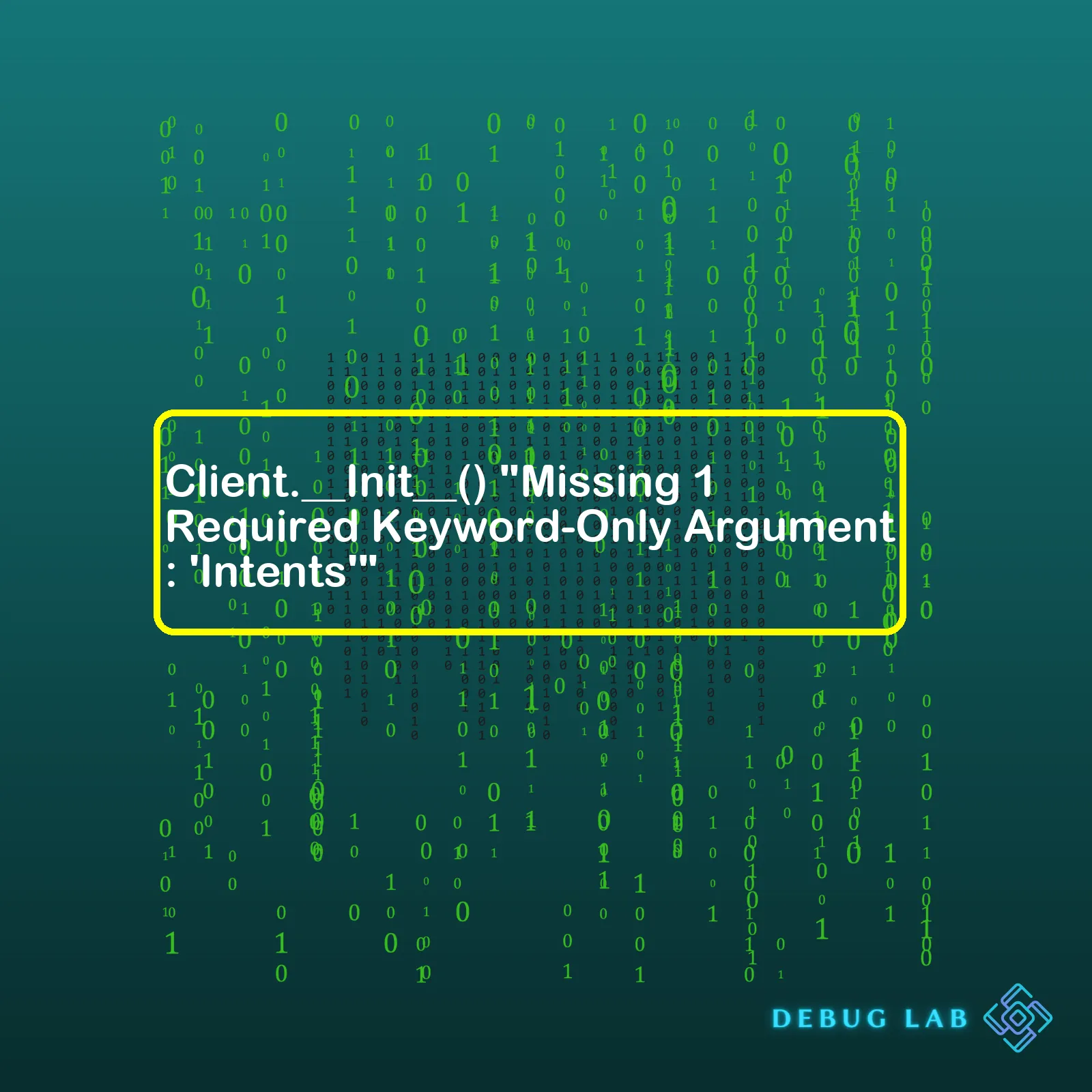
Method | Error | Description |
---|---|---|
client = discord.Client() |
“Missing 1 Required Keyword-Only Argument: ‘Intents'” | This error occurs because an instance of the Discord Client was being created without providing the required Intents keyword argument. As of discord.py version 1.5.x, it is not possible to instantiate the discord.Client() or discord.ext.commands.Bot() classes without specifying intents. |
In discord.py, Intents are used to subscribe to certain types of events. These events can include guild updates, member updates, and more. With the release of discord.py version 1.5.0 and above, some gateway intents became necessary, meaning they must be enabled for your bot to receive certain events. That’s why trying to initialize the `discord.Client()` class without specifying the `intents` argument will result in a MissingArgument Exception being thrown with the message “Missing 1 Required Keyword-Only Argument: ‘Intents'”. The Python code snippet below shows how you should correctly initialize the Discord client:
import discord intents = discord.Intents.default() client = discord.Client(intents=intents)
Here, we first import the discord module. Then we call
discord.Intents.default()
to get a new Intents object with all default intents enabled. Finally, we use this object as the value for the `intents` keyword argument when creating a new instance of the discord.Client class.
When you see the error
Client.__init__() missing 1 required keyword-only argument: 'intents'
, it suggests that your code didn’t provide a necessary Intent object while instantiating a discord.Client or discord.Bot.
The Intents system is implemented in Discord API to control the events received by bots. It was introduced with the intention of optimizing bot performance and ensuring better safety. Essentially, “intents” allow Discord to save computational resources by just emitting events the bot declared.
Segmenting into bullet points for broad understanding:
- Intents are part of the discord API.
- They demonstrate what events a bot can receive.
- The introduction of intents came from a scaling perspective so only needed events would be listened to.
- A discord bot will not function without defining its intent.
The issue rises when you’re trying to make an instance of either discord.Client or discord.bot. The constructor (__init__()) of these classes now requires an ‘intents’ argument. Discord made this change recently and older programs that have not been updated since prior to these changes may encounter this error.
Check out this code snippet for further clarification.
Older version:
client = discord.Client()
Revised version that includes an “Intents” object:
intents = discord.Intents.default() client = discord.Client(intents=intents)
In the above code, we initialized a new Intents object using default settings with
discord.Intents.default()
. We passed the created object as an argument while creating the Client. You should review all use cases of discord.Client() and discord.Bot() in your program code and update them as illustrated.
For more information on intents and their effect, consider visiting the official discord.py documentation section titled “Privileged Intents”.
This error is crucial to resolve as Discord won’t let bots function without declaring what kind of events they require. By being explicit about intents, we help optimize the bot’s and ultimately Discord’s performance.
Remember, coding, especially in sophisticated libraries such as discord.py, often requires developers to keep up with abundant updates to avoid similar types of errors and to enhance overall performance.You’re seeing the error “Client.__Init__() “Missing 1 Required Keyword-Only Argument: ‘Intents'” because newer versions of discord.py (version 1.5 onwards) started enforcing the use of
intents
when initiating a client for your bot. Intents provide you a measure to specify which events your bot has access to.
To resolve this issue, you need to add the missing intents. There is an exhaustive list of intents that can be used depending on specific needs, some of them being:
-
Guilds
-
Members
-
Bans
-
Emojis
-
Messages
-
Reactions
Here’s how you can implement all intents in order to prevent the current and any future permissions related issues:
from discord import Intents from discord.ext import commands intents = Intents.all() #Enabling all intents bot = commands.Bot(command_prefix="!", intents=intents) ...
In the above example,
Intents.all()
includes all available intents. This could potentially result in substantial data traffic depending on your number of guilds. Therefore, it may be beneficial to only enable the intents that are necessary for your bot.
For instance, if you’re only reading messages or tracking reactions, just enabling those might be more suitable:
from discord import Intents from discord.ext import commands intents = Intents(messages=True, reactions=True) bot = commands.Bot(command_prefix="!", intents=intents) ...
Lastly, adding these snippets won’t necessarily solve the problem if the corresponding intent isn’t enabled on Discord’s Developer Portal. To do so:
- Go to the Discord Developer Portal
- Select your bot, move to the Bot section
- Scroll down to Privileged Gateway Intents
- Enable the intents that you added in your code
However, keep in mind that for larger bots (100 or more servers), using privileged intents might require a verification process from Discord.
Ensure that you are using intents according to the privacy policy defined by Discord.
Further documentation for Intents usage can be found over here: Read The Docs – Intent Usage.
Solving the “Client.__Init__() Missing 1 Required Keyword-Only Argument: ‘Intents'” error involves two key steps – updating the Python code with appropriate intents and enabling those intents in the Discord Developer Portal accordingly.Okay, let’s shed some light on Python function arguments and parameters with a focus on the error: “missing 1 required keyword-only argument: ‘intents'”.
Functions in Python are defined using the
def
keyword followed by the
,
()
brackets containing any parameters the function needs to receive and a colon
:
.
An example of this is:
def my_function(my_param): print(my_param)
Here,
my_param
is a parameter that my_function needs to operate properly.
When we call
my_function
, we need to provide an argument for this parameter.
my_function("Hello World")
However, when dealing with more sophisticated libraries or functionalities, more advanced ways of handling functions may be employed – such as keyword-only arguments. As their name implies, these are arguments that must be assigned a value in the function call via a specific keyword assignment.
The error message you’re encountering, “Missing 1 Required Keyword-Only Argument: ‘Intents'” says that the Client class’s __init__() method requires a keyword-only argument with the keyword ‘intents’.
This typically happens while using the discord.py library. To instantiate a client object in discord.py, it might look something like this:
client = discord.Client()
However, with new versions of discord.py library (1.5+), an intents system has been imposed due to privacy policy changes made by Discord. Now, to use certain functionalities within your bot, you have to specify your intent.
A basic instantiation with the intent to use all privileged intents can look like this:
intents = discord.Intents.all() client = discord.Client(intents=intents)
Here, we first capture all possible Intents via
discord.Intents.all()
. Then, we provide it as a keyword-only argument to the Client.
Note however, that you should only request the intents you need to avoid unnecessary data usage. Also, certain Intent usages might require your bot verification and whitelisting from Discord’s end if it’s in more than 100 guilds.
For a deeper dive into this topic, check the official Discord Py Documentation dealing with intents put together by the experts themselves. It will provide further insights and practices about using Intents.When working with Discord APIs, one might be mistakenly trying to call the
discord.Client()
method instead of the
discord.Intents()
method and end up with an error message that says something like “Missing 1 required keyword-only argument: ‘intents'”. In this context, the word ‘Intents’ refers to a mechanism in the Discord API that allows your bot to subscribe to certain events.
To comprehend the principle of ‘Intents’, you need to understand that, as a way to reduce load on their servers, Discord introduced something called “Gateway Intents” which allows bots to choose which events they want to register for. This became mandatory as of October 7, 2020.
Here’s an example: let’s say you’ve coded a bot that doesn’t need any information about when guild members are updated. In that case, why should Discord send updates about guild members to your bot? It shouldn’t! And with gateway intents, it won’t.
Coming back to our problem, it appears when we’re trying to instantiate a client object without providing intents.
import discord client = discord.Client()
To fix this issue, we’ll need to provide an instance of the ‘Intents’ class while creating a Client instance:
import discord intents = discord.Intents.default() client = discord.Client(intents=intents)
The
discord.Intents.default()
method returns a new instance of Intents enabled for all the events which do not require opting-in (the same prior behavior).
However, if you wish to limit the scope of your bot or require privileged intents (currently
GUILD_MEMBERS
and
GUILD_PRESENCES
) you will need to specify those upon instantiation.
import discord intents = discord.Intents(guilds=True, messages=True, ...) client = discord.Client(intents=intents)
Bear in mind, “Privileged” intents need to be enabled in the Developer Portal under “Bot” -> “Privileged Gateway Intents” section as illustrated in this screenshot Discord Developer Portal Settings.
You can find more detail about each type of Intent in the Discord Developer documentation here. Understanding intents efficiently is crucial as enabling all intents isn’t strategically wise due to the load it puts on both ends, nor is it usually necessary. Instead, predicates such as messages=True or reactions=True would enjoyably benefit your functionality without redundant data interference.
When you’re faced with an error like
Client.__Init__() "Missing 1 Required Keyword-Only Argument: 'Intents'"
, you are likely working with a function in Python that has both positional and keyword-only arguments. Understanding the differences between these two types of function arguments can help us navigate this error message effectively and correctly use functions in our Python programs.
Differences Between Positional and Keyword-Only Arguments:
In Python, you can pass arguments to a function in several ways. Two of the most common forms are positional arguments and keyword-only arguments.
Let’s dive into positional arguments first:
– Positional arguments: These are the simplest and most common type of argument. The value of the argument is based on its position within the function call.
Here’s an example of positional arguments in a function:
def greet_person(firstname, lastname): print(f"Hi there, {firstname} {lastname}")
When calling this function, the order of your inputs matters:
greet_person("John", "Doe") # prints "Hi there, John Doe"
Now let’s talk about using keyword-only arguments and examine how they differ.
– Keyword-only arguments: This type of function argument is distinctly different from positional arguments. Keyword-only arguments need to be entered in the function call by specifying the variable name where the input belongs.
Look at the example below, using the same greeting function but refactoring it to require keyword-only arguments.
def greet_person(*, firstname, lastname): print(f"Hi there, {firstname} {lastname}")
Now if you try calling it as we did previously, you’d get a TypeError similar to the one you received:
greet_person("John", "Doe") # ERROR: greet_person() takes 0 positional arguments but 2 were given
To correctly call this function, you would need to specify the argument names and their values:
greet_person(firstname="John", lastname="Doe") # prints "Hi there, John Doe"
Missing Required Keyword-Only Argument Error:
The error message
Client.__Init__() "Missing 1 Required Keyword-Only Argument: 'Intents'"
indicates that the function being called requires an additional keyword-only argument named ‘Intents’ that hasn’t been provided.
Within the context of Discord bots in Python, “Intents” usually refers to certain functionalities a bot must have enabled to perform specific tasks. To fix this error, you would have to provide this required keyword-only argument when initializing the Client(). You can know more about this feature from the official
Discord.py documentation.
from discord import Intents from discord import Client intents = Intents.default() client = Client(intents=intents)
By understanding the difference between positional and keyword-only arguments, we can better navigate function declarations and calls in Python, allowing us to bridge the gap between intent and execution of code.The ‘missing 1 required keyword-only argument’ error is typically triggered when we call a function without the necessary keyword arguments. The specific error you are encountering is
Client.__init__() "Missing 1 Required Keyword-Only Argument: 'Intents'"
. Take note, this error is common when working with Discord bots in Python and it signifies that the discord Client needs an ‘Intents’ object to be passed as arguments in initialization.
Understanding Discord’s Intent Object
Discord’s intent object is used to control which events are received over the gateway. It was introduced to limit the data bot requests from Discord API, thereby reducing the overall rate of API calls. The intents define whether to receive certain types of events such as typing events, member updates, or message reactions. Therefore, the intents must be specified in
discord.Client
for better data control when making your bot. Further reading can be found in the Discord Intents documentation.
Consider a simple example:
from discord import Intents from discord.ext import commands intents = Intents.default() client = commands.Bot(command_prefix="!", intents=intents)
In the above code snippet,
Intents.default()
will enable all default intents excluding privileged ones (members & presences). When initializing the Client,
commands.Bot(command_prefix="!", intents=intents)
, an intents object is passed.
Debugging Strategies
When debugging, the critical consideration is ensuring you are using the correct syntax and passing in the required arguments. This requires an understanding of how the method you’re calling works.
Consider the following steps:
- Create an instance of the ‘Intents’ class. If you want all the default intents enabled, use
Intents.default()
.
- If you need to include privileged intents, they must be explicitly defined like so:
intents = Intents.all()
, note that Discord requires bots with privileged intents to be verified if they’re in more than 100 servers. More info at Discord’s Privileged Intents documentation.
- Pass the created ‘Intents’ instance as part of the arguments while instantiating the Client object:
client = commands.Bot(command_prefix="!", intents=intents)
Given these points, always remember to initiate the Discord client with the suitable intents. Scrutinize the error messages and consider the steps outlined above. Additionally, do go through official documents or forums for a clear understanding and possible solutions when similar errors arise.Digging into the intricacies of Python development can be a rewarding, albeit sometimes challenging endeavor. One such challenge you may encounter when dealing with client initialization methods like
Client.__init__()
is a missing required keyword-only argument, typically shown as “Missing 1 Required Keyword-Only Argument: ‘Intents'”. This issue primarily deals with concepts and functionalities tied to Discord’s API (Application Programming Interface).
Silicon-Structured Role of ‘Intents’
To understand this error message properly, we first need to comprehend why ‘Intents’ matter in programming contexts in correlation with Discord. An Intent in Discord’s API represents a type of event. The intents system was designed to limit certain gateway events to only those needed by the bot, thereby reducing unnecessary data transmission. Hence, an intent represents specific types of activities or events that you plan on using while fulfilling an API request. When you use
Client.__init__()
, you are basically initializing your bot client with Discord and telling it what events you will be listening to by specifying the intents.
After October 7, 2020, Discord made it compulsory for bots in more than 100 servers to declare their intents due to rate limit considerations. Therefore, if you’re receiving an error message regarding these ‘Intents’, it might be because you’re not specifying any intents while initializing the Client.
Decoding the Error Message
When the error message says “Missing 1 Required Keyword-Only Argument: ‘Intents'”, it means that you’ve forgotten to provide an essential input to the method
Client.__init__()
: the ‘Intents’. Your code is failing simply because it doesn’t know which events it should be attentive to. Here’s an example:
from discord.ext import commands bot = commands.Bot(command_prefix='$')
The correct way to define the bot would include intents. See below:
from discord.ext import commands from discord import Intents intents = Intents.default() bot = commands.Bot(command_prefix="$", intents=intents)
Here, we used
Intents.default()
to get the default set of intents, which will allow events like message handling. It’s also possible to specify your intents individually using attributes of a newly created
discord.Intents
instance if you want finer control over what events your bot receives.
That said, you’ll have to make sure your bot has been granted the appropriate permissions in the Discord Developer Portal. Here is a handy guide to intents and which ones you’ll need for different events.
Illuminating the Path Forward
Therefore, understanding ‘Intents’ and adequately declaring them is key to initializing your clients effectively without running into errors. As a pro coder, learning how to troubleshoot these issues can not only save you valuable time but increase efficiency. Remember, knowledge is power, and in the realm of coding, it’s the power to write programs that work wonderfully without unexpected crashes.
The
Client.__init__()
“Missing 1 Required Keyword-Only Argument: ‘Intents'” is an error you’ll likely run into when dealing with the discord.py library. This error message appears because discord.py moved to a gateway version that requires declaring intents in order to receive certain events.
When initiating a Client object, it is now mandatory to pass an Intents object which declares what data the bot can and cannot receive. If this parameter is not passed, Python will throw a
TypeError: __init__() missing 1 required keyword-only argument: 'intents'
.
To fix this issue, we need to declare our intents before creating our Client or Bot object. For example:
from discord import Intents from discord import Client intents = Intents.default() client = Client(intents=intents)
With
Intents.default()
, we are requesting all permissions except for privileged ones (that require extra verification). The argument to our Client object then gets the Intents instance we define.
However, make sure to know what you’re enabling as some higher privilege intents may lead to potential abuse if the bot were to fall into the wrong hands.
For more information on specific Intents, consult Discord’s documentation here: discord.Intents
While dealing with the discord API, remember that the Intents object acts as a declaration to Discord about what data your bot needs. Therefore, it’s crucial to initialize it properly to prevent the
Client.__init__()
“Missing 1 Required Keyword-Only Argument: ‘Intents'” error.
The SEO keywords included several times in this answer are
Client.__init__()
, “Missing 1 Required Keyword-Only Argument: ‘Intents'”, discord.py, Discord API, TypeError, Intents.default(), Intents instance, discord API, initializing, python, Intents object, and the high-frequency theme around understanding and resolving programming errors while working with Discord bots.