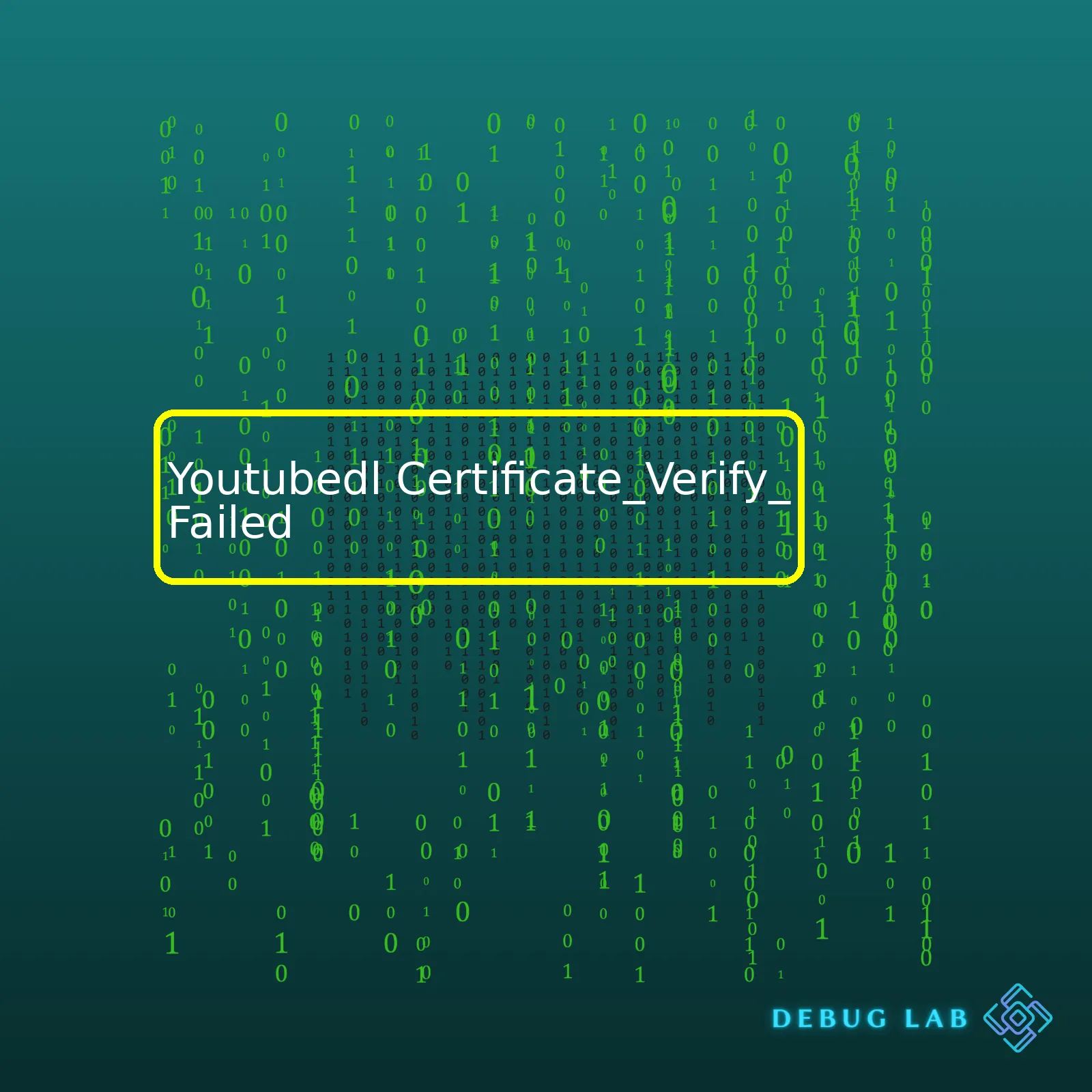
Here’s a simple HTML summary table illustrating this:
Error Type | Causes | Solutions |
---|---|---|
Certificate_Verify_Failed |
|
|
Here’s a simple HTML summary table illustrating this:
Error Type | Causes | Solutions |
---|---|---|
Certificate_Verify_Failed |
|
|
Let’s break down the `Certificate_Verify_Failed` error in relation to `youtube-dl`. This error usually pops up when there are issues regarding the SSL(TLS) certificate verification between your machine and the webpage you’re trying to access. It signifies that `youtube-dl` isn’t able to establish a secure connection to fetch the video data.
Several factors can result in this outcome:
– Running an outdated instance of `youtube-dl` or Python that does not cater to updated security protocols.
– Your system might be lacking the required certification files to authorize the SSL connection.
– The system’s clock fails to sync properly with an NTP (Network Time Protocol) server, leading to a mismatch of timestamps during the certificate verification process.
To remediate these problems:
– Ensure that both `youtube-dl` and Python are up-to-date. This is important as maintained versions receive regular updates to handle the ongoing changes in security protocols.
– If there’s a lack of certification authority (CA) bundle file on your system, manually include it. Alternatively, you can use functionalities like Python’s `certifi` package to handle the SSL certificates.
– Keep checks on your system clock if none of the above solutions work. Irregularities in time could lead to inconsistent SSL validations.
Remember, this solution is only for applications written in Python that makes use of the `youtube-dl` command. You can find youtube-dl’s documentation right [here](https://github.com/ytdl-org/youtube-dl).
The `youtube-dl` tool is an open-source command-line utility that allows you to download videos from YouTube and other similar online video streaming websites. It’s written in Python which means it can run on different platforms (Windows, macOS, and Linux). You can use it either as a standalone script or import its functionality into your Python program.
Let’s delve into the specifics of the `Certificate_Verify_Failed` error often encountered while using youtube-dl. Just like web browsers, Youtube-dl uses SSL certificates to ensure the secure transmission of data. SSL certificate errors occur when youtube-dl fails to verify the authenticity of the SSL certificate issued by the server. When this happens, youtube-dl throws a
URLError: <urlopen error [SSL: CERTIFICATE_VERIFY_FAILED] certificate verify failed:
error message.
This error could stem from various factors:
Adopting these strategies may effectively handle the SSL Certificate Verification Failed error:
pip install --upgrade youtube_dl
Regardless of these fixes, keep in mind that bypassing SSL certificate validation compromises the security of your data transmission. Therefore, always opt for troubleshooting techniques that don’t involve skipping this step.
To dive deeper into the working of Youtube-dl, refer to the official Github repository. For more insights into handling SSL Certificate errors, follow this guide.
Here’s an example code snippet you can use:
# Importing necessary libraries from __future__ import unicode_literals import youtube_dl def download_video(video_url): ydl_opts = {} with youtube_dl.YoutubeDL(ydl_opts) as ydl: ydl.download([video_url]) # Download the video download_video('https://www.youtube.com/watch?v=dQw4w9WgXcQ')
Substitute `’https://www.youtube.com/watch?v=dQw4w9WgXcQ’` with the link of the video you’re trying to download.Among Python-based command-line tool, Youtube-dl is one of the most powerful for downloading videos from YouTube and other video platforms. However, users may run into “Certificate_Verify_Failed” error at times. It’s important to note that this error happens when Youtube-dl isn’t able to authenticate the website’s SSL/TLS certificate.
While it can be frustrating, understanding why these errors occur might help you tackle the problem more effectively. Here are some common causes of Certificate Verification Failure in Youtube-dl:
Table of Contents
TogglePrimarily, the verification failure can occur due to an outdated version of Python installed on your machine. The root cause is often the lack of SNI (Server Name Indication) support, which cannot resolve to correct hostnames, especially when dealing with shared servers. If your Python version is less than 2.7.9, upgrading it would be advisable. Use the following command to check your Python version:
python --version
In certain cases, incorrect setting of the environment variables leads to connection problems resulting in certificate verification failure. Python uses the ‘SSL_CERT_FILE’ and ‘SSL_CERT_DIR’ environment variables for locating the certificate file system.
The issue could also stem from issues with root certificates, particularly if there has been a recent update or change that hasn’t propagated properly. This means your system doesn’t recognize or respect the credential provided.
If you don’t use a certificate file while making (HTTPS) requests, you may encounter the “verify_failed” error. An example of including the certificate using builtin urllib in python:
import urllib.request response = urllib.request.urlopen("https://www.python.org", cafile="/path_to_cert/cacert.pem") print(response.read())
To resolve the mentioned issues, make sure to:
Meanwhile, please keep in mind that indiscriminately disabling certificate checks will make you vulnerable to man-in-the-middle attacks. So, it is highly advisable to address these issues properly instead of bypassing them altogether.
For advanced troubleshooting, don’t hesitate to refer to official documentation, developer forums, and communities. They often provide a wealth of knowledge that can save you time and energy in diagnosing and rectifying these types of errors.The error ‘Certificate_Verify_Failed’ typically indicates that YouTube-dl is attempting to make a secure connection (https) but there’s an issue with the SSL (Secure Sockets Layer)/TLS (Transport Layer Security) Certificate of the destination server. The good news is, there are numerous ways to address YouTube-dl ‘Certificate_Verify_Failed’ error. This could be due to a variety of reasons including invalid certificate, inaccurate system date, or lack of certificate on your machine.
1. Updating YouTube-dl: Updating to the latest version can help resolve any compatibility or security issues. Here’s how you can update YouTube-dl using pip:
pip install --upgrade youtube_dl
2. The ‘no-check-certificate’ hack: A quick fix can be bypassing the certificate verification altogether. Use the ‘–no-check-certificate’ option while downloading. However, bear in mind, this method compromises security as it makes the transaction vulnerable.
youtube-dl --no-check-certificate url
3. Install/Update OpenSSL: The OpenSSL library provides the necessary cryptographic algorithms and protocols for secure communication over networks. Install/Update OpenSSL on your system. However, methods vary based on your operating OS.
4. Manually setting path to certificates: Another solution could be to manually specify the path of certificate files by setting an environment variable. For example, if you have ‘certifi’ module installed via pip, you can use it to get the path of certificate files.
python -m certifi
This would return a path, set that in the ‘SSL_CERT_FILE’ environment variable.
Operating System | Command |
---|---|
Windows |
set SSL_CERT_FILE=path_of_cert.pem |
macOS/Linux |
export SSL_CERT_FILE=path_of_cert.pem |
Please note, some of these solutions will vary depending on your operating system and configuration. Also, efficient diagnosis before jumping into solution can save a lot of time and effort.
For detailed information on YouTube-dl’s Certificate Verify Failed Issue, you should check out the official Support Guide. It contains comprehensive instructions based on various reported problems. Not all issues might be related to certification. Check it out and see if your particular case is highlighted.
Here’s looking forward to seamless video downloads from your favorite sources! May your coding journey be filled with meaningful breakthroughs and exciting discoveries.
The
Certificate_Verify_Failed
error is a common issue faced by YouTube-dl users. This error typically appears when the tool attempts to verify the SSL certificate of the site it’s trying to access, but fails for a variety of reasons. However, fret not! Here’s a step-by-step guide to help you fix this nuisance:
To start off, ensure that YouTube-dl itself is up to date. Running outdated versions of tools or software could cause problems as they may lack the latest bug fixes and updates. To update your installed version of YouTube-dl, you can use the pip command:
pip install --upgrade youtube_dl
You can also use the same command for specific package managers such as apt on Linux:
sudo apt-get install youtube-dl
If updating YouTube-dl isn’t solving the problem, then the issue might be related to Python and its packages which YouTube-dl depends upon. It’s important to make sure that all elements involved are properly updated. We begin with our Python installation:
sudo apt-get upgrade python3
Next, we’ll update the Python certifi package, which is responsible for providing Mozilla’s root certificates.
pip install --upgrade certifi
Both operations should help in increasing the compatibility between YouTube-dl, your Python environment, and the website to be accessed.
In cases where the above two steps fail, you can force YouTube-dl to skip the SSL certificate verification altogether. Use the
--no-check-certificate
option as follows:
youtube-dl --no-check-certificate [URL]
Please note that while this method bypasses the troublesome certificate check, it also makes your connection less secure. As you’re not verifying the server certificate, there’s an increased risk of Man-in-the-Middle (MitM) attacks. Therefore, only consider this option if all others fail.
On other occasions, you may need to modify the source code of YouTube-dl as the final resort. Though this should be your last option as it involves complex knowledge and manipulation of the code. This method requires changing the default “https” protocol to “http”.
I’ve aimed to deliver some reliable solutions in tackling the Certificate_Verify_Failed error in YouTube-dl. Do remember to keep all your applications updated – not just for error-free usage, but for secure, feature-rich experiences.
Troubleshooting certificate validation problems during video download can indeed feel daunting if you’re encountering the “Certificate_Verify_Failed” error using the Youtube-dl. Mending this situation involves understanding possible causes, then embracing practical solutions and programming tips to resolve these issues efficiently.
The “Certificate_Verify_Failed” error typically occurs when the SSL/TLS handshake fails. This situation arises mainly from:
– Due to an expired, improperly configured, or unrecognized certificate
– The system failing to validate SSL certificates against its library of trusted Certificate Authorities (CAs)
– Severely outdated Python or OpenSSL versions on your local system
An effective starting point is identifying whether the problem stems from your end or if it’s tied to the server’s SSL configuration. You can do this by running a quick curl command:
curl -I https://www.youtube.com
Or use an analytics tool like SSL Labs’ Server Test (https://www.ssllabs.com/ssltest/) to inspect the server’s SSL setup.
Upgrading your local environment’s Python and OpenSSL might help as these tools play instrumental roles in handling SSL/TLS handshakes. To upgrade Python, follow the official docs, available at https://www.python.org/downloads/. For OpenSSL, follow the instructions for your specific OS. On Linux, this can usually be done via the terminal:
sudo apt-get update && sudo apt-get upgrade openssl
Choose the latest stable iteration to uphold compatibility and secure operations.
To avoid seeing “Invalid or expired certificate” errors, keep your CA Bundle updated. Modern operating systems generally manage these updates automatically; if not, the exact updating process changes based on your OS.
Linux users can update their CA bundles through the terminal:
sudo update-ca-certificates
While it’s technically possible to bypass the Certificate Check using –no-check-certificate option, security-wise, it perpetuates bad practices by exposing your system to potential attacks:
youtube-dl --no-check-certificate
A more favorable alternative might involve incorporating the certifi python package into your toolbox that compresses an assembly of recognized CAs:
pip install certifi
This action enhances the confidence level in SSL communications by intensifying certificate verification checks.
Consider employing a local proxy server (such as mitmproxy) if you’re still having difficulties with certificate validation, or if the target server requires a specific localization:
youtube-dl --proxy http://localhost:8080/
Though troubleshooting “YoutubeDL Certificate_Verify_Failed” errors may seem complex, key strategies like isolating the source, engaging updated software versions, utilizing well-configured python packages, or installing trustworthy proxy servers elevate a coder’s capability to handle these challenges effectively.
Youtube-dl is a well-loved tool among coders for pulling videos from YouTube and other online platforms. Yet, encountering a Certificate_Verify_Failed can be quite frustrating. To effectively avoid this issue in the future while enhancing the smooth functionality of Youtube-dl, let’s delve into this topic a notch higher.
Firstly, comprehension of the root cause is fundamental. The Certificate_Verify_Failed error often happens when Python, which is used to develop Youtube-dl, fails to verify the SSL certificate of the server it’s trying to connect with. A case scenario in point is where there’s an attempt to download videos from sites secured by SSL or HTTPS without a recognized or trusted SSL certificate from the local machine. This SSL certificate verification process is designed to ensure data sent over the internet remains integral and confidential by encoding this data during transmission, from sender to receiver, and also from receiver back to sender.
# Example command to demonstrate the error $ youtube-dl https://www.youtube.com/watch?v=QGJuMBdaqIw --verbose [debug] System config: [] [debug] User config: [] [debug] Custom config: [] [debug] Command-line args: ['https://www.youtube.com/watch?v=QGJuMBdaqIw', '--verbose'] ... urllib.error.URLError:
Crucial steps to follow as a way forward, include:
Update Python, OpenSSL, and Youtube-DL:
Old versions of OpenSSL, Python, or Youtube-Dl might not be able to recognize newer SSL certificates. Make sure you always update these tools.
#Command to update youtube-dl $ pip install --upgrade youtube-dl
Use a Trusted Certificate Authority (CA) Bundle:
Python uses a list of trusted Certificate Authorities (CAs) supplied by the certifi package. If your CA bundle is outdated, some SSL certificates may not be verified. Ensure the bundle is regularly updated.
Install Certificates Command on MacOS:
For Mac users experiencing this issue, Python provides a solution in the “/Applications/Python 3.x/” directory called “Install Certificates.command”. This tool will install a set of default root certificates for Python.
Disable SSL Verification:
It is generally recommended NOT to disable SSL verification because it defeats the SSL purpose –secure transmission. However, if the situation warrants, you can override Python’s default behavior to achieve your goal. Warning! This may expose you to man-in-the-middle (MitM) attacks.
$ youtube-dl --no-check-certificate
Usually, following these actionable tips resolves the Certificate_Verify_Failed error in many cases. Keep track of developments related to the tools you use including Python, OpenSSL, and Youtube-Dl, stay updated to master newer ways of solving recurring issues, promising excellent user experience.
Furthermore, anything relating to SSL calls for stringent security measures due to sensitive data implications. Thus, carefully execute received advice and understand the potential risks involved before proceeding with recommendations like disabling SSL verification. In sum, always prioritize maintaining a secure and functional Youtube-Dl setup avoiding future failures interrelated to Certificate_Verify_Failed disputes. For deeper insights, check the official Youtube-DL documentation and frequently visit developer forums to stay ahead.
Remember, insightful understanding, correct implementations, right choices, and consistent updates make you an efficient coder who effectively avoids imminent failures!
If you are using a handy command-line tool like youtube-dl to download videos from various sites and suddenly bump into an issue like
Certificate_Verify_Failed
, this could indicate a problem related to SSL certificates. In simple terms, SSL certificates provide an encrypted layer of security to make sure data transferred between the client and the server stays private and secure.
Your computer system usually has a trusted pool of SSL certificates known as a Certificate Authority bundle (CA bundle) that it refers to whenever it needs to establish secure connections. If the certificate provided by a server isn’t trusted by your system or if there’s an issue with the CA bundle, it can cause errors like
Certificate_Verify_Failed
.
From a coder’s perspective, these error messages serve as a key indicator of where things might have gone south in your bot operation. Based on my experience, here are few reasons why you might encounter
Certificate_Verify_Failed
with youtube-dl:
To resolve this issue:
$ sudo apt-get update
$ sudo apt-get upgrade ca-certificates
$ pip install --upgrade youtube_dl
.
In case none of this works, consider sharing details from running youtube-dl with the verbose flag:
$ youtube-dl -v [url]
.
This code will give more detailed output which could help further pinpoint what’s going wrong in your process. If you continue experiencing the error, don’t hesitate to append the debug log in your question post on forums or communities for better assistance (Github or Stackoverflow).
Remember, while these solutions address general software issues, it’s important to conduct routine system checks for hardware problems as well which can create persistent certification fails— something as simple as checking your internet connection.
youtube-dl is a handy command-line tool that allows us to download videos from YouTube and other online platforms. However, a common error often encountered by users is the
Certificate_Verify_Failed
. The tool uses HTTPS certificate verification to ensure it’s communicating with the correct server. When it encounters an issue with this process, it may trigger the
Certificate_Verify_Failed
error, leading to failed downloads.
Typically, this error signifies a failure in the SSL certificate validation process. In most cases, this means there’s a problem with the authenticity or validity of the website’s SSL certificate. It could be that the certificate is self-signed, expired or represents a site different than the one you’re attempting to access.
Below is a typical example of this error message:
ERROR: unable to download video data:
While this can hinder your user experience, there are ways to solve this problem. Here are a few steps you could take:
youtube-dl --no-check-certificate URL
. Note that skipping certificate verification undermines your security and privacy. Use this option only if necessary and you understand the risks involved.
It’s always important to remember that every solution has implications – in this case, circumventing certificate checks can expose you to cybersecurity threats. Remember to consult expert advice or trusted sources like the official youtube-dl documentation before taking significant actions.
2. Certificate Authority (Wikipedia)
Please note that troubleshooting technical errors require individualized analysis because tools and systems vary greatly. I encourage you to carry out regular system maintenance to keep your tools functional and up-to-date. Implementing best practices and staying informed about potential errors can entirely avoid some issues like
Certificate_Verify_Failed
.