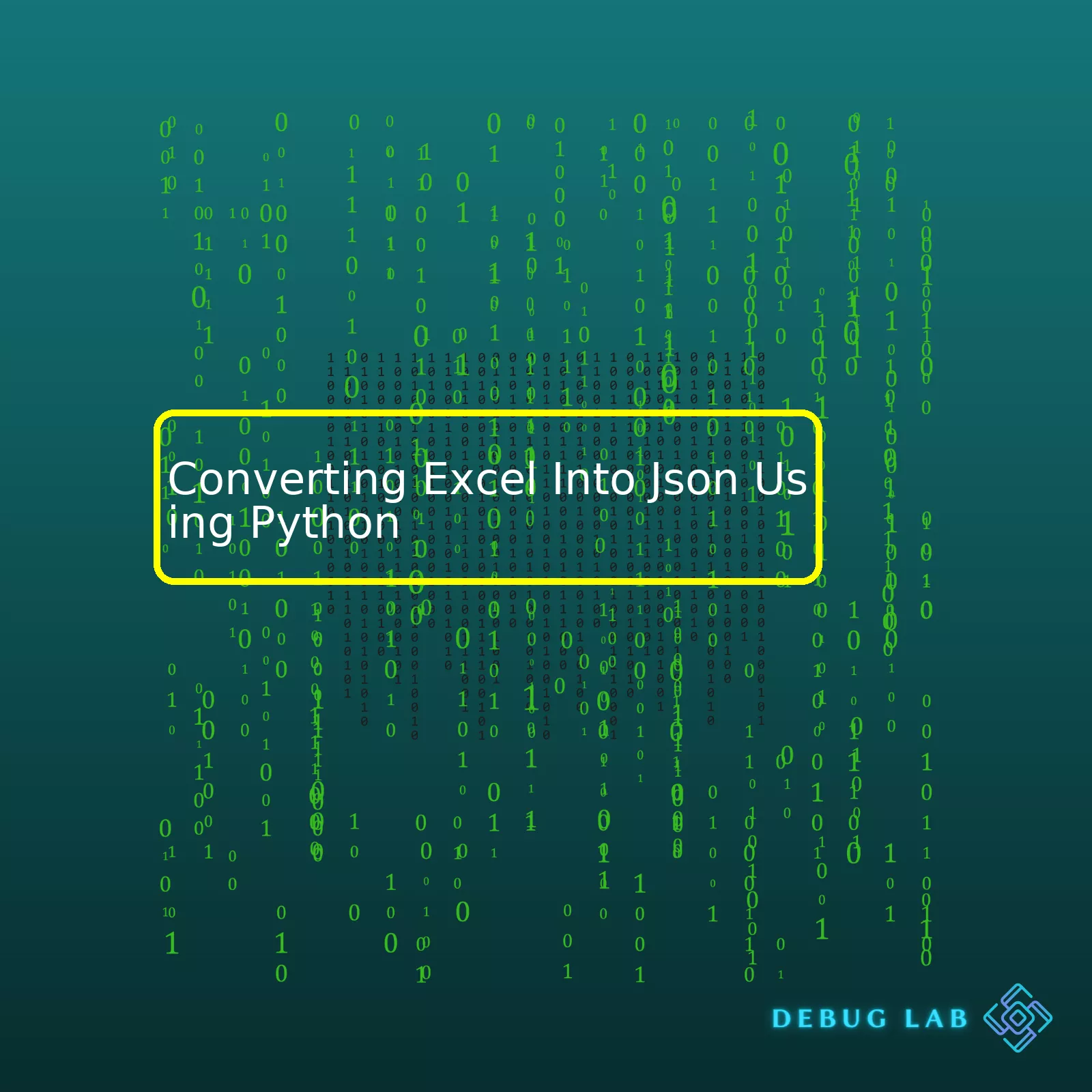
Steps | Description |
---|---|
Installing the necessary libraries | The key Python libraries needed for this task are pandas and openpyxl. Pandas provides data manipulation capabilities, whilst openpyxl is used to read and write Excel 2010 xlsx/xlsm/xltx/xltm files. |
Reading Excel data with pandas | Pandas’
x 1 1 read_excel function is used to load the Excel file into a DataFrame. The syntax is: xxxxxxxxxx 1 1 1 dataframe = pandas.read_excel('path_to_excel_file') . |
Converting the DataFrame to JSON | The DataFrame’s
xxxxxxxxxx 1 1 1 to_json function is then used to convert the DataFrame to JSON. The syntax is: xxxxxxxxxx 1 1 1 json = dataframe.to_json() . |
Writing the JSON to a file | The built-in Python
xxxxxxxxxx 1 1 1 with open.. as construct and the xxxxxxxxxx 1 1 1 .write() method can be used to write the JSON string to a file. Make sure to use the xxxxxxxxxx 1 1 1 .json file extension. |
Now, let me elaborate on the procedure outlined in the table above.
To start off the process of converting Excel into JSON using Python, there’s a requirement for specific libraries. Key among them are pandas, an open-source Python library providing high-performance, easy-to-use data manipulation structures and data analysis tools, and openpyxl, designed for reading and writing Excel 2010 xlsx/xlsm/xltx/xltm files.
Once these libraries are installed, you’ll first need to harness the power of pandas to read the contents of your Excel file. This process utilizes the
xxxxxxxxxx
read_excel
function of pandas to create a DataFrame – essentially creating a table in memory from your data. With your data now in a Python-friendly format, you’re free to manipulate and analyze it as required.
At this point, the conversion of the DataFrame to JSON comes into play. By calling the
xxxxxxxxxx
to_json
function directly on the DataFrame object, you’re able to effortlessly produce JSON output. Jumping from Excel to JSON in just two steps demonstrates the simplicity and power of using Python for data transformation tasks.
Finally, after a quick conversion, Python’s built-in abilities take over once again to write the resulting JSON string back out to a file. Simply put, the final step uses the tried-and-true
xxxxxxxxxx
with open... as
construct to set up a context for file operations, and the
xxxxxxxxxx
.write()
method to physically write the JSON data to a file on disk.
In conclusion, by exploiting Python’s rich ecosystem of data-focused libraries and its core language features, any developer can easily transform an Excel document into a JSON file. No matter where you are in your Python journey, tasks like these help you understand the real-world potency of Python in simplifying complex data manipulation tasks.
Understanding how to convert Excel data into JSON format using Python is an essential skill for large-scale data processing projects. JSON (JavaScript Object Notation) and Excel are two crucial file formats used in data analysis. Excel, a widely-used spreadsheet program, allows users to organize data in rows and columns, while JSON, a common data format with diverse language support, helps store and communicate the organized and structured data on browser-server side.
Python offers powerful libraries and modules such as
xxxxxxxxxx
pandas
and
xxxxxxxxxx
json
to manipulate, analyze, and convert these formats.
Let’s explore converting Excel into JSON using Python:
- The first step involves reading the Excel file using pandas’ read_excel() function. It reads an Excel workbook into a pandas dataframe object, maintaining all information such as column names, index, and data types.
- Once we have the data in pandas, we can quickly transform this DataFrame into a JSON document with the
xxxxxxxxxx
111to_json()
method. This will produce a string in JSON format.
- Save JSON to a file, if you need.
xxxxxxxxxx
import pandas as pd
# Read the Excel file
df = pd.read_excel('file_name.xlsx')
xxxxxxxxxx
# Convert DataFrame to JSON
json_data = df.to_json()
xxxxxxxxxx
with open('output_file.json', 'w') as f:
f.write(json_data)
The data structure of a JSON file resembles that of Python dictionaries, making it easy to read and manipulate. Meanwhile, Excel files present a tabular form of data – making it ideal for large volumes of data in structured rows and columns. Leveraging Python’s
xxxxxxxxxx
pandas
and
xxxxxxxxxx
json
libraries, you can smoothly transition between these two popular data formats, powering your data manipulation and analysis tasks. Therefore, understanding Python’s approach towards managing Excel and JSON data is critical.
For additional details on handling different excel and JSON operations, please refer to the official [`pandas`](https://pandas.pydata.org/docs/reference/index.html) and [`json`](https://docs.python.org/3/library/json.html) documentation.
Remember, while JSON and Excel files serve similar purposes, they are used in different scenarios. Excel files are better suited for human readability and manual data entry, while JSON is an excellent format for transmitting data between a client and server, due to its lightweight and easy-to-use nature. Thus knowing how to convert Excel into JSON using Python becomes a valuable tool for any coder.
The process of converting Excel files into JSON can be streamlined by using Python, an accessible and user-friendly programming language esteemed for its simplicity and power. Here are the crucial steps to achieve that-:
Step 1: Import Necessary Libraries
The first step involves importing necessary libraries into your Python environment. In this case, we need pandas, a powerful data manipulation library, to read from Excel file and convert the data into JSON.
xxxxxxxxxx
import pandas as pd
Step 2: Read Excel File
Next, you’d utilize pandas’ read_excel function to ingest your target Excel file. Suppose the name of the Excel file is ‘data.xlsx’.
xxxxxxxxxx
df = pd.read_excel('data.xlsx')
Here ‘df’ is a dataframe that holds the contents of the ‘data.xlsx’ excel sheet.
Step 3: Convert Data to JSON
Once the data is ingested into Python, the conversion into JSON is quite straightforward with pandas’ to_json function.
xxxxxxxxxx
json_data = df.to_json(orient='records')
By setting orient=’records’, the JSON structure will resemble a list of dictionaries where keys are column names and values represent data points.
Step 4: Write the JSON to a File
With the properly structured JSON string (json_data), we can create a new JSON file and transfer our data into it. Let’s say we want to create a JSON file named ‘data.json’.
xxxxxxxxxx
with open('data.json', 'w') as f:
f.write(json_data)
After executing these steps, you’ll find a new file named ‘data.json’ in your current directory where each record in excel has been transformed to a dictionary format with column headers as keys.
Common Use Table Representation
A typical conversion process is exhibited in the following table.
Steps | Python Code |
---|---|
Importing necessary library |
xxxxxxxxxx 1 1 1 import pandas as pd |
Reading from Excel |
xxxxxxxxxx 1 1 1 df = pd.read_excel('data.xlsx') |
Converting to JSON |
xxxxxxxxxx 1 1 1 json_data = df.to_json(orient='records') |
Writing to JSON file |
xxxxxxxxxx 1 2 1 with open('data.json', 'w') as f: 2 f.write(json_data) |
You could modify the steps according to your needs. For instance, if you’d like to read from specific sheets or ranges within the Excel file, pandas provides flexibility for those operations (read_excel). Similarly, customizing the structure of the final JSON file can be done through the orient parameter (to_json).Dealing with datasets is an integral part of any data analysis project, and Python provides a robust library named
xxxxxxxxxx
Pandas
to handle this. The primary use of
xxxxxxxxxx
Pandas
is for data manipulation, cleaning, analysis, and visualization.
To understand the role of the pandas package in the conversion process from Excel to JSON using Python, let’s first delve into these formats:
– Excel: Known for its spreadsheet capabilities, Excel is largely used for handling, manipulating and visualizing data. Files usually have an ‘xlsx’ extension.
– JSON (JavaScript Object Notation): It is a popular data interchange format, known for being lightweight and text-readable. It’s primarily used to transmit data between a server and web applications.
Now, diving into the role of
xxxxxxxxxx
Pandas
:
xxxxxxxxxx
Pandas
has several functions enabling a fluent and interactive interface between various file formats like Excel, CSV, SQL databases, etc., and your python code. One such function is
xxxxxxxxxx
read_excel()
. This function reads an excel file and transforms it into a DataFrame, which is two-dimensional size-mutable, potentially heterogeneous tabular data.
For example:
xxxxxxxxxx
import pandas as pd
# Reading the excel file
df = pd.read_excel('file_name.xlsx')
Once our data is within a DataFrame, we have the full power of Pandas at our disposal. We can manipulate, clean, analyze, visualize and convert this data. For our context, we’re interested in the
xxxxxxxxxx
to_json()
xxxxxxxxxx
to_json()
is used to convert the DataFrame object into JSON string.
Here is how you do that:
xxxxxxxxxx
# Converting DataFrame to json format
json_data = df.to_json(orient='records')
In the above single line of code, the
xxxxxxxxxx
DataFrame.to_json()
function is used to convert Excel data into JSON format. The term ‘records’ in the argument specifies that the resulting JSON strings should be constructed in record style, meaning they’ll look something similar to this sample output:
xxxxxxxxxx
[
{"column1":"value1_1","column2":"value2_1"},
{"column1":"value1_2","column2":"value2_2"}
]
As seen above, the Pandas package plays a crucially important role in the conversion process from excel to JSON, giving us a seamless approach to quickly transform data across different formats. As a coder, leveraging the versatility and power of Pandas to manipulate and modify data across diverse formats will significantly upgrade your productivity. Remember, more efficient data handling leads to better development speed, enhanced code quality, less memory consumption, and optimized performance.
Source:
– Pandas Documentation
Excel file is a common medium for data storage and analysis. However, when it comes to using this data for web-based applications or APIs, JSON (JavaScript Object Notation) offers a significantly lighter, readable, and easily parse-able format. Python, with its powerful libraries like Pandas and Openpyxl, can be an essential tool for converting Excel data to JSON format.
The first step involves installing the necessary Python packages. In our case, these would be ‘pandas’ and ‘openpyxl’. The installation can be done using pip, which is python’s package installer.
xxxxxxxxxx
pip install pandas openpyxl
Pandas is an open-source library providing high-performance and easy-to-use data structures, whereas openpyxl allows Python to read/write Excel xlsx/xlsm/xltx/xltm files.
After successfully installing the appropriate packages, you can begin working in your python environment to convert your Excel data into JSON.
To start, we read the Excel file using pandas:
xxxxxxxxxx
import pandas as pd
def excel_to_json(excel_file_path):
# Use pandas to read the Excel file
data_frame = pd.read_excel(excel_file_path)
Next, we convert the pandas DataFrame into JSON. It can be done by calling the ‘to_json’ function on the DataFrame object:
xxxxxxxxxx
# Convert the data frame to JSON
json_str = data_frame.to_json(orient='records')
‘orient=records’ creates a JSON array of objects, where each row in the dataframe becomes one object in the array. The keys are column names from the dataframe.
To save this JSON string into a file, use python’s regular file handling functions:
xxxxxxxxxx
# Write JSON to a file
with open('output.json', 'w') as f:
f.write(json_str)
After running this function with the specific path to your Excel file as parameter, you should have a new ‘output.json’ file in your directory – structured as per your original Excel data!
Pandas gives control over the format of the resultant json from your excel data. Let’s uncover some of it:
Argument | Description |
---|---|
xxxxxxxxxx 1 1 1 orient='split' |
Dict like {index -> [index], columns -> [columns], data -> [values]} |
xxxxxxxxxx 1 1 1 orient='records' |
List like [{column -> value}, … , {column -> value}] |
xxxxxxxxxx 1 1 1 orient='index' |
Dict like {index -> {column -> value}} |
xxxxxxxxxx 1 1 1 orient='columns' |
Dict like {column -> {index -> value}} |
xxxxxxxxxx 1 1 1 orient='values' |
Just the values array |
Please note that JSON structure also has its limitations and might not support all forms of data present in your excel file such as images or charts. For complex tasks like these, dedicated tools might need to be used.
Sure, converting Excel into JSON using Python can be a swift process with the help of Python’s built-in libraries such as ‘pandas’ for data analysis and manipulation, ‘openpyxl’ for working with Excel files and ‘json’ for parsing data to and from JSON.
Let’s break down the process into parts:
– Importing the required libraries
– Loading Excel data
– Converting Excel data to JSON
– Writing JSON data to an output file
1. Importing Required Libraries:
We will import three libraries: pandas, openpyxl and json.
xxxxxxxxxx
import pandas as pd
from openpyxl import load_workbook
import json
2. Loading Excel Data:
First, it’s necessary to read the Excel data. The pandas library has a handy function called read_excel that makes this easy. This function directly reads Excel files and transforms the data into a DataFrame object.
xxxxxxxxxx
excel_data = pd.read_excel('sourcefile.xlsx')
‘b. Converting Excel data to JSON:
Pandas DataFrame object provides a method ‘to_json’ which converts DataFrame into json format.
xxxxxxxxxx
json_data = excel_data.to_json(orient='records')
Here, ‘orient’ attribute decides the format of the resulting JSON.
– When set to ‘split’, it returns a dictionary like {index -> [index], columns -> [columns], data -> [values]}
– When set to ‘records’, it returns a list like [{column -> value}, … , {column -> value}]
– When set to ‘index’, it returns a dictionary like {index -> {column -> value}}
– When set to ‘columns’, it returns a dictionary like {column -> {index -> value}}
– For ‘values’, it returns just the values array.
3. Writing the Output File:
The final step is writing the resulting JSON data to an output file. Python’s json module provides a method ‘dump’ that writes a Python object into a JSON file.
xxxxxxxxxx
with open('outputfile.json', 'w') as json_file:
json.dump(json_data, json_file)
With ‘w’ mode, Python opens the file for writing. If the file doesn’t exist, it gets created. When the file already exists, the content gets overwritten. We’re using python’s context manager (the “with” statement) for handling our file, which ensures the file is cleaned up promptly and efficiently once it’s no longer needed.
So, there it is! You can convert your Excel file into a JSON file using Python in just these steps.When it comes to error handling during the conversion process from Excel to JSON using Python, there are several best practices a coder should follow. Python proposes several specific built-in exceptions that will handle common circumstances such as file not found errors, zero division errors, etc. This inherently makes for more robust code with more capabilities to deal with otherwise unexpected scenarios and gracefully respond to them instead of leaving an ugly system traceback.
Error Identification and Handling: One of the significant parts of dealing with conversion errors is identifying typical scenarios where they can occur. In the context of converting Excel into JSON using Python, consider these likely possible mistakes:
– When the excel file you need to convert doesn’t exist
– If the content in the Excel spreadsheet is structured incorrectly
– If the Python script running the conversion doesn’t have permission to read or write data
To proceed, let’s look at some important and applicable ways to perform error checking and handling.
Using Try/Except Blocks: In Python, we use try/except blocks to detect and handle exceptions. This allows us to intercept errors and run alternative code when they happen.
For instance, attempting to open a nonexistent file might cause a FileNotFoundError exception. Here’s how we can handle this using a try/except block:
xxxxxxxxxx
try:
with open('nonexistent_file.xlsx', 'r') as file:
# Attempt to read the file
pass
except FileNotFoundError:
print('The specified file does not exist.')
In the above case, if the specified file doesn’t exist, rather than crashing with a FileNotFoundError, the script prints a friendly message and continues to run.
We can expand the use of the try/except block to handle other issues like incorrect structure or permissions by adding more specific exceptions before finally catching every other Exception type.
Type Checking: Performing type checks is an integral part of error handling. Pandas `read_excel()` function is typically used to read Excel files in Python. That said, we must ensure the data we fetch matches our requirements (including data types). In Python, we can use the isinstance() utility to verify object types.
Data Validation: Even though the correct types might be provided, validation of the content is crucial. For example, suppose our application expects an Excel cell to configure a certain number but contains a string instead. In such cases, doing some sort of checks (like via regex validations or simple if conditions) to ensure the data conforms to a precise pattern makes our Python applications fail-safe and scalable.
Error handling comes with a learning curve and understanding of your project/application, yet it’s essential. Developers must understand the frequent areas where applications can encounter issues to provide a smooth user experience. With proper error identification and recovery mechanisms, your Python code to convert Excel to JSON will become more resilient, traceable, and maintainable. As your script gets complicated and handles more types and sizes of data, error-handling methodologies help to keep the code organized.
One great place to further explore the Python Built-In exceptions is the Python Docs Exceptions Library. Keep in mind, having descriptive error messages helps you debug better in future scenarios while also providing users with better insights if something unexpected happens.One of the most common tasks we encounter as Python developers is converting an Excel file into a JSON file. JSON files provide a flexible and efficient data structure for receiving and sending data between a server and a client, while Excel files are often used to store flat data for reports or analyses.
Despite being a simple task, there are few Python optimizations you can follow to speed up this process.
Convert Excel Into Pandas DataFrame First
When working with Excel files in Python, it’s helpful to first convert the Excel file into a Pandas DataFrame format. This allows us to take advantage of the speed and efficiency of DataFrame operations when converting your data to JSON. Here’s how you do it:
xxxxxxxxxx
import pandas as pd
def read_excel_file(file_path):
df = pd.read_excel(file_path)
return df
Pandas Options For Converting To JSON
Pandas provides several options to optimize your conversions:
- orient: Changes the JSON output format.
- date_format: Controls the format of DateTime data.
Example with specified options:
xxxxxxxxxx
def convert_to_json(df):
json_data = df.to_json(orient="records", date_format="iso")
return json_data
In this case, orient=”records” would produce a list like [{column -> value}, … , {column -> value}], which tends to be more compact and readable.
Leverage Multi-Threading
If you are dealing with multiple Excel files at once, you can make use of multi-threading. Each thread can take care of converting one .xls(x) file to a .json file concurrently. An easy way to do this in Python is by using the concurrent.futures module:
xxxxxxxxxx
import concurrent.futures
import glob
def convert_excel_to_json(file):
df = pd.read_excel(file)
json_data = df.to_json(orient='records')
with open (file.replace('.xlsx','.json'), 'w') as file:
file.write(json_data)
if __name__ == '__main__':
files = glob.glob('*.xlsx')
with concurrent.futures.ThreadPoolExecutor(max_workers=5) as executor:
executor.map(convert_excel_to_json, files)
These are few optimization techniques. However as always, balance between running time and code readability has to be maintained. +1 for ensuring properly indexed DataFrames. It ensures faster lookups. “pandas indexing explained” (source) is a very good resource to understand how indexing can improve runtimes significantly.
Benchmark before you consider optimizing. python’s timeit module (“docs.python.org“) is a quick tool for checking your solution’s efficiency.
Also remember that not all .xlsx documents are created the same: they might have different sized sheets, hidden images/comments, and so on. Different libraries may respond differently to these variations. Always validate on a subset before scaling.
These tips will definitely give you a foundation on which you can build. Happy coding!
Looking back on the journey we’ve taken together in understanding how to convert Excel into JSON using Python, it’s clear that this process is truly essential for modern data handling and management. More importantly, mastering this technique significantly enhances your Python programming skills – particularly when working with diverse data formats.
For brevity’s sake, let’s succinctly recap what we’ve learned:
Reading Excel Files:
The first step in our data conversion process begins by importing the necessary Python libraries and loading our target Excel file using
xxxxxxxxxx
pandas.read_excel()
. This function turns our Excel sheets into handy pandas DataFrame objects which can be easily manipulated.
Analysing DataFrames:
Through pandas’ built-in functions like
xxxxxxxxxx
DataFrame.head()
or
xxxxxxxxxx
DataFrame.describe()
, we gain insight into our data’s structure – a vital knowledge for quality preservation during the conversion process.
Conversion to JSON:
We accomplish our key mission of converting Excel into JSON through the powerful
xxxxxxxxxx
DataFrame.to_json()
function, choosing between different output orientations based on our specific needs.
Writing JSON Files:
Finally, we store our converted data on disk as a .json file, with the option to pretty-print it for human-friendly readability.
Understanding this crucial bridge between spreadsheet data and web-ready formats does not only improve your proficiency in Python but also opens new avenues for efficient and seamless exchange of information.
Remember some of the most common applications where switch from Excel to JSON significantly reduces complexity including:
- Powering web APIs
- Feeding JavaScript visualizations
- Storing configuration settings
With this newfound knowledge, you’re well equipped to tackle similar tasks even involving different document structures and export requirements.
For those interested in deepening their understanding, excellent resources abound online for further learning. Libraries’ documentation such as pandas ( pandas I/O ) provide valuable insights for advanced cases. Also, numerous forums (stackoverflow) present practical challenges and creative solutions shared by the coding community worldwide.
In all, keep exploring, experimenting, and expanding your horizons. There’s always more to learn in the dynamic world of Python data manipulation!