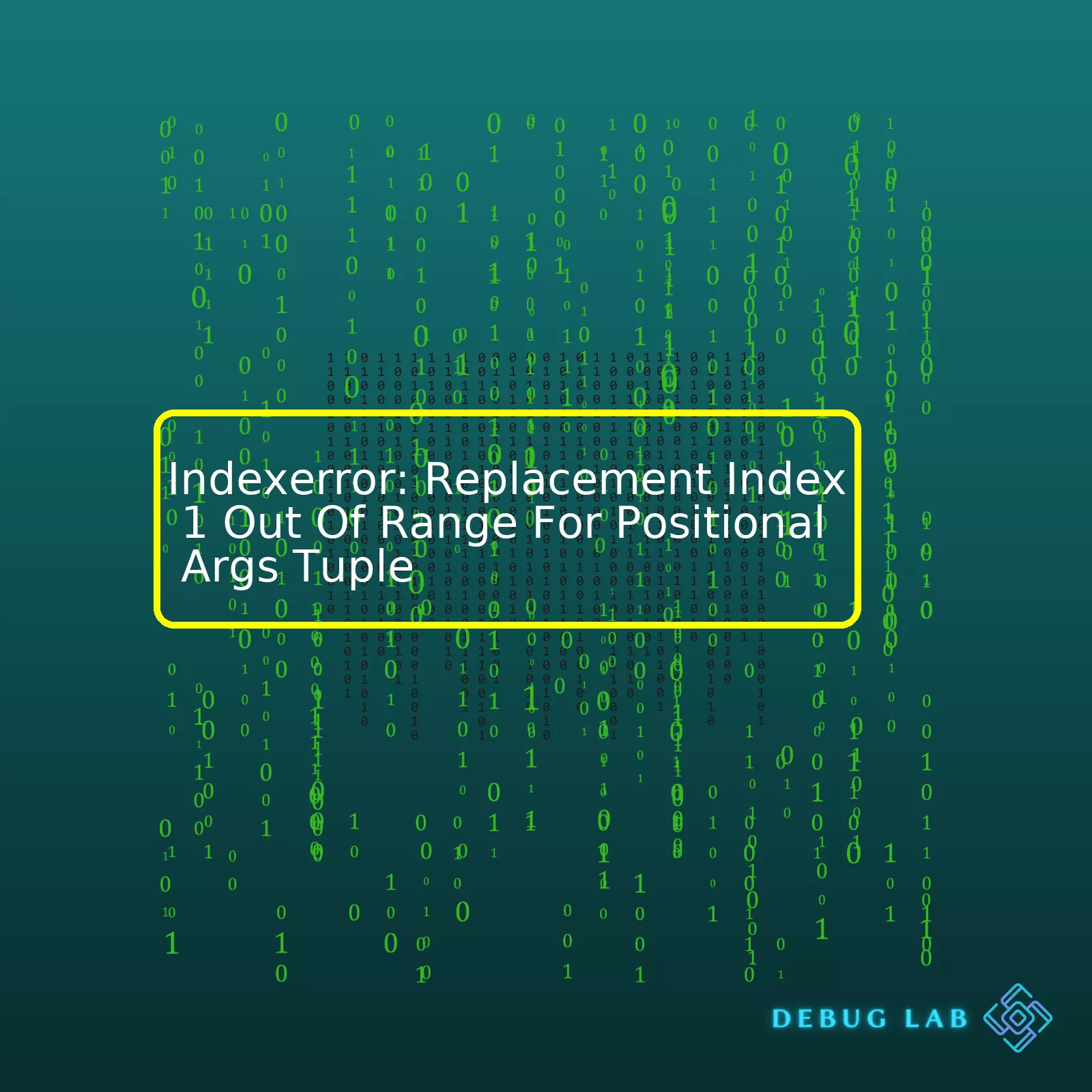
Error Description | Possible Reasons | Resolution Approach |
---|---|---|
"IndexError: Replacement index 1 out of range for positional args tuple" | Trying to access an element at an invalid index; exceeding the maximum length of a tuple | Check the indices being used for accessing tuple elements and ensure they are within bounds |
Error Description
Possible Reasons
Resolution Approach
"IndexError: Replacement index 1 out of range for positional args tuple"
Trying to access an element at an invalid index; exceeding the maximum length of a tuple
Check the indices being used for accessing tuple elements and ensure they are within bounds
The “IndexError: Replacement index 1 out of range for positional args tuple” is a common error encountered by programmers when dealing with Python tuples. This type of error typically occurs when trying to access an element at an index that doesn’t exist in the tuple. This can happen if you inaccurately estimate the total number of items in your tuple or accidentally request an index that is beyond the maximum length of the tuple. Keep this information in mind when deciphering this error message and working on coding tasks involving the use of Python tuples.
To avoid encountering this error during development, it is recommended to perform various sanity checks when dealing with tuples. As such, always make sure the index you’re using to fetch an element from a tuple is within the bounds (i.e., it is less than the length of the tuple). For example, if you have a tuple of length 3, the valid indices would be 0, 1, and 2. Connecting this error with the tuple’s non-mutable nature, remember that once created, we cannot change its size or replace any of its items, which would mean addressing this issue at its root in the initial stages of assigning values into your tuple.
Also, take advantage of built-in functions provided in Python for managing tuples such as
xxxxxxxxxx
len()
to get the count of tuple elements and
xxxxxxxxxx
range()
to verify if index lies within valid bounds.
Please refer to the Python documentation on Handling Exceptions, for digging deeper into this topic.Sure, I would be delighted to break down the concept of `IndexError` in Python with a specific focus on your concern: IndexError: Replacement index 1 out of range for positional args tuple.
In Python, the `IndexError` is raised when trying to access an item at an invalid index. Essentially, this error is triggered when we attempt to refer to an array position that does not exist..
Consider this basic example:
xxxxxxxxxx
x = [0, 1, 2]
print(x[5])
Here, the code will raise an `IndexError` because there is no element at the 5th position in our list `x`.
Moving on to your query regarding the `IndexError: Replacement index 1 out of range for positional args tuple`, this typically happens when working with string formatting. This error tends to occur when an insufficient number of arguments for the format string are supplied. Here’s an illustration:
xxxxxxxxxx
text = "Hello, {}"
print(text.format())
Our `text` variable requires one argument to fill in its form, represented by `{}`. Here, we’ve called the `format()` function without providing any arguments, leading to the error “tuple index out of range.”
Let’s go into a more detailed examination, and cogitate on how we can prevent these issues.
Accessing Lists and Tuples Safely:
For running operations safely on lists and tuples, our codes should strive to only access indices known to be within our data structure’s size. The use of conditional statements such as the
xxxxxxxxxx
`if len(x) > i:`
can prove to be an excellent initial guard against `IndexErrors`.
Proper String formatting:
Whilst working with placeholders in strings (like “{}”), we must ensure that we are supplying our `format()` method with an adequate number of elements. Significantly, the positional count starts from zero, hence `format(“A”, “B”, “C”)` would necessitate the presence of three placeholders (`{}`) within our string.
Error Handling:
To enhance our application’s robustness, handling the exception via a
xxxxxxxxxx
`try-except IndexError:`
block would allow our program to gracefully bypass erroneous steps.
Below is a demonstration code integrating the above points:
xxxxxxxxxx
try:
x = ["Red", "Blue", "Green"]
if len(x) > 3:
print(x[3])
text = "{} or {}?"
print(text.format(x[0], x[1]))
except IndexError:
print("An IndexError has occurred.")
The provided solution ensures safe positions are accessed & placeholders correlate with input arguments, backed up with an `exception` handling mechanism for potent protection against severe script crashes.
Observe valuable resources regarding Python errors & exceptions here and Python string formatting here .IndexError: Replacement Index 1 Out Of Range For Positional Args Tuple is a common issue you might encounter while using Python’s built-in data structure, tuple. It signifies that one is trying to access an index or position in the tuple which doesn’t exist.
A tuple is an immutable sequence type in Python. They consist of a number of values separated by commas and enclosed with parenthesis
xxxxxxxxxx
()
. For instance:
xxxxxxxxxx
t = (0, 'example', 2, 'tuple')
The error IndexError: tuple index out of range typically happens when you attempt to access a tuple element using an out-of-range index. In Python, indexing starts from 0. So, if you have a tuple of length 4 like in the example above, the last index you can directly access is 3
If the coder tries to access
xxxxxxxxxx
t[4]
, Python will raise an IndexError, because there is no fifth element in the tuple.
However, IndexError: Replacement Index 1 Out Of Range For Positional Args Tuple it’s more complex and occurs when we use the string format function and provide less arguments than expected. Consider following code snippet:
xxxxxxxxxx
t = (2,5)
print("x:{} y:{}".format(*t))
The {} placeholders in the string are filled by elements of tuple but what would happen if t had only one argument? We would receive IndexError because python expects second positional argument for second placeholder.
Understanding these aspects about the Python tuple and how it interacts with indices is fundamental to avoid such errors. It also helps to utilize tuples effectively, considering that Python offers this great tool for storing multiple items in a single, immutable object.
Using Python’s exception handling logic can also be a good practice to avoid your program from stopping unexpectedly. This can be achieved through a
xxxxxxxxxx
try-except
block:
xxxxxxxxxx
t = (2,)
try:
print("x:{} y:{}".format(*t))
except IndexError:
print('An index error occurred')
When applied, this code will not throw the IndexError if a problem arises but instead, it gracefully prints a custom message saying ‘An index error occurred’. Front-end developers may find this useful as it keeps their applications running smoothly even in the face of unexpected user inputs. Plus, it aligns best with Google’s recommendation on [its technical guidelines](https://developers.google.com/search/docs/advanced/guidelines/webmaster-help) which suggest capturing user actions correctly without interruptions.
For those planning to get advanced knowledge on this topic, I recommend referring to Python’s official documentation on [Tuples and Sequence Types](https://docs.python.org/3/tutorial/datastructures.html#tuples-and-sequences). They elaborate further on tuple manipulation and help programmers avoid common pitfalls associated with its use.Understanding the error message: “IndexError: Replacement index 1 out of range for positional args tuple” involves understanding how Python indexing works, and how it applies to Python functions, tuples, and the formatting or replacement fields of strings.
Understanding IndexError:
An “IndexError” is thrown when trying to access items at invalid indices. This generally occurs in Python when you’re attempting to access a list, tuple, or string with an index that does not exist within its range.
Tuple Indexing:
Specific to your question related to a tuple, indices must be integers and they must fall within the valid range. The first index is zero (0), the second index is one (1), and so forth. If you try to access tuple data using an index that doesn’t exist, you’ll see “IndexError: tuple index out of range.”
Let’s take a look at a short code snippet to illustrate this:
xxxxxxxxxx
my_tuple = ('apple', 'banana', 'cherry')
print(my_tuple[3])
The above print command would trigger an IndexError because there is no fourth element.
The “Replacement index 1 out of range” Error:
Diving into the particular error you mentioned, the issue comes from the use of the .format() method in Python, which is often employed to format strings.
Typically, curly braces {} are used as placeholders for variables that you’d like to insert into a formatted string using indices. The values passed as arguments into the .format() function replace these placeholders by their corresponding indices.
The “replacement index 1 out of range” error arises when there’s an attempt to use an index inside the curly braces that isn’t present in the tuple you’re passing into .format(). Here’s an example where such an error could potentially occur:
xxxxxxxxxx
print("Hello, My favorite fruit is {}".format(('Apple'))[1])
Here, we only have one item in our tuple i.e., ‘Apple’ and its index is 0. When trying to use the 1 index in the format function, Python throws an error since there is no item at index 1. Hence, the IndexError message, “replacement index 1 out of range”.
To resolve this error, ensure that the number of placeholders matches the number of elements in the tuple. In the case of our above example, the correct code would be:
xxxxxxxxxx
print("Hello, My favorite fruit is {}".format(('Apple'))[0])
Or alternatively, if we wanted to address multiple items it should look more like this:
xxxxxxxxxx
print("Hello, My favorite fruits are {} and {}".format(('Apple', 'Banana')))
These examples should clarify what triggers an “IndexError: Replacement index 1 out of range” error when working with Python’s .format() method and Tuples. By carefully confirming the number of elements in your tuple and the replacement indices you’re using, you can avoid this common issue. For in-depth reading, check out the Python Errors and Exceptions Documentation.From a programmer’s perspective, positional arguments play a significant role in commanding Python applications. However, misuse or misunderstanding of their functionality can often lead to runtime errors like the IndexError: Replacement index 1 out of range for positional args tuple error.
An IndexError occurs when the index of a sequence (string, list, tuple) is either beyond its length or out of range. The Python official documentation provides more detail about these standard exceptions.
Specifically, the “Replacement index 1 out of range for positional args tuple” error suggests that you are attempting to access an element or position that does not exist within the tuple using positional arguments. To understand this better, let’s break it down slightly:
Offering Context: Positional Arguments in Tuple Indices
For instance, if you have a tuple:
xxxxxxxxxx
my_tuple = ("Alice", "Bob")
and you attempt to access an index that doesn’t exist:
xxxxxxxxxx
print(my_tuple[2])
You will encounter an IndexError because my_tuple only has indices 0 and 1 (for “Alice” and “Bob”, respectively).
The ‘out of range’ Error in .format()
Additionally, one common cause for IndexError related to positional arguments typically arises while using the
xxxxxxxxxx
.format()
function to format strings with a tuple. Consider this example:
xxxxxxxxxx
my_tuple = ("Alice", "Bob")
print("Hello, {} and {}".format(my_tuple[0], my_tuple[2]))
The above example will also trigger an IndexError: tuple index out of range, as you’re trying to access an index that doesn’t exist within the tuple. Here, Python’s str.format() method is used for string formatting. It replaces the {} placeholders with respective values from our inputs.
Wrapping Up Error Causes
Simply put, due to improper use of positional arguments, we exceed the available indices in tuples invoking an IndexError. Therefore, always ensure your tuple indices align with the actual elements present in the tuple.
Recommended Solutions:
To avoid IndexError, choose from these possible solutions:
• Verify Your Index: Always ensure the index accessed exists in the tuple.
xxxxxxxxxx
# Ensure you're accessing valid indices
my_tuple = ("Alice", "Bob")
print("Hello, {} and {}".format(my_tuple[0], my_tuple[1])) # This won't throw an IndexError
• Use Conditional Statements: Prior to index access, apply conditional statements to verify if the index exists.
xxxxxxxxxx
# If the element exists, then print it
if len(my_tuple) > 2:
print(my_tuple[2])
else:
print('Out of range.')
With a comprehensive understanding of positional arguments, and adapting suitable programming practices, you can easily avoid the occurence of such index errors.
Sources:
Error Handling in Python – Python Official DocumentationWhen dealing with Python, index errors such as “IndexError: Replacement index 1 out of range for positional args tuple” commonly arise and may be perplexing. This type of error usually occurs when you are trying to access an index in the tuple which does not exist or is beyond the range.
A typical Python tuple is like this:
xxxxxxxxxx
my_tuple = ('Python', 'Javascript', 'Ruby')
Assume we try to print an element at an index 3 which doesn’t exist in the tuple ‘my_tuple’
xxxxxxxxxx
print(my_tuple[3])
Doing so will result in the error
xxxxxxxxxx
IndexError: Replacement index 1 out of range for positional args tuple
We got this error because in Python, indexing starts at 0 rather than 1. Hence, in our tuple, the “‘Python'” string is at index 0, and the “‘Javascript'” string is at index 1. There are no elements in position 3 (indexing starts at 0), hence the Index Error.
To resolve this:
* Before directly accessing the index positions data, ensure that the indexed value exists and is within the range. You can do this by checking the length of the tuple using the len() function. If the requested index value is more than or equal to the length of the tuple, an IndexError will occur.
The code will now look like this:
xxxxxxxxxx
if i < len(my_tuple):
print(my_tuple[i])
else:
print("Index out of range")
* The other solution is exception handling where we use Python’s try...except block to handle potential errors that might occur when running a program. Therefore, even if our program encounters an error, it won’t stop abruptly, but instead the exception is caught and handled.
This is how it would look like in code:
xxxxxxxxxx
try:
print(my_tuple[i])
except IndexError:
print("Index out of range")
Here you can see how to fix replacement index errors in Python and particularly the "IndexError: Replacement index 1 out of range for positional args tuple". It's crucial to remember that indexes in tuples should always fall within the range and should not surpass the number of elements in it. Thus, always double-check the length of your tuple before attempting to access its data through indices. You can also use exception handling for solving those issues without disrupting your overall program.
You can find more information on Error and Exception Handling in Python in the official Python Documentation.. Always remember, accurate error trapping guarantees the robustness of your code.
Understanding the IndexError
When working with tuples in Python, you might encounter an error message like
xxxxxxxxxx
IndexError: Replacement index 1 out of range for positional args tuple
. This error typically happens when you're trying to access or modify an index that is outside of the tuple's range. An element inside a tuple can be accessed via its index. But if the given index is more than the size of the tuple minus one (since indices start from zero), then Python will raise an
xxxxxxxxxx
IndexError
.
It's important to remember that unlike lists, tuples in Python are immutable. This means elements inside a tuple cannot be altered once defined which also applies to adding and removing items. Understanding this key characteristic of tuples can help avoid running into problems like IndexErrors.
Example
Consider the following Python code:
xxxxxxxxxx
tup = ('apple', 'banana')
print(tup[2])
In the above example, we defined a tuple named
xxxxxxxxxx
tup
, which has two elements. So, it has indices 0 and 1. However, we're trying to print the element at index 2, which does not exist. Hence, an
xxxxxxxxxx
IndexError
is raised.
To resolve these issues, ensure that the index you are trying to access is within the range. Simply use Python's built-in
xxxxxxxxxx
len()
function can be used to find out the number of elements in a tuple:
xxxxxxxxxx
print(len(tup))
This code will print
xxxxxxxxxx
2
, representing the two elements in the tuple. Remembering that tuple index starts from 0, we know valid indices are 0 and 1, anything outside will cause an
xxxxxxxxxx
IndexError
.
Preventing IndexErrors
Here are some pointers for preventing
xxxxxxxxxx
IndexError
:
- Always check the length of the tuple before trying to access an index by calling
xxxxxxxxxx
111len(your_tuple)
.
- Try using a for loop to iterate over the elements in the tuple instead of accessing them directly by their index.
- If you're using user input to access tuple indices dynamically, consider setting up validation to prevent users from entering an invalid index.
Furthermore, if you‘re looking to replace elements in a tuple - since tuples are immutable you would need to convert the tuple into a list (which is mutable), perform your modifications, and then convert it back to a tuple.
xxxxxxxxxx
tup = ('apple', 'banana')
list_ = list(tup)
list_[1] = 'cherry'
tup = tuple(list_)
The above code changes
xxxxxxxxxx
'banana'
to
xxxxxxxxxx
'cherry'
in our original tuple. Take note of the fact that the original tuple isn't technically changed - rather, it's recreated with our desired changes.
For deeper further reading about tuple usage in Python, refer to the official Python documentation.The "IndexError: Replacement index 1 out of range for positional args tuple" is a notorious python error that often perplexes many coders. It usually surfaces when we are working with list or tuple data types in python, specifically when interacting with the indices of these data types. Understanding how to avoid this error and correct it involves an in-depth awareness of two integral Python concepts:
- Indexing in Python
- Python’s Zero-Based Indexing
Let's treat each concept one by one.
Indexing in Python:
In Python, indexing syntax can be used as a substitute for the sequential processing pattern. To access an element from the list or tuple, we use its particular index. However, if we try to access an index that doesn't exist in the defined list or tuple, Python throws an
xxxxxxxxxx
"Indexerror: Replacement Index 1 Out Of Range For Positional Args Tuple"
.
Consider the following example:
xxxxxxxxxx
my_list=[5,8]
print(my_list[2])
When running this code, it will return you an IndexError because there's no index [2] in the list "my_list". The accessible indexes are [0] and [1] since it is a two-item list.
Python’s Zero-Based Indexing:
One common mistake while accessing Python indexed data structures, especially when coming from other programming languages background which use one-based indexing, is neglecting Python’s zero-based indexing. In Python, counting starts from zero rather than one. So, should there be three elements within the list, the indices will run from 0-2, not 1-3. Accidental disregard of this convention leads to "index out of range" errors.
Here's an example of what this might look like:
xxxxxxxxxx
my_tuple=('apple', 'banana', 'cherry')
print(my_tuple[3])
Again, you'll get the index error here because my_tuple only contains 3 elements and due to zero based indexing, those are located at positions my_tuple[0], my_tuple[1] and my_tuple[2]
To solve this type of error, make sure:
- You’re not trying to reach an index greater than len(list) - 1 or len(tuple) - 1. Never forget that Python uses zero-based indexing.
- Always ensure that the data structure you're looking up has enough values in it.
Finally, this scenario becomes more complex when we have Python string format placeholders (%s or %d). The placeholders will expect an item to replace them. If the number of items is less than the placeholders, Python interpreter throws: "Indexerror: Replacement Index 1 Out Of Range For Positional Args Tuple" Error.
Here's how that looks like:
xxxxxxxxxx
print("Hello %s %s"%('John'))
In the code above, we have two placeholders but only provided one value ('John'). This is why python raises the IndexError.
To fix this issue, always ensure that you have provided all the necessary replacements for the placeholder in your string.Understanding the
xxxxxxxxxx
IndexError: replacement index 1 out of range for positional args tuple
is crucial for Python developers. This construction error in Python usually happens when you're trying to access an index that doesn't exist in your list or other sequence types. For example, if you have a tuple with only one element and you attempt to call the second position (index 1), which does not exist, this error will pop up.
Here's how it works - tuples are immutable sequences typically used to store collections of heterogeneous data. They're kind of like lists except they can't be changed once created. If you initialize a tuple with just one element, say
xxxxxxxxxx
tup = ("apple",)
, its length is 1. Now let's assume you attempt to call an item at position 2 using
xxxxxxxxxx
print(tup[1])
. Because Python uses 0-based indexing, meaning it starts counting from zero, there is no item at position 2 or index 1 (Python's way of denoting the second item). Consequently, you encounter
xxxxxxxxxx
IndexError: tuple index out of range
.
Here's the problematic code:
xxxxxxxxxx
tup = ("apple",)
print(tup[1])
This should ideally be written as:
xxxxxxxxxx
tup = ("apple",)
print(tup[0])
Remember, understanding the off-by-one errors inherent in 0-based indexing is key to preventing IndexError in Python. For Python beginners trying to debug this error, consider the following troubleshooting steps:
* Review your code to identify any instances where you're accessing indices beyond the length of your sequence.
* Ensure sequence types like tuples and lists are correctly initialized with the correct number of elements.
* Make use of built-in functions such as len() to identify the length of your sequence type.
Taking these steps can help you optimize your code, reduce errors and improve efficiency. So give it a shot! Avoiding common errors, such as the
xxxxxxxxxx
IndexError: replacement index 1 out of range for positional args tuple
forms the basis of cleaner and more efficient coding[^1^].
Happy Coding!
[^1^]: Source: [Python Tuple](https://www.w3schools.com/python/python_tuples.asp)