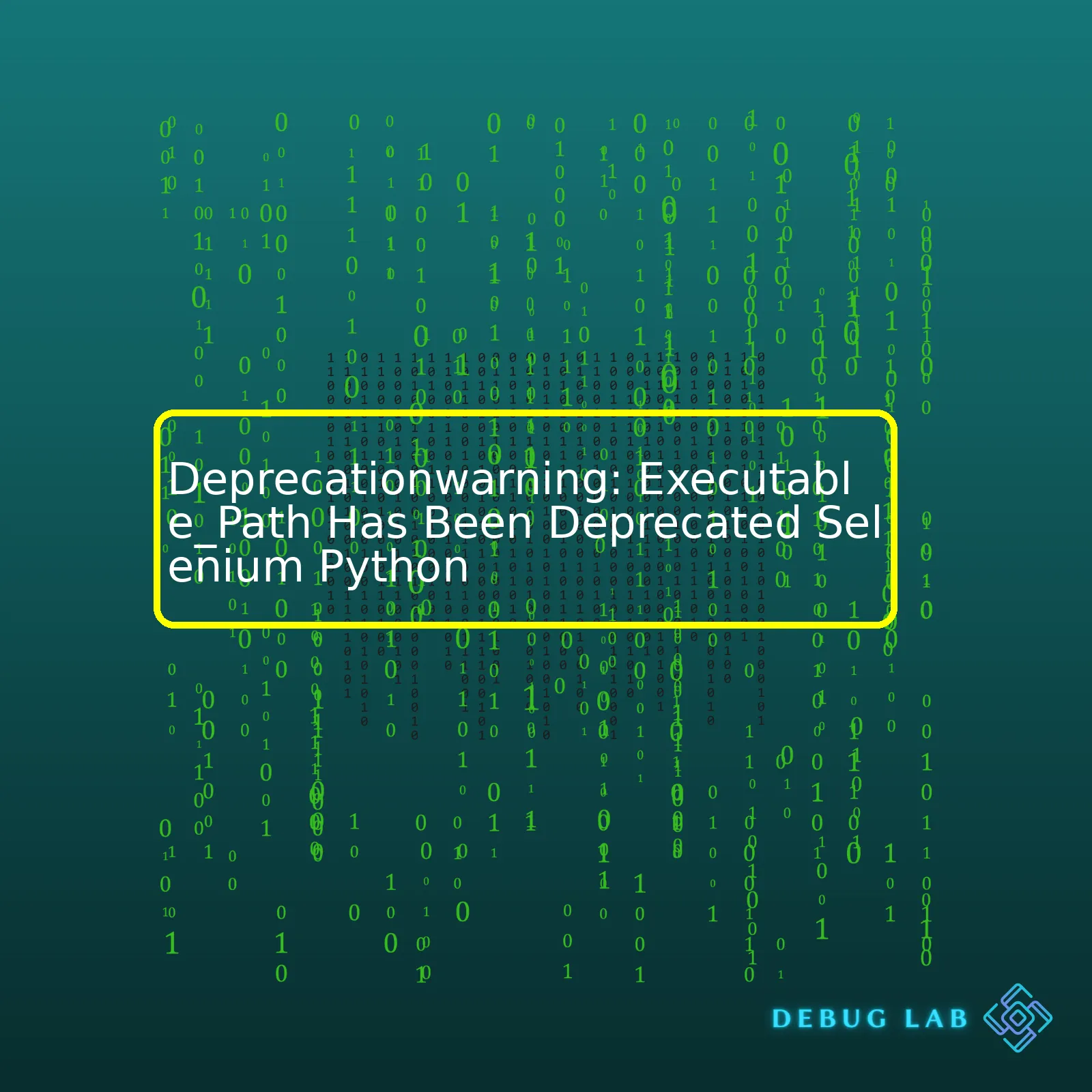
Here’s what your deprecation notice means:
Aspect | Description |
---|---|
Deprecated Feature | Executable_Path in Selenium Webdriver Python |
Implication | May cause compatibility issues or loss of certain functionalities in the future |
Solution | Use alternatives provided by Selenium Development Team |
Release Notes | In later versions, this functionality would be removed entirely, so transitioning is advisable |
Now, let me explain exactly why this happens and how you can navigate away from ‘executable_path.’ Selenium uses browser drivers (Chrome, Firefox, etc.) to connect and interact with web applications. Originally, it was necessary to specify the path of these drivers explicitly using the ‘executable_path’ argument when creating a WebDriver instance.
selenium.webdriver.Firefox(executable_path='/path/to/geckodriver')
However, this practice has changed now. For modern Selenium versions (4+), selenium will automatically discover available drivers on your system PATH environment variable. You are encouraged to add your driver executable onto your system PATH, replacing the need for ‘executable_path.’
You will create a WebDriver instance like below:
selenium.webdriver.Firefox()
In other words, the table above indicates that using ‘executable_path’ while creating WebDriver instances is deprecated and might obstruct your code execution in future Selenium versions. Instead, make sure to include your browser drivers in your System PATH for seamless Selenium operations.
For more in-depth details, kindly visit official Selenium Documentation.Sure, let’s delve into this topic.
When you use
executable_path
in Selenium Python, you might encounter a DeprecationWarning. This warning is an alert from the developers that a portion of the code (an object, method, function or argument) is at the brink of getting deprecated, meaning it is getting outdated and there may be a better option available.
In our case, using
executable_path
has been depreciated for initializing the webdriver. The origin of this warning can be traced back to updates made to the Selenium library. Python will continue executing your script with no issues despite seeing this warning, as it aims only to notify you of future changes.
After seeing this warning, as an updated approach, you should consider using Service objects from selenium.webdriver service module. Here is an example:
from selenium import webdriver from selenium.webdriver.chrome.service import Service driver = webdriver.Chrome(service=Service('/path/to/chromedriver'))
As opposed to the old approach where we used:
driver = webdriver.Chrome(executable_path='/path/to/chromedriver')
Note: replace ‘/path/to/chromedriver’ with your actual chromedriver path.
By using the
Service()
class, you stay up-to-date with the current Selenium practice and avoid these deprecation warnings.
Here’s why complying with such changes is beneficial for you:
- Staying Update: Keeping your code aligned with the latest version of libraries ensures it is never left behind.
- Avoid Breakdown: Deprecated codes may stop working in future versions, hence updating them can avoid potential breakdowns.
- Better Alternative: Updated pieces of code often come with enhanced functionality, improved performance, and better methods.
This transition is part of an ongoing effort by the community of open-source contributors to improve and update the Selenium Python Bindings along with removal of outdated elements. You can refer to Selenium Official Documentation for more details.
However, please note that if you’re dealing with older modules or scripts, you and your team should plan to make the necessary updates in codebase to avoid any possible issues post-deprecation.An intriguing shift in the Selenium framework for Python is the deprecation of the ‘executable_path’ parameter. The
Selenium
WebDriver utilizes this parameter to instantiate browser drivers like ChromeDriver, GeckoDriver, and EdgeDriver.
Transitioning into the specifics of what has changed, let’s address how Selenium usually connects with a browser driver using the ‘executable_path’ argument:
from selenium import webdriver driver = webdriver.Chrome(executable_path='/path/to/chromedriver')
This snippet initiates an instance of Chrome Browser by explicitly defining the location of chromedriver. However, this approach is experiencing a transition with the concern of ‘executable_path’ being deprecated in favor of another method that relies on browser binaries being automatically discovered from system paths.
Here are two primary issues which warrant the depreciation:
– Repetition of Code: Different instances of the WebDriver necessitate specifying the location of driver binary files with each execution. It leads to unnecessary repetition of code.
– Cross-Platform Compatibility: There may be indifferences when the script is supposed to act on distinct operating systems due to differences in file path formats.
To navigate around these detriments comes the replacement: an emphasis on adding the browser driver directories to the system PATH variable. Under this process, the WebDriver instance can be created without specifying an ”executable_path’:
from selenium import webdriver # No need to specify the executable_path driver = webdriver.Chrome()
Selenium WebDriver would then probe the system’s PATH for locating the requisite binary. Consequently, your scripts turn more portable and less error-prone. However, modifying system PATH may not always be feasible – especially in places lacking access to secured environments or limited system privileges. As a workaround, you can use WebDriver Manager for Python, which locates browser drivers that are compatible with installed browsers, downloads and uses them.
from webdriver_manager.chrome import ChromeDriverManager from selenium import webdriver driver = webdriver.Chrome(ChromeDriverManager().install())
So far, we’ve analyzed the shift away from extensive usage of ‘executable_path’ within Selenium Python framework towards non-replicative, cross-compatible methods. These alternative techniques bank either on configuring system PATH or leveraging libraries like WebDriver Manager.
Please note deprecation does not imply instant absence – it just means the element is not advised for use, nonetheless, existing as legacy support for backward compatibility. Furthermore, future versions may no longer include the deprecated feature, so it’s prudent to start preparing for a transition [Deprecation Warning](https://docs.python.org/3/library/exceptions.html#DeprecationWarning).
Also, please remember that ensuring functional Selenium scripts requires frequently downloading browser driver binaries matching the corresponding browser version which could lead to a hassle over time. Be accustomed to libraries such as WebDriver Manager can lessen manual interventions and create more performant, sustainable scripts.
Lastly, do visit the official Selenium Python bindings documentation and consider, for active community support, checking out communities like StackOverflow.
The rapid evolution of software practices imposes the necessity for staying updated to consequential changes. Thriving as a professional coder can often entail adapting to such shifts smoother than others.Whether you’re an experienced developer or new to Selenium and Python, you might have come across this warning message:
DeprecationWarning: executable_path has been deprecated
. This occurs when the web driver instance is initialized with an executable path. The
executable_path
argument in Selenium web driver classes such as
webdriver.Chrome()
,
webdriver.Firefox()
, is now deprecated and may be removed in future releases.
So, what should you do when your existing code depends heavily on it? Fortunately, as we delve deeper into this topic, you will find there is a variety of viable alternative approaches to address this deprecation and keep your applications functional and up-to-date.
One alternative approach replaces
executable_path
entirely with system’s PATH environment. The PATH is the system variable that operating systems use to locate executables from commands typed in from the command prompt. Thus, if your webdriver’s executable file i.e., chromedriver.exe or geckodriver.exe (for Firefox), is located in any directory that is part of your system’s PATH, initialization of driver instance can be done without specifying any path.
Here’s how you can implement it:
from selenium import webdriver # Make sure that ChromeDriver is added to your system's path. driver = webdriver.Chrome()
Another approach is by using WebDriver Manager for Python which allows you to manage browser drivers seamlessly. WebDriver Manager will handle the download, extraction, and requisite setup of browser-specific drivers for you. This can be accomplished by installing WebDriver Manager using pip:
pip install webdriver-manager
Then, alter your Selenium scripts to use WebDriver Manager:
from selenium import webdriver from webdriver_manager.chrome import ChromeDriverManager driver = webdriver.Chrome(ChromeDriverManager().install())
Same way for Firefox:
from selenium import webdriver from webdriver_manager.firefox import GeckoDriverManager driver = webdriver.Firefox(executable_path=GeckoDriverManager().install())
Both alternatives eliminates the need to manually update WebDriver binaries(APi to interact with web browsers).
Given these points, when confronted with the DeprecationWarning for
executable_path
, developers have several options. These alternative methods not only address the ‘deprecated’ concern but also provides simplified and modern ways to maintain interoperability of their applications with different web browsers.
For more detailed information, check out Selenium’s official documentation [source]. Don’t forget to always stay updated with latest release notes and changes, ensuring smooth transition and operation of your automated tasks/scripts.
The deprecation of
executable_path
, an attribute in the Selenium WebDriver API often used in Python scripts, has brought about notable changes to how we approach automation testing. It is imperative for Selenium library users, most especially those who use Python, to acknowledge this change and adapt their scripts accordingly.
What is ‘executable_path’ in Selenium?
The
executable_path
is a parameter that was traditionally used to specify the path where the webdriver file (like chromedriver.exe or geckodriver.exe) resided. This made it possible for Selenium to communicate with the chosen browser for web automation.
from selenium import webdriver driver = webdriver.Chrome(executable_path='/path/to/chromedriver')
This method worked without any issue but has been deprecated in the new releases of Selenium.
Impact on Selenium Scripts
‘DeprecationWarning: executable_path has been deprecated’ is a common warning message you may encounter if you are using the old method of setting up driver’s path. Script-wise, the deprecation implies that developers must modify their existing code to replace instances of
executable_path
usage. If not replaced, the script could likely fail in future versions of Selenium when the deprecated feature gets removed entirely.
Solution to Deprecated Executable_Path
The good news is Selenium’s development team has provided a more practical solution for declaring driver’s path. Now, you are advised to add WebDriver’s path to your system’s PATH variable directly instead of manually specifying it every time through
executable_path
. This is done so you can simply call the driver like this:
from selenium import webdriver driver = webdriver.Chrome()
Achieving Compatibility – Updating System’s PATH Variable
The major task now lies in updating your system’s PATH variables to include your WebDriver’s location. Below demonstrates how to update your PATH in different OS:
- Windows: Open the System Properties → Advanced → Environment Variables. Here, under system variables, look for PATH, click ‘Edit’, and add your WebDriver’s path at the end.
- Linux/MAC: In Linux or MAC, open your ‘.bash_profile’ or ‘.bashrc’ file and add the line ‘_export PATH=$PATH:/your/webdriver/path_’
Once this is done, your operating system will recognize the WebDriver on its own, and Selenium won’t require the
executable_path
separately anymore. This ensures the longevity of your scripts, making them resilient against potential issues linked to the deprecation of certain features within the Selenium WebDriver API.
To sum up, while the deprecation of ‘executable_path’ might seem like a step backward, it’s actually a move towards more efficient scripting. With your WebDriver’s path added to the system’s PATH, running Selenium scripts becomes much simpler and cleaner, aiding in improved script maintenance and compatibility with future Selenium updates.
View full documentation on Selenium here.
It goes without saying that deprecation is an integral part of software development. And quite often, when using third-party libraries like Selenium in Python, we will encounter ‘warnings’. So, right off the bat, let’s address this specific warning that you mentioned: `DeprecationWarning: executable_path has been deprecated`.
Here are a few practical tips for upgrading your codebase following this deprecation notice:
Understanding the Deprecation
Before you can effectively address any deprecation, it is crucial to fully comprehend what has been deprecated and why. In this case, Selenium’s `executable_path` is being flagged. This was previously used to specify the path of the browser driver.
According to official communications from the Selenium team, `executable_path` is being phased out. They now recommend that these binaries should be included in your System PATH. To do this, you can either manually edit your system variables or utilize browser-specific drivers that automatically find these binaries for you, such as webdriver_manager for Python.
Implementation of New Mechanism
Now that you know about the ‘why’, let’s move on to the ‘how’. You need to replace the usage of `executable_path` with the improved handling of WebDriver executables.
If you were using:
from selenium import webdriver
browser = webdriver.Chrome(executable_path='/path/to/chromedriver')
Now you can shift to:
from webdriver_manager.chrome import ChromeDriverManager
from selenium import webdriver
browser = webdriver.Chrome(ChromeDriverManager().install())
What happens here is, `ChromeDriverManager().install()` checks for the existing latest webdriver binary in cache or download it if not available and return the webdriver path which can be passed directly to `webdriver.Chrome`.
Ensuring Compatibility
With any updates or modifications to source code, it is crucial to perform rigorous testing to ascertain no subsequent modules have been impacted inadvertently.
Do verify if the updated code functions correctly with different browsers that your application supports (like Chrome, Firefox, etc.). Run comprehensive tests to ascertain this aspect.
Monitor for Other Deprecation Notices
Since software libraries are continually updated, make sure you keep an eye out for future deprecations and warnings. Constant vigilance for these notices will guarantee that your code base remains current, efficient, and free of redundancies. Please remember to regularly consult release notes, and other documentation associated with Selenium and other core libraries or frameworks that your application depends upon.
Remember, deprecation means the feature is on its way out, and although not immediately removed, it will be gone eventually. It’s wise to take action proactively, understanding the reason behind the deprecation and efficiently making required changes to ensure the sustainability of your codebase.
As a professional developer, one of my key roles is to keep up-to-date with the latest technological advancements and changes in APIs that can significantly impact my coding practices. Recently, there has been a crucial update in Selenium WebDriver’s Python bindings that developers must be aware of. The
executable_path
attribute has been deprecated. Let me explain what this means for your existing code based on Selenium WebDriver.
Deprecation is a status applied to software features or functions to indicate that they should not be used anymore, typically because they’ve been superseded by newer features or functionality. The goal is to guide developers away from older methods and ease the transition to new ones.
In Selenium Python API, this relates to the
executable_path
argument in the driver instantiation. Previously, you would define the path to your webdriver executable like this:
from selenium import webdriver driver = webdriver.Chrome(executable_path='/path/to/chromedriver')
However, this approach has been deprecated in recent versions of Selenium WebDriver.
The latest Selenium WebDriver recommends another method for specifying the driver path. Now, we need to use WebDriverManager, a tool that automates the management of binary drivers for different browsers.
First, you need to install WebDriverManager using pip:
pip install webdriver_manager
Then, you can implement this in your Selenium script as follows:
from selenium import webdriver from webdriver_manager.chrome import ChromeDriverManager driver = webdriver.Chrome(ChromeDriverManager().install())
Here,
ChromeDriverManager().install()
automatically downloads the appropriate driver version and returns the path to it.
Notably, this change requires some modifications to your legacy Selenium scripts. However, it offers several advantages:
- It automates the management of binary drivers for different browsers.
- With every execution, it checks for the latest version of the WebDriver.
- It saves significant time in setting up a new test environment by avoiding manual installations.
Fortunately, the Python community provides many resources to learn more about these updates. While the [official Selenium documentation](https://www.selenium.dev/documentation/en/) remains an auspicious starting point, several bloggers and industry experts offer their personal experiences and tricks of the trade. Remaining abreast of these developments often entails a mixture of regular study, hands-on experimentation, and collaboration with other developers.
Adapting to changes in Selenium WebDriver API contributes to maintaining a robust and efficient codebase. Although it might involve an initial learning curve, the advantages of staying updated are immense in the long-term reliability and efficiency of your automated testing system.
Innovation and evolution are at the heart of technology. Therefore, being aware and adaptable to such changes makes us better developers.Certainly, upon facing the “DeprecationWarning: executable_path has been deprecated” when using Selenium in Python, it indicates that you are using an older way to instantiate your browser driver. In recent versions of Selenium, webdriver’s
Firefox
,
Chrome
and others don’t recommend directly setting the path to the drivers’ executables by using the `executable_path` parameter.
Below is a brief example of how `executable_path` has traditionally been used:
from selenium import webdriver driver = webdriver.Chrome(executable_path='/path/to/chromedriver')
However, this approach is obsolete and can result in aforementioned deprecation warning. So, how can we rectify this situation? Instead of using the deprecated `executable_path`, we should use `service` and Python’s built-in `Service` class for creating an instance of our desired browser. Here’s what the updated code would look like:
from selenium import webdriver from selenium.webdriver.chrome.service import Service driver = webdriver.Chrome(service=Service('/path/to/chromedriver'))
But you also mentioned the desire to implement `DesiredCapabilities`. Desired Capabilities are essentially a series of key-value pairs that allow you to configure your webdriver properties such as changing browser settings or passing parameters to tweak your testing environment. They’re a powerful tool to customize your testing experience but must be used carefully.
To make use of DesiredCapabilities with the Chrome WebDriver, you would proceed in the following manner:
from selenium import webdriver from selenium.webdriver.chrome.service import Service from selenium.webdriver.common.desired_capabilities import DesiredCapabilities caps = DesiredCapabilities.CHROME.copy() caps.update({'someCapability': 'someValue'}) driver = webdriver.Chrome( service=Service('path/to/chromedriver'), desired_capabilities=caps)
We first copied the default capabilities from `DesiredCapabilities.CHROME`, then updated the copied capabilities with our own custom configurations before finally using them to create our driver.
Ultimately, transitioning away from using the deprecated `executable_path` attribute and incorporating `DesiredCapabilities` significantly increases flexibility and customization options in your test configurations. With this modification, your testing environment can be better controlled and adjusted according to your project needs. References can be found on selenium’s official documentation website here and here.In the world of software development, dealing with warnings such as the
DeprecationWarning
in Selenium Python is a standard task. These don’t necessarily break your code at first, but they inform you about elements of your code that might cease to function correctly in future versions – essentially, giving you time to make adjustments for smooth transitions.
In the case of the DeprecationWarning pertaining to
executable_path
, we need to lean onto some additional insight. The
DeprecationWarning: executable_path has been deprecated
issue arises when using web drivers in Selenium Python, where the
executable_path
argument used to specify the location of the webdriver’s binary has long been a standard approach. Recent updates, though, have flagged this use as deprecated. This warning means that Selenium is encouraging developers to adopt new strategies instead of using the
executable_path
argument.
Following good practice, there are a few paths a developer could potentially take here to keep their code base up-to-date and error-free:
- Switching to use WebDriver Manager: WebDriver Manager is a useful tool that will automatically manage the browser driver (chromedriver, geckodriver, etc.) versions and configurations for you. You can add it to your project with pip install webdriver_manager.’
from selenium import webdriver from webdriver_manager.chrome import ChromeDriverManager driver = webdriver.Chrome(ChromeDriverManager().install())
- Storing the WebDriver executables path in the System Environment Variables: Rather than specifying path in code, you can set it directly into system properties. In Windows, this would be through the Advanced System Settings.
So, when facing DeprecationWarning like this in Selenium Python, remember it’s not a cause for alarm, rather it insists on improvements. Making thoughtful choices now can help ensure your code retains its functionality in the face of changing standards.
Remember for further details do not hesitate to visit the official Selenium documentation page