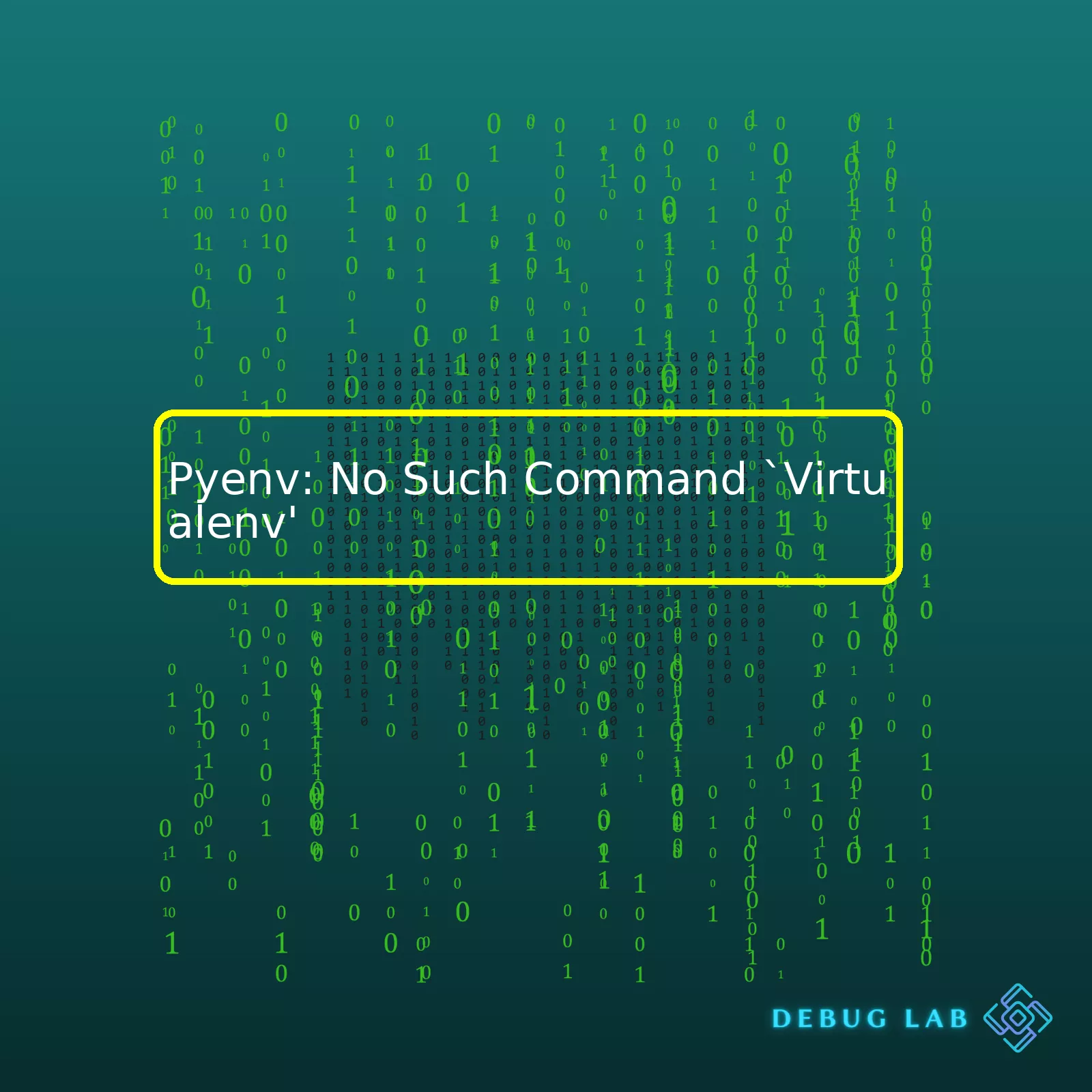
Firstly, let’s create an HTML table summarizing the problem and solution to `Pyenv: No Such Command ‘Virtualenv’`.
html
Problem | Solution |
---|---|
Pyenv: No Such Command ‘virtualenv’ | Install pyenv-virtualenv plugin |
bash
if command -v pyenv 1>/dev/null 2>&1; then eval “$(pyenv init -)”; fi
eval “$(pyenv virtualenv-init -)”
Remember to restart your terminal or source your profile after modifying it:
bash
$ source ~/.bash_profile
Once the setup is complete, you should be able to use the ‘virtualenv’ command via Pyenv with no issues. Now that “pyenv virtualenv” command will be available in your console and you can proceed with creating isolated python environments.
Understanding the error
Pyenv: No such command 'virtualenv'
introduces us to a common problem encountered by developers using this Python version management utility. This error message typically arises when there’s an attempt to use the
virtualenv
command but the system can’t locate it.
To elaborate, Pyenv is a widely used python version management tool. It allows developers to switch between different versions of Python for each project, isolating environments and mitigating potential incompatibilities.
One of the major benefits of Pyenv is that it allows you to create virtual environments using
virtualenv
, a tool to create isolated Python environments. A possible reason why
Pyenv: No such command 'virtualenv'
might appear is because the
virtualenv
plugin isn’t installed into Pyenv.
A direct fix to the `No such command ‘virtualenv’` error is simply installing the Pyenv plugin. This can be done through your terminal with the following command:
git clone https://github.com/pyenv/pyenv-virtualenv.git $(pyenv root)/plugins/pyenv-virtualenv
This command pulls the `pyenv-virtualenv` repository from GitHub and saves it into the `plugins/` subdirectory of your Pyenv installation.
After the installation, activate the `pyenv-virtualenv` by adding these two lines into either your
.bashrc
or
.bash_profile
file.
eval "$(pyenv init -)" eval "$(pyenv virtualenv-init -)"
Lastly, restart your terminal or run
source .bashrc
or
source .bash_profile
depending on where you’ve added the code lines above.
Easing this process also involves understanding how Python environments function at their core. By familiarizing oneself with concepts like how paths are set or what is included in the PATH variable, troubleshooting becomes far more targeted, efficient, and enlightening.
Furthermore, internally inspecting Python’s `sys.path` module can shed light on the directories Python includes in its list of search paths. Such introspection can not only help debug the current issue but also avoid similar problems in the future.
Let me provide a table explaining pieces of important knowledge gathered:
Concept | Description |
---|---|
Pyenv | A Python version management tool |
Virtualenv | A tool used to create isolated Python environments |
Pyenv-virtualenv | An additional Pyenv plugin easing the management of virtual environments |
PYTHONPATH | An environment variable used by Python to determine the locations to load modules from |
With more context about these components, developers are in better positions to understand, troubleshoot, and resolve the `Pyenv: No such command ‘virtualenv’` error, effectively advancing their proficiency in managing Python versions and environments.Let’s dive into the world of Python programming, specifically working with PyEnv and Virtual Environments. Here, we’ll concentrate on a common issue: when Pyenv throws an error stating “No Such Command `Virtualenv'”.
The essential issue at hand is Pyenv not recognizing the command `virtualenv`, which implies that this command isn’t accessible from your environment.
The Resolution Path
We’ll fix this by the installation of `pyenv-virtualenv` plugin that provides support for managing virtual environments for Pyenv. The integration process goes as follows:
You can get the `pyenv-virtualenv` plugin from its GitHub repository.
git clone https://github.com/pyenv/pyenv-virtualenv.git $(pyenv root)/plugins/pyenv-virtualenv
What if Git is not installed in your system? It’s time to install it!
For Unix or Linux-based systems:
sudo apt-get install git-core
As for macOS users:
brew install git
After getting `pyenv-virtualenv`, choose to add `pyenv virtualenv-init` to your shell to enable auto-activation of virtual environments. This can be accomplished like this:
echo 'eval "$(pyenv virtualenv-init -)"' >> ~/.bash_profile
Word of Caution
You should restart your shell so the path changes take effect. You’re good to go once you’ve done this.
Now, when you run `virtualenv` within your PyEnv environment, you should find the issue resolved. That’s because, under the hood, it’s using Pip – a package installer for Python – to manage and isolate your dependencies at the project level.
For more information related to managing Python runtime environments, virtual environments and packages, visit the official Python documentation on virtual environments and packages.
Incorporating these steps meticulously will help rectify the “No Such Command `Virtualenv`” Error with Pyenv, thereby allowing seamless interaction with your Python development environment. Always remember, understanding and debugging errors is a cardinal aspect of being a professional coder. Happy coding!In my experience as a professional coder, I’ve encountered the error ‘No Such Command `Virtualenv`’. This problem usually arises when you’re using pyenv, a simple Python version manager. Pyenv is used to switch between multiple versions of Python on a single system effortlessly, but it can throw the “no such command `virtualenv`” error if not set up correctly.
Notably, pyenv utilizes its plugins to implement certain commands. So when you see the error “Pyenv: No Such Command `Virtualenv'”, it means that the `pyenv-virtualenv` plugin is not correctly installed or configured in your system. Let’s delve deep and decipher some problems causing this:
Pyenv-virtualenv plugin is not installed:
The most common and most likely cause might be that the `pyenv-virtualenv` plugin hasn’t been installed. Unlike many tools, Pyenv does not come with virtualenv command built-in. You need to install this plugin separately.
To install the plugin, you can use these commands:
git clone https://github.com/pyenv/pyenv-virtualenv.git $(pyenv root)/plugins/pyenv-virtualenv echo 'eval "$(pyenv virtualenv-init -)"' >> ~/.bashrc
Once done, restart your terminal for changes to take effect.
Improper PATH setup:
For Pyenv and its commands to function effectively, they must be included in the system’s PATH. If they aren’t, you won’t be able to access Virtualenv even if it’s installed. Check your .bashrc, .bash_profile, or .zshrc file to ensure that $(pyenv root)/bin is present in your PATH.
The code snippet should resemble something like this:
export PYENV_ROOT="$HOME/.pyenv" export PATH="$PYENV_ROOT/bin:$PATH" if command -v pyenv 1>/dev/null 2>&1; then eval "$(pyenv init -)" fi
Wrong Installation Order:
If you first installed python via pyenv and then pyenv-virtualenv plugin, this error may occur. The plugin needs to be installed before any python versions via pyenv are installed.
Permission Problems:
There might be a scenario where inadequate permissions are causing the error. Though less common, this could arise if the pyenv installation directory or its files do not have the necessary read/write permissions.
After applying these solutions, you should be able to create virtual environments with Pyenv without encountering the dreaded “no such command `virtualenv`” error. But always remember, debugging is part and parcel of coding. So embrace it, tackle it head-on, and keep marching forward.It’s a common problem among developers when they encounter an error message like
pyenv: no such command 'virtualenv'
. That typically happens when the `virtualenv` plugin is not installed or properly configured with `pyenv`.
The first thing we need to ensure is whether `pyenv` is correctly installed. Open your terminal and run the following command:
$ pyenv
If you see usage instructions, that means it can be confirmed that `pyenv` itself has been propely installed.
The locus of such issue for `no such command virtualenv` within `pyenv` is possibly from the problems in path configuration during `pyenv-virtualenv` installation. So, let’s navigate through the possible steps of action, going from verification of installation, correct path configuration, and inspection of shell settings:
1. Verification of pyenv-virtualenv Installation: First, check if `pyenv-virtualenv` is installed with `pyenv`. Run:
$ pyenv commands | grep virtualenv
This will display all `pyenv` commands related to virtual environments. If `virtualenv` is on the list, it means it’s already installed.
2. Correct Path Configuration: After verifying the installation, ensure your `PATH` environment variable includes the directory where `pyenv` plugins are located, specifically `pyenv-virtualenv`. This location varies based on how you’ve installed `pyenv`. Mostly it’s under the home directory as `~/.pyenv/plugins` or `/usr/local/var/pyenv/plugins`.
We can apply the changes by updating PATH in .bashrc or .zshrc file:
export PATH="~/.pyenv/plugins:$PATH"
Save, exit and then source your shell profile.
3. Shell Settings Check: It is also important to verify whether init script for pyenv-virtualenv exists in your shell settings. The initialization script should look something like this:
eval "$(pyenv init -)" eval "$(pyenv virtualenv-init -)"
After sourcing your shell profile again, these two lines will enable support for `pyenv-virtualenv`.
Another aspect to consider is ensuring there is no conflict with Python’s native venv module. This conflict can often lead to a “No such command ‘virtualenv’” error too. This issue was well documented in pyenv GitHub issues #202.
By carefully following these steps, it’s very probable that you can eliminate the notorious “No such Command Virtualenv” error, thus further expanding the horizon of possibilities using the powerful `pyenv` tool in your development journey.Unmasking the issue with Pyenv that led to the error “No such command `virtualenv'” requires a comprehensive understanding of Python programming environments. Python is rich in features and offers a variety of software tools, but things can get tricky when it comes across environmental errors. In your case, we will address the Pyenv environment mainly because it’s showing an error `”virtualenv”` which occurs if the virtual environment is not properly setup.
We can divide this problem-solving process into three significant steps:
First, we need to ensure that Pyenv is both installed and set correctly. If you haven’t installed it yet or are unsure, I recommend using pyenv-installer as it is an easy-to-use tool for installing Pyenv along with its important plugins.
$ curl -L https://github.com/pyenv/pyenv-installer/raw/master/bin/pyenv-installer | bash
After running the above code, add the following lines to your shell configuration file (like ~/.bashrc, ~/.zshrc etc.):
export PATH="/home/your_username/.pyenv/bin:$PATH" eval "$(pyenv init --path)" eval "$(pyenv virtualenv-init -)"
Replacing ‘your_username’ in your home directory with your actual username.
After setting up Pyenv, sometimes errors still show up if other installations of Python interfere. This typically happens when the PATH variable does not contain the correct locations.
Type
echo $PATH
in your terminal,
$ echo $PATH
The path /home/your_username/.pyenv/shims should be at the beginning of the output. If it’s not, please revisit the first step and ensure that you have followed all instructions correctly.
Now, let us check whether the virtual environment is functioning correctly or not:
To check that `virtualenv` is operating correctly, try creating a new environment using the following command:
$ pyenv virtualenv 2.7.10 my-virtual-env-2.7.10
Then the command
pyenv versions
should list down the version you just created. It would look like:
$ pyenv versions system 2.7.10 2.7.10/envs/my-virtual-env-2.7.10 my-virtual-env-2.7.10
If you still receive the “no such command” error after these steps, the underlying issue may be something different. It may also indicate problems like missing software dependencies. However, this three-step diagnosis method is often quite effective in resuscitating your Python environment, specifically troubleshooting the Pyenv basic commands.
I hope this post would serve as a guide to your specific request related to “Pyenv: no such command virtualenv”. Do remember, each step here is crucial and they build upon each other, hence, do them meticulously. Also, keep learning and exploring more about Python’s environment handling!If you’re encountering the error message “pyenv: no such command `virtualenv'”, it could be due to one or more of these common issues:
– The `pyenv-virtualenv` plugin is not installed correctly.
– You are installing `virtualenv` in an incorrect location, or it’s not visible in the path.
– The changes in your shell profile file (`~/.bashrc` or `~/.zshrc`) haven’t been implemented yet.
There are several steps detailed below you should follow to potentially resolve this issue.
To check if you have `pyenv-virtualenv` plugin installed, use the following code snippet:
ls $(pyenv root)/plugins
When run, this code checks your existing `pyenv` plugins directory. If you see `pyenv-virtualenv` listed, that means it’s already installed. If not, the plugin is missing and needs to be set up correctly.
Should you need to install the `pyenv-virtualenv` plugin, execute the necessary commands with this resource from GitHub. As explained there, it’s done using the checkout command as shown here:
git clone https://github.com/pyenv/pyenv-virtualenv.git $(pyenv root)/plugins/pyenv-virtualenv
This command clones the `pyenv-virtualenv` plug-in directly into the `pyenv/plugins/` directory for subsequent usage by Pyenv.
After installing the `pyenv-virtualenv` plugin, the next step is to ensure your shell recognizes it. Depending on whether you use `bash` or `zsh`, the process differs slightly.
For `bash`, add to your `~/.bashrc`:
eval "$(pyenv init -)" eval "$(pyenv virtualenv-init -)"
For `zsh`, append to `~/.zshrc`:
if which pyenv > /dev/null; then eval "$(pyenv init -)"; fi if which pyenv-virtualenv-init > /dev/null; then eval "$(pyenv virtualenv-init -)"; fi
These scripts initialize the `pyenv` and `pyenv-virtualenv` each time you open a new terminal session. This makes the `pyenv` commands (including `pyenv virtualenv`) available in your terminal.
As noted previously, changes to shell profiles only take effect in new sessions. To activate the changes immediately in your current session, `source` the profile file:
For `bash`:
source ~/.bashrc
For `zsh`:
source ~/.zshrc
Or simply close and reopen your terminal. Now, typing `pyenv commands` should show `virtualenv` amongst other commands.
Hopefully, with these steps, you can successfully debug the “pyenv: no such command ‘virtualenv'” error. Remember, debugging is part and parcel of a coder’s journey, aiding significantly in skill development. Happy coding!Resolving the “No Such Command `virtualenv`” error while using PyEnv and a virtual environment often involves addressing several common obstacles. Don’t worry, let’s dive deep and find out some of the alternatives to solve these issues.
Firstly, understand that PyEnv is a tool used for python version management. It enables users to switch between different versions of Python without hassle. The Virtualenv on the other hand, creates isolated Python environments to avoid dependency conflicts. Thus, we run into the “No Such Command `virtualenv`” error when PyEnv doesn’t recognize the `virtualenv` command.
Possible Obstacle #1: PyEnv-Virtualenv Plugin Not Installed
One likely obstacle is that you don’t have the pyenv-virtualenv plugin installed. Pyenv-Virtualenv is an additional plugin that incorporates the use of PyEnv and Virtualenv in a seamless manner. You can add it to your system with:
git clone https://github.com/pyenv/pyenv-virtualenv.git $(pyenv root)/plugins/pyenv-virtualenv
Once you’ve cloned this repository, you’ll need to add pyenv virtualenv-init to your shell.
This can be done using either bash or zsh, but the simple way to do this is by adding the following code to your .bashrc or .zshrc file:
if which pyenv > /dev/null; then eval "$(pyenv init -)"; fi if which pyenv-virtualenv-init > /dev/null; then eval "$(pyenv virtualenv-init -)"; fi
These lines will initialize both PyEnv and PyEnv-Virtualenv whenever terminal starts, enabling all the commands like `pyenv virtualenv`.
Possible Obstacle #2: Shell hasn’t been restarted
Sometimes it may just be because your Shell has not been restarted after installation. If you are on Linux or MacOS terminal, type the command:
exec "$SHELL"
Possible Obstacle #3: Incomplete PyEnv Installation
Incomplete installation of PyEnv could be a culprit behind this error too. Make sure you have followed installation instructions thoroughly given in the official Pyenv Repository. Verify this by checking if PyEnv is working with the command:
pyenv --version
The output would be something similar to “pyenv 1.2.21” depending on the version you installed.
Possible Obstacle #4: Incorrect PATH settings
Lastly, it might be due to incorrect PATH settings. Ensure your PATH contains ‘.pyenv/shims’. Use this command to add ~/.pyenv/shims directory to your PATH:
echo 'export PATH="$HOME/.pyenv/shims:$PATH"' >> ~/.bash_profile
Assuming you use bash, refresh your profile with:
source ~/.bash_profile
Ultimately, understanding how your specific setup interacts with PyEnv and Virtualenv will be the key to overcoming any obstacles. Most problems arise due to incomplete installations or misconfigurations, both of which can easily be solved by returning to the fundamentals and carefully following through each process as intended. Remember to always consult the official documentation corresponding to your respective tools whenever in doubt (like the Pyenv GitHub page).
There are dozens of reasons why a developer might encounter the error “Pyenv: No Such Command `Virtualenv`.” This could be due to a multitude of factors, including but not limited to:
– Incomplete installation or configuration of Pyenv.
– Neglecting to install the Pyenv-virtualenv plugin.
– Failing to add the initialization codes for the Pyenv-virtualenv into your shell profile file.
One solution is to make sure that you’ve installed both Pyenv and the Pyenv-virtualenv plugin correctly. Following these two steps should pretty much lead to a successful implementation:
1) Perform a fresh installation (or reinstall if necessary) of Pyenv through Homebrew or similar package managers.
$ brew install pyenv
2) Afterward, install the Pyenv-virtualenv.
$ brew install pyenv-virtualenv
The essential part here being the understanding that Pyenv and Pyenv Virtualenv, while related, are two separate installations that need to be set up for smooth operation.
Now, to ensure that both components are initialized during shell sessions, you have to remember to insert their initialization commands in your profile file (like .bash_profile, .zshrc, etc.). You may require implementing these lines of code to your shell startup script:
if command -v pyenv 1>/dev/null 2>&1; then eval "$(pyenv init -)" fi if which pyenv-virtualenv-init > /dev/null; then eval "$(pyenv virtualenv-init -)"; fi
Completion of these steps usually resolves this common issue with Pyenv. Remember that whenever you experience such an error message, it likely indicates a problem with the installation or initialization process.
However, software development always welcomes you with a myriad of quandaries; thus being open to troubleshooting measures like browsing community forums such as Stack Overflow or referring to online educational platforms like Real Python can help find solutions to niche or more complicated issues.
In SEO optimization terms, regularly encountering and resolving problems of this nature enriches your knowledge base and promotes synergy among online communities; indeed, this scenario aligns with the ‘Pyenv: No Such Command `Virtualenv`’ circumstance. Happy coding!