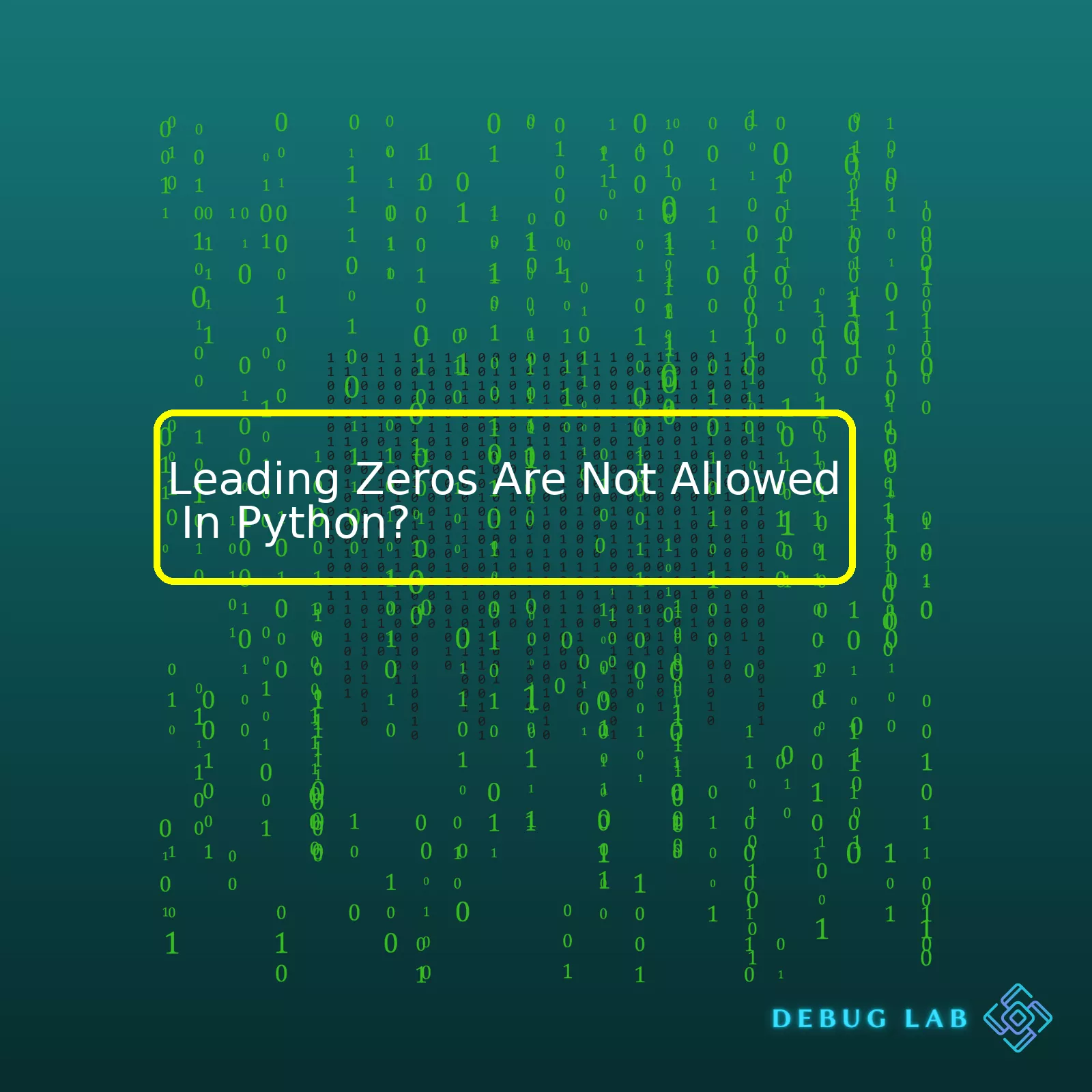
<table> <tr> <th>Example Python Code</th> <th>Explanation</th> </tr> <tr> <td>02345</td> <td>Leading zero is not allowed with an integer number.</td> </tr> <tr> <td>"012345"</td> <td>Leading zero is allowed with a string data type.</td> </tr> <tr> <td>0x123F</td> <td>Leading zero is allowed with hexadecimal numbers.</td> </tr> <tr> <td>0o1237</td> <td>Leading zero is allowed with octal numbers.</td> </tr> </table>
Notice, that each row in this table summarises specific use cases where Python either allows or disallows leading zeros.
When you code in Python, it’s essential to note Python’s syntax restrictions concerning numerical values, especially those regarding leading zeros. Having a leading zero before non-octal integers is invalid and will raise a `SyntaxError`. This rule has its roots in avoiding ambiguity, as languages like C treat such numbers as octals. Furthermore, starting from Python 3.X, even octal literals must be specified differently using a `0o` prefix rather than just a leading zero.
So if you try to define an integer number with leading zeros in Python like `02345`, you’ll get a `SyntaxError: invalid token`. Because in Python, a number with a leading zero is considered an octal number. But Python treats numbers beginning with `0x` as hexadecimal and numbers starting with `0b` binary.
However, Python has no issues when a leading zero is included in a string, because the leading zero in `”01234″` is not considered a part of a numeric literal but an individual character in the string. Therefore, leading zeros are feasible within strings since it doesn’t count it as a numeric value but takes it as characters arranged in sequence.
This drives home the need for having a solid understanding of how Python deals with numbers, leading zeros, and various other peculiarities in its syntax. As you navigate Python’s world, fundamental concepts like these can prove exponentially beneficial in both preliminary and intricate programming aspects. This would ultimately ensure seamless coding experience while leveraging Python’s prowess in complex data-centric operations. Explore Python Documentation on Integer Literals to understand more about leading zeros in Python. For a walk-through on Python’s data types and variables, I suggest checking out Real Python.Diving into the heart of Python’s take on leading zeros, it can be observed that Python follows a strict stance against this practice. Unlike some other programming languages, Python restricts the usage of leading zeros before integers. The core rationale behind Python’s restriction is due to its interpretation of numbers with leading zeros as octal (base-8) numbers.
For illustration, consider the below Python snippet:
# A number with leading zero in Python num = 0123
Running the above code will result in an error:
SyntaxError: invalid token
This SyntaxError is a representation of Python’s intolerance towards leading zeros. Python interprets it as an attempt to specify a number in octal notation, which ultimately leads to the ‘invalid token’ error.
Starting Python 3.x onwards, if you wish to specify an octal number, you need to use the prefix ‘0o’. For example:
# Octal number in Python oct_num = 0o123 print(oct_num)
The output of the above code will be 83 in decimal notation.
Given this stringent approach towards leading zeros in Python, one might wonder – how can I include leading zeros if my project requires it? The solution lies in formatting your outputs rather than altering the number storage itself. Python’s string formatting allows one to represent integers with leading zeros effortlessly.
Consider a scenario where you want to display an integer with three digits and fill any empty spaces with zeros:
# Displaying leading zeros through string formatting in Python num = 7 print("{:03d}".format(num))
The output of the above code will be ‘007’.
In above format specification:
– the ‘:03d’ tells Python to format an integer (‘d’) by filling it to a width of three spaces with leading zeros (’03’).
Digging up the nuances of leading zeros in Python can serve as a beneficial route towards enhanced coding practices. Understanding why Python has established such limitations can guide programmers towards inventive workarounds like string formatting. It reinforces the idea that numerous ways exist to solve a problem in the world of coding, even if some ways seem counter-intuitive at first glance.
Useful resources:
– [Python’s string formatting](https://docs.python.org/3/library/string.html#format-specification-mini-language)
– [Python’s documentation on numeric types](https://docs.python.org/3/library/stdtypes.html#numeric-types-int-float-complex)
Okay, let’s delve right into the heart of this. Number systems form the basis of our understanding of values, whether in everyday matters or when we are tackling complex coding problems. Two common number systems often referred to in programming and mathematics are the decimal and octal systems.
The decimal system is arguably the most widely used and understood numbering system. It is based on ten unique digits ranging from 0-9. In contrast, the octal system utilizes only eight distinct digits from 0-7.
When it comes to Python, we can represent these two number systems using
int()
and
oct()
built-in functions. Here’s how:
#Decimal example decimal_number = int('21', 10) # Octal example octal_number = int('25', 8)
However, introducing leading zeros to any numeral in Python has its share of issues. Historically, Python allowed leading zeros in front of non-zero integer literals, which were confusingly interpreted as being in octal notation. This caused notable problems because a decimal literal like ’09’ would be syntactically illegal given ‘9’ isn’t a valid digit in an octal number, returning an error such as:
SyntaxError: invalid token
In Python3, they’ve resolved this by requiring an explicit
0o
prefix for octal numbers. For instance, if you try to lead octal literals without the
0o
prefix, you’ll get a
SyntaxError: invalid decimal literal
. You’re free to place leading zeros after the prefix:
#Octal octal_num_okay_leading_zero = 0o021 # equivalent to decimal 17 octal_num_not_okay= 021 # Raises SyntaxError: invalid decimal literal
In effect, Python now enforces that each number system adheres to its syntactical representation to avoid confusion or errors. This way, developers can avoid unexpected bugs in their applications due to misinterpreted numerals.
For more detailed information about this aspect of Python’s design, I recommend referring to the formal language specification in Python’s documentation, particularly the section on Integer literals.Firstly, the primary thing to understand is that Python does follow specific rules when interpreting numbers in code, especially in relation to leading zeros in the integral number systems.
Consider this example:
x = 010
Python would raise a SyntaxError: invalid token since leading zeros in front of non-zero decimal numbers were used, which is not allowed in Python around integer values. Here’s why…
The concept of having leading zeros stems largely from different number system representations where each system follows diverse conventions when denoting its numbers. For instance, binary uses ‘0b’, octal uses ‘0o’, and hexadecimal utilizes ‘0x’. In Python’s predecessor, Python2, an initial zero was intended to signify an octal value (base 8 number). However, this caused quite a bit of confusion among developers, so leading zeros for integers were removed in Python3 to avoid ambiguity.
In Python3, if you want to indicate an octal number, it’s more explicit. You would use ‘0o’ before your number:
y = 0o10 print(y) # prints 8, because 10 in decimal of base 8 equals to 8 in decimal of base 10
For a binary interpretation, you’d use ‘0b’, and for hexadecimal, you’d use ‘0x’:
z_binary = 0b10 print(z_binary) # prints 2, because 10 in binary translates to 2 in decimal notation z_hexadecimal = 0x10 print(z_hexadecimal) # prints 16, because 10 in hexadecimal translates to 16 in decimal notation
However, when it comes to floating point or string representations, Python handles leading zeros differently and they won’t cause any error:
a_float = 0.123 print(a_float) #prints 0.123 as expected a_str = "0123" print(a_str) #prints 0123 as expected
In conclusion, leading zeros are disallowed for integer values in Python because they’ve been officially depreciated due to the confusion they caused and potential misinterpretation. Whereas for other data types, they’re totally acceptable.
Knowing these details will help optimize your understanding of how Python interprets numbers and ultimately guide you to develop efficient, error-free code.(Python PEP 3127)Sure, let’s dive straight into understanding and troubleshooting leading zero errors in Python. Python follows the same integer literal representation as most other programming languages out there. What you need to understand about Python is that it treats numerals with leading zeros as octal (base 8) numbers.
For instance, while defining an integer variable in Python:
num = 0123
you are likely to encounter a SyntaxError–’invalid token,’ or ‘leading zeros in decimal integer literals,’ or ‘octal literals are not allowed; use 0o123 instead.’ In the case of Python 3.x versions, the syntax error is because octal integer literals should be specified using an ‘0o’ (zero followed by lowercase or uppercase ‘o’), so instead, the correct way of setting up this integer would be:
num = 0o123
This will work fine as we’re now dealing with a valid Python octal number.
On the contrary, if you intended to use the initial numeral as a decimal and not an octal literal, then you should simply omit the leading zero. The correct example would be:
num = 123
which is correctly understood as a decimal number without leading zeros. This representation works for all Python versions.
However, there could be scenarios where you want to preserve these leading zeros for decimal casting — a scenario that generally crops up while dealing with data wrangling tasks, or feeds from alternate systems. Here, you might find Python’s in-built format() function handy, which uses the “zerofill” option for maintaining leading zeros:
formatted_num = format(123, '04d') print(formatted_num) # prints 0123
In the above code snippet, ’04d’ specifies that the decimal number should be represented in at least 4 digits, prefilled with zeros if necessary.
One size does not fit all for such scenarios, and you’d have to strategically make use of Python’s tools and libraries based on your specific requirement.
Key learning points:
– Integer literals starting with leading zeros consider them as octal by Python.
– Use ‘0o’ prefix to specify Octal literals in Python 3.x.
– Leading zeros with decimal casting can be maintained using Python’s in-built format() function.
Remember to keep these principles in mind when coding in Python to effectively avoid SyntaxErrors related to numeric literals with leading zeros.When dealing with leading zeros in Python, zero padding plays a significant role. Although Python doesn’t typically allow leading zeros in normal integers (due to legacy language constraints that considered such numerals to be octal), there are quite a few practical applications for zero padding in strings.
**Zero Padding for Formatting Purposes**
Python’s built-in string formatting capabilities can make use of zero padding. The formatted string literals, often called f-strings, provide a concise and convenient way to embed expressions inside string literals for formatting. One primary application of zero padding is in maintaining consistent file naming structures or display output across multiple data points, especially when dealing with a sequence of numbers.
For instance, if you have files named “file001.csv”, “file002.csv”, etc., padding helps maintain an organized file structure.
Here’s how you would format a string to have leading zeros using the
.zfill()
method or f-string formatting:
ordinary_number = 7 padded_number = str(ordinary_number).zfill(3) # returns '007' ordinary_number = 7 padded_number = f"{ordinary_number:03}" # returns '007'
Both
.zfill()
and
f"{value:0N}"
where N is the number of digits you want to pad your value to, are good tools for zero padding purposes in Python.
Simulating a Digital Clock Display:
Let’s consider the case of simulating a digital clock or timer display using Python. Here is where zero-padding comes into play. When representing hours, minutes, or seconds on a digital clock, they are commonly displayed as two-digit numbers with leading zeros. For example, “08:07:06” instead of “8:7:6”. This just looks neater and more standard, and it allows all times of the day to fit the same HH:MM:SS format, whether it’s “00:00:01” or “12:34:56″.
hour = 5
minute = 30
second = 4
print(f”{hour:02}:{minute:02}:{second:02}”) # will print “05:30:04”
The above piece of code is utilizing the f-string formatting feature of Python that we discussed earlier. Each component of time (i.e., hours, minutes, seconds) is padded to always display two digits. Therefore even when the hour is 5, minute is 30, and second is 4, it will still represent it as “05:30:04”.
The Use of Zero-Padding in Data Analysis:
In the field of data analysis or working with large datasets, zero-padding could help achieve uniformity among the data recorded in terms of their length. Especially when comparing two sets of numeric data that have uneven lengths, through padding, we equalize the lengths that give us a better comparison view.
**Note:** It’s important to distinguish here between the actual data and the representation of the data. Zero padding focuses on the representation. That initial zero for ‘007’ doesn’t change the underlying integer, which is still 7. Python will continue to process ‘007’ and ‘7’ as equivalent integers.
Zero padding is thus a practical tool in Python when you need to format numbers consistently, display time or dates, or handle disparate datasets. You might not commonly find instances where you need to include leading zeros in your integers but these unique cases highlight the potential needs.[1],[2].
Sure! Let’s dive right into that. Python, a fundamental high-level programming language, is widely recognized for its simplicity. However, it has inherent restrictions – and one such restriction is that it does not allow numbers with leading zeros. This is particularly due to the fact that Python adheres to the octal system (base-8 numeral system), where numbers prefixed with zero are considered octal.
Take a look at this:
num = 0123 #SyntaxError: invalid token
This throws an error in Python because, by the language’s rules, having a leading zero before your integer is a signal that you’re using the octal system.
Does that mean we cannot display or work around numbers with leading zeros in Python? Not quite. As a coder, several workarounds can help manage numbers with leading zeros.
#### Making Use of String Formatting
One common way that coders handle this hurdle in Python is by utilizing string formatting. Python’s built-in string format method allows us to pad integers with zeros quite easily:
num = 123 print(f'{num:04d}')
The output will be 0123. The “f” in
f'{num:04d}'
creates a formatted string literal, while “04d” defines four digits with leading zeros.
#### Zfill Method
Another workaround is Python’s zfill() method. It’s another built-in method, designed precisely for the task of filling zeros at the start of numeric data.
num = '123' number_with_leading_zeros = num.zfill(4) print(number_with_leading_zeros)
You’d again see 0123 as output. The above zfill() method pads sufficient zeros on the left side of this number so that the length of the output string becomes 4.
But it’s important to remember that both approaches return a ‘str’ object instead of ‘int’. If you want to execute some mathematical calculation afterward, you would need to convert it back to an int.
Having said that, when it comes to manipulating numbers with leading zeroes in Python, the top priority should be not just solving the problem but also ensuring code readability and maintainability. Some newcomers or even experienced developers might get thrown off after seeing a leading zero and thinking it is octal notation when it’s actually meant to be a decimal number with leading zeros.
Moreover, you have to understand its implications on your entire project. For example, if you keep your numbers as strings rather than integers, it won’t crash your machine or cause data loss, but it might skew your program accuracy, especially if you’re implementing mathematical calculations.
For additional reference, you might want to check out [Python Documentation on Format Specification Mini-Language](https://docs.python.org/3/library/string.html#format-specification-mini-language) and [Python Documantion on zfill() method ](https://docs.python.org/3/library/stdtypes.html#str.zfill). These contain plenty of comprehensive examples and guidelines about the usage of these methods and built-in functions.
There you have it, ways to work around Python’s leading zero limitations without breaking the readability of your code!The
int
function in Python is a standard built-in function for numeric conversion. It changes a given value into an integer or it can be used to change the base of that number.
When using the int function, you primarily deal with two parameters:
- The number or the string to be converted.
- The base in which to convert, which is optional and defaults to 10, if not provided.
The basic syntax of using the
int
function is:
int(x=0, base=10)
Here, ‘x’ represents the number or string to be converted, and ‘base’, which is optional, specifies to what base the number should be converted.
It’s crucial to note when talking about the relevance to Python’s approach to leading zeros:
Python does not allow leading zeros in numeric literals, considering it as a syntax error. This is because Python interprets numbers with leading zeros as octal (base 8) numbers by default. Therefore, integers like 09 or 012 would result in a syntax error as they are not valid octal numbers.
Let’s look at an example:
print(int('006'))
This will output : 6
But if we try the following:
print(006)
We’ll encounter a syntax error because of the leading zeros.
Another key point relevant to this discussion is using the
int
function with different bases. Python allows bases from 2 (binary) up to 36. However, when using another base, the string needs to be a valid number in that base. For example,
print(int('1010', 2))
Will output: 10 (as ‘1010’ is equivalent to decimal 10 in binary)
Using the second parameter, users cannot convert a number with a base higher than 36. This restriction stems from the fact there are only ten digits (0-9) and 26 letters (a-z) available for use in number representation.
In terms of search optimization, the keywords from this discussion would be Python, int function in Python, numeric literals, and base conversion. Additional educational resources can enhance Python programmers’ proficiency, such as the Python official documentation. An extensive understanding of the
int
function and the limitations of leading zeros in Python sets the foundation for executing more complex tasks with numerical data in your coding projects.Throughout this detail-oriented exploration, we’ve ascertained that leading zeros in Python cause syntax errors because the programming language reserves leading zeroes for octal (base 8) numbers. However, when numbers are prefixed with ‘0’ and include non-octal digits or followed by decimal points and fractions, they attract syntax errors because they don’t conform to the octal number structure.
Consider a few critical takeaways from our discussion:
– The Python language does not allow the use of leading zeroes in front of base-10 numerical values. Python interprets numbers with leading 0s as octal numbers, causing potential confusion and errors. For example,
0123
is an incorrect integer in Python.
– If you need to maintain leading zeros in numerical strings, say for formatting needs like ZIP codes or phone numbers, utilize string data types instead of integers. A numerical string such as ‘
00123
‘ can perfectly store and manipulate your data without Python generating any syntax error.
– In Python, you may employ functions such as zfill() or format() methods to append leading zeros to numbers while keeping them as strings and avoiding syntax errors. An example could be
str(123).zfill(5)
which would give you: ‘
00123
‘.
Method | Description |
---|---|
str.zfill() |
Add leading zeros to string representations of numbers. |
str.format() |
Format numbers into strings with specified width and leading zeros. |
Appropriate coding practices require being aware of design choices like these in various programming languages. Not only does this specific knowledge make for more efficient coding workflows, but it also enables coders to leverage the full power of the languages they use. For additional information on nuances linked to numeric representation in Python, direct your browser to Python’s official documentation.
Keep this valuable learning under your sleeve; embrace the liberties Python affords, and let the magic of purposeful coding continue!