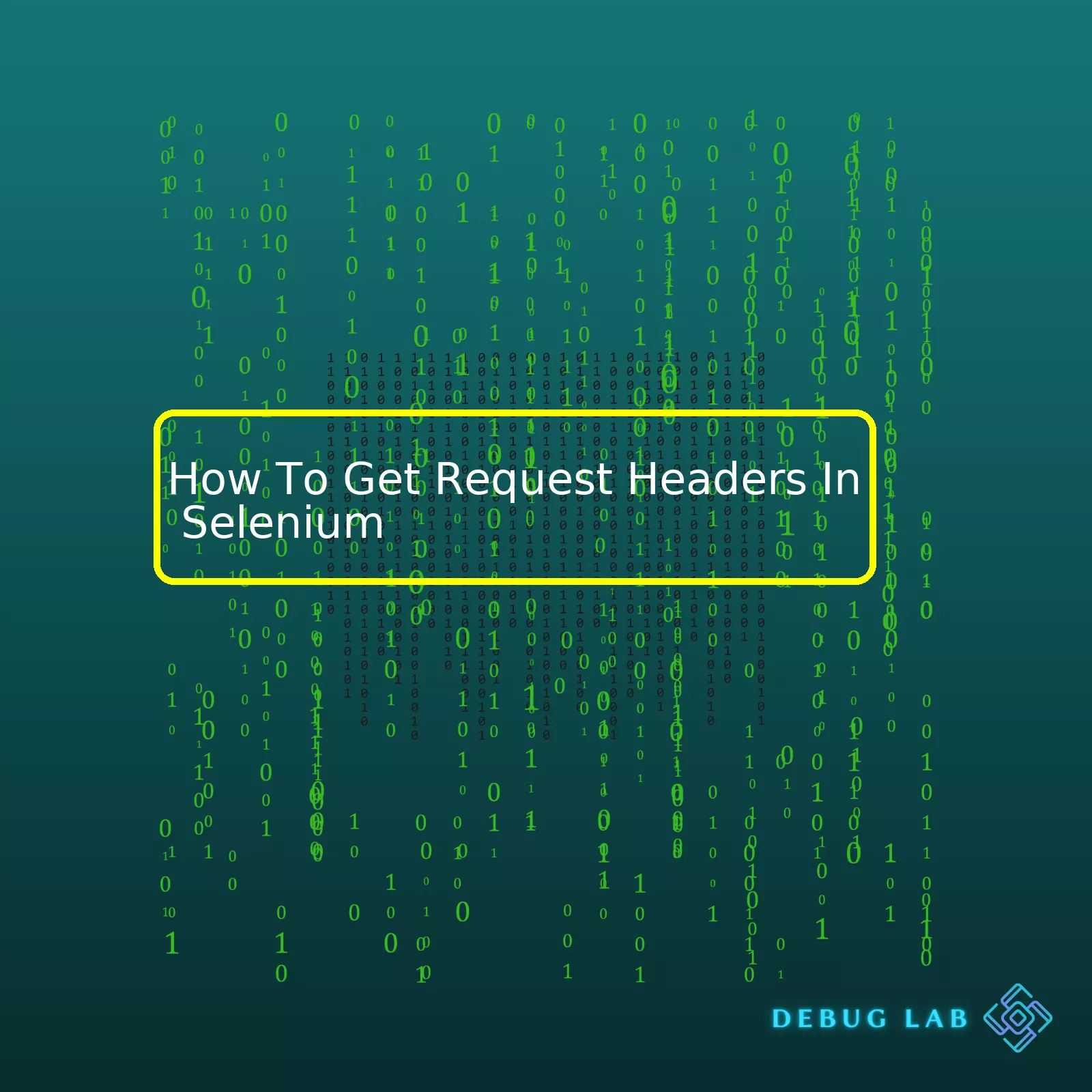
Here’s an HTML table listing down the steps involved:
Steps | Description |
---|---|
Install BrowserMob-Proxy | This involves downloading and adding the BrowserMob-Proxy into your project. It serves as a proxy server that will enable capturing HTTP requests. |
Set up Proxy Server | Use BrowserMob-Proxy to set up a new proxy server. |
Configure Selenium Webdriver | Selenium needs to be configured to leverage the newly created proxy server. |
Enable HAR Capture | Enable HTTP Archive (HAR) using BrowserMob-Proxy to start capturing headers. |
Access Request Headers | Once HAR capture is enabled, you can access request headers through the HAR logs. |
To elaborate a bit more on getting request headers with Selenium, when using tools like BrowserMob-Proxy (source), it provides you with capabilities that Selenium WebDriver does not directly offer. After setting up the Proxy server, Selenium WebDriver needs to be configured to use this server. This is accomplished via DesiredCapabilities objects or ChromeOptions for Chrome specifically.
Each browser has specific ways to deal with proxy settings.
Once HAR capture enabled using
manualHarCapture
method provided browsermob-proxy, we can navigate to the webpages and allow the proxy to collect HAR data which contains information about HTTP requests and responses.
We can obtain the headers from these logs. Here’s a quick look at how you’d do that in code:
ProxyServer server = new ProxyServer(9091); server.start(); server.newHar("request"); // Navigate to desired web page driver.get("http://www.example.com"); // Get request headers Har har = server.getHar(); for (HarEntry entry : har.getLog().getEntries()) { System.out.println(entry.getRequest().getHeaders()); }
Remember, capturing network traffic requires more resources and potentially slow down your tests. Therefore, it’s a good idea to turn off HAR capture once you’re done collecting the necessary data.Sure, delving into the world of Selenium WebDriver and request headers is an exciting journey. One very common question that people often ask is how they can get request headers while automating their tests using Selenium.
Well, it is essential to understand that Selenium is essentially a browser automation tool. In its standard form, it isn’t designed to interact directly with HTTP requests or response headers.
However, all hope is not lost! There are many indirect ways to access request headers during your Selenium testing process. Some of these methods involve integrating Selenium with tools such as BrowserMob Proxy or HAR viewer.
Let’s take a brief look at one of these workarounds using Selenium with BrowserMob Proxy:
First, you need to download and import the BrowserMob Proxy server. Once done, initiate the proxy server using:
BrowserMobProxyServer proxy = new BrowserMobProxyServer(); proxy.start();
Next, create a DesiredCapabilities object with the proxy settings and initialize your WebDriver with these settings.
DesiredCapabilities capabilities = new DesiredCapabilities(); capabilities.setCapability(CapabilityType.PROXY, ClientUtil.createSeleniumProxy(proxy)); WebDriver driver = new ChromeDriver(capabilities);
Following that, enable the HAR capture on the proxy server, navigate to your page, then retrieve and print the headers.
proxy.newHar("google.com"); driver.get("http://www.google.com"); Har har = proxy.endHar(); for (HarEntry entry : har.getLog().getEntries()) { for (HarNameValuePair pair : entry.getRequest().getHeaders()) { System.out.println(pair.getName() + ": " + pair.getValue()); } }
In this example code, we first start the proxy with
proxy.start()
. Then we set the WebDriver to use this proxy. We capture the network traffic by opening a new HAR with
proxy.newHar("google.com")
, go to our target page with
driver.get(url)
, and stop network traffic capture by ending the HAR with
proxy.endHar()
statement. The resulting structure contains all network requests made while the HAR was active, including the headers of each request.
If you’re not comfortable with dealing with proxy servers, another interesting approach is to use javascript and AJAX to send a dummy request to any URL and then abstract the request headers from that.
You could inject javascript into selenium as shown below:
JavascriptExecutor js = (JavascriptExecutor) driver; String script = " ... "; // Your JavaScript code js.executeScript(script);
So, here’s the main drawback: neither method is particularly clean or easy, but then again, what in developer life ever is? Each method has its advantages and use-cases based on what your requirements are, so choose wisely!
Remember, these workarounds are just that – workarounds. Neither is perfect and both come with their own drawbacks. But if you absolutely need this data, either of these routes will get you there. It is worth investigating further and checking out tutorials or guides like these on Selenium’s official documentation.Certainly, decoding the basics of HTTP requests in relation to getting request headers in Selenium can be an interesting topic for exploration. At the core of web testing automation, understanding the workings of HTTP requests plays a vital role.
First and foremost, let’s begin by understanding that HTTP (HyperText Transfer Protocol) functions as a request-response protocol in the client-server computing model. In simpler terms, a client, in this case a web browser, sends an HTTP request to the server–the server then responds by sending back an HTTP response.
Now, accompanied with these HTTP requests are HTTP headers – pieces of information that provide data about the requested file or the server behavior. A fundamental comprehension of such headers is required when setting up automated testing via Selenium.
// Importing necessary libraries import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.devtools.DevTools; import org.openqa.selenium.devtools.v89.network.Network; // Function to get HTTP Headers public void getRequestHeaders() { WebDriver driver = new ChromeDriver(); DevTools devTools = ((ChromeDriver) driver).getDevTools(); devTools.createSession(); devTools.send(Network.enable(Optional.empty(), Optional.empty(), Optional.empty())); devTools.addListener(Network.requestWillBeSent(), entry -> System.out.println(entry.getRequest().getHeaders())); }
The code snippet above illustrates getting request headers using Selenium WebDriver with the DevTools API. After initializing the WebDriver and DevTools objects, a new DevTools session is created. Following this, the Network domain of Chrome is enabled where all network events can be monitored. The listener function “requestWillBeSent” allows us to capture every outgoing HTTP request from the browser, fetching all request headers associated.
It is worth noting other types of HTTP request methods commonly used:
– GET: It retrieves or gets data from the server.
– POST: It sends or posts data to the server.
– HEAD: Similar to the GET method but only receives header, not the actual data.
– PUT: It updates current data on the server.
– DELETE: It removes data from the server.
With this kind of knowledge, you’re now equipped to leverage Selenium WebDriver to its full capacity. Automate dynamic tests using various HTTP Request methods and analyze their corresponding responses. Helping optimize your web application performance, while looking out for potential issues. You’ll find expansive tutorials at Selenium official documentation.
Moving further with HTTP requests, I implore you to familiarize yourself with Status Codes and Response Headers. They play a critical part in comprehending how your server reacts to certain requests. Remember, understanding these basics of HTTP requests will help you add another dimension to your automation capabilities.
You can also look into tools like BrowserMob Proxy or Fiddler which allow you to view and manipulate network traffic when combined with Selenium. This deepens your ability to analyze the state of your web application during testing.
Whether you’re achieving seamless page navigation, identifying performance bottlenecks, optimizing load times, verifying redirects or ensuring secure data transmission, HTTP requests form the crux of it all.
The process of extracting request headers in Selenium can be a bit complex, but quite rewarding when achieved correctly. The reasons for this complexity largely lie in the fact that Selenium by itself does not have a direct method to get the Request Headers. Nonetheless, there exists a clever workaround by relying on browser-based developer tools. In Google Chrome, the popular choice is an API called “Network”, from Chrome DevTools Protocol (CDP).
Let’s dive deeper into how one can utilize this technique.
Firstly, make sure you import the necessary classes and interfaces required for Chrome DevTools:
import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.devtools.DevTools; import org.openqa.selenium.devtools.v91.network.Network;
With everything set up, initiate DevTools and create a session:
DevTools devTools = ((ChromeDriver) driver).getDevTools(); devTools.createSession();
The next step is to enable Network tracking via the DevTools API:
devTools.send(Network.enable(Optional.empty(), Optional.empty(), Optional.empty()));
At this point, we can tell DevTools to start listening to the Network.requestWillBeSent events. This event will fire when a network request is about to be sent and contains request header information.
devTools.addListener(Network.requestWillBeSent(), request -> { // Voilà! We have access to the request headers. Mapheaders = request.getRequest().getHeaders(); });
Now we’re successfully listening to every request sent and obtaining all their request headers. Each time a request is sent, it captures the header and stores them into the ‘headers’ map.
Pro tip: If you specifically want to track only certain kinds of requests or URL patterns, you could include a conditional checking within the listener definition to filter out desired network calls:
if (request.getRequest().getUrl().contains("desired_pattern")) { Mapheaders = request.getRequest().getHeaders(); }
Let me underline the elegance of using DevTools API for such tasks. Not only do we get capabilities beyond WebDriver, like retrieving request headers, but we also leverage a powerful toolset used by developers worldwide directly integrated into our beloved browsers.
To read more about the Network API, follow this link Chrome DevTools Protocol – Network.
In a nutshell, while achieving this might not be straightforward with Selenium WebDriver alone, the combination with browser DevTools opens up new possibilities and allows us to extract the set of request headers easily. It underscores the power of leveraging browser-native developer tools APIs alongside Selenium. Understanding how your browser works under the hood can truly level up your automation game.
Lastly, it’s worth mentioning again that different approaches might be better suited depending on what underlying web driver you use. This particular strategy very much capitalizes on chrome’s built-in DevTools with its substantial exposure of the underlying networking operations. Other browsers may offer somewhat different routes towards reaching similar results. Good luck diving into the nitty-gritty details of your network traffic!Certainly, let’s delve into the Importance of Webdriver and Proxy Server, with a focus on How To Get Request Headers In Selenium.
Webdriver is an essential tool in Selenium’s toolset. It’s a collection of language specific bindings to drive a browser – the way it’s meant to be driven. A significant plus point about webdriver is its ability to work with modern advanced web-applications, along with providing support for traditional and modern browsers.
In the world of testing applications, Proxy server use can’t be understated as well. In a nutshell, Proxy servers act as an intermediary server, separating end-users from the websites they view. Serving as a middle ground between the client (which could be your Selenium tests) and the application under test (AUT), it reroutes requests from the client to the AUT and fetches responses back to the client. This route between the webdriver and the actual web occurrence passes through the proxy server, and all requests go through it.
Now, coming to “How to get request headers in Selenium”, you might notice that getting request headers directly using Selenium isn’t possible. This is where the interaction between the WebDriver and Proxy server comes into prominence. With programs like BrowserMob Proxy or Fiddler configured correctly, we can capture network traffic, thereby obtaining request headers.
Here’s a basic example of how one might work with Selenium and BrowserMob Proxy:
Example snippet:
from browsermobproxy import Server from selenium import webdriver server = Server("path/to/browsermob-proxy") server.start() proxy = server.create_proxy() chrome_options = webdriver.ChromeOptions() chrome_options.add_argument("--proxy-server={0}".format(proxy.proxy)) browser = webdriver.Chrome(chrome_options=chrome_options) proxy.new_har("google") browser.get("http://www.google.com") print(proxy.har) server.stop() browser.quit()
In the example above, you’re activating the BrowserMob Proxy server and setting up your webdriver instance to utilize this proxy. Then you create a new HTTP Archive (HAR) object, navigate to google.com, and print out the HAR data (where the request headers reside). The BrowserMob Proxy Py library documentation offers more details.
Notice anything? The interaction between the WebDriver and proxy server is clear here. Without these two components integrating effortlessly, acquiring request headers would be a tough nut to crack.
It’s evident that WebDriver and Proxy Server are not just crucial in the broader realm of web development but also paramount for niche operations like obtaining request headers in Selenium. Ultimately, combining the power of webdriver to drive browsers and the utility of proxy servers to monitor network traffic makes data gathering possible in a variety of intricate scenarios.In Selenium, a web automation tool, there isn’t a direct method to get HTTP request headers. But don’t worry, we do have workarounds that you can implement in just a handful of steps. Below, we will walk you through several methods, like:
– Using Browser Network Tools
– Leveraging BrowserMob Proxy
– Utilizing Python + Selenium along with BrowserMob Proxy.
So, let’s delve a bit deeper into each one.
Using Browser Network Tools
The simplest option is to use the in-built network tools in a browser like Chrome or Firefox, in combination with Selenium:
1. Navigate to the URL using Selenium.
2. Open the developer tools and switch to the ‘Network’ tab.
3. Refresh the page so the network requests show up.
4. Right-click a network request and select ‘Copy -> Copy as cURL’.
Now, these headers can be utilized or transformed into code in languages like Python, Node.js, etc.
However, this approach might not be feasible if your application sends requests internally without a full page refresh because those requests might not appear when you refresh the page. In such scenarios, we should use a proxy server, like BrowserMob Proxy.
Leveraging BrowserMob Proxy
BrowserMob Proxy is a utility tool used primarily for capturing performance data for web apps. It monitors HTTP traffic and can manipulate browser behavior and traffic also:
from browsermobproxy import Server server = Server("path_to_browsermob-proxy") server.start() proxy = server.create_proxy() profile = webdriver.FirefoxProfile() profile.set_proxy(proxy.selenium_proxy()) driver = webdriver.Firefox(firefox_profile=profile)
To capture Http Headers:
proxy.new_har("req", options={'captureHeaders': True, 'captureContent':True}) driver.get(url)
Then, this header information can be extracted from the HAR (HTTP Archive) object:
print(proxy.har)
Python + Selenium along with BrowserMob Proxy
If we’re working with Python along with Selenium, combining BrowserMob Proxy makes it easy to extract headers. This detailed post from StackOverflow provides practical steps for this setup.
Meanwhile, let’s take a look at a quick code snippet showing how this integration works:
from browsermobproxy import Server from selenium import webdriver import json # define paths browser_mob_path = "/path/to/browsermob-proxy" firefox_driver_path = "/path/to/geckodriver" # set up server and driver server = Server(browser_mob_path) server.start() proxy = server.create_proxy() driver = webdriver.Firefox(executable_path=firefox_driver_path, proxy=proxy) # create HAR archive proxy.new_har() # navigate to the web page driver.get("http://example.com") # get entries entries = proxy.har["log"]["entries"] for entry in entries: url = entry["request"]["url"] headers = entry["request"]["headers"] # clean up driver.quit() server.stop()
In the example above, we’re creating a new HAR object before navigating to the webpage. After navigation, we can simply extract the request header information from the entry.
To wrap up, harvesting data in Selenium – specifically HTTP request headers – can be achieved via different techniques such as leveraging built-in browser network tools, pivoting on a proxy server like BrowserMob, or combining python and selenium alongside BrowserMob Proxy.Selenium WebDriver is an open-source tool that lets automation engineers drive a browser natively. While it provides a plethora of capabilities to interact with web elements, it doesn’t directly support grabbing HTTP response or request details like status code or headers. To achieve this, we often need to marry Selenium WebDriver with other tools.
One method to capture request headers while using Selenium WebDriver involves integrating a proxy server into your test configuration. One such tool for this purpose is BrowserMob Proxy. This open-source utility captures performance data about web pages like load times, header sizes and information among other things.
Below is a Python-based sample script demonstrating how you can incorporate BrowserMob Proxy with Selenium to capture request headers:
Installation:
BrowserMobProxy and its python client can be installed via pip:
pip install browsermob-proxy
Make sure you downloaded the latest version of BrowserMob Proxy and specify the path in your script.
Example script:
from browsermobproxy import Server from selenium import webdriver server = Server("path/to/browsermob-proxy") # specify the path to your BrowserMob Proxy server.start() proxy = server.create_proxy() chrome_options = webdriver.ChromeOptions() chrome_options.add_argument("--proxy-server={0}".format(proxy.proxy)) browser = webdriver.Chrome(chrome_options=chrome_options) proxy.new_har("google") browser.get("http://www.google.com") # Print all request headers for ent in proxy.har['log']['entries']: print(ent['request']['headers']) browser.quit() server.stop()
In the above example, we are starting BrowserMob Proxy, creating a new proxy, setting up ChromeDriver to use this proxy, and finally instructing the proxy to start capturing traffic. We open google.com and then print out all the request headers.
Using this approach, you are capable of troubleshooting header configuration potential issues effectively. Such issues could include: improper content-type being sent, missing authentication tokens, or a cache-control header incorrectly setup. Whatever the specific issue might be, these header details will form the basis of your investigation when debugging HTTP Header related tests. For advanced header configurations, you might also need to explore Selenium’s integration with other HTTP inspection tools.
An important thing to keep in mind, however, is that this approach adds complexity to your Selenium WebDriver setup and creates additional dependencies in the forms of the browsermob-proxy server, as well as extra considerations for compatibility and reliability of the proxy server. Therefore, it should not replace traditional application logging or network traffic analysis with tools targeted towards full-stack network troubleshooting, but only used as an aid for Selenium-specific scenarios.
For more details on how to leverage BrowserMob Proxy with Selenium WebDriver, please refer to the official BrowserMob Proxy website and Selenium documentation.Getting request headers in Selenium is vital to understanding how your web app interacts with different servers, be it for API calls, fetching resources, or any kind of network requests. The ‘request headers’ contain crucial HTTP context, which helps you infer things such as authentication tokens, the accepted data type, user-agent information etc.
To get the request headers in Selenium, we need to integrate it with a proxy server like BrowserMob-Proxy. We initialize the proxy server, start it and embed its details into DesiredCapabilities – a class used by Selenium WebDriver to let you set properties for the driver.
For example,
from browsermobproxy import Server server = Server("path/to/browsermob-proxy") server.start() proxy = server.create_proxy() from selenium import webdriver cap = webdriver.DesiredCapabilities.FIREFOX cap['proxy'] = {"httpProxy":proxy.proxy,"ftpProxy":proxy.proxy,"sslProxy":proxy.proxy,"noProxy":None,"proxyType":"MANUAL","class":"org.openqa.selenium.Proxy","autodetect":False} driver = webdriver.Firefox(capabilities=cap)
Here httpProxy, ftpProxy, sslProxy define the protocol-specific proxies. After setting up the proxy server, you can navigate to the required webpage making an HTTP request using Selenium-
driver.get("http://www.someurl.com")
Now coming to the main point of evaluating success after getting these request headers.
1. **Cross Verify with Expected Headers:** You already know what headers to expect from the documentation of the webpage or API service. Check these against what we fetched using Selenium. If they match (including their values), it’s a successful checkpoint.
2. **Specific Key-Value Pairs:** Make sure the headers contain specific keys that you deem important. For instance, ‘Content-Type’, ‘Authorization’ etc. may be some headers of interest to ensure the app is working correctly.
3. **No Unauthorized Response:** Monitor the response code associated with the request. A 200 OK response indicates success while a 403/401 might mean issues with the ‘Authorization’ header, indicating Authorization failures.
Above all these points, continuous evaluation and monitoring are essential to ensure ongoing success. There are many toolsGoogle DevTools Network Monitor for example, where you can examine request headers right in your browser for isolated test cases and dig deep if anything is seeming out-of-orders.
Remember, the real strength of this method comes from its flexibility to combine it with other elements of automated testing. Hence keep refining your way of assessing success as you keep mastering the art of extracting more & more useful information from the Request Headers.
In the realm of automated web testing, Selenium plays a dominant role with its wide array of functions and capabilities to emulate user interactions. However, obtaining request headers with Selenium alone doesn’t come straight forward, which brings about certain limitations on its efficiency. This hurdle can be most effectively overcome by using a combination of Selenium with BrowserMob Proxy or HAR (HTTP Archive format) which are special utility tools that interact well with Selenium and allow to access request and response headers.
Following the detailed steps below will help you get request headers in Selenium:
-
Start off by initiating the BrowserMob Proxy server and create a new instance of it. Assign this instance to your add-on or browser extension of choice.
-
Create a new DesiredCapabilities object, where you align the capabilities of Selenium WebDriver with that of your proxy server. Here’s an example:
DesiredCapabilities capabilities = new DesiredCapabilities();
capabilities.setCapability(CapabilityType.PROXY, seleniumProxy); -
Following this, instantiate your WebDriver with these set capabilities.
-
Now when you execute any action on your WebDriver like navigating to a URL, the actions get recorded in BrowserMob Proxy, enabling you to extract the request details from it which contains the headers.
-
Retrieve the request headers by converting the captured information into a more readable HAR file format or directly from your proxy if it is enabled.
The process might appear quite elaborate at first but getting hands-on experience will make it easier and open up many possibilities for advanced testing scenarios. Remember, there is always a trade-off between power and complexity while learning something new. Once mastered, this powerful technique to get request headers can enhance your overall testing capability and efficiency.
For better understanding and direct implementation, resources like
BrowserMob Official Documentation
and
Selenium Documentation
provide in-depth knowledge about how these tools work independently as well as together.