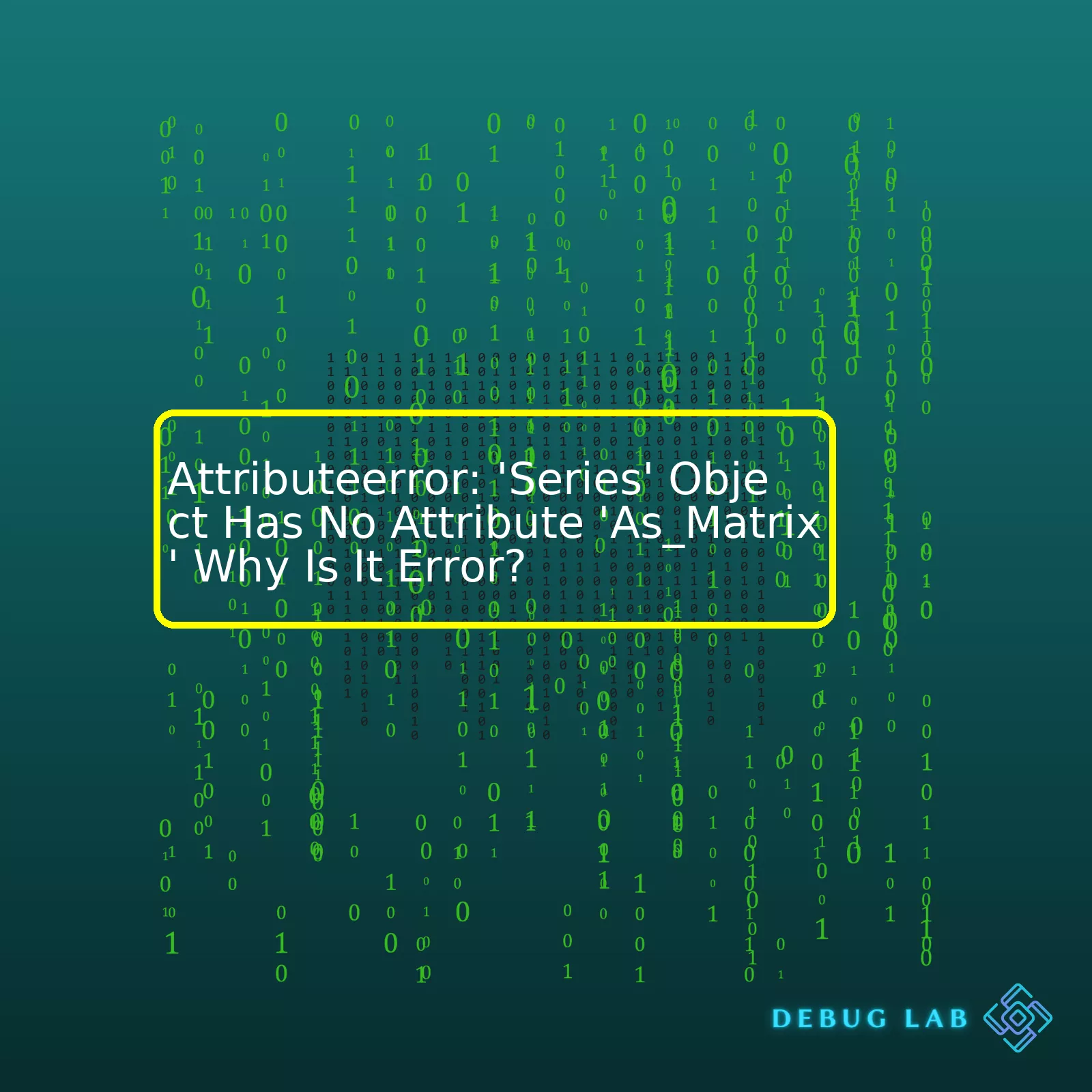
Below is a simple visualization summary in HTML table format:
Error | Reason | Solution |
---|---|---|
AttributeError: ‘Series’ object has no attribute ‘as_matrix’ | The function as_matrix() has been deprecated since version 0.23.0. | Use to_numpy() or values attribute |
The two alternatives for `as_matrix()` are `to_numpy()` or the `values` attribute – these will work suitably without error. Simply replace your previous `.as_matrix()` call with `.to_numpy()` or `.values`.
Here’s the replacement in code:
Instead of having something like:
dataset = pd.Series([1, 2, 3])
matrix = dataset.as_matrix()
You should now use either of these two options:
1. Use `to_numpy()` function:
xxxxxxxxxx
dataset = pd.Series([1, 2, 3])
matrix = dataset.to_numpy()
2. Use `values` attribute:
xxxxxxxxxx
dataset = pd.Series([1, 2, 3])
matrix = dataset.values
Both `to_numpy()` and `values` return the same output and they do the exact thing `as_matrix()` used to do which is to return the numpy representation of the dataframe.
Keep in mind, if you still come across the same error after implementing the suggested changes, it could be because you may be using an older version of pandas. I highly recommend updating your pandas library to its latest version to ensure all functions and attributes work properly. You can refer to the official pandas documentation for more details and understanding.When it comes to Python programming, especially in data analysis with libraries such as NumPy and pandas, you may sometimes encounter an `AttributeError: ‘Series’ Object Has No Attribute ‘As_Matrix’`. This error usually originates from trying to use a non-existent method,
xxxxxxxxxx
as_matrix()
, on a pandas Series object.
A pandas Series is a one-dimensional labeled array capable of holding any data type, be it integers, strings, floating points or Python objects. You are likely to obtain this when accessing a DataFrame column. As you might already know, DataFrame is a two-dimensional size mutable, potentially heterogeneous tabular data structure from the pandas library.
The method
xxxxxxxxxx
as_matrix()
was used to convert the given pandas DataFrame / Series into its numpy-array representation. However, as of Pandas 0.23.0 (released May 15, 2018), the
xxxxxxxxxx
as_matrix()
function was deprecated, and subsequently removed in later versions. That explains why you can’t find the method in recent pandas versions.
If you’re looking to convert a pandas DataFrame or Series to a NumPy array, which most probably is your objective if you’re using the
xxxxxxxxxx
as_matrix()
method, you should instead now use the more favored
xxxxxxxxxx
.values
or
xxxxxxxxxx
.to_numpy()
.
Here is how you can do it:
.values property:
xxxxxxxxxx
numpy_array = df['Column_Name'].values
In this instance, `df` is the DataFrame and `’Column_Name’` is the specific column that you are dealing with.
.to_numpy() function:
xxxxxxxxxx
numpy_array = df['Column_Name'].to_numpy()
The confusion with functions like
xxxxxxxxxx
as_matrix()
arises because of shifts and developments in libraries like pandas. Changes like these are made often due to improvements or optimizations in the library, leading to the deprecation of some functions or methods. So, always make sure to refer to the latest official pandas documentation when exploring new functions.
So, to avoid getting errors like these in future, it’s recommended to regularly update your pandas library and stay aligned with changes in the API by checking the discussions on websites like Stackoverflow or other coding forums that keep track of issues encountered by real users.As a dedicated Python developer, I frequently encounter Attribute Errors which are common while working with complex packages like Pandas. The attribute error we are specifically addressing here is
xxxxxxxxxx
AttributeError: 'Series' Object Has No Attribute 'as_matrix'
. Understanding why this error occurs surely helps in finding and applying the correct solutions.
To understand the root cause of the error, you first need to look at what attributes in Python actually mean. Attributes in Python refer to data elements or methods associated with a class. When we attempt to call an attribute that is not attached to the said Object, it raises an AttributeError. The text of the error message specifically mentions this situation: ‘Series’ object has no attribute ‘as_matrix’.
Here, the ‘Series’ is your object, a fundamental pandas data structure, and ‘as_matrix’ is supposed to be an attribute, a method in this case. But why does the error say there’s no such attribute when it should exist?
The straight answer is that the ‘as_matrix’ method has been removed in recent versions of Pandas due to several reasons. Majorly, the method was redundant because performing similar tasks could easily be done using other methods. The Pandas team announced the deprecation in Pandas 0.23.0 and finally removed it in Pandas 1.0.0 ([Pandas Documentation](https://pandas.pydata.org/pandas-docs/version/0.25.3/reference/api/pandas.Series.as_matrix.html)).
xxxxxxxxxx
# old way using as_matrix (which would produce the error message)
matrix = series.as_matrix()
# new recommended ways
matrix = series.values # yields a NumPy array
df = pd.DataFrame(series) # if you want it as a one-column DataFrame
Simply put, the reason behind the attribute error message is that the code is calling an attribute or method ‘as_matrix’ that no longer exists on pandas’ Series object due to changes in the newer versions of the package. By adapting the code to use ‘values’ or convert the series into a DataFrame, we can circumvent the issue effectively without compromising the code’s overall functionality.
I highly recommend getting comfortable with these kinds of attribute transformations so that when libraries update, certain attributes get deprecated, or even when debugging problematic portions of code, you’ll know the full extent of what needs to be corrected. Overcoming Attribute Errors requires precise understanding of how objects and their attributes function within Python’s complex universe.Ah, the perpetual confusion of AttributeError. This is a common error that Python programmers run into quite frequently, especially those fairly new to the language or working with complex data structures. An AttributeError typically means that you are trying to access or call an attribute or method that does not exist for a certain object.
Let’s take a closer look at this error message:
xxxxxxxxxx
AttributeError: 'Series' object has no attribute 'as_matrix'
. Notice that it specifically tells us that a ‘Series’ object doesn’t have the ‘as_matrix’ attribute.
A Series object comes from the pandas library, a staple in data science coding with Python. It is essentially a one-dimensional labeled array capable of holding any data type (integers, strings, floating point numbers, Python objects, etc.). You might be dealing with a pandas Series object if you’re manipulating rows from a dataframe or doing certain types of data wrangling.
But here comes the tricky part – the ‘as_matrix’ method. You see, there was indeed a time when Pandas Series had this handy method named ‘as_matrix’. However, starting with pandas version 0.23.0, which was released in May 2018, the ‘as_matrix’ function has been deprecated in favor of ‘values’ or ‘to_numpy’.
So what’s happening here? You’re simply trying to use a method on an object that no longer exists. Hence the
xxxxxxxxxx
AttributeError: 'Series' object has no attribute 'as_matrix'
.
Let’s see how we can update our code to avoid this error:
Previous:
xxxxxxxxxx
import pandas as pd
s = pd.Series([1, 2, 3])
matrix_data = s.as_matrix()
Updated:
xxxxxxxxxx
import pandas as pd
s = pd.Series([1, 2, 3])
matrix_data = s.values
Or:
xxxxxxxxxx
import pandas as pd
s = pd.Series([1, 2, 3])
matrix_data = s.to_numpy()
So, now those pesky ‘AttributeError’ tweaks should be a bit clearer. Remember, errors aren’t always your enemy. Sometimes they are just pointers showing you where you need to improve. Your programming journey will likely be filled with ‘AttributeErrors’, but that’s alright. Embrace them and learn from them. Always ensure to do regular checks on the modules and functions you’re using, especially when working with open-source libraries like pandas. Minor changes or deprecation could result in AttributeErrors. Regularly check the official pandas documentation for updated methods and attributes.To address the topic at hand, let’s first delve into what a ‘Series’ object is. In Pandas, one of the main data structures used for data manipulation is Series. It represents a uni-dimensional labeled array capable of holding data of any type (integer, float, string, python objects, etc.).
Attributes are associated with each series object, allowing access to its internal properties that help us perform different operations on a series. There exists a multitude of attributes on ‘Series’ objects like
xxxxxxxxxx
size
,
xxxxxxxxxx
dtype
,
xxxxxxxxxx
values
, and many more.
Now, onto the crux of the matter – why do you encounter an “AttributeError: ‘Series’ object has no attribute ‘as_matrix'”? The reason traces back to changes in newer versions of the ‘Pandas’ package.
The method
xxxxxxxxxx
as_matrix()
was a function associated with ‘DataFrame or Series’ objects that used to return the values as a numpy.ndarray. But it was deprecated since version 0.23.0 and was entirely removed in Pandas v1.0.0.
So, if you’re using a later version of Pandas and trying to utilize the
xxxxxxxxxx
as_matrix()
attribute on a ‘Series’ object, you’ll run into this error because this attribute doesn’t exist anymore. You’d face a similar outcome if you tried to access an attribute or method that isn’t defined on any object.
Consider the following example:
Say you have a series object, named serieA:
xxxxxxxxxx
import pandas as pd
data = [1,2,3]
serieA = pd.Series(data)
and you are trying to execute:
xxxxxxxxxx
matrix_data = serieA.as_matrix()
This will lead to Python throwing an AttributeError, saying that the ‘Series’ object has no attribute ‘as_matrix,’, just like your issue.
Instead, to get the matrix representation of a Series object, use the
xxxxxxxxxx
.values
or
xxxxxxxxxx
.to_numpy()
attribute:
xxxxxxxxxx
matrix_data = serieA.values
or
xxxxxxxxxx
matrix_data = serieA.to_numpy()
Both
xxxxxxxxxx
.values
and
xxxxxxxxxx
.to_numpy()
will return the same numpy.ndarray:
xxxxxxxxxx
array([1, 2, 3])
In sum, it is crucial to stay updated with changes in the libraries you use, be cautious in adopting deprecated methods, and always check which versions your project requirements need. For more details, you can refer to the official Pandas documentation.
Sure, let’s dive into the nuances of pandas DataFrame as_matrix() and .values functionality in Python coding. Let’s imagine a typical error like the one you’ve described: “
xxxxxxxxxx
AttributeError: 'Series' object has no attribute 'as_matrix'
“.
In essence, what’s happening here is that you’re trying to use a function/method “as_matrix()” that no longer exists or is deprecated in later versions of pandas library. The alternative method that should be used in place of it is “.values”.
The ‘as_matrix()’ function was a part of older versions of the pandas library, specifically before version 0.23.0. Its purpose was to convert a pandas Series into a numpy array. However, with the updates and improvements in the pandas library, developers found more efficient ways to get the data values from a DataFrame or Series and as_matrix() was deprecated in favor of .values.
So, if you originally had code that looked like:
xxxxxxxxxx
df.as_matrix()
You can replace it with:
xxxxxxxxxx
df.values
It is therefore critical to adapt to these changes not just for compatibility purposes but also to stay updated with the latest methodologies provided by the updated libraries.
Key Differences – as_matrix() vs .values:
Criteria | as_matrix() | .values() |
---|---|---|
Version Support | Supported in pandas versions till 0.23.0 | Supported in all versions; recommended way of equivalent operation as per latest version. |
Functionality | Converts pandas data frame / series to numpy matrix | Returns numpy representation of the DataFrame/Series. Where possible, the output is a view on df/values. |
Deprecation status | Deprecated since version 0.23.0 of pandas. | Not Deprecated currently |
By replacing as_matrix() with .values, you’ll ensure your code works seamlessly without raising any ‘AttributeError’, while keeping up with the current recommended practices from the pandas team.
I’d suggest checking out the official [Pandas Documentation](https://pandas.pydata.org/pandas-docs/stable/reference/api/pandas.DataFrame.html) for detailed, comprehensive lists of attributes and methods applicable to DataFrame objects.
Although this recommended change from as_matrix() to .values may seem minimal, it’s an essential step as stringently defined structures and practices confirm quality software development. The transformation reiterates the relevance of an up-to-date coding approach, yielding easier debugging and lower cost maintenance in the long run. But also, to assure your Python codes are more readable, maintainable, and adhering to the latest trends and best practices in the Data Science community.
Sure, I’d be delighted to provide an in-depth take on the attributes and methods of Series objects in Pandas, more specifically why the AttributeError: ‘Series’ object has no attribute ‘as_matrix’, may occur.
To start with, let’s dig into what a Series object is. In the Pandas library, a Series is a one-dimensional ndarray with axis labels. This data structure is insanely handy when dealing with vectorized operations and handling null values.
Here’s a quick overview of some common attributes and methods:
* The `
xxxxxxxxxx
.shape
` attribute gives us the dimensionality of the DataFrame.
* The `
xxxxxxxxxx
.size
` attribute shows us the number of elements.
* The `
xxxxxxxxxx
.values
` method will return a Numpy representation of the Series.
* The `
xxxxxxxxxx
.index
` method allows us to access or set the index labels.
* The `
xxxxxxxxxx
.head()
` and `
xxxxxxxxxx
.tail()
` methods fetch the first 5 and last 5 items in the Series respectively.
Diving into the main topic, you might encounter the following error message:
xxxxxxxxxx
AttributeError: 'Series' object has no attribute 'as_matrix'
Why is this happening? It’s because the `
xxxxxxxxxx
as_matrix()
` function was removed from Pandas, as per its documentations.
The reason behind its removal stems from making the code clearer and more efficient. Previously, the `as_matrix()` function was used to return the values of the DataFrame or Series object as a NumPy array. However, it could lead to confusion as there were instances when it wouldn’t return the dtype as expected.
Now, instead of using `
xxxxxxxxxx
as_matrix()
`, Pandas recommends using two alternative methods depending upon your use-case:
1. For getting a NumPy array, use the `
xxxxxxxxxx
.values
` property:
xxxxxxxxxx
numpy_array = series_obj.values
2. Alternatively, if you want to ensure that the returned value is definitely a NumPy array and also wish to specify a dtype, use the `
xxxxxxxxxx
.to_numpy()
` method:
xxxxxxxxxx
numpy_array = series_obj.to_numpy(dtype='float32')
As you can see, not only are these methods more intuitive, but they make the underlying process self-explanatory for the user. This enhances readability and aids subsequent maintainers of the code.
For example, suppose you have a pandas Series named “my_series”:
xxxxxxxxxx
import pandas as pd
# create a Series
my_series = pd.Series([2, 4, 6, 8])
In older versions of pandas, you would convert the Series object to a NumPy array using ‘as_matrix’:
xxxxxxxxxx
# Older versions (not recommended)
numpy_arr = my_series.as_matrix()
However, if attempted now, the aforementioned AttributeError occurs.
Let’s update the code snippet above to correctly handle conversion to a numpy array using the ‘.values’ property or ‘.to_numpy()’ function:
xxxxxxxxxx
# Current version using .values
numpy_arr_values = my_series.values
# Current version using .to_numpy()
numpy_arr_to_numpy = my_series.to_numpy()
By keeping up with the updates in different libraries and understanding the reasoning behind them, we can write clearer and more effective code. This continuous learning surely keeps our life as a programmer interesting!Certainly! This particular error, “AttributeError: ‘Series’ object has no attribute ‘as_matrix’, usually occurs when programmers try to utilize the “as_matrix” function that the Pandas library in Python used to offer. It’s essential to understand how this function worked, why it was depreciated and what alternatives we have today.
Understandably, if you’re accustomed to using the “as_matrix” function, it can be unnerving to suddenly stumble upon an AttributeError. But fear not, because I’ll lead us on a productive journey of explanation and alternative solutions.
Pandas .as_matrix function
The
xxxxxxxxxx
"as_matrix"
was a method in the Pandas library that allowed users to convert their data into numpy.ndarray format:
xxxxxxxxxx
import pandas as pd
s = pd.Series([1, 2, 3])
data = s.as_matrix()
print(data)
However, owing to its ambiguous nature—since it could convert input to either ndarray or matrix formats—it was deprecated in version 0.23.0 and eventually removed after version 1.0.0. Thus, trying to use it now will result in an “AttributeError.”
Solutions
Here are two effective solutions for this issue:
1. Utilizing .values attribute:
The
xxxxxxxxxx
.values
attribute returns the Series or DataFrame as a numpy.ndarray:
xxxxxxxxxx
import pandas as pd
s = pd.Series([1, 2, 3])
data = s.values
print(data)
2. Using .to_numpy() function:
Internally,
xxxxxxxxxx
.values
uses
xxxxxxxxxx
.to_numpy()
, which can provide more control over the output format (for certain dtypes). See the example below:
xxxxxxxxxx
import pandas as pd
s = pd.Series([1, 2, 3])
data = s.to_numpy()
print(data)
So, while the abrupt disappearance of as_matrix might seem like stumbling upon an unknown territory, rest assured that with .values and .to_numpy(), we’ve got swift, suitable substitutes to rely on. Never fret when you see AttributeErrors! They often unravel new ways of doing things better.
Need more info about pandas lib? Here is the official documentation. Need understanding on numpy? Try visting the numpy documentation.
Ready to explore deeper? Here is a resourceful guide on how to convert Pandas DataFrame to Numpy array.The error that surfaces as
xxxxxxxxxx
AttributeError: 'Series' object has no attribute 'as_matrix'
is a classic headache for many programmers utilizing the pandas python library. Now, one might wonder about the reason behind its occurrence. This error primarily sprouts from attempting to use the deprecated method
xxxxxxxxxx
as_matrix()
on a pandas series object. The Pandas Series object has removed the
xxxxxxxxxx
as_matrix()
function as it no longer aligns with the streamlined usage mandates of the library.
The primary purpose of the
xxxxxxxxxx
as_matrix()
function was to return the pandas series or data frame contents as they are with no modifications. But with evolving programming requirements and improving library versions, the shift towards creating easily maintainable code steered towards discarding this method in favor of other enhanced methods.
Here’s a glimpse into the method that led to the error:
xxxxxxxxxx
import pandas as pd
s = pd.Series([1, 2, 3])
mat = s.as_matrix()
print(mat)
Should you try executing the above code block now, it’d throw up an AttributeError, displaying that the ‘Series’ object has no attribute ‘as_matrix.’
Instead of using the
xxxxxxxxxx
as_matrix()
method which is no longer part of pandas directives, you can switch over to using alternatives such as the
xxxxxxxxxx
values
attribute or the
xxxxxxxxxx
to_numpy()
function. Here’s how it is done,
xxxxxxxxxx
import pandas as pd
s = pd.Series([1, 2, 3])
mat = s.values # alternative 1
print(mat)
mat_toNumpy = s.to_numpy() # alternative 2
print(mat_toNumpy)
Both these alternatives yield similar results while bypassing the AttributeError issue arising due to usage of the outdated method ‘as_matrix’. Striving to use more current and supported features not only contributes towards writing cleaner, more efficient code but also circumnavigates problem territories like such AttributeErrors.