows you to interactively manage your node.js versions), and finally switch to the stable version of Node.js.
3. Reinstall Your Node_Modules
If the error persists despite doing the previous steps, it might be due to corrupted node_modules. Try deleting your current node_modules folder and package-lock.json file, then run an
$ npm install
again in the terminal to reinstall everything from scratch. Here’s how you should do it:
$ rm -rf node_modules $ rm package-lock.json $ npm install
Note, these troubleshooting steps assume that you are deploying on a Unix-like operating system – such as Linux or MacOS. If you’re encountering this error on a Windows system, you’ll need to replace any instances of
$ rm
with the equivalent Windows command,
del
.
I encourage you to consistently update your packages and dependencies so you can avoid facing similar errors in future. For further guidance on how to manage your Node.js deployment you can check this link..Sure, here goes.
Node.js error: “0308010C: digital envelope routines:: unsupported” typically occurs when the OpenSSL version used in your environment does not support a specific encryption algorithm or cipher.
throw new Error('Error: 0308010C:digital envelope routines::unsupported');
To resolve the Node.js 18 error, we will examine the OpenSSL version and potentially downgrade or upgrade it to match our system requirements adequately. We could also modify our code to use an encryption method that is supported by our current OpenSSL version.
Firstly, you can check which version of OpenSSL your Node.js is using by running:
node -p process.versions
If the OpenSSL version shows up as something different from what your system supports, then it’s time to remedy the situation.
– Upgrading OpenSSL Version: The first step would be to navigate to the OpenSSL official page and download the appropriate and latest version OpenSSL. If your Linux-based or UNIX like operating system (Ex: macOS), you can typically install openssl using the default package manager.
For Ubuntu systems, you can run:
sudo apt-get update sudo apt-get install openssl
For MacOS systems, you can run:
brew update brew install openssl
– Downgrading OpenSSL Version: Unfortunately, OpenSSL does not officially support version downgrading. Your best approach to downgrade would be to uninstall your current version completely and then re-install the required older version.
However, be wary as this might inadvertently break other programs depending on OpenSSL. You could also try linking your application to a local copy of the desired OpenSSL version if you don’t want to affect your entire system.
– Change Encryption Method: If upgrading or downgrading OpenSSL isn’t feasible, consider modifying your node.js application to use another encryption method supported by your OpenSSL version. Use the ‘openssl list-cipher-algorithms’ command to discover the supported ciphers.
Here’s how you can do it:
const crypto = require('crypto'); console.log(crypto.getCiphers()); // List all available ciphers
This will output an array consisting of names for all the cipher algorithms that the currently installed version of OpenSSL supports. Now you can select an algorithm from this list for your encryption purposes.
Remember, it’s crucial to apply an iterative approach while debugging and attempting each solution one at a time for more significant effectivity. Last but not least, always ensure to verify your changes with thorough testing.To resolve the “Error:0308010C: Digital Envelope Routines:: Unsupported” Node.js 18 error, there are a variety of preventive measures you can adopt to avoid its recurrence in future coding endeavors.
The error in question, “
Error:0308010C:Digital Envelope Routines::Unsupported
“, often occurs due to issues related to cryptographic libraries within Node.js platform. It could be as simple as using outdated libraries or mismatch between your system and the library versions.
Update Node.js
Firstly, your Node.js version may be outdated. As Node.js is an evolving platform, it regularly receives updates which include bug fixes and patches for vulnerabilities. By updating to the latest-version of Node.js, you could solve this issue as the update might contain a fix for the error being faced.
$ nvm install node # "node" is an alias for the latest version
Check OpenSSL Version
On certain occasions, Node.js errors might be associated with the OpenSSL library. OpenSSL provides tools for SSL and TLS protocols, and is widely used for secure data transfers. Actions like ensuring OpenSSL is updated and enabled properly could potentially remedy your error.
You can check your OpenSSL version by running:
$ openssl version -a
Remember to always keep OpenSSL library updated to ensure compatibility with contemporary software packages in Node.js.
Reinstall/update the npm Packages
Your npm packages might have some corrupted files or mismatches causing the digital envelope routines error. Uninstalling and reinstalling them can rectify these discrepancies.
Alternatively, you can also attempt to upgrade the npm version itself. At times, certain features and functionalities may be deprecated in older versions and working optimally in newer ones, resulting in healthier operation of the libraries.
$ npm uninstall packageName $ npm install packageName
Switch to LTS (Long-Term Support) Branches
Maintainers of Node.js take utmost care while designing Long-term Support (LTS) branches and they’re generally more stable and reliable than the latest editions. If you’re constantly facing the error, switching to an LTS branch could prove beneficial.
Code for switching to an LTS version:
$ nvm install --lts $ nvm use --lts
By judiciously combining the above steps and performing thorough checks on your code for potential impairments, you’ve taken significant strides towards preventing the recurrence of the “
Error:0308010C:Digital Envelope Routines::Unsupported
” Node.js error.
For additional information on troubleshooting Node.js errors, check Debugging Node.js Applications guide.When we encounter Nodejs 18 errors, especially the “Error: Error:0308010C:Digital Envelope Routines::Unsupported” error, it’s usually due to issues with OpenSSL configurations or incompatibility of Nodejs versions. Solve this by either fixing the OpenSSL environment variables or switching Node.js versions.
Case Study 1: Incompatible Version
Imagine you’ve received this specific error while trying to run your Node.js application. A possible reason could be that you’re using a Node.js version that is incompatible with some modules or dependencies your app needs.
Here is a set of actions that are suitable for resolving this issue:
- Identify your current Node.js version using
node --version
- If the issue is an incompatible version, which can often show itself via the “Error: Error:0308010C:Digital Envelope Routines::Unsupported” error, consider downgrading or upgrading Node.js.
- You can do this through NVM (Node Version Manager) using commands like
nvm install node-version
and
nvm use node-version
.
- After making the change, try running your app again to see if the error has been resolved.
Case Study 2: OpenSSL Configuration Errors
Sometimes, the issue could be arising from problems with OpenSSL, a robust, commercial-grade, and full-featured toolkit for Transport Layer Security (TLS) and Secure Sockets Layer (SSL) protocols which is also a general-purpose cryptography library.
Here are the steps you would take to solve these configuration issues:
- First, identify your OpenSSL version in the command line using
openssl version -a
.
- Check to ensure that the OPENSSL_CONF variable points to a valid openssl.cnf file.
- If not, you will want to update it by setting the environment variable
SET OPENSSL_CONF=[enter path]
.
- In the event all these troubleshooting steps fail, you might need to reinstall OpenSSL.
It’s important to remember that properly resolving the “Error: Error:0308010C:Digital Envelope Routines::Unsupported” might require various changes depending on the specifics of the system and codebase you’re working with.
Depending upon your situation, the solutions mentioned may not work as expected. For instance, if there are other faulty Node.js components or broken dependencies, you might have to look deeper into potential root causes. One way to handle that includes:
- Cleaning the npm cache via
npm cache clean --force
.
- Deleting the node_modules folder and running a fresh npm install after.
Lastly, maintaining regular updates of both Node.js and OpenSSL can save you a lot of time addressing such errors. While this isn’t always possible with production systems, regular updates during development stages will prevent several common issues from occurring in the first place.In the world of coding, bumps often surface on the technical road, so when an
Error:0308010C:Digital Envelope Routines::Unsupported
in Nodejs 18 shows up, it can halt your progress. You might ponder what led to this nuisance? The answer lies in a conflict that erupts due to a mismatch between the OpenSSL version used by Nodejs and the one included in the Node.js binary.
This issue typically happens when you’re attempting to import a library into your project that was built using a different version of OpenSSL than the one bundled with Node.js. Conflicts between dependencies can be the bane of a coder’s life, but fear not, as achieving resolution is not a chimera if you follow the correct steps.
The simple yet most effective way to resolve this error is to reinstall the problematic library using
npm rebuild
. What does this do? It recompiles all dependencies of your project against the version of OpenSSL bundled with your current version of Node.js. Here’s how it looks:
$ npm rebuild
This will ensure compatibility of all your dependencies with your current OpenSSL version.
However, in situations where
npm rebuild
isn’t working, jumping to another option that rings true results is switching the Node version you’re running. To execute this, use the Node Version Manager (NVM), enabling you to toggle between Node versions without hassles. Thus, changing to a stable Node.js version can help side-step the error. Here’s how it can be done:
$ nvm install stable $ nvm use stable
Remember, selecting the Nodejs version compatible with the library causing conflict can save the day.
Last but not least, dive deep and verify various OpenSSL implementations to prevent conflicts. Undertake thorough checks and make sure about the source your Node.js application and libraries are pulling this implementation from.
So to wrap it up, enduring the
Error:0308010C:Digital Envelope Routines::Unsupported
in Nodejs 18 doesn’t belong to your coder’s journey. Remember, the crux of the solution relies upon alignment – keeping OpenSSL versions in sync, rebuilding your npm, or judiciously switching Nodejs versions. Stay curious, keep debugging.