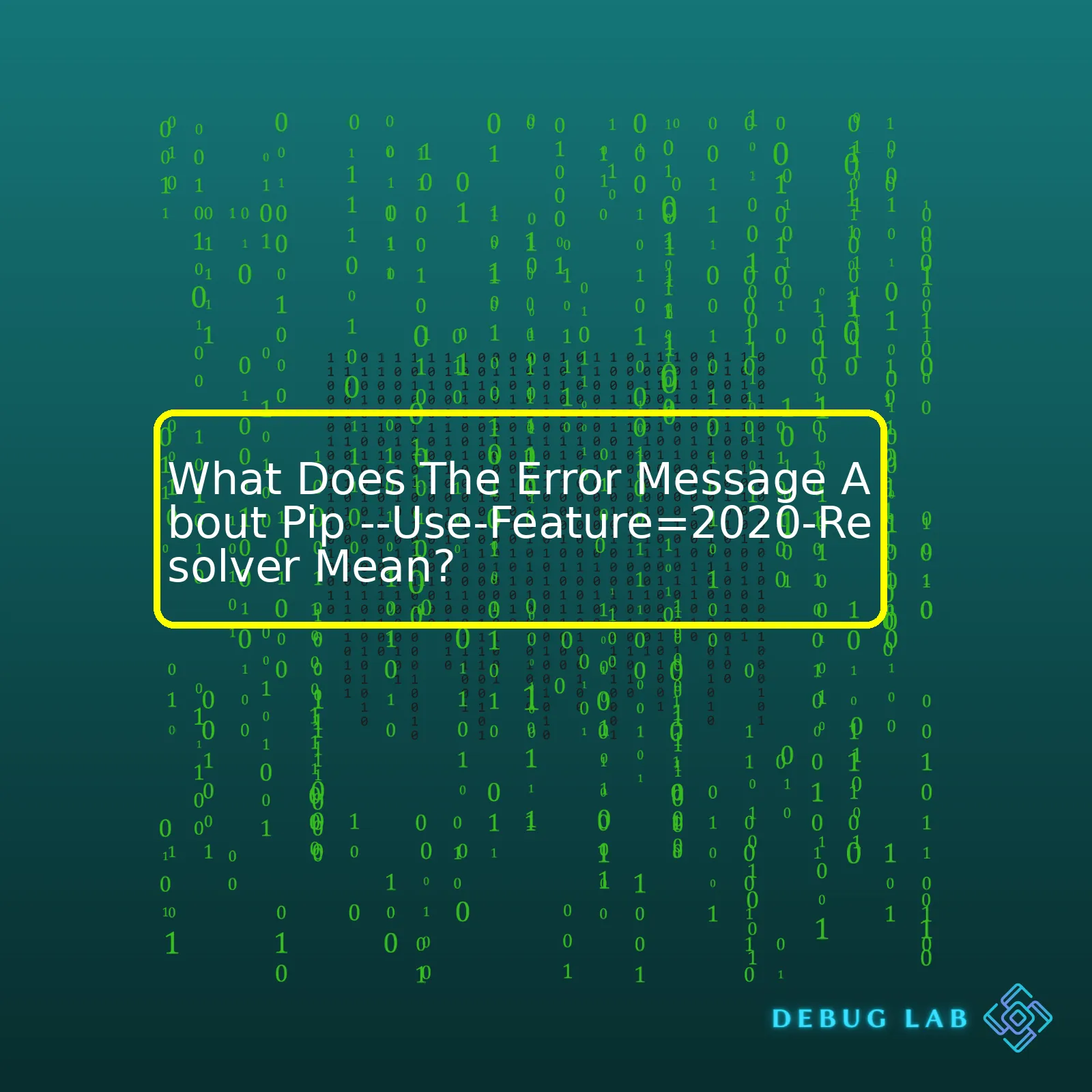
Error Message | Meaning | Solution |
---|---|---|
xxxxxxxxxx |
Points to a new resolution algorithm in pip. | Update to pip 20.3 or higher, you won't need to use it explicitly anymore. |
Error Message
Meaning
Solution
--use-feature=2020-resolver
Points to a new resolution algorithm in pip.
Update to pip 20.3 or higher, you won't need to use it explicitly anymore.
The error message
xxxxxxxxxx
--use-feature=2020-resolver
signifies that pip is trying to implement a new resolution algorithm planned for the version 20.3, which was introduced to address specific shortcomings of the earlier dependency resolver. This newer “2020-resolver” is more robust and can avoid cases where pip would previously get stuck in an infinite loop while trying to figure out package dependencies, particularly when there are conflicting ones.
However, some developers started to see this message upon running pip install commands because pip included the new resolver as an optional feature starting from version 20.2, hence the name ‘
xxxxxxxxxx
--use-feature=2020-resolver
‘. To give users ample time to test and adjust to the new resolver, pip initially kept both the old and the new resolver. Users had to add the flag
xxxxxxxxxx
--use-feature=2020-resolver
explicitly to opt into using the new resolver.
But now, pip 20.3 and onwards come with the new resolver by default and users do not need to use
xxxxxxxxxx
--use-feature=2020-resolver
anymore. If you’re still seeing this warning message, it must be because you haven’t updated pip. Update your pip to version 20.3 or higher and this message will no longer appear. However, do note the new resolver may cause issues if your package has a complicated set of dependencies. Always be cautious while upgrading your environments, and test adequately before updating in a production environment.
For more details, you can refer to the official Python information about this changeon their website here. You also find relevant information in the Pip documentation.
When you encounter an error message about
xxxxxxxxxx
pip ‐‐use‐feature=2020‐resolver
, it essentially means that you are dealing with a new feature of the pip Python package installer known as the dependency resolver. This feature, introduced in 2020 (which is why it’s called
xxxxxxxxxx
2020‐resolver
) is designed to evaluate and enforce the dependencies between packages, ensuring that all of these dependencies are met correctly.
xxxxxxxxxx
‐‐use‐feature=2020‐resolver
forces pip to use this new dependency resolution algorithm instead of the traditional one. It was created primarily to solve previously tricky problems where packages had conflicting dependencies([source](https://pyfound.blogspot.com/2020/07/new-pip-resolver-to-roll-out-this-year.html)).
The error relates to this kind of issue:
can't install package X, version Y because it depends on package A version B and you have package A version C installed which is incompatible This is an example of the sort of conflict that the new resolver was meant to handle, and by enforcing the usage of the new resolver, pip can ensure that all dependencies are met properly, and if not, provide helpful error messages. The error surfaces when there's a serious conflict which pip can't automatically resolve. Withoutxxxxxxxxxx
111--use-feature=2020-resolver
, pip might have tried to install/upgrade packages in a way that broke other packages. With this option, it's more cautious and requires user intervention instead.
Let’s illustrate this with a simplified code snippet:
xxxxxxxxxx
111pip install mypackage --use-feature=2020-resolver
Here, the line of code specifically instructs pip to use the aforementioned 2020 resolver feature while installing the theoretical "mypackage". If a dependency-related error occurs, pip will likely output a message indicating what the specific problem is, like our first example.
As of pip 20.3,
xxxxxxxxxx
111--use-feature=2020-resolver
is now the default behavior, so you probably won't be using this flag unless for some reason you need to revert to older behavior ([source](https://pip.pypa.io/en/stable/user_guide/#changes-to-the-pip-dependency-resolver-in-20-2-2020)).
So essentially, encountering a
xxxxxxxxxx
111pip ‐‐use‐feature=2020‐resolver
error means your attempt at installing or upgrading a Python package has run into issues regarding conflicts in package dependencies. The error prompts you to scrutinize your package requirements and make necessary corrections, once again confirming the importance of keeping clean, conflict-free dependencies in Python programming environments.Typically, the
xxxxxxxxxx
111Pip --use-feature=2020-resolver
error message appears when there's an issue with dependencies in your Python environment. This option was added in Pip 20.2 to offer users a simple way of enabling the new dependency resolver.
What is Pip's Dependency Resolver?
Pip's dependency resolver is a feature that determines how pip decides which versions of libraries or packages are compatible with your project. It analyzes all the installed and required packages and resolves any conflicts between them. The goal is to avoid incompatible versions of packages being installed together.
The
xxxxxxxxxx
111--use-feature=2020-resolver
switch forces pip to use its new, updated resolver.
Causes Behind The Error
In most cases, this warning or error is due to one of the following causes:
- Incompatible Package Versions: You might be trying to install a package version that conflicts with the version of another package you have installed. This could happen if one package depends on v1.x of another package, but you already have v2.x installed.
- Unfound Packages: Your Python environment may not find some required packages. These might be missing from Python Package Index (PyPI), or your configuration may be incorrect.
- Conflict with Local Libraries or Environment: If you are using virtual environments, any local/global site-packages may lead to conflicts causing such errors. Specifically, two different versions of the same library in different locations can cause trouble during the installation process.
- Error in the Setup Script: A bug in a setup script in a package could also potentially trigger this issue.
Source codes are not included for these explanations since these are logical concepts based on how Python and Pip function.
Resolving The Issue
Here's how you can generally approach resolving the
xxxxxxxxxx
Pip --use-feature=2020-resolver
error situation:
- Understanding the Details: Always start by closely examining the error message details. Pip will generally provide some insightful information within the traceback. The error generally includes the names of conflicting dependencies.
- Examining Installed Packages: You can run the command
xxxxxxxxxx
111pip freeze
to get a list of all currently installed packages and their versions. Compare these with the required packages and versions of your new module to identify any evident mismatches.
- Cleaning the Environment: If possible, try to clean your Python environment. Uninstall unwanted libraries and packages. You can even consider setting up a separate virtual environment for your specific project. This provides a cleaner installation process.
- Contacting Package Maintainers: If the conflict involves recent versions of widely used packages, it's worth checking their repositories for reported issues or contacting the maintainers. They should have better insights into the specific requirements and compatibility issues.
This should help clarify what the error message about pip --use-feature=2020-resolver means, why it appears and how you go about resolving it. Remember, while these steps work in many cases, there may be instances where further troubleshooting and detailed analysis of your specific Python environment is required.Firstly, it's essential to understand what the
xxxxxxxxxx
--use-feature=2020-resolver
message is all about. Python's package installer, Pip, introduced a new dependency resolver feature in 2020 to address some long-standing issues pertaining to how dependencies are resolved. While this new approach should largely improve the way dependencies are handled for most users, its potential impact and unexpected consequences resulted in widespread concerns, leading to plenty of interest in interpreting the error message.
Let's dive deeper into the logic behind the
xxxxxxxxxx
--use-feature=2020-resolver
switch, its impacts and the ramifications of any potential errors.
The primary change here is that the new 'resolver' is more strict in resolving dependencies compared to its predecessor. It installs dependencies based on their compatibility with all other requirements of your project. This can prevent certain bugs from manifesting themselves at runtime. However, sometimes it might generate an error related to conflicts between packages or versions which aren't immediately visible to you as a coder.
The error message concerning
xxxxxxxxxx
--use-feature=2020-resolver
typically arises when pip encounters conflicting dependency requirements that it cannot reconcile. A conflict may emerge due to diverse reasons like:
- packages specifying less accurate version details
- conflicting requirements imposed by different packages
- dependencies that need a particular version of a package.
These conflicts impede pip from finding a suitable combination satisfying all necessities, which results in the mentioned error.
Here's a common structure of the error message associated with
xxxxxxxxxx
--use-feature=2020-resolver
.
xxxxxxxxxx
The conflict is caused by:
The user requested ...
... is provided by ...
Could not find a version that aligns with these requirement constraints.
Astute analysis of the error message often provides clear insights into the conflicted modules and their respective versions. For instance, if different parts of your application require different, incompatible versions of the same library, this error can point out the discrepancy clearly.
So, what does this mean for you as a developer? One of the main impacts would be having to invest time in hunting down these compatibility issues between packages already used in your applications, but it also provides benefits:
- You gain better predictability and security in terms of your project's dependencies.
- It forces rigorous package management, prompting you to pick reliable, updated packages.
- You avoid runtime bugs that can arise from unresolved or improperly resolved dependencies.
Despite the possible upfront difficulties, it encourages good practice in managing your application's dependencies leading to dependable, bug-free code. Explicit error messages will provide clarity about problematic packages, making your troubleshooting tasks significantly easier.
Remember, the official pip documentation provides a comprehensive guide about the resolver and how to handle potential issues during the transition period. Resources from experienced Python coders on platforms such as StackOverflow can be referenced to help navigate through complex dependency issues.
For instance, here's a simple way to check what packages create conflicts inside your virtual environment:
xxxxxxxxxx
pip check
In many cases, updating the relevant packages to their latest versions often helps resolve the discrepancies. However, do keep in mind that each case is unique, and some situations may demand different solutions.
This fundamentally shifted paradigm has turned a previously less severe issue (improperly handled dependencies) into an explicit problem that requires immediate attention. While it may initially seem inconvenient, it has a positive long-term effect - encouraging developers towards a solid foundation for robust code and well-managed dependencies.If you've ever executed a Python pip command and encountered the error
xxxxxxxxxx
Pip --Use-Feature=2020-resolver
, it could have left you puzzled. But fret not, we are here to decode this for you!
The
xxxxxxxxxx
--use-feature=2020-resolver
flag is a feature preview introduced in pip version 20.2. It acts as the new resolver's switch, allowing pip to use a different strategy when identifying package dependencies (requirements). However, why does it display an error?
Firstly, let’s understand what pip does. Pip is a popular package installer for Python. It is responsible not only for installing individual packages but also for managing a complex network of interdependencies between these packages. Now, if pip encounters a problem whilst trying to resolve these dependencies, it will log out an error message. Presented with the
xxxxxxxxxx
--use-feature=2020-resolver
error, one can ascertain that there's a conflict with the installation or upgrade process in Python‘s package ecosystem.
In response to such conflicts, pip might even fall back to a default ‘first come, first served‘ solution approach, which could possibly result in malfunctioning software due to unsuitable package version combinations. Therefore, the 2020 Resolver upgrade purported to reduce the chance of installing incompatible package versions by rejecting certain installation requests in situations where it identifies unresolvable conflicts.
Now that we have a base understanding of
xxxxxxxxxx
--use-feature=2020-resolver
, let's hone in on some key troubleshooting tactics:
1. Check Python Version: Ensure the Python version you're working with is up to date. While pip attempts to keep up with all Python version updates, using the most recent version of Python provides maximum compatibility.
Command to check python version:
xxxxxxxxxx
python --version
2. Update Pip: Inspect your pip version by executing
xxxxxxxxxx
pip --version
. If it's not the latest version, conduct an upgrade with
xxxxxxxxxx
python -m pip install --upgrade pip
. The 2020-resolver is a pretty recent feature and won't work with older pip versions.
3. Review Python Packages: Go through the current packages installed in your system by running
xxxxxxxxxx
pip list
or
xxxxxxxxxx
pip freeze
. This allows you identify the packages causing dependency conflicts via their versions, further assisting you in troubleshooting.
4. Simplify Requirements: If there exist too many dependencies, simplifying your requirements.txt file might be beneficial. Keep the list as minimal as possible, including only primary packages required; pip will automatically handle the auxiliary ones.
While these steps generally take care of the issue, it's important to mention that issues related to package dependency are dependent (no pun intended) on the types of packages involved. Hence, specific cases may require more nuanced solutions[^1^].
[^1^]: Read more about Pip’s dependency resolution
Ultimately, the goal remains to enhance and streamline the development experiences within Python, and tackling errors like
xxxxxxxxxx
--use-feature=2020-resolver
greatly contribute towards it. As a coder, understanding these complexities beneath the simple commands fundamentally elevates your skills and adaptability.
Yes, this is a fascinating element. The pip --use-feature=2020-resolver error message primarily means that there's an issue with how pip is attempting to handle dependencies in your Python project. Even more so, it seeks to illuminate any conflicts between different versions of the same package required by multiple other packages. When encountered, it can pose quite the challenge, but thankfully, there are ways to prevent and resolve this.
To better comprehend what's happening, let's delve into the pip resolver feature, which was updated back in 2020.
The
xxxxxxxxxx
--use-feature=2020-resolver
flag is part of a significant pip change brought about last year to enhance how the package manager resolves package dependency conflicts. Before this modification, pip would install packages in the order they were found in the requirements file, even if later packages negated the dependencies installed earlier. This created problematic discrepancies and conflict scenarios. The 2020 resolver update was hence implemented to improve the installation order and constraints, making pip smarter when figuring out dependency resolution.
Now, when faced with the pip --use-feature=2020-resolver error, there exist some key prevention strategies:
1) Regularly Update Packages:
Promoting regular updates to your packages enables you to stay current with your dependencies. This act seems subtle, yet it lessens the chances of running into dependency problems or conflicts significantly.
2) Use Virtual Environments:
Creating isolated environments for each of your Python projects limits dependency clashes caused by different projects requiring diverse versions of the same package. You can use tools like venv or virtualenv to manage these dedicated spaces.
For instance, to create a virtual environment using venv, you would use the following command:
xxxxxxxxxx
python3 -m venv /path/to/new/virtual/environment
And to activate it:
On Unix or Linux:
xxxxxxxxxx
source /path/to/new/virtual/environment/bin/activate
On Windows:
xxxxxxxxxx
\path\to\new\virtual\environment\Scripts\activate
3) Specify Exact Package Versions:
Also, consider declaring exact package version numbers in your requirements file. By doing this, you control the versions installed by pip, mitigating the likelihood of receiving the --use-feature=2020-resolver error. It can be as simple as the example below in your requirements.txt file:
xxxxxxxxxx
numpy==1.19.2
pandas==1.1.3
matplotlib==3.3.2
If you've taken all these steps into account and you're still encountering the --use-feature=2020-resolver error, I'd recommend examining the full error message and logs delivered by pip. These logs provide specific details about which packages and version conflicts are causing the problem. You can then utilize this information to understand where exactly the conflict arises from and seek a suitable solution.The error message about pip `--use-feature=2020-resolver` typically means there are incompatible dependencies within your Python project. Essentially, pip is having difficulty figuring out which versions of which libraries can live together peacefully.
In August 2020, with the introduction of Pip version 20.2, a new resolver was released that was intended to be less flexible than its predecessor, with the goal of reducing bugs arising from incompatible libraries.
So when you see this error message, it's telling you that pip has detected a possible issue with the way your libraries are interacting and it wants you to use the 2020-resolver feature to help sort it out.
While this might sound intimidating if you've never encountered it before, do not fear; taming the beast is simple once you understand what it does.
But before I provide the steps on how to use pip’s `--use-feature=2020-resolver`, let’s look at what exactly the Resolver does:
- The Resolver attempts to simplify dependency management by automatically determining which software versions are compatible with each other.
- Instead of just installing requested packages like older versions of pip, Resolver digs deeper into these packages’ dependencies as well.
- Ultimately, Resolver is designed to ensure the entire dependency tree of a project is consistent in terms of package versions.
Now, let's go through how to correctly use pip’s `--use-feature=2020-resolver` feature:
1. Open your command line prompt. This could be Terminal for macOS users, Command Prompt for Windows users, or any substitute that you prefer.
2. Make sure you're using the correct version of pip. To check your pip version, use the following command:
xxxxxxxxxx
pip --version
If you're not running pip version 20.3 or above, you'll need to upgrade. To do so, use the following command:
xxxxxxxxxx
python -m pip install --upgrade pip
3. Once your pip is upgraded, you can use the 2020-resolver feature. Just add `--use-feature=2020-resolver` at the end of your usual pip commands. For example:
xxxxxxxxxx
pip install pandas --use-feature=2020-resolver
4. Upon executing the command, pip will perform an additional step of checking for inconsistencies among all dependencies of the package specified before installation. If any inconsistencies are discovered, pip will raise an error detailing the version conflicts.
5. To fix conflicting dependencies, you would usually need to manually adjust the versions of the problematic packages.
You should now better understand what it means when pip asks you to use the 2020-resolver feature, and feel comfortable doing so. In fact, using pip’s `--use-feature=2020-resolver` could save you from facing bigger headaches down the road caused by pesky library incompatibilities.
Of course, if you still encounter errors, consider reviewing the official Pypi documentation on [how to manage package dependencies](https://packaging.python.org/guides/managing-dependencies-in-python/).
When you come across the error message relating to `pip --use-feature=2020-resolver`, it is typically because pip, Python's default package manager, received a significant update in 2020. This update introduced something known as pip's 'resolver', which has direct implications for Python's dependency management.
Python dependency management refers to how Python handles different packages that your codebase needs to execute successfully. These packages often depend on other packages, forming a tree-like structure. For example, if you install Django (a high-level Python Web framework), it would require pytz (a Python library to deal with timezones). So, pytz would be a dependency of Django.
Previously, pip did not have a strong resolution mechanism. This meant that it would cheerfully install and upgrade packages, sometimes causing problems when different packages had conflicting dependency requirements. In the worst-case scenarios, this could even break your project.
This is where Pip’s new dependency resolver comes into play:
- The new resolver: Following PEP 458 and PEP 480, pip's new resolver was introduced to rectify issues from pip's old resolver. Now, before installing packages, it tries to understand all the packages that are dependent, verifies versions and ensures they are compatible, thus avoiding any conflicts.
- Error Code (--use-feature=2020-resolver): The flag `--use-feature=2020-resolver` was a user option given during an intermediate period to switch between the old and the new resolver. This helped users gradually adapt to the new resolver before it became the default in pip 20.3. Therefore, if you are seeing this error, it suggests that you're using a flag or version of pip where the new resolver isn't available by default.
So, what do you do about this error message? You'll need to simply upgrade your pip to version 20.3 or above, where the `--use-feature=2020-resolver` warning is unnecessary as the new resolver is used by default. To upgrade pip, just run the following command line instruction:
xxxxxxxxxx
python -m pip install --upgrade pip
Having done so, the error message regarding `pip --use-feature=2020-resolver` should no longer occur, and pip will use the new resolver to handle dependencies more efficiently and avoid conflicts between packages in your Python projects.
For more details, check out pip's official guide on the changes to the pip dependency resolver.As a developer, seeing a cryptic error message like
xxxxxxxxxx
Pip --use-feature=2020-resolver
can certainly throw you off track. But fear not, this particular message isn't as daunting or complex as you might initially think. It's all about understanding the underlying issue and addressing it accordingly.
Essentially, the
xxxxxxxxxx
pip --use-feature=2020-resolver
, relates to a new resolver feature introduced by Python’s pip as part of its version 20.3 release. Python packages world often requires the installation of numerous interconnected and dependent packages, which is where this new resolver shines. Its primary role is to determine the compatibility amongst these related dependencies during an installation process.
A notable feature of this new resolver is that it will refrain from installing incompatible packages, which significantly reduces any potential conflicts that may otherwise arise. This stark contrast from previous versions of pip installations, which wouldn’t prevent the installation of conflicting packages, ultimately leading to troublesome situations.
If you’re encountering the
xxxxxxxxxx
pip --use-feature=2020-resolver
error message, it implies that there are some issues with package dependencies in your current Python environment setup. Addressing such errors involves troubleshooting each problem one at a time:
Consider running
xxxxxxxxxx
pip check
to check for discrepancies.
Fix detected inconsistencies using
xxxxxxxxxx
pip install
or
xxxxxxxxxx
pip uninstall
.
Look into the documentation of various conflicting packages to find compatible versions.
Or even isolate your project dependencies through virtual environments.
Remember - Stack Overflow1 is a fantastic place to get help if you're still stuck or unsure on what the error means or how to fix it. Even better, this community platform often comes forward with ample solutions provided by developers worldwide who faced the same challenges. So don't panic! Reach out, research, test, learn and overcome!
Moreover, it's important to understand that getting error messages are totally normal, especially in the world of coding. They should not daunt or deter you, but instead seen as a learning experience and a chance to improve your debugging skills. Embrace them and use every opportunity they present to become a better coder. The more you know about errors like
xxxxxxxxxx
pip --use-feature=2020-resolver
and their resolutions, the easier and faster it'll be to crack any problems they may bring along next time.
Relevant references:
Stack Overflow | www.stackoverflow.com |
Pip Documentation | https://pip.pypa.io/en/stable/user_guide/ |
Sample codes referred:
xxxxxxxxxx
pip check
pip install {packageName}
pip uninstall {packageName}