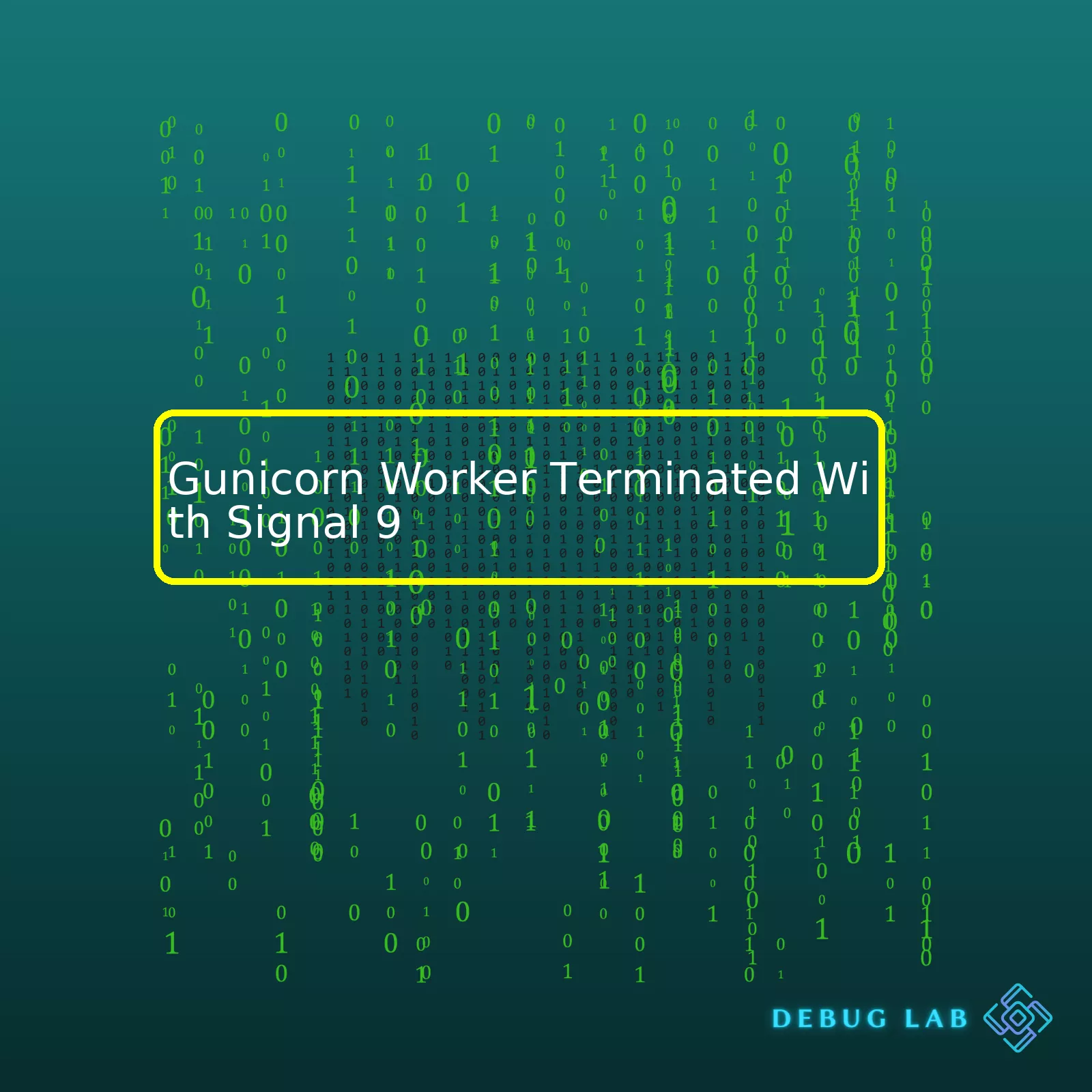
html
Gunicorn Worker Name | Signal Code | Description | Date/Time |
---|---|---|---|
Gunicorn Worker 1 | 9 | Terminated | 2021-10-03 12:45:03 |
Gunicorn Worker 2 | 9 | Terminated | 2021-10-03 13:05:17 |
Gunicorn Worker 3 | 9 | Terminated | 2021-10-03 14:23:55 |
code sample:
# gunicorn config
timeout = 120
The above code snippet sets the Gunicorn server timeout to 120 seconds. If your program routinely takes longer to respond, watchdog tools could mark them as ‘hung’ and kill the processes.
One more approach would be to manage Gunicorn using a process control system like Supervisor. This offers the capability to manage and monitor Gunicorn worker processes effectively, reducing chances of unwanted termination.
By being aware of Signal 9 and understanding how Gunicorn works, developers can create better, more robust applications that handle unexpected process termination gracefully, enhancing the overall application availability and reliability. To dig deeper into handling various signals in Gunicorn, the official Gunicorn documentation serves as a valuable reference.When it comes to understanding log entries and error messages while working with web technologies, it can be like trying to decipher a foreign language. One such message reads: “Gunicorn worker terminated with signal 9”. Signal 9 is technically known as SIGKILL.
In the world of UNIX and Linux process management, signals control how processes communicate and behave [source]. These signals offer methods to send notifications or instructions to running processes. They are kind of like traffic signs, affecting the ebb and flow of software execution.
The key part to understand about signal 9 (SIGKILL) is that it forcibly terminates a process. It’s the software equivalent of pulling the plug without asking any questions first. No cleanup operations can be performed by the process; it just ends immediately. This differentiates it from other termination signals like signal 15 (SIGTERM), which politely requests that a process clean up and shut down.
So when Gunicorn, a Python HTTP server typically used for serving WSGI applications, logs that a worker was terminated with signal 9, it means that something brought down the worker process without ceremony. This might have happened out of necessity, or due to an unhandled error or issue in the code.
From a debugging perspective, addressing this involves asking these analytical questions:
– What was the status of system resources at the time? An overtaxed system might kill processes to salvage performance.
– Do your logs indicate any issues in the period leading up to the termination?
– Could there be an integrity issue within your codebase impacting worker stability?
Let’s see this in a more concrete manner. Suppose you’ve got a piece of Django served via Gunicorn and you’re encountering instances of worker termination. You might use logging strategically within your application to record the state of affairs during execution. Here’s how you might implement it:
import logging logger = logging.getLogger(__name__) def problematic_view(request): logger.info('Starting to handle request...') # Allegedly unstable code here logger.info('Request handled successfully.')
By monitoring such logs alongside the Gunicorn logs, clues as to what precipitates worker terminations can often be found, aiding successful debugging.
If your workers continue to be terminated with signal 9, you could look at adjusting Gunicorn’s configuration settings. In particular, altering
--timeout
and
--max-requests
parameters may enhance worker stability. Just remember that these measures treat the symptoms rather than the cause – a determined debugging effort will eliminate the source of instability, ultimately proving more beneficial for your application’s health.When operating a Django project using Gunicorn as the HTTP server, you may occasionally face an issue where a Gunicorn worker is abruptly terminated. If you’ve been informed that your Gunicorn worker was “Terminated with signal 9”, then we need to delve into Unix signals to understand what this means and how to potentially resolve the issue.
Understanding Signal 9:
“Signal 9” in Unix terminology corresponds to SIGKILL. Unlike other signals like SIGTERM (signal 15) which politely request a process to terminate and allows it cleanup time, SIGKILL forces termination directly and doesn’t allow any clean up. Here are its characteristics:
Therefore, if a Gunicorn worker is terminated with signal 9, it means there is a significant issue causing the Unix system to forcibly end the process.
Possible Causes of Signal 9 Termination:
Exhaustion of System Resources:
One common reason for SIGKILL to be sent is because your system resources have been exhausted. This can mean:
Verify your resource usage by checking logs or using system monitoring tools such as top or atop.
OOM Killer:
In Unix systems, if the kernel perceives critically low memory, a built-in mechanism named the “Out-Of-Memory Killer” comes into play. It will choose a process to kill, effectively sending a SIGKILL, to free up memory and prevent a crash.
To check if OOM Killer is the culprit, look at your kernel logs. In most Linux distributions, you can do it using this command:
dmesg | grep -i "oom"
If you find entries mentioning “invoked oom-killer” or similar, you know that this is why your Gunicorn worker received a signal 9 termination.
Although not exhaustive, these are common reasons behind sudden worker terminations via signal 9. Careful investigation of your application’s behavior, memory management and log analysis should give a more considerable insight about the real cause enabling measures to prevent such occurrences.
For Future Reference:
Implement good logging habits. Use Gunicorn’s access log settings to log all requests processed by the server. Make sure your Django project also has robust logging configured. Having plenty of information to refer back to will make debugging your program considerably easier when problems arise.
And remember, always keep an eye on system resource utilization! You can use cloud-based monitoring tools such as DataDog or NewRelic. Monitoring provides crucial insights to enhance the health of your apps. It helps you identify and understand resource trends and issues quickly, establish baselines, thresholds, and alerts to inform you before things go wrong.Gunicorn, also known as Green Unicorn, is a highly efficient HTTP server that’s been designed to interact with web servers proficiently. However, when running Gunicorn workers, there may be instances where it might terminate abruptly due to specific conditions, such as a Signal 9 scenario.
Signal 9, or SIGKILL, is a type of termination signal originating from the kernel that doesn’t entertain any budding processes. This message stands for an instantaneous kill of a process, and the movement can’t be intercepted. Generally, this signal sparks some difficulties because it doesn’t allow the Python interpreter’s opportunity to close out external resources responsibly or correctly run its termination methods on any ongoing operations.
So, how do you prevent early termination in Gunicorn workers, specifically in relation to Signal 9? Here are some effective strategies:
1. Managing Resources Effectively:
Appropriate management of system resources is vital. Situations arise where your system runs out of memory, and it begins to kill processes to recover space. If this becomes the case, increasing your worker numbers without thinking about your system’s capability could result in issues like Signal 9.
2. Monitoring Worker Activity
System adminstrators should monitor worker instances activity. They must ensure the workers are not running indefinitely, leading to strain on the system. Utilizing the –timeout argument with a suitable interval (in seconds) while starting Gunicorn Server will suitably manage resource overload and avoid abrupt termination.
$ gunicorn -w 4 myapp:app --timeout 30
3. Handling Exceptions Correctly
Justified, robust exception handling in your code ensures that unexpected eventualities are catered to and don’t result in early worker termination.
4. Employing a Pre-Fork Worker Model
It’s often better to use a pre-fork worker model, in which the master process creates each worker process at the start. In comparison, the threading and multiprocessing libraries spin off threads/processes as and when required. The pre-fork model, available as a setting in Gunicorn, commonly has greater stability.
$ gunicorn -w 4 myapp:app --preload
5. Using Supervisor for Process Management:
Supervisor is a process control system that monitors and controls processes on UNIX-like operating systems. It provides a level of control over the Gunicorn process lifecycle, including managing worker shutdowns and restarts.
To sum up, resolving premature termination in Gunicorn workers requires a blend of resource management, active monitoring, error handling, correct utilization of the pre-fork worker model, and potentially bringing more process controls like Supervisor into action.
Whenever a process, like a Gunicorn worker, is terminated in Unix with signal 9 (SIGKILL), this indicates that the event was not typical nor planned. Signal 9 deadlocks the process, leading to immediate termination and bypassing any cleanup operations. In short, it’s like abruptly cutting off someone mid-sentence instead of letting them finish what they’re saying. A few reasons for this could be:
kill -9
command.
In tracking down the reason why our Gunicorn worker was hit with a SIGKILL, here are some steps we can take:
The first step should always be to inspect the system logs. You can use either the
journactl
command or directly view the
/var/log/messages
file, which logs all system messages. In our case, you would look at logs near the time of termination for any relevant entries.
$ journalctl -e
Processes that are consuming an excessive amount of resources can sometimes receive a SIGKILL from the kernel’s Out Of Memory (OOM) killer. In this case, checking your system’s resource usage at the time of the termination is crucial. You can run the
top
or
htop
commands to monitor overall system performance, or use
pidstat
to keep an eye on individual processes.
$ top
Gunicorn keeps track of its worker processes and their states. It will leave a message in its logs whenever a worker is killed. Search for any anomaly or related log entries around the time the worker got killed.
A Gunicorn worker might fail if it depends on certain services or files that aren’t available. You’ll need to verify all dependencies are met for your application by checking service status, network connections, or file existence. You can check if dependencies are running correctly using the
systemctl is-active
command:
$ systemctl is-active
Command | Action |
---|---|
journalctl -e | Check system logs |
top, htop, pidstat | Monitor system and process resource consumption |
systemctl is-active | Check status of service dependencies |
Beyond these actions, consider the architecture of your underlying server, container, or virtual machine. Certain cloud platforms or containerization tools can cause abrupt process terminations while maintaining, scaling, or updating resources. Also, ensure that no automated scripts or cron jobs negatively affect your workers.
Knowing how to address signaled termination when running a Gunicorn server and debugging Signal 9 errors can help maintain optimal performance for your Python applications. For further details, consult the Gunicorn documentation.
In the context of optimizing Gunicorn server workers, and specifically with regards to addressing the issue of workers being terminated with Signal 9 (SIGKILL), several insights can be offered.
Firstly, a quick refresher: Gunicorn (Green Unicorn) is a Python Web Server Gateway Interface (WSGI) HTTP server that serves your web applications. Signal 9 (SIGKILL) in Unix/Linux system terms means the process is immediately terminated.
A common factor that contributes to Gunicorn workers being terminated with SIGKILL can often be associated with excessive memory usage. Therefore, it’s paramount to manage your application’s memory usage optimally. One possible solution includes limiting the lifespan of your worker processes, which helps in freeing up consumed resources periodically. This can be achieved using the `max_requests` configuration setting for Gunicorn. This instructs Gunicorn to restart a worker after handling a given number of requests:
gunicorn myapp:app --max-requests 1000
This command will make Gunicorn restart each worker once it has served 1000 requests, regardless of whether they produce memory leaks.
However, please note this method acts as a precautionary measure rather than a cure for problematic code and leaky libraries. Optimizing the codebase of your application for efficient memory usage should always be the priority.
Another recommended practice is to right-size your Gunicorn workers. Normally, you allocate Gunicorn workers depending on available CPU cores. However, if your application uses high memory, it might be advisable to use fewer workers than CPU cores. Now let’s have a look at the appropriate formula to get an idea of how many workers you should use:
Workers = (2 x $num_cores) + 1
But remember, these are just general guidelines. The exact number must be tuned according to your actual workload and resources.
A practical way of adapting instances would be using auto-scaling based on pre-set matrix like CPU utilization or memory footprint if you’re running your infrastructure on cloud distributes systems such as Google Cloud Platform (GCP), Amazon Web Services (AWS), etc. Huge tech companies like Instagram also leverage adaptive pre-fork model where parent process monitors workers and scale up or down according to the load, a technique effective against memory overcommitment.
If these measures don’t solve the problem, consider profiling your application. Tools like cProfile or Py-Spy can give you insights into parts of your code that could be causing memory leaks. Remember to analyze your dependencies as well; third-party libraries can sometimes introduce unexpected side effects.
Traces from tools would provide constructive insights and point out exactly in your source code where the most time was spent. These spots in code are probably your culprits for memory consumption or CPU starvation.
To sum up, maximizing the efficiency of your Gunicorn server workers mainly involves managing memory usage effectively, right-sizing your worker processes, and judiciously evaluating your application and dependencies. Each step perfectly complements the other and we cannot overemphasize the importance of understanding your application workflow in identifying specific bottlenecks.
Remember, there never is a one-size-fits-all solution to mitigating issues revolving around worker termination with Signal 9. It largely depends on various factors that interact in complex ways, including your application’s specifics, scenarios of usage, and the environment in which it runs. Do not hesitate to seek help from performance audit tools to aid your diagnosis.
Action Point | Description |
---|---|
Effective Memory Management | Monitor and limit memory usage. Consider metrics-based autoscaling. |
Sensible Worker Allocation | Ensure the allocation of Gunicorn workers is optimal for your server’s size and application’s nature. |
Investigation and Profiling | Analyse codebase and dependencies carefully using profiling tools to find bottlenecks. |
Despite its versatility and friendliness to beginners, Python still poses challenges when it comes to maintaining the stability of your web server. Frequent occurrences of Signal 9 errors can be particularly frustrating as they lead to termination of Gunicorn workers which disrupts service.
In Unix-like operating systems, a signal is a software interrupt delivered to a process. Signal 9 is SIGKILL, which forces immediate termination of a process. When a Gunicorn worker is terminated with this Signal 9, it indicates that your Python web server abruptly closed a process—something which is generally unfavorable for system stability. Here are some best practices to maintain your Python web server stability with respect to these errors.
Arguably one of the most important aspects is having an optimized Gunicorn configuration. This involves determining the appropriate number of worker processes, types of workers (sync or async), and timeouts (source). High traffic applications may require more workers while read-intensive applications benefit from async workers.
# example Gunicorn config command = '/usr/bin/gunicorn' pythonpath = '/myapp' bind = '127.0.0.1:8000' workers = 4 worker_class = 'gevent' timeout = 30
Overutilization of resources by your application processes could result in Signal 9 errors – if you’re out of memory, the Linux OOM Killer might kill your Gunicorn processes! Monitor your system’s CPU usage, memory, disk I/O, etc. There are tools like `top`, `htop`, and `vmstat` that come built-in on many UNIX-based systems which can be used for real-time monitoring. Also look at adjusting ulimits and correctly sizing your machine to avoid running out of system resources.
Gunicorn’s –access-logfile and –error-logfile options allow logging all requests and errors respectively. You should consider enabling log rotation so old logs don’t accumulate and fill up your disk space. The Python standard library’s `logging.handlers.RotatingFileHandler` class makes this easy.
import logging from logging.handlers import RotatingFileHandler logger = logging.getLogger('my_logger') handler = RotatingFileHandler('/var/log/my_logger.log', maxBytes=2000, backupCount=10) logger.addHandler(handler)
In addition to logging, setting up alerting for when certain errors occur or when error rates pass certain thresholds can help detect and debug issues before they affect too many users.
If Gunicorn workers are frequently being killed, there could be code-level issues present. Perhaps there are memory leaks, infinite loops, tasks that take too long to run within the defined timeout, etc. Analyzing your logs from –access-logfile and –error-logfile will provide more insight into what was happening just before each worker termination.
In conclusion, maintaining the stability of a Python web server involves careful tuning of Gunicorn settings, diligent resource management, and thorough code-level troubleshooting—all while keeping accurate error records. Teaming all these best practices together will ensure your web service runs steadily and securely and that Gunicorn worker terminations become a thing of the past.
It’s crucial to understand that when a Gunicorn worker gets terminated with signal 9 (SIGKILL), it implies the worker was abruptly and forcefully stopped, without giving it the chance to clean up or finish any ongoing processes. This can occur due to multiple reasons including:
To investigate these issues, employ tools like GDB and strace to debug at the process level. You could also set timeouts within Gunicorn using:
workers = 3 timeout = 120
Remember, It’s not advisable to have arbitrary long or no set timeouts as this leads to memory exhaustion.
Furthermore, modifying your software to support multithreading might provide significant benefits. Multithreaded applications exhibit graceful handling of multiple requests simultaneously.
Consult Gunicorn’s documentation for more details on possible configurations related to worker processes. Furthermore, consider reading their debugging guide to gain an in-depth understanding of worker behaviors.
Finally, monitoring tools like NewRelic or Sentry can provide insightful details about the history leading up top the point where the worker got killed, aiding you in spotting patterns and understanding if specific requests tend to trigger the problem.
Recognizing these elements surrounding the phenomena of a ‘Gunicorn worker getting terminated with signal 9’ can offer deep insights into your application’s behavior. Implementing the detailed suggestions can protect your application from unexpected shutdowns while providing a consistent user experience.