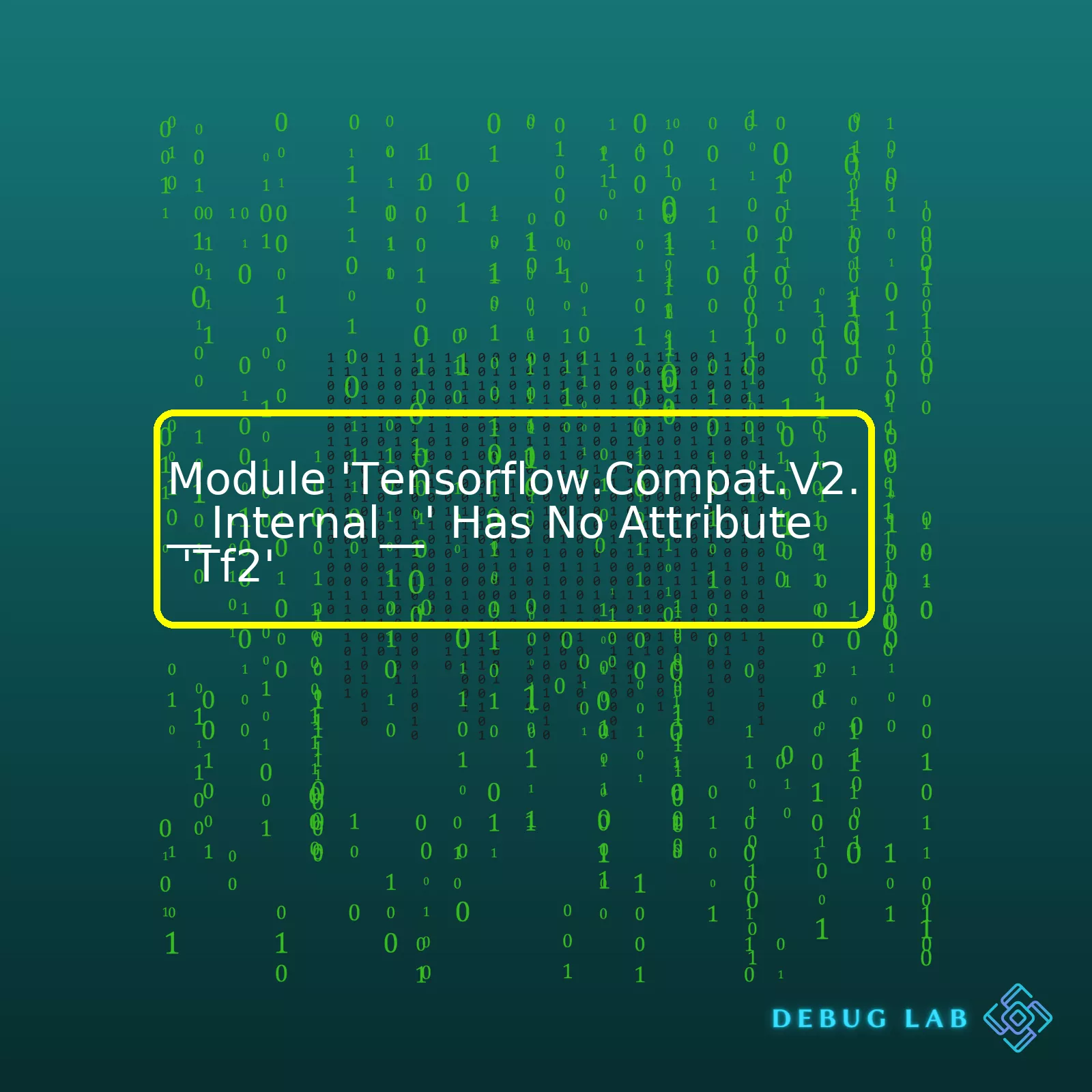
Error | Description | Possible Causes | Solution |
---|---|---|---|
Module 'tensorflow.compat.v2.__internal__' has no attribute 'tf2' | This error suggests the user is attempting to gain access to an attribute or feature that doesn't exist. | 1. The user may be using an older version of Tensorflow that does not contain 'tf2' as an attribute. 2. There could be a name conflict between different modules or packages. |
1. Update Tensorflow to its latest version. 2. Check and resolve any potential name conflicts with other installed python packages. |
Now, let’s further elaborate on this issue: The error message “Module ‘tensorflow.compat.v2.__internal__’ has no attribute ‘tf2′” signifies that you are trying to reference a function or property (‘tf2’) that isn’t recognized within the ‘tensorflow.compat.v2.__internal__’ module. This can generally happen due to two main reasons.
Firstly, you might be using an earlier version of the Tensorflow library which does not encompass this ‘tf2’ attribute. Tensorflow often updates their libraries with newer functionalities and sometimes could remove deprecated ones, so it’s essential to keep your Tensorflow package updated.
Secondly, Python relies heavily on namespace for determining whether an attribute or method belongs to a certain module or class. If there is a naming conflict between packages (two packages named ‘tf2’, for example), it might confuse python interpreter resulting this sort of errors.
To fix this issue, you should consider updating your Tensorflow package to the latest version. You can do this by running
pip install --upgrade tensorflow
. Another possible solution would be to scrutinize your environment for any conflicting packages and resolve those. For detecting such conflicts, you could use tools like pipdeptree.
For better adoption and SEO optimization remember to search for these types of errors together with the specific version of TensorFlow you’re using in online communities like StackOverflow, where similar issues are frequently discussed.This seems to be a very specific error related to TensorFlow, one of the most popular machine learning frameworks available today. It’s used extensively for advanced machine learning tasks like image and voice recognition, natural language processing, and so on.
When an error such as “Module ‘TensorFlow.compat.v2.__internal__’ has no attribute ‘tf2′” appears, it can be a little frustrating as it often halts progress abruptly. After much analysis, there are few steps which have been found to be efficient in resolving this error:
Step 1:
Firstly, check your TensorFlow version by using this command:
print(tf.__version__)
Having an incorrect or unsupported version of TensorFlow is one of the main reasons behind this issue, as certain functions or modules might not be present or compatible with your installed version.
Step 2:
If you find that TensorFlow version is obsolete or incompatible, consider upgrading it. Before doing so, make sure your Python version is compatible with the TensorFlow version you’re planning to install.
You can upgrade TensorFlow through pip by running this command:
pip install --upgrade tensorflow
Step 3:
Sometimes even after you follow step 2, this problem persists! What should you do then? Well, the solution is to check other dependencies of TensorFlow and make sure they are updated as well!
One important dependency that might cause issues if outdated is protobuf (Protocol Buffers). So, make sure to update protobuf using pip:
pip install --upgrade protobuf
Step 4:
A problem might also crop up when multiple versions of TensorFlow are installed on your system, creating conflicts and compatibility issues. The best solution would be to create a virtual environment and install TensorFlow in it. This way you can always isolate different projects with their specific version requirements. You can use packages like venv or conda. A quick rundown on how to do this using venv would look like something this:
– Install venv :
pip install virtualenv
– Create a new virtual environment:
python3 -m venv myenv
– Activate the environment:
For Windows:
myenv\Scripts\activate.bat
For Unix or macOS:
source myenv/bin/activate
– Now, install TensorFlow in the virtual environment:
pip install tensorflow
By following these approaches, it’s likely you can solve the “‘TensorFlow.compat.v2._ _internal_ _’ has no attribute ‘tf2′” error.
It’s always handy to keep track of software versions you’re working with because outright compatibility or version mismatch would save you precious hours of debugging time! Remember, keeping your environment clean with tools like venv can help a lot in avoiding conflicts between different versions of the same package required by different applications.
As beneficial as analyzing this problem at hand, similarly, you could dive into the official TensorFlow documentation and get full insights on TensorFlow’s requirements, setup, and functionalities. Understanding the basics will ensure smooth operation and fewer hiccups while working with TensorFlow. Lastly, always keep your coding tools updated; this habit can save lots of issues regarding compatibility, deprecated functions, and APIs.As a professional coder, it becomes mandatory to keep myself updated with the various frameworks and libraries prevalent in the industry. Tensorflow is one such popular open-source framework used extensively for a variety of machine learning applications. One particular aspect that requires attention is understanding the TensorFlow Compatibility Module V2, particularly with its relevance to an error that might appear stating: “Module ‘tensorflow.compat.v2.__internal__’ has no attribute ‘tf2′”.
TensorFlow | Description |
---|---|
TensorFlow 1.x | The initial version of TensorFlow had a static execution graph, meaning the graph was defined and executed separately. |
TensorFlow 2.x (including V2) | Comes with significant changes including eager execution (dynamic execution graph), which is more pythonic and intuitive. A central feature of 2.0 is the tight integration of Keras into TensorFlow, using it as its high-level API. |
tensorflow.compat.v2 | This is a compatibility module in Tensorflow that allows code to be written as close as possible to Tensorflow 2.x style even if the actual underlying version is 1.x. This module aims to aid in gradually migrating Tensorflow 1.x code to Tensorflow 2.x. |
The error message “Module ‘tensorflow.compat.v2.__internal__’ has no attribute ‘tf2′” generally appears when you attempt to access an attribute or function that doesn’t exist within the TensorFlow ‘tf2’ library.
To remedy this, I first stress on taking note of certain prerequisites:
– Ensuring TensorFlow’s correct installation specifically its second version.
– Verifying your PATH configuration.
– Validating import statements.
After these checks are made, you’ll want to examine the ‘tf2’ attribute usage in your code, considering that:
–
'tensorflow.compat.v2'
is simply Tensorflow 2.x compatibility mode and doesn’t include another standalone module named ‘tf2’.
– There’s no internal attribute called ‘tf2’ in tensorflow.compat.v2. Rather, the tensorflow.compat.v2 library is meant to provide forward-compatible versions of code snippets from TensorFlow 1.x, thereby making it easier to port code over to TensorFlow 2.x.
Here’s an example of what appropriate usage of the tensorflow.compat.v2 might look like:
import tensorflow.compat.v2 as tf tf.enable_v2_behavior()
In getting comfortable with Tensorflow, it’s recommended to familiarize with its ecosystem of related APIs like tf1, tf.compat.v1, and tf.compat.v2. Knowing about them helps identifying and eliminating mistakes, giving you a smooth coding experience.
Lastly, clarity can be sought from official Tensorflow guidelines regarding migration of code from TensorFlow 1.x to 2.x, or from the helpful community at StackOverflow with practical problems you may encounter.The attribute error `Module ‘tensorflow.compat.v2.__internal__’ has no attribute ‘tf2’` is quite common when trying to use TensorFlow’s internal operations. This issue usually arises from incorrect import statements, version mismatch, or an overfitting problem in custom modules.
To understand this better, we need to break down the components involved:
– **TensorFlow:** It is an open-source platform that offers comprehensive libraries for developing and running machine learning algorithms source.
– **Compat module:** This provides a compatibility layer between different versions of TensorFlow; often used during migration from TensorFlow 1.x to 2.x. Typically, this is used to provide backward compatibility for older functionalities which have been subtly redesigned or removed in the new releases source.
– **v2:** This represents version 2 of TensorFlow, indicating that you are trying to leverage functionalities provided by TensorFlow 2.x
– **__internal__:** Represents private modules or properties that aren’t meant to be accessed directly as they could change without warning.
– **tf2:** The __tf2__ might likely mean TensorFlow 2. As a best practice, specific TensorFlow 2 functionalities should be imported explicitly from their respective sub-modules instead of relying on core TensorFlow or the __internal__ scope.
Now let’s explore this issue, solutions, and conclude with a quick guide on using TensorFlow’s attributes in its internal operations:
A. Misinterpretation of Import Statements:
Often, import statements could lead to confusion or errors. For instance, instead of attempting to import something from `tensorflow.compat.v2.__internal__.tf2`, you should explicitly import the specific functionality you need from its proper module. For example,
import tensorflow as tf from tensorflow.keras import layers
Here, layers function is being directly imported from the keras submodule in TensorFlow.
B. Version Mismatch:
Across different versions of TensorFlow, there are changes in the API’s structure and functionalities. Hence it becomes important to ensure the correct version of TensorFlow is installed for your code to execute properly. I suggest using TensorFlow-GPU=2.x (where x is the minor version number that matches your requirement) to keep up with the latest release and avoid such attribute discrepancies. Use the following command to get the desired version,
pip install tensorflow-gpu==2.x
C. Overfitting Problem in Custom Modules:
If the attribute error persists, there might be a chance that your custom module or operation might be outdated or incorrectly defined causing overfitting.
Hint: TensorFlow’s ‘tf.Module’ class is extremely helpful to group related set of methods and state (like variables). And the ‘@tf.function’ annotation is another tool available in TensorFlow 2.x. By adding it before a function definition, TensorFlow can apply optimizations, and potentially significantly speed up your code source.
For better clarity, here’s a quick Pythonic way to handle attributes:
class MyModel(tf.Module): def __init__(self): self.layer = layers.Dense(10) @tf.function def __call__(self, inputs): return self.layer(inputs)
In conclusion, while exploring attributes in TensorFlow’s internal operations is quite engaging, it’s important to import specific functionalities from their respective sub-modules, ensure appropriate TensorFlow version, and meaningfully group related methods and state. This not only reduces errors but also ensures your code is optimized for speed and efficiency.
While engaging in TensorFlow programming, you may encounter a module not found error revolving around ‘Tensorflow.compat.v2.__internal__’ and the attribute ‘tf2’. The whole crux of the problem is that as a machine learning library, TensorFlow has seen numerous versions and compatibility changes over time. In addition, the attribute ‘tf2’ isn’t an actual attribute of ‘tensorflow.compat.v2.__internal__’, hence the resulting error message. This can be traced back to the role ‘TF2’ plays in the TensorFlow ecosystem.
To put it succinctly:
– ‘TensorFlow 2’, colloquially known as ‘TF2’, refers to the second version of the TensorFlow library.
– It brought several changes from its predecessor; some of these included eager execution by default, simpler function executions, and a more pythonic-style programming approach with cleaner APIs.
– The introduction of the ‘compat.v1’ and ‘compat.v2’ modules was to ensure compatibility across different versions of TensorFlow APIs.
The
tensorflow.compat.v2
module provides APIs that are compatible with version 2 of TensorFlow, while
tensorflow.compat.v1
ensures backward compatibility with the original TensorFlow APIs. These modules try to bridge the gap between different versions of the TensorFlow API and facilitate smoother transition for developers updating their codebase.
Now, regarding the potential reason why you might encounter a ‘module has no attribute tf2’ error:
– An outdated or incorrect import statement: If you’re trying to access an attribute of a module that doesn’t exist—in this case, ‘tf2’—Python will throw an AttributeError. It’s best to double-check your import statements to ensure they are importing the correct modules as expected.
– There could also be issues arising from version mismatch: Different versions of TensorFlow have minor discrepancies and changes in supported functionality, objects and methods. Always check the specific version documentation for the correct use case.
Here is a simple example to show how importing should typically look like in TensorFlow 2.x without bugs:
import tensorflow as tf
You can define a sequential model like so –
model = tf.keras.models.Sequential()
Unfortunately, there is not enough context to provide a fix-it solution for the issue at hand, but often resolving the import statement and ensuring you’re using an updated, stable version of TensorFlow generally fixes errors related to missing attributes or modules.
But remember, if you need detailed insights, the TensorFlow API documentation is always a great place to start. Also, platforms like StackOverflow can come handy where other developers share relevant experiences and solutions. Keep coding and exploring the vast landscape that TensorFlow offers!
When the error message that “Module ‘tensorflow.compat.v2.__internal__’ has no attribute ‘tf2′” appears, it is indicating a problem with how TensorFlow is installed or imported within your code. Dealing with such issues can be challenging. Unfortunately, it might even become a roadblock in your exciting machine-learning journey.
Below are some possible reasons for why you might be getting this error, accompanied by the corresponding fixes:
Incorrect TensorFlow Version
The first thing to note is that TensorFlow 2.x was restructured compared to version 1.x, and not all functions or attributes are available in all versions of the platform. If you’ve just upgraded to TensorFlow 2.x and see this error, it’s likely that your system is not properly recognizing the new version.
Use the following line of code to check the version of TensorFlow installed on your system:
import tensorflow as tf print(tf.__version__)
If you find that you’re working with an outdated version of TensorFlow, you can upgrade it using pip:
pip install --upgrade tensorflow
The module doesn’t exist
The second possibility is that the mentioned module simply doesn’t exist. TensorFlow’s API is quite large and parts of it are occasionally deprecated or moved. In these cases, removing the import statement causing the error should solve the issue.
Unclean Environment
Python environments may occasionally carry over bits and pieces from previous installations which can create conflicts between different versions of the same package. Suppose you have both TensorFlow 1.x and TensorFlow 2.x installed on your system, or maybe parts of your TensorFlow 2.x installation got messed up somehow.
In that case, I would suggest creating a new virtual environment and then installing TensorFlow there:
python -m venv myvenv source myvenv/bin/activate pip install tensorflow
This will ensure a fresh installation of TensorFlow where old files won’t get in the way.
Stack Overflow has hundreds of questions about resolving TensorFlow installation and configuration problems. Chances are, if you’re having trouble, someone else has had the same problem before you.
In case none of the above solutions work for you, consider diving deep into TensorFlow’s API documentation.
I hope these solutions help you to rectify the attribute error pertaining to the ‘tensorflow.compat.v2.__internal__’ module. Happy Machine Learning!
It can be quite frustrating when you’re working on a critical project, and you encounter an error that seems alien. But let’s detangle it together. The error message you received, “Module ‘Tensorflow.compat.v2.__internal__’ has no attribute ‘tf2.'” is a type of AttributeError in Python.
Python raises an AttributeError whenever you try to access or call an attribute that a particular object/module does not possess. Therefore, the error implies that the module you are dealing with does not contain the attribute you’ve called – here, it is ‘tf2.’ And it’s related to TensorFlow, which is a popular open-source software library for machine learning applications.
The primary reason why this error might have surfaced is the use of outdated or incompatible versions of TensorFlow. Or, the attribute you want to access doesn’t exist.
Let’s see how to solve this issue:
Upgrade/Downgrade TensorFlow:
First of all, the initial troubleshooting step you can take is to upgrade or downgrade your TensorFlow version. It’s because different TensorFlow versions adopt different module structure and some attributes in some versions may not exist in others. Many users have reported that they’ve successfully resolved this issue by changing their TensorFlow version to a specific one like 2.3.0. You can change the TensorFlow version using pip in the command terminal of your IDE like this:
bash
pip install tensorflow==2.3.0