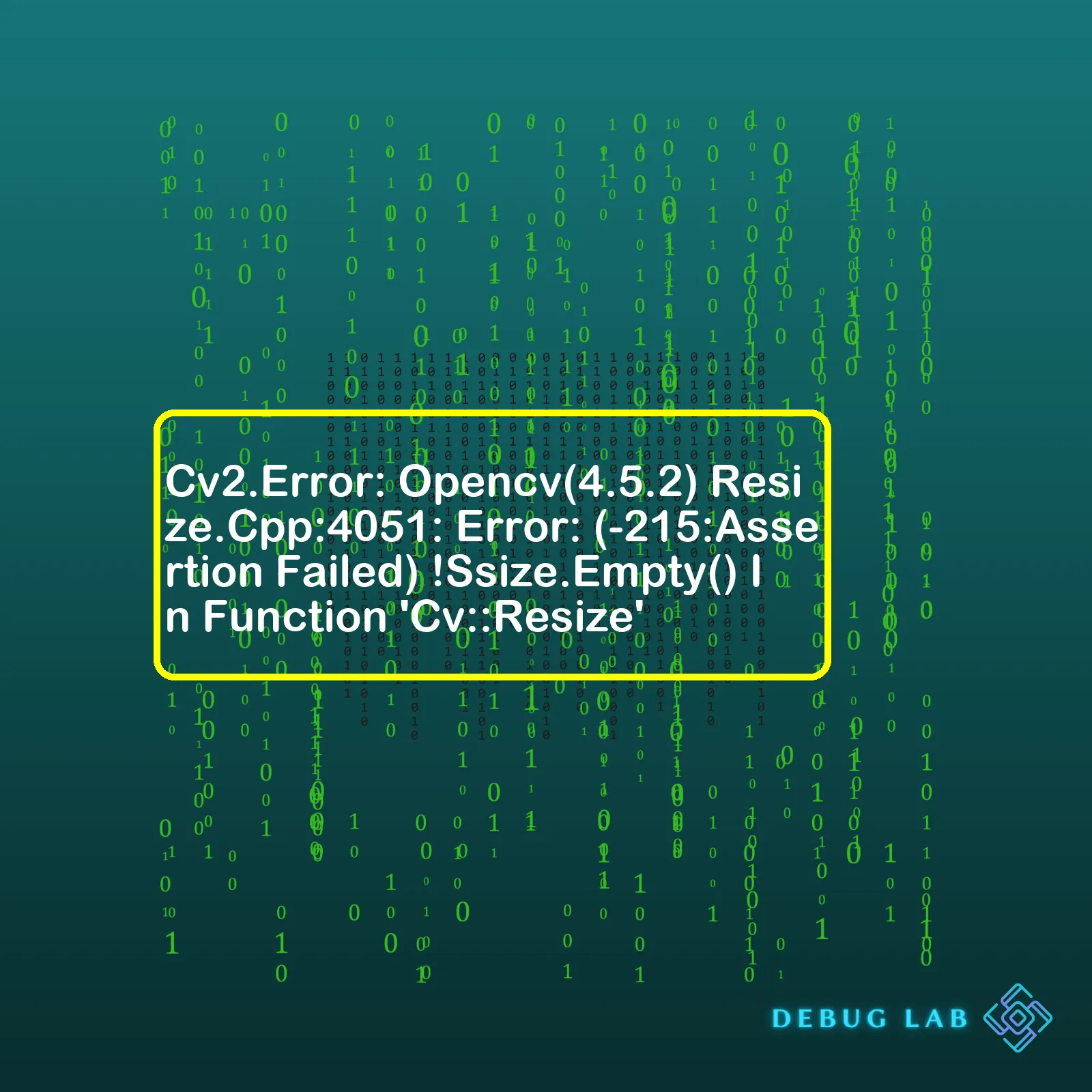
Error | File | Description |
---|---|---|
Cv2.Error: OpenCV(4.5.2) | Resize.cpp:4051 | This is an error indicating that the size parameter passed to the resize function is empty. |
This error typically occurs when the Cv2 `resize()` function in OpenCV receives an empty array or an array of size zero. This could be due to an incorrect image path, resulting in the function trying to process an inexistent image, thus leading to this error. It’s also possible that an operation prior to `resize()` may have led to unexpected empty results. For example, you are attempting to read an image file that does not exist or the path breaking due to platform differences (Windows uses backslashes, while Unix-based systems use forward slashes).
Here’s an empirical way you could avoid the problem:
import cv2 image_path = "" image = cv2.imread(image_path) if image is None: print("Could not read image.") else: resized_image = cv2.resize(image, (100, 100))
In this code block, we first attempt to read the image at `image_path` using `cv2.imread()`. If the image is unreadable or the file doesn’t exist, the method returns `None`. We check for this condition and print an error message, ensuring a non-empty image is handed off to cv2.resize().
For understanding more about working with images I suggest heading over to the Official OpenCV Python Tutorials. Remember to always verify your inputs before passing them into functions to avoid potential runtime errors and breakdowns.
Understanding and rectifying the Cv2.Error: Resize.Cpp:4051 in OpenCV involves a thorough comprehension of OpenCV’s resize method and its required conditions.
OpenCV (Open Source Computer Vision Library) is an open-source computer vision and machine learning software library that contains more than 2500 optimized algorithms.source It’s used for processing images and videos to detect and recognize faces, identify objects, classify human actions, track camera movements, extract 3D models, and much more.
Prior to diving into the error itself, let’s first figure out what the “resize” function does.
Resizing an image can be achieved using the OpenCV function `cv2.resize()`. The syntax of this function is as follows:
res = cv2.resize(img, dsize[, dst[, fx[, fy[, interpolation]]]])
Arguments:
– img: [required] source/input image
– dsize: [required] desired size for the output image
– fx: [optional] scale factor along the horizontal axis
– fy: [optional] scale factor along the vertical axis
– interpolation: [optional] flag that takes one of the methods
The cv2.error: Opencv(4.5.2) resize.cpp:4051 corresponds to an Assertion error, stating that !Ssize.empty() in function ‘cv::resize’. This essentially means that the source image which should not be empty, as per OpenCV’s required conditions for the resize function, is unfortunately empty. An image file could be empty due to various reasons like Incorrect path, Invalid file, or lack of proper read permissions.source
To fix this error we need to ensure the source image path is valid and the image is loaded correctly before executing the cv2.resize() operation on it. Let’s illustrate this with a Python code snippet.
import cv2 image_path = '/path/to/image.jpg' # Use assertion to check if the image path exists assert os.path.exists(image_path), 'Image path not found!' # Read the image img = cv2.imread(image_path) # Assert that image is loaded assert img is not None, "Image failed to load" # Resize image resized_img = cv2.resize(img, (200, 200))
In this snippet, if either the image path is incorrect, the image doesn’t exist at that path, or the image loading fails, an assertion error will be raised with an appropriate message indicating what exact problem occurred. Implementing checks before attempting the resize operation can often help in quickly pinpointing and resolving the issues causing cv2.Error: Resize.Cpp:4051.
The error message you’re encountering,
cv2.error: OpenCV(4.5.2) resize.cpp:4051: error: (-215:Assertion failed) !ssize.empty() in function 'cv::resize'
, is usually raised when the function
cv::Resize
in the OpenCV library gets an empty input image upon attempting to resize it.
This often manifests due to three main reasons:
– The image file cannot be found at the specified location.
– There’s misspelling or incorrect use of case-sensitive letters in the image filename or its extension.
– The loaded image file has a corrupted or unsupported format.
To ensure these aren’t the causes for your problem, you can check the following:
Verify your image path:
Make sure your file path is correct. Absolute paths are less prone to mistakes compared to relative paths.
Example code:
import cv2 img = cv2.imread('/absolute/path/to/image.jpg')
Validate existence of your image file: Ensure that the image file genuinely exists at the specified path by using Python’s built-in
os.path
module.
Example code:
import os if os.path.exists(file_path) and os.path.isfile(file_path): print("File found!") else: print("Image not found.")
Check file extension: It’s crucial to verify that the image file name and extension are both correct and spelled properly. Remember that file names and extensions are case sensitive on certain operating systems.
Inspect if your image data is corrupted or unsupported: After confirming the existence of the image file at the specified path with the correct name and extension, we should view the loading of the image to see if it was successful and the returned object is not empty. In OpenCV, if image loading fails, it doesn’t raise an exception, but rather returns None.
Example code:
img = cv2.imread('image.jpg') if img is None: print('Image load failed!') else: print('Image loaded successfully.')
Additionally, you might want to confirm whether your image format is supported by OpenCV. For instance, although OpenCV supports most of standard image formats like JPEG, PNG, TIFF, etc., it may stumble over new, proprietary, or uncommon ones.
By taking these steps systematically, it becomes inevitable where exactly the exception originates from – either failing to locate the requested image file, misspelled image filename/extension, or attempting to work with an unsupported or damaged image file. Following this approach will lead you to address the root cause effectively and accordingly.When attempting to use the cv2.resize() function in OpenCV 4.5.2, the error
Cv2.Error: OpenCV(4.5.2) resize.cpp:4051: error: (-215:Assertion failed) !ssize.empty() in function 'cv::resize'
is thrown when the source image you’re trying to resize is empty or has not been loaded properly.
To resolve this error, there are several debugging techniques that you can apply. Focusing on the code structure, application flow, and data inputs can bring about an effective debug process.
– **Check The Importation of Image**
To start with, it’s essential to confirm if the image file you’re dealing with has been imported successfully. An absence of errors during the importation process does not inherently entail a successful importation.
import cv2 # load image img = cv2.imread('file_path') # check if image is loaded if img is None: print('Image could not be loaded.') else: print('Image is loaded.')
If “Image could not be loaded” is printed, ensure your file path is correct. Python treats file paths differently when they begin with a slash (“/”). Instead of starting from the current directory, it starts from the root directory. It might cause issues if the file structure varies between your local machine and host server.
– **Validate Your Image Data**
Even though an image file was loaded successfully, certain circumstances might render the file useless; for example, only having black pixels.
# check if the image is just black pixels if cv2.countNonZero(cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)) == 0: print('The image is just black pixels.')
Any image only comprising black pixels will produce a zero count, revealing that the image is notably flawed.
– **Verify the Resize Parameters**
You may get an assertion failed exception if you are attempting to resize the image to a size that’s either too large or identical to its original size. Make sure your parameters specify a size apart from these extremes.
– **Applying Exception Handling Techniques**
Lastly, encapsulating your resize function inside a try-except block helps catch exceptions, providing detailed feedback about what went wrong. But bear in mind that this insulation wouldn’t necessarily solve the problem but instead make troubleshooting handy.
try: resized_img = cv2.resize(img, (x_dim, y_dim)) except Exception as e: print(f'Resize Exception: {e}')
By implementing these techniques, you’ll be able to effectively debug and overcome the
Cv2.Error: OpenCV(4.5.2) resize.cpp:4051: error: (-215:Assertion failed)
. If the problem persists, refer to the official OpenCV Documentation as it might necessitate a more extensive understanding of the particular functionalities, especially if you’re working with unique or exceptional datasets.
Resources:
– stackoverflow: How to check if an image has visible elements?
– Learn OpenCV – A comprehensive tutorial series on Computer Vision using OpenCV
First of all, the error message you’re encountering is related to OpenCV’s `cv::resize` function. Here’s a brief screencap of this error:
cv2.error: OpenCV(4.5.2) resize.cpp:4051: error: (-215:Assertion failed) !ssize.empty() in function 'cv::resize'
To help you understand what this error message signifies and ways to tackle it, let’s dissect its two components.
1. Assertion Failed:
The assertion that has failed here is
!ssize.empty()
. In other words, OpenCV expected the source image (that was meant to be resized) not to be empty- however, it was. Therefore, the problem lies within an empty or non-existing source image used as an input for the `resize` function.
2. Function ‘cv::resize’:
This part of the error message simply indicates that the error occurred within OpenCV’s `cv::resize` function. Typically, such an error is raised when there’s something inappropriate with the parameters the function receives. In our case, the source image appears to have been the problematic parameter.
Now, shifting to the resolution of this issue, here are a few possible areas you should inspect within your code:
• Check the Image Load Process: Prior to the resizing operation, ensure the image path provided reads correctly, and the image object gets created successfully.
• Validation: To alleviate the likelihood of accidentally passing an uninitialized or empty image to `resize`, add pre-validation steps. This can include null-checking conditionals or utilizing exception handling methodologies.
• Image File Format/Corruption: Make sure that the image file isn’t corrupted and is a format supported by OpenCV e.g., JPEG, PNG etc.
• Solution in Python: For instance, upon using Python, an error-checking step like this may look like:
import cv2 import os image_path = '/path/to/image.jpg' # Verify if the image path exists if os.path.exists(image_path): # If it does, load the image img = cv2.imread(image_path) # Then, make sure the image exists before attempting resize operation if img is not None: res = cv2.resize(img, dsize=(54, 140), interpolation=cv2.INTER_CUBIC) else: print('Image not loaded') else: print('Path does not exist')
The takeaway point from this error is: the `(-215:Assertion Failed)` error is frequently an indication of some argument related issue. Specifically, regarding the `!ssize.empty()` error, it emphasizes how you must ensure the source image being passed into your `cv::resize` function actually exists and has been correctly loaded.
Moreover, the beauty of OpenCV lies in its highly optimized funtions that provide almost real-time solutions. Understanding these functions and how they handle specific cases can streamline your computer vision projects and keep unforeseen errors at bay. Refer to the OpenCV documentation available here to dive deeper into the use-case-specific functionalities of functions like `cv::resize`.There is a particular issue in OpenCV applications that can cause a considerable amount of confusion and error if not addressed correctly. The error message thrown by the application usually looks something like this:
cv2.error: OpenCV(4.5.2) resize.cpp:4051: error: (-215:Assertion failed) !ssize.empty() in function 'cv::resize'
The reason this error occurs is that the source image provided to the `cv::resize` function is empty. Usually, it is because the path to the image you are trying to load is incorrect or the image does not exist at the specified location. To resolve this, first, check if your file path string is correct. [source] You could use `os.path.exists(filepath)` to verify whether an image exists at a given file path.
Delving into the subject of common troubleshooting steps for cv2 errors, we’re going to outline some potential solutions for resolving the Cv2.Error: OpenCV(4.5.2) Resize.cpp: 4051 error message. This particular error is triggered by an assertion check in cv::resize function that ensures the source image is not “empty”.
Let’s first decode part of the error message:
!ssize.empty() in function 'cv::resize'
The “!ssize.empty()” expression relates to a check being performed to confirm whether there is a valid or non-null source image (ssize). The ‘!’ symbol means “not” in C++. Thus, !ssize.empty() means that the function is asserting the source size should not be empty. When this function encounters an empty source image or invalid size, the AssertionError is raised.
A simple Python script using cv::resize might look like this:
import cv2 img = cv2.imread('image.jpg') resized_img = cv2.resize(img, (100, 100)) cv2.imshow('Resized Image', resized_img) cv2.waitKey(0) cv2.destroyAllWindows()
When the mentioned Cv2.Error occurs, this often indicates an issue with the image you’re attempting to resize. Here are a few possible scenarios and their fixes:
1. Invalid image path:
Make sure that you’re providing a valid image path to the cv2.imread() function. If the function can’t find the image at the specified path, it will return an empty matrix leading to a resize error.
Check your current working directory and ensure your image path corresponds appropriately.
Here’s a very basic way to check if your image has been loaded correctly where img is the variable name you have used to store the image:
if img is None: print("Image not loaded") else: # continue with the rest of the code
2. Incorrect spelling or extension of the image file:
If the image filename or its extension is misspelled, it won’t load correctly resulting in an empty source matrix. Validate your spelling and case sensitivity for the image filename and also verify that you’ve included the correct extension (.jpg, .png etc.).
3. Image not available in the directory:
Ensure that the image you want to read and resize actually exists in the defined path. Moving or deleting files can sometimes cause this issue.
4. File Permission Issues:
There may be cases when you do not have appropriate permissions to access the file. You may need administrative permission or need to change the ownership of the file if it’s located in a restricted directory.
In conclusion, understanding the origin of the
cv::resize
error is key to troubleshooting. In most cases, the error arises due to issues with the source image – it may not exist, may be incorrectly named or spelled, or may reside in an incorrect location.
You can refer to OpenCV’s official documentation
, it gives a complete list of functions including
cv2.resize
offered by OpenCV for handling images.Preventing cv::Resize assertion failures in OpenCV can help to enhance the performance and functionality of your code. When working with OpenCV, resizing an image is a common operation, but there can be instances when your code fails due to some issues. One such issue where error or failure may occur is when there is an assertion failure at ‘cv::resize’.
This kind of error essentially indicates that your source image object might probably be empty, and as a result, OpenCV fails to resize the object, hence leading to the cv2.Error.
Understanding the error message is the first step towards prevention – The Cv2 error (-215: Assertion failed) suggests two primary possibilities.
Firstly, this error may occur when you’re trying to operate on an image that does not exist, potentially because the image’s file path has been incorrectly specified.
Secondly, this error can also stem from an unsuccessful image reading process due to unsupported image format or corruption of the image file.
To prevent `cv::resize` assertion failures, Here are a few effective preventive measures –
- Ensure the correct file path: Make sure that the image file path is correctly specified in the imread function. You need to cross-verify whether the relative or absolute path given to the imread function is accurate. This is critical as providing an incorrect file path would cause the imread function to return an empty matrix because it won’t find any image to read.
- Check image format: Your image file format might not be compatible with OpenCV imread function. It’s recommended to use widely supported formats like .jpg or .png to prevent any likelihood of incompatibility issues. If you’re using a different image format, try converting it into supported ones.
- Validate with ‘None’ checks: Checking if the image data loaded into the application is empty by asserting the variable holding the image data should not be None before further processing of the images. For example,
img = cv2.imread('path/to/image.jpeg') assert img is not None, "Image couldn't be loaded"
This line will give out an assertion error if the image was unable to load for any reason.
- Confirm valid Image: Ensure that your image is not corrupt. A corrupted image file could lead to an unsuccessful image reading process. In this case, try using an alternate image.
- Debugging: Debugging the code can be beneficial to pinpoint the exact location or section causing the problem. Techniques like stepping through the code, print debug information, or use logging.
Here is an illustration of loading an image using the correct path:
img = cv2.imread('correct/path/to/image.jpeg')
Make sure the ‘image.jpeg’ exists in the provided path before running the code.
The effectiveness of these steps often depends on accurately diagnosing the cause behind your error. Therefore, understanding the different reasons this error can occur in the first place is critical. For more knowledge about debugging and error messages in OpenCV, one can refer to online resources available here.
Among the many error messages you may encounter during your journey through OpenCV (Open Source Computer Vision Library), one that can be particularly cryptic is
Cv2.Error: Opencv(4.5.2) Resize.Cpp:4051: Error: (-215:Assertion Failed) !Ssize.Empty() In Function 'Cv::Resize'
.
Firstly, understanding the context of this error message requires some insight into the purpose of the ‘resize’ function in OpenCV. This function is used to resize images in specific ways, such as by adjusting the width and height. An important principle to note here is that an image must have a spatial size for the resize function to work. If no spatial size exists, then there’s nothing to resize, which leads to an assertion failure.
When you encounter the Cv2.Error, it’s indicating that the resize operation was invoked on an image object that doesn’t contain anything – in other words, an empty object. The “!ssize.empty()” clause is the check for emptiness, with the “!” operating as a logical NOT – if the size is NOT empty, proceed; if it is empty, abort and throw an error.
So, how could you address this issue?
#import packages import cv2 # Always ensure that the path of the image file is correct. img = cv2.imread('FILE_PATH.jpg') # Test whether the image file is successfully loaded. if img is not None: # Only then execute the resize function. resized_img = cv2.resize(img, (new_width, new_height))
According to the snippet, you can add a condition to test whether the image file is properly loaded by checking if ‘img’ is not Null.
Avoiding common pitfalls like typos or incorrect paths is essential in preventing this error. Make sure you are adequately checking that your image objects are fully instantiated before performing operations on them. Lastly, the error could arise from a more complex issue like memory limitations or bottlenecks in your system that prevent successful image loading [source]. Studying these aspects will set you on the path to handling this Cv2.Error successfully. Stay patient and methodical in your debugging and remember experience is the best teacher!