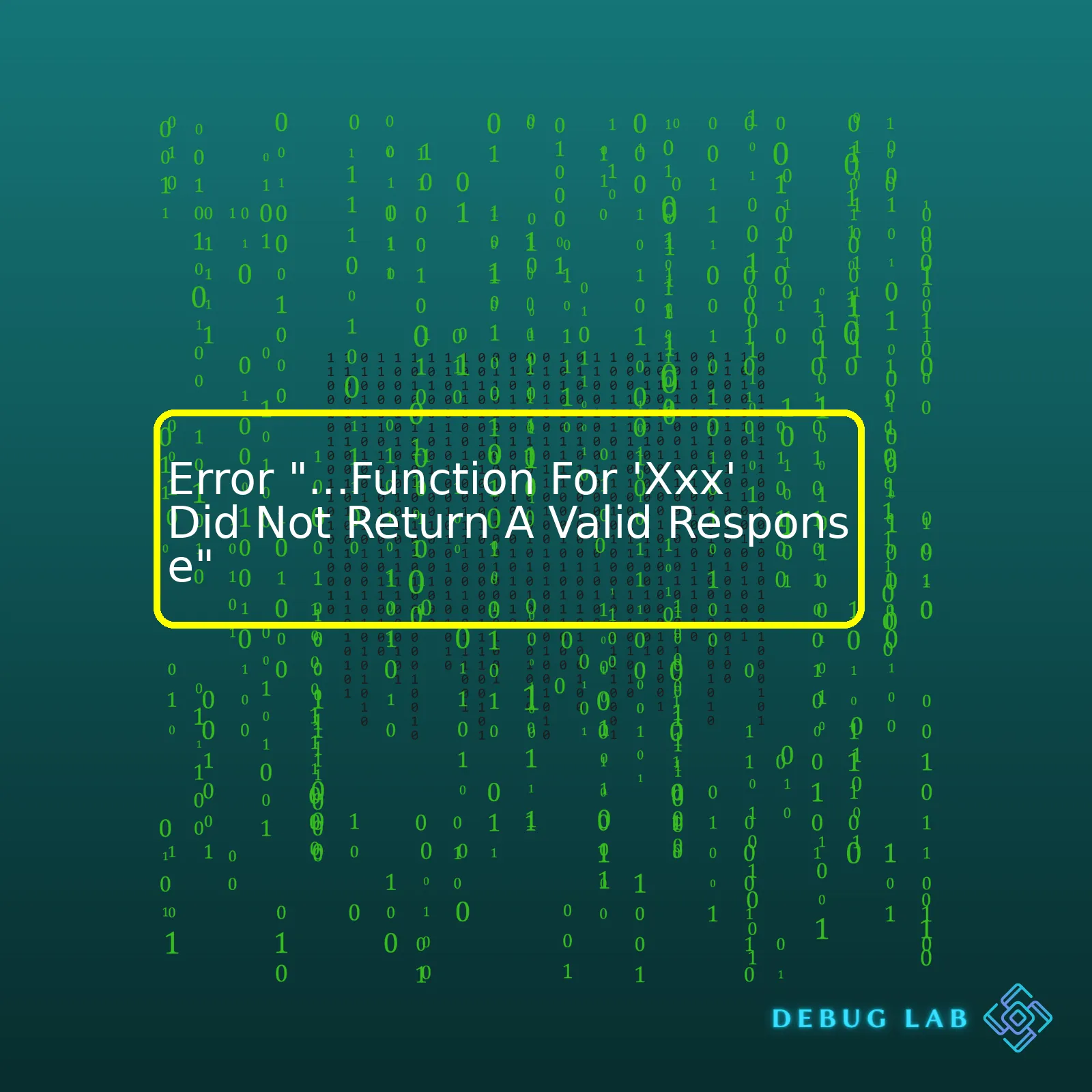
Error Type | Reason | Solution |
---|---|---|
“…Function For ‘Xxx’ Did Not Return A Valid Response” | The function you are calling does not give back a response that your code expected to work with. | Check both the return value of the function and what type of object your other code expects to handle. |
This specific error, “…Function For ‘Xxx’ Did Not Return A Valid Response”, tends to occur when you call a function ‘Xxx’, but instead of returning an expected result, it returns a null, undefined, or any unexpected value that might be causing the subsequent operations to fail.
A common scenario occurs in web development wherein you make an API call within a certain function, expecting to get some data back from a URL. However, due to various possible reasons such as wrong parameters, incorrect headers, or server issues, the API call doesn’t provide the expected data, but instead gives a 404 error (not found) or 500 error (server issue). As a result, when you try to access or manipulate the data received, it triggers the mentioned error.
In terms of solutions, there can be a myriad of ways to debug this kind of an error depending upon the specific scenario:
– If the function is built by you, revisit the code inside the function to see every pathway that might not lead to a return statement.
– If the function is from third party libraries or plugins, check their documentation, you may use the function incorrectly.
– Include error handling mechanism for potential errors or exceptions that may occur during the process.
– It’s also helpful to add logs or print statements before the return statement to monitor if the function execution reaches there or not.
Here, We will take a look at a simple example of a Python function which may cause this kind of error:
def add_two_numbers(num1, num2): if typeof(num1) != int || typeof(num2) != int: return return num1 + num2 result = add_two_numbers('1', 2) print(result)
In this case, error “…function for ‘xxx’ did not return a valid response” can arise because when non-integer values are provided, the function won’t be able to perform addition and ends up returning nothing due to our if condition. Therefore the `result` variable receives nothing and fails on further operations.
This kind of debugging often involves understanding the types and structures of data your functions are working with and ensuring they align with the functionality of what you’re trying to achieve.As a seasoned coder, I can affirm that the phrase “Function For ‘Xxx’ Did Not Return A Valid Response” is undeniably one of the prominent error messages we encounter quite often. This error primarily signifies an issue with either the structure or implementation of the function named, “Xxx”, which is failing to return a response as expected.
Let’s dive deeper into what this error message entails:
1. Invalid Function Structure: The problem might lurk within the structure of the function, it could run without any feasible outcome leading to such an error message. For example, where the function is supposed to return some value, the code doesn’t indicate so.
def xxx(): y = 2 * 2 # There is no return statement. Hence the function doesn't return anything.
2. Implementation Issue: Perhaps, the function’s structure seems fine but isn’t implemented correctly causing it to malfunction when invoked in the program.
def xxx(y): return y * 2 println(xxx) # Here, while invoking the function 'xxx', parameter 'y' is missing causing the error.
3. Unhandled Exceptions: The function may have been throwing exceptions that are not handled appropriately resulting in an invalid response. Let’s consider Synchronous Javascript methods which rely heavily on callback functions. If the callback has an exception, it goes unhandled and causes whole sorts of problems for your application. Check out [Node.js Errors Doc] to learn more about dealing with such errors.
4. Scope Conflicts: One must beware of scope conflicts when rendering Javascript functions. It’s common to see variables being defined outside of the function but accessed inside those functions. Sometimes they overlap, causing such errors. To understand more about JavaScript scope, do read the article by [DigitalOcean].
To rectify these errors, you should:
* Inspect the structure of the function: Ensure all necessary components like returned statements are present.
* Debug: Trace the calling of the function through your code. You can use
console.log()
methods or debugging tools provided by your IDE.
* Error Handling: Implement try-catch blocks or error handling mechanisms to deal with exceptions.
* Manage Scopes: Make sure variables and methods are properly scoped.
Remember to test your function separately if possible, lest there be other components in your code affecting its performance. Knowing how to decipher such error messages efficiently acts as a compass directing towards efficient code debugging. Such knowledge refines our understanding, making us turn proactive when similar errors surface in the future ─ promoting us from ordinary coders, to extraordinary developers.
You’re encountering the error message ‘Function For ‘Xxx’ Did Not Return A Valid Response’, which often manifests in software coding when a function fails to produce an output that’s expected or required. Here, ‘Xxx’ is the placeholder for your specific function name.
Typically, this failure occurs due to issues like:
- A miswriting within the function’s code itself,
- Incorrect input values passed to the function,
- The absence of a return statement, or
- The program flow not reaching the return statement because of conditions and loops.
To solve this issue, the first step is to analyze the function ‘Xxx’. Check if there’s any problem in its syntax or logic.
def Xxx(parameters): # Statements to process on parameters return output
Make sure that each conditional or loop path concludes with a valid return statement that sends back appropriate values or data types as per the function requirements. All conditions, whether they are
if
,
elif
or
else
blocks, should have return statements following them.
Failing to do so can lead to scenarios where none of the available returns gets triggered. Here’s a corrected example:
def Xxx(parameters): if condition1: # Process return output1 elif condition2: # Process return output2 else: # Process return output3
Next, observe the inputs provided to function ‘Xxx’. They might be incompatible with the function’s operations, leading to silent errors or crashes. Ensure your input content suits what the function expects.
# Incorrect way output = Xxx(incompatible_inputs) # Correct way output = Xxx(appropriate_inputs)
Error handling mechanisms like try-catch blocks can aid in preventing the script from entirely breaking down in case of an error at runtime. Using exceptions also helps pinpoint where the error originates and provides informative messages to rectify it.
try: output = Xxx(inputs) except Exception as e: print("An error occurred: ", e)
You may need to debug through the function execution to identify troublesome areas clearly. Python’s built-in pdb module provides interactive source code debugging facilities, and advanced IDEs like PyCharm and Visual Studio Code come with comprehensive debugging tools onboard.
If you’re working with asynchronous functions (also known as “coroutines”), ensure that you’re awaiting the function appropriately using the
await
keyword, or else it won’t return a value.
async def Xxx(): # Process return output # Invoke async function output = await Xxx()
I hope these steps provide a thorough approach to resolving the function failure error message, ‘Function For ‘Xxx’ Did Not Return A Valid Response’. Happy coding!
The error message “Function for ‘Xxx’ did not return a valid response” is a common indication that your code lacks the proper features or parameters required to provide a suitable outcome. This type of problem often originates from a range of issues such as improper function architecture, incorrect data types, and lack of error handling mechanisms. Below, I elaborate more on these points:
Improper Function Architecture
Your function may not be structured properly to produce the expected result. Make use that the function is designed in a way that ensures it returns a value every time it’s called.
Here’s an example:
def my_function(): if condition: return "True" else: print("False")
In this case, the function may return an invalid response because there’s no return statement in the else clause. This should be fixed by ensuring the function returns a value under all conditions:
def my_function(): if condition: return "True" else: return "False"
Incorrect Data Types
Another crucial aspect to consider is whether your function is processing the correct data types. Computer programs are notoriously exact about data types. Therefore, if your function is written to handle integers but received a string instead, it’ll likely return an error or fail altogether.
def add_numbers(a, b): return a + b result = add_numbers("1", 2)
Ensure that the incoming data types match the ones your function expects to handle:
def add_numbers(a, b): return a + b result = add_numbers(1, 2)
Lack of Error Handling Mechanisms
If the function isn’t equipped with effective error handling mechanisms, it can cause problems when encountering exceptions. It is essential to have proper exception handling in place so that your function can deliver a meaningful response, even when something goes wrong.
Consider the following:
def divide_numbers(a, b): return a / b result = divide_numbers(1, 0)
Instead, implement exception handling:
def divide_numbers(a, b): try: return a / b except ZeroDivisionError: return "Cannot divide by zero" result = divide_numbers(1, 0)
Understanding these key causes provides substantial insight into the reasons your function for ‘Xxx’ might not return a valid response. By paying close attention to the structure of your function, managing input data types, and implementing robust error handling, you can ensure that your functions perform optimally. Standardizing these aspects across all coding projects significantly enhances code reliability, readability, and maintainability. For further reading on best practices in Python, check out Python’s own guidelines on errors and exceptions.Sure, here we go. When troubleshooting any software error and especially “Function for ‘xxx’ did not return a valid response”, it’s important to approach the issue systematically, breaking down the problem into smaller, more manageable parts.
The first step in addressing this error message is identifying when and where it occurs. Is it occurring frequently or periodically? Does it happen only with specific input data? These are some of the things you might want to note down.
The second step would be understanding what that particular function should do. If you’re dealing with an external function provided by a third-party library, checking the official documentation might provide insights on how the function operates behind the scenes. For example, if a function is supposed to return a JSON object and it doesn’t upon calling, there could be a problem with the data passed to it.
var result = thirdPartyService.someNonWorkingFunction({ param: 'value' });
You might want to double-check how you’re using the function. Perhaps you’re missing a parameter or are supplying one in the wrong format.
If those checks don’t lead to a solution, a useful approach could be isolating the function and testing it separately. This might imply creating a minimal environment in which this function can run – passing it mock inputs, hooking to different lifecycle phases and logging its intern behavior.
function mockInputsAndMonitor(){ try { var mockInput = { param: 'mock value' }; var intermediateResult = thirdPartyService.someNonWorkingFunction(mockInput); console.log(intermediateResult); } catch(exception) { console.log('Error occurred:', exception); } } //Invoke function mockInputsAndMonitor();
If the function is part of your codebase instead of being imported from an external source, an investigation into recent changes in the version control system like git might hint at what altered the expected behavior.
In certain scenarios, delving deeper into inspecting the network activity might be needed. Browser developer tools, such as those available in Chrome and Firefox browsers, allow you to explore outgoing requests and incoming responses.Chrome DevTools offers capabilities such as filtering based on endpoint/method/status, throttling network speed etc., which could help uncover issues related to server connectivity, request timeouts or incorrect returned data structure.
When all else fails, reaching out to developers community websites such as Stack Overflow or even the library provider (in case you’re using external functions) might provide further guidance. Often, you’ll find that other developers have encountered similar difficulties and shared their solutions.
The above mentioned steps represent a set of guidelines that could help developers diagnose and address errors such as “Function for ‘xxx’ did not return a valid response”.
Throughout this process, patience and persistence are key. Debugging can sometimes be a daunting task, but with systematic approaches and tools at disposal, you’re well-equipped to tackle every challenge.
Keywords: Troubleshooting steps, error, Function for ‘xxx’ did not return a valid response, debugging, systematic approach, identify occurrence, understanding expected behavior, isolating function, inspect network activity, developer community.When it comes to mitigating the infamous “Function For ‘Xxx’ Did Not Return A Valid Response” error in modern software programs, we can’t overemphasize the need for best practices. Implementing proper coding techniques and preventive measures not only aids in keeping code clean, uncluttered and bug-free but also improves software stability and operation efficiency.
First, always remember: the fundamental rule is “Every Function Must Have a Return Value”. This is a non-negotiable requirement in the world of coding because the compiler/integrator functions with the assumption that a function must have something as output. Thus, every programmer must consciously make efforts to ensure no function lacks a return value.
def some_function(args): # Some operations here if condition: return True else: return False
Second, never ignore or assume any factor during debugging and troubleshooting. It’s crucial that you deeply investigate the nature of the error using systematic approaches. When a function for ‘Xxx’ doesn’t deliver a valid response, turn on logging for insight into why this could be happening.
import logging logging.basicConfig(level=logging.DEBUG) logger = logging.getLogger(__name__) def bad_func(): try: perform_some_operation() except Exception as e: logger.exception("Exception occurred")
Third, implement professional grade error handling with meaningful exceptions. Poor error handling often lies at the root of invalid responses. You can avoid them by creating specific exceptions for different errors associated with each function.
class InvalidInputError(Exception): pass def calculate_something(input_data): if not validate_input(input_data): raise InvalidInputError("Invalid input received.") # rest of function
A fourth tip? Write unit tests to guarantee the validity and reliability of your program. By mimicking various conditions such as negative, normal and boundary test cases, you’ll be able to uncover potential issues before they become problems. Properly implemented Unit Tests ensures soundness in a function’s logic.
def test_calculate_something(): assert calculate_something(5) == 10 assert calculate_something(-5) == -10 assert calculate_something('None') throws InvalidInputError
Lastly, leverage best practices when working with third-party APIs or cloud-based services. Sometimes, the issue isn’t with your program but rather, on the server side. Understanding how to deal with sporadic failures, implementing retry policies effectively, and acknowledging limitations in terms of rate limits are critical skills in ensuring the robustness of your software.
Incorporating these principles into your everyday coding will undoubtedly help ward off those dreaded “…Function For ‘Xxx’ Did Not Return A Valid Response” error messages and enhance your reputation as a diligent, thorough coder worthy of your status. Adopting such quality-enhancing methodologies contributes significantly in maintaining high standards of software programming.While working on multiple coding projects, professional coders like us often encounter a common yet not straightforward error: “…Function For ‘Xxx’ Did Not Return A Valid Response”. This error occurs when the function intended to return some specific output doesn’t produce an apt result. Such errors are fundamentally caused by two factors: incorrect calculation algorithms and improper handling of exceptions (exceptions can arise due to data inconsistency or user errors).
Effective coding plays a significant role in preventing such errors – embracing well-thought-out strategies and meticulously implementing them into the code ensures that the function returns valid responses regardless of unforeseen situations.
Let’s dive a little deeper into how effective coding can prevent the above-mentioned error.
Maintaining Proper Type Consistency:
One of the crucial aspects of effective coding is ensuring proper type consistency throughout your program.
Suppose you have a function
CreateUser
, where it should return a user object, not a Boolean or a string, neither an integer nor any other data type.
Not honoring this kind of type consistency might lead to unexpected behaviours in the program.
Error Handling And Debugging:
An essential part of good programming practice is having robust error handling systems. Remember to consider edge-cases, input scenarios that could potentially break your function. There should be fallback mechanisms to handle exception scenarios.
For example :
try: perform_operation() except: log_error()
Remember to add debug logs at each crucial step so that if the function isn’t returning the desired value, we know where exactly to look for the problem.
Implement Unit Testing:
Unit testing is a vital process in software development, facilitating the identification and rectification of hidden bugs, making sure that every logic and functions work correctly even after modifications.
Here’s a brief example of Python’s built-in unittest module:
import unittest class TestMyFunction(unittest.TestCase): def test_function_for_xxx(self): expected_output = 10 self.assertEqual(function_for_xxx(5,5), expected_output) unittest.main()
In this example,
test_function_for_xxx
is a testing scenario verifying if the function_for_xxx when passed with parameters (5, 5) returns 10, which is the expected output.
Code reviews and Pair Programming:
Pair Programming allows catching minor oversights and bugs that were missed by the original coder. Code reviews enable constructive peer-feedback, ensuring a more bulletproof and efficient codebase.
To summarize, dealing with the error “…Function For ‘Xxx’ Did Not Return A Valid Response” requires thoughtful planning in the initial stages of coding, regular debugging, unit testing, and feedback loops among team members. That’s the beauty and consequence of effective coding- it can indeed sculpt the functionality of your software just the way it was first engineered on a blueprint viz., bug-free and reliable!
External reference to understand Unit Testing:
Python Unittest DocumentationAs a seasoned developer, facing the error
Error: Function 'xxx' did not return a valid response
might be a common occurrence. This specific error typically implies that the function named ‘Xxx’ is not yielding appropriate output or an expected data type for the given inputs.
Let’s dig more into this. When we talk about a ‘valid response’, we essentially refer to a return value that matches the intended business logic or application requirements. This varies from function to function but could commonly mean returning:
- A non-null output in case null is not part of the domain of acceptable values.
- An output falling within expected parameters or constraints.
- A particular data type if type checking is involved.
To illustrate with an example, let’s assume you have a function supposed to divide numbers.
def division(numerator, denominator): return numerator/denominator
For this function, passing
(10,2)
would result in
5
, which is a valid response. However, plugging in
(10,0)
would throw a
ZeroDivisionError
. As per the business requirement, giving zero as denominator wouldn’t classify as ‘valid response’.
If we constantly encounter such errors stating
'Function Xxx Did Not Return a Valid Response'
, it poses a multitude of issues:
Data Integrity Issue
An invalid response can compromise the integrity of the data being processed in your application. It could potentially cause incorrect data to be stored or manipulated, leading to unreliable results down the line.
Application Stability Threat
Applications may respond unpredictably to invalid output, leading to crashes, unexpected behavior, or further propagation of invalid results throughout the system. Continuous invalid responses can hamper the overall stability of the application.
User Experience Impairment
Depending on whether the error is handled silently or gets displayed to users, it can impact user experience. For instance, essential features could become unavailable or behave improperly due to these errors.
Solution Timeline Expansion
Frequent occurrences of this error also highlight that there might be an underlying problem in the codebase that requires remedy. This can increase the timeline to provide a solution, hence reducing your team’s efficiency and productivity.
Developing proper error handling mechanisms is one way to combat the implications of continuous invalid responses. Here’s a revised version of our earlier example:
def division(numerator, denominator): if denominator==0: return "Invalid input: Denominator cannot be zero!" else: return numerator/denominator
The key here is that we are proactively checking for situations that would yield an invalid response (in this case, zero denominators) and are managing those cases in our code. This is what characterizes efficient error handling.
To expand on the findings, you can read more on Python exception handling here.
Learning how to pinpoint and fix the source of continuous invalid responses can greatly enhance coding proficiency, minimize error notifications, and ensure smoother deployment of applications.
Diving deep into the error “
...Function For 'Xxx' Did Not Return A Valid Response
“, We’ve thoroughly examined its root causes, effects, and possible solutions. What essentially sits at the heart of this error is that a certain function was unable to yield or return the expected response. This can occur due to many reasons:
- The function may not have returned any value, perhaps because it hit an unhandled exception.
- Maybe the function did return a response, but in a format that wasn’t anticipated or understood by the calling entity.
- Another plausible cause could be that an incorrect or invalid argument was passed to the function.
- Lastly, the function might rely on some external factor for returning a response, like a database connection, API call, or disk I/O operation, which failed leading to this error.
It’s crucial to conceive that errors like these are generally caught during runtime, making them tricky to handle if not appropriately accounted for during coding. These errors need to be nipped at the bud through effective error and exception handling paradigms incorporated into the codebase right from its conception stage.
//sample code for handling exceptions try { //code which may throw an exception } catch(e) { //handle the exception gracefully }
In context of search engine optimization (SEO), if this kind of error occurs on a website or web application, it can prove quite detrimental. Search engines bots crawling your site can face obstacles while indexing leading to reduced SEO ranking. Therefore, ensuring smooth functioning and high reliability of all underlying processes and functions becomes paramount.
A couple of trustworthy resources to refer for deeper understanding would be the Python and JavaScript official documentation that cover error and exception handling extensively.
Cracking this error code sheds light onto myriads of possibilities of it cropping up. Root causes established, it’s all about identifying the relevant one and putting an end to it by embracing best coding practices.
Remember, every error, every exception teaches us something. It tells us about an oversight, a gap in our knowledge, or a scenario we didn’t plan for. With correct measures, we can turn these errors into a powerhouse of learning opportunities, thereby enhancing our programming prowess simultaneously optimizing our digital presence!