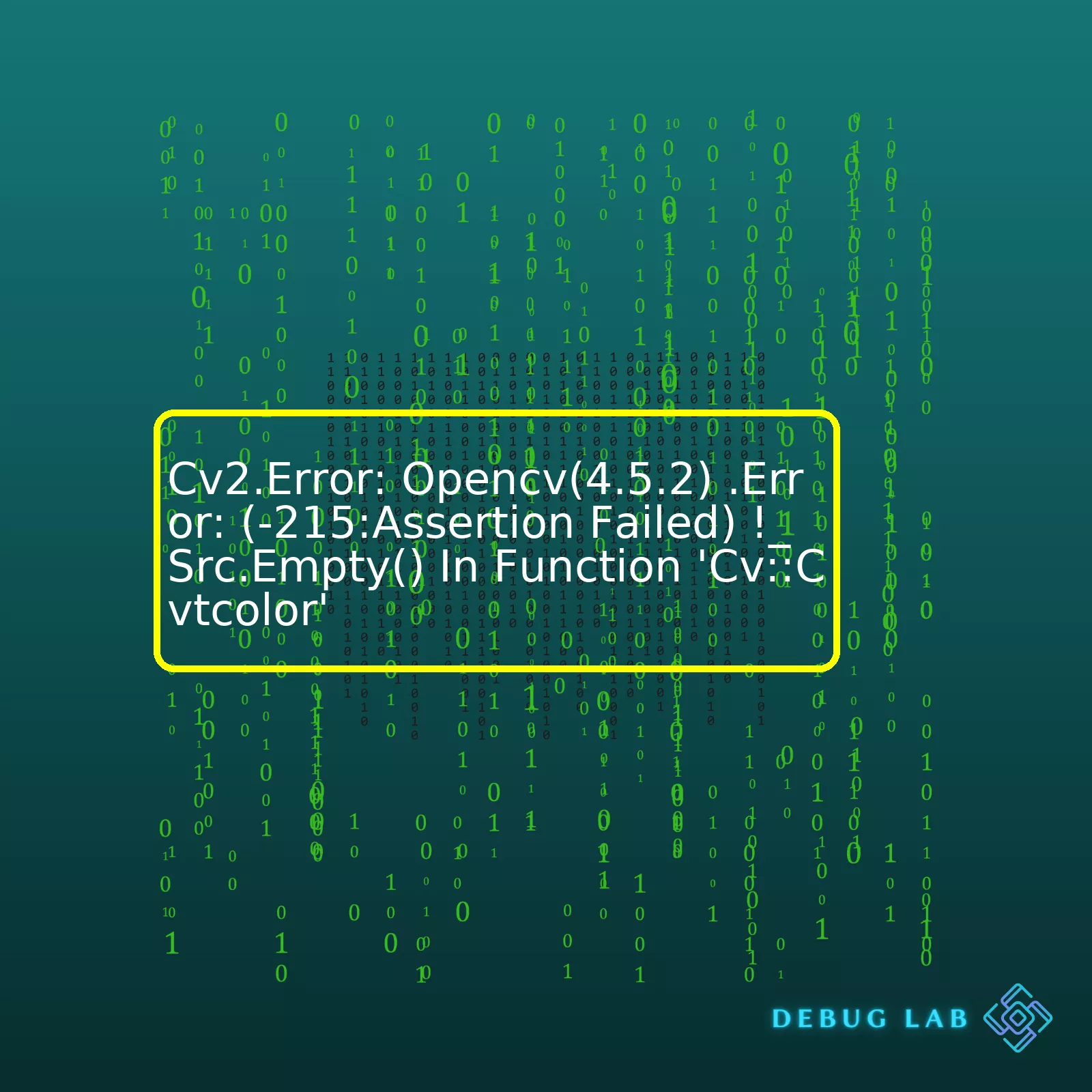
Cv2.Error: Opencv(4.5.2) .Error: (-215:Assertion Failed) !_Src.Empty() In Function 'Cv::Cvtcolor'
in Python typically occurs when the source image that’s being utilized is either missing or doesn’t get loaded correctly. In such a scenario, OpenCV isn’t able to find any data to process—triggering an assertion error to highlight the issue.
Let’s breakdown this verbose error message:
1.
Cv2.Error
: It denotes the library (in this case, OpenCVv2), indicating where the error originated from.
2.
Opencv(4.5.2)
: It reveals the version of the OpenCV library you’re using.
3.
.Error
: It’s the error type that was thrown by the function.
4.
(-215:Assertion failed)
: It stands for the specific error description demonstrating that an internal condition, which was expected to be true actually turned out to be false triggering the assertion.
5.
!_src.empty()
: This part checks if the source contains some data—in other words, it checks whether the source image is empty or not.
6.
In function 'cv::cvtColor'
: It shows you the method or operation at which this error occurred–in this case, during color conversion (
cvtColor
).
This summarized information can now be represented as a table in HTML format. Here is an example of an HTML table representing this breakdown:
Term | Description |
---|---|
Cv2.Error |
Represents the library (OpenCVv2)—the origin of the error. |
Opencv(4.5.2) |
Version of the OpenCV library used. |
.Error |
Type of error occurred. |
(-215:Assertion failed) |
Error description revealing an unfortunate (false negatives) event—an internal condition thought to be right proved wrong, thereby setting off the alarm. |
!_src.empty() |
A boolean checker scrutinizing whether the source data is empty or available. |
In function 'cv::cvtColor' |
Highlights the method where the error occurred—a color conversion. |
The occurrence of this error can be largely attributed to incorrect file path, wrong image file name or the image data simply failing to load. To troubleshoot this, ensure your file directories are precise, the filename and its extension are spelled accurately, and the image is successfully loading before running processing methods such as
cvtColor
.
It’s crucial to keep these points in mind while working with OpenCV, because errors related to
cv::CvtColor
or other similar functions can interrupt your workflow greatly. But once you understand the structure of these error messages, and know how to rectify them, you’d significantly reduce debugging time.Sure! The error message in OpenCV version 4.5.2, `Cv2.Error: (-215: Assertion Failed) !_Src.Empty() In Function ‘Cv::Cvtcolor’` typically occurs when the source image (denoted by `_Src`) is not successfully loaded or read into memory. Simply put, you’re trying to perform operations on an image that doesn’t exist or couldn’t be found.
To debug this issue, let’s analyze it step-by-step:
Here Is Essentially What The Error Message Says:
Cv2.Error: - Tells us there was an error in OpenCV. Assertion Failed - Such indicates a condition expected to be true has returned as false. !_Src.Empty() - This is the condition that failed. Here, we're checking whether the source object (_Src), which should hold our image, is actually not empty. If it's empty (which is an issue), the return would be false triggering the assertion. In function 'cv::cvtColor' - Specifies exactly where in the code/executed functions the error occurred.
When Does This Happen?
The above error is thrown within an operation using
cv2.cvtColor()
function which converts an input image from one color space to another. It arises when the following conditions meet:
– The input path of the image is incorrect.
– The input file is not an image or is a corrupted image file.
– There are issues with read permissions of the file.
Diving Deeper:
The
cv2.cvtColor()
function accepts two arguments: the image and the color space conversion code. For instance:
img = cv2.imread('image_path') gray_img = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
If the image located at `’image_path’` does not exist or cannot be read for any reason, the `cv2.cvtColor()` function will try to transform a null object, throwing the, `(-215: Assertion Failed) !_src.empty() in function ‘cv::cvtColor’`, error.
How To Resolve Cv2.Error:
Use Debugging Checks:
Before your `cv2.cvtColor()` function, add a conditional statement to check if the image has been loaded correctly:
img = cv2.imread('image_path') if img is None: print('Failed loading image') else: gray_img = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
Ensure The Correct Image Path:
Make sure the string representing your image file path is correct. Absolute paths are better in avoiding path-related errors compared to relative paths.
Check File Permissions:
Lastly, verify your Python script has the appropriate permissions to access and open your image file.
Pro Tip:
Remember ‘cv2.imread()’ returns ‘None’ when it can’t read an image. Whenever the `-215:Assertion Failed` error surfaces, first confirm `imread()` is reading the image properly.
In debugging, understanding each error message and its origin simplifies the process. The Cv2.Error could be daunting at first but once dissected, points us precisely to what went wrong. So there you have it, a comprehensive resolution for the `(-215:Assertion Failed)` error in the OpenCV library. Now go ahead and fix those bugs like a champ!
The OpenCV “assertion failed” error in the `cv::cvtColor` function describes an issue where the source (src) file for CV2 is empty. This error typically happens when the image you attempt to load does not exist at the location specified or is improperly loaded from its source. Let’s dive deeper into why it occurs and how you can fix it.
When working with OpenCV, you might encounter an error message that reads like: Cv2.Error: Opencv(4.5.2) .Error: (-215:Assertion Failed) !_Src.Empty() In Function ‘Cv::Cvtcolor’. Understanding what an Assertion error is, aids us in debugging the problem.
Understanding the Error
The Exception `-215` generally refers to an assertion failure due to the src.empty(). Essentially, it flags when an image file input is missing or not found. The “CvtColor” function, on the other hand, stands for convert color space – which is a typical operation for changing the color space of an image file like BGR<->Gray, BGR<->HSV etc. If your source image isn’t properly loaded or doesn’t exist at the specified location, no conversion possible, hence causing this assertion fail error.
Pressure Points That Can Cause This Error:
• Image file not located in the directory as specified by its path.
• The filename or extension of the image is typed incorrectly.
• The image data has not been correctly loaded into the variable assigned.
Solutions to these situations include ensuring your pathname and filename is correct, your image file is in the right directory, and your code effectively loads the image data.
How to Fix this Error
You may want to analyze the following aspects:
• Verify the Image Path: The error could stem from a typing error within your image file’s pathname. Ascertain the image file the OpenCV function should work with is indeed situated in your defined path.
import os print(os.path.exists('/path_to_your_file/your_file.png')) # Replace with your actual path & filename #Should return True if file exists
• Check the Filename and Extension: You may have spelled your filename or extension incorrectly. It’s worth cross-checking the name and typology for accuracy.
img = cv2.imread('file.jpg',0) # Ensure 'file.jpg' matches your filename exactly, including the extension
• Analyze Loading of Image Data: If you’ve verified your image’s pathname and filename, then it’s crucial to check whether your image data is properly loaded into the variable assignment. To troubleshoot, print the variable holding the image immediately after trying to load in the image.
img = cv2.imread('file.jpg',0) print(img)
If the terminal returns “None”, it indicates that your image hasn’t been loaded properly. However, if an array of numbers prints, you will know that the image data has been correctly read into the variable.
In conclusion, remember that the “-215:Assertion Failed” error is likely related to your source image either being missing or improperly loaded. Ensuring your image file’s proper placement and accurately loading the data are vital steps in resolving this issue. Maintain care to prevent typos while scripting image pathnames; a trivial typo might be the root cause of the exception.
Follow the methods mentioned above to debug the error patiently and logically. Continue probing on stackoverflow, stackoverflow.com, or similar platforms for very specific issues concerning the matter. Ensure to build a stronghold over OpenCV basics, and you’ll resolve the matter in no time!
For further reference about the OpenCV Error: (-215:Assertion failed) !\_src.empty() in function ‘cv::cvtColor’, check out the official OpenCV Documentation.
Note: These tips methodically address the potential causes of the Assertion error. However, errors can be project-specific and may require unique solutions.If you’ve navigated through the coding labyrinth of OpenCV and arrived at the troublesome function error message `CV::cvtColor`, fret not! Often, this warning beacon goes off due to assertions failing within this specific function. It can be vexing, opaque, and deciphering it may feel like modern hieroglyphs. But, as Sherlock Holmes once said, ‘the world is full of obvious things which nobody by any chance ever observes’, so let’s tackle this together.
FIRST THINGS FIRST
First, let’s get a basic understanding of the error message.
cv2.error: OpenCV(4.5.2) .error: (-215:Assertion failed) !_src.empty() in function 'cv::cvtColor'
This error indicates that the program couldn’t find the image you’re trying to use in cvtColor(). By exclaiming an _src.empty() issue, it’s telling us that our source (the image we’re using) appears to be empty. Before resorting to complex troubleshooting, let’s guide ourselves with the following possibilities.
IMAGE PATH ISSUES
The most common cause for such kind of error is an incorrect or incomplete image file path. Whatever image you’re loading, make sure:
- The image is present in the correct directory.
- The spelling of the image file exactly matches with what you have specified in imread() function.
For instance:
img = cv2.imread('image.jpeg')
In this case, ‘image.jpeg’ should exist in the same directory where your script is running. If it’s in another location, the complete directory path needs to be mentioned. Case sensitivity could also trigger this error, so be wary of upper and lower case mix-ups!
ERROR IN THE IMG PROPERTY
Yes, an incorrect or inadequate property assigned to the img can kick off this error. Confirm whether:
- You’re making use of the proper syntax.
- All required properties and methods are correctly assigned.
Imagine the scenario:
img = cv2.imread(img) gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
This may return an error because ‘img’ only serves as a placeholder. Make sure you define all needed properties and methods to read, capture, and transform frames or images.
TROUBLE WITH THE CVTCOLOR FUNCTION
Sometimes the problem isn’t the image per se, but how the cvtColor function is implemented. Review if:
- Your color space conversion code is spot on.
- The src (source) parameter isn’t empty.
Here is an example snippet:
img = cv2.imread('image.jpeg') grey_img = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
In the above snippet, ensure that the image ‘image.jpeg’ loads successfully before being passed to the cvtColor function. The grey_img line converts the image into gray scale using cvtColor() function.
Remember, the issue is generally around the region from where the image data is acquired, and the inputs to the cvtColor function. By focusing your inquiry there, you would have crossed halfway past the Rubicon.
Stay hungry, keep coding! For further references, do check out the official OpenCV documentation.
In OpenCV, the error message “cv2.error: OpenCV(-215:Assertion failed) !_src.empty() in function ‘cv::cvtcolor'” is often encountered by developers when the source of the image or the video inputted into the cvtColor() function is empty. The cvtColor() function in OpenCV is used for converting images from one color space to another. When it comes with an argument that is not valid or does not contain any image data, it raises this assertion error.
Primary reasons producing these types of errors:
- File Path Issue:
OpenCV might be unable to locate the image file if either the path provided is incorrect or the image does not exist at the specified path. This leads to the creation of an empty matrix, which when passed as an argument to cvtColor() causes it to raise an AssertionError.Exception code:import cv2 image = cv2.imread('incorrect/path/to/image.jpg') new_image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
If your system can’t find ‘image.jpg’ at the mentioned path, it will return None and result in a ‘_src.empty()’ warning.
- File Opening Issues:
The issue can also occur while dealing with video files or webcam feeds because the cv2.VideoCapture() method you’re using may not have access to your camera or it’s being used by any other process simultaneously.In such cases, the video feed might not be accessible leading to an empty frame.An example:
import cv2 video = cv2.VideoCapture('wrong/path/to/video.mp4') ret, frame = cap.read() gray_frame = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
Here, if ‘video.mp4’ doesn’t exist at the given path, cv2.VideoCapture() would fail to open the video and will return False. Hence, ret will be False and frame will be None, triggering a _src.empty() warning.
Resolution Method:
You should confirm if your specified path is accurate and that the relevant permissions for accessing the files or resources are granted. You could add a check to verify if the image or video is loaded correctly before further processing. Example:
import cv2 # Check if the video is opened correctly if not video.isOpened(): print(f"Error opening video file") else: # Read until video is completed while(video.isOpened()): # Capture frame-by-frame ret, frame = video.read() if ret == True: # Display the resulting frame cv2.imshow('Frame', frame) # Break the loop else: break
Here, isOpened() method is used to confirm whether the VideoCapture was successfully initiated or not.
From the above analysis, we see how accuracy in the filepath, correct loading of assets and granting of appropriate permissions helps in preventing ‘_src.empty()’ errors in OpenCV. Thus, it’s crucial to understand the underlying reasons of any specific command exceptions/erros in order to effectively debug and resolve them. Understanding software and tools at a deep level empowers anyone to use them more effectively and efficiently.The error message
Cv2.Error: Opencv(4.5.2) .Error: (-215:Assertion Failed) !_Src.Empty() In Function 'Cv::Cvtcolor'
usually means that the image you are trying to perform the Cv2 function on has not been loaded or initialized correctly, thus yielding an empty source (i.e., `_Src.Empty()`). The `Assert` command in OpenCV primarily works by halting the execution of the program if it encounters an error. It does this to prevent additional errors from occurring during the course of the function’s execution.
Let’s dive deeper into understanding each part of the error:
–
-215:Assertion Failed
: This is the assert statement that has failed. An assertion is a sanity-check that verifies whether the conditions expected are true. If the condition turns out to be false, the program will stop and generate an error.
–
!_Src.empty()
: The `Empty()` function checks whether the matrix/source (in this case, your image) is empty or not. The “!” is a logical NOT operator. Thus, when it says `!_Src.empty()`, it implies that it expects the source to NOT be empty.
–
In Function 'Cv::Cvtcolor'
: This indicates where exactly the error occurred. In this scenario, `cv::cvtColor` was the function being executed when the assertion failure occurred. This function converts an input image from one color space to another.
Here is how the typical structure of the code might have looked like before the error:
import cv2 img = cv2.imread('path_to_your_image') gray_img = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
The call to `imread` is supposed to read the image file from your specified ‘path_to_your_image’ and store it as a matrix in `img`. Later, `cvtColor` tries to convert that image into a grayscale image. Therefore, if `imread` fails to load the image, `img` doesn’t hold any data. Consequently, converting `img` to grayscale becomes impossible and thus the assertion fails resulting in the given error.
Tactics for troubleshooting such errors can include:
- Incorrect Path: One common mistake is specifying an incorrect path to the image file. Ensure you’ve entered the accurate path, and the image file exists at that location. You can print the image that was read to check if it was loaded successfully.
- Inaccessible File: Another problem could be poor permissions, or for some other reason the program isn’t able to access your image file. Try manually checking the image file’s location and if possible, moving the image into the same directory as your Python file. You can then provide just the image filename to `imread` instead of a full path.
- Corrupt Image File: The issue could stem from a corrupt image file or unsupported image format. Try loading a different image file and see if you still encounter the error.
- Absolute Vs Relative Paths: Finally, be aware of whether you’re using relative paths vs absolute paths. A relative path is in relation to the current working directory, whereas an absolute path gives the full location. It would be helpful to double-check which you’re using.
print(img)
If the output is `None`, then your image file wasn’t loaded properly.
By thinking through these possibilities, you should be more prepared to tackle the
Cv2.Error: Opencv(4.5.2) .Error: (-215:Assertion Failed) !_Src.Empty() In Function 'Cv::Cvtcolor'
assertion error!
For further exploration into handling images with OpenCV, consider visiting the following [online resource](https://docs.opencv.org/3.4/d3/df2/tutorial_py_basic_ops.html) which provides a comprehensive tutorial on basic operations involving images using OpenCV library.OpenCV 4.5.2 cv2.error: (-215: Assertion failed) !_src.empty() in function ‘cv::cvtColor’
This error is typically linked to OpenCV not being able to locate or open the file you specified, which results in an empty matrix being sent in for processing. The options below can help you fix such errors.
Solution 1: Check Your File Path
It’s critical to double-check your image/video input files paths. Absolute paths are more reliable and less prone to errors than relative paths. Here is a quick example:
import cv2 # Provide absolute path img = cv2.imread("/home/user/images/pic.jpg") cv2.imshow('Image', img) cv2.waitKey(0)
In case your file path contains blank spaces or special characters, encapsulate it in raw string Python format by using the r keyword, as shown:
img = cv2.imread(r"/home/user/images/my pic.jpg")
To validate if the file was loaded correctly by imread, consider checking if the output is None:
if img is None: print("Check your image path. The file could not be loaded.") else: cv2.imshow('Image', img) cv2.waitKey(0)
In this way, you will get informative feedback if anything goes wrong with loading the image.
Solution 2: Update Your Library Versions
Ensure that you have the most recent versions of relevant libraries. This includes numpy and OpenCV itself. Use the command
pip install --upgrade opencv-python-headless
to update OpenCV and similarly for numpy use
pip install --upgrade numpy
.
Solution 3: Check your webcam
If you’re capturing frames from a webcam, check if its index is set correctly. A value of 0 (or -1) usually points to the default camera. If others are connected, indices starting from 1 onwards represent those:
cap = cv2.VideoCapture(0) # default camera
Also, verify whether your webcam is available and isn’t busy with another application. Adding a check after initializing the capture object can help diagnose the problem.
if not cap.isOpened(): print("Could not open webcam")
Furthermore, make sure to release the VideoCapture instance properly once done. Not doing so may keep the webcam occupied and cause trouble opening in the future.
cap.release()
These solutions should resolve most occurrences of the cv2 error related to ‘cv::cvtColor’. Always bear in mind to validate your inputs before processing them further. Additionally, staying updated with the latest library versions helps evade compatibility issues and leverage improved functionalities.
For more specific help, check the official OpenCV documentation here.Certainly, let’s delve deep into the technical aspects of the cv::cvtColor function and understand its relevance to the error `cv2.error: OpenCV(4.5.2) .error: (-215:Assertion Failed)! _src.empty() in function ‘cv::cvtColor’`.
The cvtColor function hails from the C++ library of OpenCV (Open Source Computer Vision Library). It is a critically valuable function for image processing that primarily converts images from one color space to another.
Code example:
cv::Mat inputImage = cv::imread("input.jpg"); cv::Mat outputImage; cv::cvtColor(inputImage, outputImage, cv::COLOR_BGR2GRAY);
In the above snippet, we load an image using `cv::imread` function, and `cv::cvtColor` is employed to convert the color space of this image from BGR to grayscale.
Now, let’s evaluate the error message which reads: `(-215: Assertion Failed)! _src.empty() in function ‘cv::cvtColor’`:
• This alert pops up when the cv::cvtColor function doesn’t find an input image or the input image isn’t loaded correctly.
• An exclamation mark before `_scr.empty()` indicates it is checking if the source image is not empty.
• The error `-215` refers to an assertion fail, which means a condition expected by the program was found to be false at runtime.
What triggers this issue? A number of reasons could trigger it, predominantly it can be due to the incorrect path to the image file, or the image just doesn’t exist in the provided location.
Consider the following example:
cv::Mat img = cv::imread("NonExistent.jpg"); cv::Mat outputImg; cv::cvtColor(img, outputImg, cv::COLOR_BGR2GRAY);
Here, the problem erupts because we attempt to read an image “NonExistent.jpg” which isn’t there in the first place. Hence, when attempting to apply cv::cvtColor to an empty matrix `img`, the program throws an error.
Ways to handle it:
• Ensure that the image exists in the correct path
• Implement condition checks to see if the image has been loaded correctly
Example:
cv::Mat img = cv::imread("input.jpg"); if(img.empty()) { std::cout << "Could not open or find the image!\n" << std::endl; } else { cv::Mat outputImg; cv::cvtColor(img, outputImg, cv::COLOR_BGR2GRAY); }
Here, before moving ahead to work with the image, a check is implemented to see whether the image has loaded without any glitches. If something goes wrong while loading (like an incorrect filename), then the program will output a message to indicate the issue instead of directly slipping into the exception.
Understanding the basics of cv::cvtColor and the issues associated with OpenCV's implementation provides a solid base to leverage this function effectively in your computer vision pipeline.
Additional details on the utility and application of the cv::cvtColor function can be visited at the official OpenCV documentation page.The cv2.error: opencv(4.5.2) .Error: (-215:Assertion Failed) !_src.empty() in function 'cv::cvtcolor' is a classic error message you will come across when working with OpenCV library in Python. A detailed understanding of the error, its root causes and efficient ways to solve it, are crucial to proficient coding.
This error can be quite daunting but if you know where to look, it's got simple solutions.
Typically, it arises when there's an assertion failure, which occurs when a precondition - that input must not be empty - isn't met before calling the function
cv::cvtColor()
. Essentially, OpenCV is telling you that it was expecting to receive something but found nothing, hence, it crashed.
Imagine trying to bake a cake and realizing halfway through that you have no flour! This is how OpenCV feels when it encounters this error.
Now, let's delve deeper into identifying common situations that lead to this error:
* The image file you're trying to open doesn't exist or the path specified is inaccurate.
* The image file may exist but it's corrupt or unsupported by OpenCV.
* The image was loaded improperly, resulting in an empty matrix.
To solve this, ensure that:
* Image path specified is correct.
img = cv2.imread('correct/path/to/image.jpg')
* Image file is not corrupted and in a format supported by OpenCV.
* Check that the image loads properly and isn't empty. You can confirm this by printing the array right after loading:
print(img)
If these suggestions don't mitigate the issue, go through your code again meticulously checking for typographical errors, improper file paths, unsupported codecs, or inappropriate use of functions.
Getting more familiar with your libraries and tools will definitely aid in quickly identifying and fixing such hitches. And finally, always remember to consult the official OpenCV documentation or online communities like StackOverflow when you're stuck; chances are someone else has had the same problem and it's been solved!
Always apply best practices when handling digital media and data in order to avoid running into such errors. Happy coding!