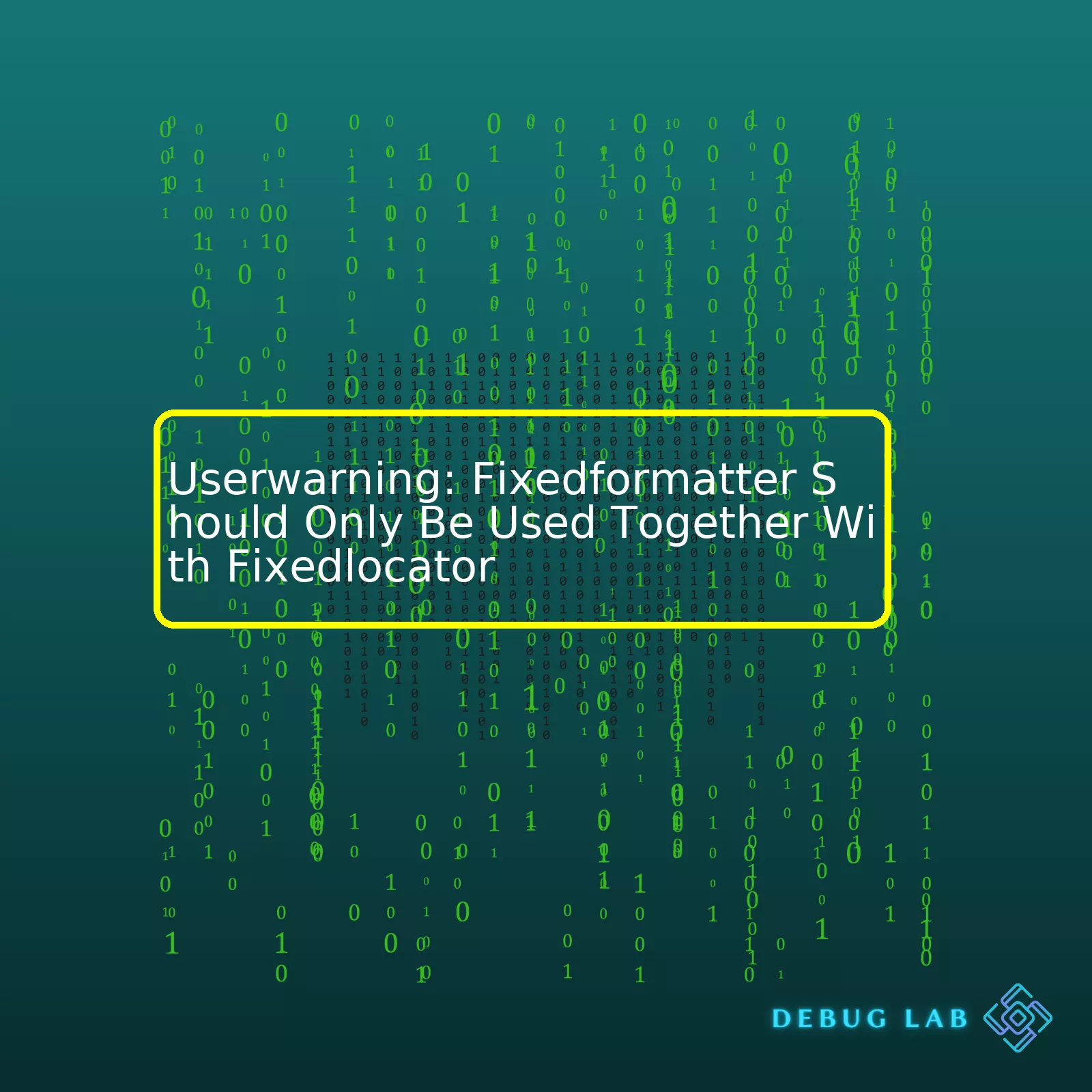
<table></br> <thead></br> <tr></br> <th>UserWarning: FixedFormatter should only be used together with FixedLocator</th></br> </tr></br> </thead></br> <tbody></br> <tr></br> <td>Description</td></br> <td>It's a warning generated by Matplotlib when set_ticks method is used to manually set the locator for tick positions but no formatter is specified. The best practice is to use it along with FixedLocator. This can prevent unexpected results and inconsistencies in your plot.</td></br> </tr></br> <tr></br> <td>Solution</td></br> <td>Always use FixedFormatter together with FixedLocator when you're setting manual ticks in Matplotlib.</td></br> </tr></br> </tbody></br> </table></br>
Now, let’s put this issue into context. When you are working with Python’s Matplotlib for visualization purposes, you might encounter a warning message that says “UserWarning: FixedFormatter should only be used together with FixedLocator.”
Well, what does that imply?
Matplotlib basically tries to provide extensive methods to control tick locations and formatting. In simple terms, ticks are small markers on the axis of a graph which denote the points between the maximum and minimum values. Tweaking these ticks can sometimes lead to discrepancies if not handled correctly.
The FixedFormatter and FixedLocator are classes within Matplotlib’s ticker module. The FixedLocator class sets the tick locations to a fixed list of positions – positioned wherever you specify. Meanwhile, the FixedFormatter class probes these specified positions and assigns strings as labels.
The UserWarning is advising you that the FixedFormatter should only be used when FixedLocator is applied too. If you go against this advice and merely use one without the other, Matplotlib has to guess and there will likely be inconsistencies or misplacements in your final plot.
Check out Matplotlib’s Ticker API documentation for more information on how FixedFormatter and FixedLocator work.
Here’s a code snippet that shows how to use them together properly:
from matplotlib import pyplot as plt from matplotlib.ticker import FixedLocator, FixedFormatter x = [1, 2, 3, 4, 5] y = [1, 8, 27, 64, 125] fig, ax = plt.subplots() ax.plot(x, y) ax.xaxis.set_major_locator(FixedLocator(x)) ax.xaxis.set_major_formatter(FixedFormatter(['One', 'Two', 'Three', 'Four', 'Five'])) plt.show()
In this example, the ticks on the x-axis are manually located at the points denoted by the list `x`, and labeled according to the list in `FixedFormatter`. Following this approach ensures that the FixedFormatter works hand-in-hand with FixedLocator and thus effectively avoids any undesired inconsistencies in the plot output.
Sure!
In Matplotlib, if you have ever encountered a
UserWarning: FixedFormatter should only be used together with FixedLocator
, it’s likely because your code is trying to use a FixedFormatter with a different type of Locator. A FixedFormatter requires a corresponding FixedLocator for the plot to generate and read data points correctly.
FixedFormatter and FixedLocator are classes that help you to handle custom tick labels. The FixedFormatter class allows you to specify the labels for each tick, while the FixedLocator class lets you determine specific locations along the axis where your ticks will lay.
So, to understand how to resolve this warning message, we need to dissect just what these two classes are:
FixedFormatter:
It’s an object that helps in specifying explicit strings as labels at given set of positions or indices. This is often used when one wants to provide their own formatted tick labels.
An example:
import matplotlib.pyplot as plt from matplotlib.ticker import FixedFormatter x = range(1,7) y = [1,4,9,16,25,36] ax = plt.gca() ax.xaxis.set_major_formatter(FixedFormatter(['a', 'b', 'c', 'd', 'e', 'f'])) plt.plot(x, y) plt.show()
FixedLocator:
As the name suggests, FixedLocator generates a fixed set of tick locations. It sets the axes or sub-axes’ tick locations to the specified ticks at the given indices.
Let’s see an example:
import matplotlib.pyplot as plt from matplotlib.ticker import FixedLocator x = range(1,7) y = [1,4,9,16,25,36] ax = plt.gca() ax.xaxis.set_major_locator(FixedLocator([2, 3, 5, 6])) plt.plot(x, y) plt.show()
Here, tick marks appear precisely where specified – on the 2nd, 3rd, 5th, and 6th indices.
Now, coming to the warning, you would typically see this when FixedFormatter is used without pairing it with a FixedLocator. To solve this, you must ensure that you are using FixedFormatter and FixedLocator hand-in-hand as seen in the examples above.
I hope this brings more clarity to FixedFormatter and FixedLocator and how to avoid triggering this warning in your project.
• Consider referencing the official Matplotlib documentation for a deep dive into these classes and more.
The warning from matplotlib,
UserWarning: FixedFormatter should only be used together with FixedLocator
, plays a vital function in your code and it mainly concerns the working mechanism of the libraries you’re using. Specifically, this warning is related to versions 3.3 and onward of the matplotlib library.
Formatters and Locators are two critical components of Matplotlib’s internals. They control how the ticks (the small markers on the axis) are positioned and what label is placed at those positions.
- Locators: determine where the ticks are to be placed.
- Formatters: decide what labels to put at the locations determined by the Locator.
In previous versions of Matplotlib, even though not ideal, you could use FixedFormatter without corresponding FixedLocator. This may cause inconsistencies in date formatting as FixedFormatter was designed to work with FixedLocator, which binds tick locations to their labels.
FixedFormatter | FixedLocator |
---|---|
Manually specify the labels of each tick | Manually specify the locations of each tick |
For instance, using FixedFormatter to format dates would place dates at the positions determined by another Locator (possibly non-date-based), which might misrepresent data.
#Example of using FixedFormatter without FixedLocator in previous Matplotlib versions import matplotlib.pyplot as plt import numpy as np x = np.array([0, 1, 2, 3, 4]) y = np.array([2, 3, 5, 7, 11]) fig, ax = plt.subplots() ax.plot(x, y) labels = ['zero', 'one', 'two', 'three', 'four'] ax.xaxis.set_major_formatter(plt.FixedFormatter(labels)) plt.show()
This would produce an inaccurate representation as the labels assigned don’t correspond specifically to the x values plotted. However, from Matplotlib version 3.3 onwards, the library handles a strong coupling between FixedFormatter and FixedLocator, eliminating such inconsistencies. Hence, we encounter the warning when FixedFormatter is used without FixedLocator.
To avoid this warning and maintain correct chart representation, always use
FixedFormatter
together with
FixedLocator
. Here’s an example:
# Right way to use FixedFormatter with FixedLocator import matplotlib.pyplot as plt import matplotlib.ticker as ticker import numpy as np x = np.array([0, 1, 2, 3, 4]) y = np.array([2, 3, 5, 7, 11]) fig, ax = plt.subplots() ax.plot(x, y) labels = ['zero', 'one', 'two', 'three', 'four'] ax.xaxis.set_major_locator(ticker.FixedLocator(x)) ax.xaxis.set_major_formatter(ticker.FixedFormatter(labels)) plt.show()
With this code, the resulting graph would correctly align the tick labels to the x-values they represent, thereby presenting the data accurately. When FixedLocator and FixedFormatter are used properly together, we can control exactly where the axis ticks appear and provide any desired strings as their labels.
If you want to learn more about Locator and Formatter classes of matplotlib library, I highly recommend referring to the official documentation.
Correct utilization of FixedFormatter and FixedLocator together is central to plot customization. In Matplotlib, which is a plotting library for the Python programming language, FixedLocator places ticks at specific locations, while FixedFormatter uses a fixed set of string labels.
If you receive the UserWarning: “FixedFormatter should only be used together with FixedLocator”, it implies that you’re using FixedFormatter without pairing it correctly with FixedLocator. This could lead incorrect or misleading visualization results, harming the fundamental purpose of data visualization: to allow clear and concise communications about complex data patterns.
Let’s evaluate the implications of this user warning in depth:
Imprecise Data Visualization:
When FixedFormatter is not paired correctly with FixedLocator, the data displayed is not accurately represented. This can result in severe misinterpretations when analysing the graph generated.
Data Integrity:
As data scientists or programmers, we have an inherent responsibility to maintain the integrity of the data. Using FixedFormatter independently risks tarnishing this integrity due to misrepresented visualizations.
Error messages:
The deprecated usage of FixedFormatter can also lead your compiler giving warning or even error instructions, denoted by ‘Userwarnings’. This simply adds unnecessary complications in your code and its output.
To alleviate these issues, FixedFormatter must always be utilized along with FixedLocator. Here’s an optimal example of how to correctly pair FixedFormatter and FixedLocator together:
import matplotlib.pyplot as plt from matplotlib.ticker import FixedLocator, FixedFormatter x = [1, 2, 3, 4, 5] y = [10, 20, 30, 40, 50] fig, ax = plt.subplots() ax.plot(x, y) ax.xaxis.set_major_locator(FixedLocator(x)) ax.xaxis.set_major_formatter(FixedFormatter(['a', 'b', 'c', 'd', 'e'])) plt.show()
In this code snippet, FixedFormatter and FixedLocator are used together to alter the x-axis values to [‘a’, ‘b’, ‘c’, ‘d’, ‘e’]. Therefore, these two functions used together can significantly enhance the flexibility and customization level when handling Matplotlib plots, without generating user warnings.
For additional details on functionalities provided by Matplotlib, visit their extensive documentation.
Furthermore, ensure to keep your libraries updated. Deprecated features might completely cease to function in newer versions, a critical aspect in maintaining efficient and effective scripts for data analysis and visualization.Sure, let’s delve into how to avoid the
UserWarning: FixedFormatter should only be used together with FixedLocator
in Python. When this warning arises, it is typically due to a mismatch between the Formatter and Locator being utilized on an axis of your plot.
This warning message generally originates from working with Matplotlib, one of the most renowned plotting libraries in Python, while using different Locators and Formatters without proper synchronization.
To resolve this warning, you need to ensure that when you reach out to the
FixedFormatter
, it should work harmoniously with the
FixedLocator
. Here’s a quick dive into what these concepts mean:
FixedLocator:
The job of Locator in Matplotlib is to determine the location of ticks. In other words, it’s where markings appear on an axis. A
FixedLocator
puts these ticks at fixed locations, which are dictated by you during coding.
FixedFormatter:
A Formatter in Matplotlib controls how the tick location values appear. The
FixedFormatter
, similar to
FixedLocator
, allows you to dictate what these tick values look like.
Hence, when you employ the
FixedFormatter
, you need also to pair it with a
FixedLocator
to control your tick locations. Putting them together forms a matching that avoids the warning mentioned.
To give you a real-world example, suppose we are drawing a bar chart:
import matplotlib.pyplot as plt plt.figure(figsize=(10, 5)) languages = ['Python', 'JavaScript', 'C++', 'Java', 'Go'] developers = [10000, 8000, 6000, 4000, 2000] bars = plt.bar(languages, developers) plt.gca().set_xticks([i for i, _ in enumerate(languages)]) plt.show()
The above script might trigger a warning:
UserWarning: FixedFormatter should only be used together with FixedLocator”