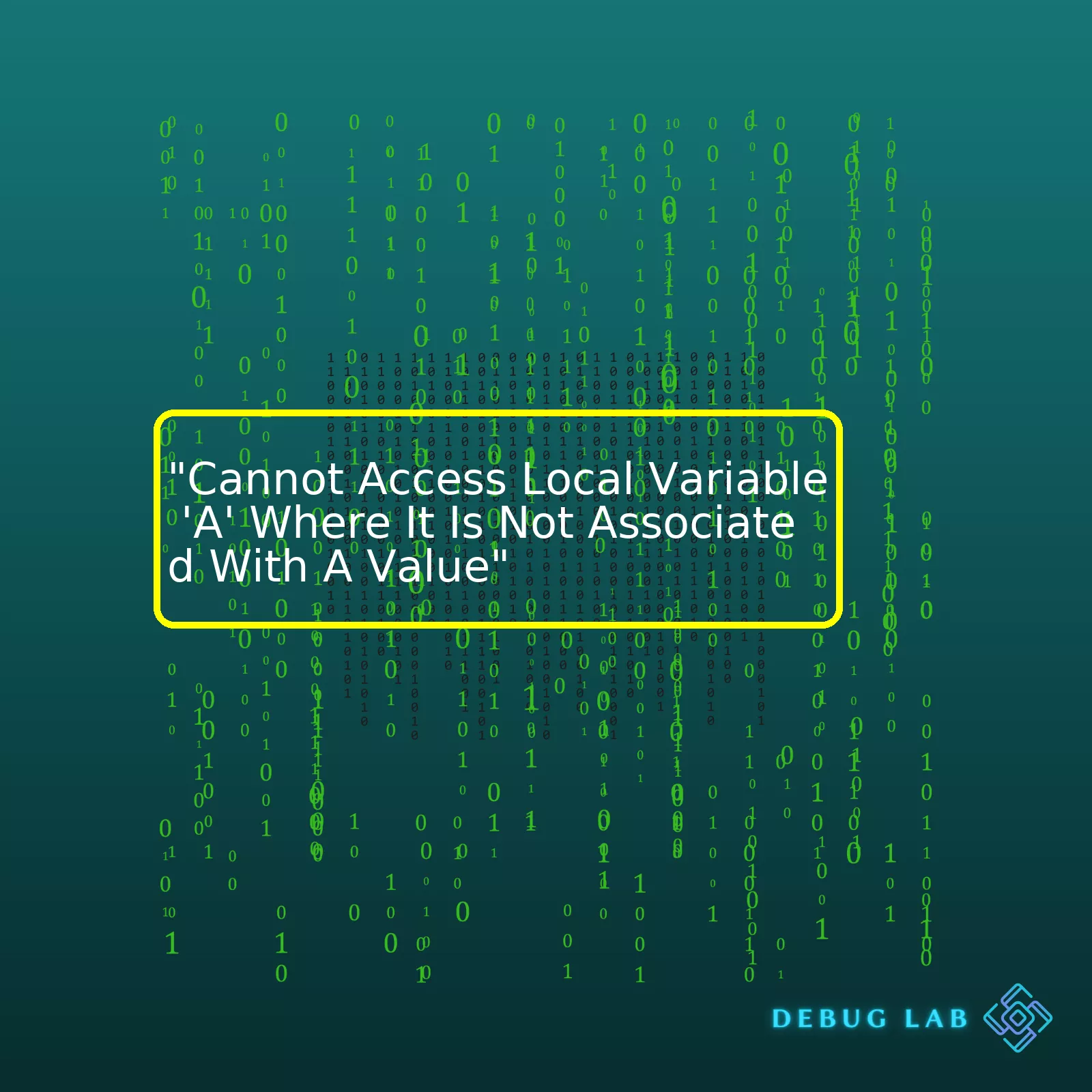
Error Type | Description | Common Causes | Solutions |
---|---|---|---|
"Cannot Access Local Variable 'A' | An error that occurs when a program tries to use a variable not assigned any value. | Declaring a local variable without assigning it a value, then attempting to use it. | Ensure all local variables are initialized with a value. |
Error Type
Description
Common Causes
Solutions
"Cannot Access Local Variable 'A'
An error that occurs when a program tries to use a variable not assigned any value.
Declaring a local variable without assigning it a value, then attempting to use it.
Ensure all local variables are initialized with a value.
The issue of “Cannot Access Local Variable ‘A’ Where It Is Not Associated With A Value” is quite a common one amongst programmers, especially those who are either novice or have recently switched from a different programming paradigm. In essence, it pertains to a situation where a local variable, in this case referred to as ‘A’, has been referenced in the code but is not associated with any value.
In most programming languages, trying to access an unassigned local variable triggers a compiler or runtime error. This would include languages like C#, Java, and Python, among others. They don’t accept usage of unassigned local variables mainly because it helps prevent certain types of programming errors by ensuring that a variable always holds a valid value before it’s used. For example, in JavaScript, if you try to use a variable that hasn’t been declared yet, it will simply evaluate to ‘undefined’, but in C# it will give out an error.
Common causes of the error include declaring a variable inside a function but failing to assign it a value before using it, or using a variable outside its scope. The obvious way out of this predicament is to ensure that every time you declare a variable, you should assign a value to it within its usable scope. Doing so will avoid any such issues, allowing your code to execute smoothly without returning any exceptions, hence resulting in more predictable and reliable software.
Analyzing your code for uninitialized variables and correcting them can be tedious at times, most IDEs (Integrated Development Environment) provide built-in tools to help detect these kinds of problems before even executing your code. For instance, Visual Studio gives a compile-time error whenever it finds an uninitialized local variable.
In short, remember: Always initialize your local variables, and ensure that you’re using them within their appropriate scope.
As a seasoned developer, I’ve often found myself debugging through cryptic error messages. One among them is the infamous “Cannot Access Local Variable ‘A'” message. When we dive deeper into this error, it generally implies that a particular variable defined within a function or a block of code doesn’t hold any associated value or it’s trying to be used outside the scope it was defined in.
Understanding Local Variables
Before getting to the root of the problem, let’s take a quick review of what local variables are again or refresh our memory. In the world of coding –
- Local variables are those which are defined within a function or a block.
- They can only be accessed and manipulated within the same function or block where they’re declared.
- If you attempt to access them outside their defined scope, you’ll encounter an error.
Analogy
A handy way to understand this concept is by drawing comparisons with real-world situations. Think of it like your house – if you leave something inside your house (let’s say your phone), it’s still there when you go back in. But when you’re outside, you cannot just reach out and use it. The thing here is that unless spatial constraints are defied, anything kept within a certain boundary stays in there, and its interaction from outside is limited.
Code Sample
In Python, for example, accessing a local variable outside its scope would look like this:
xxxxxxxxxx
def my_function():
a = 10
print(a)
Running this code leads to an error since ‘a’ is not defined in the main program but only inside the ‘my_function’ function.
Causes And Solutions
To move forward, we need to trace two particularly common reasons why you’re seeing the ‘Cannot Access Local Variable ‘A’ error:
- The variable hasn’t been assigned any value yet: If you try to access a local variable without assigning any value to it beforehand, you’ll meet this error. Check thoroughly if the variable is equipped with a value before it’s called in your code.
- The variable is being accessed outside its scope: As mentioned above, local variables can only be accessed within their defined scope. Make sure you aren’t accessing the variable outside the function or block it was defined in.
A solution to the first scenario might look like this:
xxxxxxxxxx
def my_function():
a = 10
print(a)
my_function()
This ensures that you’re accessing ‘a’ after it has been assigned a value and within the same function where it was defined, dodging both issues that cause the error.
It’s important to remember that every language handles scoping differently, so you must refer to its specific documentation for rules pertaining to variable handling.
Please note: Avoiding these pitfalls will save you a lot of time and frustration. So, always make sure to declare variables within the correct scope, assign them values before accessing, and keep track of where all you’ve referred to them in the code.
Sure, let’s decipher this!
If you’re getting an error like
xxxxxxxxxx
"Cannot Access Local Variable 'A' Where It Is Not Associated With A Value"
, it implies that your Python code is attempting to use a local variable that has not been given a value within the scope in which it is being called.
Scopes and variables are significant aspects of any programming language. It’s important to understand that the scope of a variable defines its accessibility or visibility from different parts of code.
Python’s scopes are implemented as dictionaries that map variable names to the objects they refer to. These dictionaries stacked approximately as:
– The innermost scope has local variables and free variables from inner functions.
– The scopes of enclosing functions, which are searched starting with the nearest enclosing one,
– The next-to-last scope is the module’s globals,
– The outermost scope is a namespace that contains built-in names.
To back up a little bit, let’s discuss a few fundamental concepts:
1. Local Variables:
Local variables only exist within the function in which they are declared. Once the function is completed, these variables cease to exist.
Let’s consider an example:
xxxxxxxxxx
def func():
a = 10
print(a)
func()
print(a)
In the above code, if you attempt to invoke
xxxxxxxxxx
print(a)
outside the function (
xxxxxxxxxx
func()
), you’d receive an error stating that ‘a’ is not defined. This is due to ‘a’ being a local variable to the function and thus, it doesn’t exist outside
xxxxxxxxxx
func()
.
2. Global Variables:
On the contrary, global variables are available throughout your entire code body. This means they can be accessed both inside and outside of functions. Here’s an illustrative piece of code:
xxxxxxxxxx
a = 10 #Global variable
def func():
print(a)
func()
print(a)
Running this piece of code won’t spark any errors because ‘a’ is globally accessible to both
xxxxxxxxxx
func()
and the space outside
xxxxxxxxxx
func()
.
Getting back to your question: Cannot Access Local Variable ‘A’ Where It Is Not Associated With A Value – There could be two potential reasons:
1. Out Of Scope:
It might be that the variable ‘A’ is local to another region where it’s been defined (like in a different function or method), and you’re trying to access it in a place where it isn’t visible or accessible as per its scope.
2. No Variable Initialization:
The more likely trigger for this error is that you might have forgotten to initialize the variable ‘A’, yet you’re aiming to use it in your python code.
Imagine having a recipe that calls for eggs, but you forgot to buy them at the store. You can’t commence the recipe because you missed a prime ingredient. Similarly, if ‘A’ does not have a preceding value assigned to it, making an attempt to access it will result in an error.
For further reads on these concepts, I recommend you check out the following hyperlinks:
– Understanding Python Scope
– Python Global Variables
From this in-depth probe, it’s essential to note that handling variables carefully by understanding their scopes can prevent many bugs while writing python scripts!One of the commonly faced issues in programming is the error message “Cannot access local variable ‘A’ where it is not associated with a value”. This error usually means that you’re attempting to use a local variable “A” before assigning it a value. In many programming languages, declaring a variable doesn’t automatically associate it with a value.
Here, let’s break down some possible causes and how to address them:
Using Variable Before Initialization
This issue most commonly arises when a declared variable is used before it’s been initialized with a value.
For example, in Python, if we declare a variable
xxxxxxxxxx
a
and try to print it without initializing, it will lead to an error:
xxxxxxxxxx
a
print(a)
To resolve this issue, initialize your variable with a value prior to referencing it:
xxxxxxxxxx
a = 10
print(a)
Variable Scope
If using a locally declared variable outside its scope can result in this error. The rules for variable scoping vary across languages.
In C#, for example:
xxxxxxxxxx
{
int a = 10; // Local scope
}
Console.WriteLine(a); // Outside the scope
The solution would be ensuring that the variable is in the same or broader scope where it’s being referenced, or passing the variable through as a parameter, if applicable.
Branching Structures
Within branching structures (like if-else or switch-case statements), usage of uninitialized variables may go unnoticed until runtime.
Consider this C# snippet:
xxxxxxxxxx
int a;
if(condition)
{
a = 10;
}
Console.WriteLine(a);
The problem arises when
xxxxxxxxxx
condition
doesn’t evaluate to true, leaving
xxxxxxxxxx
a
uninitialized.
To fix such issues, always see to it that all branches assign a value to any variable before its downstream references.
Data Type Mismatch
While this wouldn’t necessarily produce the “variable not associated with value” message, it can similarly halt execution. Variables must be initialized with values meaningful within their declared type contexts. Incorrect data type assignments could potentially lead to this issue.
For corrections, ensure you are respecting each variable’s data type during assignments.
Error | Possibly caused by | Solution |
---|---|---|
“Cannot access local variable A where it is not associated with a value” | Using variable before initialization | Initialize your variable with a value prior to its reference. |
“Cannot access local variable A where it is not associated with a value” | Variable Scope | Ensure the variable is in the same or broader scope where it’s being referenced, or pass the variable through as a parameter, if applicable. |
“Cannot access local variable A where it is not associated with a value” | Branching Structure | Ensure all branches assign a value to any variable before its downstream references. |
“Cannot access local variable A where it is not associated with a value” | Data Type Mismatch | Make sure you are respecting each variable’s data type during assignments. |
Remember to regularly check your code integrity by running tests and handling exceptions properly. Understanding the root of these errors promotes cleaner, tighter code and leverages compiler error messages towards faster troubleshooting.
Link: “Use of unassigned local variable – Stack Overflow” provides more context about errors related to the use of uninitialized local variables.It seems like you’re hitting an error something along these lines: “Cannot Access Local Variable ‘A’ Where It Is Not Associated With A Value”. Simply put, this error means that your variable has been declared but not given any value. This makes it undefined and therefore inaccessible. In programming terms, we refer to these kinds of variables as uninitialized.
Now, let’s look into some debugging techniques we can apply to fix such errors:
Use default values: One simple remedy is assigning a default value when declaring the variable. For example:
xxxxxxxxxx
int a = 0;
This not only declares “a” as an integer but also initializes it with value “0”, which ensures our variable is always associated with a value.
Be aware of control flows: Quite commonly, this error occurs due to the structure of different control flows in your code, particularly conditional (if-else) statements. Often, a programmer might declare a variable inside one block of an if-else statement. This makes the variable accessible only within that specific block. If you try to use the variable outside this block, it may not have been assigned a value yet and throw the TypeError.
For instance:
xxxxxxxxxx
int a;
if (someCondition)
{
a = 10;
}
// Here "a" could still be uninitialized
In such situations, ensure that all paths through your code will initialize the variable, or consider declaring and initializing the variable before entering the control flow.
Using ‘out’ or ‘ref’ parameters correctly: If you’re coding in a language like C#, you may face this error when trying to use ‘out’ or ‘ref’ parameters without properly initializing them.
If you’re dealing with ‘ref’ parameters, make sure that the variable has been initialized before it is passed as a method argument:
xxxxxxxxxx
void TestMethod(ref int b)
{
...
}
int a = 0;
TestMethod(ref a);
If you’re handling ‘out’ parameters, ensure the variable is assigned a value inside the method it gets passed to:
xxxxxxxxxx
void TestMethod(out int b)
{
b = 10;
...
}
int a;
TestMethod(out a);
Analyze compiler warnings: Most modern compilers are pretty smart and they provide warnings about possible problems in your code even before they develop into runtime errors. Analyze compiler warnings and messages as they can give you important hints about uninitialized variables.
Note: Even though JavaScript and certain other languages allow use of uninitialized variables and auto-initialize them as ‘undefined’, it’s a common best practice across multiple programming languages to always initialize your variables.
There’s another important note to be made here; these debugging techniques are diagnostic practices. They are intended to help identify and consequently fix issues regarding uninitialized variables. If used appropriately, they’ll guide you towards producing clean, working code efficiently. I hope these methods provide you with the practical knowledge required to tackle the issue at hand! If you have further queries or concerns, don’t hesitate to ask!One common error that Python developers face is the “UnboundLocalError”. This occurs when you try to reference a local variable before it has been assigned any value. Simply, Python can’t find anything associated with the name you’re trying to access.
Python’s execution order matters, as the language reads code from top to bottom. If you attempt to call a local variable before defining it, you may receive an error like this:
xxxxxxxxxx
UnboundLocalError: local variable 'a' referenced before assignment
To understand it better, let’s look at an example:
xxxxxxxxxx
def example():
print(a)
a = "Hello, world!"
example()
In this case, variable “a” was called by the print function before its assignment, leading to UnboundLocalError. A straightforward check for troubleshooting this issue is to ensure that all variables are assigned a value before they are accessed in your code. For instance, this could be fixed by simply moving the variable assignment above the print statement:
xxxxxxxxxx
def example():
a = "Hello, world!"
print(a)
example()
There are other scenarios where UnboundLocalError can appear, such as:
– Changing a global variable in a function (fix by using the `global` keyword before the variable).
– Assigning a value inside an if statement but accessing it outside of it, and the conditional returns False (solution: put a broader scope for the variable assignment).
Python uses something called namespaces to keep track of variables. Namespaces can be thought of as containers of names. In contexts like functions, a new namespace (a.k.a local namespace) is created isolate variables that live inside that specific context. For global variables, Python uses what is referred to as the global namespace.
Let’s see an example where UnboundLocalError arises due to manipulation of a global variable in a function:
xxxxxxxxxx
a = "Hello, world!"
def change_a():
a = "Goodbye, world!"
change_a()
print(a)
The function
xxxxxxxxxx
change_a()
raised an error because Python treats variable
xxxxxxxxxx
a
as a local variable, since an assignment operation on it is performed within the function’s local namespace scope.
We can resolve this by declaring
xxxxxxxxxx
a
as a global inside the function block:
xxxxxxxxxx
a = "Hello, world!"
def change_a():
global a
a = "Goodbye, world!"
change_a()
print(a) # prints 'Goodbye, world!'
While Python provides helpful mechanisms like local scopes and the `global` statement for handling variable assignments, the best approach is always to ensure your program’s logic assigns and references variables correctly. Remember, understanding a language’s rules and intricacies is key to writing clear, concise, and error-free code.The “Cannot access local variable ‘A’ where it is not associated with a value” error in Python usually arises when you are trying to use a variable before it has been assigned a value. This can occur for several reasons, including if the variable is only defined under certain conditions, or it is initialized after being called.
Here are some best practices to avoid such an error:
1. Always Initialize Your Variables:
Initializing your variables before using them is the basic habit you should incorporate into your coding routine. Not doing this might lead you to face the “Cannot access local variable” error. For instance,
xxxxxxxxxx
def function():
print(a) # Here, 'a' is not yet defined
a = 5 # 'a' should be defined before usage
function()
You should define `a` before using it in your print statement.
xxxxxxxxxx
def function():
a = 5 # Now, here 'a' is defined before usage
print(a)
function()
2. Ensure Variables Are Defined In All Control Flow Paths:
Certain situations may demand defining your variable within conditional blocks such as “if-else” statements. In these cases, make sure ‘a’ is defined in all possible control paths.
For example, this will raise an error,
xxxxxxxxxx
def function(input):
if input == 1:
a = 5
print(a)
function(0)
To resolve, ensure ‘a’ is defined in all control flow paths,
xxxxxxxxxx
def function(input):
if input == 1:
a = 5
else:
a = 0
print(a)
function(0)
3. Avoid Using Local Variables Globally:
In Python, a variable declared inside a function (local variable) has scope only within that function. Hence, trying to access it globally will raise errors.
For instance, this code is problematic:
xxxxxxxxxx
def function():
a = 10
print(a)
To resolve, you can return ‘a’ from your function and then use its value.
xxxxxxxxxx
def function():
a = 10
return a
print(function())
Or alternatively, you could define a global variable:
xxxxxxxxxx
a = 10
def function():
global a
a = 20
print(a)
function()
print(a)
Following these practices can help prevent the “Cannot Access Local Variable Where It Is Not Associated With A Value” error. These examples and tips provide a broad guideline for configuring the variable scopes correctly and should equip you with the appropriate knowledge to handle such errors during your Python development. You can find more information about variable scopes and error handling in Python on websites like Python Documentation.
When dealing with scope-related problems in programming, like the error “Cannot Access Local Variable ‘A’ Where It Is Not Associated With A Value”, there are several alternative approaches that could help you to overcome such an issue. We understand that scoping rules can vary from one programming language to another. For the purpose of this discussion, let’s focus on the C# programming language.
Consider the following piece of code:
xxxxxxxxxx
public void SomeMethod()
{
int a = 10;
{
int a;
a = 20;
Console.WriteLine(a);
}
Console.WriteLine(a);
}
This code will return a compile-time error stating “A local or parameter named ‘a’ is already defined in this scope”. Here, the variable a is declared twice in the same method with one inside nested scope and one in enclosing scope. The problem is because we’re trying to redeclare it within the nested scope which is causing the conflict.
Alternative Approach One: Unique Variable Names
One straightforward approach to solving this issue would be using unique names for each variable.
xxxxxxxxxx
public void SomeMethod()
{
int a = 10;
{
int b;
b = 20;
Console.WriteLine(b);
}
Console.WriteLine(a);
}
In this updated version of the code, we’ve renamed the second declaration to ‘b’ instead of ‘a’, effectively nullifying the conflict.
Alternative Approach Two: Using Different Types for your variables
Another approach could consist of using different types for your variables. Variables with different data types even if they share the same name, won’t conflict.
xxxxxxxxxx
public void SomeMethod()
{
int a = 10;
{
string a;
a = "20";
Console.WriteLine(a);
}
Console.WriteLine(a);
}
In this instance, the two ‘a’ variables don’t conflict because one is of type ‘int’ and the other is of type ‘string’. But you need to remember these are treated as completely different variables.
Alternative Approach Three: Properly managing scopes
Properly managing scopes also plays a crucial part in avoiding these issues. As provided in this Microsoft documentation, Local functions (functions defined inside other methods) are a way to define and call methods privately; they can access local variables, which helps to control variable’s accessibility and visibility.
xxxxxxxxxx
public void SomeMethod()
{
int a = 10;
void InnerMethod()
{
int a;
a = 20;
Console.WriteLine(a);
}
InnerMethod();
Console.WriteLine(a);
}
In this case, the use of an inner function entirely isolates the inner ‘a’ variable from the outer one, thus achieving our goal of having two ‘a’ variables without a scoping error.
These three alternatives highlight the flexibility offered by C# when dealing with scope-related issues. You can choose an option based on your requirement or preferences while working on a project. When confronted with any error in your coding journey, consider understanding it deeply rather than quickly jumping into debugging it, and I’m sure you’ll discover many new insights about programming every single time.When dealing with the issue of “Cannot access local variable ‘A’ where it is not associated with a value”, one must remember that scope plays a significant part in this type of error. As with any coding language, Python treats things inside functions as local unless otherwise specified.
For instance, consider this erroneous piece of code:
xxxxxxxxxx
def local_test():
print(A)
local_test()
In the above example, you may expect to find a global variable A which isn’t been declared, hence Python sends an error message: “UnboundLocalError: local variable ‘A’ referenced before assignment.”
Conversely, should the variable have been defined within the same function, such as demonstrated here:
xxxxxxxxxx
def local_test():
A = 10
print(A)
local_test()
The code now works perfectly due to the fact that the variable ‘A’ is known within the function’s scope.
There are several ways we can resolve this issue:
Method 1: Declare your variable outside of your function or class
xxxxxxxxxx
A = 10
def local_test():
print(A)
local_test() # outputs: 10
Here the variable ‘A’ has been declared globally making it accessible anywhere within your code, including inside of functions.
Method 2: Use a return statement in your functions
xxxxxxxxxx
def local_test():
A = 10
return A
print(local_test()) # outputs: 10
Returning ‘A’ ensures that even though it is a local variable to the function, its value can still be accessed outside the function by calling the function itself.
These examples demonstrate how the scope of a variable might affect its accessibility and also guide developers to fix such errors.
If you would like to learn more about variable scope in python, this is a great article to start with.