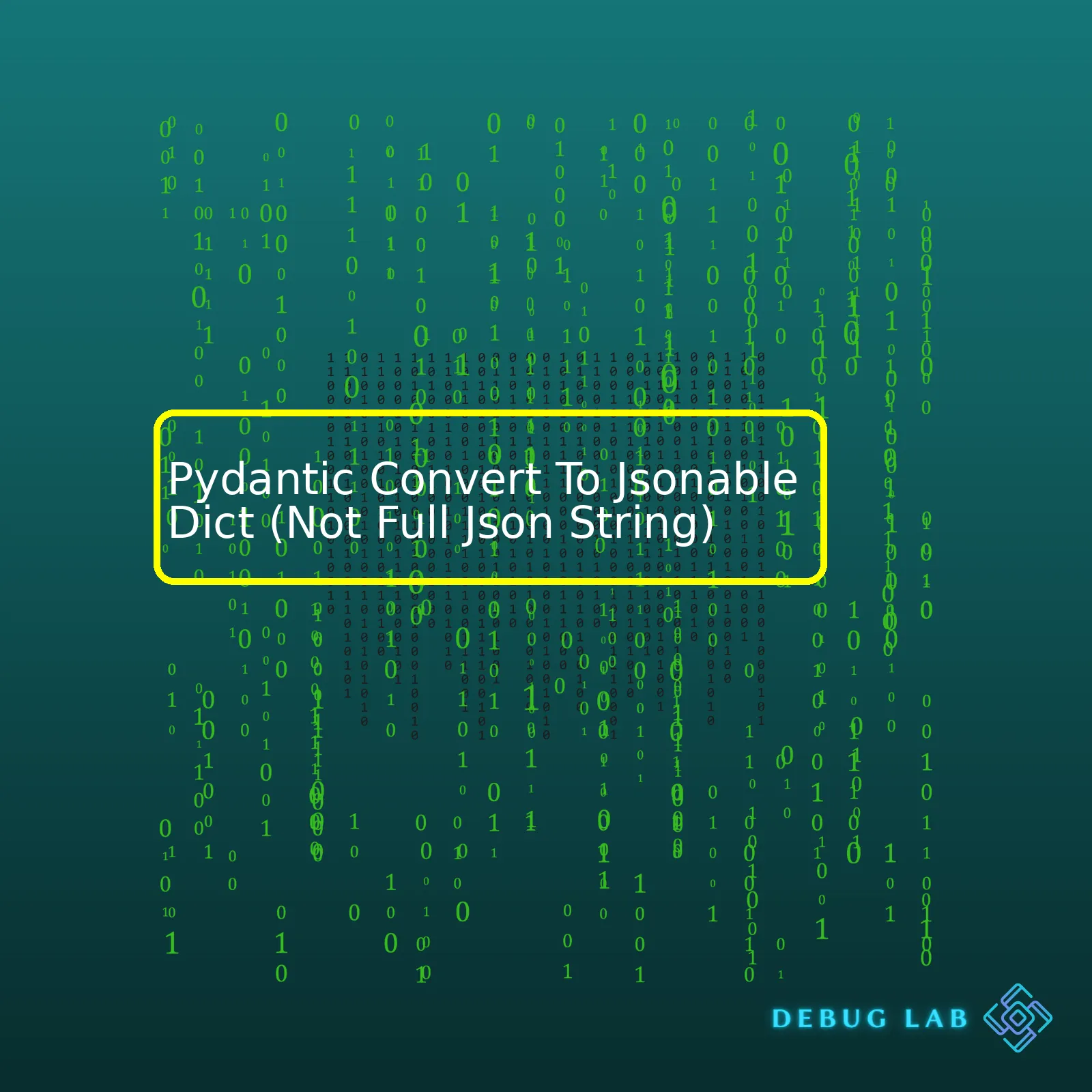
Functionality | Description |
---|---|
Pydantic Models | They are classes that validate and enforce type constrains on the input data. This ensures safety and reliability. |
.dict() Method | An instance method to convert the Pydantic model into a Python dictionary. |
.json() Method | An instance method to convert the Pydantic model into a JSON formatted string. |
Jsonable Encoding | It is the process to convert a Pydantic model into a Python data structure that can be serialized into a JSON format string. |
Now, let’s give you more insight into how this works. Pydantic libraries provide developers with some built-in methods to handle JSON data easily. Specifically,
.dict()
helps in converting a Pydantic model into a Python dict while
xxxxxxxxxx
.json()
converts the object into a full JSON string.
However, when it comes to Pydantic’s ‘jsonable encoding’, it refers to transforming the Pydantic model into a Python data structure that is capable of being turned into a valid JSON format string. In technical terms, we call these ‘JSON serializable objects’. These include simple types like dicts, lists, strings, integers, etc., all of which are readily accepted by the
xxxxxxxxxx
json.dumps()
method in Python.
Take this example:
xxxxxxxxxx
from pydantic import BaseModel
import datetime
class UserModel(BaseModel):
name: str
joined_at: datetime.datetime
user = UserModel(name='John Doe', joined_at=datetime.datetime.now())
print(user.dict())
Here, we have a simple `UserModel` form Pydantic which contains `name` (a string) and `joined_at` (a datetime object). When we create an instance of `UserModel` and call
xxxxxxxxxx
.dict()
on the instance, the data is converted into a Python dictionary.
But if you pay attention, the ‘datetime’ object within the dictionary doesn’t fall under ‘jsonable’ objects as it throws TypeError if we try to serialize it using
xxxxxxxxxx
json.dumps()
. It needs to be converted into a ‘string’ representation to make it JSON serializable or ‘jsonable’ – hence we need ‘jsonable’ encoding to avoid such conflicts during serialization.
Pydantic, a data parsing library for Python, aims to provide data validation using Python type annotations. It’s heavily used in FastAPI and other modern Python web frameworks for handling request/response schema definitions. Pydantic ensures that the input data conforms to a pre-defined model or type.
JsonableDict is a term often used in Pydantic circles to describe a Dictionary that can be safely converted into a JSON string using
xxxxxxxxxx
json.dumps()
function, without any additional conversion or manipulation required.
Converting to JsonableDict
In Pydantic, the model instance dictionary returned by the
xxxxxxxxxx
.dict()
method on a Pydantic Model is essentially JSON serializable or “JSONable”. We define our Pydantic model and then convert an instance of it to a dictionary (or JSONableDict).
xxxxxxxxxx
from pydantic import BaseModel
class User(BaseModel):
id: int
name: str
email: str
user = User(id=1, name='John Doe', email='john@example.com')
jsonable_dict = user.dict()
print(jsonable_dict)
This code will output:
xxxxxxxxxx
{'id': 1, 'name': 'John Doe', 'email': 'john@example.com'}
The resulting dictionary (
xxxxxxxxxx
jsonable_dict
) can now be serialized into a JSON string, or used anywhere else where we need a JSONable dictionary.
Difference between JsonableDict and Full Json String
A JSON object corresponds to a dictionary in Python. Therefore, when you use the
xxxxxxxxxx
.dict()
method from Pydantic, you’re not getting full JSON string, but a dictionary that can be easily converted to a JSON string because it fits the structure. A JsonableDict can be manipulated as any regular Python dictionary until it is serialized using
xxxxxxxxxx
json.dump()
or
xxxxxxxxxx
json.dumps()
.
Here is how you would transform the above example’s JsonableDict to a JSON string:
xxxxxxxxxx
import json
...
json_string = json.dumps(user.dict())
print(json_string)
This code outputs the full JSON string:
xxxxxxxxxx
{"id": 1, "name": "John Doe", "email": "john@example.com"}
In a nutshell, with Pydantic models being able to quickly turn them into JsonableDict offers flexibility – you have a dictionary that is JSON-ready, but can still be handled as a normal Python dictionary with all its functionalities if needed. Turning it into a full JSON string finalizes it into a format ready for document storage databases or HTTP responses, but losing the flexibility Python dictionaries might offer.
To further your understanding about Pydantic and JSON serialization, I would recommend going through the official Pydantic documentation.
When working with Pydantic, there comes a time when you may want to convert your models to jsonable dictionaries, instead of fully serialized JSON strings. This might be particularly useful in cases where you need to operate on the data natively in Python, before possibly sending it off as JSON elsewhere.
The key method that we’re going to look at in detail is
xxxxxxxxxx
dict()
. It’s a built-in mechanism provided by Pydantic for converting Pydantic BaseModel instances into python dictionaries.
Take a look at this simple example:
xxxxxxxxxx
from pydantic import BaseModel
class User(BaseModel):
id: int
name: str
user = User(id=1, name="John Doe")
user_dict = user.dict()
print(user_dict)
In this example, you can observe how the Pydantic BaseModel instance
xxxxxxxxxx
user
gets converted into a dictionary using the
xxxxxxxxxx
dict()
method. The output would then be:
xxxxxxxxxx
{'id': 1, 'name': 'John Doe'}
Now you have a dictionary representation of your Pydantic model object, ready for any manipulation or processing in a dictionary-friendly environment!
You can even control what data enters your final dictionary by passing extra arguments such as ‘include’, ‘exclude’, and ‘by_alias’ to the
xxxxxxxxxx
dict()
method.
‘Include’ and ‘exclude’ can filter out keys from the dictionary while the ‘by_alias’ argument uses the alias provisioned in your Pydantic class definition – thus enabling greater control over the process of conversion.
Consider:
xxxxxxxxxx
class User(BaseModel):
id: int
name: str
class Config:
alias_generator = lambda field: 'u_' + field
allow_population_by_field_name = True
user = User(id=1, name="John Doe")
user_dict_with_alias = user.dict(by_alias=True)
print(user_dict_with_alias)
This will yield:
xxxxxxxxxx
{'u_id': 1, 'u_name': 'John Doe'}
So, we’ve reviewed the mechanics of conversion underpinning Pydantic’s ability to transform BaseModel instances into jsonable dictionaries. This provides a great deal of flexibility when dealing with complex data formats – especially if you need an intermediary stage that isn’t purely JSON.
Want more details? Check out Pydantic’s documentation on model usage. It goes deeper into these concepts, and covers additional areas like ORM mode, etc. Always remember, tailor your strategy based on your specific data needs for maximum efficiency!Ah, it’s quite a fascinating journey diving into working with complex objects in Python, especially when you’re utilizing a powerful library like Pydantic. When we talk about using Pydantic to convert these elaborate objects into a format that’s more friendly for JSON manipulation but not necessarily entirely to JSON string representation, there are several considerations and approaches we can take.
Firstly, it’s essential to understand why Pydantic is so handy. It assists in data parsing, providing us with an effective way to define how our data should be constructed with Python 3.6+ type annotations. Moreover, it fashions your data classes with built-in validation to keep the data structured and consistent.
Here, we can look at converting a Pydantic object (e.g., BaseModel) to a dictionary capable of being easily converted to a full JSON string:
xxxxxxxxxx
from pydantic import BaseModel
class User(BaseModel):
name: str
age: int
# Creating an instance
user = User(name="John Doe", age=30)
# Converting to dictionary
user_dict = user.dict()
#prints {'name': 'John Doe', 'age': 30}
print(user_dict)
The
xxxxxxxxxx
dict()
method comes with Pydantic’s BaseModel, enabling you to convert the model into a dictionary effortlessly. The brilliant part, though, is that it doesn’t stop there. With Pydantic, you have control over how this conversion process happens using parameters such as
xxxxxxxxxx
include
,
xxxxxxxxxx
exclude
, and
xxxxxxxxxx
by_alias
to dictate what information gets added, excluded, or transformed.
However, there could be an instance where you might need to have nested Pydantic models, adding a bit more complexity. Let’s consider another example:
xxxxxxxxxx
from pydantic import BaseModel
from typing import List
class Car(BaseModel):
brand: str
year: int
class User(BaseModel):
name: str
age: int
cars: List[Car]
car1 = Car(brand='Tesla', year=2020)
car2 = Car(brand='Ford', year=2018)
# Creating an instance
user = User(name="John Doe", age=30, cars=[car1, car2])
# Converting to dictionary
user_dict = user.dict()
Even here, Pydantic takes care of the heavy lifting, handling the nested models effectively while turning them into a dictionary. Additionally, using
xxxxxxxxxx
parse_obj
function, you can reverse the action of converting back to Pydantic models from the dictionary.
Now coming to SEO optimization, writing maintainable and easily-readable code not only bolsters productivity but improves web visibility. While the search engine bot crawls your website’s content, quality content includes clear, concise, and well-formatted code, promoting better rankings[^1^].
During the coding phases, optimizing for variables, methods, and class names by being descriptive, brief, and leaving no room for ambiguity land a significant impact in making your codes SEO friendly. Further, usage of comments significantly helps engines recognize the structure and functionality, accelerating the indexing processes dramatically.
Also, leveraging highly sought keywords within your commentary and writing thorough alt attributes provides valuable context to search engines, enhancing your code comprehension and SEO simultaneously[^2^]. However, use them wisely, unnatural or excessive keyword stuffing might get flagged as spammy.
Overall, mastering the art of working with intricate objects in Python using Pydantic involves understanding the tool’s unique capabilities and tactfully applying its functionality where it matters most. Afterall, coding is as much an art as it is science!
[^1^]: “Why Clean Code is Important for Your Website’s SEO,” DevriX,
[^2^]: “How to provide meaningful code-based SEO clues,” BuiltVisible,
Converting a Pydantic Model to a Jsonable Dict
In essence, here’s the basic syntax to make use of Pydantic’s `jsonable_encoder` to convert your Pydantic model to a dictionary:
xxxxxxxxxx
from pydantic import BaseModel, jsonable_encoder
class MyModel(BaseModel):
name: str
age: int
data = MyModel(name="John Doe", age=30)
json_data = jsonable_encoder(data)
print(json_data) # Outputs: {"name": "John Doe", "age": 30}
Neverthless, this outputs a dictionary filled with primitive types like strings, integers, floats, and booleans that mirror their JSON equivalents. Note though, that the types here are solely those familiar to Python. But what if we have other complex datatypes such as date and time objects?
Making Use of Jsonable Dicts with Complex Datatypes
Picture having a datetime value in your Pydantic model and intending to preserve it when converting to a dictionary representation. Implementing the same steps, this time with a datetime field, looks like the following:
xxxxxxxxxx
from datetime import datetime
from pydantic import BaseModel, jsonable_encoder
class MyModel(BaseModel):
name: str
age: int
timestamp: datetime
data = MyModel(name="John Doe", age=30, timestamp=datetime.now())
json_data = jsonable_encoder(data)
print(json_data)
Visualizing the resulting dictionary from the print statement demonstrates that the datetime object is transformed into a string. This is automatically performed by the jsonable_encoder function, ensuring compatibility with JSON standards.
However, we can instruct Pydantic to retain specific complex types. Communicating to `jsonable_encoder` that it should spare DateTime objects entails using the `custom_encoder` parameter, as illustrated below:
xxxxxxxxxx
json_data = jsonable_encoder(data, custom_encoder={datetime: str})
This coding instance tells `jsonable_encoder` to slide past `datetime` objects and not transform them into strings. Consequently, while remaining compliant with JSON syntax, the resulting dictionary will maintain the `datetime` object in its original form.
Durable Types Over Full JSON Strings
Evidently, Pydantic’s Jsonable dict capability passes beyond the mere advantages of using full JSON strings. Maintaining datatype identities during the conversion puts forward the benefit of preserving your model’s integrity. From preventing needless type casting to retaining actual python objects, this methodology allows your dictionary to perform as a more potent, dynamic, and robust variant of a traditional JSON object.
Moreover, the option to customize your own desiring encoders leads to remarkable extensibility. For example, suppose you’re handling a custom class in your model that isn’t ordinarily compatible with JSON syntax. In that case, you can construct a customized encoder for the class and pass this along to the `jsonable_encoder`. Accordingly, you’ll obtain a structured and explicit mapping just in one go, instead of an error or unexpected results.
These aforementioned grounds serve as unmistakable reasons why Pydantic’s Jsonable dict beats working with full JSON strings. The pervasive flexibility and supportive design presented by this feature will allow you to build loosely coupled and scalable systems with unprecedented simplicity.Without a doubt, Pydantic has evolved as a robust data validation and settings management library for Python. To implement a declarative representation, one of the common methods involve leveraging Pydantic’s inherent capabilities to convert validated data – say, in this case, models – into JSON-compatible dictionaries (Not full JSON string).
With Pydantic Models
Before we delve deeper, let’s understand what Pydantic models are. They allow the use of Python 3.6+ type annotations for data validation and serialization/deserialization. This process allows you to declare the shape and type of your data using classes.
To convert a Pydantic model into a dict that can be jsonified, the model instance has an inbuilt
xxxxxxxxxx
.dict()
function. Here’s how you use it:
xxxxxxxxxx
from pydantic import BaseModel
class UserModel(BaseModel):
name: str
age: int
user = UserModel(name='Foo', age=42)
user_dict = user.dict()
In this code snippet,
We start by importing the necessary modules. Our Pydantic class,
xxxxxxxxxx
UserModel
, is created with two fields: name and age. Then, we create an instance of this class. Finally, the variable
xxxxxxxxxx
user_dict
stores our dictionary.
The
xxxxxxxxxx
.dict()
method will output only JSON serializable types; any value or field annotated as
xxxxxxxxxx
bytes
will be encoded using base64, datetimes as iso-format strings, etc.
Configure Your Models
There may be instances where you require full control over the conversion process. The
xxxxxxxxxx
111Config
class in Pydantic becomes useful here.
Here’s an example using Config:
xxxxxxxxxx
from pydantic import BaseModel
class UserModel(BaseModel):
name: str
age: int
class Config:
json_encoders = {
datetime.datetime: lambda v: v.isoformat(),
# Other conversions
}
user = UserModel(name='Foo', age=42)
user_dict = user.dict()
In this example, Pydantic’s
xxxxxxxxxx
Config
class comes in handy if we want all datetime objects to be serialized using the ISO format. You can introduce further custom encodings as required.
Additional Arguments
The
xxxxxxxxxx
111.dict()
method provides additional parameters such as ‘include’, ‘exclude’, ‘by_alias’ etc. These parameters could further refine your data representation.
For example,
xxxxxxxxxx
user_dict = user.dict(include={'name'})
This would include just the ‘name’ field in the final dictionary.
For more insights into Pydantic model conversions, explore its official documentation.
By practical implementation of Pydantic features, we can generate a presentation layer suitable for different scenarios. It not only ensures a cleaner way of handling data but also brings the flexibility of tuning data representation as per the needs and requirements. However, be advised that these operations should be performed keeping in mind the computational costs associated with large data sets.Moving from ORM models to JSON-compatible dictionaries is a common necessity for any developer dealing with web applications. This could be to send data to a client application, like a frontend JavaScript view, or perhaps to store the data in a serialized format. The Pydantic library in Python facilitates this efficiently and effortlessly.
To create a JSONable dictionary from a Pydantic object, you’d want to use
xxxxxxxxxx
dict()
method provided by pydantic BaseModel.
Let’s take an example of a typical User model,
xxxxxxxxxx
from pydantic import BaseModel
class User(BaseModel):
id: int
username: str
email: str
In order to create a JSON compatible dictionary, it would look something like this:
xxxxxxxxxx
user = User(id=1, username='JohnDoe', email='johndoe@test.com')
json_compatible_dict = user.dict()
print(json_compatible_dict) # Output: {'id': 1, 'username': 'JohnDoe', 'email': 'johndoe@test.com'}
Note that this process does not generate a full JSON string, instead, it creates a Python dictionary that can be readily converted to a JSON string if you decide you need it later. However, It’s lightweight, less memory-intensive, and faster because no actual serialization takes place until needed.
But what about nested models? Glad you brought that up. Nested models are also handled gracefully by pydantic.
Consider this model setup,
xxxxxxxxxx
class Address(BaseModel):
street: str
city: str
zipcode: str
class User(BaseModel):
id: int
username: str
email: str
address: Address
Again, conversion happens the same way:
xxxxxxxxxx
address = Address(street='123 Elm Street', city='Springfield', zipcode='12345')
user = User(id=1, username='JohnDoe', email='johndoe@test.com', address=address)
json_compatible_dict = user.dict()
print(json_compatible_dict)
# Output:
# {
# 'id': 1, 'username': 'JohnDoe', 'email': 'johndoe@test.com',
# 'address': {'street': '123 Elm Street', 'city': 'Springfield', 'zipcode': '12345'}
# }
Great! So our structure remains intact. It is worth noting that Pydantic can handle more complex relations and hierarchies as well.
Now, keep in mind that ORM models may have certain types of data that aren’t JSON serializable. For instance, dates and datetimes, binary data, UUIDS, etc. In fact, the main reason serializers exist is to convert non-serializable types into a format that can be serialized. Thankfully, Pydantic came prepared, and it handles difficult special cases including recursive models (trees), complex collections such as tuples and frozensets, and even custom types and type annotations!
The longer I work with Python and APIs, the more I find myself gravitating towards these utility libraries like [Pydantic](https://pydantic-docs.helpmanual.io/) that make the development process smooth and hassle-free. This transformation process becomes particularly handy when building scalable applications following patterns such as the Repository-Pattern, where separating the domain model from the persistence model can be crucial for keeping your code maintainable.
The power of Pydantic lies in its ease of use for data parsing and the enforcement of type annotations. For Python programmers, Pydantic is priceless because it allows them to effortlessly validate and deserialize complex data types and ensure consistency in their code base.
Now, let’s delve into the use cases and key benefits of using Jsonable dict with Pydantic:
Use Cases:
- Enhancing Serialization:
In some instances, the process of serialization can be problematic due to non-serializable types like datetime or UUID. Pydantics’s
xxxxxxxxxx
jsonable_encoder
function comes to the rescue here, as it converts these non-JSON serializable types to JSON serializable forms.
Let’s look at a source code snippet that illustrates this:
xxxxxxxxxx
from pydantic import BaseModel
from fastapi.encoders import jsonable_encoder
class Item(BaseModel):
name: str
description: str = None
price: float
tax: float = None
item = Item(name="Foo", price=42.23)
json_compatible_item_data = jsonable_encoder(item)
- Complex Data Types:
For complex delivery payloads, Pydantic models themselves may contain sub-models resulting in deeply nested structures. Utilizing the
xxxxxxxxxx
jsonable_encoder
function ensures that these deep, recursive structures convert seamlessly into JSON-friendly dicts.
Follow the simple example below which demonstrates the usage of Jsonable dict in converting nested Pydantic model instances to a compatible dictionary in JSON format:
xxxxxxxxxx
from pydantic import BaseModel, Json
from fastapi.encoders import jsonable_encoder
from typing import Optional
class SubItem(BaseModel):
id: int
value: str
class MainItem(BaseModel):
name: str
description: str = None
subitem: SubItem
json_field: Optional[Json]
subitem = SubItem(id=123, value="A valuable item")
item = MainItem(name="Foo", description="A foo item", subitem=subitem, json_field={"key": "value"})
json_compatible_item_data = jsonable_encoder(item)
Key Benefits:
By employing Pydantic’s
xxxxxxxxxx
jsonable_encoder
function, we bring about efficiency and accuracy in our codebase. Particularly,
- Data Integrity: Enforcing type checking promotes consistent data structure across your application.
- Easier Troubleshooting: Validation errors are crystal clear, aiding in debugging any issues quickly.
- Hassle-free Serialization: Pydantic makes handling complex or custom data types effortless.
- Efficient Code: The simplicity of using Pydantic often results in more compact and efficient code.
You may learn more about Pydantic and its various applications here.The Pydantic library in Python is an interesting topic to cover. It’s a data parsing library that uses Python annotations for data validation and setting model attributes.
A noteworthy feature of Pydantic is the conversion ability from Pydantic models to jsonable dictionary but not full JSON string. This ensures that the output is a Python dictionary with values that are naturally JSON serializable like plain Python objects, such as integers, lists, and nested dictionaries.
Here’s a basic illustration:
xxxxxxxxxx
from pydantic import BaseModel
class User(BaseModel):
name: str
age: int
u = User(name='John Doe', age=30)
json_dict = u.dict()
In this example,
xxxxxxxxxx
User.dict()
method transforms the Pydantic model to a dictionary where all its values can be dumped into a JSON format.
It’s important to note that `dict()` does not return a full JSON string, but it outputs a Python dictionary understandable by the `json.dumps()` standard library method.
This makes it simpler for developers to use the data in further processing or transformations before finally encoding it to a JSON format.
For instance,
xxxxxxxxxx
import json
json_str = json.dumps(json_dict)
Looking forward to diving deeper, the dict() method can take several arguments to control its behavior. Some options include:
– ‘
xxxxxxxxxx
include
‘: Only the attribute names presented in the ’include’ set will be included.
– ‘
xxxxxxxxxx
exclude
‘: Exclude attributes whose names are declared in the ‘exclude’ set.
Again, here’s how we use them:
xxxxxxxxxx
json_dict = u.dict(include={'name'})
json_dict = u.dict(exclude={'age'})
The usefulness of converting Pydantic models to a jsonable dictionary lies in its flexibility and the precise control it provides over what gets included or excluded in the resultant dictionary.
To wrap up, utilizing Pydantic to convert Python models to a jsonable dictionary is a powerful tool when dealing with structured data. It permits you to have all the benefits of type hinting while still being able to quickly and effortlessly transform your structured Python data models into something that can be readily transformed into a JSON string. Thus, offering an easy, reliable, and efficient way of managing and manipulating structured data based on definitions.
You might want to check out the official Pydantic Documentation for an exhaustive understanding.