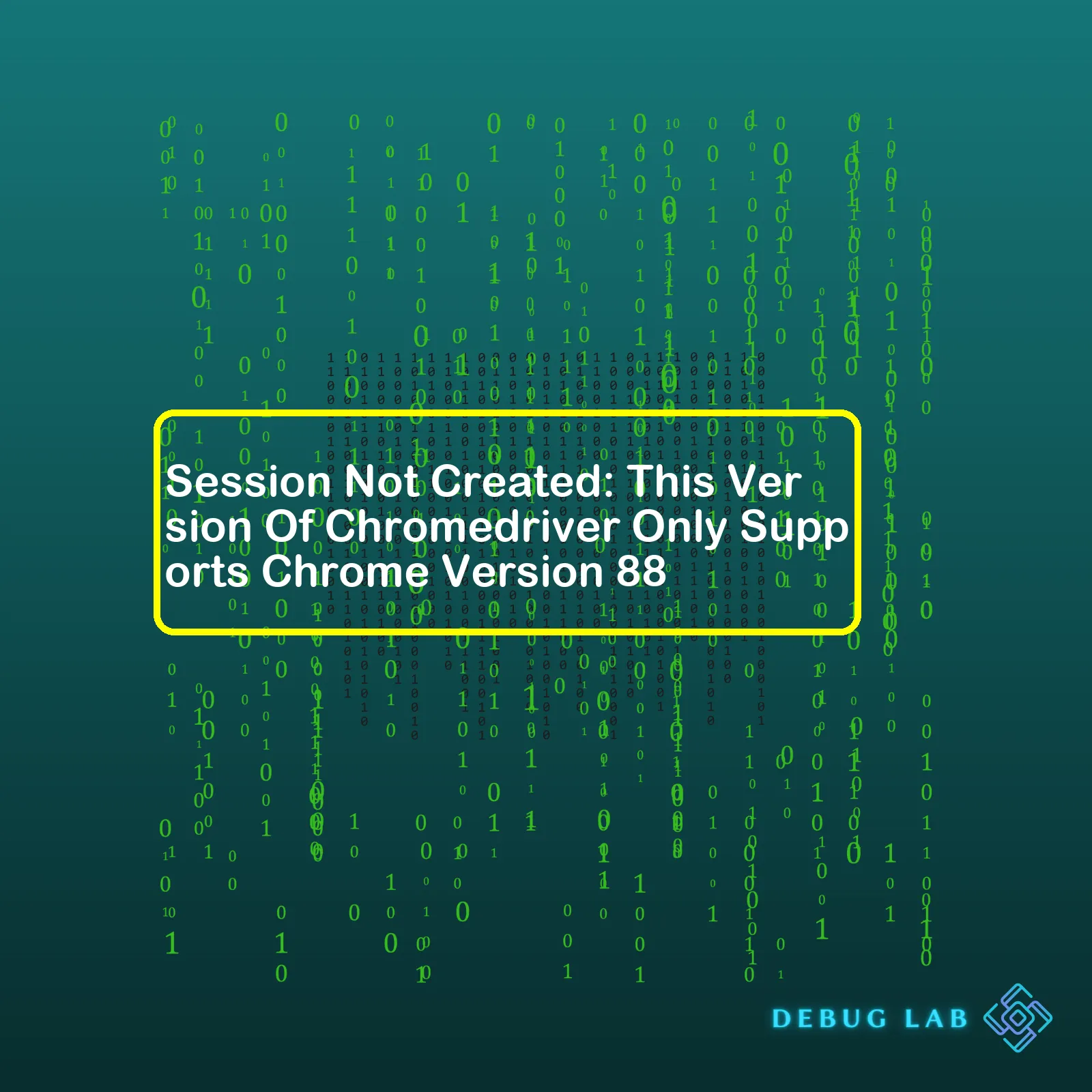
Error | Description | Solution |
---|---|---|
x 1 1 Session Not Created: This Version Of Chromedriver Only Supports Chrome Version 88 |
This error is triggered when the Chrome version on your machine and the ChromeDriver version are not compatible. | To resolve this issue; revert back to the previous version of Chrome or update ChromeDriver to the version that supports your current Chrome. |
The error
xxxxxxxxxx
Session Not Created: This Version Of Chromedriver Only Supports Chrome Version 88
is a common inconvenience many developers face, particularly in the fields of web development and automation testing. Having the incorrect version of ChromeDriver installed for the version of Chrome can cause communication mishaps between your code, the ChromeDriver, and Chrome itself.
ChromeDriver is a standalone server which implements WebDriver’s wire protocol for Chromium, essentially serving as the bridge between our programs and the Chrome browser for automation purposes. When an updated version of Chrome gets new features or updates existing ones, the relevant commands in ChromeDriver need to be updated too so as to keep pace. Hence, it is critical that ChromeDriver and Chrome versions are compatible.
When you see this error, it usually means that there’s an inconsistency between the version of Chrome installed on your system and the version of ChromeDriver your application is using. Specifically, the ChromeDriver version is designed for Chrome Version 88 and your machine has a different one. Consequently, the sessions cannot be created successfully.
To resolve this issue, you have two main options. First, you could downgrade your Google Chrome installation to Version 88 if possible, allowing the preexisting ChromeDriver to interface effectively with the desired browser build. However, bear in mind that older versions of software can potentially expose your system to security threats due to known vulnerabilities. Alternatively, you could choose to upgrade your ChromeDriver to a version that supports your currently installed Chrome version. Periodically updating your drivers to their latest stable versions is often recommended as part of good maintenance practices.
For instance, If your chrome browser’s version is 92, you might want to get the ChromeDriver 92 as they are considered to be compatible.
You can download latest drivers from the ChromeDriver download page.
xxxxxxxxxx
Note:
Do remember to add the path of the newly downloaded ChromeDriver to the system environment variable – PATH.
After a successful upgrade or downgrade, consider restarting your system or at least your automation app to apply the changes effectively. You should be now capable of creating successful WebDriver sessions without encountering this error.
If you are dealing with the error message “Session Not Created: This Version Of Chromedriver Only Supports Chrome Version 88” in Selenium, it’s crucial to understand that this issue arises from a mismatch between the versions of Chrome browser and the ChromeDriver.
Selenium WebDriver makes direct calls to the browser using each browser’s native support for automation. For browsers like Chrome, they require a separate executable driver which needs to be synchronized with the browser version installed on your system. In this particular case, the installed ChromeDriver only supports Chrome version 88. Therefore, if the version of your installed Google Chrome browser is not matching version 88, then the error occurs.
xxxxxxxxxx
121WebDriverException: Message: session not created: This version of ChromeDriver only supports Chrome version 88
2
To solve this issue, you need to follow these steps:
- Determine the version of the Google Chrome browser installed on your machine. You can easily find this by going to the “Help” section, then click on “About Google Chrome”. This page displays the current version of your Chrome browser.
- If your Chrome version is higher or lower than 88, you should download the compatible ChromeDriver from the Chromedriver download page.
- Replace the old ChromeDriver executable file in your project or in your system path with the new downloaded version.
- Implement the usage of the new ChromeDriver in your Selenium script. Here’s a quick Python code for instance:
xxxxxxxxxx
141from selenium import webdriver
2
3driver = webdriver.Chrome('/path/to/new/version/of/chromedriver')
4
- The “/path/to/new/version/of/chromedriver” represents the location where you saved the newly downloaded ChromeDriver. It should be replaced with your actual path.
- Lastly, re-run your Selenium script to confirm that the “Session not created” error has been resolved.
Keeping your Chrome browser and ChromeDriver versions aligned will prevent such errors from happening. A good practice could be setting automated alerts for when a new update releases, so you can adapt your testing environment correspondingly right on time.
Please remember to also check the compatibility between the Selenium WebDriver version you’re using and the browser driver version, as mismatches there might cause similar errors.
By proactively managing these dependencies, you’ll make your web automation journey with Selenium more stable, scalable, and enjoyable!
Typically, when you encounter the error message “Session Not Created: This version of Chromedriver only supports Chrome Version 88”, it signifies an inconsistency between the versions of Google Chrome and Chromedriver you’re utilizing.
Underlining Reasons
The principal reason for this issue could be due to Chromium project. As part of its decided procedures, each Chromedriver version aligns with a specific release of the Google Chrome Browser. So, if your Chromedriver supports only Google Chrome version 88, it’s suggestive that the web automation operations can work best with that browser variant.
To put simply:
- The Chromedriver was expressly built for the Google Chrome Web Browser.
- Different versions of Google Chrome require different versions of Chromedriver. This is because as Google Chrome gets updated, new features are added, bugs are fixed, and certain operations are altered; hence, a correct version of Chromedriver with compatible functionalities has to be maintained.
Solutions
Avoid the striking of another similar problem in the future by adopting the following suggestions:
- Manually maintain the compatibility of the two. This means checking the current Chrome Browser version installed on your system (
xxxxxxxxxx
111Help → About Google Chrome
), then downloading the corresponding Chromedriver from the Chromium website.
- Use Webdriver Manager. It automatically manages the browser drivers (Chrome, Firefox, Microsoft Edge, etc.) versions according to your browser’s version. The process includes checks for updates, downloads, and sets up browser drivers automatically, effectively resolving version mismatches.
Code Example: Using Webdriver Manager
Here’s an example of how to use Webdriver Manager with Selenium to launch Google chrome:
xxxxxxxxxx
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import io.github.bonigarcia.wdm.WebDriverManager;
public class Test {
public static void main(String[] args){
WebDriverManager.chromedriver().setup();
WebDriver driver = new ChromeDriver();
driver.get("https://www.google.com");
}
}
This would eliminate the need to manually download the Chromedriver matching the installed Google Chrome version as WebDriverManager handles it efficiently.
Frequently Auto-Updating Google Chrome
If you have allowed auto-updation for Google Chrome, and the existing code uses an older Chromedriver, you might face a variation of the aforementioned issue, i.e., “session not created: This version of ChromeDriver only supports Chrome version X”, where ‘X’ is the previously compatible but now outdated version number. Consequently, keeping your drivers updated is paramount for streamlined operations.
Hence, to keep your test environment consistent, stable, and not encounter such sessions or version support errors, ensure a match between the version of Chromedriver and Google Chrome by manually maintaining compatibility or by dynamically managing it using Webdriver Manager.
Chromedriver is an essential component when running automated tests on Chromium browsers such as Google Chrome. It’s a standalone server that interacts with the Webdriver in a specific version of Chromium. If there’s a mismatch between the Chromedriver and Chromium versions, there could be unexpected behaviors. One common issue you might come across is the
xxxxxxxxxx
SessionNotCreatedException
error with the message: “This Version Of Chromedriver Only Supports Chrome Version 88”. This happens when your automatic testing tool is trying to communicate with a Chromium browser using a different version of Chromedriver.
Error Type | Description |
---|---|
xxxxxxxxxx 1 1 1 SessionNotCreatedException |
You might encounter this error when there’s a version mismatch between Chromedriver and your Chromium browser. The WebDriver cannot successfully create a secure session due to this discrepancy. |
The impact of using unsuitable versions of Chromedriver can be wide-ranging:
- Incompatibility issues: You might face functionality problems as some operations might not work as expected due to compatibility issues.
- Unreliable test results: Tests run on an incompatible setup are less reliable. The tests may fail or experience undiscovered problems due to these underlying disparities.
- Increased debugging time: Mismatched versions could lead to time-consuming troubleshooting, possibly requiring you to dig into unanticipated system levels.
- Slower test execution: Different versions may affect test execution performance which could slow down your overall project.
Generally, each Chromedriver version is designed to support various chrome versions. So, if you’re running Chrome 88, ensure you’re using the corresponding Chromedriver version. Here’s an example of how you can check and match Chromedriver and Chrome versions:
xxxxxxxxxx
// Using Linux bash shell
// Check Chrome version
google-chrome --version
// Update Chromedriver version to match Chrome
wget https://chromedriver.storage.googleapis.com/<VERSION>/chromedriver_linux64.zip
unzip chromedriver_linux64.zip
Consistently keeping your Chromedriver up-to-date will significantly reduce the risk of encountering inconveniences associated with incompatible versions. Tools and libraries like webdriver_manager automatically manage drivers for Python, saving you from these potentially detrimental impacts.
You can also use online tools such as Chromedriver Download and Version Selection to help you select the suitable Chromedriver version depending on your installed Chrome version.
Having accurate versions formulates a robust foundation for any successful automatic testing environment. It assures consistent behavior throughout your testing processes, resulting in reliable results.
Selenium WebDriver had made it easy for web developers and testers to automate browser activities. One of the key challenges that users of Selenium WebDriver face is compatibility issues between the WebDriver version and that of the browser.
For example, receiving a “Session Not Created: This Version of Chromedriver Only Supports Chrome Version 88” error means there is a mismatch between the Chromedriver version and the Google Chrome Browser version installed on your machine. It can be tricky to automatically align WebDriver and browser versions, especially if your project involves multiple browsers and systems.
Identify the Problem Version Mismatch
The first step in resolving this issue is identifying the mismatched versions:
– The
xxxxxxxxxx
"This version of ChromeDriver only supports Chrome version XX"
indicates your ChromeDriver version.
– Use this command to check your Google Chrome’s version:
xxxxxxxxxx
chrome://settings/help
Resolve the Issue
To resolve the issue, consider these two options:
1. Update ChromeBrowser:
The most straightforward fix would be to update your Chrome browser to match your current chromedriver. You can accomplish this by going to Google’s official site to download and install the latest chrome browser. However, sometimes, you just cannot control the version of the browser especially when you’re working with a browser in a shared environment.
2. Download Compatible ChromeDriver Version:
Alternatively, download a compatible ChromeDriver version consistent with the installed Chrome Browser version.
Visit ChromeDriver Downloads Page where a list of available versions will be displayed. Select and download a release that matches your specific Chrome Browser version. After downloading, replace the existing one on your local machine.
Automating Matching Process
While the methods mentioned above work, they require manual intervention each time there is a selenium driver upgrade or browser version change. An effective way to automatically align the configurations is using webdriver managers.
Below is a Python code snippet that demonstrates the use of the Webdriver Manager for Chrome:
xxxxxxxxxx
from selenium import webdriver
from webdriver_manager.chrome import ChromeDriverManager
driver = webdriver.Chrome(ChromeDriverManager().install())
In this example, we’ve employed the `webdriver_manager` package. Whenever a webdriver instance gets initiated, the `ChromeDriverManager().install()` command checks for the latest version of the browser installed in your system and downloads the compatible WebDriver binary if not present, or if the version is outdated.
Remember, while solving this issue always ensure any change you make does not break other parts of your application or affect its overall performance.Being a coder, I’ve had my share of experience with WebDriver dependencies and the challenges they can bring about. In this case, it appears you’re getting an error saying “Session Not Created: This version of ChromeDriver only supports Chrome version 88.” To interpret this error, it’s actually saying that the version of ChromeDriver that’s installed in your system is meant to work with Chrome Browser Version 88.
The underlying reason for this error message may be because the Chrome browser automatically updates to its newest version when connected to the internet. However, it doesn’t automatically update ChromeDriver, which is a separate component from the browser itself. Thus, leading to a mismatch between the two versions.
Firstly, confirm we are on the same page by checking if your Chrome is updated or not. Type “
xxxxxxxxxx
chrome://settings/help
” in your Chrome address bar and press Enter. You’ll see the information about the current version and whether your Chrome needs an update.
Then, here is how you can update your ChromeDriver:
Finding the right ChromeDriver version:
You can look for the appropriate version of ChromeDriver that matches your current browser version in the specific format ‘ChromeDriver xx.xx’. Google maintains all the previous releases of ChromeDriver on their repository (LINK), where you can download and install them.
Download and replace existing Chromedriver:
After you have discovered the suitable ChromeDriver for your Chrome version:
1. Download the corresponding ChromeDriver version binary.
2. Unzip the downloaded file and extract the ‘chromedriver.exe’ driver.
3. Finally, replace the current ‘chromedriver.exe’ with the one recently downloaded to the appropriate location in your system where the older version currently resides.
Using WebDriver Manager:
If you don’t want to manually handle the ChromeDriver versioning, you can also use WebDriver Manager libraries such as “webdrivermanager” for Python or “WebDriverManager” for Java. These tools will automatically match the browser and driver versions. They download the drivers binaries/executables in an automated way. Here’s how you can do it in Python:
xxxxxxxxxx
from webdriver_manager.chrome import ChromeDriverManager
from selenium import webdriver
driver = webdriver.Chrome(ChromeDriverManager().install())
or in Java:
xxxxxxxxxx
import io.github.bonigarcia.wdm.WebDriverManager;
WebDriverManager.chromedriver().setup();
WebDriver driver = new ChromeDriver();
Remember, updating your ChromeDriver isn’t just about fixing this problem – it also ensures better compatibility and smoother functioning of your automated testing process, enhancing your overall coding efficiency!
Do implement these suggestions to keep your program running effortlessly while aligning your Chrome and ChromeDriver versions.In the world of web automation testing, keeping your browser and web driver versions synchronized is of utmost importance. More often than not, discrepancies between these two variables lead to the aggravating instance of session creation failure, prominently error messages like “Session Not Created: This Version Of Chromedriver Only Supports Chrome Version 88.”
There are several benefits behind maintaining your chrome browser and chromedriver in sync:
Compatibility:
– The main reason you should keep your browser and driver versions in sync is compatibility. Different versions of browsers and drivers have different functionalities or lack thereof. For example, a driver made for an older version of a browser might not be able to support newer features rolled out in a latest browser release.
– The specific error message you cited,
xxxxxxxxxx
"Session Not Created: This Version Of Chromedriver Only Supports Chrome Version 88"
pops up when there’s a mismatch between the version of the Chrome browser and Chromedriver. By keeping the versions synchronise, we can avoid such errors.
Stability:
– A properly synced browser-driver pair ensures stability during test executions. Mismatches could possibly fluctuate automated test behaviour leading to unpredictable and unreliable test outcomes.
– Discarding significant numbers of test runs due to session initiation failures would not only incur unnecessary efforts and resources but also hamper the flow and productivity of the testing process.
Achieving Better Test Results:
– Keeping your browser version harmonized with your driver’s promotes obtaining accurate and improved test results, since appropriate interfaces would be exercised as devised.
Maintenance:
– Keeping browser-driver harmony reduces maintenance efforts. As you update one component, the respective component needs updating as well. Maintaining them separately may lead to unnecessary troubleshooting which might consume a chunk of valuable time that can be invested elsewhere.
To tackle the “Session Not Created” kind of issue, always ensure that the Chrome browser and Chromedriver versions match. You can check the current version of your Chrome Browser by navigating to
xxxxxxxxxx
"chrome://version/"
in the address bar and hitting enter.
Next, download the matching version for Chromedriver from the official ChromeDriver downloads page. Replace the existing Chromedriver executable with the newly downloaded match.
Code Sample:
xxxxxxxxxx
from selenium import webdriver
# specify path to chromedriver download
PATH = "/path/to/your/chromedriver"
driver = webdriver.Chrome(PATH)
# navigate to a website
driver.get("https://www.yourwebsite.com")
Additionally, consider automating the synchronisation process with available solutions or use continuous integration (CI) servers, these are already equipped to handle such problems automatically. They regularly update both browser and driver versions ensuring harmonised interaction.
Therefore, it is evident that alignment of your browser and driver is crucial for smooth operations in automated testing. It not only assures steady test runt but also minimises potential hiccups in the testing processes thereby increasing efficiency, promoting accurate test results and reducing maintenance overhead. Trust me, the harmony achieved here would make the entire testing symphony super melodious. Happy Coding!Sure, the error message, ‘Session Not Created: This Version Of Chromedriver Only Supports Chrome Version 88,’ indeed suggests that you’re using a chromedriver that’s compatible only with Chrome version 88. Yet, if your system runs another Chrome version, a disconnection occurs, creating this issue.
Delve into these troubleshooting tips to eliminate such issues:
Verifying Your Chrome Browser Version
Firstly, verify your current Google Chrome browser version. Open your browser and go to chrome://settings/help, your browser version number is available there. Examples include 87.0.4280.141 or 94.0.4606.61.
Downloading an Compatible ChromeDriver
Knowing your chrome browser version is crucial as it helps identify what version of ChromeDriver to download. Say if you’ve got Chrome version 87, download ChromeDriver 87.XX.XX.XX.
Use the ChromeDriver downloads page to do this. Always ensure you have a compatible pair between Chrome and ChromeDriver to prevent the stated error.
Updating Your ChromeDriver Path
After downloading the correct ChromeDriver version, make sure your code can access it by correctly setting the ChromeDriver path. Here’s a python example on how to set it:
xxxxxxxxxx
from selenium import webdriver
# put the correct path to your chromedriver below
driver = webdriver.Chrome('C:/path/to/your/chromedriver')
Automating the ChromeDriver Setup Process
Consider automating the setup process. In Python, the webdriver manager package handles driver related issues efficiently. With it, there’s automatic matching of the ChromeDriver version to the browser version followed by the necessary Chromedriver updates.
Here’s an example of how to use webdriver manager to automatically use the correct ChromeDriver:
xxxxxxxxxx
from selenium import webdriver
from webdriver_manager.chrome import ChromeDriverManager
driver = webdriver.Chrome(ChromeDriverManager().install())
Following Regular Updates
Google Chrome auto-updates can lead to sudden changes in your browser version, presenting difficulties while running your scripts unless you keep everything updated. Make a habit of regularly updating your ChromeDriver to match your browser version for smoother operations.
By following these measures diligently, you’ll skip the hassle often linked to the ‘Session Not Created: This Version Of Chromedriver Only Supports Chrome Version 88’ error and streamline your coding process impeccably.When it comes to the issue of “Session Not Created: This Version Of Chromedriver Only Supports Chrome Version 88”, I recommend updating your Chromedriver and Google Chrome to their latest versions.
The issue arises when there’s a mismatch between Chromedriver and Google Chrome versions, as the error message indicates. This is because Chromedriver is a separate component from Google Chrome that needs to be compatible with the browser version you’re using. Simply put, if they’re not compatible – you can encounter the ‘Session Not Created’ error.
xxxxxxxxxx
# Here is how you update ChromeDriver within the terminal
$ webdriver-manager update
Moreover, you could also specify a path to the Chromedriver executable that matches your installed Chrome version manually. Alternatively, dynamically get the right driver for your system using a service like ChromeDriver Download.
xxxxxxxxxx
# Here's how you can specify the path to Chromedriver
from selenium import webdriver
webdriver.Chrome('/path/to/your/chromedriver')
Remember to replace ‘/path/to/your/chromedriver’ with the actual path to your Chromedriver executable.
xxxxxxxxxx
# Here's a Python package that simplifies managing Chromedriver
# It automatically downloads the driver that corresponds with your Chrome version
pip install chromedriver-autoinstaller
import chromedriver_autoinstaller
chromedriver_autoinstaller.install()
Checking and making sure both these components are regularly updated prevents possible incompatibility errors from arising. In fact, working with outdated versions opens up risks to potential security vulnerabilities and reduced functionality. So it’s best to keep them updated for optimized performance.
Keep an eye on official websites and resources where Chromedriver and Google Chrome publish updates or news regarding recent releases. These sites should have detailed instructions regarding installation procedures fit to your specific OS and environment.
Google’s official Chrome Browser website, as well as the Chromedriver Help Center, are great places to start.
Remember, the key to smooth, streamlined software development is consistent updates and compatibility checks. Make sure your tools and platforms are synchronized to create a seamless coding experience.