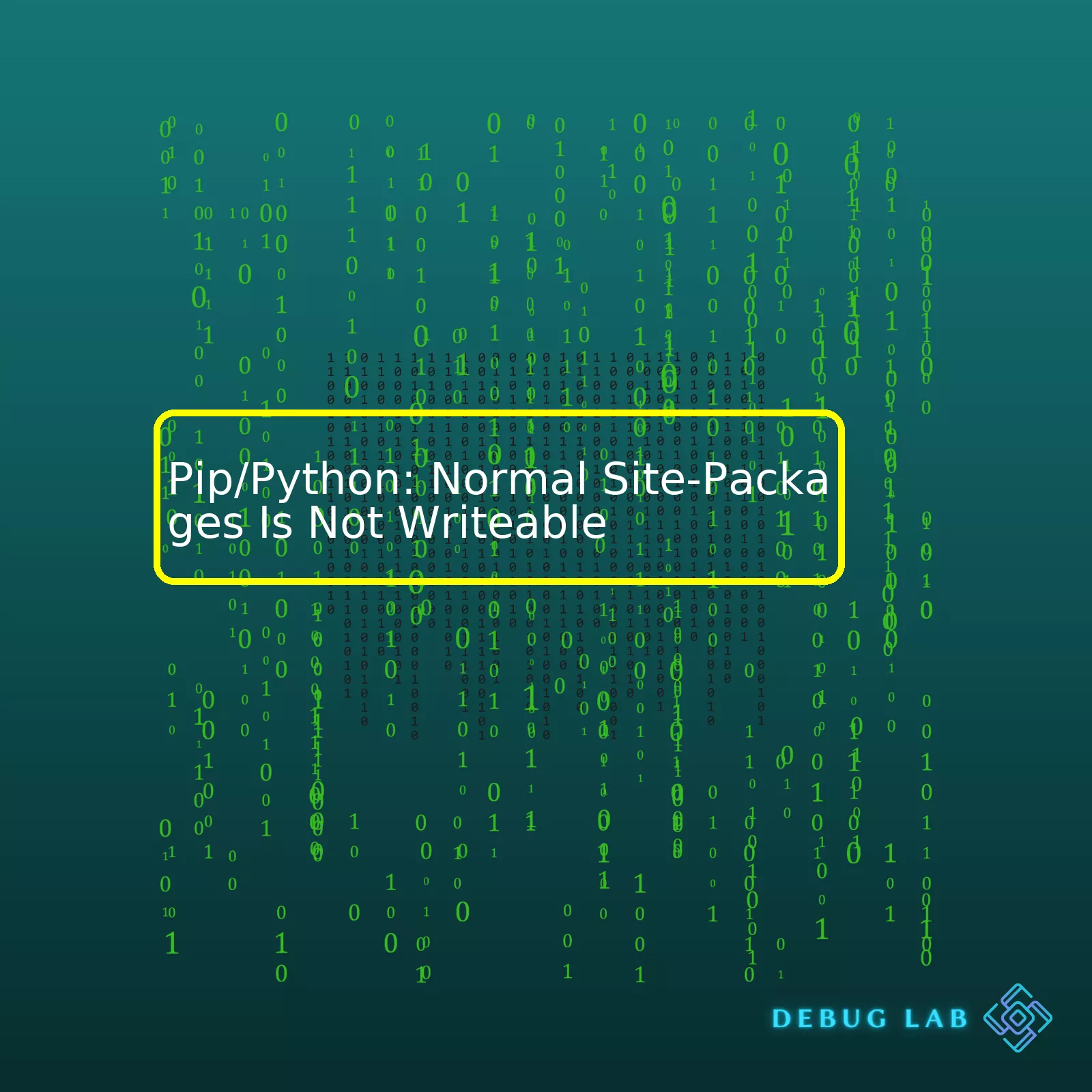
Creating a table summarizing this scenario, we could use the following HTML code:
<table>
<tr>
<th>Scenario</th>
<th>Problem</th>
<th>Root Cause</th>
<th>Solution</th>
</tr>
<tr>
<td>Pip/Python: Normal Site-Packages Is Not Writeable</td>
<td>Error while installing Python packages via pip</td>
<td>Insufficient permissions to write in site-packages directory</td>
<td>Install packages with correct privileges or use a virtual environment</td>
</tr>
</table>
In dealing with this issue, there are mainly two possible solutions. The first resolves around adjusting folder permissions to permit the necessary access. However, changing system-wide permissions can be risky and is generally discouraged. Therefore, it’s more advisable to opt for the second solution: leveraging Python’s dedicated package environment systems, like venv or virtualenv. These set up isolated environments where your Python projects can reside, avoiding the aforementioned permission issues.source. For instance, setting up a new virtual environment can be as straightforward as running, in command line,
xxxxxxxxxx
python3 -m venv /path/to/new/virtual/environment
source. Optimizing our workflow around such environments imparts an additional benefit of keeping our projects’ dependencies well-scoped and organized. With separate environments, you don’t need to worry about version conflicts between different projects or tampering with your system’s Python installation.Sure, I’d love to dig into the topic of Normal Site-Packages in Python and how this interfaces with pip, particularly when you encounter issues like “Normal Site-Packages is Not Writeable”.
So, let’s start from the basics. In a normal Python installation, there’s a directory called
xxxxxxxxxx
site-packages
, where pip installs any libraries and dependencies for your project. This includes things like NumPy, Django, Flask, etc.
However, sometimes while installing packages using pip, you might face a permission error such as:
xxxxxxxxxx
ERROR: Could not install packages due to an EnvironmentError: [Errno 13] Permission denied: '/usr/local/lib/python3.7/site-packages/six.py'
.
This means your current user does not have write access to the
xxxxxxxxxx
site-packages
directory.
Let me break it down further:
– The role of pip in this ecosystem is quite simple yet critical. Pip is a package manager for Python that helps you manage all these libraries and dependencies efficiently. It communicates with PyPi (Python Package Index) to download and install packages, which are added to the aforementioned
xxxxxxxxxx
site-packages
directory.
– Now, the Python
xxxxxxxxxx
site-packages
folder can be regarded as a depot for Python libraries. When you ‘pip install’ a package, it essentially places the downloaded package into this particular directory. And here’s the catch! If this directory is not writable by the current user, then pip simply cannot perform its task.
– Consequently, if your Normal Site-Packages folder is not writable, you get the kind of error I mentioned above. Reasons for this issue may revolve around operative systems like Linux and macOS, which prevent unauthorized writing on system directories. Yes, your Python
xxxxxxxxxx
site-packages
falls under a protected system folder!
But rest assured, we also have solutions. Here are a few workarounds I can suggest:
– Use a virtual environment. A virtual environment (venv) creates isolated environments for Python projects. This means each one will have its own
xxxxxxxxxx
site-packages
directory. In this way, you can bypass the permission issues arising within the global
xxxxxxxxxx
site-packages
. You simply create a new venv, activate it, and install your pip package there. Code snippet goes something like this:
xxxxxxxxxx
python3 -m venv ./venv
source venv/bin/activate
pip install your-package
– Use the
xxxxxxxxxx
--user
flag while installing packages. What this does is that it installs the package(s) specifically for the current user, thus evading the need to write in the global site-packages. It’s as simple as running
xxxxxxxxxx
pip install --user your-package
.
To check on permissions and find whether a directory is writable or not, you can use the below command on Unix-based systems:
xxxxxxxxxx
ls -ld /path/to/directory
Remember, understanding your Python environment and handling permissions proficiently are crucial for problem-free coding endeavors. The concept of Normal Site-Packages alongside insights into pip functioning equips us essentially in treading across complex Python development set-ups.
For more information about how to use pip, Python’s package installer, and detailed knowledge about the Python filesystem including
xxxxxxxxxx
site-packages
, refer to the official Python Docs or Python Packaging User Guide.
What is “Site-packages” in Pip/Python?
The term “site-packages” refers to a sub-directory of the Python standard library where third-party packages are installed. This directory location may slightly vary, based on your operating system and Python distribution. For example, for Windows users with Python 3.7 installed, it can typically be found at
xxxxxxxxxx
C:\Python37\Lib\site-packages
.1
Exclusive Rights to Site-packages: A Deep Dive
By design, any interactions within site-packages require write access permissions. Included but not limited to, are activities such as adding new Python libraries, uninstalling existing modules, or updating the current packages.
In the standard setup of pip and other Python package management systems, they rely on these write access permissions to function properly.
For instance, when you run:
xxxxxxxxxx
pip install requests
Pip will attempt to download the ‘requests’ package, compile it if necessary, and store it inside the site-packages folder. At each stage of this process, pip needs exclusive write access to the site-packages directory.
Python Site-packages Directory: Non-Writeable Issue
You might encounter an error message that states “normal site-packages is not writeable” when trying to install a package using Pip. The issue arises due to lacking rights needed writing to the directory structure housing the site-packages.
A number of factors can potentially trigger this error:
- Running Python in a virtual environment but having conflicting python binaries in the PATH.
- If the user doesn’t have administrative-level access to the machine running the script.
- The site-package directory permissions were changed manually.
- Changes were introduced into how directory security and ownership operates, due to a recent update of your operating system or Python environment.
To address these issues, the following solutions are applicable:
- Modify the PATH environment variable to resolve any path conflict. Use
xxxxxxxxxx
111which python
on Unix/Linux/macOS or
xxxxxxxxxx
111where python
on Windows to see the paths the OS consults for executable files.
- Run your scripts with elevated privileges (e.g., sudo on Unix/Linux), or ask your System Administrator to provide the required access.
- Reset your site-packages directory permissions. Depending on your OS and Python installation, resetting these permissions vary.
- Reinstall your Python environment or roll back the system update introducing the changes. Whilst these are drastic measures, they’ll often resolve any disruptive changes.
Remember to discontinue using Python with root privileges. Running scripts related to internet access especially downloads in a superuser mode poses security vulnerabilities and it’s therefore advisable to use Virtual Environments.3 An excellent tool to manage multiple isolated Python environments is virtualenv.4The write permissions play a crucial role in
xxxxxxxxxx
Pip
/Python when it comes to installing and managing Python packages. A quick primer: Pip is a package manager for Python, useful for installing and managing software libraries written in Python. It connects with an online repository of public and private packages, making it easy for Python users to install virtually any Python package online.
By design, Unix-like operating systems authenticate all file access by checking permissions associated with each file. Each file and directory has an owner and an associated group. The system checks permissions for the owner, group, and everyone else – often encapsulated as User, Group, Owner (UGO) – each time a file operation attempt is made.
These permissions fall into three categories:
- Read: This allows you to open and read a file or list the contents of a directory.
- Write: This allows you to modify a file or directory.
- Execute: This allows you to run a program file or traverse a directory tree.
Any problem related to file operations can be traced back to these permissions.
When it comes to pip installations, the write permission is pivotal. If your Python installation’s site-package directory doesn’t have adequate permissions, pip will not be able to write the files during the installation process. Thus, the error “normal site-packages is not writable” will occur.
Taking it further with user-site installation:
To solve this predicament, pip offers the
xxxxxxxxxx
--user
option which installs packages in a Python user install directory for your platform. Typically
xxxxxxxxxx
~/.local/
, or
xxxxxxxxxx
%APPDATA%\Python
on Windows.
Running pip with the
xxxxxxxxxx
--user
option alters the installation location. The location becomes your home directory, a place where you typically have write permissions. That way, no special system privileges are needed.
Here’s how you might install requests library using the user option:
xxxxxxxxxx
pip install --user requests
This command takes the ‘requests’ library and installs it into the user directory, bypassing issues with the normal site-packages directory.
The workaround of Virtual environments:
Another common workaround for write permission problems is the use of virtual environments (
xxxxxxxxxx
venv
).
Virtual environments are isolated Python workspaces which can have different packages installs without interfering with each other or the system-wide Python installation. Since the user controls the venv, the pip in that venv can freely write packages to it.
xxxxxxxxxx
python3 -m venv my_env
source my_env/bin/activate
Then you could freely install packages in your venv without encountering write permission issues.
Overall, write permissions are fundamental in pip/Python because they control when and where packages can be installed. Whether it’s granting more permissions or cleverly bypassing restricted areas by using the
xxxxxxxxxx
--user
option or venvs, understanding and adjusting to your specific environment’s permissions can make all the difference in smooth and effective package management.
Dealing with the “Normal Site-Packages Is Not Writeable” error in Pip/Python could be a bit daunting, particularly if you’re not acquainted with Python’s package management structure. This issue arises predominantly when pip does not have the required permissions to install packages into the site-packages directory.
Before we dive deep into the solution, let’s first understand what site-packages is. It’s a directory within your Python installation that holds installed packages for that specific version of Python.
Causes
The chief reason behind this error is the lack of necessary permission to write files into Site-packages. This often happens:
- When you’re trying an installation without using a virtual environment, or
- After using
xxxxxxxxxx
111sudo
to install packages which modifies the ownership and permissions of certain directories.
A Possibility: Using virtual environments
One simple and clean way of circumventing these problems is by employing Python Virtual Environments. This solves the problem by creating isolated environments to run and manage Python and its packages individually for each project. We can create a Python virtual environment using the
xxxxxxxxxx
venv
module as outlined below:
xxxxxxxxxx
python3 -m venv /path/to/new/virtual/environment
After activating the virtual environment (with
xxxxxxxxxx
source /path/to/new/virtual/environment/bin/activate
), you can freely install Python packages without facing any permission issues.
Changing Ownership or Permission
In case you’re not utilizing a virtual environment, modification of the site-packages directory’s ownership could solve this issue. Use the code snippets below from a terminal to do so:
xxxxxxxxxx
sudo chown -R $USER /path/to/site-packages
Additional precautions might involve adding write permissions to the site-packages directory:
xxxxxxxxxx
sudo chmod -R u+w /path/to/site-packages
Replacing “/path/to/site-packages” with the actual path to your site-packages directory.
However, take note that changing the owner or permission of system files/directories comes with risk and it’s generally not advisable as it may potentially break some functionalities. It is recommended to opt for the usage of Python Virtual environments as mentioned above.
A core principle: Avoid usage of Sudo
Always avoid the use of
xxxxxxxxxx
sudo
for installing Python packages with pip. Doing so might cause Python or pip-related tasks to require root permission which isn’t best practice due to potential security concerns and the fact that user-level applications should not need root privileges.
Instead, choose to install packages either under a virtual environment or using the
xxxxxxxxxx
--user
flag which installs packages into your local user directory.
xxxxxxxxxx
pip install --user package-name
Hopefully, understanding the core issue causing the “Normal Site-Packages Is Not Writeable” error and following the possible measures will assist you in rectifying it efficiently while promoting better practices handling Python packages. Remember that each situation has unique factors and circumstances so ensure that you’re adjusting the solutions provided to fit your precise scenario. You might find Python’s guide on installing Software helpful. Additionally, information about managing Python packages with pip and venv might come handy.Python’s package manager pip installs Python packages in a directory known as site-packages. Sometimes, a permission issue may arise where the user does not have write access to the site-packages directory owing to various reasons like multiple Python versions, virtual environments, or non-admin rights.
Granting write access to normal site-packages is crucial for pip to install or upgrade any specific module within Python ecosystem. Here is how we can secure write access:
Virtual Environment
The recommended way of solving the issue is by using Python’s virtual environment for your project. This would create an isolated environment that does not interfere with system-wide Python setup and hence, mitigates permission issues.
To create a virtual environment, navigate to your project directory then run
xxxxxxxxxx
python -m venv env
To activate it:
xxxxxxxxxx
source env/bin/activate
(for Unix or MacOS)
or
xxxxxxxxxx
.\env\Scripts\activate
(for Windows)
Once the virtual environment is active, try installing your package via pip again and it should work without any permission issues.
The –user Parameter
If a virtual environment is not suitable for your use case, there is another solution. When installing a package via pip, add the parameter –user after the pip install statement.
xxxxxxxxxx
pip install --user <your-package>
This instructs pip to install the package at a location where the current user has write access to. One point to consider here is that modules installed this way will only be available to the current user, and not to other users on the system.
Changing Directory Permissions
A more drastic but less safe measure is to change the permissions of the site-packages directory globally:
xxxxxxxxxx
sudo chmod -R o+w /path/to/site-packages
This aids in granting write permissions to the user for site-packages directory. It is critical to remember that changing directory permissions can have unforeseen repercussions, and I caution against it unless absolutely necessary.
Making sure the pip version is up-to-date
Sometimes problems in dealing with packages installation or upgrading can be related to outdated pip versions. Therefore, keeping updated addition to aforementioned solutions can mitigate such issues.
xxxxxxxxxx
python -m pip install --upgrade pip
In general, the suggested methods can provide easy solutions to getting write access to site-packages directory when employing pip for package distribution in Python. Remember, altering permissions should be your last resort due to potential security concerns. It’s always better to go for safer options like creating a virtual environment which adds an extra layer of isolation and security while dealing with Python packages.
We’ve all been there before, coding in Python and you encounter a setup snag when trying to install new packages with pip. For some reason, your site-packages directory is not writable. As a professional coder, I’ll take you through some steps to troubleshoot Python package installation issues, particularly focusing on situations where the normal site-packages directory is appearing as not writeable. These bullet-pointed solutions are designed to be quickly digestible, enabling you to fast-track your route back to productive coding.
The Error Message
First off, let us pin down the problem. Typically, you might meet an error like this when trying to install or update a Python package:
xxxxxxxxxx
ERROR: Could not install packages due to an EnvironmentError: [Errno 13] Permission denied: '/usr/local/lib/python3.7/site-packages/some-package'
Consider using the '--user' option or check the permissions.
This error is indicating that pip couldn’t perform its operation because the
xxxxxxxxxx
site-packages
directory, where Python packages are stored, is read-only for the current user. The
xxxxxxxxxx
errno 13
specifies ‘Permission denied,’ which further confirms this.
Solution 1: Use the –user flag
Now, let’s delve into possible solutions:
One specified by the error message itself is the use of the
xxxxxxxxxx
--user
flag during package installation. This will install the package into a user-specific site-packages directory, rather than the global one:
xxxxxxxxxx
pip install --user package-name
The only downside of this approach is that this package will only be available for the currently signed-in user and not system-wide. Which is often fine in a development context.
Solution 2: Change the directory permissions
An alternative approach can be changing the permissions of the global site-packages directory, allowing the pip operation to proceed. To do this, execute:
xxxxxxxxxx
sudo chmod -R 777 /usr/local/lib/python3.7/site-packages/
However, this opens up the directory to writing by any user or process on the system, posing a security risk. A safer way would be to change the ownership of the site-packages folder to the current user like so:
xxxxxxxxxx
sudo chown -R $USER /usr/local/lib/python3.7/site-packages/
But this again has a caveat; it may interfere with system-managed package installations or updates.
Solution 3: Use a Virtual Environment
If you’d like a cleaner, encapsulated approach that doesn’t involve modifying system-level configurations, you could use Python’s built-in virtual environment (venv). Here essentially, each project gets its own local “copy” of Python and pip, eliminating permission issues and ensuring package versions specific to each project.
To create a virtual environment, navigate to your project directory and run:
xxxxxxxxxx
python3 -m venv venv-name
Then activate it:
For Unix or MacOS, run:
xxxxxxxxxx
source venv-name/bin/activate
And for Windows:
xxxxxxxxxx
.\venv-name\Scripts\activate
Now you can install packages without worrying about conflicts or permissions:
xxxxxxxxxx
pip install package-name
Troubleshooting package installation issues in Python can be frustrating at times, but the above techniques should have you covered for most occasions when dealing with unwriteable site-package directories. Each comes with its trade-offs, and considering these carefully in choosing the most fitting solution will save you significant wrench time down the road.
Indeed, having issues with site-packages directory permissions for Python can definitely be a nuisance. But fret not! I’ve got you covered on that bit. Before we delve into the solutions, let’s understand what this error means.
When you see the error “Pip/Python: Normal Site-Packages Is Not Writeable,” it is indicative that Python’s directory for storing packages (site-packages) has restricted write access. This primarily happens due to two reasons:
– You’ve installed Python as an administrator or via a package manager like brew on MacOS and you’re trying to install a Python package as a non-sudoer.
– Python is trying to install in the global site-packages directory instead of the local one.
Let’s go step-by-step on how to fix this error:
Step 1: Verify your Python and Pip installation
First, we need to confirm that both Python and Pip are properly installed in your system. As a pro-coder, I always suggest checking the version of your software to see if they’re correctly installed using the Python and Pip command-line interface:
For Python:
xxxxxxxxxx
python --version
For Pip:
xxxxxxxxxx
pip --version
If you have trouble accessing either of these interfaces, revisit their respective installation steps.
Step 2: Understanding Virtual Environments in Python:
Oftentimes, using Python’s venv module—a built-in feature since Python 3.3—solves many problems related to permission issues. It creates isolated spaces where you can install Python packages without affecting the global Python setup, ensuring no conflicts between different versions of the same package, and, needless to say, also taking care of the unwriteable site-packages directory issue:
To create a virtual environment:
xxxxxxxxxx
python -m venv name_of_your_venv
And then, to activate it:
On Windows:
xxxxxxxxxx
.\\name_of_your_venv\\Scripts\\activate
On Unix or MacOS:
xxxxxxxxxx
source name_of_your_venv/bin/activate
After activating the environment, any package you install using pip will be placed in the site-packages directory of the virtual environment, avoiding any conflicts with the global site-packages directory.
Step 3: Installing Packages Locally Using the User Scheme
If you don’t want to use virtual environments, another approach would be to install the package locally using the user scheme. Pip provides the handy –user flag which lets you install packages in a directory within your user account:
xxxxxxxxxx
pip install --user name_of_python_package
This mitigates the need to write inside the global site-packages directory, once again making the seemingly persistent ‘not writeable’ message dissipate.
These practices should solve the permission issue for the normal site-packages, enabling smoother coding in Python.
*Note*: For further guides, check out the official documentation on Python Packaging User Guide by Pip.As a final note on the topic of Pip/Python: Normal Site-Packages Is Not Writeable, it is crucial to remember that Python’s
xxxxxxxxxx
pip
is not just a package manager. It’s an effective tool that allows you to install, uninstall, upgrade, and manage Python packages effortlessly. Whenever you come across the error “Normal Site Packages is not Writeable,” don’t panic! It can typically mean one of two things:
- You’re trying to modify or install in a directory where your current user account doesn’t have write access.
- Your Python environment isn’t correctly configured, often when using a virtual environment or when having multiple versions of Python installed.
Usually, there are several ways to fix this issue. You could attempt to modify the directory permissions to allow the current user to write or execute commands with administrative privileges. Keep in mind that these changes should be applied judiciously.
An alternative solution is to utilize a Python virtual environment. A virtual environment sequesters and isolates your project’s working scope, preventing any issues related to permission or version conflicts among many Python versions/packages installed.
Example:
The below command creates a new virtual environment named ‘myenv’ and then activates it which will solve the normal site-packages not writable problem.
xxxxxxxxxx
python3 -m venv myenv
source myenv/bin/activate
Also, bear in mind that Python scripts require read and write permissions for proper execution, and some file systems (like NTFS) or network shares do not support setting specific Unix-style file permissions. Having adequate knowledge in managing permissions would help a great deal in fixing the ‘Normal Site-Packages is Not Writeable’ issue.
Here’s a brilliant guide on Linux file permissions if you feel like exploring the subject deeply.
Remember, gaining a good grasp on Python’s pip and understanding how it interacts with your environment could significantly enhance your Python programming experience and make troubleshooting easier when such problems arise. Happy coding!