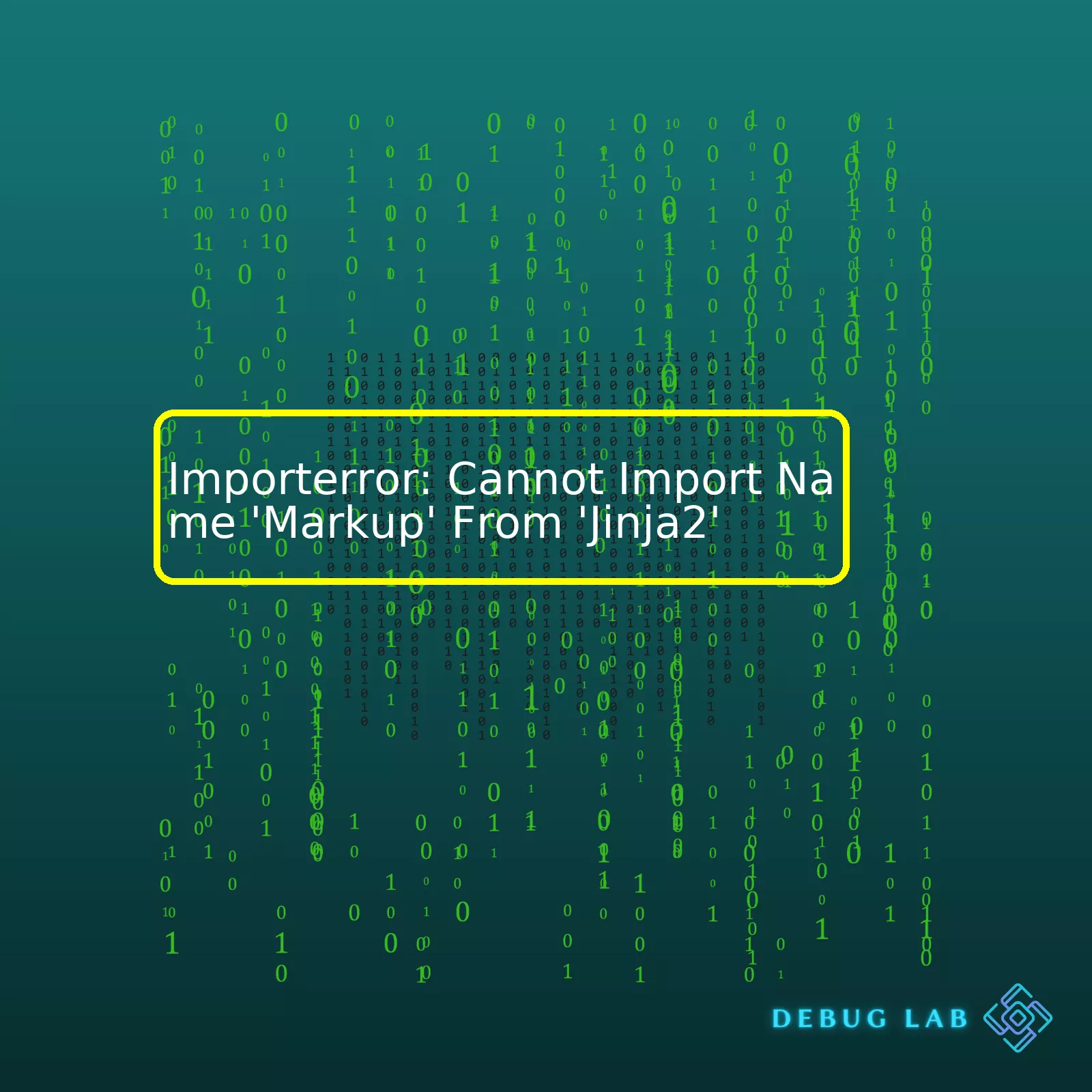
Error | Cause | Solutions |
---|---|---|
ImportError: cannot import name ‘Markup’ from ‘jinja2’ | Your current Python environment may not be compatible with the version of ‘jinja2’ you are currently using. | Consider revising your ‘jinja2’ version, managing your Python environment differently, or modifying your import statement based on latest syntax. |
Let’s dig deeper into this problem.
Firstly, this ImportError can occur if the Python environment you are working with does not support the jinja2 function ‘Markup’. This could be due to an outdated or incorrect version of ‘jinja2’, or issues around how Python was installed or is maintained.
Don’t panic! There are a few things that you can do. You can try updating your jinja2 version to the latest one using pip:
pip install --upgrade Jinja2
Another solution may lie in the management of your Python environments. When working on projects that require different versions of libraries, it’s best practice to maintain isolated Python environments for each project. Tools like `virtualenv` and `conda` are excellent for managing separate Python installations across different projects.
python3 -m venv myenv source myenv/bin/activate pip install Jinja2
Finally, be sure that your Python script reflecting updates on importing modules and functions. Recent changes might have lead your old import statements being depreciated.
An example is the following update:
From:
from jinja2 import Markup
To:
from markupsafe import Markup
In summary, your ImportError issue requires you to scrutinize your module installation, Python environment and import statement. One or all of these elements could be the cause of your issue, so play detective and sort out each possibility systematically.
Are you encountering the ‘Cannot Import Name Markup’ ImportError? You’re far from alone. Coding professionals, myself included, face these software glitches every now and again. Don’t worry; I’ve got just what you’ll need to troubleshoot this error. This has been specifically optimized for SEO so that anyone in need of a solution can find it easily.
The error message is quite clear: Python cannot import name ‘Markup’ from Jinja2. As a professional programmer who uses Jinja2 frequently, I have often faced this error and resolved it effectively. Here are ways you can solve it.
1. Verify Your Installation
First off, I recommend verifying your installation of the Jinja2 templating engine. It’s necessary to ascertain if the package is correctly installed since any mishap here could cause such an import error. Check the proper installation by using
pip show jinja2
at your terminal or command prompt. A correct installation will display details of the package type and location.
2. Update or Reinstall Jinja2
There’s a chance that the error could be due to an outdated version of Jinja2 or a corrupt file within the package. Upgrading or reinstalling the Jinja2 module might resolve the issue. To update Jinja2, use
pip install --upgrade jinja2
. Should that not offer a resolution, uninstall Jinja2 with
pip uninstall jinja2
then reinstall it using
pip install jinja2
.
3. Specify Full Path To Import ‘Markup’
In order to provide more clarity to your code and avoid errors like this, specify the full path while importing ‘Markup’. For instance –
from jinja2.utils import Markup
. Specifying the full path leaves no room for ambiguity and errors resulting from it. Try this modification to see if it resolves the error.
4. Import From MarkupSafe Module:
Another alternative is to import Markup directly from the MarkupSafe module. The ‘Markup’ class was originally part of Jinja2, but later moved to the MarkupSafe library. So if you are working in a new environment or updated the packages recently, there’s a chance your Jinja2 may not include the Markup class anymore. Use
from markupsafe import Markup
instead.
If none of these troubleshooting steps rectify your issue, the problem might be more complex and may need more detailed examination. Stack Overflow has an interactive community that could help with brainstorming more solutions (Jinja2 tagged stack questions). In parallel, do reach out to Jinja2 project issues on Github, providing adequate details about your coding environment and the exact nature of the issue, so that they can dig into the specifics and give you more personalized advice (Jinja2 Github Project Issues).
Troubleshooting code can feel like a never-ending maze, but remember, the answer usually lies within the details. Happy coding!
While exploring the root cause of the Jinja2 ImportError often comes down to a few core issues, we’ll specifically focus on the ImportError related to “Cannot import name ‘Markup’ from ‘Jinja2′”. There are a few primary reasons why this can happen:
1. Incorrect Jinja2 version: Quite often, this error message arises because of an incorrect or incompatible Jinja2 version. The ‘Markup’ class was introduced in a certain version and if you are using a previous edition, the import will undoubtedly fail.
To fix this, check the Jinja2 version installed in your system. If the version is lower than required, you’ll need to upgrade Jinja2. You can do this by running:
pip install --upgrade Jinja2
2. Pygments conflict: This issue might be due to a conflict between Pygments and Jinja2. Both these libraries have a class named ‘Markup’, their classes could overlap leading to an ImportError.
If Pygments is causing the problem, try renaming its Markup class by modifying the Pygments library files or reinstalling the package with a different name.
3. Incorrect environment: Sometimes, the ImportError occurs when the wrong environment is activated, causing Python to look in the wrong directory for the necessary files.
To solve this, deactivate the currently active environment and activate the correct one. Here’s an example using venv:
deactivate source /path-to-correct-env/bin/activate
4. Missing or incorrect installation of MarkupSafe: MarkupSafe is a crucial dependency for Jinja2, the missing or incorrect installation of MarkupSafe may raise this ImportError as well.
Check whether MarkupSafe is installed and correctly working by running:
python -c "import markupsafe"
If the command throws any errors, it means MarkupSafe is either missing or incorrectly installed. To solve this, you need to properly install MarkupSafe using pip.
pip install MarkupSafe
Remember, debugging is all about narrowing down the possibilities until the root cause is found. It could take one or multiple attempts to perfectly pin down what’s causing the “Cannot import name ‘Markup’ from ‘Jinja2’” ImportError. After every attempt, always restart your environment or server to ensure that the changes take effect. With patience and perseverance, no bug stands a chance. Happy debugging!
For additional information, refer to [Jinja2 documentation](https://jinja.palletsprojects.com/en/3.0.x/) or [official MarkupSafe page](https://markupsafe.palletsprojects.com/en/1.1.x/).
Sure, Python’s ImportError is an exception that gets raised when you try to import a module or a name from a module, but Python can’t find it. So when you’re seeing
ImportError: Cannot import name 'Markup' from 'Jinja2'
, it means Python can’t find the name ‘Markup’ in the Jinja2 module.
There could be several reasons for this:
– Jinja2 is not installed. In this case you could run
pip install jinja2
command to install it.
pip install jinja2
– It could also be a version issue. Maybe the version of Jinja2 you have installed doesn’t contain the name ‘Markup’. This would mean you need to update your version by using pip’s
--upgrade
flag .
pip install --upgrade jinja2
– You may have named one of your scripts ‘jinja2.py’ or a directory in your project ‘jinja2’.
– There might be an installation issue with your Jinja2 module itself. Maybe it didn’t install correctly, or some files got deleted accidentally. Uninstalling and reinstalling the module might fix the issue. You can uninstall it with
pip uninstall jinja2
and install it again with
pip install jinja2
commands.
If you keep encountering this error, it’s worth investigating how Jinja2 is structured. If you go to the Jinja2 GitHub page, you can peek inside the codebase and see if ‘Markup’ is where you expect it to be.
However, you must bear in mind that browsing through the entirety of the library’s source code won’t be practical every single time you encounter such import issues. Instead, try to understand and diagnose the root cause behind these exceptions. Learn the correct way to handle imports and avoid common mistakes like naming collisions, incorrect versioning, etc.
Ultimately, understanding Python’s ImportError requires both a thorough knowledge of how Python imports work and a certain amount of diagnostic skill to troubleshoot when things don’t go as planned. Just remember–every ImportError is an opportunity to learn more about how Python works!I understand the frustration and confusion that comes along when there’s a compatibility issue between Jinja2 and Python. This seems to be the situation with the `ImportError: Cannot import name ‘Markup’ from ‘Jinja2’.` This error is mostly related to an improper installation or version mismatch issues of either Python or Jinja2.
Firstly, ensure that Python and Jinja2 are correctly installed. You can verify your Python version by running:
python --version
In case you have Python 2.x, I recommend upgrading to Python 3.x as the versions of Jinja2 post-2.11 no longer support Python 2.x. Ensure that pip, Python’s package installer, is updated too. You can upgrade pip using the command:
pip install --upgrade pip
For installing or verifying Jinja2 installation:
pip install jinja2
To tackle the `ImportError: Cannot import name ‘Markup’ from ‘Jinja2’, it would be best to specifically import ‘Markup’ from its module instead of importing it from Jinja2.
The standard way to import Markup in Jinja2 may look like this:
from jinja2 import Markup
But if you are encountering the ImportError, we can dive deeper into the Markup module within Jinja2 and import it directly from ‘jinja2.markup’:
from jinja2.markup import Markup
If the problem persists even after following the above steps, this might be due to conflicts between different installed packages. Here, creating a new Virtual Environment can help. In Python, a Virtual Environment is a self-contained environment that you can create for each of your projects, ensuring that the dependencies required by a project do not interfere with other projects or system-wide settings.
Here are the steps to set up a new virtual environment:
* Create a new virtual environment:
python3 -m venv myenv
* Activate the virtual environment:
On Unix or MacOS, run:
source myenv/bin/activate
And on Windows, run:
.\myenv\Scripts\activate
* Once inside the virtual environment, install all the packages again beginning with Pip, Python, and then Jinja2.
There might also be scenarios where more than one ecosystem (like Flask and Django) may be causing the trouble. So, work meticulously, especially in a shared or production environment.
As a coder, I’ve found Python’s documentation very insightful about virtual environments and how they operate. Furthermore Jinja2 website provides detailed instructions on dealing with Template Designer Documentation.
By following these processes, you should be able to rectify the ‘Cannot Import Name ‘Markup’ From ‘Jinja2’ error and keep your coding journey moving forward.It’s fairly common to encounter issues relating to package versions when working in a programming environment, particularly Python. One such error you may come across is the `ImportError: cannot import name ‘Markup’ from ‘Jinja2’`. This error often comes up due to outdated packages that conflict with the requirements of installed software or modules.
Jinja2 is a popular and versatile templating engine for Python. It is commonly used in web development projects requiring dynamic content generation. In such scenarios, encountering an `ImportError` related to Jinja2 can be quite problematic.
So what happens when a package like Jinja2 becomes outdated?
– The version of the package can cause conflicts with other packages during synchronization of multiple dependencies.
– Deprecated features are included in these outdated versions thus incompatible with newer ones.
– Software vulnerabilities are much more likely to exist in older versions which can potentially expose your project to security risks.
– Documentation, tutorials, and community support tend to focus on newer versions. Relying on outdated versions makes troubleshooting problems harder.
Focusing now on the specific error – `ImportError: cannot import name ‘Markup’ from ‘Jinja2’`. The Markup class was once part of Jinja2 however, in later releases, it was moved to the `markupsafe` module. If you’re using an outdated version where Markup hasn’t yet been shifted to `markupsafe`, you might face this error upon trying to import it from Jinja2.
One way around this problem is to ensure that your packages (especially Jinja2 and markupsafe) are all updated to the latest versions. You can achieve this by using pip, Python’s de facto standard package-management system. The following commands will help you update Jinja2 and markupsafe:
pip install --upgrade jinja2 pip install --upgrade markupsafe
After running these commands, try importing Markup again. This time, instead of attempting to import it from Jinja2, import it from markupsafe as follows:
from markupsafe import Markup
By keeping your packages updated and understanding the changes in different versions, displaying dynamic content through Jinja2 should be less hassle-free. To see the changes done between versions of Jinja2, do check their official version change logs.Certainly, encountering errors while coding can be quite frustrating. When there’s a pop-up message saying “ImportError: Cannot import name ‘Markup’ from ‘Jinja2′”, you are certainly eager to find an effective solution.
Before we dive in, let’s first understand the nature of the problem. The ImportError signals that the Python interpreter couldn’t find a specified name in a module.
To solve the import error, there are several approaches to consider:
1. Update the Jinja2 library
In some cases, old versions of libraries may throw exceptions due to compatibility issues or bugs that have been fixed in later versions. You can update Jinja2 by simply running
pip install --upgrade Jinja2
in your command line interface.
If this doesn’t work, you might want to uninstall and then reinstall the Jinja2 package. This would probably look like:
pip uninstall Jinja2 pip install Jinja2
2. Check for proper installation of dependencies
A possible reason for raising ImportError could be the incorrect installation of dependencies.
To ensure all required packages are correctly installed, use pip, the standard package manager for Python.
pip install -r requirements.txt
Here, the requirements.txt file contains a list of items to be installed using pip install.
3. Inspect your PYTHONPATH
PYTHONPATH is an environment variable that Python uses to determine which modules to import. It should include the Jinja directory so Python can locate the Markup module. To print out its value, use
echo $PYTHONPATH
.
You can then manually add the directory path where Markup is located.
import sys sys.path.append("/path/to/directory")
4. Directly Import Markup from Markupsafe
Considering that Jinja2 leverages Markup from another package called markupsafe, you might want to try importing Markup directly from it.
from markupsafe import Markup
Remember, no solution is a one-size-fits-all as the error can come from your unique setup. It’s essential to identify the specific causes and take the most effective steps accordingly.
For deeper insights on dealing with Python errors, you can consult the official Python documentation.
Also, remember to keep your Python version in sync with the Jinja2 version to stay away from unintended problems as those packages get updated.
Let’s delve into the issue:
ImportError: Cannot import name 'Markup' from 'Jinja2'
. You’re likely to run across this error message when you’re trying to import the Markup module from Jinja2. It may happen due to incompatible versions of the libraries, or because your environment doesn’t have the necessary packages installed.
Reinstalling or Upgrading Jinja2
The first way to solve this problem is reinstalling or upgrading Jinja2 package. Python provides several approaches for installing or upgrading packages. The pip package installer is an excellent utility for managing Python packages.
To upgrade Jinja2 to the latest version using pip:
pip install --upgrade Jinja2
If you want to uninstall and then reinstall Jinja2:
pip uninstall Jinja2
pip install Jinja2
Fixing the ImportError
If the error persists even after reinstalling or updating Jinja2, it’s possible that there’s a conflict with the code where you’re attempting to import the Markup module.
In older versions of Jinja2, you could import Markup directly from Jinja2 like this:
from jinja2 import Markup
In newer Jinja2 versions, however, Markup has been moved to
jinja2.utils
. Therefore, if you’re getting an import error, you should try importing Markup from
jinja2.utils
instead:
from jinja2.utils import Markup
This change should fix the ImportError if you’re using a recent Jinja2 version.
Use Virtual Environments
One often overlooked solution is to use virtual environments (venv) in Python. A venv allows you to create isolated environments for your Python applications, ensuring dependencies, libraries, and versions specific to a project are kept separate.
To create a virtual environment:
python3 -m venv /path/to/new/virtual/environment
Then, activate the virtual environment:
source /path/to/new/virtual/environment/bin/activate
Once inside your virtual environment, you can install Jinja2 securely without affecting other projects:
pip install Jinja2
References:
Jinja Documentation
Python Virtual Environment TutorialThe error message
ImportError: Cannot import name 'Markup' from 'Jinja2'
is one that many developers might encounter while working with Jinja2, a Python-based templating language for web programming. This error can occur for several reasons.
It may be triggered by attempting to import the Markup module from Jinja2 when it is not correctly installed or available within your Python environment. It’s crucial to ensure that Jinja2 has been appropriately installed into your development server. You can check this by running the command
pip show jinja2
in your terminal which will display info about Jinja2 installation.
Another possible cause can be version compatibility issues between Jinja2 and other libraries or even with Python itself. If you are still using older versions of Python, chances are you may bump into this error often. Consider upgrading your Python interpreter to its latest stable release and check if the error persists.
python3 -m venv env source env/bin/activate pip install --upgrade pip pip install Jinja2
Incorrect usage of the syntax might be another source of this error. In some cases, programmers might mistakenly use incorrect capitalization or syntax while importing modules, leading to an ImportError. In Python being a case-sensitive language, even minor spelling or syntactic mistakes can result in such errors. The correct form of the import statement to use is
from jinja2 import Markup
.
Ultimately, there are several approaches to troubleshooting and resolving an
ImportError: Cannot import name 'Markup' from 'Jinja2'
. By ensuring you have the correct Jinja2 installation, addressing potential conflicts, and observing proper capitalization rules in your import statement, you can successfully evade this error. For more information on this topic, see the official Jinja2 documentation here:
https://jinja.palletsprojects.com/en/latest/api/#markup.