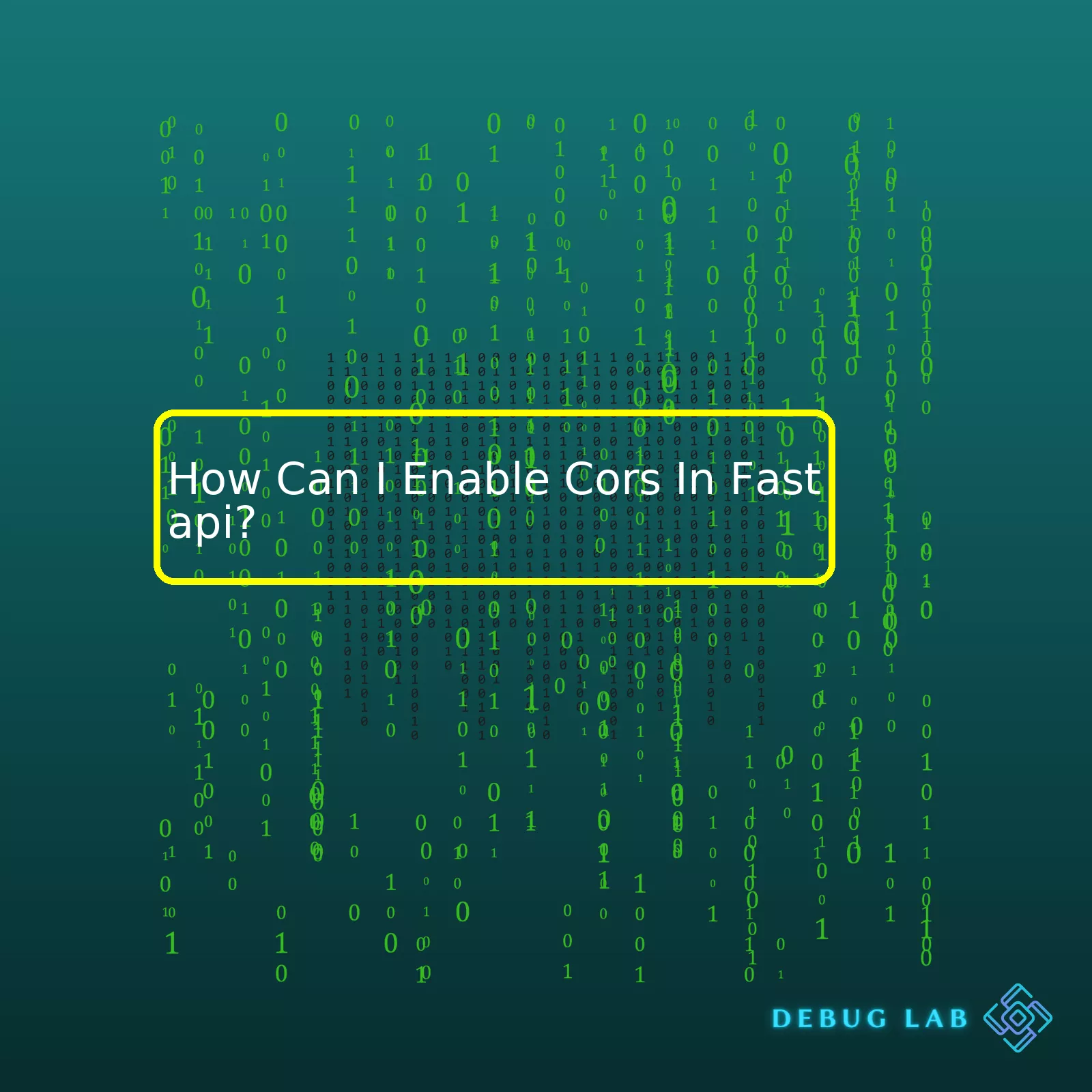
First of all, let’s generate the summary table:
html
Package | Description |
---|---|
fastapi.middleware.cors.CORSMiddleware |
This middleware helps us configure CORS settings for our project |
First of all, let’s generate the summary table:
html
Package | Description |
---|---|
fastapi.middleware.cors.CORSMiddleware |
This middleware helps us configure CORS settings for our project |
The concept of enabling CORS in FastAPI revolves around the CORSMiddleware, an inbuilt FastAPI library to manage these configurations. Let’s dig into the explained step-by-step process:
1.
from fastapi import FastAPI
2.
from fastapi.middleware.cors import CORSMiddleware
Above code lines import the necessary libraries. For enabling CORS, we will add ‘CORSMiddleware’ to our FastAPI application and mention the allowed origins as:
3.
app = FastAPI()
4.
origins = ["http://localhost:3000",]
Here, localhost:3000 is used as an example; replace this with the correct client-side URL or keep it [“*”] to allow all origins.
5.
app.add_middleware( CORSMiddleware, allow_origins=origins, allow_credentials=True, allow_methods=["*"], allow_headers=["*"],)
In the add_middleware method, along with the allowed origins, other parameters are also mentioned:
* allow_credentials allows session cookies from the client side to be sent to the server and get a response.
* allow_methods permits HTTP methods.
* allow_headers gives permission for headers according to incoming requests.
Whenever a browser does a cross-origin request, firstly it sends a preflight request before making the actual request. The preflight request helps the browser figure out whether the actual request is safe to send. These preflight-requests are built by the browser and handle itself without exposing APIs. Here, allow_methods and allow_headers become handy for managing HTTP methods and headers.
FastAPI uses Starlette for web routing which makes route level configuration possible too. In such cases, a decorator ‘@app.options(“/”, include_in_schema=False)’ uses the options method for handling preflight-requests manually.
Refer to the official FastAPI documentation for more in-depth wisdom about handling CORS config in your FastAPI applications.While working with FastAPI, enabling CORS (Cross-Origin Resource Sharing) is vital to ensure the browser can make safe HTTP requests to APIs from a different server or domain. In short, it solves a common problem in web development known as cross-origin or cross-domain requests.
The FastAPI framework has an easy-to-use middleware component for enabling CORS functionality, simply known as CORS middleware. This middleware provides a way to fit CORS related headers into the FastAPI application’s responses, which administrate how and when a client (like a web browser) can access a certain resource.
Let’s dive straight into the steps on how we can enable CORS in FastAPI:
Firstly, import the necessary components by adding this line at the start of your FastAPI application code:
from fastapi import FastAPI from starlette.middleware.cors import CORSMiddleware
Secondly, initiate your FastAPI app in the traditional way:
app = FastAPI()
Now, it’s time to add the CORS middleware. You need to include the list of allowed origins which will have permission to interact with your API.
Here’s an example:
origins = ["http://localhost", "http://localhost:8080", "https://yourfrontenddomain.com"] app.add_middleware( CORSMiddleware, allow_origins=origins, allow_credentials=True, allow_methods=["*"], allow_headers=["*"], )
In this snippet:
By following these steps, you’ve configured CORS policy for your FastAPI application.
Although this is a straightforward setup for allowing CORS within your FastAPI project, always remember to modify parameters such as `allow_origins` based on the environment (development/production) rules, and to secure sensitive data exposure.
For more detailed information about CORS in FastAPI, check out the official documentation. There you’ll find additional options for the CORS middleware – such as `expose_headers`, `allow_origin_regex`, `max_age` – and explanations on their usage.
Enabling Cross-Origin Resource Sharing(CORS) in FastAPI brings a host of benefits for every project. It not only enhances interaction with resource servers but also ensures the validity of requests while warding off security threats.
The importance of enabling CORS extends to:
Now, let’s turn our attention to enabling CORS in FastAPI. It’s simpler than you may guess. FastAPI provides a middleware specifically aimed at handling CORS permissions, eliminating the need for manual set up.
The
CORSMiddleware
is included in the
fastapi.middleware.cors
module, which enables the application to include CORS handling with just a few lines of code. Here is a straightaway example illustrating how it can be set up:
from fastapi import FastAPI from fastapi.middleware.cors import CORSMiddleware app = FastAPI() origins = [ "http://localhost.tiangolo.com", "https://localhost.tiangolo.com", "http://localhost", "http://localhost:8080", ] app.add_middleware( CORSMiddleware, allow_origins=origins, allow_credentials=True, allow_methods=["*"], allow_headers=["*"], )
In this code snippet, you declare a list of origins that are permitted to interact with your server’s data. For each declared origin, you enable all methods (such as GET, POST, PUT) and all headers by specifying them as “*”. The
allow_credentials=True
attribute allows cookies to be supported for cross-origin requests.
This setup ensures securer and efficient interaction between varying servers, prioritizing the recognized origins and adjusting in response to cross-origin requests. You can always modify these settings to match the specific requirements of your web application.
For additional information and further reading, consult the FastAPI official documentation on CORS.
Configuring Cross-Origin Resource Sharing (CORS) in FastAPI gives adjacent sites the ability to interact with your application. Here, it’s noteworthy that CORS is a security feature that limits how and when content from other websites can interact with yours.
In FastAPI, adding CORS middleware is relatively straightforward with the
CORSMiddleware
. Notably, when configuring or using the
CORSMiddleware
, you will need to determine your origin policy—specifically what hosts should be allowed to connect.
from fastapi import FastAPI from fastapi.middleware.cors import CORSMiddleware app = FastAPI() origins = [ "http://localhost.tiangolo.com", "https://localhost.tiangolo.com", "http://localhost", "http://localhost:8080", ] app.add_middleware( CORSMiddleware, allow_origins=origins, allow_credentials=True, allow_methods=["*"], allow_headers=["*"], )
Now, let’s break down some of this setup:
–
FastAPI()
: This calls and instantiates the FastAPI application.
–
origins[]
: This list defines which origins are accepted. You can provide specific domains here or use “*”, though the latter should only be used for development due to security concerns.
–
add_middleware()
: This is where we are configuring our CORS policy:
–
allow_origins=origins
: The list of origins defined above.
–
allow_credentials=True
: Allows cookies to be included in requests.
–
allow_methods=["*"]
: Any HTTP method is accepted.
–
allow_headers=["*"]
: Any HTTP header is accepted.
More advanced configurations involve setting up certain constraints around headers and non-specified methods. For instance, if you want just set GET and POST as the accepted methods, and only allow specific headers, you could alter the configuration thusly.
app.add_middleware( CORSMiddleware, allow_origins=origins, allow_credentials=True, allow_methods=["GET", "POST"], allow_headers=["Authorization"], )
These settings are largely determined by your application needs; remember that overly-permissive CORS policies can introduce security vulnerabilities. By using FastAPI’s
CORSMiddleware
, you’ll get significant flexibility in creating a CORS policy suiting your project’s unique demands.
For more information about CORS and its implementation in FastAPI, check out these resources from the FastAPI documentation , and Mozilla MDN Web Docs.Behind the scenes of any modern web application, there comes a host of different technologies and protocols to ensure seamless communication between the client and server, one such protocol being the Cross-Origin Resource Sharing (CORS). However, working with CORS can sometimes result in issues which need careful troubleshooting. Enabling CORS in FastAPI might encounter several issues, including:
– No ‘Access-Control-Allow-Origin’ Header
– Multiple CORS Origin
Let’s dissect each issue and suggest ways on how you can navigate them.
No ‘Access-Control-Allow-Origin’ Header
As the error message suggests, the response from your server does not include the necessary ‘Access-Control-Allow-Origin’ header allowing your client application to access the resource.
FastAPI has built-in support for handling CORS, thanks to the `fastapi.middleware.cors.CORSMiddleware`. Adding this middleware to your app will allow you to specify CORS configurations, including the “Access-Control-Allow-Origin” header.
You can add the middleware in your main FastAPI initialization using this code snippet:
from fastapi import FastAPI from fastapi.middleware.cors import CORSMiddleware app = FastAPI() # Middleware configurations origins = [ "http://localhost", "http://localhost:8080", ] app.add_middleware( CORSMiddleware, allow_origins=origins, allow_credentials=True, allow_methods=["*"], allow_headers=["*"], )
This piece of code tells FastAPI to include an “Access-Control-Allow-Origin” header in all responses, with its value set to either “http://localhost” or “http://localhost:8080”. This should resolve the ‘No Access-Control-Allow-Origin Header’ issue.
Multiple CORS Origins
Dealing with multiple possible request origins can be another source of CORS problems in FastAPI. You may wish to accommodate a situation where your front-end application runs in different environments with varying base URLs, thus needing more than one origin in the list of allowed origins.
Instead of hardcoding these origins, you can read them dynamically from a configuration file or environment variable.
Consider an example where we store our allowed origins as a comma-separated string in an environment variable:
import os from fastapi import FastAPI from fastapi.middleware.cors import CORSMiddleware app = FastAPI() # Fetching the origins from an environment variable origins = os.getenv("ALLOWED_ORIGINS").split(",") app.add_middleware( CORSMiddleware, allow_origins=origins, allow_credentials=True, allow_methods=["*"], allow_headers=["*"], )
In the updated version of our app initialization code, the origins are read from the “ALLOWED_ORIGINS” environment variable and then split into a list using the comma as a separator. This method allows greater flexibility and eases management of allowed origins for your application.
However, if you still encounter problems despite adopting the right procedural measures, it might be useful to check out the following recommendations MDN docs about implementing CORS properly. Always keep in mind that the browser security model is inherently tied to the concept of origins, and trying to circumvent it might lead to a whole array of other potential security issues. Stick to the rules of the game, and you should see your FastAPI app playing nice with CORS.When we talk about FastAPI, CORS – Cross-Origin Resource Sharing is a significant aspect that you need to understand. It can be quite daunting at first, but adding CORS is relatively simple and brings enormous benefits in protecting your web application against potential threats and allowing valid cross-origin requests.
FastAPI by default doesn’t allow CORS middleware for APIs, that means any request coming from a different origin would be rejected. But we have the option to explicitly enable it.
FastAPI uses an
add_middleware()
method to add middlewares including CORS middleware through which you can manage cross-origin requests. Here’s how:
from fastapi import FastAPI from starlette.middleware.cors import CORSMiddleware app = FastAPI() origins = [ "http://localhost:5000", ] app.add_middleware( CORSMiddleware, allow_origins=origins, allow_credentials=True, allow_methods=["*"], allow_headers=["*"], )
This makes use of FastAPI’s
.add_middleware()
function to enable CORS for certain origins. The important arguments to this function are:
–
CORSMiddleware
: This is the middleware class provided by Starlette, which FastAPI bases on.
–
allow_origins
: A list of origins that should be permitted to make cross-origin requests. For example, the frontend domain that would interact with the API.
–
allow_credentials
: Allow the response to be shared with sending credentials.
–
allow_methods
: Specifies the method or methods allowed when accessing the resource in response to a preflight request.
–
allow_headers
: Indicates which HTTP headers can be used during the actual request.
FastAPI offers even more customization according to your needs. In the give code snippet, we allowed all methods and headers from the mentioned origins, but you can specify only those you wish as follows:
app.add_middleware( CORSMiddleware, allow_origins=origins, allow_credentials=True, allow_methods=["POST", "GET"], allow_headers=["Content-Type"], )
Here we will allow only POST and GET methods, and responses should contain the ‘Content-Type’ header. You can change these parameters as per your requirements.
Incorporating this into your FastAPI setup will ensure flexibility while maintaining safety standards. By knowing how to manipulate the parameters of the CORSMiddleware class, you’re prepared to handle security issues associated with CORS effectively.Indeed, in modern web applications, ensuring a secure data connection between servers is crucial to protect sensitive user information. For this reason, Cross-Origin Resource Sharing (CORS) has become pivotal in enhancing application security across various technology suites. If you’re using FastAPI, a high-performance Python framework that offers single, simple, and direct routes, here’s how you can implement CORS.
FastAPI boasts starlette as an undertone, which briskly cooperates with the cors module to make this possible. To enable CORS, during the initial setup of your FastAPI application, take advantage of the
add_middleware
function inside FastAPI.
Here is how we practically apply it:
from fastapi import FastAPI from fastapi.middleware.cors import CORSMiddleware app = FastAPI() origin = [ "http://localhost:8080", # Allow localhost for development purposes ] app.add_middleware( CORSMiddleware, allow_origins=origin, allow_credentials=True, allow_methods=["*"], allow_headers=["*"], )
In the code snippet above, using the
app.add_middleware()
method, we instruct FastAPI to use the middleware of the
CORSMiddleware
module. We have also defined some rules within it.
–
allow_origins
: This defines accepted origins – or clients – for our server. It should be noted that any website or URL included in the list will now send requests to your FastAPI application without interference from the same-origin policy.
–
allow_credentials
: By enabling this option, cookies will be supported with requests they accompany.
–
allow_methods
: Here, we endorse all methods by setting it to “*”, alternatively, if only specific HTTP methods are needed for your application, this is the place to specify.
–
allow_headers
: This option enables all headers; however, similar to methods, feel free to select those necessary for your application requirements.(source)
Remember, when correctly placing CORS policies, they create an effective barrier preventing unsolicited third-party sites from gaining unwarranted access to precious user data. They boost the site’s resilience towards cross-site request forgery (CSRF), cross-site scripting (XSS), and data injection attacks. Therefore, ensuring these security checks are a consequence of the due diligence needed for safe and reliable web application deployment. Specifically focusing on FastAPI implementation, the
CORSMiddleware
provides a robust solution delivering an accessible approach to such vital configurations.
To enable Cross-Origin Resource Sharing (CORS) in FastAPI, there is a need to understand the essence of CORS and why it’s a fundamental aspect of today’s Web APIs. CORS is a security feature that allows or restricts web applications running on one origin to access resources from another origin. It’s used when a browser makes a request to a server with a different origin (domain, scheme, or port).
FastAPI has a built-in middleware for managing CORS requests, which seamlessly integrates with Starlette, the ASGI framework upon which FastAPI is built. With just a few easy steps, you can enable CORS on your FastAPI application.
The following code illustrates how to include FastAPI’s CORS middleware:
from fastapi import FastAPI from starlette.middleware.cors import CORSMiddleware app = FastAPI() app.add_middleware( CORSMiddleware, allow_origins=["http://example.com"], # List of sites allowed to make CORS request allow_credentials=True, allow_methods=["*"], # All HTTP methods allow_headers=["*"], # All headers )
In the
add_middleware()
function, multiple parameters are passed, each controlling a particular facet of the CORS policy:
* `allow_origins` is a list containing all origins that are permitted to make cross-origin requests. In production, this should only include the URLs of trusted websites; in the example, it’s set to `[“http://example.com”]`.
* `allow_credentials` is a Boolean value indicating whether to support cookies on requests. If true, it enables browsers to support cookies along with cross-origin requests.
* `allow_methods` is an array of HTTP methods (like GET, POST, PUT, etc.) that are allowed while making the cross-origin request.
* `allow_headers` list specifies the HTTP headers that the client is allowed to use in the actual request.
These practices provide a standard way of enabling CORS in FastAPI, however it is worth noting some best practices for CORS configurations, particularly in a production environment.
Source: FastAPI Documentation
FastAPI
application, and add the
CorsMiddleware
to your application’s middleware stack using the
add_middleware()
function.
A typical use case might look like:
from fastapi import FastAPI from starlette.middleware.cors import CORSMiddleware app = FastAPI() origins = ["http://localhost", "http://localhost:8080",] app.add_middleware( CORSMiddleware, allow_origins=origins, allow_credentials=True, allow_methods=["*"], allow_headers=["*"], )
Note: Remember that CORS policies are based on trust. In the origins list, we’ve placed accepted origins which are trusted by us. You should replace it with your own origins as per requirements and shouldn’t enable all methods and headers if not necessary due to security reasons.
The parameters hold the following meanings:
With its scope clearly defined, integrating advanced capabilities such as CORS in your FastAPI application becomes less complicated and easy to comprehend. For more details and specific controls over CORS, please refer to FastAPI Documentation.