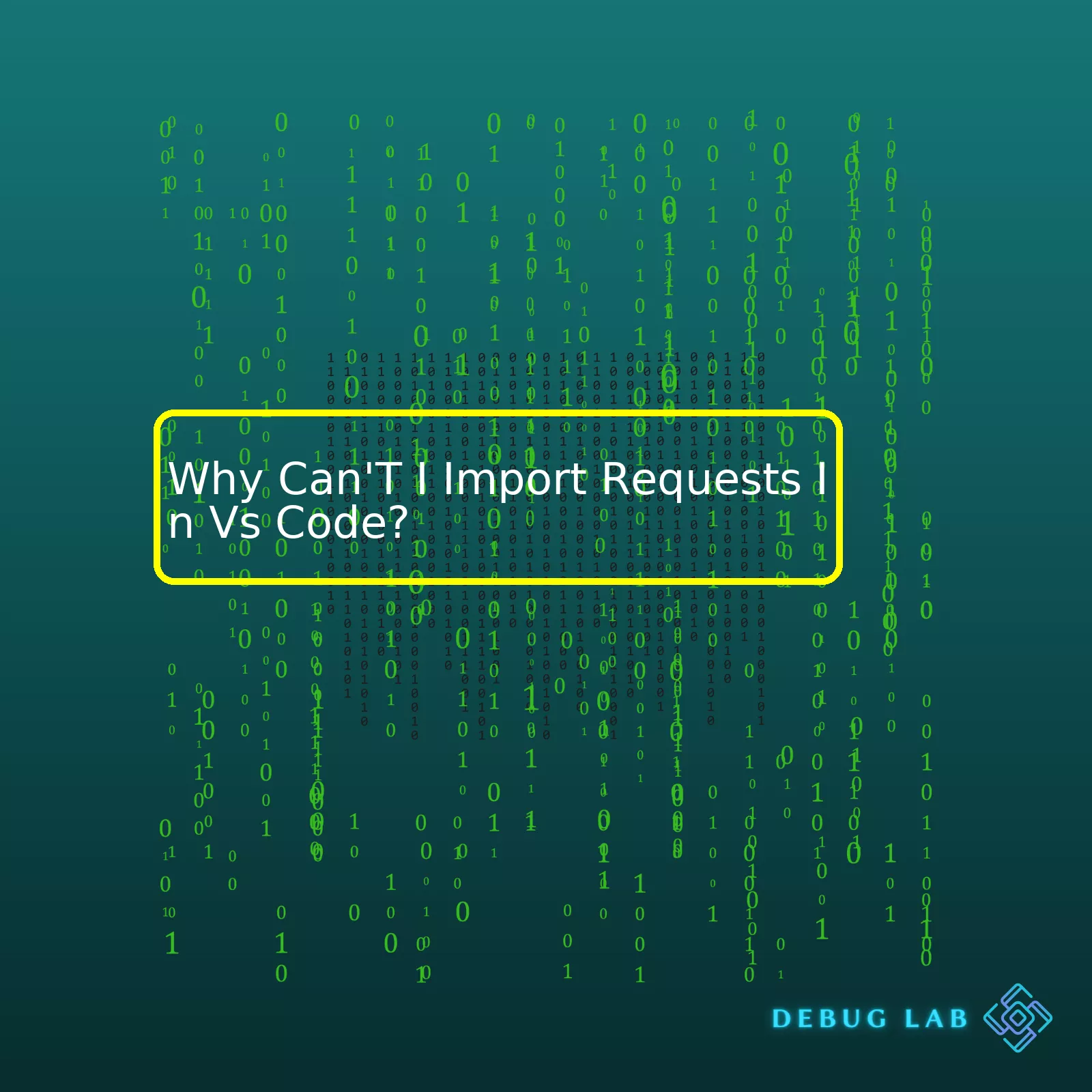
Issue | Possible Reason | Solution |
---|---|---|
Can’t import ‘requests’ in VS Code | Pip not installed or wrong Python interpreter is selected | Install pip and select the correct Python interpreter |
‘requests’ library is not installed | You didn’t install the required library to your Python environment | Use Pip to install the ‘requests’ library |
Not using the right environmental variable | The installed library isn’t recognized because of different environments variables | Change the environmental variable where pip installs packages |
The prompt “Why Can’t I Import Requests In VS Code?” usually pops up when you’re using Python in Visual Studio Code and trying to write a code block that requires importing the external library named ‘requests’. One of the major reasons you might be experiencing this problem is perhaps because pip, which is Python’s package manager, isn’t correctly set up. To solve this, make sure that pip is properly installed in your development environment.
python -m ensurepip --upgrade
Another reason could be that the Python interpreter selected within Visual Studio Code isn’t the one where pip has installed the ‘requests’ library. Make sure the interpreter selected in VS Code matches the interpreter where ‘requests’ is installed. If ‘requests’ library is not already installed, use pip to install it.
xxxxxxxxxx
pip install requests
Lastly, you may have installed the ‘requests’ library with pip, but are trying to import it in Python in a different environment from the one where pip installed the package. Ensuring proper environment consistency is important. It’s often good practice to use virtual environments in Python to maintain consistency across all dependencies relevant to your specific project.
Let’s take a look at an example of changing the environment variable where pip installs your library files. First, we can create a virtual environment scoped to our project:
xxxxxxxxxx
python3 -m venv /path/to/new/virtual/environment
Once the virtual environment is set up, we can activate it like so:
For Windows,
xxxxxxxxxx
.\venv\Scripts\activate
Or for Unix or MacOS,
xxxxxxxxxx
source venv/bin/activate
Now, any libraries you install with pip while this environment is active will be scoped just to this environment.
Remember, programming in Python using Visual Studio Code should be a walk in the park once every software necessary for Python codes to run smoothly are properly installed and configured. So, if you’re getting an error for such an issue, just revisit your configurations and confirm you’ve got everything right [source].
On this note, addressing these potential causes would certainly aid in resolving the problem of being unable to import ‘requests’ in Visual Studio Code. Remember, the objective is not to simply resolve the issues encountered, but to understand why they occur in the first place. This would assist you in mitigating similar problems in the future and overall, propel your growth on the journey to mastering Python coding in Visual Studio Code.Let’s analyze possible issues related to the problem: “Why Can’t I Import Requests in VS Code?”
Firstly, requests library is not a built-in Python package. It’s an independent project created by Kenneth Reitz and it must be separately installed.
You can install it using pip:
xxxxxxxxxx
pip install requests
Or if you are using Python 3:
xxxxxxxxxx
pip3 install requests
If you have already installed requests but you’re still facing import errors, there might be multiple causes for this problem:
One common issue arises when virtual environment usage comes under consideration:
• If you’re working in a virtual environment and you have installed requests there, but your VS Code is set to use the global Python interpreter, you won’t be able to import requests until you change the interpreter to the one associated with your virtual environment.
Steps | Description |
---|---|
Inspect the Bottom Bar | Your current Python interpreter is displayed on the right side of the status bar at the bottom of the window. |
Command Palette | Open the Command Palette (Ctrl+Shift+P) and type ‘Python: Select Interpreter’. |
Select Interpreter | Choose the interpreter that corresponds to the virtual environment where you installed requests. |
Another potential issue is related to multiple Python versions installed on your machine. If you’ve installed requests using one version of Python (let’s say Python 2.7), but you’re trying to import it in a file that uses a different Python interpreter, you’ll encounter an import failure. To confirm this, check which python interpreter is being used by the VS Code. You can do this by opening the Command Palette and typing ‘Python: Select Interpreter’.
An additional mitigation technique relates to the PATH:
• The PATH environment variable could not be properly set up to include the directory containing the requests module.
When Python tries to import a module, it looks at the directories listed in sys.path. So, before importing requests, print out sys.path to see where Python is looking for modules:
xxxxxxxxxx
import sys
print(sys.path)
The output will be a list of directories. If the directory where requests is installed doesn’t appear on this list, add it manually with:
xxxxxxxxxx
sys.path.append('path_to_directory')
Every time a Python program runs, it maintains a system path that shows the directories it’s looking in for imported modules. By printing sys.path, we get these locations. If the directory where requests is located isn’t listed, we can manually append it to sys.path, substituting ‘path_to_directory’ with the relevant directory.
Finally, consider re-installing Requests or even Python itself, as unexpected hiccups like corrupted files could naturally lead to packages like requests failing to import.
For more assistance, the Python Software Foundation provides a comprehensive guide on how to install Python packages.
Remember to consider further searches and readings, exploring diverse experiences shared by developers. Websites like Stack Overflow often provide valuable insights that help solving coding issues.
Hopefully, with these insights, you can avoid future headaches when attempting to import the requests library into VS Code. Stay persistent and experiment with these techniques to ensure smooth implementation of your code.The inability to import requests in VS Code can be directly linked to the configuration or setup of your Python environment. This might happen because your VS Code is making use of a different Python interpreter than the one where your
xxxxxxxxxx
requests
library is installed.
What is a Python environment?
A Python environment is akin to an isolated container where you install libraries and frameworks that your Python project will use. It keeps the dependencies used by different projects separate by creating isolated Python virtual environments for them.
When you create a new environment, you’re creating a separate Python installation that has its own files, resources, and modules. This allows you to have a distinct set of libraries for every project, ensuring compatibility among the modules used in each of your projects, and mitigating conflict between modules having different versions.
Versus Code and Python environment
VS Code is a highly configurable source code editor. It has built-in support for languages such as Python. However, most of the time when we install a package using pip—Python’s package installer—we install it globally on our system. But when we work with VS Code, we sometimes work in a unique Python virtual environment. If we don’t explicitly tell VS Code the path to the Python interpreter within this environment, it might not recognize the packages installed within.
Therefore, whenever you try importing the
xxxxxxxxxx
requests
module in VS Code, it might fail although you’ve already installed it using pip on your global Python interpreter.
The Solution?
You need to point VS Code to the Python interpreter where the
xxxxxxxxxx
requests
library exists. Here’s how:
• Open your VS Code
• Go to command Palette (You can get it by either pressing F1 or use shortcut Ctrl+Shift+P)
• Type: ‘Python: Select interpreter’
Then you will see a list of Python interpreters available for selection. Choose the right interpreter where your
xxxxxxxxxx
requests
library is installed.
In the case that the correct python interpreter is already selected and you still cannot import the
xxxxxxxxxx
requests
module, this means you probably have not installed it in that environment. In that case you can install it by simply opening the terminal in VS Code and typing:
xxxxxxxxxx
pip install requests
Update: The version on the pip of your package could vary depending upon the python environment you are currently working on, thus using the following command might help you:
xxxxxxxxxx
python -m pip install requests
This ensures that you’re using the pip associated with the current Python interpreter.
Knowing how to handle and manage your Python environment effectively is key to running successful Python projects. Tools like venv allow you to maintain separate environments easily, and understanding the integration between your code editor (like VS Code) and these environments will ensure smooth development process.
Sources:
VS Code Environments,
Requests Documentation
If you are having trouble importing the
xxxxxxxxxx
requests
package in Visual Studio Code (VS Code), it typically means that the package has not been installed in your current Python environment. This could be a global environment or a virtual environment, depending on your setup.
The
xxxxxxxxxx
requests
package doesn’t come bundled with Python. It is an external library which needs to be manually installed. Generally, when installing external packages for use in Python projects, we make use of Python’s built-in package manager: PIP (Python Package Index). This makes sure that our Python interpreter can find and utilize these packages at runtime.
xxxxxxxxxx
pip install requests
This command goes out to Python’s online package repository (PyPi) and fetches the latest version of the specified package, in this case, `requests`.
However, if the package is installed globally and VS Code is utilizing a specific virtual environment, then you may still face issues. Virtual environments have their own isolated Python installations and don’t have access to global site-packages by default.
There are two primary solutions to this problem:
1. You can activate the virtual environment and then install the ‘requests’ package within it.
2. Change the Python interpreter which VS Code is using.
These can be achieved as follows:
- Activating the Virtual Environment & Installing ‘requests’
Firstly, you need to understand how to activate your existing virtual environment. This is dependent on whether you have used venv, pipenv, pyenv or another tool. Without activation, any packages you install via PIP will be into your global site-packages which might not be utilized by VS Code. After you activate the respective environment, you can install ‘requests’ like so-
xxxxxxxxxx
pip install requests
Here is an example of activating the environment and installing ‘requests’ if you use venv
xxxxxxxxxx
source env/bin/activate #activate your environment first
pip install requests #then install requests
- Changing the Python Interpreter in VS Code
In some cases, changing the interpreter that VS Code is targeting can resolve these type of import issues. This is because each python interpreter has its modules and knowing which interpreter you’re using can help debug the issue.
To change the interpreter, follow these steps on the official VS code documentation.
Remember, managing dependencies is crucial for maintaining clean, manageable, and production-grade code. Coding is an art form, and just like colors in a painting work together, packages in your code should also harmonize together. Take note to handle and verify your dependencies correctly and troubleshoot issues appropriately when they arise.
By understanding Python’s dependency management, you can conquer import errors like “Cannot Import Requests”. It’s all about aligning your Python interpreter, the environment, and ensuring the necessary packages are installed where required. Remember to document every step; transparency is key to avoid confusion in larger teams.
Your team members can run the same commands to ensure continuity and similarity throughout your project. Happy debugging!In order to use any Python library such as `requests` through Visual Studio Code’s (VSCode) editor, the library has to be installed within the Python environment that VS Code is using. You might experience an issue like being unable to import `requests` in VS Code due to problems such as:
– The Python interpreter configured in your workspace settings doesn’t have the `requests` module installed.
– Your VS Code is pointing towards a different Python interpreter in which you haven’t installed the `requests`.
– You might also experience this problem if `Pylint`—which is used for linting in VS Code—is installed globally instead of in a specific virtual environment.
Here are possible misconfigurations and solutions:
Possible Misconfiguration: Wrong Python Interpreter
You might have selected the wrong Python interpreter in your Visual Studio Code. If the interpreter doesn’t have ‘requests’ module installed, then you can’t import it in your code. To resolve it,
xxxxxxxxxx
-> Open Command Palette (Ctrl+Shift+P)
-> type "Python: Select Interpreter"
-> select the correct interpreter that has 'requests' installed
Possible Misconfiguration: Pylint Installed Globally
If you have `Pylint` installed globally or without activating the specific virtual environment where `requests` is installed, you may face this issue. To fix it, ensure that you install `Pylint` in the specific virtual environment where `requests` is installed. Use your terminal to navigate to the project directory, activate the virtual environment and then install `Pylint`:
xxxxxxxxxx
pip install pylint
Possible Misconfiguration: Module Not Installed in Current Environment
Your current Python environment may not have the ‘requests’ module installed. You should run the following command in your terminal:
xxxxxxxxxx
pip install requests
Remember to execute this command in the same Python environment that is set in your Visual Studio Code.
For persistent issues, consider viewing the official VSCode Python troubleshooting guide. To understand better how to manage Python environments in Visual Studio Code, check this official guide.You might encounter errors when trying to import modules such as Requests in VS Code due to a variety of reasons. Here are likely causes:
Environment Path Issues
Working with multiple Python versions can often cause confusion with pathing. If the Python interpreter used by the VS Code is different from what’s accessing the Requests library, an error will occur.
Packages Not Installed
It could be that the Requests library was not installed correctly or at all. Standard Python installations don’t come bundled with additional packages like Requests.
Virtual Environment Misconfiguration
If you’re using a virtual environment such as venv or pipenv and it doesn’t have the Requests library installed, this might cause the “import requests” instruction to fail.
Below are possible ways to troubleshoot this issue:
Checking Your Python Version
To ensure that VS Code is using the same Python version as the one involved with Requests, use the following commands:
xxxxxxxxxx
python --version
This command checks the version of Python in your system. Then, within VS Code, activate the Python interpreter by typing the command:
xxxxxxxxxx
"name_of_the_python_interpreter" --version
If those two commands display different versions, then that suggests VS Code isn’t using the Python interpreter where “requests” package is installed.
Installing Packages
If the Requests library isn’t installed, you need to do so through pip:
xxxxxxxxxx
pip install requests
This installation should be done in the terminal within the VS Code.
Virtual Environment Configuration
In case you’re using a virtual environment, make sure that you’ve exported it in the correct way. To ensure that the ‘requests’ library is actually installed within your virtual environment, activate it first:
xxxxxxxxxx
source ./name_of_your_environment/bin/activate
Then make sure to install the Requests library within this virtual environment:
xxxxxxxxxx
pip install requests
The table below summarizes these common error sources and solutions:
Error Sources | Solutions |
---|---|
Environment Path Issues | Ensure VS Code uses the Python version that has the Requests library |
Packages Not Installed | Install the Requests library through pip |
Virtual Environment Misconfiguration | Export the virtual environment appropriately and ensure to install Requests in it |
Concerning unpacking syntax and command errors, issues associated with syntax usually stem from misunderstanding the coding language’s rules. Remember, Python utilizes indentation to differentiate codes. For instance, inconsistent use of tabs and spaces for indentation can result in syntax errors. On the other hand, command errors typically occur when the interpreter fails to understand what’s demanded due to typos or incorrect usage of commands or functions.
If you’re having trouble troubleshooting, you can seek assistance at the online communities dedicated to Python and VS Code, such as [StackOverflow] and the [Python Documentation].
Lastly, always remember the importance of debugging your code line-by-line or checking out various tutorials for using IDEs like VS Code efficiently.When it comes to importing requests in VS Code, a few key issues could possibly arise that might be causing the trouble:
1. You’re not working with the correct virtual environment.
Multiple Python installations can co-exist on a single system without conflicting as they’re installed in their respective environments. If you attempt to import the “requests” library without first selecting the correct Python interpreter for your virtual environment where “requests” is actually installed – otherwise known as activating the environment – you’ll often encounter an ImportError.
In VS Code, ensure you’re working in the correct environment by selecting the associated Python interpreter from the Command Palette (
xxxxxxxxxx
Ctrl+Shift+P
, then enter ‘Python: Select Interpreter’). The selected Python interpreter in use by VS Code should have the `requests` module installed.
2. Requests installation has not been done correctly or at all.
It’s possible that the “requests” package itself isn’t installed in your virtual environment. To troubleshoot this, activate your Python environment in VS Code terminal and try installing requests with pip:
xxxxxxxxxx
pip install requests
Then try to import it in your script again.
3. Your source code files are named improperly.
Python modules should not be named after standard Python libraries. For instance, if you name your Python file `requests.py`, it may conflict with the `requests` library that you are trying to import leading to unexpected errors. Make sure no such conflicts exist while trying to import `requests`.
According to the official Python docs, the first file found along `sys.path` that matches the import statement would be sourced in. Therefore, if your custom scripts share names with any of the standard library packages like `requests`, Python will mistake your file for the intended library and subsequent instantiation calls would fail (since your script presumably does not define the functionalities provided by the actual library).
By analyzing these points, evaluating your current situation and taking the required actions accordingly, you can remedy the problem of not being able to import `requests` into your VS Code environment. Remember, coding often involves troubleshooting and refining your process, but the reward is a smoothly operating, issue-free coding experience.
While using the sophisticated editor like VS Code, some new coders might experience difficulties with importing certain libraries, such as ‘requests.’ If you’re one of them asking – Why Can’t I Import Requests in VS Code?, The root cause most likely lies within Python’s virtual environment configuration. Virtual environments are critical for a tidy workspace since they allow each project to have its home-grown dependencies, irrespective of other Python projects.
Creating and Activating a Virtual Environment
In an interactive shell, invoke the
xxxxxxxxxx
venv
module as a script to create a virtual environment. Use the below command:
xxxxxxxxxx
python3 -m venv tutorial-env
This command will create the tutorial-env directory if it doesn’t exist and also includes an interpreter that can run the python code directly. To activate this on Unix or MacOS, use:
xxxxxxxxxx
source tutorial-env/bin/activate
Installing Packages Inside a Virtual Environment
The requests library can be installed within the virtual environment scope by using pip. Remembering that you’ve activated your virtual environment, install the ‘requests’ package using the following command:
xxxxxxxxxx
pip install requests
Python Extension in VS Code
VS Code’s Python extension does a magnificent job in interpreting which Python interpreter to apply for executing scripts, linting, debugging, etc., by considering the available
xxxxxxxxxx
.venv
,
xxxxxxxxxx
pyenv
,
xxxxxxxxxx
virtualenv
environments in your workspace.
Selecting the Correct Python Interpreter on VS Code
To make the VS Code Python extension operate inside the virtual environment, you need to manually pick which interpreter to employ. Be sure to select the interpreter that’s stored inside your project folder (typically
xxxxxxxxxx
.venv
or something similar), owing to the fact that each project ought to possess its independent virtual environment.
To change the interpreter, just click on the left-hand corner and choose the interpreter from your
xxxxxxxxxx
.venv
directory. After doing this, try importing “requests” again and behold the magic that happens!
If you still face problems while importing the requests library, refer to the official VS Code documentation for more detailed information related to Python Environments.
Issues with importing requests in VS Code might stem from a variety of potential problems but predominantly, it’s an environment problem where VS Code is unable to recognize the installed packages. Some actionable steps that can be implemented to resolve this issue include:
- Ensure that you have the requests package installed in the same Python environment which your project also refers to. This can be validated by checking the Python interpreter being used. You may set the correct interpreter like this:
xxxxxxxxxx
131{
2"python.pythonPath":"path-to-your-python"
3}
on your settings.json file.
- The use of virtual environments and making sure that you are using the correct python interpreter from within the Virtual Environment. You should activate the virtual environment like so:
xxxxxxxxxx
111source venv/bin/activate
for UNIX and Linux, or
xxxxxxxxxx
111.\venv\Scripts\activate
for Windows, then check if you have requests.VS Code Python Environments
- Make sure you install the requests library using pip directly from the terminal inside Visual Studio Code. For example, type:
xxxxxxxxxx
111pip install requests
- You could ensure that requests module has been correctly installed in your Python site-packages directory by navigating to it and verifying its presence. A quick way to locate your site-packages directory is by running
xxxxxxxxxx
111python -c "import site; print(site.getsitepackages())"
in your terminal or command prompt.
Incorporating these solutions should effectively resolve the “Can’t Import Requests In VsCode” error and allow the seamless interaction between the requests module and your Python environment within the Visual Studio Code.
All these above-mentioned solutions for solving importing request issues in VS Code underline the importance of understanding how Python interpreter works in correlation with virtual environments and packages installation. This knowledge is crucial not only for working with the ‘requests’ library but also forms the foundation of effective Python programming utilizing VS Code.
Further, it’s suggested to refer detailed tutorials to understand how modules and packages work in Python. One good resource would be The Python Documentation or several other online tutorials that explain various aspects of installing, importing and using different Python packages and dealing with common errors.
xxxxxxxxxx
113131Sample table showcasing different solutions (Professional recommendation):
2+---------------------+----------------------------------+
3| Solution | Suitable If |
4+---------------------+----------------------------------+
5| Set correct path | Python interpreter path is wrong |
6+---------------------+----------------------------------+
7| Use virtual env | You're working on many projects |
8+---------------------+----------------------------------+
9| Install via pip | requests is not installed |
10+---------------------+----------------------------------+
11| Check site-packages | Couldn't find where requests is |
12+---------------------+----------------------------------+
13
Always taking these insights into account and applying strategies based on the context will surely make you more proficient and productive in coding, especially when employing Integrated Development Environments (IDE) like Visual Studio Code for Python programming.