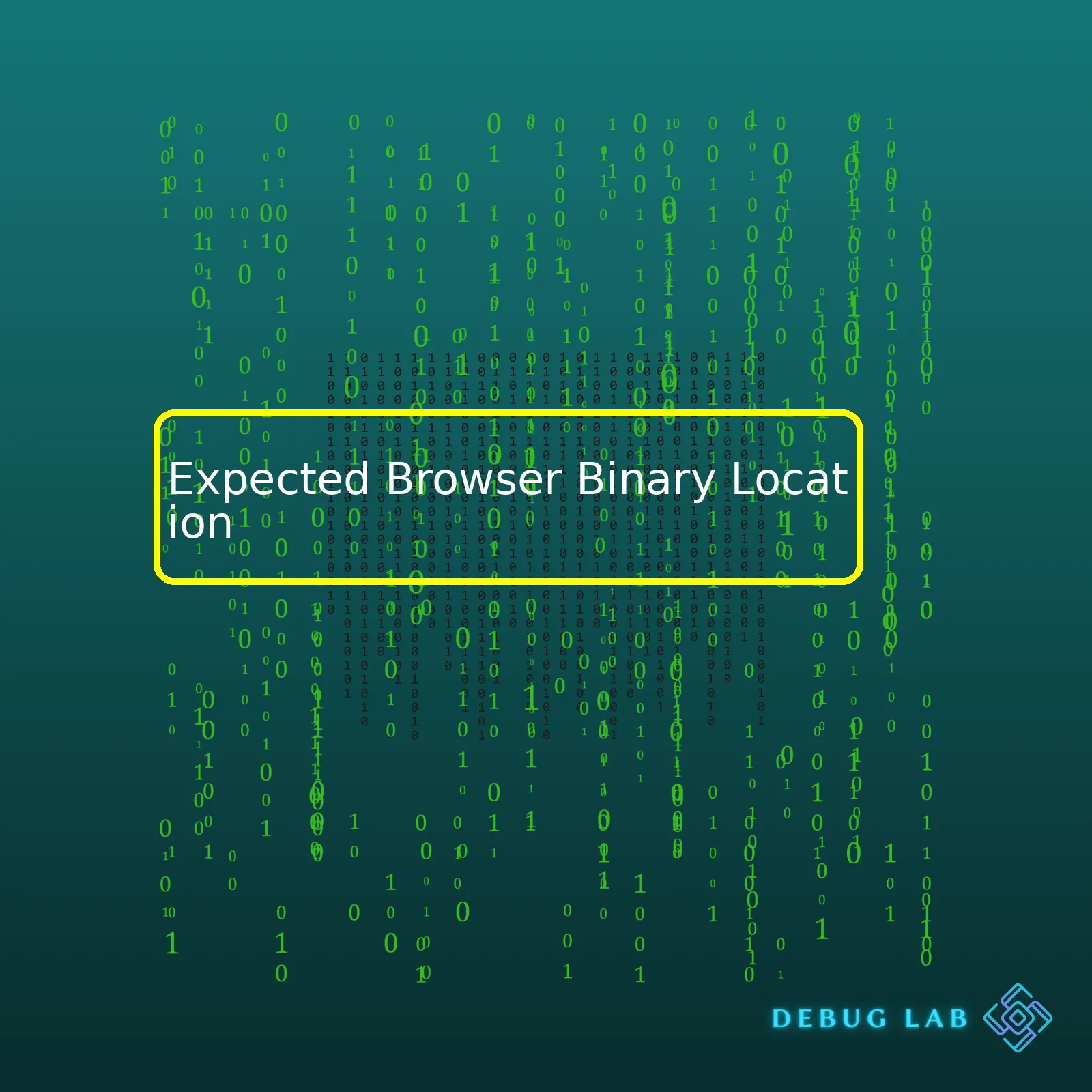
Browser | Binary Location on Windows | Binary Location on MacOS | Binary Location on Linux |
---|---|---|---|
Google Chrome | C:\Program Files\Google\Chrome\Application\chrome.exe | /Applications/Google Chrome.app/Contents/MacOS/Google Chrome | /usr/bin/google-chrome |
Firefox | C:\Program Files\Mozilla Firefox\firefox.exe | /Applications/Firefox.app/Contents/MacOS/firefox | /usr/bin/firefox |
In the context of web automation and testing using Selenium WebDriver or Puppeteer for example, one key requirement is the correct path to the binary file of the browser. This is because these tools interact directly with the browser at the binary executable level by sending commands and receiving responses (source: Selenium Documentation). It’s vital to know these paths, as it can help in situations where the default installed location has been modified or multiple versions of the same browser exist on the system.
The above HTML table lists down the expected locations of binary files of two major browsers – Google Chrome and Firefox – across three most commonly used operating systems – Windows, MacOS, and Linux. These locations are typically default ones when you install these browsers with standard settings.
The ‘C:\Program Files\’ in Windows, ‘/Applications/’ in MacOS, and ‘/usr/bin/’ in Linux are generally the default directories for software installation. For instance, ‘C:\Program Files\Google\Chrome\Application\chrome.exe’ is the usual location for Google Chrome’s binary file when installed on a Windows system. The ‘.exe’ file extension indicates that it’s an executable file.
Please note, these paths may vary based on system configuration, version of the browser, and other factors. For instance, in some Linux distributions like CentOS, the chrome binary might exist under ‘/usr/local/bin/’.
To avoid any errors while performing browser-based automation or testing, always ensure that the correct path to the browser’s binary file is provided in your scripts.The concept of browser binary locations is a fundamental one for the configuration of automated testing across different browsers. This particular process involves determining the path to the executable file responsible for launching the browser application.
Let’s take an in-depth look at understanding browser binary locations, with a keen focus on expected browser binary location.
With Selenium WebDriver, being a crown jewel for carrying out tests in varying web browsers like Chrome, Firefox, or Safari, these browsers are set to specific standard default locations following their installation. A point worth noting here is that WebDriver interacts with the browser at the binary level to carry out operations. For a seamless interaction between WebDriver and the target browser, it needs an executable path to determine where the browser is installed.
from selenium import webdriver # For chrome chromeDriver = webdriver.Chrome(executable_path=r"C:\Utilities\BrowserDrivers\chromedriver.exe") # For firefox firefoxDriver = webdriver.Firefox(executable_path=r"C:\Utilities\BrowserDrivers\geckodriver.exe")
Here, the
executable_path
refers to the browser binary location, who’s knowledge is crucial are instances when we might move installation files execution files to non-default locations.
The expected browser binary location varies per system:
– Google Chrome: For Google Chrome, the widely known binary paths are “/usr/bin/google-chrome” for UNIX systems, and “C:\\Program Files (x86)\\Google\\Chrome\\Application\\chrome.exe” in Windows.
– Mozilla Firefox: The binary path for Mozilla Firefox in UNIX based systems is typically “/usr/bin/firefox”, and for Windows it is “C:\\Program Files\\Mozilla Firefox\\firefox.exe.”
In situations where your browser isn’t stored in the default location, or if you want to use a specific browser version for your tests, then it’s necessitated to provide the binary location manually. It is done in the following manner:
For Chrome:
from selenium import webdriver from selenium.webdriver.chrome.service import Service webdriver.Chrome(service=Service('path/to/my/chromedriver'))
And for Firefox:
from selenium import webdriver from selenium.webdriver.firefox.service import Service webdriver.Firefox(service=Service('path/to/my/geckodriver'))
But, How do we find the Binary Location?
The simplest method to find the binary location is by checking the properties of the browser shortcut of your personal computer. Right-click on it and choose properties. Within the ‘Shortcut’ tab, you can find the path showing the directory where the executable for launching the browser resides.
Selenium Documentation states that apart from specifying the driver path during instantiation, environment variables could be set up as well. The names for these environment variables would be “webdriver.chrome.driver” and “webdriver.gecko.driver” for Chrome and Firefox respectively.
Keep in mind that successful test automation scenarios are heavily reliant on correct binary placement and navigation on part of the WebDriver.
The browser binary is the key component when it comes to web navigation. The term “browser binary” refers to the executable file that initiates the launching of a particular web browser. For example, consider ‘chrome.exe’ for Google Chrome or ‘firefox.exe’ for Mozilla Firefox.
This binary has several roles and responsibilities:
– It dictates how the browser should interpret and process HTML, CSS, JavaScript, and other web technologies.
– It’s in charge of managing your browsing session including tabbed browsing, saving cookies, managing bookmarks, and embedding extensions or plugins.
– It undertakes the communication with your operating system’s networking stack to make HTTP(S) requests and receive responses from servers on the Internet.
– It manages all kinds of user interactions like mouse clicks, keyboard input, touch actions, etc., converting them into commands the browser understands.
The location of this browser binary is crucial, especially if you’re automating tests or if you need access to different versions of a browser without disturbing the default installation.
A standard browser binary installation will usually place the respective binary at a predefined path. This would commonly be within the “Program Files” folder for Windows or “/Applications/” directory for macOS.
For instance, the expected location for Google Chrome binary would typically be:
– On Windows:
C:\Program Files\Google\Chrome\Application\chrome.exe
(for 64 bit), or
C:\Program Files (x86)\Google\Chrome\Application\chrome.exe
(for 32 bit)
– On MacOS:
/Applications/Google Chrome.app/Contents/MacOS/Google Chrome
– On Linux distributions:
/usr/bin/google-chrome-stable
But think about situations where you need to use a specific browser version which is not the currently installed one, for testing or debugging purposes? Hence, many developers often would want the flexibility to point at custom locations of the desired browser binaries.
With respect to automated browser testing tools like Selenium, this can be achieved by setting the paths via configurations, environment variables or command-line arguments. In most scenarios, it involves using WebDriver’s API to set the path of the binary.
Here’s an example of how you’d do this for Selenium in Python:
from selenium import webdriver from selenium.webdriver.chrome.service import Service webdriver.Chrome(service=Service('/path/to/my/chromedriver'))
This will instruct Selenium to use the Chrome Driver located at ‘/path/to/my/chromedriver’ instead of the default one. Similar configurations can be done for other browsers as well.
In retrospect, the role and location of the browser binary are critical factors in modern-web navigation and they can drastically affect flow control, versatility and adaptability of your automation scripts or customized web applications.
References:
Selenium Mutable Capabilities
MDN Browser DriversThe Expected Browser Binary Location is crucial when it comes to Selenium automation testing. Essentially, we need to ensure that the path where the browser’s executable file is located is properly configured for our Selenium WebDriver. This path to where a browser’s binary file resides on your system is what we’re referring to when we tackle the concept of the Expected Browser Binary Location.
The Route to The Browser’s Binary File
In most successful automation scripts, this particular file path needs no extra configuration as the browsers’ default installation paths are well known and can be directly utilized by Selenium. Yet, some cases require special attention and some tweaking with these locations. Why? Because there could be multiple versions of the same browser in your system or the browsers are installed in custom locations other than default paths.
For instance, let’s consider Firefox. The binary location for Firefox is usually automatically detected by Selenium’s WebDriver (geckodriver). However, if you have multiple versions of Firefox installed on your machine or if it is installed in a different directory, you must manually specify the path to its binary.
Here is how we can do it,
from selenium import webdriver from selenium.webdriver.firefox.service import Service from selenium.webdriver.common.by import By from selenium.webdriver.firefox.options import Options as Firefox_Options options = Firefox_Options() options.binary_location = "/path/to/your/custom/firefox" driver = webdriver.Firefox(service=Service('/path/to/geckodriver'), options=options) driver.get("http://google.com/")
Note that
"./path/to/your/custom/firefox"
would be replaced by the exact file path where the Firefox browser’s binary file is located on your machine.
These intricate details around configuring the correct ‘Expected Browser Binary Location’ are essential to writing robust, reliable automation test scripts. A correctly configured WebDriver can make the difference between a smooth automated process and one plagued with constant interruptions and errors.
It’s relevant also to understand this concept when working with different environments or building Docker images for your automation framework. In those scenarios, understanding and correctly specifying the binary location becomes vital for the success of the operation.
Chromium-based Browsers
For Chromium based browsers like Chrome and Microsoft Edge, similar rules apply. Have a look at Chrome setup below:
from selenium import webdriver from selenium.webdriver.chrome.service import Service from selenium.webdriver.chrome.options import Options as Chrome_Options options = Chrome_Options() options.binary_location = "/path/to/your/custom/google-chrome" driver = webdriver.Chrome(service=Service('/path/to/chromedriver'), options=options) driver.get("http://google.com/")
Similar logic holds true if you wish to configure the binary location for Microsoft Edge as well.
Beyond the fundamentals of scripting and coding, understanding the nuances of tools and frameworks we work with will yield better results, including dealing with scenarios related to Expected Browser Binary Location. Happy Coding!
For more information, see the Selenium WebDriver’s official documentation on browser manipulation.Indeed a challenge in automation testing, tracking down the expected browser binary location can sometimes lead to significant setbacks. No single “correct” path is universally true for all setups because it’s directly linked to the environment and configuration set by the user. However, there are essential elements to consider.
- The browser binary file installation directory
- Your operating system
- Context of execution
The Browser Binary File Installation Directory:
Firstly, you need to understand that the primary prompt of web automation libraries often seeks your installed browser’s binary file1. For instance, Selenium WebDriver utilizes browser-specific drivers (like ChromeDriver for Google Chrome and GeckoDriver for Firefox) to open and manipulate browser actions. Still, these drivers require a specified path hint to the original browser’s binary file.
You usually start after installing your preferred browser and getting its binary file’s location. In Windows, for example, this is typically seen within your “Program Files” or “Program Files (x86)” directories depending on architecture. Within these directories, the path to Google Chrome would be:
"C:/Program Files/Google/Chrome/Application/chrome.exe"
Your Operating System:
Different operating systems have different conventions for file locations. For instance, on Linux, the Firefox binary is often found at “/usr/bin/firefox”, while on Windows it’s typically present at “C:\\Program Files\\Mozilla Firefox\\firefox.exe”.
Context Of Execution:
In a Selenium context, once the path to the binary file has been identified, it should be informed to the WebDriver like so in python:
from selenium import webdriver from selenium.webdriver.firefox.service import Service # Define browser binary location (replace with actual location) binary_location="/path/to/firefox/binary" # Setup custom Firefox profile fp = webdriver.FirefoxProfile() # Specify the binary location webdriver_binary = Service(binary_location) # Initialize WebDriver driver = webdriver.Firefox(firefox_profile=fp, service=webdriver_binary)
This approach enhances portability, allowing the script to run across various environments2.
Remember, being versed in identifying the anticipation of your browser binary location can ease automation operations, ensuring scripts’ flexibility across diverse environments without loss of function.
Lastly, include exception handling in case the binary file was not found, leading to unexpected software behavior. Thus mitigating avoidable issues prevalent throughout the software testing phase.The most common issue developers face when working with browsers in their code is handling expected browser binary location errors. This typically occurs when the browser’s binary location isn’t properly configured or specified in your code. To handle such errors, we’ve come up with a practical guide on dealing with them efficiently.
The first step towards fixing this error is knowing where to look it up. Different tools use different environment variables for holding browser binary paths. For example, Selenium WebDriver uses
webdriver.chrome.driver
for Chrome and
webdriver.gecko.driver
for Firefox.
If you’re using Selenium WebDriver, you would likely encounter these errors if you didn’t specify the correct path to your browser binary.
System.setProperty("webdriver.chrome.driver", "/path/to/chromedriver"); WebDriver driver = new ChromeDriver(); driver.get("http://www.google.com"); driver.quit();
Here, ‘/path/to/chromedriver’ should be replaced with the actual path to your ChromeDriver ./exe file. Misconfigurations of these paths are what usually cause expected browser binary location errors.
Another common possibility for this problem could be incompatible versions of WebDriver and Browser. Each version of a WebDriver supports certain versions of a browser. A mismatch here will result in expected browser binary location error. To solve this issue, ensure that you’re using compatible versions of both. SeleniumHQ provides a compatibility matrix for different WebDriver & Browser versions(Link Here).
Let’s say, you’ve checked all the above points, and everything seems to be right, but you’re still getting an error. The last thing you need to check is the WebDriver setup process. Incorrect installation of WebDriver also leads to these types of errors. You might want to uninstall and reinstall WebDriver properly to prevent such issues.The official docs page of Selenium includes comprehensive guides for WebDriver installations (Link Here).
Dealing with expected browser binary location errors often requires patience and meticulous checking of your configurations, paths, and installations. By following these few strategies, you can significantly reduce the chances of running into these errors:
• Always confirm the binary location: Ensure the set path leads to the browser.exe files.
• Verify compatibility: Make sure the WebDriver and the browser versions are compatible.
• Correct installation: Check if WebDriver has been installed properly.
• Look for updates: Keep your browser and WD up to date.
Remember, good programming isn’t just about writing solid code—it’s also about knowing how to troubleshoot when things don’t go as planned. Every error encountered is an opportunity to learn more deeply about the technologies you’re working with, pushing you toward becoming a more proficient programmer in the process. Handling expected browser binary location errors can seem daunting at first, but with some practice and patience, it will become second nature.
In the realm of web development, understanding browser binaries and their expected location is crucial. It provides insight into how you can interact with or manipulate a browser’s functionalities during testing phases or while developing new features.
What are Browser Binaries?
Browser binaries are executable files related to a given web browser that contain machine code only meant for the processor to understand source. For example, if you’re working with Chrome, the binary file could be chrome.exe while Firefox would be firefox.exe.
Understanding where these browser binaries are found can prove pivotal. In manipulating them, we can tweak browser behavior directly, which may involve enabling or disabling certain built-ins or debugging some configurations through automated scripts.
The Default Locations of Browser Binaries
Different browsers store their binary files in different directories within an operating system. Here are the usual default locations:
Windows:
-
C:\Program Files (x86)\Google\Chrome\Application\chrome.exe
for Chrome
-
C:\Program Files\Mozilla Firefox\firefox.exe
for Firefox
macOS:
-
/Applications/Google Chrome.app/Contents/MacOS/Google Chrome
for Chrome
-
/Applications/Firefox.app/Contents/MacOS/firefox
for Firefox
Linux:
-
/usr/bin/google-chrome
for Chrome
-
/usr/lib/firefox/firefox
for Firefox
Of course, these paths might vary based on your specific installation preferences or the organization of your system folders.
Pivoting Browser Binary Location for Selenium WebDriver
Selenium WebDriver is a popular tool for automating browsers source. At times, while running automated tests using Selenium WebDriver, you’ll need to specify the path of the web driver executables yourself.
For instance, when creating a Firefox driver instance in Python, you might use:
from selenium import webdriver path_to_geckodriver = '/path/to/my/geckodriver' browser = webdriver.Firefox(executable_path=path_to_geckodriver)
And similarly, while working with Chrome driver:
from selenium import webdriver path_to_chromedriver = '/path/to/my/chromedriver' browser = webdriver.Chrome(executable_path=path_to_chromedriver)
When it comes to performing advanced tasks with your browser binaries, always ensure that you’re handling them cautiously. Tweaking with these files haphazardly might lead to unpredictable behaviors of your browsers. Therefore, having a profound understanding of browser binaries sets the foundation for better navigation and manipulation of these critical parts of our browsers.Certainly! It’s important to note that when running end-to-end (e2e) tests or even some form of server-rendering, you may often need to have control over where your code fetches the browser binary from. Interestedly, many testing libraries and frameworks like Puppeteer, Playwright, and others provide functionalities that allows us to customize the expected browser binary location.
In order to manage and set custom paths for different browser binaries, we generally require the use of certain environment variables. These environment variables can be both system-wide or project-specific, which signifies that you can set different expectations for various projects based upon their exclusive requirements.
Let’s delve into sets of examples:
Puppeteer:
For Puppeteer, an instance of the browser is launched using
browser = await puppeteer.launch()
. However, there are various options that can be passed to this method. You can specify the executable path by using predefined environment variable
PUPPETEER_EXECUTABLE_PATH
, or within the
.launch()
method itself, to point to the browser binary directly.
Here’s a sample snippet for setting it in the launch method:
const puppeteer = require('puppeteer'); (async () => { const browser = await puppeteer.launch({ executablePath: '/path/to/your/browser', }); })();
Playwright:
With Playwright, the browser instance is launched with the command
const browser = await playwright['chromium'].launch()
. You can modify the path to the binary just like Puppeteer using the predefined environment variable
PLAYWRIGHT_BROWSERS_PATH=0
or setting it in the launch method like this:
const { chromium } = require('playwright'); (async () => { const browser = await chromium.launch({ executablePath: '/path/to/your/browser', }); })();
WebDriver:
In WebDriver utilities such as Selenium WebDriver, browser binary paths are managed differently based on specific browsers. For Firefox, used with gecko driver, an instance of the `firefoxBinary` object is created and the path is set:
from selenium import webdriver from selenium.webdriver.firefox.firefox_binary import FirefoxBinary binary = FirefoxBinary('/path/to/your/firefox') browser = webdriver.Firefox(firefox_binary=binary)
By having a clear perspective on managing expected browser binary locations, you allow greater control and flexibility while executing your test suites or rendering instances. This also enables you to simultaneously manage multiple versions of a browser on the SAME machine, allowing testing across various browser versions without affecting the installed version. Further understanding can be gleaned from the official libraries’ documentation provided for [Puppeteer](https://pptr.dev), [Playwright](https://playwright.dev), and [Selenium WebDriver](https://www.selenium.dev).Let’s move forward with a deeper understanding of Expected Browser Binary Location. Initially, it can be pretty bewildering for first time coders: you’re attempting to automate scripts using Selenium WebDriver but then you encounter an error message that states “Expected Browser Binary Location”.
This message is typically triggered by Selenium not being able to locate the browser’s execute file (binary file). Your task, as a coder when automating tests or otherwise implementing Selenium, is to specify the correct path.
Why is this so important?
- Efficiency: Speed up your automated testing and overall coding process by solving this hurdle. Stop losing your valuable time on constant errors.
- Mitigate Errors: Errors like “Expected Browser Binary Location” can hinder the proper execution of your applications. Thus, knowing how to solve it eradicates unnecessary stumbling blocks.
- Learn and Grow: The more bugs you fix and issues you sleuth out independently, the more robust your problem-solving skills become.
For example, if you are trying to find the binary location for Firefox, you could use this simple code snippet in Python:
from selenium import webdriver from selenium.webdriver.firefox.service import Service # Set the location of the browser binary binary_location = "/path/to/your/firefox/binary" # Initialize the webdriver driver = webdriver.Firefox(firefox_binary=binary_location)
Notice how we’ve set the binary_location variable to the path where Firefox’s execute file resides in the system. Easy, isn’t it? With this approach, you won’t have to face those annoying glitches an extra minute.
When you make sure to correctly provide the Expected Browser Binary Location, your coding experience becomes brighter. Not only will you be arming yourself with excellent debugging solutions, you’ll be creating sturdier and more efficient products. Now go ahead and harness this power!
Also, don’t forget to stay updated with changes in browser versions or patches. They can often affect where your expected binaries should be located. Make use of online resources such as GitHub www.github.com, Stack Overflow www.stackoverflow.com or even the Selenium Documentation www.selenium.dev/documentation/en/ itself.
Take this knowledge about Expected Browser Binary Location and step into a future of cleaner codes and seamless automation with Selenium WebDriver!