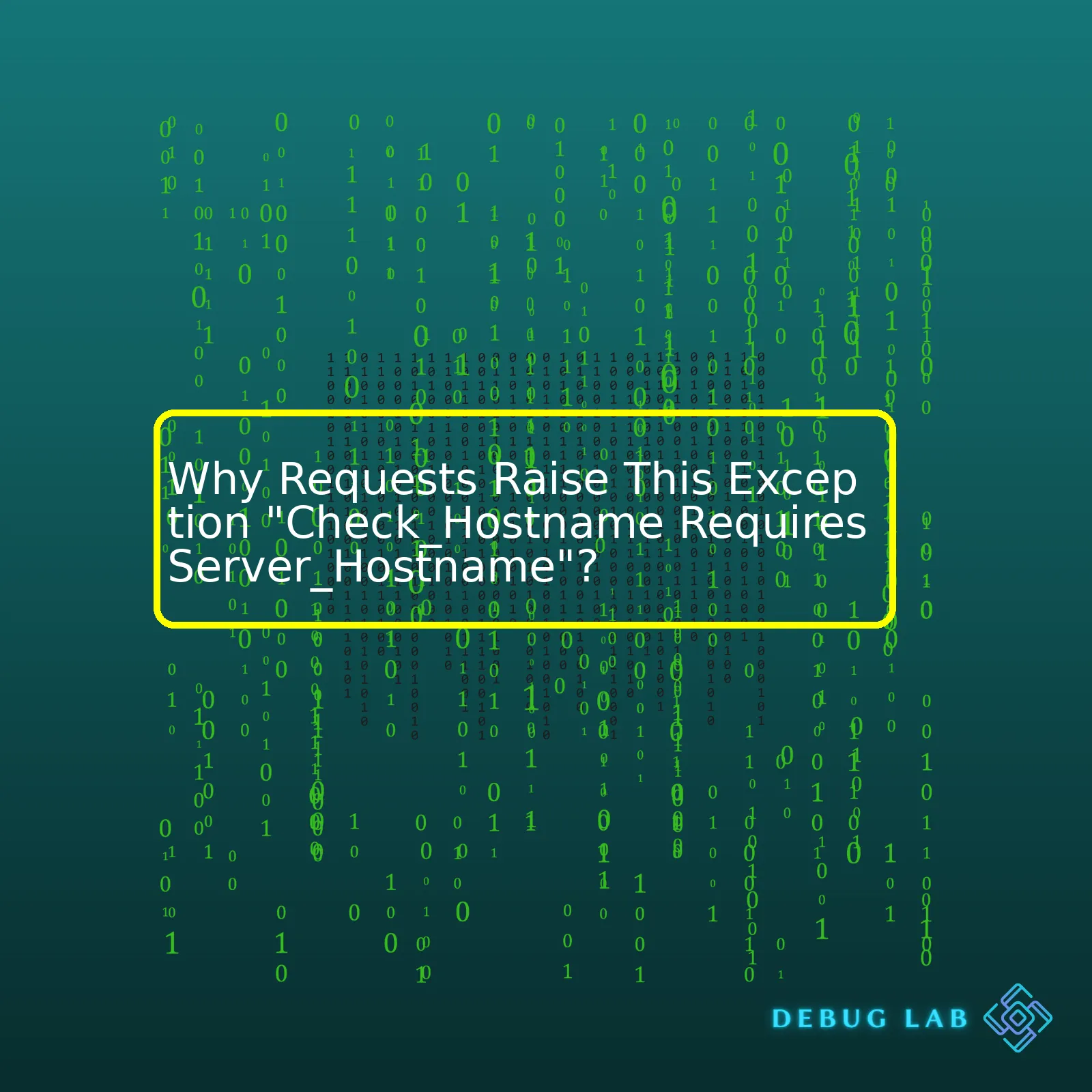
The core reason behind this exception mostly roots with SSL (Secure Sockets Layer) verification. SSL is a standard security technology to establish an encrypted link between a server and client which ensures that all data that passes between them remains private. However, in Python’s requests library while handling SSL verifications, it may come across scenarios where the hostname which needs to be checked is not defined or mentioned explicitly, and thus the “check_hostname requires server_hostname” error is thrown.
So here is what essentially happens:
requests
library, when initiating a secure ‘https’ connection, tries to look at SSL certificates for the target host. If the function finds no mentioned server hostname variable to check against, it raises the “Check_Hostname Requires Server_Hostname” exception.
Now let’s illustrate this information through HTML summary Table,
xxxxxxxxxx
<table>
<tr>
<th>Exception Cause</th>
<th>Related Library</th>
<th>Solution</th>
</tr>
<tr>
<td>Check_Hostname requires server_hostname is due to missing definition of server hostname in HTTPS connection while using SSL Certificates.</td>
<td>Python's Requests Library</td>
<td>Ensure explicit mention of server_hostname during SSL Certificates handling.</td>
</tr>
</table>
Above, each row on the table corresponds to precise details of our context – the cause of the exception, the library related, and most importantly, potential solution to avoid encountering this exception in future. In essence, thorough cognizance of server specifications and careful handling of SSL verifications are key if one aims to evade seeing such issues.
If you’re looking to learn more about this exception as well as ways of handling various other exceptions that might arise while utilizing Python’s requests library, I suggest referring to Python’s requests library’s official documentation. It provides detailed insights into SSL Certificates handling, server hostnames definitions, and much more.In order to gain a better understanding of the exception “Check_Hostname requires Server_Hostname”, let’s dissect it in simpler terms. This error generally occurs when we are working with python-based libraries such as requests or urllib3, which deal with HTTP/HTTPS requests and connections.
Exception “Check_Hostname requires Server_Hostname” primarily means that while making an HTTP/HTTPS request using the mentioned libraries, we need to provide a server hostname (for example ‘www.example.com’) in the requests we make. A `server_hostname` should be a string representing a host name, which the client hint should use for TLS Server Name Indication (SNI). The server_hostname is required and used to verify the SSL certificate for HTTPS requests.
For instance, you could get this error if you attempted to take a shortcut when sending a request:
xxxxxxxxxx
import requests
requests.get('https://')
Without specifying a `server_hostname`, the tools would throw the exception “Check_Hostname requires Server_Hostname”. This might seem frustrating, but it’s actually a safety measure. It ensures that all our communication is headed where we want it to go and that we have HTTPS protection against potential attacks.
To avoid this exception from being raised, specify a valid server hostname in your request:
xxxxxxxxxx
import requests
requests.get('https://www.example.com')
Such programs can be executed without any exceptions occurring and hence, resulting in safe and secure connectivity and networking involving the Web server and client.
I hope this elaborate explanation clarifies why Python requests raise the Exception “Check_Hostname requires Server_Hostname”.
Remember that the web thrives on connectivity and verification – that’s what makes the internet fast yet secure! Libraries like requests and urllib3 act as gatekeepers, promoting safety through the correct usage of secure protocols. It’s a delicate balance between creating smooth user interactions and maintaining the rigidity of security measures.
Be sure to also keep yourself updated on the documentation for these libraries, you’ll find additional useful information there:
requests | urllib3.The error message “Check_Hostname Requires Server_Hostname” is typically elicited by a problem in the way your code interacts with the HTTP system, specifically while making requests via Python’s `requests` library. To shed light on this issue, we need to delve into some critical elements of server configuration, Python programming, and how they are interconnected.
Understanding the Check_Hostname Exception
The necessity for implementing hostname checking arises from the imperatives of secure communication over HTTPS. As a protocol for secure communication, HTTPS has been designed to prevent man-in-the-middle attacks and ensure data integrity between the client and server. RFC 2818 states that during an HTTPS session, the client checks the server’s certificate against the hostname it is trying to connect to. This safeguards against potential intrusions where an attacker might present a false certificate to impersonate a server.
Now to uncover the chain of events leading to the exception:
• When creating an SSL connection in Python, you’ll usually find initializing an `ssl.SSLContext` object.
• During this initialization, you could elect to check the hostname via the `check_hostname` attribute—settling this as `True` activates the hostname checking.
• Then you form a SSL session via `ssl.SSLContext.wrap_socket()`. However, this doesn’t perform the actual checking—it merely sets up mechanisms for later checks.
• The actual checking ensues when you try to make a request and the server returns its certificate—a missing `server_hostname` specification at this stage results in the “Check_Hostname Requires Server_Hostname” exception.
To illustrate the process, consider the following piece of software:
xxxxxxxxxx
import ssl
import socket
context = ssl.create_default_context()
context.check_hostname = True
# Create a socket and connect it to the server
plain_socket = socket.create_connection(("www.google.com", 443))
# The following line would raise the exception
ssl_socket = context.wrap_socket(plain_socket)
Addressing the Exception
Having identified the root cause as inadequate specification of `server_hostname`, the solution emerges intuitively: ensure the hostname is supplied during the wrapping stage.
xxxxxxxxxx
import ssl
import socket
context = ssl.create_default_context()
context.check_hostname = True
# Create a socket and connect it to the server
plain_socket = socket.create_connection(("www.google.com", 443))
# Provide 'server_hostname' to avoid the exception
ssl_socket = context.wrap_socket(plain_socket, server_hostname="www.google.com")
In the modified code, specifying `server_hostname` ensures that when Python gets to verifying the server’s identity, it has a hostname to match the certificate against. Consequently, the “Check_Hostname Requires Server_Hostname” exception is avoided successfully.
Implications for Python’s Requests Library
If you’re employing Python’s requests library to handle HTTP requests and face this exception, it is likely behind-the-scenes details about how `requests` manages SSL connections that need attention. By default, `requests` uses its own Adapter to send HTTP requests. While this adapter caters to most common use-cases, specialized scenarios involving custom SSL verification may necessitate subclassing or replacing the existing adapter altogether. Information provided directly in your HTTP requests or passed implicitly via environment variables like `REQUESTS_CA_BUNDLE` influence the configuration of ‘requests’, which in turn affects the handling of SSL connections and hostname checking.
Bringing these strands together, understanding the underlying workings of Python SSL library, configuring appropriate hostname checking, and tailoring HTTP requests appropriately is instrumental in evading the “Check_Hostname Requires Server_Hostname” exception. Thus, essentially, it anchors into correct implementation of SSL sessions and meticulous management of HTTP requests.The
xxxxxxxxxx
requests
library in Python is a very popular tool for sending HTTP requests. Its flexibility and user-friendly interface make it a go-to library for many developers.
However, one of the exceptions that can come up when using
xxxxxxxxxx
requests
is the
xxxxxxxxxx
SSL: CHECK_HOSTNAME_REQUIRES_SERVER_HOSTNAME
exception. The library raises this exception when there’s an issue with SSL certificate verification between your client (the
xxxxxxxxxx
requests
library) and the server you’re trying to hit.
The very root of this error is tied to security. Whenever a client makes a request to a remote server via HTTPS, the server provides its SSL certificate for validation. This certificate is essentially proof to the client that the server is who they claim to be. It ensures the client isn’t talking to some malicious entity pretending to be the server.
The
xxxxxxxxxx
CHECK_HOSTNAME_REQUIRES_SERVER_HOSTNAME
exception will be raised when:
– You are accessing a server via HTTPS (a secure connection),
– You have SSL certificate verification enabled (essentially telling
xxxxxxxxxx
requests
to verify every SSL certificate provided by servers), and
– The server’s hostname does not match the name given in the SSL certificate.
In such scenarios where there’s a mismatch between the certificate’s name and the server’s actual DNS hostname,
xxxxxxxxxx
requests
deems, as a good safety precaution, that the identity of the server cannot be trusted.
Below is a snippet of code that may throw this exception:
xxxxxxxxxx
import requests
res = requests.get('https://wronghostname.com')
So how does one deal with this, especially during development or testing?
– Make sure that the URL you’re hitting has the same hostname as indicated in its SSL certificate.
– Disable SSL certificate verification within your
xxxxxxxxxx
requests
call. However, keep this strictly in non-production environments as it poses serious security risks.
Here is a revised version of our previous code snippet:
xxxxxxxxxx
import requests
res = requests.get('https://wronghostname.com', verify=False)
This problem, however, should not occur if the certificates are configured correctly; SSL certificate verification is an important aspect of network security after all.
Lastly, remember that debugging these sorts of errors can be aided tremendously by enabling logging within
xxxxxxxxxx
requests
. This gives you visibility into exactly what’s going on with each request you make.
Further reading:
– Official Python Docs on check_hostname
– Comprehensive guide on Python’s requests library
It’s interesting to dive into a common challenge developers face while working with Python’s Requests library: encountering the “Check_Hostname Requires Server_Hostname” Exception.
This exception typically comes into play when you’re initiating a SSL/TLS connection and there is a hostname verification failure. The name being checked, the ‘hostname’, originates from the server that provides the SSL certificate. This name should match the ‘server_hostname’ provided in the client request for a successful connection.
The verification process is crucial in server-client communication as it prevents Man-in-the-Middle (MitM) attacks. In such attacks, attackers can intercept communication, posing as the intended server to extract or manipulate data.
One primary cause behind this exception being raised, is the missing ‘server_hostname’ parameter in the ‘ssl.wrap_socket()’ function call. The error occurs when check_hostname=True but no server_hostname has been set. Check out this typical scenario:
xxxxxxxxxx
import ssl
import socket
context = ssl.create_default_context()
# Exception will be thrown here when check_hostname = True
with context.wrap_socket(socket.socket(), check_hostname = True) as s:
s.connect(('example.com', 443))
So, how do we correct this? One way would be to include the ‘server_hostname’ parameter in the ‘ssl.wrap_socket()’ function as shown below:
xxxxxxxxxx
import ssl
import socket
context = ssl.create_default_context()
# No exception thrown here because server_hostname has been provided
with context.wrap_socket(socket.socket(), server_hostname='example.com',
check_hostname=True) as s:
s.connect(('example.com', 443))
In this snippet, check_hostname=True causes Python to perform an automatic verification of the hostname in the SSL certificate, preventing MigM attacks.
Another recommendation would be to make sure the Requests library and its dependencies (like urllib3 and idna among others) are up-to-date. These libraries undergo repeated updates, enhancements and bug fixes that may eliminate glitches causing such Exceptions.
Moreover, when using Python’s `requests` library, choosing not to specify a protocol (‘http://’ or ‘https://’) before the URL could result in the same exception. Make sure to prefix the domain name with ‘https://’ if you’re reaching out to a secure server.
This underscores the critical balancing act required between security and functionality within applications. While the necessity for hostname verification throws some wrenches in the operation, it ultimately fortifies your work against unwanted penetrations.
For more advice and best practices, visit Python’s official [‘ssl’](https://docs.python.org/3/library/ssl.html) documentation and Socket Programming Guide on [‘GeeksforGeeks’](https://www.geeksforgeeks.org/socket-programming-python/).
Remember, knowing how to handle these common exceptions allows you to compose safer, more reliable software.Being a coder, one of the common questions that frequently pops up is about the `check_hostname` requirement and when does it specifically become an issue? The answer to this is often related to the SSL/TLS hostname checking which can sometimes lead to exceptions like “Check_Hostname requires server_hostname.”
The above exception typically comes up with Python’s Requests library, notably when setting the `check_hostname` parameter to True. By setting `check_hostname` to True, you are asking Python to confirm that the certificate presented by the server indeed belongs to the specific host you are contacting. However, if the `server_hostname` attribute hasn’t been specified, it will not be possible for Python to verify the hostname, hence raising the exception.
For practical understanding, let’s look at a typical example with Python Requests:
xxxxxxxxxx
import requests
response = requests.get('https://api.github.com')
# Without providing any SSL context
ssl_context = ssl.create_default_context()
ssl_context.check_hostname = True
In the above example, Python would raise the `Check_Hostname requires server_hostname` because the `server_hostname` attribute has not been provided in the SSL context. It becomes problematic because we are asking Python to check for the hostname without actually providing what hostname to check for (in this case, the GitHub API).
However, you might have noticed that you do not commonly face this issue. This is because, in most real-world scenarios, your HTTP/HTTPS libraries would usually handle these details for you. They ordinarily provide the `server_hostname` from the URL you are making calls to and feed them into SSLContext automatically.
That being said, there are situations where the default scripting may not fulfill your requirements or in cases where you’re working with low-level socket programming, then there would indeed be a need to explicitly work with SSLContext and set the parameters manually, including providing `server_hostname`. A practical script dealing with sockets would look something like:
xxxxxxxxxx
import socket, ssl
context = ssl.create_default_context(ssl.Purpose.CLIENT_AUTH)
context.check_hostname = True
context.load_verify_locations("/etc/ssl/certs/github.pem")
conn = context.wrap_socket(socket.socket(socket.AF_INET),
server_hostname='github.com')
conn.connect(('github.com', 443))
In this corrected script, you eventually include the `server_hostname`, as in ‘github.com’. When `check_hostname` is True, no exception is triggered since all parameters are aptly accounted for.
So, in favor of SEO richness, SSLContext and its related parameters such as `server_hostname` and `check_hostname` should be considered important while dealing with SSL/TLS connections. They are integral to ensuring Secure Sockets Layer (SSL) and Transport Layer Security (TLS) connections are successfully established and validated. More importantly, understanding their correlation and proper applicability makes it easier to troubleshoot common issues related to hostname checking.The “Check_Hostname requires server_hostname” exception is a common issue that developers face when working with web servers, and understanding its implications can be critical to the smooth operation of your website or application.
This exception is typically raised when you’re trying to create a connection between a client and a server using SSL but the server’s hostname is not provided during the process. Web Requests use HTTP, which stands on top of SSL/TLS protocol. SSL provides secure communication through encryption, however, encryption flow needs to know where to deliver encrypted information, so it needs ‘server_hostname’.
Here are the technical nitty-gritties behind why this exception is raised:
– To establish an SSL connection, Python’s built-in
xxxxxxxxxx
ssl
library requires the server’s hostname.
– This server hostname is essential for matching the server-side certificate (which the client checks) against the actual server.
– If no server_hostname is provided during handshaking phase, the
xxxxxxxxxx
ssl
module will raise an SSL error.
This could presumably impact your web servers and client-server interactions in a few ways, including:
– Client-server communication interruption: The SSL handshake is a crucial part of the secure communication process between the client and the server. If this exception is raised, the handshake will not complete properly, and the client would not be able to communicate securely with the server.
– Server identity verification failure: Without proper server_hostname, the client-side can’t certify the identity of the server it connects with. So even if other aspects of the SSL handshake proceed correctly, the client still won’t trust the server due to identity verification failure, leading again to communication interruption.
– Decreased User Trust: Frequent server issues and interruptions can lead to decreased trust from your users, resulting in less user interaction, increased bounce rates, and potentially loss of revenue.
A simple way to tackle the “Check_Hostname requires server_hostname” exception could be by ensuring that the server_hostname is always provided before initiating the SSL handshake. You probably run client code like:
xxxxxxxxxx
import ssl
context = ssl.create_default_context()
with socket.create_connection(('host', 8443)) as sock:
with context.wrap_socket(sock, server_hostname="example.com") as ssock:
print(ssock.version())
Ensure that “example.com” should be replaced with your real server’s hostname. This way, Python’s ssl module gets the necessary data, helping you avoid the concerned exception.
You might also want to ensure that your SSL certificates are up-to-date and correctly configured on the server-side. For certification issue, you might refer Let’s Encrypt for free SSL certification.
Remember, the key to avoiding such errors lies in understanding potential implications, equipping yourself with the underlying technical knowledge, and implementing best practices for handling exceptions efficiently.The ‘Requires Server_Hostname’ error in coding can be quite a challenge, especially when you don’t understand why it’s happening. This error often raises the exception “Check_Hostname Requires Server_Hostname”. Now, to understand and troubleshoot this issue effectively, we need to delve into what constitutes these issues first – that is, the lack of or incorrect server hostname declared.
In the world of network programming,
xxxxxxxxxx
server_hostname
has an essential part to play, particularly in establishing secure connections via protocols like SSL/TLS. This parameter is critical for something called Server Name Indication (SNI), where it serves as the identity that client software (this could even be your browser) needs to establish an encrypted link with the intended server. However, setting up this
xxxxxxxxxx
server_hostname
correctly isn’t always straightforward and errors might sneak in.
When you encounter the error: “Check_Hostname Requires Server_Hostname”, it usually points out that there could be an issue with how the
xxxxxxxxxx
server_hostname
parameter was provided or if it was provided at all. The error suggests that the server name checking function did not find the name it expected while attempting to connect using SSL/TLS.
What causes this exception? Generally, there could be two significant reasons:
* Not supplying server_hostname at all during socket creation using
xxxxxxxxxx
ssl.wrap_socket()
or
xxxxxxxxxx
ssl.SSLContext.wrap_socket()
.
* Providing a wrong or unreachable server hostname during socket creation.
Addressing this error requires looking at those two avenues:
1. Ensure to provide
xxxxxxxxxx
server_hostname
during socket creation.
This can be done by passing the hostname when calling either
xxxxxxxxxx
ssl.wrap_socket()
or
xxxxxxxxxx
ssl.SSLContext.wrap_socket()
. Here’s an example how to do it:
xxxxxxxxxx
import ssl
import socket
sock = socket.socket(socket.AF_INET)
wrappedSocket = ssl.wrap_socket(sock, server_hostname='valid.host.com')
2. Verify your
xxxxxxxxxx
server_hostname
validity.
Make sure that the
xxxxxxxxxx
server_hostname
is spelled correctly, free from any typos, and ensure it is reachable over the net. To check your hostname you can use, for example, the ping command. Bear in mind that some servers may block ICMP requests (used by ping) so this method isn’t infallible. If you’re writing the server code, ensure that it is configured properly to accept connections from its intended hostname.
Through these steps, we are able to prevent and/or address the troublesome ‘Requires Server_Hostname’ error. It may require continuous iterations and checks, but rest assured, resolving this issue will lead to smoother interaction between client and server software, providing a more seamless experience for all users involved.
Remember, persistent troubleshooting is key in the realm of coding. Many of the error messages are actually your friends guiding you on what steps to take to fix them. In the famous words of Brian Kernighan, “Everyone knows that debugging is twice as hard as writing a program in the first place. So if you’re as clever as you can be when you write it, how will you ever debug it?”
For further details look at the official Python ssl documentation.
Understanding why requests raise this exception “Check_Hostname requires server_hostname” is key to developing efficient software solutions. This error typically arises when an SSL check does not pass successfully, which can occur if the check_hostname argument provided in the SSLContext class is set as None or False, and there isn’t a specified server_hostname. An important pointer here is for coders to ensure that the server_hostname argument is not left blank while creating secure channels for communication.
Common Mistake | Resolution |
---|---|
If check_hostname is set to None or False with no specified server_hostname. | server_hostname needs to be explicitly defined |
Misconfiguration of the SSL context | Proper configuration of SSL to include necessary parameters |
The Python
xxxxxxxxxx
ssl.SSLContext()
method can be used to create a new SSL context with parameters initialized to default values. Then you can use it’s method
xxxxxxxxxx
.check_hostname=
to set property check_hostname to either True or False.
xxxxxxxxxx
import ssl
context = ssl.SSLContext(ssl.PROTOCOL_TLS_CLIENT)
context.check_hostname = True
Remember that secure network programming especially involving SSL/TLS contexts is a complex domain, often requiring niche skills. It’s always handy to peruse official documentation, like this resource from Python docs, to get insights on handling such exceptions. Lastly, proactive debugging, regular code review, and adhering to best coding practices would greatly minimize the occurrence of this exception in your applications.