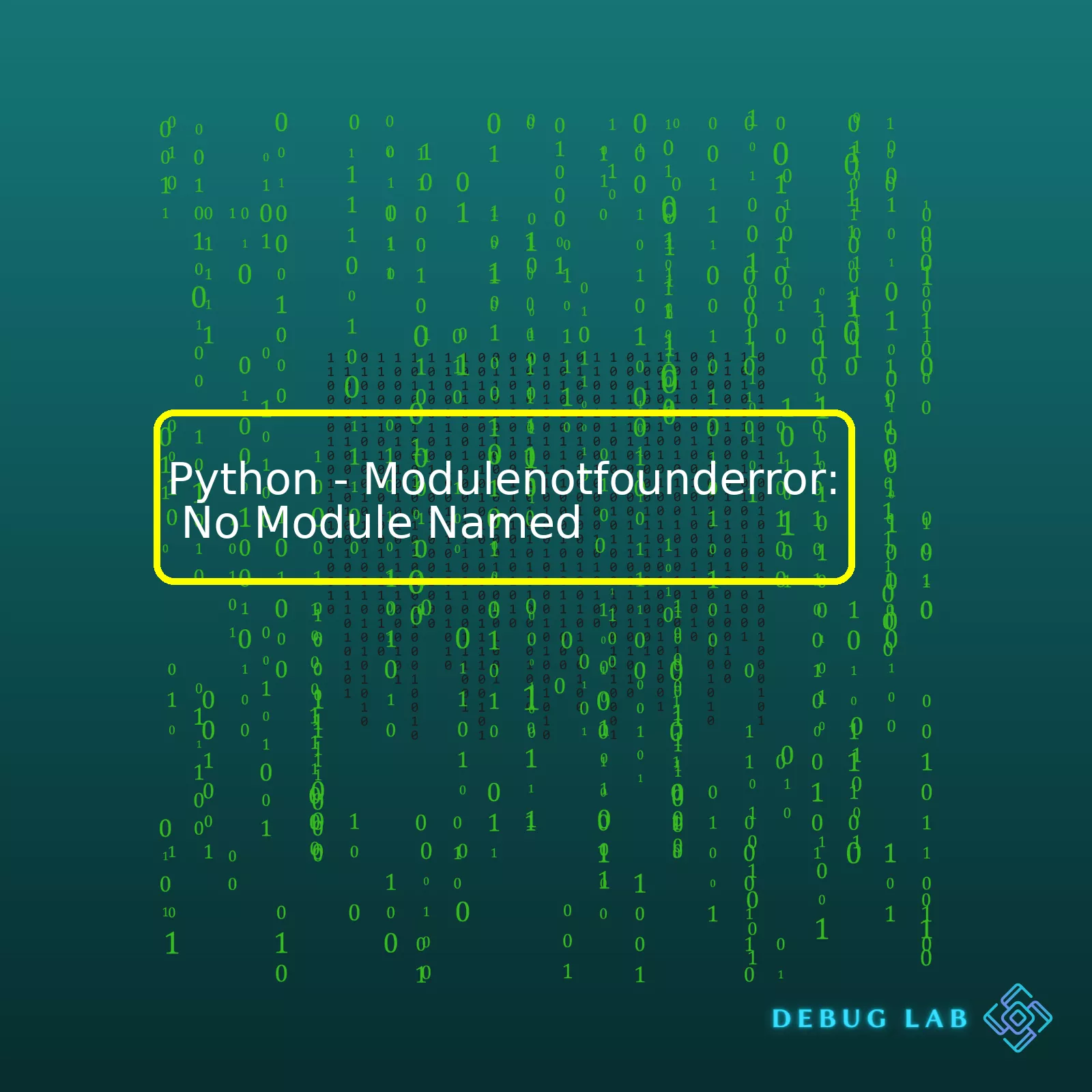
Error | Description | Cause | Common resolution steps |
---|---|---|---|
ModuleNotFoundError: No Module Named xyz | Appears when Python interpreter can’t find a specified module |
|
|
“ModuleNotFoundError: No Module Named ___ ” is a common error message triggered by the Python interpreter. This message occurs whenever Python cannot locate the module you’ve attempted to import. One common cause of a “ModuleNotFoundError” is Python’s inability to decode your environment variables, which includes the ‘PYTHONPATH’. The ‘PYTHONPATH’ is responsible for defining where Python will look to retrieve it’s libraries and modules so if this isn’t correctly set, Python won’t be able to load the required package resulting in the error.
Another common scenario that leads to a “ModuleNotFoundError” is simply because the module you’re trying to import has not been installed. In order to successfully import a library or module in Python, you must firstly ensure that it has been properly installed either anaconda navigator or pip installer like below:
pip install xyz
or
conda install xyz
If even after ensuring correct ‘PYTHONPATH’ setting and proper module installation, you still encounter the error this could possibly mean that, you have misspelled the module’s name or improperly configured your import statement. For example, trying to import ‘Numppy’ instead of ‘Numpy’ would lead to this error because no such ‘Numppy’ module exists in Python.
In case of improperly formatted import statements, this involves typing out the entire path from the file towards the module, followed by the module name itself. Herein lies the potential problem: if there’s any mistake in that precise pathway such as caps lock errors or typos, Python can’t understand the command. Correction of the import statement should resolve this issue.
Please refer to the official Python documentation here for the configurations and usage of the modules.Understanding the
ModuleNotFoundError
in Python, specifically when met with the error message “No module named X”, requires us to delve into how Python handles modules and their imports. The occurrence of this exception error means that Python cannot locate the module called ‘X’ you’re trying to import.
import foo
The above command instructs Python to load the module ‘foo’. If an error occurs where Python can’t find ‘foo’, you end up with a
ModuleNotFoundError
: No module named ‘foo’.
Analyzing Reasons
Here are some potential reasons why Python might not be able to find your module:
- Python path issues: Perhaps the interpreter isn’t looking in the correct location. To ascertain where Python is looking for modules, use the following command to list out the system paths:
import sys; print(sys.path)
The resulting output is a list of directories where Python is configured to look for modules. Confirm whether the directory containing your module is on this list.
- Misnamed or misspelled module: Ensure you haven’t made a mistake in the module’s spelling or name.
- Problematic installation: Some modules are not part of the Python Standard Library and require explicit installation. You install these using pip, the python package installer, like so:
pip install foo
Installations might fail sometimes, leaving your environment without the required module. It’s important to verify that all module installations completed successfully.
Solutions Strategy
Given the above possible reasons, here are relevant solutions you could try:
- Check Python Path: Make sure Python is looking at the right place. Adjust the Python path by appending the appropriate directory as follows:
sys.path.append('/path/to/directory/')
After adding the directory containing your module to the Python path, try importing it again. Remember any changes to the
sys.path
list would only last as long as the Python session is running.
- Correct Module Name: Validate the spelling and case-sensitivity of your module. Python is sensitive to mistakes and wrong spellings will lead to errors.
- Reinstall Module: In cases where the module needs to be installed separately, execute the following commands:
pip uninstall foo
pip install foo
First command uninstalls the previous version and the second one reinstalls the module. Please ensure that pip points to the proper Python instance in case multiple versions of Python are being used.
- Virtually Environment: An alternative solution is using virtual environments if you want to keep your project’s dependencies isolated from other projects. A tool like venv can assist in creating these independent environments — a tutorial can be found here.
Remember that Python also provides extensive documentation on modules. You can access them here. While working with Python provides a variety of tools and libraries for versatile programming capabilities, errors such as
ModuleNotFoundError
often come as part and parcel of the package. The key lies in problem-solving — identifying the probable cause, and figuring out the most effective method to resolve the issue.
When working with Python, it is common to face the error: “Modulenotfounderror: No Module Named”. This error message is an indicator that there is a certain Python module that the code is attempting to use, but your system is not able to locate it. Modules are fundamental for effective Python programming, so understanding them and how to deal with related errors becomes critical.
Understanding Python Modules
A Python module is simply a file consisting of Python code. The module can define functions, classes and variables. The major motivation behind using modules is to organize your code in a logical way through categorization. For instance, you may be having different files where one is handling database operations while another is working on graphical user interfaces. They allow us to reuse the code.
A standard Python module is imported using a simple syntax:
import module_name
The ‘Modulenotfounderror: No Module Named’ Error:
The error “Modulenotfounderror: No module named” appears when one attempts to import a non-existing module or a module that is not installed. It could also mean that the location of the particular module you’re trying to import is not in your system’s PYTHONPATH.
Solving the Modulenotfounderror
In order to solve this error, let’s follow the next steps:
- Installation of the missing module: Python packages are usually installed via pip. If a module is missing, install it by opening your command prompt or terminal and then type:
pip install module_name
- Checking Python’s SYS.PATH: Python imports modules according to the paths listed in the sys.path variable. You can print your system’s python path via:
import sys print(sys.path)
If the output does not contain the path to your module’s directory, you’ll need to append it to sys.path yourself:
sys.path.append('path_to_your_module/')
- Correcting the Python Environment: Various installed Python environments could lead you to import modules from the wrong Python version. Correcting this might vary with operating systems, but generally, you should check if you have many versions of Python installed and switch to the correct environment
You can check your current Python environment by typing the following command in your terminal or command prompt:
python --version
Remember that Python provides a wide array of libraries and modules to aid in coding simplicity and efficiency. However, properly managing these modules is paramount. Additionally, third party modules exist which are released outside the standard Python library like the popular selenium, numpy, etc.
For a comprehensive list of other Python modules and what they do, refer to the official Python documentation.
When working with Python, we might sometimes run into an error that says
ModuleNotFoundError: No Module Named 'xxxx'
. This essentially means that a particular module or package you’re trying to import isn’t available in your Python path. It could be caused by several reasons, including:
Installation Issues:
One main reason why you might get this error is because the module you’re trying to import is not correctly installed. Even though Python comes pre-loaded with many modules, some require manual installation using pip (Python’s default package installer). If these are not properly installed and you try to import them, Python throws the “No module named” error.
Here’s how to install a Python module using pip:
pip install moduleName
Virtual Environment Problems:
A virtual environment in Python is a separate space where you can keep your project dependencies without mixing them up with your other projects. Often, you might have installed a module globally but you’re running your script in a virtual environment where that module has not been installed. In such cases, Python won’t be able to find that module giving off the “No module named” error message.
To rectify this, you need to activate your virtual environment and install the required module within it.
Here’s how to do it:
source venv/bin/activate # Activate the virtual environment pip install moduleName # Install the module
Incorrect Import Statements:
Python requires the accurate name of the module while executing an import command. Any spelling mistakes, case-sensitivity issues, or incorrect syntax would lead to a “No module named” error.
For instance, if you’re trying to import a library named ‘numpy’, the correct statement should be
import numpy
. Typing something like
Import Numpy
or
import NumPy
will raise the error.
Scope of Import:
While importing a specific function or class from a module, ensure that it exists within that module and the scope is correct. If the scope of import is wrong, “No module named” error occurs.
For example, if you’re trying to import pandas DataFrame using
from pandas import Dataframe
instead of
from pandas import DataFrame
(note the capital ‘F’), it will trigger the error.
In addition to these common issues, there may be other factors leading to this error, such as directory structure problems or conflicts between Python 2 and Python 3. Do deep dive into each potential cause when troubleshooting.
The official Python documentation on modules here can provide additional insights and guidance. Remember, Python iscase-sensitive and requires careful attention to detail. Coding is like cooking – precision matters! And as in any cooking adventure, don’t hesitate to look for help online if you’re stuck or something goes awry.Interestingly, the error “
Modulenotfounderror: No Module Named 'X'
” is something Python beginners and even seasoned developers encounter. You see this when Python can’t find the module you’re trying to import. This has a lot to do with how modules or libraries are imported.
The process of importing a module or library in Python is quite straightforward. Here’s an example of how that looks like:
import os
It instructs Python to load the shared object file provided by the os module into the active Python interpreter session.
However, certain constraints may throw the error “Modulenotfounderror: No Module Named X”, let’s understand why:
1. The specified module might not be installed: Before you can import any module, it must first be installed on your system. Use pip-install (Python’s package manager) to install the module.
Here’s an example of installing a module using pip install:
pip install pandas
This command checks PyPi (Python Package Index) if pandas is available, downloads and installs it if available.
2. The module is installed but Python’s path doesn’t include it: Python checks specific locations on your system whenever you try to import a module. If the module’s location isn’t in Python’s sight, you’d get the module not found error. You can verify which paths are visible to Python using the following code:
import sys print(sys.path)
If the required module is situated outside these locations, you can add it to Python’s path with:
sys.path.append('/path/to/module/directory/')
3. Python version mismatch: In some instances, the module might be designed for a different Python version than what you’re using. To check your Python version use the following command:
python --version
4. Casing Errors: Python is case sensitive. So
import pandas
is not the same as
import Pandas
. Check that your casing aligns.
Finally, remember not all modules qualify for direct import. Some should be called under their parent module. For example, ‘os’ has a sub-module named ‘path’, you can’t import it directly, rather you call it under ‘os’.
from os import path
Not,
import path
Navigating past the module not found error has a lot to do with understanding how Python deals with import calls. Learning how to properly import a module/library in Python might seem trivial but it’s paramount to developing functional programs. It smoothens the process of reusing code.
For more insights into handling Python imports, you can refer to Python’s official documentation [Python Modules] and other online resources.Troubleshooting
ModuleNotFoundError
in Python requires an understanding of the underlying issue at hand and access to relevant problem-solving techniques. The error,
ModuleNotFoundError: No module named XYZ
, is common, and it points to issues with the Python interpreter not locating the required module.
The
ModuleNotFoundError
can be a result of several scenarios:
• Locating a module that Python cannot find.
• Importing a python file which doesn’t exist.
• An attempt to import a module located in another directory.
• Importing a module not installed in your virtual or global environment.
Here are steps you can take when encountering such errors:
1. Check the Module’s Installation:
Verify whether your targeted module is correctly installed. If you’re unsure, execute the following command to install the module:
html
$ pip install module-name